Building a Java Application: A Complete Guide
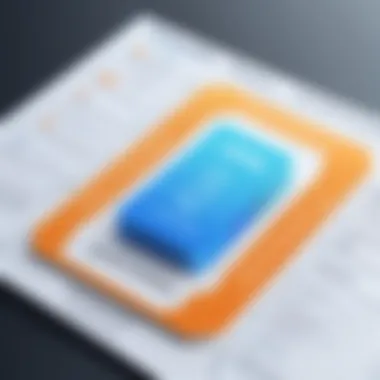
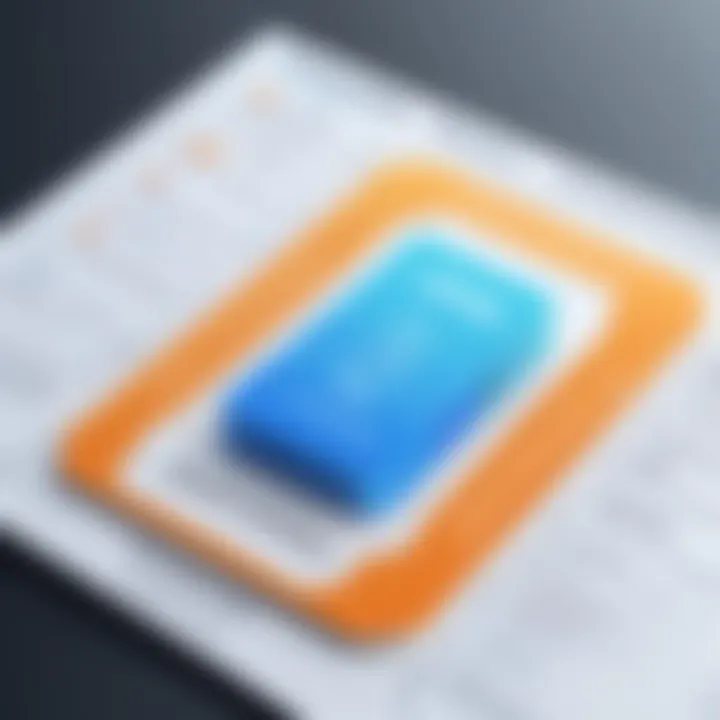
Overview of Topic
Building a Java application is a task that many individuals and organizations undertake to harness the power of one of the most popular programming languages in the world. Java is widely recognized for its versatility, platform independence, and vast ecosystem. This guide aims to break down the intricate processes involved in creating a Java application, from setup and development to deployment and maintenance.
The significance of Java in the tech industry canât be overstated. It serves as the backbone for numerous applications ranging from mobile apps to large-scale enterprise systems. Understanding the foundational principles and systematic approaches to building applications is crucial for both newcomers and experienced programmers.
Historically, Java was introduced by Sun Microsystems in 1995, and since then, it has evolved significantly. From its initial version, Java has undergone changes that have introduced new features and improved performance. Today, it is managed by Oracle Corporation and continues to thrive in diverse sectors.
Fundamentals Explained
Core Principles
To build a successful Java application, a developer should grasp key concepts such as object-oriented programming (OOP), which is the backbone of Java itself. OOP principles like encapsulation, inheritance, and polymorphism empower developers to create modular and reusable code.
Key Terminology
Familiarizing oneself with the terminology used in Java is important. Terms such as
- JVM (Java Virtual Machine): The runtime environment that allows Java bytecode to be executed.
- JDK (Java Development Kit): The complete toolkit for developing Java applications, including the compiler and libraries.
- IDE (Integrated Development Environment): Tools like Eclipse or IntelliJ IDEA that facilitate the coding process.
Basic Concepts
A solid understanding of variables, data types, control structures, and error handling is essential. These elements provide the building blocks for creating more complex applications. Developers must learn how to manage inputs and outputs efficiently, as well as how to handle exceptions to ensure that their applications run smoothly.
Practical Applications and Examples
Real-World Case Studies
Consider a banking application where secure transactions are paramount. In this scenario, an understanding of multi-threading in Java can greatly enhance the applicationâs performance. Developers must consider factors such as data integrity and user session management to create a robust solution.
Demonstrations and Hands-On Projects
Building a simple CRUD (Create, Read, Update, Delete) application using Spring Boot is a practical way to implement learned concepts. This project allows developers to understand frameworks and how they expedite application development.
Code Snippets
Hereâs a basic example of setting up a Spring Boot application:
This snippet demonstrates the main entry point of the application and lays the foundation for further development.
Advanced Topics and Latest Trends
Cutting-Edge Developments
The tech landscape is ever-changing, and this holds true for Java as well. Emerging trends like microservices architecture and serverless computing are shaping how applications are developed and deployed.
Advanced Techniques
Incorporating frameworks like Hibernate or Spring for dependency injection can significantly streamline the development process. Moreover, modern Java versions have introduced features like lambda expressions and the stream API, which aids in writing cleaner and more efficient code.
Future Prospects
Looking ahead, Java continues to be a strong player in the tech industry. Its community is robust, and with ongoing updates, the language is aligned to meet future demands, especially in cloud-native development and AI integrations.
Tips and Resources for Further Learning
Recommended Resources
To deepen your understanding of Java, consider exploring the following:
- Books: "Effective Java" by Joshua Bloch, a staple for both novices and pros.
- Online Courses: Platforms like Coursera and Udemy offer a range of Java courses for different levels.
Tools and Software
Utilize tools such as Maven for project management and JUnit for testing to improve workflow efficiency. These tools can help in automating tasks and managing dependencies effectively.
Understanding Java Applications
In todayâs tech-savvy world, understanding Java applications is crucial for anyone looking to navigate the realms of programming and software development. This journey begins with a clear comprehension of what Java applications are, as they stand at the intersection of functionality and user interaction. Java enables developers to create robust applications that are not just limited to the desktop but extend to various platforms, providing flexibility like no other.
Focusing on Java applications is essential not just for academic purposes but also for its practical applications in industry. By exploring different types of Java applications, developers can pinpoint which application type suits their needs. Itâs more than just learning to code; itâs about crafting solutions that are efficient, scalable, and user-centric. With Java being an object-oriented language, it embodies a design philosophy that promotes modularity and reusability, which are pivotal in todayâs fast-paced development environments.
This section will cover the definition and various types of Java applications, examining each with an analytical lens to uncover their unique features and advantages. Let's delve deeper into what makes Java applications an integral part of modern software development.
Definition of a Java Application
A Java application is essentially a program or software developed using the Java programming language. It can operate on any system that has the Java Runtime Environment (JRE) installed, thus ensuring a high level of portability across different platforms. These applications are written in Java programming code and compiled into bytecode which the Java Virtual Machine (JVM) interprets, allowing it to run on any machine without needing to be recompiled for each individual operating system.
The beauty of Java lies in its versatility; it allows developers to create everything from simple standalone applications to complex web-based programs. This adaptability makes it a prime choice in the programming world.
Types of Java Applications
Java applications can be broadly categorized into several types, each serving different needs and preferences. Here are the main categories:
- Standalone Applications
Standalone applications are traditional desktop applications that operate independently on a user's machine. An example might be a text editor or a media player. These applications have a distinct advantageâthey can execute without being connected to the internet, providing users with reliability and ease of use.One key characteristic of standalone applications is their simplicity in installation and use; users can run them right from their desktops with minimal fuss. However, they are limited in scope, as they canât leverage server resources or implement cloud functionalities, which could restrict their capabilities in today's interconnected world. - Web Applications
Web applications are the backbone of the online experience. These applications run on servers and are accessed by users via web browsers. With features like dynamic content and user interaction, web applications have become the default choice for many businesses.
A primary benefit is their ability to reach users anywhere, at any time, as long as there's an internet connection.The unique feature of web applications is their ability to handle multiple users simultaneously, which is crucial for businesses seeking to expand their reach. However, they require constant internet connectivity, making them less ideal for offline scenarios. - Enterprise Applications
These are large-scale applications meant for use in an organization. They cater to the needs of businesses and might include components like customer relationship management (CRM) and enterprise resource planning (ERP).Their scalability is a standout characteristic; as a company grows, enterprise applications can evolve to meet changing demands. While they can be a wise investment for any large organization, their complexity can be a double-edged sword, requiring rigorous maintenance and updates which can be resource-intensive. - Mobile Applications
Mobile applications are designed for mobile devices and have become increasingly important with the rise of smartphones. They offer users functionality right in their hands, delivering services ranging from social media to banking.A key feature here is accessibility; users can interact with applications on the go, a convenience that is hard to beat. Nonetheless, developers must consider various mobile operating systems, which can complicate development and increase testing time.
Setting Up Your Development Environment
The importance of establishing a robust development environment cannot be overstated. This phase sets the stage for every project you undertake in Java. Like building a house, a solid foundation is crucial to support all activities that come later. Getting it right at this stage can save developers from a world of headaches down the line.
A well-set development environment enables developers to write efficient code, debug issues effectively, and manage projects seamlessly. Without the right tools and configurations, even the most talented developers can struggle, leading to decreased productivity and frustrations. This section looks into the key aspects of setting up your Java environment, including selecting an Integrated Development Environment (IDE), installing the Java Development Kit (JDK), and fine-tuning your IDE for optimal performance.
Selecting an Integrated Development Environment (IDE)
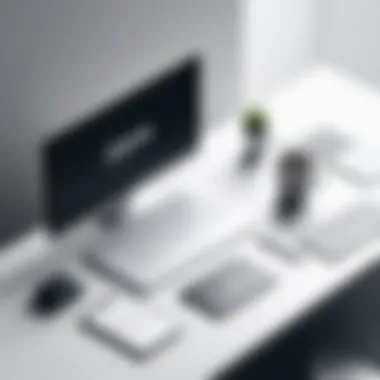
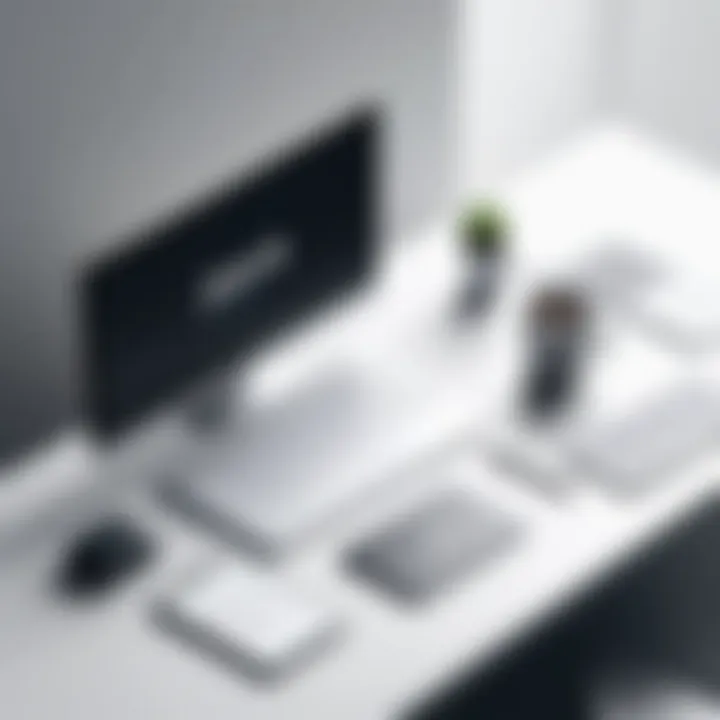
Choosing an IDE is a pivotal step in your Java development process. It can influence not just how easily you can write and read code, but also impact debugging, project management, and collaboration with other developers. Here, we explore a few popular choices in detail.
Eclipse
Eclipse stands as one of the most well-known IDEs in the Java community. The flexibility it offers is often highlighted as a key aspect. Many developers appreciate that Eclipseâs plugin system allows for extensive customization.
One key characteristic that tends to draw developers is its strong community support. This means finding help or resources on specific issues is generally just a search away. However, despite its strengths, Eclipse often has a steeper learning curve, especially for newcomers.
One unique feature is its built-in support for different programming languages through plugins. This makes it versatile, but on the downside, it can sometimes feel a bit bloated with features that some users may never use.
IntelliJ IDEA
IntelliJ IDEA has gained a loyal following due to its intelligent code analysis features. Many would argue it's smarter than your average IDE, understanding your code and offering suggestions for improvements.
The standout characteristic is its user-friendly interface, making it easy to navigate, even for those who arenât tech-savvy. This personal touch is an appealing aspect for many developers.
One unique feature of IntelliJ IDEA is its refactoring capabilities, which are both powerful and intuitive. However, this IDE is not without drawbacks; the ultimate version can be pricey, and some may find certain features unnecessary for simple projects, making it less appealing for novices.
NetBeans
NetBeans is another IDE that offers a solid foundation for Java development. It's especially praised for being free and open-source. This inclusion makes it accessible for students and hobbyists alike.
One of its key characteristics is out-of-the-box ease of use, often eliminating the need for a steep learning curve. NetBeans features clear and straightforward menus. Yet, it may lag behind in terms of the extensive plugin ecosystem that Eclipse boasts.
A unique aspect of NetBeans is its ability to work with various languages, not just Java. However, some developers note that it can sometimes lack the performance efficiency and advanced functionalities that IDEs like IntelliJ offer, especially for larger projects.
Installing the Java Development Kit (JDK)
Once you've selected an IDE, the next step is to install the Java Development Kit (JDK). This essential toolkit contains everything you need to develop in Java, from the JAVA compiler to essential libraries. The installation is relatively straightforward; you just need to download the appropriate version from Oracle's website or a third-party distributor and follow the instructions. Keep in mind that there are different JDK versions available, so aligning the version with your specific project requirements is crucial.
Configuring Your IDE for Java Development
After installation, itâs time to configure your IDE properly. Different IDEs have unique settings, but there are common steps you should follow. You might want to set your workspace directory, adjust settings for compiler compliance level, and integrate version control systems like Git. These configurations will customize your workspace to suit how you work best.
Additionally, take advantage of the various built-in features most IDEs offer for Java development. This could include setting up code templates for faster coding or utilizing debugging tools to streamline error detection.
In summary, setting up your development environment is not just a preliminary step but a critical component that can influence the overall quality and efficiency of your Java applications. By carefully selecting your IDE, installing the JDK, and configuring your tools, you equip yourself for a more seamless development experience.
Designing Your Java Application
Designing your Java application is a crucial step in the software development lifecycle. It's not merely about writing code; it's about crafting a blueprint that guides the entire process of application development. A thoughtful design phase lays down the foundation for future development, testing, and maintenance. Without proper design, a project can spiral out of control, leading to chaotic code structure and unforeseen bugs.
When it comes to designing a Java application, there are several important considerations to keep in mind. First, clarity in requirements is essential. You need to know what exactly you want your application to achieve. Involving stakeholders during this phase can ensure that the end product meets their expectations.
Next, you should effectively leverage architectural patterns and principles. These design frameworks help in organizing code and can make your application adaptable for future changes. Not only does this streamline your development process, but it can also minimize technical debt.
Creating a Functional Specification
A functional specification is essentially a detailed description of the functionalities your application will provide. It's like a roadmap that outlines what hilltops you wish to conquer in your software journey. Creating one forces you to think critically about each feature and its value to users.
There are several key elements to consider when drafting a functional specification:
- User Stories: What would the users want from your application?
- Functional Requirements: Specify how each feature should function. This can include inputs, outputs, and user interactions.
- Use Case Scenarios: These can illustrate how a user would typically navigate through the application.
A well-thought-out functional specification saves time down the line, preventing unnecessary reworks and helping ensure all team members are on the same page.
Architectural Patterns
Leveraging architectural patterns during the design phase permits developers to reuse successful layouts of software that have stood the test of time. Two frequently used patterns in Java application design are Model-View-Controller (MVC) and Service-Oriented Architecture (SOA).
Model-View-Controller ()
The Model-View-Controller (MVC) architecture is pivotal for designing robust applications. It segments the application into three interconnected components: the Model handles the data, the View manages the display, and the Controller acts as an intermediary.
One key characteristic of MVC is its separation of concerns. This means that changes made in one layer wonât heavily impact the others, allowing developers to work on different aspects simultaneously. For example, a frontend developer can modify the UI without worrying about the backendâs logic. This makes it a beneficial choice for collaborative environments where team members specialize in various areas.
However, MVC isn't without its downsides. Sometimes, developers might find themselves navigating complicated data flows, which can increase the learning curve for newcomers. Nonetheless, the advantages greatly outweigh the disadvantages, establishing MVC as a stalwart choice in Java application design.
Service-Oriented Architecture (SOA)
On the other hand, Service-Oriented Architecture (SOA) focuses on building applications from a collection of services. Each service operates independently, communicating over well-defined protocols. This approach is advantageous for larger projects that need scalable and flexible solutions.
A notable characteristic of SOA is its loose coupling of components. It allows various parts of the system to evolve independently, adapting to technological advances without a complete overhaul of the existing system. This is particularly beneficial in environments where rapid changes are the norm.
However, with these benefits come challenges. The management of multiple services can get complex, requiring resources and proper governance to maintain order. Additionally, when services communicate over a network, performance might be hindered, especially if the communication isn't optimized.
Class and Object Design
Class and object design revolve around structuring your code to maximize readability and maintainability. Every class should follow the single responsibility principle, ensuring it has only one reason to change. This increases the longevity of your code and makes updates more manageable. Objects, as instances of classes, represent real-world entities, making it intuitive for developers to grasp the relationships and behaviors within the application.
Thoughtful design decisions at this phase will serve as a bedrock for the implementation, testing, and maintenance processes that follow, paving the way for a successful Java application.
Implementing Your Application
Implementing your application stands as a cornerstone in the journey of building a Java program. This stage transforms your meticulous planning and design into a living, breathing software solution. Itâs where the rubber meets the road. Without effective implementation, all that careful design work could be nothing more than hypothetical. Itâs the stage where you start coding, building functionality, and breathing life into your ideas.
Several key elements define this phase. Firstly, writing your initial Java program is fundamental. This is the pivotal moment where you take your concepts and translate them into code, setting the groundwork for your application. Secondly, utilizing Java libraries and frameworks helps streamline development. These tools can save time, reduce bugs, and simplify complex tasks. Lastly, managing dependencies with build tools is crucial. Proper dependency management ensures that your project runs smoothly, avoiding conflicts that can arise from library incompatibilities.
Ultimately, the implementation phase is where you kick start the actual coding process, making this segment essential for anyone looking to develop robust Java applications.
Writing Your First Java Program
Getting started with your first Java program can be a thrilling experience, much like taking your first step into a new world. This program often serves as a simple "Hello World" application, where you display a message on the screen. Not only does this teach the syntax of Java, but it also helps you to understand the compilation and execution process. Hereâs a straightforward snippet:
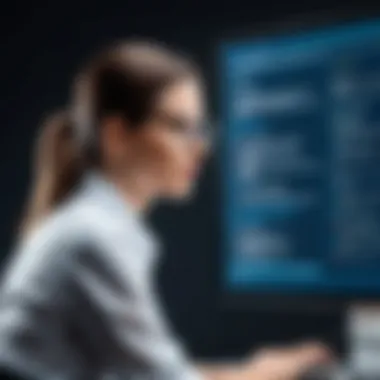
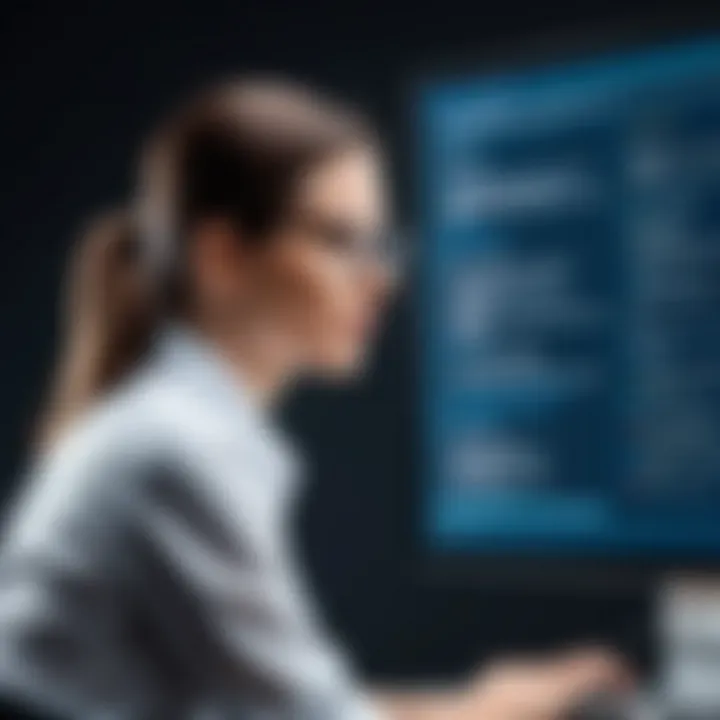
When you run this code, the output will show Hello, World! on the console. This simple act encapsulates a larger journey: coding every small feature, debugging issues along the way, and eventually creating a finely-tuned application. Starting small allows you to build confidence, experiment with syntax, and gradually tackle more complex problems.
Utilizing Java Libraries and Frameworks
Java boasts a rich ecosystem of libraries and frameworks, each designed to enhance productivity and broaden functionality. They are indeed invaluable tools in a developer's toolkit.
Java Standard Libraries
The Java Standard Libraries offer a comprehensive set of tools that simplify common programming tasks. These libraries provide ready-made classes and functions, making it easier for developers to accomplish a range of operations without reinventing the wheel. Some standout features include data structures like ArrayLists and HashMaps, utility classes, and input/output capabilities.
The inclusion of these libraries is a common and beneficial practice in Java application development. One unique aspect is how they come bundled with the Java Development Kit (JDK), ensuring theyâre available out-of-the-box with your Java installation.
However, while they cover a variety of needs, advanced tasks might require additional frameworks, which can lead to clutter if one isn't careful.
Spring Framework
The Spring Framework is one of the most popular choices among Java developers, known for its modular architecture. Its primary function is to simplify the complexities of Java EE for enterprise applications. The Inversion of Control (IoC) container it employs allows for highly maintainable and testable applications.
What sets Spring apart is its comprehensive suite of features, including Spring MVC for web applications, Spring Boot for rapid setups, and Spring Data for simplified database access. Though powerful, the learning curve can be steep for newcomers, making it potentially daunting at first glance.
Hibernate ORM
Hibernate ORM is pivotal in managing database interactions within Java applications. It provides a framework for object-relational mapping, seamlessly converting data between incompatible systems. This abstraction allows developers to work with database records as simple Java objects without dealing with the complexities of SQL directly.
A distinguishing feature of Hibernate is its caching strategy which can drastically improve performance by minimizing database access. However, it comes with its own set of challenges; misconfiguration can lead to poor performance or unexpected behavior, demanding a thorough understanding of ORM concepts.
Managing Dependencies with Build Tools
As projects grow in complexity, managing dependencies becomes crucial. Here, build tools streamline the process, making it easier to manage the libraries that your project depends on, ensuring everything works harmoniously.
Maven
Maven is a widely-used build automation tool in the Java ecosystem. It emphasizes convention over configuration, meaning it follows predefined structures for project management, which can significantly reduce setup time. Maven not only manages project dependencies, but also automates the build process, testing, and deployment. Itâs especially beneficial for large projects, making it a popular choice among developers in this article.
However, Maven can feel a bit rigid for simpler tasks, which might overwhelm newcomers or those working on smaller projects that donât require extensive configuration.
Gradle
Gradle is another powerful tool, often praised for its flexibility and performance. It leverages a Groovy-based domain-specific language to define builds, allowing developers to write custom scripts easily. Gradle combines the best of both worlds â XML configuration simplicity from Maven and the capability for complex scripting.
One standout feature is its incremental build capabilities, which significantly speed up the build process. On the flip side, this flexibility may lead to confusion if not properly documented, especially for teams with varied skill levels.
In concluding this section, implementing your Java application is an intricate yet rewarding endeavor. With the right tools and methodologies in hand, developers can navigate this process smoothly, ensuring the creation of robust applications that meet user needs.
Testing Your Java Application
Testing a Java application isnât just a box to tick; it's an essential part of the development process that ensures your application runs smoothly and meets user expectations. Imagine crafting a beautiful piece of furniture â would you sell it without making sure the joints are secure and the finish is even? The same principle applies to software. Itâs about guaranteeing that every component functions as intended, catching bugs before they make their way to users.
Effective testing can significantly reduce errors, enhance performance, and boost overall user satisfaction. As software becomes more complex, the urgency of robust testing rises to the forefront. Neglecting this vital phase can lead to costly fixes down the line and damage to your reputation.
Importance of Testing
At the heart of testing lies assurance. By systematically verifying features, you provide a safety net that catches issues before they have the chance to escalate. It also leads to increased confidence among users in your applicationâs reliability. Ultimately, testing nurtures a culture of continuous improvement, where feedback is not merely tolerated but embraced.
Additionally, catching errors early in the development cycle is much cheaper than fixing them after deployment. Statistics often point to the phenomenon known as the cost of delay. It highlights that a defect detected during later phases can exponentially cost more to resolve compared to one found during initial development.
Unit Testing in Java
Unit testing is a crucial aspect of ensuring the reliability of your application. This involves testing individual components â or units â of code to verify that each part performs its designated function correctly.
JUnit
Since its inception, JUnit has become synonymous with unit testing in Java. One of its primary contributions is its structured approach to testing methods and functionalities. A defining characteristic of JUnit is its use of annotations, which simplifies test cases. A standout feature of JUnit is its ability to run tests in isolation. This means that if one test fails, it doesnât halt the entire testing process. Such isolation helps in pinpointing issues more accurately. In terms of popularity, JUnitâs ease of use makes it an attractive choice for both novices and seasoned developers.
Advantages of using JUnit include:
- Integration with IDEs: Many integrated development environments support it seamlessly, allowing for smooth execution of tests.
- Rapid feedback: Developers receive immediate notification of any issues, which speeds up the iterative process of development.
- Wide community support: A steep learning curve is often mitigated by a plethora of online resources and community discussions.
However, utilizing JUnit does come with some downsides. For instance, it may not handle complex test scenarios without additional configuration.
Mockito
In tandem with unit testing, Mockito serves as an invaluable companion for simulating the behavior of complex objects. Its main role is to allow developers to create mock objects that mimic real ones but operate under controlled conditions. The key characteristic of Mockito is its focus on simplicity, hence itâs typically easy to set up and execute tests.
One unique aspect of Mockito is its fluent interface, meaning that developers can chain method calls, creating more readable tests. This clarity puts developers in the driverâs seat when verifying that their components are interacting correctly.
Benefits of employing Mockito include:
- Decoupling of tests: By replacing real dependencies with mocks, you can test only the specific unit in question, without interference.
- Flexibility: Mocking allows for testing various use cases without needing the entire application stack.
- Streamlined test maintenance: If an external dependency changes, only the mocked references need updating.
Nonetheless, the degree of abstraction it offers can sometimes mask real interactions, which is a potential drawback when striving for comprehensive testing.
Integration Testing Strategies
Integration testing takes a step further by evaluating how various components of an application interface with each other. Unlike unit testing, which checks isolated elements, integration testing assesses the combined functionality of interconnected modules. This kind of testing can reveal problems that may not surface during unit tests, such as data flow issues or authentication errors between components.
There are several strategies developers can adopt for integration testing, including:
- Big Bang Testing: Combining all components at once and checking the system as a whole. This method is straightforward but can be challenging to debug if failures occur.
- Incremental Integration: Adding components gradually and testing each addition. This can either be done in a top-down or bottom-up fashion, allowing for more manageable debugging.
- Contract Testing: This is crucial in microservices architectures, ensuring that services adhere to agreed-upon interfaces.
In summary, rigorous testing during the development of a Java application plays an indispensable role in maintaining quality. By leveraging tools like JUnit and Mockito, alongside smart integration testing strategies, developers are better positioned to produce robust, efficient applications that meet user needs.
Deploying Your Java Application
Deploying a Java application is like putting the finishing touches on a beautifully crafted piece of art. Itâs not just about writing code, but about bringing that code to life in a real-world environment where users can engage with it. This process is crucial as it determines how well the application performs, its availability, and its scalability. A proper deployment strategy can lead to better user experiences, enhanced performance, and long-term maintainability of the application.
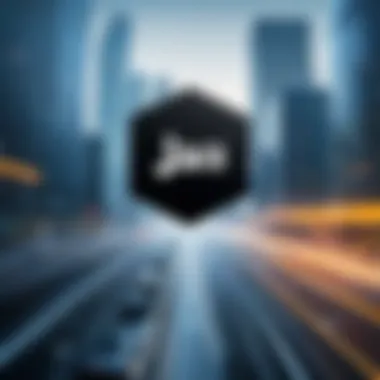
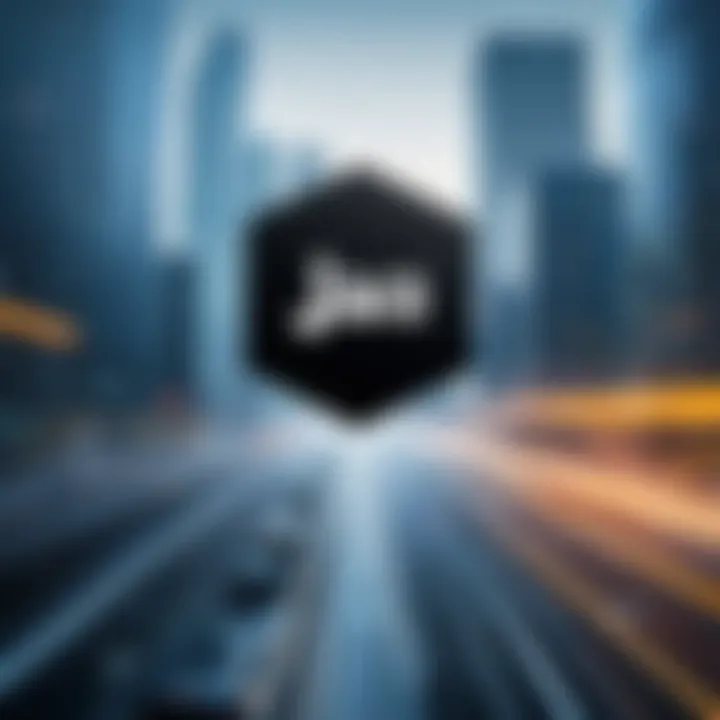
Choosing the right deployment method influences the architecture of the application and even affects the development process itself. Whether you implement the app on-premises or in the cloud, each choice offers unique advantages and comes with its own set of challenges. This section dives into the core deployment options available for Java applications.
Understanding Deployment Options
When it comes to deploying a Java application, two primary options often arise: on-premises and cloud deployment. Understanding the nuances of each plays a vital role in making a thoughtful decision suited to your application's specific needs.
On-Premises Deployment
On-premises deployment involves setting up the application within the internal network of an organization. This means that the entire infrastructureâincluding servers, storage, and networkingâis owned and managed by the organization.
One major aspect of on-premises deployment is the control it provides. Organizations that have strict security policies, such as financial institutions or healthcare providers, often opt for this deployment method to maintain tight control over data accessibility and compliance. Furthermore, it can lead to lower long-term operational costs as there are no ongoing fees for cloud services, although initial setup costs can be significant.
However, there are some downsides. Organizations are responsible for all maintenance, including updates, backups, and hardware issues. If infrastructure issues arise, the users might face substantial downtime. In sum, while on-premises deployment offers control and security, it also requires a commitment to ongoing management and potential additional costs associated with hardware and support.
Cloud Deployment
Cloud deployment refers to hosting the application on virtual servers managed by a third-party providerâlike AWS or Azure. In this setup, users access the application over the internet instead of running it on local servers.
One notable aspect of cloud deployment is its flexibility. Companies can scale resources up or down as needed, paying only for what they use. This adaptability allows for rapid response to fluctuating demands, which can be particularly beneficial for applications experiencing variable user loads. Cloud platforms often come with built-in redundancy and disaster recovery features, enhancing the reliability and availability of applications.
That said, cloud deployments can introduce concerns around data privacy and compliance, as sensitive information may be stored on external servers. Additionally, relying on a third-party cloud service can lead to vendor lock-in issues, making it challenging to migrate to different platforms in the future. Ultimately, cloud deployment allows for speed and scalability but requires careful consideration of security and long-term accessibility.
Creating a Deployment Package
The deployment package is the bundle that contains all the necessary files and configurations to run your Java application in a designated environment, whether on-premises or in the cloud. Creating a dependable deployment package is crucial, as it ensures that when you deploy, all dependencies, libraries, and configurations are intact and functional.
This package typically includes compiled code, configuration files, and resources such as images and third-party libraries. Using tools like Maven or Gradle simplifies the process of managing dependencies and creating deployment packages, making the job much less burdensome.
Hereâs a quick checklist for creating a deployment package:
- Compile your Java files.
- Include all necessary libraries and dependencies.
- Add configuration files tailored for the deployment environment.
- Include scripts for starting and stopping services.
- Test the package in a staging environment before going live.
Continuous Deployment and Integration (/)
Continuous Deployment and Integration (CD/CI) practices automate and streamline the deployment processes in Java development. This also plays a pivotal role in maintaining high-quality code.
By integrating CD/CI into the deployment workflow, Developers can automatically test code changes before theyâre merged. This reduces the chances of errors in production environments and allows for rapid delivery of features and updates, which is essential in todayâs fast-paced digital landscape.
Implementing these practices involves moving away from manual deployments, which can lead to delays and potential human error. Instead, teams can focus on writing code and delivering value rather than on the logistics of deploying that code.
With effective CD/CI practices, teams can achieve faster feedback loops, maintain better code quality, and ultimately create a more resilient application, leading to an overall enhanced user experience.
"Continuous Deployment turns deployment into a non-event, which is the goal we aim for in modern software development."
Maintaining Your Java Application
Maintaining your Java application is a crucial aspect often overlooked during the initial development phases. Once a project is deployed, it doesnât simply run on its own; it needs continual attention. This section delves into the importance of maintenance, highlighting why it's more than just fixing bugs, and how it contributes to the longevity and performance of your application.
Firstly, regular maintenance helps in bug identification and resolution. As users interact with your application, they might encounter issues that didn't surface during testing. Fixing these bugs promptly not only enhances user satisfaction but also improves system stability. A system riddled with unresolved bugs is likely to frustrate users and can lead to erratic behavior.
Moreover, maintenance includes updating libraries and frameworks used within your application. Software libraries often undergo changes or enhancements. If your application relies on outdated libraries, it risks compatibility issues and lacks the benefits of performance improvements or new features. Not to forget, keeping dependencies up-to-date aligns with industry standards and security practices, making your application less vulnerable to external threats.
Performance monitoring is yet another aspect of maintenance. Staying on top of how well your application performs in a production environment is essential. Whether itâs response times or resource usage, a drop in performance can indicate underlying problems that need addressing.
Regular maintenance isnât just about fixing whatâs broken; itâs about enhancing and future-proofing your application.
In essence, maintenance is a key component that ensures your Java applications remain functional, relevant, and competitive in a fast-changing technological landscape. Now letâs explore the specific elements of maintaining your Java application.
Bug Fixing Protocols
Establishing effective bug fixing protocols is paramount in maintaining your Java application. Without a clear strategy, addressing bugs can become a chaotic process, leading to unforeseen issues down the road. Hereâs a closer look at how to streamline this task.
- Identify the Bug: Utilize logging mechanisms to pinpoint issues. Java provides a robust logging framework that helps capture exceptions and errors throughout application execution.
- Reproduce the Issue: Ensure that the bug can be consistently reproduced. Itâs essential to understand the userâs actions that led to the failure.
- Fix the Issue: Once diagnosed, implement a solution. Ensure that the fix doesnât introduce new vulnerabilities or break existing functionality.
- Test the Fix: After applying the fix, thoroughly test to confirm that the issue is resolved and no new problems emerge.
- Update Documentation: Reflect changes in your documentation. This can be vital for future reference.
Establishing a responsive communication channel for users to report issues also aids in quicker bug detection and resolution, fostering a community-driven approach to application maintenance.
Updating Libraries and Frameworks
Keeping libraries and frameworks updated is like replacing old parts in a well-used machine. Over time, software components can become outdated, causing compatibility issues, security vulnerabilities, and reduced performance. Hereâs why regular updates are not just beneficial but essential:
- Security: Many libraries frequently release patches to fix vulnerabilities that could be exploited by malicious users. By regularly updating, you reduce the risk of security breaches.
- Performance Improvement: New versions of libraries often come with enhancements that boost efficiency and speed. Utilizing the latest versions can dramatically improve application performance.
- Feature Enhancements: New releases can also introduce new features or functionalities that you can leverage to enhance your application's capabilities.
Regularly check for updates through package management tools, such as Maven or Gradle, and read the release notes to understand changes.
Performance Monitoring Techniques
Monitoring performance is akin to checking the pulse of your application. Knowing how it performs under various loads is essential not only for user satisfaction but also for reliability. Here are effective strategies for performance monitoring:
- Application Performance Monitoring Tools: Utilize tools like New Relic or AppDynamics to get insights into application performance metrics. These tools provide visibility into transaction times, memory usage, and other key indicators.
- Stress Testing: Create scenarios to test how your application behaves under high traffic or load. Identifying limiting thresholds can prevent performance breakdowns during peak usage times.
- User Feedback: Encourage users to report performance issues. Sometimes, external insights provide different perspectives than internal testing.
- Regular Code Reviews: Encourage a culture of regular code reviews. Sometimes, inefficient code can slip through the cracks, leading to performance issues that could have otherwise been avoided.
Keeping a vigilant eye on performance will help you ensure your application runs like a well-oiled machine, ensuring a seamless experience for users.
Future Trends in Java Development
The landscape of Java development is ever-evolving, shaped by both technological innovations and changing user needs. As we look into the future, it becomes crucial for developers to be aware of these trends. Recognizing emerging technologies not only helps in staying relevant but also enhances the ability to create applications that resonate with modern demands. This section discusses several significant elements that are changing the face of Java.
Exploring Emerging Technologies
In the rapidly changing world of software development, technologies rise and fall like tides. Java is not untouched by this flux. Several emerging technologies are steering Java development in exciting new directions:
- Cloud-native Java: More applications are being designed to optimize their performance in cloud environments. Tools like Kubernetes and Docker are now indispensable for deploying Java applications efficiently.
- Microservices Architecture: Gone are the days of monolithic applications. Microservices offer the flexibility to develop, scale, and maintain individual components in isolation. This is not just a trend; it's becoming essential for building resilient systems.
- Reactive Programming: In a world where speed and responsiveness are paramount, reactive programming paradigms such as Project Reactor and RxJava are gaining traction. They allow developers to build systems that are more responsive and capable of handling massive data streams.
- Artificial Intelligence: Java is making inroads in the realm of AI and machine learning. Frameworks like Deeplearning4j enable developers to integrate predictive analytics and intelligent features into their Java applications.
Understanding these emerging technologies is vital, as they will have profound implications on development practices, project architecture, and deployment strategies.
The Role of Java in Modern Application Development
Even with the shifts in technology, Java continues to hold its ground as a staple in software development. Its longevity can be attributed to several core advantages that make it suitable for modern application needs:
- Robust Ecosystem: Java benefits from a rich ecosystem of libraries and frameworks that enable quick and efficient application development. Tools like Spring and Hibernate simplify complex tasks, allowing developers to focus more on logic rather than boilerplate code.
- Cross-Platform Compatibility: The mantra "Write once, run anywhere" remains a significant selling point. Organizations can save resources by deploying the same Java application across various platforms without needing extensive modifications.
- Safety and Security: With cyber threats on the rise, Java's built-in security features such as automatic memory management and type checking make it a reliable choice for building secure applications.
- Continuous Growth: The Java community is vibrant and continuously evolving, fueled by passionate developers and frequent updates. This ensures the language adapts to modern needs while maintaining its foundational strengths.
In the ever-changing sphere of technology, Javaâs adaptability and resilience continue to make it a favorite among developers.
As Java keeps stepping into new territories, developers must adapt their skills and knowledge to leverage what Java brings to the table in modern application development.