Mastering React: A Complete Guide for Developers
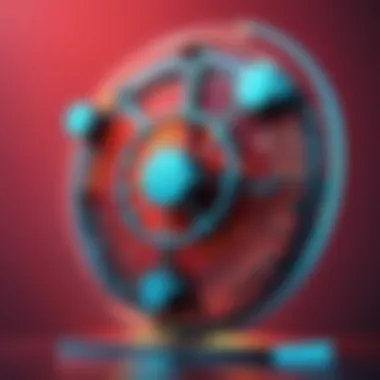
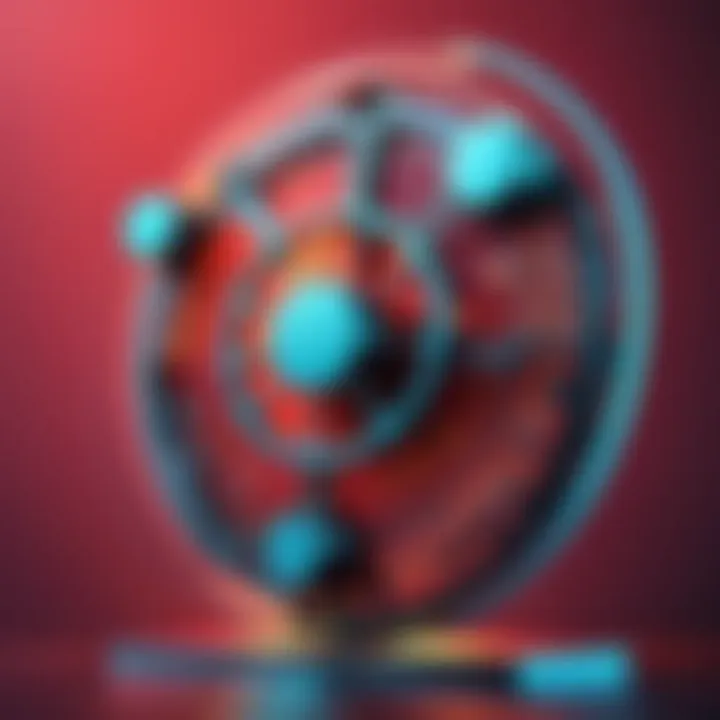
Overview of Topic
Building web applications is becoming more integral in today’s tech landscape. Among the myriad of tools available, React stands out like a beacon for developers aiming to create dynamic interfaces with efficiency and ease. React, a JavaScript library for building user interfaces, was developed by Facebook and has undergone significant evolution since its inception in 2013. Its component-based architecture not only encourages code reusability but also promotes an organized approach to development.
The significance of React in the tech industry cannot be overstated. As companies continue to demand faster and more responsive applications, React has surged in popularity due to its robust ecosystem and vibrant community. The ability to seamlessly integrate with other libraries and frameworks makes it an adaptable choice for diverse projects, ranging from single-page applications to complex progressive web apps.
"React’s flexibility and performance have made it a quintessential tool in web development today."
In terms of its historical context, React was released by Facebook as an internal tool to optimize the user interface of its platforms, particularly for managing complex data states. Over time, the library's open-source model has attracted millions of developers, leading to a rich repository of resources and a thriving community, which are essential for learning and problem-solving in real-world applications.
Fundamentals Explained
At its core, React operates on several principles that are crucial for both new and seasoned developers. Understanding these fundamental concepts can significantly bolster one’s capacity to utilize React effectively.
Core principles
- Component-Based Architecture: React breaks down user interfaces into reusable components, allowing developers to encapsulate data and behavior, promoting organization.
- Declarative UI: Instead of manipulating the DOM directly, React allows developers to design views based on the state of the application, making it easier to anticipate changes and manage updates.
- Unidirectional Data Flow: Data flows in one direction, leading to predictable outcomes and easier debugging.
Key terminology
- JSX: A syntax extension allowing HTML-like syntax in JavaScript, providing a clear and concise way to create UI elements.
- Props: Short for properties, these are how data is passed between components.
- State: An object that holds information about the component’s current situation.
Practical Applications and Examples
Real-world applications of React showcase its versatility and performance. Companies like Facebook, Instagram, and Netflix utilize React to deliver scalable and efficient user experiences. Practical examples can help elucidate how this is achieved.
Demonstration
In building a simple React application, you might start with component creation:
This snippet showcases a basic functional component, a fundamental building block of a React application.
Advanced Topics and Latest Trends
As technology continues to evolve, so does React. New features, such as React Hooks, have transformed how we manage state and side effects in functional components. This shift simplifies component logic and encourages best practices.
With the rise of server-side rendering and static site generation, frameworks like Next.js are gaining traction. These advancements enable faster load times and better SEO performance, which are essential for modern web applications.
Tips and Resources for Further Learning
For those eager to deepen their understanding of React, several resources exist:
- Books: "Learning React" and "React Up & Running" provide foundational and advanced material.
- Online courses: Platforms like Udemy and Coursera offer comprehensive courses ranging from beginner to expert levels.
- Communities: Engaging with forums on Reddit or the Reactiflux Discord channel can provide insights and support from other developers.
Tools to consider:
- Create React App: A boilerplate tool for starting new React projects.
- Redux: A state management library that integrates well with React for managing complex data flows.
By familiarizing yourself with these resources, tools, and foundational concepts, you will be better equipped to tackle challenges and innovate in the exciting world of React development.
Prelims to React
In the ever-evolving landscape of web development, knowing the tools and frameworks available is paramount to creating effective, scalable applications. React is at the forefront of this movement, regarded as one of the most popular libraries used for building user interfaces. It's remarkably potent yet simple enough for newcomers to grasp quickly. This article's section on React serves as an important foundation, guiding readers through its intricacies, benefits, and practical applications.
As the digital world increasingly pushes for interactive and dynamic designs, React fulfills a critical role. Understanding React isn't only about learning to code—it’s about fostering a mindset that embraces component-driven designs and efficient state management. Moreover, React's ecosystem is vibrant, with an abundance of libraries and tools that support and enhance its functionality, further expanding its appeal to developers.
This section paves the path towards grasping essential concepts, enabling a seamless transition into more advanced topics later in the guide.
What is React?
React is a powerful JavaScript library created by Facebook for building user interfaces, particularly single-page applications. At its core, React promotes the idea of building modular components, which can be reused throughout an application. Each of these components encapsulates its own rendering logic, meaning that managing an application's UI becomes much more straightforward. Instead of dealing with convoluted codebases filled with mixed responsibilities, React encourages developers to create discrete building blocks, leading to clearer and more maintainable code.
The Virtual DOM is a distinctive feature of React. This lightweight copy of the actual DOM allows React to determine what parts of the UI need to be updated or rendered anew. Rather than updating the entire UI every time a change occurs, React efficiently calculates the minimal changes needed. This efficiency is a significant reason behind React's speed and performance, making it suitable for building high-performance applications.
Why Choose React for Web Development?
Selecting the right framework for web development can be daunting, yet React makes a strong case with several compelling reasons:
- Component-Based Architecture: Developers can create encapsulated components that can be reused, which simplifies both development and future updates.
- Reusable Code: Once a component is built, it can be used across different parts of the app, minimizing redundancy and making maintenance easier.
- Strong Community Support: With a vast ecosystem and a thriving community, React provides a wealth of resources and tools—including libraries like Redux for state management.
- SEO-Friendly: React can be rendered on the server side, making it more accessible for search engines to crawl and index content, a key aspect for businesses.
- Speed and Performance: Thanks to its Virtual DOM implementation, applications can perform optimally, even with rich user interactions.
The decision to choose React ultimately rests on the specific needs of a project. However, its robust features and the support from its community certainly stack the odds in its favour, making it an ideal choice for modern web development.
Setting Up Your Development Environment
Setting up your development environment is the backbone of any programming project. It’s like laying down a solid foundation before you start constructing a house. If you've ever jumped into a project without the right tools or configurations, you'll know that it can lead to a heap of headaches down the line. In the context of React applications, ensuring that your environment is correctly configured allows for smoother development and quicker debugging.
A properly prepared development space not only sets the stage for your coding but also helps you utilize various technologies efficiently, ranging from JavaScript frameworks like React to tools such as npm and Node.js. Having everything in place can significantly enhance productivity and can unearth potential issues before they bloom into bigger problems.
Prerequisites for Setting Up
Before rolling up your sleeves and diving into React, there are a few prerequisites you should tick off your list. Getting the basics straight will save you from much stress later. Here are some key points to consider:
- Basic Understanding of JavaScript: React is a JavaScript library; hence, familiarity with its syntax and core concepts is essential.
- Familiarity with HTML and CSS: Since you'll be rendering components that form the visual aspect of your application, a grasp of HTML and CSS is necessary.
- A Code Editor: Popular choices include Visual Studio Code, Atom, or Sublime Text. Pick one that feels comfortable to you.
- Version Control: Knowing Git can be beneficial for tracking changes and collaborating with others.
Thinking ahead by equipping yourself with these skills helps establish a smoother coding experience and aids in the transition to more advanced React concepts.
Installing Node.js and npm
Node.js and npm are the dynamic duo that drives modern JavaScript development. Node.js provides the JavaScript runtime needed to run your applications outside the browser, while npm serves as the package manager to install libraries and additional tools you may require. Here's how to get them:
- Download Node.js: Navigate to Node.js's official site and download the version suitable for your operating system. Most users go for the LTS version, as it's more stable.
- Install Node.js: Once downloaded, open the installer and follow the instructions. The installation of Node.js will automatically include npm.
- Verify Installation: After installation, open your terminal or command prompt and type in:You should see version numbers for both Node.js and npm if everything is set up correctly.
With Node.js and npm at your command, you're armed with all you need to fetch React and its libraries, ensuring your experience unfolds without a hitch.
Creating Your First React Project
Now that your environment is primed and ready to go, it’s time to create your first React project. The most expedient way to spin up a new React application is by using Create React App, a comfortable CLI tool that handles the configuration and setup for you. Here's a step-by-step guide to get started:
- Open Terminal: Begin by launching your terminal or command prompt.
- Navigate to the Desired Directory: Use the command to navigate to the folder where you want to set up your project.
- Run Create React App Command: Execute the command:Replace with your chosen project name.
- Change into the Project Directory: After installation, switch to your project directory:
- Start the Development Server: Finally, launch your application by using:This will open your browser and display your new app, ready for exploration.
Once your first React application is out of the box, you have the fundamental structure for building your components and integrating your ideas into code.
Remember: Each step you take in setting up your environment and running your first project is not just about following instructions; it’s about laying a strong foundation crucial for successful development in the future. Prior preparation can save you from plenty of head-scratching moments.
Through careful setup and running simple commands, you're well on your way to becoming proficient in React!
Understanding React Components
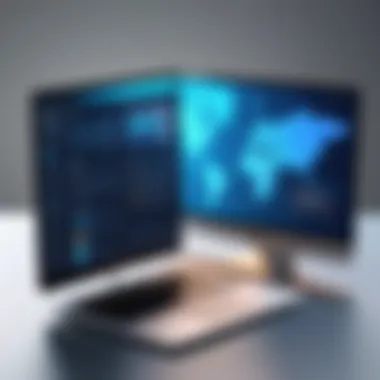
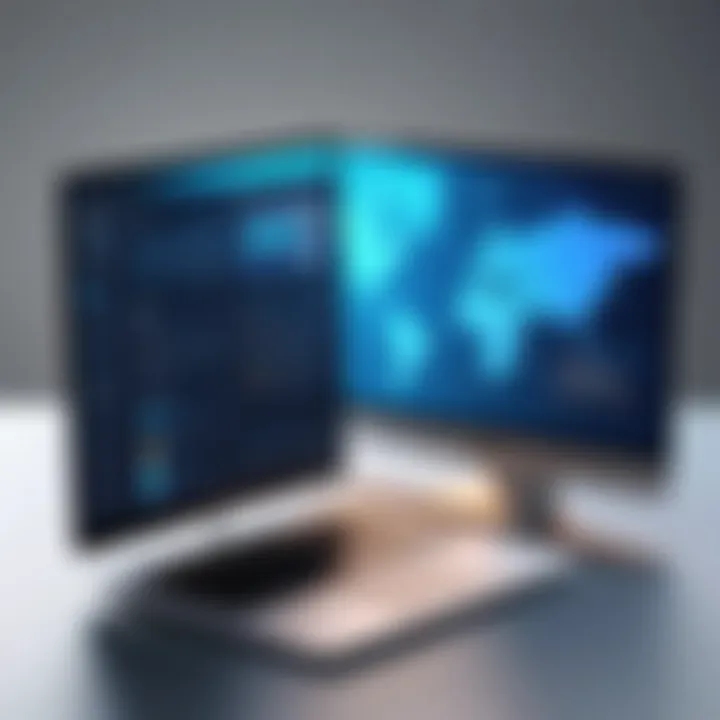
Components are the backbone of any React application. They are reusable, self-contained units that dictate how the UI behaves and appears. If React was a well-oiled machine, components would be the gears making it turn smoothly. Grasping the concept of components is crucial as it sets the stage for mastering React. You’ll find that working with components allows for a modular structure, making development and maintenance more manageable.
Functional Components vs. Class Components
In the realm of React, understanding the distinction between functional and class components is vital. Functional components are simpler and have become the preferred choice for many developers. They are JavaScript functions that return JSX. This straightforwardness fosters better readability and ease of testing. For instance, a functional component is often like a quick sketch of a character in a story - direct, concise, and focused.
On the flip side, class components provide more structure and can manage their own state through lifecycle methods. They can be compared to the full-blown narrative arcs we create in a novel - rich with detail but sometimes needing more attention to craft. However, the growth of Hooks in React has pushed developers towards functional components, even for complex applications.
In summary, while class components have their place, functional components are often more in line with modern React development, emphasizing simplicity and efficiency.
Props and State Management
When it comes to communication within components, props and state play significant roles. Props (short for properties) are read-only attributes passed from parent components to child components. You can think of them as the letters that a character receives in a story, providing crucial information that allows them to react accordingly. For example, if a button needs to know its label, it can receive this information via props.
State, on the other hand, is all about internal data that can change over time. It’s akin to the character’s emotions that can ebb and flow based on the plot twist; this internal state is defined and managed within the component itself. Using the useState hook, you can define state in functional components:
The seamless interaction between props and state is fundamental for creating dynamic web applications, allowing React developers to efficiently manage their data flows.
Lifecycle Methods in Class Components
Lifecycle methods are essentially the orchestration of a class component’s lifecycle, dictating how components behave at specific points of their existence. These methods tell a component when to mount, update, and unmount. When a class component is created, it's like preparing for a show. You have a cast of characters (your component), you rehearse how they'll act in different situations, and then you perform.
For example, is called after a component is first rendered, similar to when the curtain rises for the first act. You can fetch data or set up subscriptions here. In contrast, acts as the closing scene, where you clean up any remaining tasks, such as removing event listeners.
Even though functional components now utilize hooks for managing effects, knowing these lifecycle methods is essential for understanding React at a deeper level, especially when debugging or maintaining older codebases.
Understanding these key concepts of React components lays a strong foundation for building interactive and efficient web applications. The way components interact, share data through props, manage their own state, and respond to the lifecycle methods can significantly shape the development process.
Ultimately, mastery over these concepts allows developers to code with confidence, creating applications that are not only functional but also scalable.
Working with JSX
JSX, which stands for JavaScript XML, plays a pivotal role in React development. It's not just a syntax choice; it's a paradigm shift that allows developers to write HTML structures in JavaScript code. This integration bridges the gap between visual representation and the underlying logic of an application. Embracing JSX can fundamentally streamline the React development process, rendering the building of user interfaces not only intuitive but also efficient.
What is JSX?
JSX is essentially a syntax extension for JavaScript that resembles HTML. With JSX, components can define their structure using a familiar format, making it easier for developers to visualize how their components fit together.
One of the significant advantages of using JSX is the visual appeal it brings to the code. The clarity of combining markup and logic in a single file facilitates easier reading and maintenance. Rather than juggling between different files and formats, developers can keep the UI code and its behavior closely linked. However, it's worth noting that JSX is not mandatory when working with React. Developers can still create components using plain JavaScript, albeit at the cost of readability. For instance:
Compared to JSX:
The second option is certainly easier to grasp at first glance.
Embedding Expressions in JSX
JSX allows embedding expressions within curly braces. This feature significantly enhances its dynamism. By integrating JavaScript expressions directly into JSX, developers can create more interactive and compelling UIs.
For instance, you might want to display a user's name dynamically. Here’s how it can be done:
This flexibility makes JSX powerful, as it enables the incorporation of complex conditions and lists effortlessly. For example, you can conditionally render components based on a state variable, like so:
Important: Remember, only expressions can be embedded within the curly braces in JSX. Statements like or loops cannot be used here. If conditional logic is necessary, consider using ternary operators or functions that encapsulate the logic.
Styling Components with JSX
Styling in JSX can also present unique possibilities. To apply CSS styles, there are primarily two approaches: inline styling and external CSS files.
Inline Styling
In JSX, you can directly apply styles using an object:
Here, the style syntax resembles JavaScript object syntax. This approach is beneficial for dynamic styling based on props or state. However, inline styles have their limitations, such as not supporting pseudo-classes or media queries.
External CSS
For traditional styling, you can still link to external CSS sheets. This method keeps your JSX clean and separates markup from styling concerns, adhering to best practices. You might link a CSS file like:
Then in your CSS file, simply style your component classes as you normally would:
Managing State and Events
Managing state and events is crucial in React development. It’s where the magic happens, allowing your applications to react dynamically to user interactions. In simple terms, state represents the data that influences a component's rendering. When this data changes, the UI responds accordingly. Effective state management ensures your application remains fluid, responsive, and user-friendly.
For instance, consider a shopping cart application. As a user adds or removes items, the number in the cart needs to update instantly. Here, managing state effectively ensures that the displayed information matches the user's actions. Without proper state management, components could render stale data—definitely not what you want when aiming to create a seamless user experience.
Using the useState Hook
The hook is a fundamental building block when dealing with state in functional components. When you call , it returns an array containing two values: the current state and a function to update that state. This hook replaces the need for class components in many cases, simplifying how you manage state.
In the above example, when the button is clicked, updates the value. The UI is re-rendered automatically, reflecting the new state. This illustrates how the hook streamlines the process of handling state changes without the boilerplate of class components.
Handling User Input and Events
Understanding how to handle user inputs and events is another crucial aspect of state management. React makes this straightforward. By assigning event handlers to your components, you can easily capture and respond to user actions, such as clicks, key presses, or form submissions.
For example, imagine a simple form where users enter their names. You might want to update state every time they type something:
Notice how feeds the input’s current value back into the component’s state via . This is an example of controlled components, where form elements are bound to React state, ensuring that all changes are reflected right away in the UI. Leveraging these techniques enables developers to create intuitive interfaces that respond to user behavior immediately.
"In React, managing state is like maintaining the pulse of your application; if it's not in sync, everything loses rhythm."
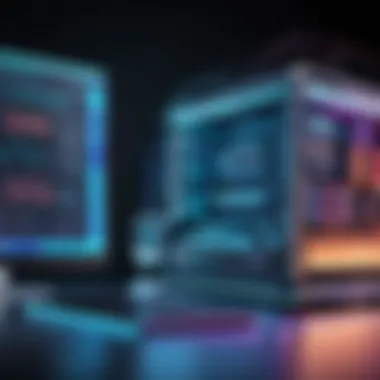
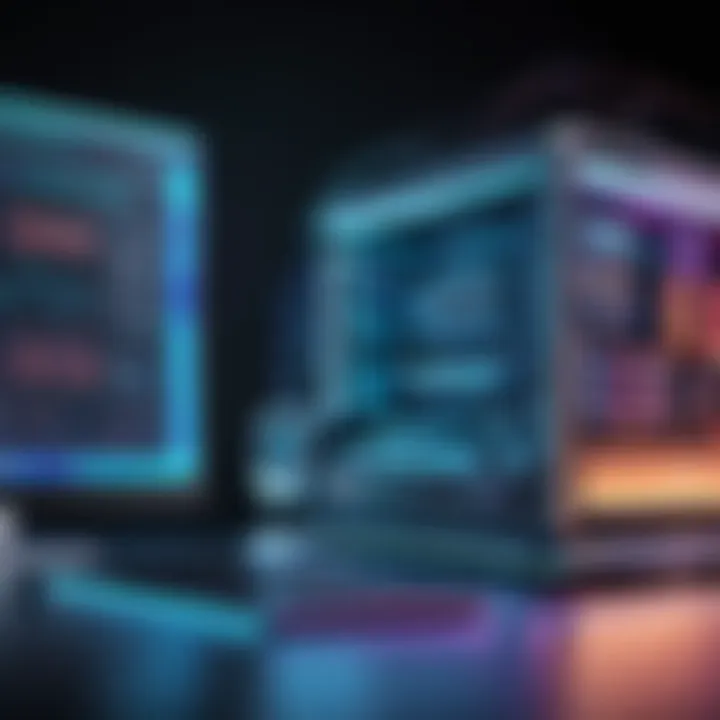
Ultimately, mastering state and events in React empowers developers to build dynamic, responsive applications that provide a seamless user experience. Whether it be through the hook or effective event handling, these concepts are foundational to a sophisticated understanding of React.
Data Fetching in React Applications
Data fetching is a cornerstone of modern web development, making it arguably one of the most critical elements in building React applications. Simply put, an application can often feel lifeless if it lacks real-time data updates or the ability to respond dynamically to user requests. In React, managing data effectively means the difference between a seamless user experience and an app that feels stagnant or unresponsive.
When you think about it, nearly every modern app needs to grab data from somewhere. Whether it’s pulling in user profiles, product listings, or the latest news articles, understanding how to fetch and handle that data smoothly within a React application is paramount.
Benefits of Data Fetching in React
- Real-time Updates: Data fetching allows your application to update in real time, ensuring users always get the most current information. This keeps user engagement high and ultimately improves user satisfaction.
- Decoupling UI and Data Logic: React's architecture promotes a clear separation between UI and data fetching logic. This not only enhances the maintainability of your applications but also leads to a clearer understanding of how data flows within your app.
- Enhanced Performance: Efficient data fetching can greatly improve the performance of your application. Techniques like lazy loading can help load only the data that is needed initially, reducing unnecessary overhead.
While there is much to gain, developers also have to grapple with a few considerations:
- Error Handling: Properly managing errors that may arise from failed API calls is essential. Without effective error handling, users may encounter unexpected behavior or blank screens.
- Loading States: Indicative loading states can enhance user experience by informing users that data is being fetched. Users appreciate knowing that an action is being processed, rather than leaving them in the dark regarding the status of their requests.
As you explore the topic of data fetching within React, let’s break down two common methods developers use to make HTTP requests: the Fetch API and the Axios library.
API Requests with Fetch
The Fetch API is a modern interface for making network requests in web applications. Since it is built directly into the browser, developers can access it without needing to download or manage any third-party libraries. The simplicity of syntax alone can be quite alluring.
Here's a quick example demonstrating how to use Fetch to request data:
This snippet shows how to request data from an API using Fetch. The process involves checking the response status, converting it to JSON, and handling any potential errors along the way. All in all, Fetch provides a straightforward yet highly effective means for data fetching.
Using Axios for HTTP Requests
While Fetch is an excellent native option for handling requests, many developers prefer using Axios, a promise-based HTTP client that can simplify certain aspects of the data-fetching process. What makes Axios particularly compelling is its intuitive API, automatic JSON data transformation, and built-in support for older browsers.
Here’s an example of making the same data request using Axios:
The Axios function here simplifies the request flow. Notably, it directly returns the response data, which can make the code cleaner and easier to follow.
In summary, data fetching in React is not merely an operational necessity; it also plays a pivotal role in ensuring that applications can provide engaging and user-centric experiences. Whether you opt for Fetch or Axios, understanding the nuances in how each handles requests, responses, and errors is key to building robust React applications.
Routing in React
Routing is at the heart of building interactive applications using React. As web applications grow larger, so does the need for managing different views or pages without reloading the entire page. React's virtual DOM makes this smoother and faster, but without routing, you might run into a real pickle when trying to navigate through your app’s various components. In short, implementing routing allows users to seamlessly navigate within your application, providing a more cohesive experience overall.
Foreword to React Router
React Router is the go-to library for adding routing capabilities to your React apps. It's incredibly handy because it allows you to manage navigation between your components using a declarative approach. This means you can think about your app in terms of routes and components, rather than the mechanics of rendering specific views. For instance, with React Router, your definitions of routes can become part of the component structure itself.
"Routing is crucial for an organized web application. Keeping your code structured ultimately leads to maintainability."
In a basic setup, you’d start by installing React Router. Here's how to do it:
From there, you can import , , and to manage your routes. Consider this basic example:
This snippet outlines a straightforward implementation where the app switches between the and components based on the URL. The component ensures that only one of the routes is rendered at a time, preventing display chaos.
Dynamic Routing Techniques
Dynamic routing is a step further, enabling your application to adjust routes in real-time based on user interactions or data. This flexibility is especially valuable when dealing with data-driven applications, where the content might change based on user input or API responses.
Let’s say you are building a blogging platform. Each post could have a unique URL based on its ID. With React Router, you can define a dynamic route that captures this ID:
In this case, is a wildcard that can match any number. Now, when a user navigates to , the component can use that ID to fetch the corresponding post data. Inside the component, you can access the ID like this:
Dynamic routing offers a more tailored experience for users, giving them access to detailed content while keeping your URL structure neat and manageable.
Summary
In short, understanding routing in React is pivotal for developing web applications that are both functional and user-friendly. React Router shines as a robust solution for integrating various components into a fluid navigation experience. From static routes to dynamic routes, mastering this aspect can elevate your application considerably, making it intuitive and resourceful.
Performance Optimization Techniques
In the realm of web development, especially when working with extensive libraries like React, the need for speed and efficiency cannot be overstated. When a React application is sluggish, users may abandon it faster than you can say "long loading times." Performance optimization isn’t just a luxury; it’s a necessity that can make or break a user’s experience. The right optimizations enhance responsiveness and keep the application running smoothly, ensuring users remain engaged.
Why Optimize React Applications?
When crafting a web application, one must consider how performance directly affects user satisfaction. A well-optimized React application is significantly more pleasant to navigate. Here are key points to consider when discussing the importance of optimization:
- User Retention: Users won’t stick around for slow load times or unresponsive interfaces. Studies show that even a one-second delay can lead to decreased engagement.
- SEO Benefits: Search engines prefer faster-loading websites. Optimizing your application can improve your site's visibility in search results, which is crucial for driving traffic.
- Resource Efficiency: Efficient apps consume less bandwidth and server resources. This lowers operational costs and enhances scalability, as more users can access your application concurrently.
- Competitive Advantage: In a crowded market, a high-performance application can set you apart from competitors. Users are more likely to recommend a fast and reliable application to peers.
"If you build it, they will come, but only if it’s easy to use and loads fast."
Memoization with React.memo
React comes bundled with tools that programmers can harness to enhance performance. One such tool is React.memo, a higher-order component that optimizes functional components. But what does that mean in practical terms? Let’s break it down:
- Preventing Unnecessary Re-renders: By default, functional components will re-render with every parent component update. can help prevent this by memorizing the result of a rendered component. If the props have not changed, React skips the rendering process, which can substantially speed up your application.
- Use Cases: It’s particularly effective for components that receive complex objects as props or maintain information that doesn’t change frequently. For instance, if a component only shows user details but doesn't change with every keystroke in an input field elsewhere, memoization is the way to go.
- Implementation: Implementing is straightforward. Here’s a simple example:
Using this method, only re-renders if the prop changes, effectively improving performance.
In essence, employing can sharpen your application’s responsiveness. It’s crucial to understand when and where to use memoization to avoid unintended side effects, such as stale data or excessive optimization if the calculation to update is relatively trivial.
By focusing on these performance optimization techniques, developers can not only improve the end-user experience but also pave the way to maintainable and scalable applications.
Testing React Applications
Testing is not just a box to check; it's an essential piece in the puzzle of software development, particularly when building applications using React. In a web environment that's constantly evolving, ensuring that your application behaves as expected is paramount. As React applications become more complex, the need for robust testing strategies also grows. Implementing effective testing practices can provide numerous benefits, including reducing bugs, improving code quality, and boosting developer confidence.
When you test your React applications, you’re essentially setting up a safety net that makes it easier to introduce new features or modify existing ones without fear of breaking the entire system. This proactive approach helps you catch errors early in the development process, saving time and costly troubleshooting later on. Whether you're a student learning the ropes or a seasoned IT professional, understanding testing will greatly enhance your ability to create reliable applications.
Prelims to Testing Libraries
In the React ecosystem, several libraries can help you with testing, each bringing its own flavor. Jest, for instance, is a widely-used testing framework that integrates seamlessly with React applications. It is the default testing library that comes with Create React App and provides a rich set of features including a test runner, a powerful assertion library, and support for mocking.
React Testing Library is another key player, designed with a focus on testing components in a way that resembles how users interact with the applications. Its philosophy is that tests should reflect user behavior rather than implementation details, which aids in maintaining a more stable and consistent testing suite as your application evolves.
Here are some key points to consider regarding testing libraries:
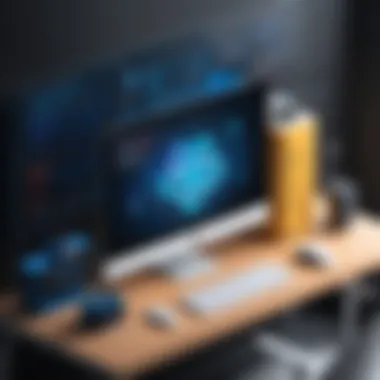
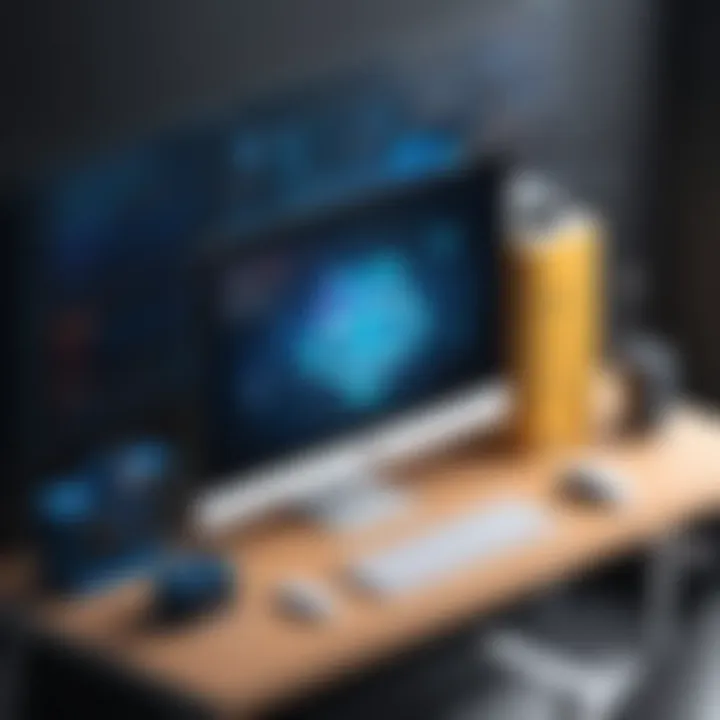
- Ease of setup: Many libraries like Jest come with zero-config setups to get you started quickly.
- Community support: They have strong communities, meaning you'll find plenty of resources, tutorials, and solutions to common problems.
- Rich ecosystem: Most libraries can be used together, allowing for flexible and powerful combination of tools to match the specific needs of your application.
Using the right testing library will bolster your developing process, ensuring fewer issues in the long run and maintaining a high standard for quality.
Creating Unit Tests with Jest
Unit tests are essential for protecting individual units of code—be it functions, components, or methods. By ensuring each unit works as intended, you create a foundation for more complex interactions within your application. Jest makes creating these tests straightforward.
To get started with Jest, first ensure you have it installed and configured in your React project. The provided commands during the setup with Create React App usually take care of this part for you. Here’s a basic example of how you can write a simple test for a React component:
In this snippet:
- You import the necessary functions from React Testing Library.
- The method is called to create an instance of your component.
- You then check whether a specific text is present in the document using the method.
- Finally, the function checks if the text actually exists in the rendered component.
This simplicity is one of Jest's strengths and allows you to focus on writing tests efficiently without getting bogged down in boilerplate code.
Deploying Your React Application
When you’ve toiled away on your React web application, it feels a bit like biting your nails in anticipation. That moment when you deploy is not just a cherry on top; it’s what turns your labor into something usable and, more importantly, accessible to the world. This section underscores the significance of effectively deploying your application, along with providing clear steps and choices that lie ahead.
Deploying your React application embodies the culmination of your development journey. Often, developers overlook this step, thinking that simply building the project is enough. However, proper deployment can significantly affect performance, user accessibility, and even SEO rankings. Taking the time to understand the deployment process can help mitigate common pitfalls that might leave your application unusable in the wild.
Preparing for Deployment
Before you hit the big red button for deployment, there’s groundwork to consider. Here are key points to ponder before going live:
- Build the Project: Create an optimized production build of your React app using the following command:This command generates a directory containing optimized files ready for performance.
- Environment Variables: Make sure your environment variables are properly set. If you have different APIs or settings for development and production, adjust accordingly.
- Static Assets: Confirm that all static assets, like images and CSS, are referenced correctly within your code. Incorrect paths can lead to broken components post-deployment.
- Testing: Run final tests on your application. This includes verifying functionality and checking console errors or warnings. You don’t want to throw your project out there only to find it has a few bugs.
Preparing thoroughly can save you from headaches after deploying, ensuring everything operates smoothly from day one.
Choosing Deployment Platforms
Now that you’ve prepped your application, the next big step is picking a platform for deployment. Some options stand out, each with its own sets of pros and cons:
- Netlify: Known for its ease of use, Netlify takes the cake for deploying front-end applications. Continuous Deployment (CD) is a breeze, giving you instant updates with every code push.
- Vercel: This platform shines when dealing with serverless functions. Perfect for JAMstack applications and efficient deployments that handle scaling matters well.
- Heroku: Primarily known for backend applications, but still a solid choice if your React app requires additional server-side support. Its scaling flexibility can be quite beneficial.
- GitHub Pages: A no-fuss option for those who just need a simple static page. If your project is purely client-side, this could be an ideal choice without any server considerations.
When choosing a platform, keep an eye on factors such as project size, scaling needs, costs, and the ease of integration with your current development workflow.
"Choosing the right deployment platform could make or break your application’s performance. Do your homework!"
In summary, deploying a React application is an intricate process that goes well beyond pressing the deploy button. Proper preparation and selection of a hosting platform can set the stage for success. With this knowledge under your belt, you’re set to launch your application into the digital realm, where users can appreciate your hard work!
Common Challenges and Troubleshooting
Building applications using React can be a thrilling endeavor, but it's not without its share of hurdles. Identifying these common challenges helps developers navigate the often choppy waters of this JavaScript library, ensuring smoother sailing in their coding journey. This section sheds light on vital aspects of troubleshooting in React—lighting a path through complexities that can arise during development. Understanding the intricacies of these challenges not only equips developers with the tools to tackle issues but also sets a foundation that leads to cleaner, more efficient code.
"The right tool for every job makes the work lighter."
Troubleshooting indeed serves as one of those essential tools. When developers encounter issues, it's crucial to not only fix them but to comprehend the root cause. This knowledge can lead to major improvements not just in that specific instance but in future work as well.
Identifying Common Errors in React
Errors in React can crop up unpredictably, often turning what should be a straightforward task into a frustrating puzzle. Some common errors include issues with missing dependencies, incorrect props, or failure to bind methods correctly. These problems can lead to application crashes or unexpected behaviors, making it vital to identify them swiftly.
To shed light on these common pitfalls:
- Missing Dependencies: This is often encountered when using hooks like . If you neglect to include dependencies, it could lead to unexpected behavior in your component. The console may not always give clear feedback regarding these issues.
- Invalid Props: Providing the wrong data type for props can also lead to errors. For example, if you pass an object where a string is expected, your component won't function as intended.
- State Management Fails: Mismanaging state can throw a spanner in the works. Subtle mistakes in deeply nested state updates, particularly when using class components, can lead to bugs that are tough to trace.
By keeping a close watch on these issues, developers can enhance the overall stability of their applications.
Best Practices for Debugging
Debugging is, without doubt, a necessary component of the development cycle. A combination of tools and techniques can ease the debugging process, leading to quicker resolutions and higher efficiency. Here are several best practices for debugging in React:
- Utilize Browser Developer Tools: Familiarizing oneself with the Chrome Developer Tools or Firefox Developer Tools can really provide insights into how your application behaves. The React DevTools extension can be a game changer, enabling you to inspect the hierarchy of components, check their state and props, and understand the component lifecycle.
- Break Down the Components: If you're facing a bug, consider isolating the component that's causing issues. Simplifying your components allows you to test smaller pieces of code, making it easier to spot errors without the noise of surrounding logic.
- Error Boundaries: Introduced in React 16, error boundaries enable you to catch JavaScript errors anywhere in your child component tree. Implementing them can provide a fallback UI that prevents your whole app from crashing.
- Logging: Sometimes, a good ol’ print statement is the way to go. Utilizing console.log strategically in your code can help track down where things are going awry.
By adhering to these practices, developers not only fix existing problems but also lay a groundwork for more vigilant coding moving forward. React applications, like any other complex software projects, require ongoing attention to detail. With dedication to understanding common challenges and employing effective debugging techniques, developers can significantly elevate the quality of their web applications.
Advanced React Concepts
As you venture into the realm of React, diving deep into advanced concepts is paramount for creating sophisticated applications. The knowledge of these concepts helps in building scalable, maintainable, and performative web applications. Let’s explore two critical aspects of advanced React development: the Context API and custom hooks for reusability. Understanding these elements not only enhances your skills but also equips you to tackle real-world challenges effectively.
Understanding Context API
The Context API is a powerful feature that offers a way to share values (like user authentication, themes, or language preferences) between components without needing to pass props down manually at every level. This can become particularly useful in larger applications where prop drilling can lead to cumbersome, inefficient code.
With Context API, you create a context object that holds the state. This context can then be accessed by any component that subscribes to it. Below is a basic example of how to set up and utilize the Context API:
In this example, the wraps around your application or a part of it, effectively making the and accessible to any child components that consume the context. The result is cleaner and more manageable code. It indeed streamlines the process of component data sharing, ensuring you can maintain focus on the functionality and design rather than on data passing inefficiencies.
Context API is not a replacement for state management libraries like Redux; instead, it serves as an excellent alternative for managing global state in simpler apps, striking a balance between ease of use and functionality.
Adopting the Context API not only reduces complexity but also fortifies the reusability of your components. Balancing its application is important, though. Using it indiscriminately can result in performance issues if not handled with care, especially in multi-level tree structures where updates re-render all consumers of the context.
Custom Hooks for Reusability
Custom hooks allow developers to extract component logic into reusable functions. The beauty of React’s hook system lies in its ability to facilitate this reusability without compromising the underlying functionality of the component itself. This leads to a cleaner codebase and better organization of logic shared across components. It breaks complexity into bite-sized pieces that can be easily managed.
Creating a custom hook is a straightforward process. Here’s a simple example that demonstrates how to create a custom hook for managing form inputs:
You can now utilize this custom hook in any of your components, promoting consistency and reducing redundancy:
With custom hooks, you can encapsulate behavior and logic specific to your needs, enhancing the adaptability of your components. It also lends itself well to testing, as you can test the hook independently from the component. Moreover, it aids in maintaining a clean separation of concerns, making each piece of code more functionally oriented rather than tightly coupled with component logic.
Finale and Future Trends
In wrapping up our exploration of React, it’s essential to acknowledge the critical role that the conclusion and the anticipated trajectory of React development play in both the present and future landscape of web applications. The significance lies not only in summarizing what’s been discussed but in offering a forward-looking perspective that can influence your current projects and future endeavors.
As developers venture forth, armed with insights gathered throughout this guide, the takeaway is much deeper than just technical knowledge. Embracing an adaptive mindset, especially in a rapidly evolving field, can set one apart. This realization of ongoing learning resonates heavily within the React community, where updates and improvements are frequent.
"Staying ahead in React means not just knowing the library, but understanding how its evolution shapes web standards and practices."
Recap of Key Takeaways
To ensure that the core concepts resonate, here’s a brief recap:
- Understanding React Components: Familiarity with functional and class-based components is crucial for building scalable applications.
- State Management Skills: The use of hooks like and has become standard practice for simple to complex state management solutions.
- Importance of Routing: Leveraging React Router effectively enhances user experience through dynamic route handling.
- Performance Matters: Mastering performance optimization techniques, including memoization and lazy loading, can significantly improve application efficiency.
In essence, building proficiency in these areas will empower developers to tackle real-world challenges, creating robust applications that deliver exceptional user experiences.
Emerging Trends in React Development
Looking ahead, several trends in the React ecosystem are noteworthy and deserve attention:
- Concurrent Mode: This feature allows React to prepare multiple versions of the UI at once. As developers adopt this, they experience smoother visuals and more responsive applications, particularly during heavy data loads.
- Server Components: This upcoming paradigm enables developers to render components on the server and only deliver the necessary content to the client, reducing the size of JavaScript bundles and improving load times.
- React Suspense: The ability to show loading states until components are fetched or ready promotes better UX practices, especially in the era of speed and interactivity. By using Suspense, developers can prepare users for dynamic content loads without interruptions.
- Improved Tooling: As the ecosystem matures, new tools and libraries continue to emerge for enhancing development productivity. From state management libraries like Recoil to enhanced testing frameworks, developers have an arsenal of options to streamline their workflow.
- Integration with TypeScript: More projects are being built with TypeScript, improving code quality and developer experience. The type safety offered can lead to fewer runtime errors, making it increasingly popular among seasoned developers.
In summary, staying abreast of these emerging trends will be critical for developers aiming to harness the full power of React. By continually adapting to new methodologies and tools, one can ensure that their skills and applications remain relevant in an evolving digital landscape.