API Development in Python: A Detailed Exploration
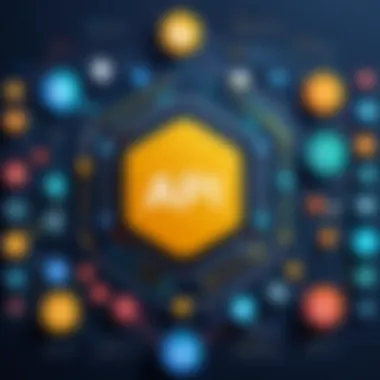
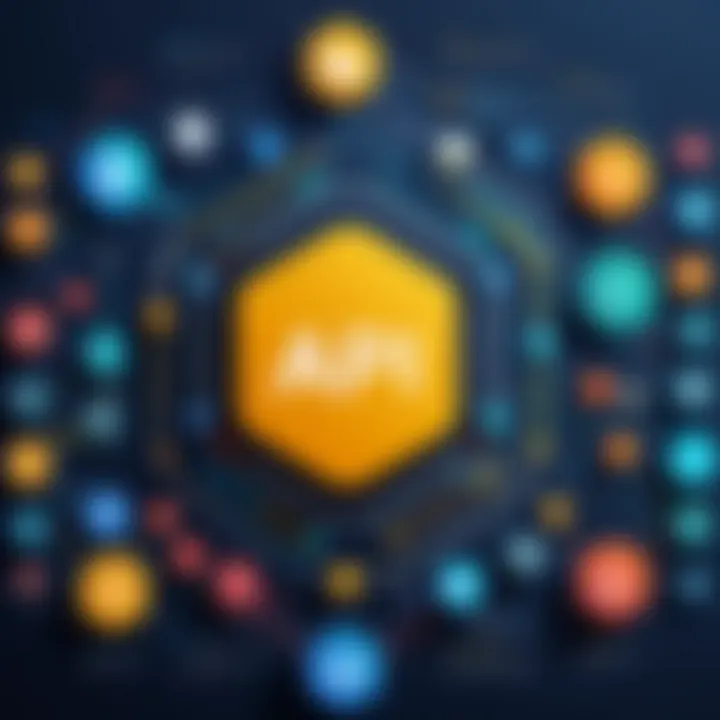
Overview of Topic
API development is a critical aspect of modern software architecture. It involves creating robust systems that facilitate communication between different software applications. The significance of API development in today's tech industry cannot be understated. With rapid digital transformation, organizations need reliable and efficient methods to interact with their services, making APIs increasingly essential.
The evolution of APIs traces back to the early days of computing. Originally, they were used primarily to allow different software to function together and share data. As the internet expanded and cloud computing emerged, APIs have been adapted to work with distributed systems. They now serve as the backbone of modern web applications.
Fundamentals Explained
To engage with API development using Python, it is key to understand some core principles. APIs act as the only way for external clients to access a software program’s functionalities without exposing the underlying mechanics.
Key Terminology
Here are some important terms relevant to API development:
- Endpoint: The URL where an API can be accessed.
- Request: A message sent by a client to the server asking for data.
- Response: The data returned by the server.
- JSON: A commonly used data format for APIs.
- REST: An architectural style for dealing with networked applications.
The complexities of APIs narrow down to a few foundational concepts. These include resources, data formats, and communication protocols.
Practical Applications and Examples
API development is not theoretical. Numerous real-world applications rely on APIs for their functionality. For example, social media apps utilize APIs to access user data or to interact with other services.
Case Studies
- Twitter API: It allows developers to build applications and access Tweets programmatically.
- Google Maps API: It is pivotal in location-based services within many applications.
Hands-On Project
To illustrate practical implementation, consider creating a simple RESTful API using Flask:
This lightweight application showcases how simple the process of creating an API can be.
Advanced Topics and Latest Trends
With the passage of time, API development has seen numerous advancements. Trends include the rise of GraphQL, providing greater flexibility compared to REST APIs. Another trend is the greater emphasis on security and rate limiting to protect resources from misuse.
Future Prospects
The future for API development looks promising. Integrating artificial intelligence into APIs is setting the stage for intelligent communication between applications. Programs that learn from user interactions can lead to more refined experiences.
Tips and Resources for Further Learning
For those keen to dive deeper, various resources can enhance understanding and skills:
- Books: RESTful Web APIs by Leonard Richardson.
- Online Courses: Check platforms like Coursera and Udacity.
- Tools: Postman for testing APIs and Swagger for documentation.
Seek information on sites like Wikipedia for well-rounded knowledge.
API development influences how digital systems interact. Understanding its inner workings enables seamless integration of new features.
As discussed, Python serves as a powerful tool in building APIs, complemented by a community rich in resources. Every step taken in this guide pushes you toward enhancing your skills in API development.
Prologue to API Development
API development is an essential aspect of modern software engineering. The reliability and interoperability of applications today heavily depend on the use of Application Programming Interfaces, or APIs. Understanding how APIs function is crucial for developers, particularly in the context of API development in Python. This introduction delves into key facets that merit discussion.
Definition and Importance of APIs
APIs, or Application Programming Interfaces, are varied sets of rules that allow different software applications to communicate with each other securely and effectively. They serve as an intermediary enabling functionalities and data exchange without requiring developers to understand the complexities of the underlying code. APIs have become integral in building robust, modular, and scalable applications in our digitally-connected world, where speed and efficiency are paramount. They allow for the integration of third-party services, resulting in enriched functionality. This enables developers to focus on core application features without delving into aspects best handled by other services.
Overview of API Types
In API development, acknowledging the existence of various types of APIs is fundamental.
- REST APIs: REST stands for Representational State Transfer, which has distinct principles defining its adaptability and scalability. REST APIs mainly utilize HTTP methods, consistently retrieving or modifying resources located at unique URLs, making it familiar and easier to use in web-based applications. Its key characteristic is the use of standard HTTP requests, making data fetching intuitive. This contributes significantly to the prevalence of REST as the chosen architecture in many development projects due to its simplicity and efficiency. However, its stateless nature can lead to complexities when managing sessions or contexts in more intricate setups.
- SOAP APIs: Simple Object Access Protocol, or SOAP, emphasizes a strict protocol for message format using XML. This structure allows for high security, which can be beneficial in sensitive data transactions. A significant characteristic of SOAP APIs is the endpoint where they communicate through predefined rules. Their formal contract requirements may seem advantageous; however, designing and implementing SOAP can often prove more intricate than RESTful approaches. Therefore, developers must weigh its merits against the higher complexity involved, especially in pyhton.
- GraphQL APIs: GraphQL presents an evolution in API structure, allowing clients to specify precisely what data they need. This flexibility makes it especially useful in terms of bandwidth, as clients can retrieve only relevant data in a single request instead of making multiple calls, which is common in traditional REST APIs. Its unique feature lies in the ability to reduce over-fetching and under-fetching of data. Despite its growing popularity, GraphQL brings challenges in caching strategies and requires robust management due to its dynamic nature.
As APIs become more embedded into our applications, understanding these basic types assists in making informed design decisions. This exploration sets the foundation for a deeper look into API development and Python's role in creating such interfaces, moving us forward into the richness of this domain.
Python's Role in API Development
Python's integration into API development signifies not only a growing trend but also a level of practicality and efficiency in creating and maintaining web services. This section delves into various aspects identifying why Python is an exceptional language for developing APIs, focusing on its simplicity and the vast ecosystem it brings to the table. Readers will appreciate an insight into the advantages Python offers in the context of API development, helping to demystify its core value propositions.
Why Use Python for APIs
Ease of Learning
One of the most compelling reasons to use Python for APIs is its ease of learning. Designed with clarity in mind, Python's syntax mimics human language, enabling newcomers to grasp fundamentals quickly. This characteristic lowers the barrier to entry for those who might be intimidated by programming languages. As such, both beginners and experienced developers find Python productive and efficient.
Advantages of Python’s simplicity include:
- Reduced boilerplate code significantly speeds up development.
- Enhanced readability fosters better collaboration among team members.
- An active community frequently shares resources for overcoming hurdles in the learning process.
The generally lower learning curve can lead to faster prototyping, enabling projects to advance more quickly through design and iteration phases. However, while this accessibility is typically an advantage, the simplicity sometimes attracts criticism for lacking advanced features inherent in other languages.
Rich Libraries and Frameworks
Python's diverse collection of libraries and frameworks presents considerable benefits in the realm of API development. Tools such as Flask, Django, and FastAPI equip developers with essential abilities for crafting robust API endpoints. Each framework caters to different needs; for instance, Flask enables flexibility in projects where customizability is paramount, while Django offers a more opinionated approach with built-in features for development.
Some notable features include:
- Extensive libraries enable tasks such as database management and data validation, thereby minimizing redundant work.
- Community support allows quick resolutions to queries or issues, making learning and building références more manageable.
- Frameworks often provide prebuilt functionalities, leading to decreased implementation times and improved product stability.
Despite these strong points, developers sometimes need to navigate the choice of framework carefully. Each brings its own learning curve, coding styles, and knowledge requirements, necessitating consideration in the project design phase to ensure alignment with developer skill sets and project goals.
Python Versions and API Compatibility
Python evolves iteratively, with major version releases impacting API practices regularly. Yet, compatibility with APIs is generally maintained, making transitions manageable for development teams. The time invested to adapt code with revised versions contributes positively to pushing forward evolving standards in development.
Periodic updates and community discussion forums champion best practices for ensuring compatibility when updating programs. Developers can take proactive measures to future-proof APIs by understanding potential pitfalls associated with version changes and testing extensibility early in the development process.
Setting Up the Development Environment
Setting up the development environment is a crucial page in the journey of API development. This preparaion stage can highly influence the smoothness and efficiency of the entire designing and implementing process. By establishing a well-organized environment, you enhance productivity and reduce common coding errors early. It involves selecting crucial tools and libraries to work with, along with a capable Integrated Development Environment (IDE) that maintains the workflow regular and straightforward.
Essential Tools and Libraries
Virtual Environments
Virtual environments play a vital role in Python API development. They allow you to create isolated environments in which you can install packages independently from the system-level installations. This characteristic mitigates risks of version conflicts that may arise when different projects require different package versions. By managing dependencies more effectively, virtual environments keep your projects more maintainable.
A famous library for creating virtual environments in Python is , which comes built-in with Python 3. You can create a new virtual environment using a simple command in the terminal:
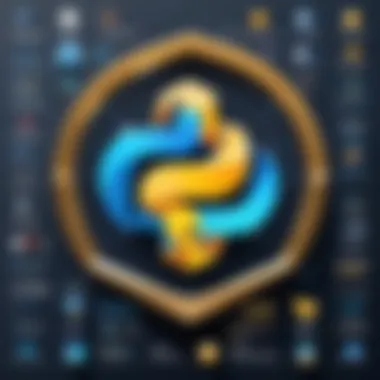
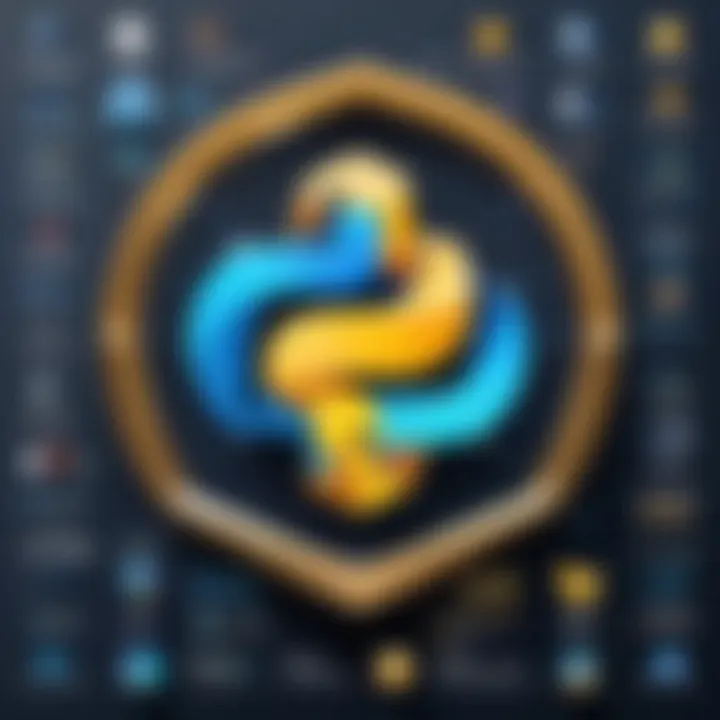
This creates an isolated environment named 'myenv'. However, it is also worth noting that your choice of a virtual environment library should reflect the complexity and scale of your projects and team dynamics. A few disadvantages involve an extra layer of abstraction, which some newcomers might find confusing at first.
Package Management with pip
Another significant component of creating a solid development environment is package management, mainly through the usage powered by . Pip simplifies the task of finding and installing Python packages from the Python Package Index (PyPI). Dependence management becomes increasingly easier when étions happen maintanance with pull for many libraries without worrying about others implementations.
You can make a file that lists all your project dependencies. Using the following command, you can install specified packages straight from this file:
The flexibility that pip provides makes it suitable for developers at every level, allowing for easy package configurations. Nevertheless, reliance on too many packages could lead to bloated project dependencies, so it's best to utilize only what's necessary.
Selecting an IDE for API Development
Choosing the right IDE can greatly impact your productivity during API development. A capable IDE serves multiple functionalities, including code suggestions, debugging tools, and project management interfaces. Finding one that suits your working style will streamline your workflow and provide a better coding experience.
Popular IDEs for Python
Some popular IDEs include PyCharm, Visual Studio Code, and Atom. PyCharm, developed by JetBrains, offers a dedicated environment specifically for Python. It contains intelligent code editors, strong debugging utilities, and project navigation.
Visual Studio Code, on the other hand, is lightweight yet incredibly powerful in functionality. Strong community extensions can customize it for any workflow needs, making it ideal for nearly any programming task, including API development. This flexibility remains a major strength of the IDE.
Each IDE choice comes with its respective strengths, but the learning installed might require some time; that's completely normal as each relate differently totally based on personal preferences and objectives during the developmental stradegy.
Configuration Recommendations
Proper configuration of your IDE can help significanty in increasing productivity. At the star, ensuring the integrated terminal has the project’s virtual environment activated can aid in providing an immediate and connected programming atmosphere, that keeps tasks orderly. Versatility in themes and optimally aligning toolbars for favorites will redirect focus straight into tasks without many distractions.
Every configuration aspect, if aligned according to personal mental flow could define clarity in finding necessities lacks unnecessarily struggling to trackapp functionalities. Using features, such as keyboard shortcuts and multi-row code edits, boosts workflow and minimizes friction, assisting developers focus mainly on coding.
Clear set up of your Python development environment can prevent multitude of mistakes and misconfigurations in throughout remaining journey in ineffective project builds.
Choosing the Right Framework
Selecting the appropriate framework for API development in Python is a critical decision. It shapes how effectively the project meets its objectives and how maintainable it becomes. Each framework brings its own set of benefits, trade-offs, and learning curves. When making this choice, several considerations play an important role:
- Project Objectives: The complexity of the API being developed can dictate the need for either a lightweight or a more robust framework. Frameworks like Flask may serve relatively simple applications well, while Django offers comprehensive features suitable for more intricate projects.
- Community and Support: Well-established frameworks often have extensive community support and better documentation. Flask and Django enjoy large active communities that facilitate troubleshooting and offer numerous third-party libraries.
- Performance and Scalability: Some frameworks may have basic performance advantages. FastAPI is known for its asynchronous capabilities that improve responsiveness under load. Careful evaluation based on anticipated API traffic can save significant refactoring work in the later stages.
Understanding these factors enables developers to make informed decisions that will affect not only the initial development but also the future prospects of the API. In the following sections, we’ll closely examine two key frameworks: Flask and Django, and provide insight into alternatives like FastAPI and Tornado.
Flask vs.
Django for API Development
Both Flask and Django have emerged as front-runners in the world of Python web development. Each presents its distinct qualities that cater to different types of projects.
Flask is a micro-framework, promoted for its simplicity and lightweight nature. It is flexible and allows developers to implement only necessary components, making it ideal for those starting with APIs. However, this can lead to increased complexity as the application scales unless the right structures are put in place from the start.
On the other hand, Django provides a full-featured framework. Its structure offers a built-in ORM, authentication framework, and admin panel. This helps developers launch fully functional APIs quickly but can result in added bloat for those needing just simple functionality. Django is beneficial for team projects requiring uniformity and quick scaling due to its consistent practices and patterns.
Other Frameworks to Consider
FastAPI
FastAPI has gained rapid popularity due to its support for asynchronous programming and automatic generation of interactive API documentation. It utilizes modern Python features like type hints to ensure elegant and maintainable code. FastAPI emphasizes performance, often outperforming older frameworks. This speed coupled with automatic request validation attracts professionals focused on cutting-edge technologies. Moreover, the easy integration with asynchronous libraries opens significant opportunities for enhancing API efficiency.
- Key Characteristic: The ability to perform asynchronous operations.
- Unique Feature: Automatic generation of interactive API docs.
- Advantages: High performance, swift deployment recognition, and ease in handling high requests.
- Disadvantages: A relatively new framework might equate to lesser community resources compared to Flask/Django.
Tornado
Tornado is another consideration that stands out for its ability to handle a large number of simultaneous connections. It is particularly preferred for WebSocket APIs or applications needing long-lived connections due to its non-blocking network I/O. Tornado shines in scenarios requiring real-time functionalities.
- Key Characteristic: Handling thousands of open connections just lessthan traditional frameworks.
- Unique Feature: Its non-blocking mechanisms that make it suitable for WebSocket applications or long polling tasks.
- Advantages: Robust performance for real-time applications.
- Disadvantages: More complex to set up compared to Flask and could be overkill for simpler endpoints.
Knowing these frameworks' specific aspects will guide developers depending on their project requirements and help ensure they make an educated decision in API development utilizing Python.
Designing Your API
Designing your API is a crucial phase in the development process. Effective design involves defining how the API will function and how it will respond to user requests. Focused planning leads to better maintainability and user experience. Logical design minimizes code accidents and reduces bugs. As you explore specific elements, consider usability, efficiency, and clarity.
Defining Endpoints and Resources
Endpoints serve as points of interaction in your API, representing various resources. When defining endpoints, it is vital to think about how they align with the underlying data model. Each endpoint should correspond meaningfully to operations on the data. Use clear naming conventions to imitate standard database interactions. Some best practices include:
- Use nouns for resource names. For instance, endpoints such as or are easily understood.
- Adopt a hierarchical structure. Instead of having flat structures, use nested patterns like .
- Consistent 'versioning.' Indicate version in the URL like . This approach will help avoid disruption when you update your API.
This clear definition offers both developers and users a strong understanding of how to work with your API and effectively manage resources.
Implementing RESTful Principles
Adopting RESTful principles in API design ensures that the interfaces are stateless, cacheable, and uniformly defined. REST, or Representational State Transfer, centers around resources and utilizes standard HTTP methods like GET, POST, PUT, and DELETE. Here’s how these principles benefit your API design:
- Statelessness: Each request from the client should contain all necessary information for the server to fulfill that request. This reduces server complexity and enhances scalability.
- Uniform Interface: Limiting the architectural constraints helps to allow the et of APIs to function in a coherent manner. It ensures users approach API integrations uniformly.
- Cacheability: Improve performance by enabling clients to cache responses. If responses are cacheable, clients can reuse stored responses to reduce extensive server requests.
Applying these RESTful criteria creates an intuitive API that enhances usability and encourages efficient interaction among users, making it vital for any modern web application.
In summary, a well-designed API ensures smooth interactions and enhances overall application communication. Prioritize thoughtfulness in endpoint definition and RESTful adherence, which cultivates a robust API architecture.
Implementing API Functionality
Implementing API functionality is a critical step in the development process. This section focuses on the immediate interaction between API users and the backend. It encompasses how clients send requests, how servers process these requests, and how responses are delivered back to clients. By ensuring that API functionality is correctly implemented, developers can streamline user experiences and enable applications to perform efficiently.
The complexity involved in managing HTTP requests and data serialization has far-reaching implications on performance. It is vital to grasp these elements to ensure a reliable and high-performing API. Challenges such as handling data formats, content negotiation, and error handling are common. Thus, understanding the nuances of these topics will equip developers with the skills to build robust APIs.
Handling HTTP Requests
HTTP requests are the backbone of API communication. When a client initiates a request, it relies on the HTTP protocol to communicate with the server. Each request includes specific information like method (GET, POST, PUT, DELETE), headers, and potentially a body with additional data.
From a Python perspective, the popular library provides a simple way to handle HTTP requests. This library allows developers to construct requests without needing to manage the underlying details of sockets or network configurations.
Here’s a slight overview of HTTP methods commonly used in APIs:
- GET: Retrieve data from the server. The client does not send data; it only asks for information.
- POST: Submit data to the server. Typically used for creating new resources.
- PUT: Update existing resources or create them if they do not exist.
- DELETE: Remove a specified resource from the server.
By effectively handling HTTP requests, developers can ensure that users can access and manipulate resources seamlessly.
Data Serialization Techniques
Data serialization is another key element in API functionality. It transforms complex data structures into a format suitable for sending over the network. This is crucial because APIs typically communicate using strings since network protocols are based on text.
There are two commonly used formats for data serialization in APIs: JSON and XML.
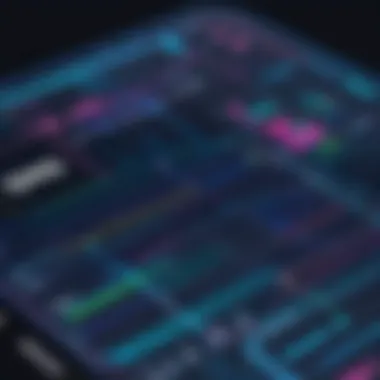
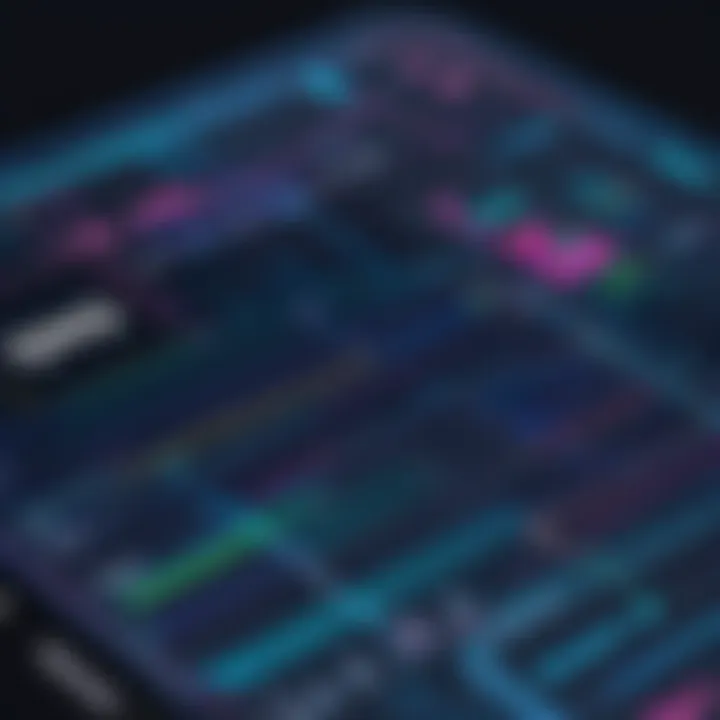
Using JSON
JSON, or JavaScript Object Notation, is a lightweight format popular in web APIs. Its main characteristic is its simplicity and readability. Because JSON is easy to write and parse, it has become the go-to choice for most modern APIs.
The unique feature of JSON is its ability to represent data structures using human-readable text, which makes it advantageous for debugging. Furthermore, since many programming languages can easily parse JSON—including Python—it facilitates the interchange of data between clients and servers with minimal friction.
However, while JSON has many advantages, there are limitations. For instance, representation of more complex data types, such as data models or date objects, can be less straightforward. Additionally, sometimes it lacks built-in support for namespaces, which can complicate matters in larger data exchanges.
Using
XML, or eXtensible Markup Language, is another common format used for data serialization. It offers a more verbose structure, facilitating the representation of complex data and hierarchical relationships. This has made XML a popular choice for systems that require document validation and schema definitions.
One of its distinguishing features is the ability to integrate additional meta-information using attributes, making it more verbose than JSON. XML also supports namespaces effectively, allowing more significant diversity in data representations.
Nevertheless, the advantages of XML come at a cost. Its verbose nature can lead to increased payload size, making it less efficient compared to JSON in most typical use cases. Due to its complexity, XML is generally not the preferred format for simple APIs where speed and size are priorities.
In summary, decoding data serialization techniques and handling HTTP requests are fundamental components of API functionality in Python development. Understanding these aspects leads to more effective and sophisticated API solutions.
Testing Your API
Testing is a crucial component in the API development life-cycle. Effective testing ensures that your API functions correctly and meets user expectations. This includes verifying that endpoints return accurate data, perform effectively under different conditions, and are secure from vulnerabilities. By employing rigorous testing procedures, developers can identify issues early, enabling smoother functionality and improved user satisfaction. Moreover, regular testing aids in keeping the software aligned with evolving business requirements and technology trends.
Unit Testing Strategies
Using pytest
pytest is a widely adopted testing framework for Python that simplifies API unit testing. Its flexibility allows testers not just to verify code functionality but also to produce detailed output for debugging purposes. A standout feature is its extensive use of plugins to extend the core functionality, making it adaptable for various projects.
In API testing, pytest supports the creation of modular tests that can be reused across different test cases. This reduces redundancy and quickens the testing process.
Another reason why pytest is popular is its ability to manage test environments with minimal configuration, making it an accessible choice for beginners and experts alike. Its vibrant community also contributes to numerous libraries that enhance its capabilities, providing good documentation and resources.
Mocking External Requests
Mocking external requests is a best practice in API testing that prevents dependence on external services, allowing for more controlled test environments. This methodology involves simulating responses from third-party services, enabling developers to test an API's integration points without dealing with live data or unforeseen outages.
By utilizing libraries like unittest.mock in Python, developers can create precise replicas of external responses necessary for testing without needing the actual API to function. This approach has a notable advantage: it can greatly reduce test time by eliminating network dependency.
However, one drawback includes the potential distinction between mocked responses and live environments, which might lead to a disconnect over time. Constant updates and sophisticated mocks are necessary for keeping tests relevant and useful, yet the benefit of having faster and reliable tests generally outweighs the challenges involved.
Integration Testing Approaches
Integration testing focuses on how different parts of an API work together seamlessly. This aspect ensures that the components are compatible and meet intended functionality, dedicating efforts toward verifying the interaction pathways across system parts. Ensuring coherence between multiple microservices and verifying the end-to-end scenarios become vital tasks here.
Units may pass individual tests while integration tests reveal clashes not evident in isolation. Techniques for integration testing embrace real-world simulations to evaluate user interactions with the API, enhancing the effıcacy of the API's deployment significantly. Proper implementation of this phase contributes to reliable systems in production environments.
Documenting Your API
Documentation is a vital aspect of API development. A well-documented API ensures that users, whether they are developers or end-users, can understand how to integrate and interact with it. Without clear documents, using the API can quickly become a frustrating endeavor. It sets the expectation of what functionality exists, how it operates, and what security measures are in place. Additionally, proper documentation can reduce support requests and enhance user experience. In essence, it serves as a roadmap for anyone looking to engage with the API.
Importance of Documentation
Documentation plays a crucial role for several reasons. Above all, it promotes usability. When users can quickly locate and understand how to use the API, they are more likely to adopt it in their work. Clarity in documentation reduces the learning curve, allowing new users to start interacting within a short time frame. Furthermore, it contributes to error reduction. Clear outlines for input and output formats prevent misunderstandings that could lead to coding errors.
Moreover, it enables easier onboarding for teams. When you bring in new developers, having detailed records of functionalities, endpoints, and data structures aids them in getting up to speed effectively. An additional noteworthy factor is the implications for maintainability of the project. Updating existing systems usually involves revisiting the API. Having a solid documentation base can facilitate straightforward updates and maintenance processes.
Tools for API Documentation
Various tools exist to assist in the API documentation process. Each of these tools offers unique features that cater to specific needs.
Swagger
Swagger effectively streamlines the documentation process. It provides an interactive playground where developers can not only view documentation but also test endpoints directly. A key characteristic of Swagger is its ability to automatically generate documentation based on the code. This feature greatly simplifies maintaining records as subsequent changes in code automatically reflect in the documentation.
- Unique Feature: Swagger’s interactive UI allows real-time feedback, making it easier for developers to understand how to use the tools provided.
- Advantages: Its automatic generation feature means less manual input and error potential, which aids productivity.
- Disadvantages: Swagger’s heavy reliance on certain formats can be limiting in diverse implementation scenarios.
Postman
Postman serves as a versatile tool that reinforces the documentation process. Not only does it allow for API testing, but it also provides documentation generation capabilities. A core aspect of Postman is its collaboration feature, which enables teams to engage with API projects in a shared environment. All workers can provide insights into collective documentation, allowing for a more comprehensive and clear API structure.
- Unique Feature: The ability to synchronize documentation with the development team in real-time promotes reliability.
- Advantages: Its user-friendly interface lets even less experienced developers generate documents easily.
- Disadvantages: Some users may find that relying heavily on Postman might not integrate as smoothly with all development environments, affecting efficiency on larger projects.
Proper documentation can drastically improve efficiency in API adoption and maintenance. It remains an investment in your API’s usability, reliability, and existing performance.
Deploying Your API
Deploying an API is a crucial step in the development process. This part puts the API into a live environment, allowing it to be utilized by clients. It connects the final product with users or other applications. Understanding deployment strategies can significantly impact accessibility, content delivery, and response times. Selecting the right method and environment for deployment brings many benefits, including scalability, reliability, and efficient resource management. Many factors must be considered, such as the desired traffic load, maintenance effort, and budget constraints.
Selecting a Hosting Environment
Cloud Services
Cloud services present a valuable option for hosting APIs. Providers like Amazon Web Services, Google Cloud Platform, and Microsoft Azure have made significant advancements in providing robust, scalable solutions for deployment. The key characteristic of cloud services is their ability to offer on-demand resources. Developers can scale resources up or down according to their needs with little hassle.
A unique feature of these services is flexibility. This means that developers can manage their applications explicitly based on traffic trends without heavy investments in hardware. The advantages of using cloud services include reduced costs, improved uptime, and a global presence through data centers. However, there are disadvantages, mainly concerning data privacy and potential vendor lock-in. Before commitment, it is vital for teams to evaluate these aspects thoroughly.
On-Premises Options
On-premises hosting involves deploying the API on hardware that is physically located within an organization. This option can be appealing for businesses that prioritize security or have strict compliance requirements. One significant trait of on-premises options is control; organizations maintain complete oversight over their infrastructure, which many believe translates to enhanced security and performance.
A unique feature here is the customizability. Businesses can create an environment fit specifically for their applications or industry needs. However, this option has downsides, particularly in terms of high initial costs and ongoing maintenance challenges. Overall, companies need to weigh these advantages against the requirements of their projects when considering on-premises solutions.
Continuous Deployment Practices
Continuous deployment is a software development practice where changes to an API are automatically deployed to production after passing specific tests. This approach ensures that updates are frequent and integration is seamless. Using tools like Jenkins, CircleCI, or GitHub Actions, developers can facilitate continuous integration or delivery.
Implementing continuous deployment brings notable benefits. Firstly, it allows for rapid development cycles, enabling teams to adapt quickly based on user feedback. Secondly, it aids in consistent quality, providing a safety net through automatic testing processes that identify issues before deployment.
A primary consideration in this practice is infrastructure: an automated deployment pipeline requires thorough configuration and sometimes a robust architecture to ensure seamless operations. Teams must balance the pursuit of speed with the quality assurance needed for successful API deployments.
Securing Your API
API security is crucial for maintaining the integrity and confidentiality of the data exchanged between clients and servers. With the increasing prevalence of cyberattacks, it is essential to reinforce your API against possible vulnerabilities. This section examines common security threats lurking in the realm of API development and offers best practices for solidifying defenses.
Common Security Threats
APIs, by their nature, expose functionalities and data to the outside world. This accessibility, while advantageous, invites diverse security threats.
- Injection Attacks: These occur when an attacker sends malicious code through an API. This code can manipulate back-end databases or leverage resources, often leading to unauthorized data access or service disruption.
- Authentication Breaches: Weak or improperly implemented authentication mechanisms can allow attackers to impersonate legitimate users, resulting in unauthorized actions on the API.
- Insufficient Rate Limiting: Without proper controls, an API can become overwhelmed by excessive requests which may lead to denial-of-service attacks.
- Data Exposure: Inadequate safeguards around data can lead to sensitive information leakage. Operative points like response data must be correctly sanitized.
- Man-In-The-Middle (MITM) Attacks: Here, attackers intercept data exchanges, allowing them to read or modify the information being exchanged between parties.
Detecting and addressing these threats falls within prudent API development practices.
Best Practices for API Security
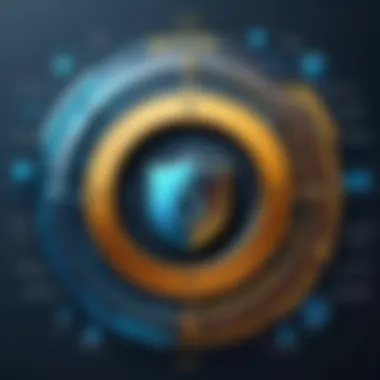
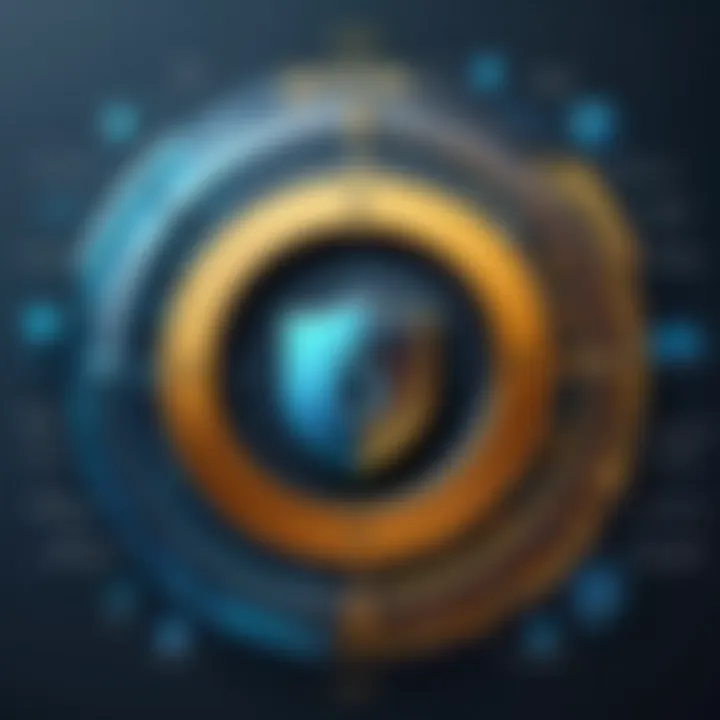
Implementing rigorous security protocols is indispensable. Key strategies can fortify API interfaces against threats.
Authentication Mechanisms
Robust authentication mechanisms can serve as a formidable barrier against unauthorized access. Typically, these systems utilize standards such as OAuth 2.0 or JSON Web Tokens (JWT).
- Key Characteristic: The decentralized nature of OAuth 2.0 facilitates not just user guest functionalities but also scope limitations. Many experienced developers tend to opt for OAuth 2.0 given its proven framework and widespread use.
- Unique Feature: With OAuth 2.0, resource owners grant third-party applications limited access to their resources without exposing their credentials. This offers flexibility for app interactions and leads to better security in multi-tenant environments.
- Advantages/Disadvantages: While OAuth 2.0 brings robust authentication, it necessitates meticulous implementation to prevent risks associated with tokens. JWTs also introduce the potential for token tampering but can be configured securely if crafted with a key that remains secret.
Rate Limiting
Implementation of rate limiting represents another strong line of defense. By controlling how frequently clients can call the API, it helps ensure resource usage remains within sustainable bounds.
- Key Characteristic: This strategy enables developers to define a ceiling for API requests per user within a specific timeframe.
- Unique Feature: If users reach their limit, the API can deliver error messages, informing them they have been rate-limited. This provides substantive feedback while safeguarding resources and user experience.
- Advantages/Disadvantages: Rate limiting genuinely curbs abuse since it manages server load and interaction levels. However, misplaced limits on legitimate users can hinder use cases, such as real-time applications.
Governments of developer ecosystems universally recognize the weight of API security. Vigilant observance of recommended practices and hardening approaches enhances safe and effective API interaction. By continuously monitoring and revising API security protocols, developers ensure compliance to evolving technologies and threat vectors.
Monitoring and Maintaining Your API
Monitoring and maintaining an API is crucial in ensuring its functionality and performance over time. An API operates as a gateway, facilitating communication between different systems or applications. If left unmonitored, the API can develop issues that may affect user experience or lead to downtime.
The benefits of diligent monitoring are numerous. Firstly, it aids in identifying performance bottlenecks. For example, if an API slows down, the root cause can often be determined through effective monitoring techniques. Secondly, it helps in ensuring uptime. An API needs to be accessible; downtime translates to lost opportunities and revenue. Regular maintenance practices can prevent these issues before they escalate.
Additionally, security considerations come into play. Monitoring an API can reveal any fraudulent activities, unauthorized access attempts, or data breaches. Such insights are essential, especially in an era when cyber threats are increasingly sophisticated. Furthermore, an observant development team can adapt more swiftly to user demands or changing conditions from upstream APIs. Adjusting the monitoring strategy is often necessary to cater to changing business requirements.
Regularly monitoring your API not only proves how effective it is but also ensures it meets user expectations and business needs.
Tools for API Monitoring
Various tools are available for API monitoring, each tailored to cater specific needs. Popular choices include:
- Postman: Primarily known for testing, it also offers features for monitoring APIs.
- New Relic: This tool provides insights into front-end performance and server-side metrics, making it vital for comprehensive API health monitoring.
- Prometheus: An open-source monitoring system that captures metrics from configured targets at specified intervals, providing a flexible means to monitor APIs.
- Datadog: A cloud monitoring platform that connects with various applications, offering the ability to visualize metrics and set alerts.
Choosing the right tool depends on organizational needs. Understanding what metrics to monitor is equally important, be it uptime, response times, or throughput.
Performance Optimization Techniques
Ensuring optimal performance of an API is an ongoing task. Several techniques can be employed to achieve this. First, caching can significantly reduce load times and improve user experience. Solutions like Redis or Memcached store data that can be reused, minimizing the need to repeat prior calculations or fetch data from a database repeatedly.
Improving load balancing can also optimize an API's performance by distributing workload efficiently among multiple servers. Tools such as NGINX can be beneficial here.
Moreover, employing throttling practices helps in restricting the number of requests a user can make to an API in a certain timeframe. This prevents abuse and improves reliability for all users.
Moreover, it is essential to continuously evaluate and refine the API’s underlying code to enhance performance efficiency. Engaging in code reviews can detect potential exploits or sluggish areas that need further optimization, leading to an overall more resilient API.
In summary, constant monitoring combined with sophisticated optimization techniques can ensure that an API remains robust and effective.
Real-World Use Cases
In the realm of API development, real-world use cases serve as a critical intersection between theoretical knowledge and practical application. APIs are no longer just technical components; they are essential facilitators of functionality in a diverse range of applications, enabling seamless data exchange and interactivity. Understanding successful use cases informs developers about possible integrations, problem-solving strategies, and industry trends, ultimately contributing to more robust and efficient systems.
APIs enable organizations to connect and extend their services, engage users effectively, and improve their operational workflows. Here are some key elements that outline the importaance of real-world use cases:
- Demonstrate Effectiveness: Concrete examples of API implementations show what can be achieved, thus guiding developers in creating effective solutions.
- Highlight Best Practices: Analyzing use cases showcases methodologies that have worked well in real life, giving insight into time-saving techniques and common pitfalls to avoid.
- Industry Insights: Contextual knowledge from various industries assists developers in modeling their projects according to existing standards and practices.
"The costs in time and money for building and managing an API pales in comparison to the advantages gained in agility, flexibility, and performance."
Real-world use cases illuminate not just the results but also the strategic thinking behind successful implementations, proving invaluable across multiple scenarios.
Successful API Implementations
When examining successful API implementations, several instances stand out in their ability to bridge gaps between complex systems while offering tangible value. Technologies like Twitter's API have fostered a rich developer ecosystem, enabling countless applications to connect with Twitter. Businesses leverage such functionality to boost user engagement and streamline heat to their channels:
- Twitter API: This API allows developers to access Twitter data for analytics, marketing, and social engagement, displaying how accessible data can stimulate innovation.
- Google Maps API: Many businesses use this service for location-based functionalities. Integrated into apps, it accommodates numerous requests, displaying real-time map information, routing, and geographic context directly within user applications.
- Stripe API: Stripe offers payment processing solutions that simplify transactions. Businesses adopt this to enhance their commerce platforms with built-in checkout experiences.
Such use cases demonstrate that real-world API implementations are vital resources for reinforcing best practices and fostering innovation. They are examples to enable understanding of various applications within different niches.
Case Studies in Various Industries
API utility spans across a broad spectrum of sectors. Distilling insights from actual scenarios can reveal much about functional nuances and cross-industry applicability:
- E-commerce: Companies like Shopify harness APIs for third-party app integration. This approach enhances their core services by allowing additional functionalities like loyalty programs or AI-generated recommendations.
- Finance: Santander has developed APIs for granting developers access to bank functionalities like account information and payment processing. Integrating security layers keeps customer data safe while extending service offerings in FinTech.
- Healthcare: The Epic Systems’ API showcases how healthcare organizations facilitate secure, interoperable data sharing across entities, improving patient care and administrative efficiencies through integrated health data.
- Social Media: Instagram's API allows developers to manage content while maintaining attribution, a scenario that brings commercial opportunities to creators by tying their emphasis on engagement metrics to robust reporting capabilities.
Each of these case studies clearly exhibits diverse API implementations impacting substantial areas. By exploring them, IS professionals get well-rounded templates applicable in their projects, helping in grasping the necessary landscape for innovation and improvement.
Future Trends in API Development
Understanding future trends in API development is crucial for developers and organizations that rely on APIs for their operations. As the digital landscape is continually evolving, APIs are becoming increasingly central to business strategies. Insights into upcoming trends help developers make informed decisions, optimize workflows, and ensure seamless integrations. Below, we will discuss significant trends shaping the future of API development, focusing on microservices and emerging technologies.
The Role of Microservices
Microservices architecture represents a shift from traditional monolithic applications towards a more agile and scalable software development approach. This style breaks down applications into smaller, independent services.
Here are some key aspects of the role of microservices in API development:
- Enhanced Flexibility: By decentralizing functionalities, microservices allow for better flexibility in updating or replacing specific components without disturbing larger systems.
- Improved Scalability: Individual microservices can be scaled based on demand, optimizing resource usage and response times.
- Faster Deployment: Smaller codebases can be released more frequently, leading to rapid innovation cycles and reduced time-to-market.
- Technology Diversity: Development teams can choose different technologies or programming languages best suited to specific microservices, promoting efficiency.
However, in adopting microservices, there are core considerations such as managing inter-service communication, ensuring data consistency, and handling large-scale deployments effectively. Robust API documentation and monitoring tools play an essential role in overcoming these challenges.
Microservices architecture enhances software development by providing a scalable, flexible, and efficient framework that adapts easily to changes in business requirements.
Emerging Technologies and Standards
Staying up to date with emerging technologies and standards in API development is essential. As new tools develop, so do the capabilities they offer. Below are several pivotal trends:
- OpenAPI Specification: This standard continues to gain traction, empowering developers to define RESTful API services with great clarity, enhancing communication across teams.
- GraphQL: Unlike traditional REST APIs, GraphQL gives clients the power to request just the data they need. This flexibility fosters performance improvements and a better developer experience.
- API Gateways: These act as intermediaries, securing and managing API traffic. Their implementation is crucial for monitoring, logging, authentication and can provide usage analytics for each API endpoint.
- AI-Driven APIs: The growth of artificial intelligence and machine learning is sparking the creation of more intelligent APIs. Businesses will utilize such services for predictive analytics, user personalization, and automating processes.
- Containerization Technologies: Docker and Kubernetes support microservices by ensuring consistent environments, making deployments faster while improving DevOps practices.
As these technologies evolve, developers must remain vigilant and adaptable. Continuous learning will be key to effectively utilizing these trends within API development projects. This vigilance is critical not just for technical proficiencies but also for maintaining competitive advantages in today's market.
Finale
In this final section of the guide, we consolidate the understandings gleaned from earlier discussions on API development utilizing Python. The importance of comprehending an effective API structure becomes evident as we navigate the digital landscape, especially for those engaged in the fields of software engineering and IT. With firms increasingly relying on APIs to facilitate seamless integration and interaction between disparate systems, having a proficient grasp of API development in Python is pivotal.
One key element emphasized throughout this guide is the versatility of Python itself. Whether one is crafting robust back-end services or employing high-level abstractions through frameworks like Flask or Django, Python grants developers the tools necessary to innovate quickly and efficiently. Furthermore, understanding how to document, test, and secure APIs stands as just as significant as designing endpoints. Security measures like rate limiting and authentication help protect valuable data.
By now, readers should be mindful of best practices surrounding RESTful principles, data serialization methods, and testing strategies. Mastery of these concepts affords developers the ability to build scalable and functional services tailored for a variety of applications. API optimization is another central aspect, ensuring high performance and response times in live environments.
Merging functional implementation with pragmatic security while deploying solutions forms the crux of a successful API architecture. The information conveyed throughout the article offers ample resources and techniques to achieve such integration effectively, with insights that cater to the unique demands of modern programming scenarios.
"Understanding the fundamentals of API development in Python enables developers to adapt to challenging demands in tech, distinguish themselves consistently in their skills, and deliver outstanding solutions to their audience."
Lastly, as the technology playground evolves, adapting one's approach towards API development stands as essential for all programmers. Keeping pace with emerging trends, enhancing knowledge through practice, and establishing strong development routines continues to plot accurate career pathways forward.
Summary of Key Points
- Understanding API types like REST and GraphQL is crucial for effective development.
- Python stands out for its ease of use, rich libraries, and powerful frameworks suitable for various API architectures.
- Proper documentation aids usability and support, essential for public-facing APIs.
- Security best practices greatly minimize risks for both the API solutions as well as user data.
- Monitoring and optimization are vital for performance in production settings.
Call to Action for Developers
As you close this comprehensive overview, consider taking actionable steps to reinforce your learned skills. Get creative with small projects built on the concepts discussed. Initiate frameworks with Flask or Django, explore data serialization via JSON, and do not neglect the implementation of security measures throughout your processes. Keep pushing the boundaries of your understanding. Explore communities like Reddit for forums on specifics and trends in API development.
The market constantly evolves with technology. Thus, staying current and sharpening your expertise progressively becomes a continual voyage. Participate in open-source contributions, reading industry-relevant articles, and partnering in collaborative projects. Such initiatives may position you advantageously in a competitive landscape, yielding opportunities for gathering indispensable industry insights and further refining your coding acumen.