Mastering Background Blur Techniques in Android Apps
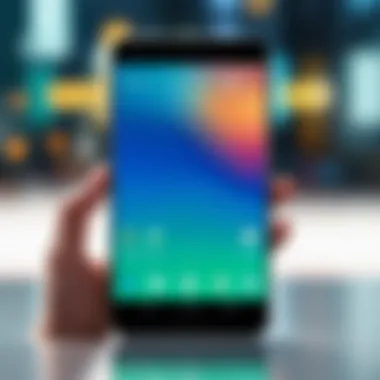
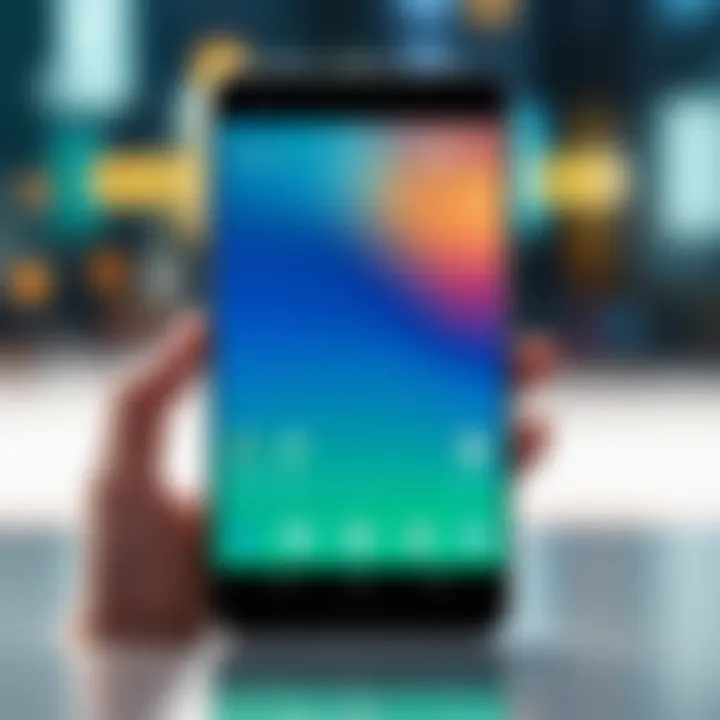
Overview of Topic
Blurring background images is an essential technique in Android development. It improves user experience and elevates the aesthetic quality of applications. This subject is relevant for applications requiring focus on foreground elements while subtly integrating the background. Understanding various methods of achieving this effect can significantly enhance visual designs, thus increasing user engagement.
Historically, image blurring techniques have evolved with advancements in both hardware and software capabilities. Early methods employed simple algorithms with basic effects. More recently, modern libraries and frameworks offer sophisticated solutions that cater to high-quality image processing and user interface design.
Fundamentals Explained
To effectively implement blurring, developers must grasp the core principles of image processing. At its core, blurring is achieved by averaging pixel values within a certain area. Key terminology includes:
- Bitmap: An image file format that stores pixels in a grid.
- Gaussian Blur: A common method of blurring, derived from the equation of the Gaussian function.
- Alpha Channel: Refers to the luminosity of each pixel, influencing transparency and blending decisions.
These concepts lay the foundation for practical applications. Familiarity with various algorithms related to pixel manipulation is essential.
Practical Applications and Examples
Real-world applications of blurred images include creating elegant UI elements and enhancing focus on content by de-emphasizing background details. Examples are text overlays where background clutter needs softening. Implementation often involves using libraries such as Android’s RenderScript or OpenGL for performance optimization.
Here’s a code snippet for using RenderScript to blur a bitmap:
Having clear demonstrations fosters a deeper understanding of how to adapt these techniques into real applications.
Advanced Topics and Latest Trends
Recent trends in Android development involve moving towards more GPU-driven and real-time performance enhancements. Developers are encouraged to explore new methods that harness machine learning approaches for smarter blurring effects. This includes the TensorFlow Lite model for background separation, allowing for dynamic adjustments based on context.
Additionally, there are ongoing discussions in developer communities about optimizing existing methods to balance output quality and processing demands. Techniques like fast Gaussian blur have surfaced as they're designed to operate with reduced computational power while maintaining visual fidelity.
Tips and Resources for Further Learning
To deepen knowledge in this area, you may consider several resources:
- Books: “Learning Android Application Development”
- Online Courses: Websites like Coursera or Pluralsight offer comprehensive classes on Android design practices.
- Tools and Libraries: Experiment with libraries such as Picasso or Glide for image handling in applications.
Updated methodologies and best practices can be frequently sourced from forums like Reddit and also through documentation on Wikipedia.
By investing time in understanding and applying advanced blurring techniques, developers can noticeably elevate application aesthetics and enhance overall user experience.
Understanding Image Backgrounds in Android
The effective use of images in Android applications is crucial for creating an engaging user experience. Background images can enhance aesthetics and encapsulate the essence of an application. Developers must understand this aspect as it serves as the foundation for visual layout and affects user interactions. Selecting the appropriate background can significantly impact how users perceive an app. A well-crafted background lays the groundwork for elements placed on top, ensuring that interfaces are not only functional but also cohesive.
The Importance of Background Images
Background images play a vital role in branding. An image can convey a message or evoke emotions associated with the business. Aesthetic appeal captivates users, enabling applications to stand out in an overcrowded market. Moreover, background images contribute to usability, guiding the user’s attention to primary content areas. The integration of appealing backgrounds can lead to better user engagement, helping in retaining users. The blend of design and usability influenced by proper background choices should not be overlooked.
Types of Background Images
When considering background images in Android applications, two primary categories arise: static and dynamic backgrounds. Each type has its unique attributes and implementations, which can facilitate diverse use cases. Understanding them thoroughly helps developers tailor their design strategies to effectively meet application goals.
Static Backgrounds
Static backgrounds are fixed images that remain unchanged during the app's interaction. Their key characteristic lies in simplicity. They offer a direct approach in design, making adhered them particularly appealing in many scenarios. Static backgrounds facilitate easy incorporation without requiring intensive resources for real-time changes. The distinct feature of static images is their reliability. They demand minimal processing power, enhancing performance on lower-end devices and conserving battery life.
However, static backgrounds may lack an element of motion that captivates users over time. This lack meticulous engagement can sometimes lead to users becoming accustomed to their surroundings. Care must be taken in their selection to ensure they harmonize well with other visual elements.
Dynamic Backgrounds
Dynamic backgrounds, in contrast, are images that shift or animate to provide a more immersive experience. This interactivity can engage users and create a rich visual context for the application. The main advantage of dynamic images lies in their ability to adapt and evolve, keeping content fresh and appealing. This responsiveness can turn a commonplace app into an extravagant experience, appealing to modern user expectations. Therefore, uses of dynamic backgrounds are crucial as they help setters for engaging experience.
However, dynamic backgrounds may come at the cost of increased resource usage. They can impose higher demands on processing power and memory, possibly affecting performance on less capable devices. Developers must strike a balance between visual impact and application performance when deciding which background type to use.
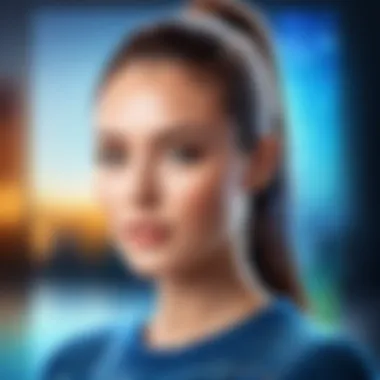
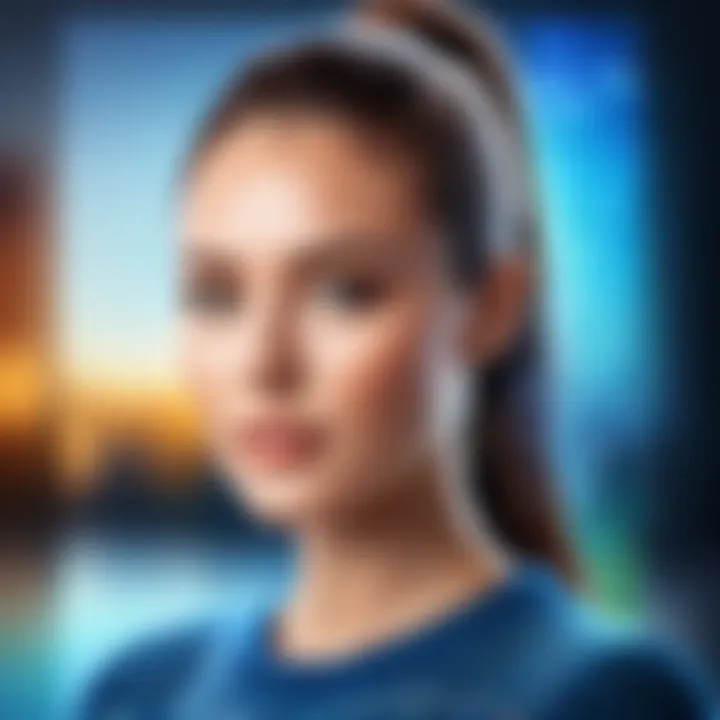
In summary, choosing the right type of background image is central to successfully enhance the Android application's experience and overall engagement with users.
Developers and designers should weigh the pros and cons of static versus dynamic backgrounds and select the one that aligns with the application's objectives.
Techniques to Blur Background Images
Blurring background images has become an aesthetically pleasing and practical solution in modern Android app development. It holds importance in improving user interfaces by providing visual depth without overwhelming the main content. Techniques available vary in complexity and purpose. They can cater to simple projects or more intricate designs.
Using Built-in Android Functions
Bitmap Processing
Bitmap Processing stands as a fundamental method within Android for manipulating images. This technique allows developers to edit pixels directly, making it an attractive option for image blurring. The key characteristic of bitmap processing is its low-level operation. This accommodates precise control over how the blur effect is applied. Due to its flexibility, many recognize this method as a beneficial choice for finer adjustments to image detail and dynamic manipulation in real-time.
However, Bitmap Processing can also present challenges. One major disadvantage is its higher memory demand, especially with larger images. Developers must manage memory efficiently to avoid application performance degradation. Overall, bitmap processing proves to be valuable for different image alteration strategies.
RenderScript Tool
RenderScript serves as a powerful Android tool for high-performance computation, particularly suitable for processing large images efficiently. It is recognized for harnessing multi-core processors, which drastically optimizes image blurring tasks. The unique feature of RenderScript allows it to execute focused image processing tasks in an optimized manner on different classes of hardware.
A primary advantage of using RenderScript includes enhanced processing speed. This, however, comes with a tradeoff. Developing with RenderScript can be complex, which some developers may find daunting. Therefore, evaluatng the right scenario for its use is vital in achieving seamless image manipulation within Android applications.
Employing External Libraries
Glide
Glide is a well-regarded image loading library that focuses on efficiency in delivering images onto display. It simplifies the process for developers when it comes to blurring images as part of the loading process. Glide excels because it handles caching automatically, which can enhance performance significantly during different states of image retrieval.
This library can also gracefully manage ongoing image transformations, which keeps the UI running smoothly. One must be cautious, though, as some may perceive Glide’s learning curve as challenging. When deep customization is needed, Glide may not provide the straightforward, customizable interface preferred for some specific uses.
Picasso
Picasso represents another popular image loading library designed to make the handling of image blurring easy. It is tailored for swift image rendering and supports various transformations. Picasso automatically adjusts image size and provides features like tag managing and placeholder indicating, making it user-friendly for many developers.
Despite its advantages, a few drawbacks should be taken into account. Picasso focuses primarily on operations requiring ease of use, and this might limit flexibility when drastically transforming images. It requires careful consideration for intricate effects and nuanced modifications. Understanding these tools supports developers realizing tailor-made results for their projects.
Implementation Steps
Creating the Android Project
Creating a new Android project is the first significant step in the process of blurring images. Establishing an organized project framework allows developers to manage code and resources effectively, leading to a more fluent development process.
Setting Up Gradle
Gradle serves as a powerful build automation tool and as a central place for managing project dependencies. Properly setting up Gradle is vital for obtaining libraries crucial to image processing. One key characteristic of Gradle is its biofacilitation of modular development, allowing developers to add specific libraries without extensive manual setup. For the task of blurring images, Gradle simplifies fetching libraries such as Glide or Picasso that support image manipulation. While Gradle brings convenience to dependency management, it can also slow down build times if not configured properly due to the extensive checks it performs. However, the advantages it presents in project structure and library integration outweigh the possible downsides if optimized correctly.
Configuring Manifest
Configuring the manifest in an Android project is essential for defined application behavior and property. The AndroidManifest.xml file describes components and permissions. It highlights the key characteristics of any project, including activities and their compatibility. By leveraging the configuration settings, developers can ensure that their applications possess the necessary permissions to access and modify images. This step shows its significance because improper manifest configurations can lead to runtime errors or unauthorized access issues. So while the manifest might seem trivial, it lays the groundwork for robust application features: if your manifest settings are incorrect, entire functionalities could fail to work. Therefore, careful attention during this stage results in a strong operational baseline for any application's development process.
Coding the Blur Functionality
After setting up the project structures, the next logical step is to focus on coding the functionality required to actually apply the blur effect on the images. This stage requires precision to deliver quality output.
Loading the Image
Loading an image is the first snippet of code to addressing the blur effects. Properly loading images dramatically influences how users will experience the application. Efficiently managing how images are brought into memory can greatly affect performance and responsiveness. Libraries like Glide are liked for their ease in image loading and caching. This characteristic is essential because slow loading times can frustrate users, disrupting their interaction with apps. Implementing adept image loading techniques like efficient caching is vital. Inefficient image loading can lead to memory management Java exceptions, crashing the app and leading to a negative user experience. Therefore, this step ensures both a starting visual engagement and maintains good app performance.
Applying the Blur Effect
Once an image is loaded, the blur effect needs to be applied accurately. The application of this functionality can radically enhance aesthetic appeal. By specifying parameters, such as the blur radius, developers shape how pronounced the blur will appear. This key aspect allows customization for various scenarios, making it more beneficial for creating nuanced visuals tailored to the individual application's context. Introducing external libraries into this process allows ease of implementation, exhibiting a notable unique feature in speed-to-market designs. It is important then to watch for disadvantages ccoming with outside library dependencies. If not managed correctly, future library updates could affect existing implementations, so keeping them under review is beneficial. Hence, safely applying the blur with well-defined code leads to both functional and visual success for developers’ ongoing projects.
Best Practices for Image Blurring
Blurring background images can be an effective way to enhance an application’s aesthetic appeal. However, it is crucial to approach the blurring with best practices that ensure both performance and visual quality remain intact. The importance of following best practices, especially in terms of memory management and maintaining a consistent user experience, cannot be overstated.
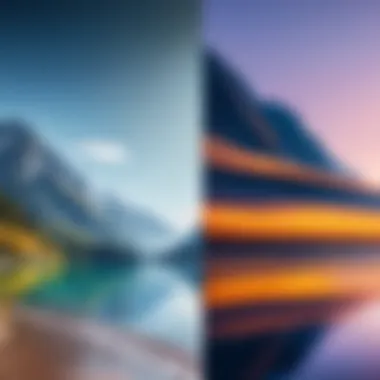
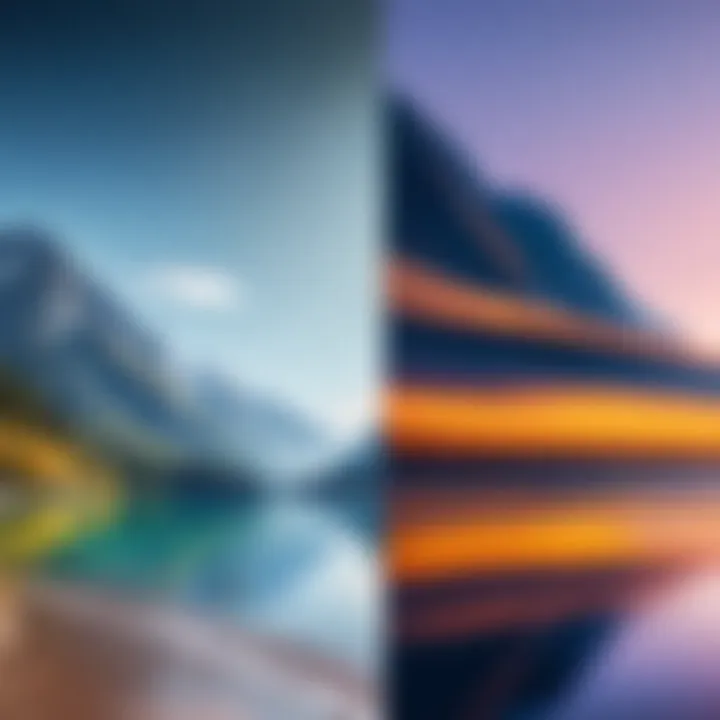
Performance Considerations
Memory Management
Memory management plays a pivotal role in the success of background image blurring. When you apply a blur effect to images, you often process large bitmap files. If the memory is not managed properly, it can lead to crashes or stuttering in applications. In Android, using optimized image sizes and formats reduces memory requirements significantly. A key characteristic is the need to unload images that are no longer required. This will free up memory and help maintain the optimal functioning of the app.
Effective memory management contributes to smoother performance and can greatly affect overall user satisfaction.
Unique features like Android’s built-in memory management tools can help avoid complications. They automatically handle image caching, optimizing RAM usage. However, one disadvantage is that developers may not have full control over these processes.
Processing Time
Processing time directly affects the responsiveness of apps. The time taken to apply the blur effect should be minimized to ensure that user interactions remain fluid. A common issue arises if high-resolution images are processed synchronously on the main thread, which can lead to noticeable delays. A beneficial strategy involves doing these processes asynchronously or using background services. This allows the UI to remain responsive while heavy lifting happens in the background.
Different image processing techniques impact processing time; for example, RenderScript can exploit multiple threads, which generally accelerates the blur operation. While this is beneficial, developers must be cautious, as this complexity can introduce performance bottlenecks if not managed properly.
Visual Consistency
Maintaining Style
Maintaining style through blurring effects ensures the application’s overall aesthetic is unified. A distinctive design language elements often revolves around image aesthetic consistency. If a blurred image differs in style inconsistently throughout the app, it can create disruption for users. Popularity arises from retaining certain elements, even when applying blur. Hence, design should be consistent, making it easier to recognize different parts of the application.
One unique feature of maintaining style is ensuring that blurred images fit seamlessly into any existing color palette or font style. A well-matched image creates continuity, increases user comfort and retains a clean appearance throughout.
User Experience
User experience cannot be compromised, even when aiming for a more visually stunning application. A well-blurred background can elevate focus on important UX elements, but if not done well, it can also impede usability. When blurring images, developers must keep in mind how the blur may affect readability and interaction with overlaid elements. It is important that the information presented remains legible and clickable.
Thus, unique features such as customizable opacity levels contribute significantly to user experience. Users find clickable elements easier to analyze when blurred images don’t create distracting visual obstacles. Yet, one must always weigh the advantages of aesthetics against usability when implementing blends.
Through clear attention to these detailed best practices, developers can effectively enhance their application’s design. Adhering to both performance and visual consistency principles ensures that blur effects optimize rather than diminish user experiences.
Troubleshooting Common Issues
Understanding the common issues that programmers face is essential for successful implementation. When blurring background images in Android, issues can lead to unmet visual objectives or stability problems. This section will discuss the primary challenges: ineffective blur effects and application crashes. We'll explore potential causes and remedies for each problem.
Ineffective Blur Effects
A key element is recognizing why blur effects might not function as expected. Several factors contribute, notably image quality and blur parameters.
Check Image Quality
Image quality directly impacts blur effect success. Low-resolution images often result in unsatisfactory blur, failing to produce the potent cohesive look every app desire. High-detail images ensure that details become subtly diffused but still recognizably appealing.
Moreover, practical coding decisions may hinge on file formats utilized. For example, .png formats maintain life-like quality better than .jpg files, which often lose clarity after texture change. Thus, selecting the proper image type is pivotal for good performance in this article. In summary, high-quality images lead to better blending results, making this aspect critical in Android image processing.
Adjust Blur Parameters
Adjusting blur parameters considerably affects how the final image appears. Parameters such as blur radius, must be modified depending on the image and desired outcome. A high radius provides heavier diffusion while a low radius preserves some detail.
Frequently, developers overlook fine-tuning values. An effective choice in this article is maintaining dynamic controls in your initial setup. The unique feature of fine parameters will call for adaptability as overall conditions might change. Tuning parameter settings lets developers adapt the intensity of effects in bearable settings, reducing the chances of failure.
Application Crashes
Application crashes represent a notable hurdle during development. Common factors contributing to crashes include unhandled errors and poor file management. Adequately diagnosing these errors is pivotal.
Inspect Logcat
Logcat serves as a crucial diagnostic tool in Android development. It provides real-time information about the ongoing operations including warnings, errors, and other pertinent logs related to the application.
Focusing on warnings or exceptions encountered during runtime helps developers hone in on problematic areas. Often, neglected insights can cascade into broader app maintenance challenges. Thus, incorporating Logcat inspection is an astute and footway to preemptively identify potential choke points in the application environment.
Review Code Implementation
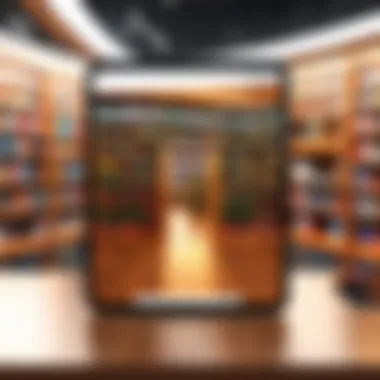
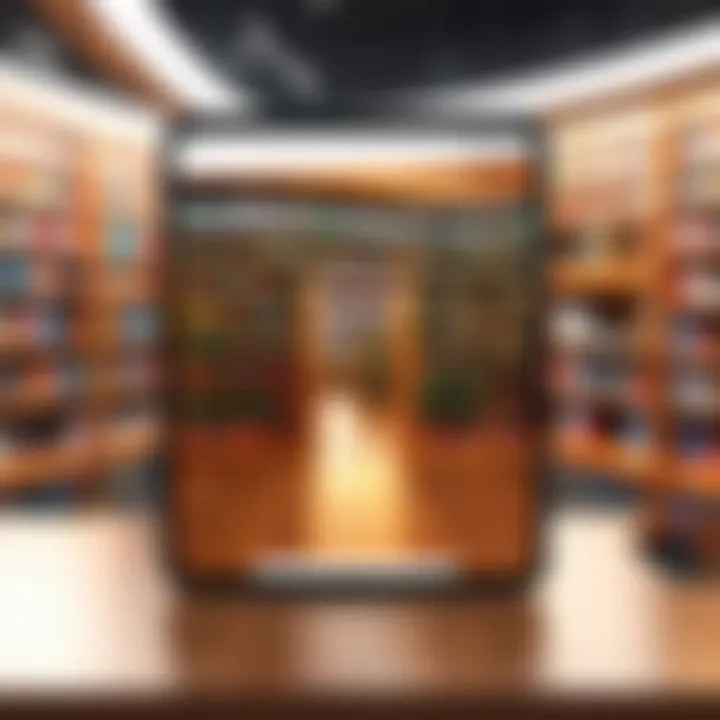
Reviewing the code implementation addresses concerns with any operation failure or crashes. It's beneficial to understand how one's code interacts and the logic underpinning operations runs through. Verifying method calls and data types can reveal primary coding errors.
Furthermore, clean and scalable code proves advantageous in debugging practices. As an intricate aspect, distinct segments gracefully connect rational thought to effective user experience: attention to structural choices greatly smooths the overall flow within projects. Proper reviews lead to budding clarity and robust app infrastructures in coding practices throughout this article.
Advanced Techniques
Understanding advanced techniques in blurring background images in Android applications is key for developers aiming to create an engaging user experience. These methodologies not only enhance the visual quality but also allow for adaptability to varied user interfaces and scenarios, making apps more appealing. Ignoring advanced techniques can limit the creativity and effectiveness that developers can bring to their national apps.
Creating Custom Blur Filters
Custom blur filters are potent tools for developers who want to implement unique and tailored blur effects. The key aspect of this functionality is providing users with flexibility over the blurring process, thus enabling an engaging interface while not oversaturating the visual output.
Kernel Methods
Kernel methods involves using convolution matrices to create a blurring effect. The importance of kernel methods lies in their flexibility. Developers can design a filter targeting specific areas or aspects of an image effectively.
The key characteristic is its precision. By modifying the kernel size and values, one can achieve anything from subtle softening to heavy distortions. The application of kernel methods often leads to realistic effects.
However, it comes with some disadvantages. Computationally, large kernel operations can prove taxing on the device, especially for complex images. Developers should factor this in during implementation. Balancing visual appeal with performance is crucial here.
Shader Programming
Shader programming is another essential aspect when considering advanced techniques. This method engages the graphics hardware directly, which leads to real-time effects with performance efficiency. This contribution can enhance animations and transitions significantly due to its design being lightweight and fast.
The key characteristic of shader programming is its real-time handling capability. This allows developers to directly manage how every pixel is rendered. This kind of control can lead to very creative output.
The downside surfaces depending on graphical hardware as not all devices uniformly support advanced shaders. Older or entry-level devices might struggle with complex shaders, impacting user experience adversely. This must be assessed ahead of launching applications.
Animations with Blurring
Integrating animations with blurring techniques can amplify user engagement and create smoother transitions across an application. Animation effects significantly complement background blurs, helping to maintain attention or guide users when navigating through different environments.
Transition Effects
Transition effects serve as a method of maintaining user attention while changing views within the application. These effects play a vital role because they reduce sudden changes and provide a smoother visual experience.
The key characteristic is that they can be seamlessly integrated, often enhancing the perceived speed and smoothness of the app. Such an approach can lead to heightened user satisfaction.
Positives include the strengthening of visual hierarchies, but there can also be negative aspects. Heavy use of transitions can become overwhelming and lead to distractions. Moderation is important here.
Dynamic Blurring
Dynamic blurring enables developers to have blur effects change based on user interaction or scene shifts. This method contributes to the goal of responsive design. Whenever the context changes, the blur adapts, enriching the visual narrative.
The key attribute here is its dynamism which offers more than just visual softness. Spaces become adaptable, resonating with user interactions in real-time.
On the flip side, this complexity can bring performance challenges for lower-end devices. Thorough testing must occur across devices to ensure uniform performance and visual effects.
Incorporating advanced techniques aids in achieving both aesthetic value and functionality within Android applications.
End
The importance of the conclusion in this article emphasizes the comprehensive nature of strategies to blur background images in Android. It serves as the final synthesis of knowledge, tying together various methodologies discussed in previous sections. Developers and learners alike benefit from understanding how different techniques and libraries can enhance their applications' aesthetics.
The process of blurring background images is essential for both user experience and visual appeal.
In recapping significant points, the conclusion highlights the effectiveness of using built-in Android features alongside popular libraries such as Glide and Picasso. Integrating these tools results in optimized performance without sacrificing visual quality.
The considerations regarding future trends in image processing are crucial. As technology evolves, developers must remain vigilant about new techniques and patterns that emerge within the realm of background blurring. These innovations can lead to enhanced user interfaces, changing how applications engage users and represent information. Taking interest in these inclusions allows developers to stay ahead of the curve in an ever-expanding field.
Recap of Important Points
- The significance of background blurring for visual impact and user experience in applications.
- Diverse tools and methodologies available, such as bitmap processing, RenderScript, and third-party libraries.
- Best practices ensure performance and quality.
- Troubleshooting common issues related to ineffective blur effects and app crashes.
- Advanced techniques exploring custom filter creation and animation integration.
Future Trends in Image Processing
Looking ahead, several trends are emerging in the field of image processing. These may involve:
- AI-driven image enhancements: Intelligent applications that automatically improve image quality and implement real-time blur effects.
- Augmented reality integrations: Using blurred backgrounds effectively can enhance user interactions within AR environments.
- More efficient processing methods: Keeping up correctness and semantics without heavy resource demands.
- Mobile GPU advancements: Further leveraging hardware improvements can make more complex implementations feasible.
As developers, keeping an eye on these trends helps not only to adapt but also inspires innovation in application design.