Building and Managing a Python API Server Effectively
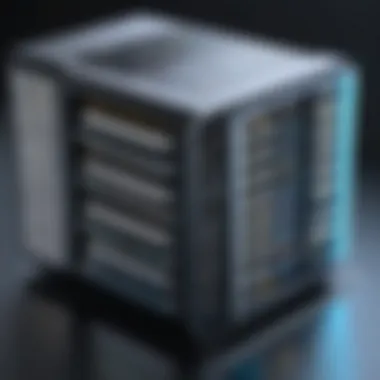
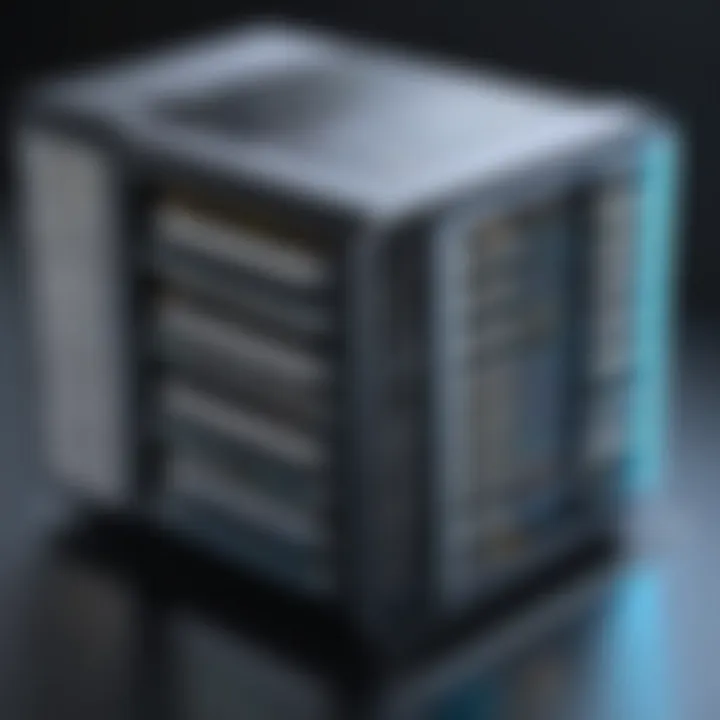
Overview of Topic
Prologue to the main concept covered
The rise of digital services has pushed the need for effective data exchange and communication between systems. An Application Programming Interface (API) is a vital component facilitating this interaction. A Python API server specifically utilizes the Python programming language to construct these interfaces and handle requests. It enables developers to create services that can communicate seamlessly with other applications, making it an essential skill in modern software development.
Scope and significance in the tech industry
The significance of building and managing Python API servers cannot be understated. As businesses increasingly rely on web services, APIs serve as integrated systems for applications, allowing them to work together. This integration enhances user experience and operational efficiency. Moreover, with Python's popularity due to its simplicity and versatility, developing API servers has become more accessible. Strengthening skills in this area can lead to increased job opportunities and project possibilities in various tech domains.
Brief history and evolution
The evolution of API servers has paralleled technological advancements in software engineering. Initially, APIs were merely functions and methods in libraries. However, as complexity grew, the need for structured interactions emerged. Over time, REST (Representational State Transfer) and subsequently GraphQL became standard paradigms for web APIs. The adaptation of Python, a language known for its readability and rich ecosystem, made it a go-to choice for developers. Today, numerous frameworks like Flask and Django facilitate quick and efficient API development, with significant community support.
Fundamentals Explained
Core principles and theories related to the topic
When developing an API server, comprehension of core principles is fundamental. Key concepts include:
- RESTful architecture: This style of software architecture is based on stateless communications. Resources are identified through URIs and accessed using standard HTTP methods.
- Endpoints: Specific paths at which APIs can be accessed, corresponding to actions or resources.
- JSON: JavaScript Object Notation is commonly used for data interchange between applications due to its lightweight format.
Key terminology and definitions
Familiarity with terminology is critical for understanding API development. Important terms include:
- Client: An application or service that sends requests to the server.
- Request: A message sent by a client to access a resource on the server.
- Response: The server’s reply to the request, often containing the requested data or status information.
Basic concepts and foundational knowledge
Understanding HTTP methods is a foundational aspect of API development. The four primary methods are:
- GET: Retrieve data from the server.
- POST: Send data to the server.
- PUT: Update existing data.
- DELETE: Remove data from the server.
Each method plays a crucial role in shaping how applications interact with each other, making it essential to grasp their appropriate usage.
Practical Applications and Examples
Real-world case studies and applications
API servers are used extensively across industries. For instance, Twitter offers an API that allows developers to post tweets, retrieve user data, and engage with its platform programmatically. Similarly, e-commerce sites like Shopify provide APIs to manage products, inventory, and orders programmatically, enhancing customer engagement.
Demonstrations and hands-on projects
Building a simple API can often be a great way to understand the practical applications. Using Flask, a lightweight framework in Python, you can create a basic API easily. A minimal example could look like this:
Code snippets and implementation guidelines
Building upon the basic example, a well-structured API should handle errors and enforce security measures. Incorporate error handling and input validation to ensure robustness. Also, consider using libraries like Flask-RESTful to enhance your API development process.
Advanced Topics and Latest Trends
Cutting-edge developments in the field
API management tools are becoming more advanced. Platforms like Postman and Swagger provide user-friendly interfaces for designing and documenting APIs, allowing developers to manage their workflows more effectively.
Advanced techniques and methodologies
Techniques such as rate limiting are now crucial in managing API usage, ensuring that resources are not overwhelmed by excessive requests. Additionally, versioning strategies are essential for maintaining backward compatibility as services evolve over time.
Future prospects and upcoming trends
The future of API development leans towards microservices architecture, where applications are broken down into smaller, manageable services. This modular approach enhances scalability and maintainability, adapting better to modern development practices.
Tips and Resources for Further Learning
Recommended books, courses, and online resources
- Books: "Flask Web Development" by Miguel Grinberg and "RESTful Web APIs" by Leonard Richardson.
- Courses: Online platforms like Coursera and Udemy offer great courses focusing on API development with Python.
Tools and software for practical usage
Some prevalent tools include Postman for testing APIs and Swagger for documenting them. Utilizing these tools can improve both development speed and end-user experience.
Remember, building a robust API involves continuous learning and adaptation to new practices and technologies.
Intro to Python API Servers
In the contemporary landscape of software development, API servers have emerged as a vital component, facilitating communication between different software applications. The integration of APIs enables the interaction of diverse systems, catering to a wide range of needs from simple data retrieval to complex transactional processes. Understanding how to efficiently build and manage these servers, particularly in Python, opens a multitude of opportunities for developers seeking to enhance service architectures.
Understanding APIs
APIs, or Application Programming Interfaces, serve as intermediaries that allow different applications to communicate with each other seamlessly. In a typical web environment, an API can expose certain functionalities of an application over the web, granting access to the underlying data and services. This abstraction layer not only simplifies the complexity associated with software interactions but also fosters innovation. The successful utilization of APIs can lead to quicker development cycles, enhanced integrations, and improved user experiences.
With APIs, developers can
- Create rich client applications that communicate with server-side resources,
- Enable third-party integrations that expand functionality and user reach,
- Leverage existing services without reinventing the wheel, thus saving valuable time.
Why Use Python for API Development
Python has gained prevalent use in API development for various reasons. Its simplicity and readability make it an ideal choice for new learners and seasoned professionals alike. Some advantages of using Python for building APIs include:
- Rich Ecosystem: Libraries and frameworks like Flask, Django, and FastAPI provide robust tools and features, streamlining the development process.
- Rapid Prototyping: Python’s flexible syntax allows for quick iterations, making it easier to build and test ideas swiftly.
- Strong Community Support: A vibrant community means plentiful resources, tutorials, and solutions to common issues can be readily accessed.
Key Features of API Servers
The significance of identifying key features in API servers cannot be overstated. These features directly impact both the performance and usability of the API. A well-designed API server should not just deliver data, but also ensure that it does so in a dependable and efficient manner. Therefore, understanding these features is crucial for developers and IT professionals who aim to build high-quality Python API servers. Here are the key attributes that contribute to a successful API server.
Statelessness
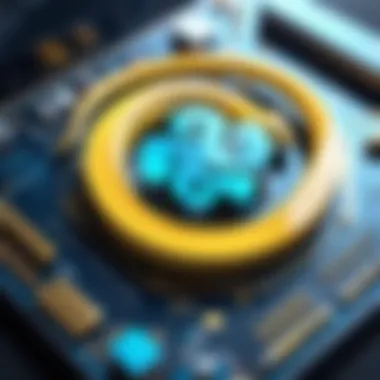
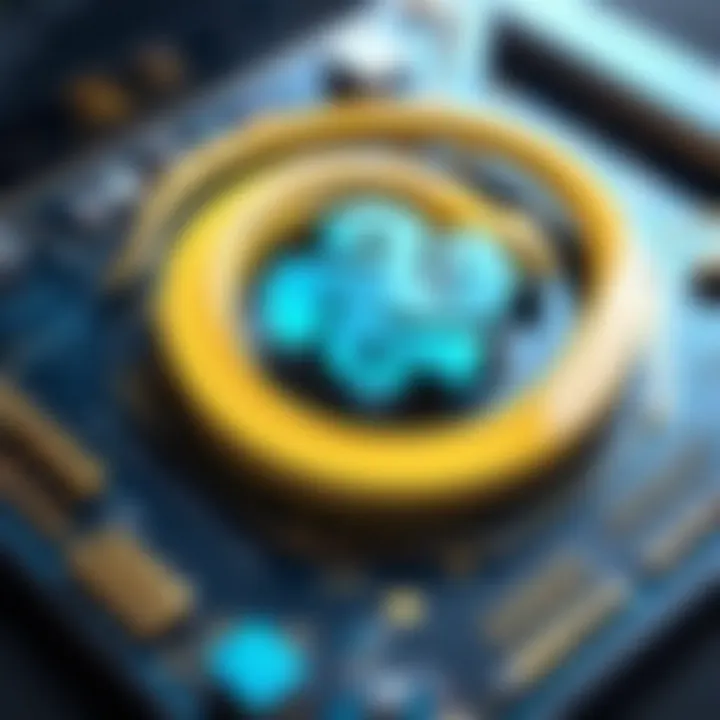
Statelessness is a core feature of RESTful architecture. In a stateless API server, each request from a client must contain all the information the server needs to process that request. This approach eliminates the need for the server to retain data about client sessions. The main advantage here is scalability. Since there is no session information stored on the server, it can handle more simultaneous requests. This design simplifies server management and improves performance under high load.
Statelessness can also enhance fault tolerance. When a server instance fails, there is no loss of session data, and clients can simply reconnect to another server instance without any disruption. Moreover, this feature aligns well with cloud-based environments where resources can be dynamically allocated based on demand.
Scalability
Scalability relates to the ability of an API to handle increased loads effectively. An effective API server must be capable of expanding its resources to manage growing requests and data traffic without sacrificing performance. There are two main types of scalability: vertical and horizontal.
- Vertical scaling involves upgrading the existing server’s hardware capabilities.
- Horizontal scaling, on the other hand, refers to adding more servers to distribute the load.
Python frameworks such as Flask and FastAPI are designed for horizontal scalability, making them suitable for building systems that need to scale as demand grows. Careful architecture planning, like implementing load balancers, also plays a significant role in ensuring that the server can manage scaling efficiently.
Interoperability
Interoperability is the ability of an API to engage with different systems and platforms. This is increasingly essential as services become more integrated. An interoperable API allows different software applications to communicate with one another, regardless of how they are built or the technologies they use.
For a Python API server, using standards such as JSON and XML ensures that it can work well with various clients. Additionally, adopting common protocols like HTTP and WebSocket enhances the API's ability to interface with a larger array of services.
To summarize, each of these features plays a vital role in determining the effectiveness of a Python API server. Statlessness contributes to scalability and fault tolerance, while scalability itself is crucial for performance under load. Interoperability ensures that diverse systems can work together, making the API more useful in integrated environments.
Frameworks for Building Python API Servers
In the realm of Python development, the choice of frameworks for building API servers is pivotal. Each framework brings unique features that can substantially impact the development process, performance, and scalability of the applications. Understanding these frameworks helps developers select the one that aligns with their specific needs and projects. The following sections dive into three prominent frameworks: Flask, Django REST Framework, and FastAPI. Each offers distinct advantages and considerations that developers should be aware of.
Flask
Flask is often appreciated for its simplicity and lightweight nature. It follows a minimalist approach that allows for easy expansion through a variety of extensions. This flexibility makes Flask ideal for small to medium-sized applications and for developers who prefer fine-tuning their applications with more control.
Key Features of Flask:
- Microservice Architecture: Flask is designed to support microservices, allowing developers to easily create small, manageable services.
- Jinja2 Templating Engine: This feature provides developers with the ability to create dynamic web pages easily.
- Extensive Documentation: The Flask community maintains comprehensive documentation, which is beneficial for both beginners and experienced developers.
Flask's learning curve is relatively mild, allowing new developers to experiment without a significant investment in time or resources. However, when scaling, developers might need to implement custom solutions, which can increase complexity.
Django REST Framework
Django REST Framework (DRF) is an extension of Django tailored specifically for building APIs. It is known for its robustness and comprehensive features that simplify the development of RESTful APIs. DRF is suitable for larger applications requiring complex functionalities and comprehensive user authentication.
Key Features of Django REST Framework:
- Built-in Authentication: DRF provides various authentication mechanisms, ensuring that securing APIs is straightforward.
- Serialization: The serialization process in DRF simplifies data representation, making it easier to convert complex data types to and from JSON.
- Admin Interface: Its built-in admin interface allows for easy manipulation of content and user data.
The main advantage of DRF is its capability to handle larger project complexities while adhering to established security standards. The downside may be its steep learning curve in comparison to Flask, particularly for those unfamiliar with Django.
FastAPI
FastAPI is a modern web framework that is gaining ground due to its superior speed and ease of use. It harnesses Python's type hints and offers automatic interactive API documentation generated by Swagger. This framework is particularly appealing for applications that require high performance and quick deployment.
Key Features of FastAPI:
- Asynchronous Support: FastAPI's async capabilities allow for handling multiple requests simultaneously, which enhances performance significantly.
- Automatic Data Validation: The framework automatically validates request data using Python type hints, reducing potential errors.
- OpenAPI Integration: FastAPI integrates seamlessly with OpenAPI, enabling automatic generation of detailed API documentation.
FastAPI stands out among other frameworks in terms of speed and performance, making it an excellent choice for API services where efficiency is paramount.
Comparative Overview
When comparing Flask, Django REST Framework, and FastAPI, it becomes apparent that the choice largely depends on the project requirements.
- Flask is beneficial for those who require minimalism and flexibility, as it lets developers build applications piece by piece.
- Django REST Framework excels in comprehensive features, suitable for larger, more complex applications that demand strict security and robust functionality.
- FastAPI is ideal for high-performance needs, especially for applications that extensively leverage asynchronous programming.
Designing API Endpoints
Designing effective API endpoints forms a crucial part of building an API server. Well-thought-out endpoints can enhance usability, ensuring that clients can interact with your server efficiently. The design process involves adhering to principles that promote clarity and maintainability. A robust endpoint design aids developers in swiftly understanding how to utilize the API without excessive documentation.
RESTful Principles
RESTful architecture is a guiding principle for designing API endpoints. REST stands for Representational State Transfer, and it relies on a stateless communication protocol—typically HTTP. In a RESTful design, APIs are structured around resources, and each resource is identified by a unique URL. This is important because it allows for clear organization of resources, making it easier for clients to navigate your API.
Key features of REST include:
- Uniform Interface: This simplifies architecture by ensuring that all interactions are made through standard HTTP methods like GET, POST, PUT, and DELETE. Each method denotes specific actions, enabling a predictable way to interface with the API.
- Statelessness: Each API call must contain all the information needed to understand the request. This fosters scalability, as it allows the server to process requests without maintaining session information between calls.
- Cacheability: Responses must define themselves as cacheable or non-cacheable. This can lead to enhanced performance, reducing the need for repeated server queries.
Following RESTful principles not only streamlines interaction but also sets a clear expectation for how consumers will integrate with the API.
CRUD Operations
CRUD stands for Create, Read, Update, and Delete. These four operations represent the basic actions that can be performed on resources in a RESTful API. Implementing these operations involves mapping them to the respective HTTP methods:
- Create is handled by the POST method. This is used to create new resources.
- Read corresponds to the GET method, fetching the resource data as required.
- Update involves the PUT or PATCH methods, modifying existing resources.
- Delete uses the DELETE method to remove resources.
Understanding CRUD is essential because it lays the foundation for data manipulation within the API. Moreover, it promotes consistency across different endpoints, making it intuitive for users to interact with the server.
Versioning APIs
API versioning is critical for maintaining backward compatibility while evolving the API. As applications grow, changes in the endpoint or data structure may disrupt existing clients. Hence, versioning allows for the introduction of new features or fixes without impacting those who use older versions.
Several strategies exist for versioning, including:
- URI Versioning: Including the version number in the endpoint. For example, "/api/v1/resource" makes it clear which version is in use.
- Query Parameters: Adding a version number as a parameter in the query string, like "/api/resource?version=1.0".
- Header Versioning: Clients specify the desired version via custom headers, though this can be less visible than other methods.
Choosing a versioning strategy depends on the particular needs and audience of the API. Nevertheless, it is advisable to document the versioning method clearly, ensuring that users can easily find the appropriate details for their specific setup.
Implementing Authentication and Authorization
Implementing authentication and authorization is critical in the development of any API server, including those built with Python. The primary objective of these mechanisms is to protect sensitive data and ensure that only authorized users can access specific resources. In an era where data breaches and compromised accounts are prevalent, robust authentication and authorization methods can be the differences between secure applications and vulnerable ones. Furthermore, they help maintain the integrity of user sessions and safeguard against various cyber threats.
Securing an API involves not just confirming the identity of a user but also determining what they are permitted to do. This dual process is vital for establishing trust in your application. Implementing these systems requires thoughtful consideration of various factors, including the types of users, collaborations with third-party services, and compliance with industry standards.
Token-Based Authentication
Token-based authentication is a widely used method in API development. In this scheme, users authenticate themselves with their credentials, such as a username and password. Once authenticated, they receive a token, typically a JSON Web Token (JWT), that serves as proof of their identity for subsequent requests.
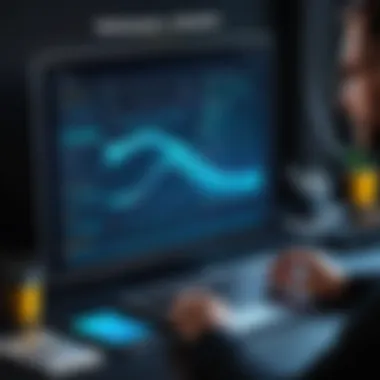
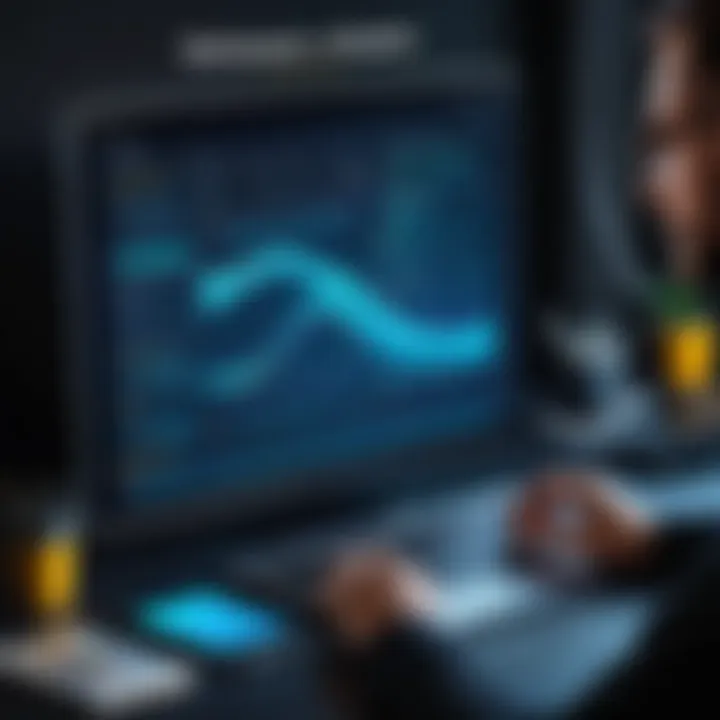
Tokens are sent along with each API call, enabling the server to verify the user's identity without needing to check credentials repeatedly. This process improves both security and performance. Tokens have several advantages:
- Stateless: The server does not store session data, leading to lower resource usage.
- Scalability: With tokens, you can easily distribute the load across multiple servers.
- Cross-domain support: APIs can be accessed from different domains without dealing with cookies and browser restrictions.
However, developers should also understand the implications of token expiration and revocation, as these factors can impact user experience and security.
OAuth 2. Overview
OAuth 2.0 is another critical aspect of API authentication that allows secure delegated access. This protocol enables third-party applications to obtain limited access to user accounts on an HTTP service, without exposing user passwords. It is widely used by well-known services like Google, Facebook, and GitHub.
OAuth 2.0 operates through a series of defined roles, including the resource owner, resource server, client, and authorization server. The workflow generally follows these steps:
- User authorization: The user interacts with the client application and is redirected to the authorization server.
- Granting access: The user agrees to permit the client access to their resources, resulting in the issuance of an access token.
- Resource access: The client uses this token to access the user's resources on the resource server.
The main benefits of OAuth 2.0 include:
- User control: Users can manage what data they share with third-party applications.
- Limited scope: The access granted can be custom-tailored, minimizing the risk of over-privileged access.
JWT Implementation
JSON Web Tokens (JWT) are a compact, URL-safe means of representing claims to be transferred between two parties. In the context of API authentication, they are often used to convey the user's identity and claims without the need for the server to store session information.
Implementing JWT in your Python API can be achieved through libraries such as . Here’s a simple example of how to create and verify a JWT:
In this example, we generate and validate a token that includes the user ID and an expiration time. Implementing JWT can enhance the authentication process by providing a streamlined and secure user experience.
In summary, authentication and authorization are foundational components in API security. By employing methods like token-based authentication, OAuth 2.0, and JWT, developers can ensure their applications are more secure and provide better user experiences.
Performance Optimization Techniques
In developing a Python API server, performance optimization is crucial. It enhances the overall speed and efficiency of the application, leading to a better user experience. As APIs become integral to many applications, ensuring they perform well is not just a technical preference but a necessity. Techniques for performance optimization help in reducing latency, increasing throughput, and ensuring stability under load. By employing these methods, developers can maximize resource utilization and minimize operational costs. Below are key strategies for optimizing the performance of a Python API server.
Caching Strategies
Caching is one of the most effective strategies for improving API performance. By storing frequently accessed data closer to the point of use, caching reduces response times and decreases the load on the server. It can be implemented at different levels:
- In-memory Caching: This involves storing data in memory for quick access. Libraries like Redis or Memcached are often used for this purpose. They provide high-speed data storage, making retrieval extremely fast.
- HTTP Caching: Utilizing HTTP headers such as , developers can instruct clients and intermediate caches on how to handle responses. For instance, marking responses as cacheable can drastically improve performance for static resources.
- Application-Level Caching: Within the API, certain computations can be stored to avoid redundant processing. This helps in scenarios where data does not change frequently.
Implementing caching requires a balanced approach. While it can improve performance, developers must also ensure cache coherence, managing expiration and validation efficiently.
Database Optimization
Databases often become a bottleneck in API performance. Optimizing database queries can lead to significant improvements. Here are some strategies:
- Indexing: Properly indexing database tables can dramatically speed up query responses. Indexes allow the database management system to locate data without scanning the entire table.
- Query Optimization: Analyzing and rewriting queries for efficiency can reduce processing time. The use of joins, subqueries, and avoiding unnecessary fields can enhance performance.
- Connection Pooling: Managing database connections using pooling keeps the application responsive. Instead of opening a new connection for every API request, pooled connections can be reused.
- Data Sharding: For very large datasets, sharding can distribute data across several databases, thus improving load times and performance. This requires careful management but can be beneficial for scalability.
Load Balancing
Load balancing distributes incoming API requests across multiple servers. This technique improves application availability and reliability. Key aspects include:
- Horizontal Scaling: Adding more servers can handle increased traffic. Load balancers distribute requests, ensuring no single server is overwhelmed.
- Failover Systems: A good load balancing strategy includes failover capabilities. If one server fails, the system can redirect requests to available servers without interruption.
- Session Stickiness: Often, it's necessary to route a user’s requests to the same server. Implementing session stickiness helps maintain user sessions without losing state.
Load balancing can be achieved through hardware or software solutions, including Nginx and HAProxy. Choosing the right method depends on the specific use case and traffic patterns.
Efficient performance optimization techniques can make a significant difference in how users interact with an API. Well-optimized servers not only enhance user experience but also save costs in the long run.
Testing Your API Server
Testing an API server is crucial to ensure that the server behaves as expected and meets user requirements. A well-tested API increases reliability and helps in identifying issues early in the development process. This is especially important as clients rely on the API to interact with services. Proper testing not only enhances the quality of the service but also improves user experience.
One of the primary benefits of testing is the early detection of bugs. This means problems can be fixed before they reach production, minimizing disruptions for end-users. Another significant advantage is providing documentation on how the API behaves under different conditions. This can be useful for both developers and users.
Moreover, testing ensures that all features of the API are working correctly. Different methods, such as unit tests and integration tests, provide specific scopes of validation. It is important to have a structured approach to testing, incorporating both automated and manual testing strategies to achieve thorough coverage.
Testing ensures that the API is reliable, performant, and secure before being released into production.
Unit Testing
Unit testing focuses on individual components of the API. This involves testing each function or method to confirm it behaves correctly in isolation. Unit tests check for valid inputs, and responses and confirm that corner cases are also handled appropriately.
Python provides several frameworks for unit testing, such as and . These frameworks allow developers to write tests that can be executed regularly, ensuring consistent performance over time. Additionally, unit tests are typically easier to maintain because they should be simple and direct.
Example of a simple unit test using :
Integration Testing
Integration testing examines how various components of the API work together. This goes beyond unit testing by ensuring that integrated modules function as intended. This type of testing can reveal issues such as data flow problems and interaction flaws between different parts of the API.
Using tools like Postman or pytest, integration tests can simulate actual requests to confirm that all components respond correctly when combined. A comprehensive set of integration tests minimizes the risk of failure when they are deployed in a live environment.
Integration tests help verify that endpoints return the expected responses and that they can communicate effectively with any databases or external services the API relies on.
Postman for API Testing
Postman is a powerful tool for API testing, allowing developers to create and organize tests easily. It provides a user-friendly interface to send requests and receive responses. Postman's ability to use collections and environments makes it very flexible for testing different scenarios and configurations.
Developers can create tests within Postman by writing scripts that validate the returned data. This functionality enables automation of testing processes, ensuring consistent results every time changes are made to the API.
To get started with Postman:
- Create a new request in Postman.
- Select the HTTP method and add the endpoint URL.
- Configure any required headers or body data.
- Execute the request and observe the response.
Postman is great for exploratory testing, allowing developers to quickly validate their endpoints without writing extensive scripts. Overall, its integration into the development workflow can significantly enhance the API testing process.
Security Considerations
In today's digital world, the security of an API server is paramount. A breach can lead to data leaks, unauthorized access, and irreparable damage to an organization’s reputation. Therefore, understanding key security considerations is essential when developing and managing a Python API server. Implementing robust security measures not only safeguards sensitive user information but also builds trust with users and clients. There are several critical components to focus on, such as data encryption, handling SQL injections, and rate limiting.
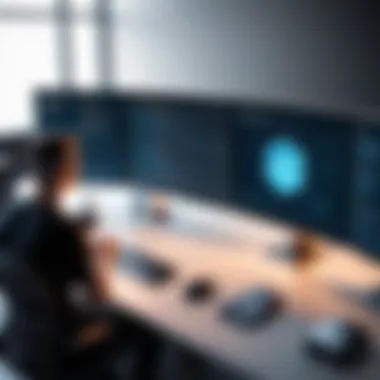
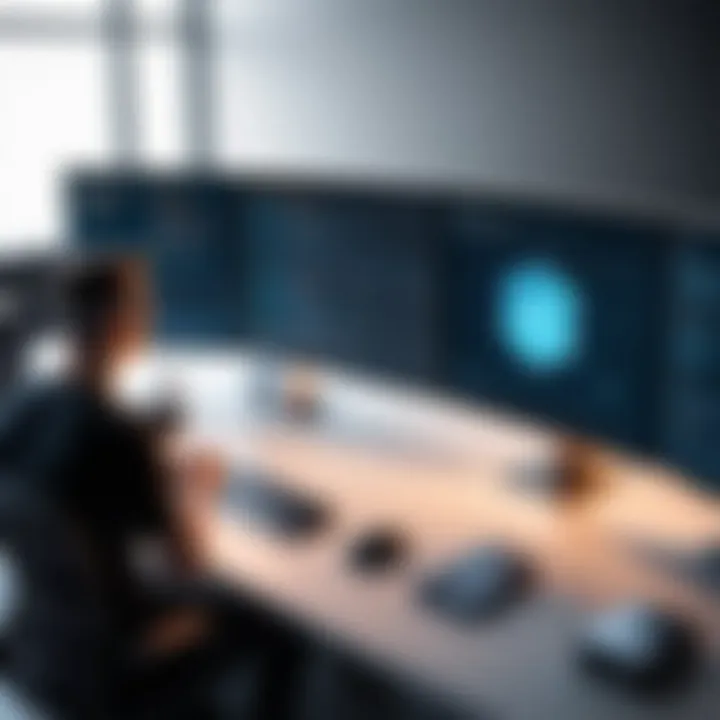
Data Encryption
Data encryption plays a pivotal role in protecting data in transit and at rest. When an API transmits data, it is open to interception by malicious actors. Secure Socket Layer (SSL) should be employed to encrypt data exchanged between the client and server. This ensures that even if data is intercepted, it remains unreadable without the proper decryption key.
Beyond transport-level encryption, it is also vital to consider encrypting sensitive information, such as passwords and personal identification numbers. Using strong algorithms, like AES (Advanced Encryption Standard), will enhance the security of stored data.
"Encryption is not only crucial for data protection but also a compliance requirement for many regulations like GDPR and HIPAA."
Handling SQL Injections
SQL injection is a common exploitation technique where an attacker can manipulate queries to gain unauthorized access to database information. To combat this threat, it is necessary to utilize parameterized queries or prepared statements. This practice ensures that user input is correctly handled and executed as data only, rather than executable code.
Additionally, it is advisable to validate and sanitize all user inputs. Implementing a robust error handling strategy can also assist in identifying and mitigating unintended SQL injection vulnerabilities.
Implementing security practices throughout the development lifecycle is crucial in defending against these threats.
Rate Limiting
Another significant aspect of API security is rate limiting. This technique restricts the number of requests a user can make to the API within a specified timeframe. By setting limits, an API can combat abusive behaviors, including denial-of-service (DoS) attacks, which overwhelm the server with excessive requests.
Additionally, rate limiting helps in ensuring fair usage of resources among users. Common implementations of rate limiting involve using tokens, where each request consumes a token, and once depleted, the requests are denied until a reset period.
Deployment Strategies
Deployment strategies are crucial for ensuring that a Python API server operates efficiently and is readily accessible to users. The choice of deployment method can significantly influence the performance, scalability, and reliability of the API. Therefore, understanding various deployment options can aid developers in making informed decisions. This section will explore three key areas: Cloud Deployment Options, Dockerizing Your API, and Monitoring and Logging.
Cloud Deployment Options
Cloud deployment of Python API servers offers various advantages. It provides flexibility and the ability to scale according to traffic demands. Major cloud providers, such as Amazon Web Services, Google Cloud Platform, and Microsoft Azure, offer robust infrastructure to host applications. By using cloud services, developers can leverage managed solutions, reducing the burden of server management.
When considering a cloud deployment, a few factors should be taken into account:
- Cost structures: Understand the pricing model of your chosen provider to avoid unexpected charges.
- Ease of integration: Choose a platform that seamlessly integrates with other services you might need.
- Support and documentation: Opt for a provider that offers ample support and clear documentation.
Using cloud deployment also allows for easier implementation of CI/CD (Continuous Integration and Continuous Deployment), enhancing the development workflow.
Dockerizing Your API
Docker has become a popular choice for deploying applications because it simplifies the process of packaging applications into containers. Containerization isolates the application and its dependencies, ensuring consistent behavior across various environments. By Dockerizing your Python API, you can achieve greater efficiency in deployment and scaling.
Key benefits of using Docker include:
- Isolation: Each API instance runs in its container, reducing conflicts between dependencies.
- Portability: Docker containers can run on any machine that has Docker installed, providing flexibility in deployment.
- Scalability: Docker can efficiently manage multiple instances of your API server to handle increased loads.
To dockerize your Python API, you can create a that specifies the base image, the application code, and how it should run. Here is a simple example:
Monitoring and Logging
After deploying your API, it is critical to ensure its health and performance. Monitoring and logging provide valuable insights into the API's operations, allowing you to quickly identify and address issues.
Incorporating a monitoring solution helps track various metrics such as:
- Response times: Understanding how quickly your API responds to requests can unveil potential performance bottlenecks.
- Error rates: Monitoring error rates can help highlight issues before they escalate into larger problems.
- Traffic patterns: Analyzing traffic can aid in forecasting load and planning scaling operations.
Tools such as Prometheus for monitoring and ELK Stack (Elasticsearch, Logstash, and Kibana) for logging can be invaluable assets in maintaining a healthy API environment.
Monitoring your API is like putting a safety net under a tightrope walker; it is essential to catch issues before they fall into production.
Documentation and User Support
Effective documentation and user support are crucial elements in the realm of API development. They serve not only as a guiding framework for users but also contribute significantly to the API's longevity and usability. When developers create an API, they should see documentation as an essential part of the product rather than an afterthought. High-quality documentation improves the overall experience of using the API, reducing friction for end-users or developers integrating the API into their applications.
Benefits of Comprehensive Documentation
- Clear Instructions: Proper documentation provides detailed instructions for using the API, covering everything from setup to usage examples. This clarity minimizes user confusion and enhances satisfaction.
- Troubleshooting Guide: A well-structured documentation accompanies users when they face problems. Having a dedicated troubleshooting section can save time and effort for both developers and users.
- Onboarding New Users: Good documentation is invaluable for onboarding new users. It allows them to get up to speed quickly, increasing their confidence in using the API.
- Influence on Adoption: APIs with comprehensive and clear documentation are more likely to be adopted by other developers. If a potential user finds the documentation lacking, they may look elsewhere.
Considerations for User Support
User support complements documentation by addressing issues that users may encounter in real-time. While documentation can often answer questions, there will always be unique cases that need direct intervention from support teams. Efficient user support ensures that these cases don’t become obstacles to successfully using the API.
Importance of API Documentation
API documentation not only informs users about available features but also dictates how they will interact with the API. High-quality documentation typically includes:
- Overview: A concise summary of what the API does.
- Authentication Details: Information on how to authenticate and access the API.
- Endpoint Descriptions: Each API endpoint should have detailed descriptions, including parameters and response formats. This specificity helps in understanding how to make valid requests.
- Examples: Providing code snippets and usage examples can vastly improve the learning curve.
- Error Messages: Clear explanations of error messages that users may encounter help users quickly troubleshoot their issues.
Tools for API Documentation
Choosing the right tools to create API documentation is critical. Here are some popular tools that can streamline the documentation process:
- Swagger/OpenAPI: This tool allows you to create a standard specification for your API. It visually documents the endpoints and allows for interactive testing.
- Postman: Known for its API testing capabilities, Postman also provides tools for generating documentation from API collections, making it easier to keep documentation updated.
- GitHub Pages: For those familiar with Git, GitHub Pages offers a straightforward way to publish documentation directly from a repository.
In summary, investing time and resources into documentation and user support can yield substantial benefits. Creating a solid foundation in documentation not only reflects well on the API but also fosters a supportive developer community.
Future Trends in Python API Development
In the rapidly changing realm of software development, understanding future trends in Python API development is crucial. As technology evolves, so do the needs of businesses and developers. Organizations are continually looking for more efficient, scalable, and secure ways to create and manage APIs. This section explores important trends that are shaping the development landscape and discusses their implications.
Serverless Architectures
Serverless architecture has gained traction in recent years due to its ability to simplify deployment and scale applications efficiently. With serverless models, developers can focus on writing code without managing the underlying infrastructure. This approach is particularly relevant for Python API developers, as it allows for rapid development cycles and cost-efficiency.
Benefits of serverless architectures include:
- Automatic Scaling: Serverless solutions automatically adjust resources based on demand. This ensures ideal performance during peak usage without the need for manual intervention.
- Cost-Effectiveness: Users are billed only for the resources they consume, which can significantly reduce costs compared to traditional hosting options.
- Faster Development: Developers can deploy applications quickly, fostering innovation and reducing time to market.
Despite the advantages, there are considerations to keep in mind. There may be vendor lock-in, which limits flexibility. Additionally, debugging in a serverless environment can be more complex due to abstracted layers. It is essential to assess the specific needs and capabilities of your API project before adopting this model.
Microservices Approach
The microservices architecture is another trend that is influencing Python API development. This approach involves breaking down applications into smaller, independent services that focus on specific functionalities. Each microservice can be developed, deployed, and scaled independently.
The advantages of adopting a microservices approach include:
- Improved Maintainability: Smaller codebases are easier to manage and update, allowing for quicker iterations and enhancements.
- Technology Agnosticism: Development teams can use different technologies for different services, enabling the selection of the best tools for each task.
- Resilience: Since each service operates independently, the failure of one does not compromise the entire application.
However, managing multiple services can introduce complexities, such as ensuring consistent communication between them. It requires a thoughtful orchestration strategy, often implemented with API gateways and service discovery tools.
"Understanding these trends allows developers to make informed decisions about the architecture that best meets their needs."