Building Robust Web Applications with Java: A Guide
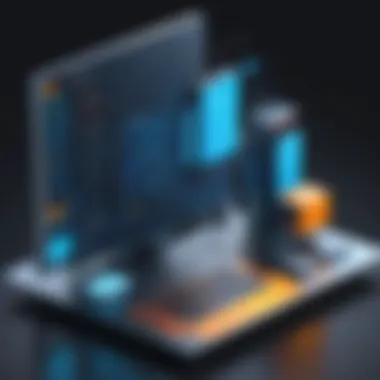
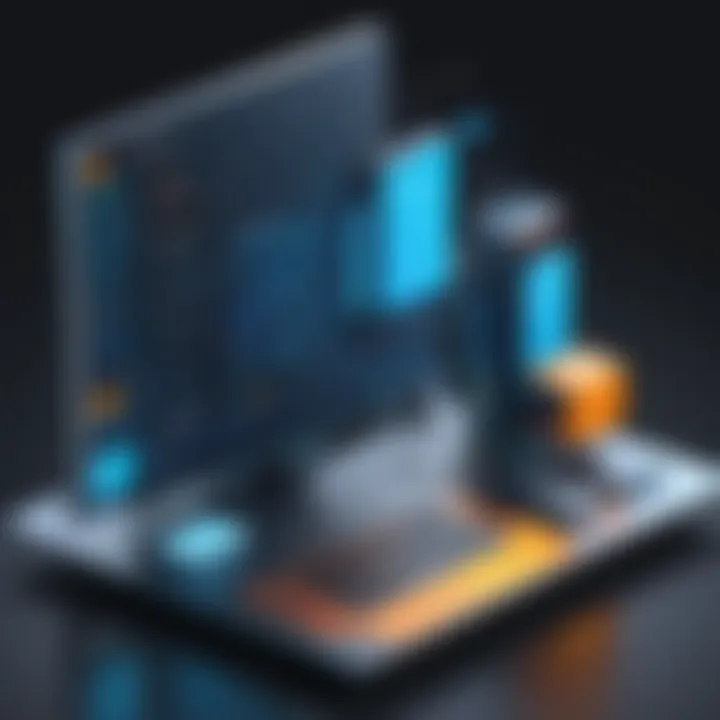
Overview of Topic
In the world of software development, the creation of web applications has emerged as a critical area of focus. Java, known for its portability, robustness, and strong community support, plays a central role in this realm. This overview will shed light on the essential components of Java web application development and its relevance in the tech industry today.
Intro to the main concept covered
At its core, creating a web application in Java entails combining programming skills with concepts of web technologies. It encompasses everything from the back-end processes that handle business logic to the front-end interfaces that users interact with. Understanding these areas forms the foundation for any aspiring developer.
Scope and significance in the tech industry
In the modern landscape, Java is ubiquitous. It powers everything from enterprise-level applications to mobile applications. Its significance lies not only in being a versatile language but also in its ability to integrate seamlessly with various frameworks, enhancing productivity and project scalability. For instance, Javaâs Spring framework, known for simplifying Java enterprise applications, is widely used among developers aiming to create secure and maintainable web apps.
Brief history and evolution
Java was first released by Sun Microsystems in 1995 and has since evolved remarkably. The introduction of Java EE (now Jakarta EE) paved the way for robust enterprise solutions. Over the years, Java has adapted to shifts in technology: from servlets and JSP in the early days to modern architectures like microservices today. Each transition marked a response to the increasing demand for more efficient and reliable web applications.
Fundamentals Explained
Building a solid web application in Java starts with grasping some core principles and theories that underpin the development process.
Core principles and theories related to the topic
A primary tenet is the separation of concerns, which emphasizes dividing the application into distinct sections, each handling specific responsibilities. This approach enhances maintainability and allows teams to work more efficiently. Moreover, understanding Object-Oriented Programming (OOP) conceptsâlike inheritance, encapsulation, and polymorphismâis key, as they streamline code organization and promote reusability.
Key terminology and definitions
- Servlet: A Java program that runs on a server and handles requests and responses.
- JSP (JavaServer Pages): A technology for creating web content dynamically, allowing for easy integration of Java code.
- Framework: A pre-built collection of code and resources that provide a foundation for building applications. Examples include Spring and Hibernate.
Basic concepts and foundational knowledge
Before diving into coding, knowledge of HTTP, APIs, and how web servers function is essential. Java-centric frameworks often abstract many of these details but understanding them will enhance problem-solving skills when issues arise.
Practical Applications and Examples
To solidify understanding, itâs crucial to explore how concepts translate into real-world applications.
Real-world case studies and applications
An example could be an e-commerce platform built using Spring Boot. This project would illustrate how Java handles backend processing for product catalog management, user authentication, and order processing through RESTful APIs.
Demonstrations and hands-on projects
One can start with a simple project like creating a CRUD (Create, Read, Update, Delete) application. This allows for practice in managing database interactions driven by user inputs through a web interface.
Code snippets and implementation guidelines
This simple servlet responds with "Hello, World!"âa first step into servlet programming.
Advanced Topics and Latest Trends
As the world of web application development matures, new trends and technologies emerge.
Cutting-edge developments in the field
Microservices architecture is one notable trend. It enables developers to build applications as a suite of small services, each running in its own process, which can be deployed independently. This contrasts with traditional monolithic applications and offers greater flexibility.
Advanced techniques and methodologies
Incorporating DevOps practices ensures smoother deployment and integration processes. Automation tools like Docker and Jenkins allow for continuous integration and continuous deployment (CI/CD), optimizing workflows and reducing time to market.
Future prospects and upcoming trends
Looking ahead, the rise of cloud-native applications suggests that Java will further intertwine with cloud technologies. This will necessitate developers to become familiar with cloud platforms such as AWS or Azure.
Tips and Resources for Further Learning
Continuous learning is paramount in the fast-paced world of technology.
Recommended books, courses, and online resources
- "Effective Java" by Joshua Bloch is a must-read for mastering Java best practices.
- Coursera offers courses specifically tailored to Java web development and the Spring framework.
- Online platforms like Stack Overflow or Redditâs programming communities can be invaluable when facing specific coding challenges.
Tools and software for practical usage
Utilizing development environments like IntelliJ IDEA or Eclipse can enhance productivity while coding. Additionally, Git for version control remains a vital tool for managing changes in your codebase.
"Learning to code is not an end, but a journey; embrace the challenges, and the growth will follow."
The journey into Java web application development is a rewarding one, filled with opportunities to create impactful and robust applications in todayâs digital landscape. Engaging with this vast ecosystem requires dedication and curiosity, but the skills acquired will undoubtedly pay dividends in oneâs career.
Understanding Web Applications
In the digital landscape of today, web applications have become lifelines for businesses, educational institutions, and individual users alike. They serve as platforms for interaction, transaction, and information dissemination. The significance of understanding web applications cannot be understated, especially for those diving into web development using Java.
Web applications are software systems that are accessed via web browsers, enabling users to perform a variety of tasks online. They range from simple platforms like a personal blog to complex systems like a full-fledged e-commerce site. Grasping what constitutes a web application is vital as it lays groundwork for understanding how to develop, deploy, and maintain them effectively.
Definition and Importance of Web Applications
At its core, a web application can be defined as a client-server software application that users interact with primarily through a web browser. Unlike traditional desktop applications, which run locally on a userâs computer, web apps operate on remote servers and are accessible from any device with internet connectivity. This model not only offers convenience but also flexibility, as users donât need to install software directly onto their devices. With real-time updates and cloud storage, users can engage seamlessly with the application from anywhere.
The importance of web applications is multifold:
- Accessibility: Users can access applications anytime and anywhere, breaking geographical barriers.
- Upkeep and Maintenance: Centralizing the application on a server means updates and fixes can be distributed immediately, eliminating the need for users to perform updates themselves.
- Cross-Platform Compatibility: A well-designed web application can run on any operating system that supports a browser, streamlining development and reaching wider audiences.
"Web applications play a crucial role in shaping user experience in the digital age, acting as a bridge between users and the information they seek."
Key Components of Web Applications
When delving into the intricacies of web applications, there are several key components to consider that are fundamental to understanding their structure and functionality:
- Frontend: This is the visual part of the web application. It's what users interact with and includes everything from buttons to text boxes and images. Technologies such as HTML, CSS, and JavaScript play a crucial role here.
- Backend: The behind-the-scenes operations that make a web app function are handled by the backend. This includes server setups, databases, and application logic, often programmed in languages such as Java, Python, or PHP.
- Database: This is where all the data of the web application is stored. Users' information, application configurations, and content all reside in a database, making it essential for a web appâs functionality.
- Server: The server hosts the web application, managing requests from users and serving the correct responses.
- APIs (Application Programming Interfaces): APIs allow various software components to communicate with each other, enabling integration of different services and allowing the web app to perform complex operations with ease.
In summary, understanding these components not only assists in getting a firm grip on how web applications function but also guides developers in designing more effective, scalable, and user-friendly applications. Grasping these concepts is crucial for anyone venturing into Java web development.
Why Choose Java for Web Development
When it comes to web development, selecting the right programming language can make or break the project. Java has established itself as a reliable tool in the toolkit of countless developers. Its rich ecosystem, robust frameworks, and cross-platform capabilities contribute to its prominence in building web applications. This section will delve into why Java stands out among other languages, touching on its stability and performance, the array of frameworks available, and its ability to operate seamlessly across diverse platforms.
Stability and Performance
Java has built a reputation for stability, which is a key selling point for many businesses. The language is designed to handle significant load with minimal hiccups, making it an ideal choice for enterprise-level applications that demand reliability. Developers often refer to "garbage collection"âJava's method of automatic memory managementâwhich helps in optimizing memory usage. This not only boosts performance but also reduces the amount of manual coding required to prevent memory leaks, enabling developers to focus on higher-level applications and features.
"Stability in development translates to minimized downtime and improved user satisfaction."
With multithreading capabilities, Java allows multiple operations to run simultaneously, making applications responsive and efficient. For businesses, this translates to better user experience and can lead to increased customer satisfaction, leading to long-term retention. Moreover, with advancements like the Just-In-Time (JIT) compiler, Java applications can run faster and more efficiently than ever.
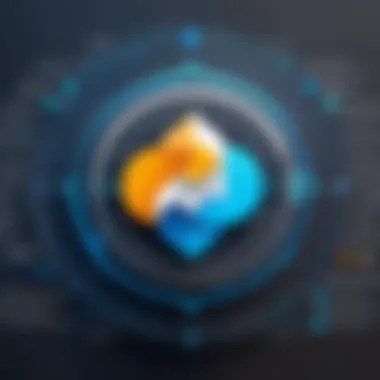
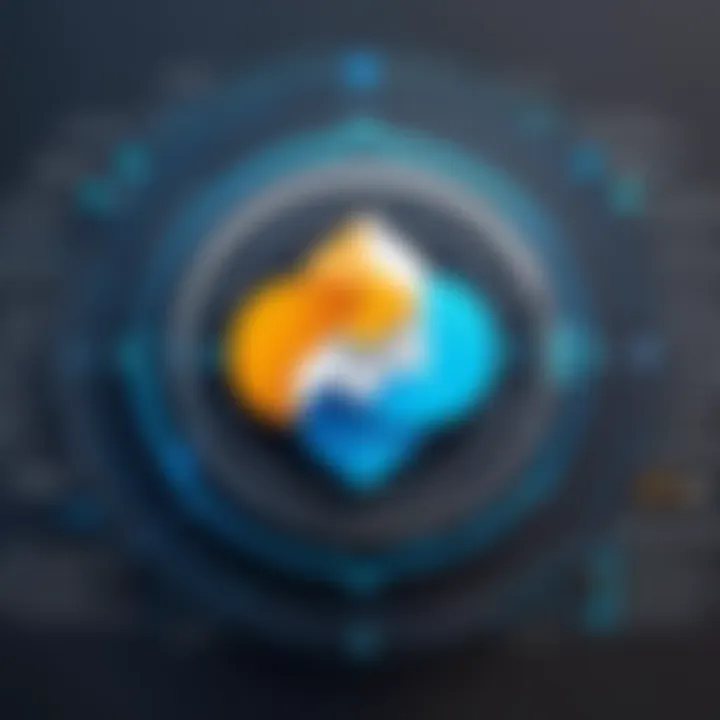
Wide Range of Frameworks
Java's versatility is further underscored by the wide array of frameworks available, each tailored for specific use cases. Among them, some of the most prominent include Spring, Hibernate, and JavaServer Faces (JSF). These frameworks not only streamline development processes but also come packed with pre-built functionalities that allow developers to build complex applications without re-inventing the wheel.
- Spring Framework: Known for its dependency injection feature, Spring simplifies the complexities of enterprise-level apps. With extensions for building web apps like Spring MVC and Spring Boot, developers can create RESTful services and microservices with less effort.
- Hibernate: A framework designed for object-relational mapping, it abstracts the complexities of database interactions. Using Hibernate, developers can manipulate data with far less boilerplate code, allowing for a cleaner and more maintainable codebase.
- JSF: This is particularly useful for building component-based user interfaces. It promotes the reuse of UI components, making the development of user interfaces quicker and more efficient.
Choosing Java means having a flexible toolbox that can manage various project demands.
Cross-Platform Capabilities
One of Java's most appealing characteristics is its promise of "Write Once, Run Anywhere" (WORA). This highlights the language's ability to compile code into bytecode, which can then be run on any operating system equipped with a Java Virtual Machine (JVM).
This cross-platform capability is crucial in today's tech landscape where applications need to function on diverse devicesâfrom desktops to mobile. It allows developers to reach a broader audience without scaling separate codebases for different platforms.
Furthermore, frameworks like Spring can also be deployed on various server environments, enhancing the adaptability of Java applications to specific business needs. This flexibility encourages innovation and helps developers respond to unforeseen requirements with agility.
In summary, Java offers a unique combination of stability, a wide range of frameworks, and cross-platform capabilities. By choosing Java, developers are equipping themselves with a powerful arsenal to tackle modern web development challenges, be it for startups or well-established enterprises. The higher-level functionalities provided by Java frameworks create an environment conducive to building robust web applications while ensuring smooth performance.
Setting Up Your Development Environment
Establishing a solid development environment is like laying the foundation of a house; itâs crucial for the stability and functionality of your Java web application. A well-configured environment not only enhances productivity but also minimizes friction when coding and debugging. Each choice you make plays a critical role, from selecting the right Integrated Development Environment (IDE) to installing necessary tools like the Java Development Kit (JDK). Without this, even the best code will struggle to shine.
Choosing an Integrated Development Environment (IDE)
When jumping into Java web development, choosing an IDE feels like picking a trusty sidekick. You want something that offers you a straightforward interface but flexes just enough muscle to tackle challenging tasks without breaking a sweat. Popular options include Eclipse, IntelliJ IDEA, and NetBeans, each comes with its pros and cons.
- Eclipse is renowned for its versatility and extensive plugin ecosystem. It's a great choice if you're keen on tailoring your environment to specific needs.
- IntelliJ IDEA, on the other hand, offers smart code assistance and is often praised for its intuitive interface. Many developers find that it increases their productivity right off the bat.
- NetBeans is known for its ease of use and integrations, making it a solid option for those who may prefer a smoother learning curve.
In summary, your IDE choice boils down to personal preference and specific project needs. Evaluate them against your workflow to ensure you find the right fit for you.
Installing Java Development Kit (JDK)
The JDK is the heartbeat of your Java applications. It provides essential tools to compile, debug, and run your programs. Installing JDK might be a bit tedious for some, but it's a task thatâll pay off in spades. When picking a JDK version, go with the latest long-term support (LTS) version from Oracle or OpenJDK.
An important step during installation is to set the JAVA_HOME environment variable, which can be a headache if overlooked. This variable tells your system where to find the JDK, allowing various tools to work without you having to lift a finger. Take time to ensure everythingâs working as it should using the command line. Just type and and see if they return the expected results.
Configuring Build Tools
Configuring build tools is essential, serving as the backbone for managing dependencies and automating tasks such as compiling code and creating packages. In the Java ecosystem, you have two heavyweight contenders: Maven and Gradle. Each tool comes with its quirks and strengths.
Maven
Maven can feel like the straight-laced cousin in the family, known for its rigid structure and convention over configuration philosophy. It's fundamentally designed for standardized project setups. One key characteristic of Maven is its central repository, which allows developers to easily add and manage dependencies. This feature means you'll spend less time hunting down libraries and more time writing code.
Mavenâs pom.xml file is where the magic happens; it defines project details, dependencies, build configurations, and more. Itâs a good choice for larger projects that benefit from a uniform project structure. That being said, it poate become cumbersome when your project requires a custom setup, leading to verbose configuration files.
Gradle
Gradle steps into the limelight as more versatile and flexible than Maven. It uses a groovy-based DSL, allowing you to script your build processes, giving you creative freedom in customizing your build to match your needs. One of its pronounced characteristics is its performance; Gradle provides incremental builds, meaning it only rebuilds parts of your project that have changed. This saves time, especially on larger projects.
Another unique aspect is its ability to handle both Java and non-Java projects seamlessly, making it a favorable choice for diverse tech stacks. Gradleâs configuration files can be clearer and more intuitive, but for newcomers, the learning curve might feel steep at first.
Overall, the choice between Maven and Gradle hinges on your project requirements and your personal preference for flexibility versus structure. No matter which you choose, ensuring proper configuration can lead to a smoother development process.
Exploring Java Web Frameworks
Java web frameworks play a crucial role in simplifying the development process, offering tools and structures that streamline tasks for developers. As the landscape of web applications continues to evolve, the choice of a framework can significantly impact both productivity and end results. Understanding different frameworks not only helps in selecting the right tools but also in adopting best practices that ensure the efficiency and maintainability of web applications.
Frameworks provide essential components that cater to various aspects of application development, such as routing, templating, and data handling. Moreover, they encapsulate company standards and industry trends, allowing developers to focus more on building features rather than wrestling with low-level coding issues. This balance of higher-level programming with the flexibility to manage intricate details makes frameworks indispensable in todayâs coding ecosystem.
Spring Framework Overview
Spring Framework, a robust and versatile toolkit known widely in the Java community, offers solutions for both web and enterprise-level applications. It is designed to simplify Java development by providing a comprehensive programming and configuration model.
Spring Boot
Spring Boot represents a significant evolution in the way applications are built with Spring. The main aspect to appreciate about Spring Boot is its ability to get projects up and running quickly, thanks to its convention-over-configuration approach. This characteristic means developers do not have to write boilerplate code or configurations, often leading to faster development cycles.
Key Feature:
One standout feature of Spring Boot is its embedded servers, such as Tomcat or Jetty. This allows developers to package the application as a self-contained unit, which can simplify deployment.
Advantages:
- Rapid application development with minimal configuration.
- Seamless integration with the Spring ecosystem, leading to easily reusable modules.
- A vibrant community around Spring Boot provides abundant resources and plugins.
Disadvantages:
- Newcomers might find the abstraction introduces a learning curve, as understanding the Spring ecosystem principles is still important.
Spring
Spring MVC is another vital component of the Spring Framework, dedicated specifically to web applications. It helps in building web applications with sophisticated routing and powerful handling of HTTP requests.
Key Feature:
Spring MVC uses a Model-View-Controller architecture, which separates the concerns of application data, presentation, and business logic. This separation can lead to more maintainable code and easier future enhancements.
Advantages:
- Flexible and highly configurable, allowing for tailoring solutions to specific needs.
- Strong support for RESTful API development, fostering modern web applications.
- Built-in support for data binding and validation, streamlining the development process.
Disadvantages:
- The complexity of configuration can be daunting for learners unfamiliar with the framework's methodologies.
JavaServer Faces (JSF)
JavaServer Faces is a server-side component-based framework designed to facilitate the development of user interfaces for Java web applications. JSF simplifies UI development by employing reusable UI components, making it efficient for large-scale applications. Many organizations appreciate its integration with other Java standards, providing a consistent and cohesive approach to building web applications. However, JSF can be seen as less flexible than newer frameworks, potentially leading to over-engineering in simpler projects.
Play Framework
The Play Framework stands out as a reactive web framework that emphasizes simplicity and developer productivity. Its unique feature lies in its ability to serve dynamic content with an asynchronous model, which can lead to more responsive applications. This framework supports both Java and Scala, appealing to a wider audience of developers. One notable advantage is its built-in hot reload capability, enabling changes to be seen immediately, thus improving the development experience. However, this framework may not have as extensive a community or plugin ecosystem as more established frameworks like Spring.
Developing Your First Java Web Application
The journey of crafting your inaugural Java web application holds significant weight in both your learning and development trajectory. This section is crucial as it lays the groundwork for understanding how to bring application concepts to fruition using Java. Knowing the steps involved in development not only enhances your technical skills but also boosts your confidence in tackling real-world projects. Each element in this section plays a pivotal role, offsetting gaps in theoretical knowledge by providing practical application.
Project Setup and Structure
When starting a new Java web application, establishing a well-organized directory structure is vital. A clean and consistent project structure helps in maintaining clarity as the project grows in size. Typically, a Java web application follows a convention of separating various components that might include resources like configuration files or libraries, Java classes, and web assets like HTML, CSS, and JavaScript files.
Here's a basic structure you might consider:
Having this structure helps you to quickly find and manage files as you develop further. Itâs like having a well-organized garage; when everything is in its place, you can see the tools at your disposal without chaos ensued.
Configuring Dependencies
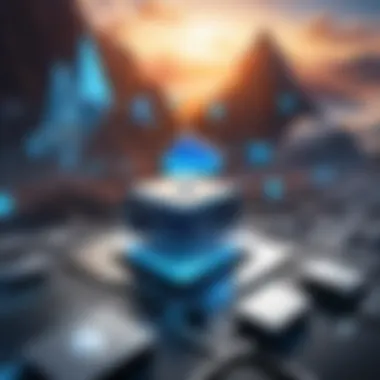
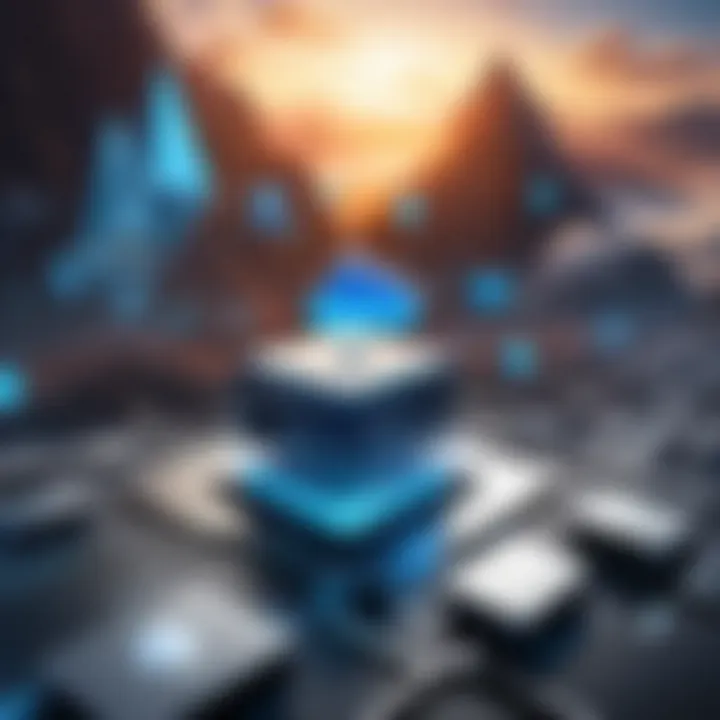
Dependency management forms the backbone of a streamlined Java development process. Utilizing tools such as Maven or Gradle can help you eliminate manual management of libraries and ensure that everything is properly referenced without the risk of conflict.
In Maven, for instance, you'll find the file essential for defining your projectâs dependencies. Hereâs a simple example:
The libraries specified here allow your application to leverage the functionalities of Spring Boot and Hibernate effectively. This way, you are not constantly searching for the latest library version; instead, they are handled automatically. It's a time-saver and sure beats having to dig around for the right version of a library whenever you set up a new project.
Building RESTful Services
In todayâs tech landscape, crafting RESTful services has become a standard practice for web applications. By building these services, you enable your application to communicate efficiently over the web. RESTful services rely on a stateless protocol, making them scalable and performant.
To route and manage requests, you can use annotations provided by Spring Boot. Below is a quick example to illustrate the creation of a simple REST controller:
This controller listens for GET requests directed at the endpoint and responds with a simple greeting. Building such RESTful services not only enhances interactivity but also fosters a client-server architecture where different components can communicate smoothly. Remember, concrete implementation and extensive testing of these services will yield robust applications ready to handle real-world demands.
"The ability to build RESTful services is a key skill in modern web development, allowing applications to be versatile and responsive to user requests."
Engaging in creating your first web application is a gateway to understanding the practicalities and challenges of web development. By methodically approaching project setup, managing dependencies, and developing RESTful services, you position yourself for long-term success in the Java ecosystem. As you grow more comfortable with these fundamentals, your journey into more complex applications will feel less daunting.
Frontend Integration with Java
In todayâs web landscape, marrying the frontend and backend is akin to crafting a beautiful symphony. Itâs crucial, especially when building web applications in Java, to ensure that the two halves dance harmoniously together. The integration of frontend technologies not only enriches user experience but also enhances functionality.
When developing web applications, one has to consider how the user interacts with the application. A seamless frontend paired with a robust Java backend can make the difference between an application that feels clunky and one that glides effortlessly. Using popular frameworks like Angular or React helps in building responsive user interfaces that can significantly elevate the engagement level. Furthermore, the dynamism they offer stands in stark contrast to older, more static designs.
Using Frameworks like Angular and React
Both Angular and React have emerged as frontrunners in creating sophisticated user interfaces. Angular, backed by Google, is a full-fledged framework that provides a comprehensive solution to building client-side apps. Its two-way data binding feature ensures that changes to the model and view are automatically synchronized. This capability allows developers to create a more interactive experience without excessive boilerplate code. On the other hand, React, spearheaded by Facebook, champions a component-based architecture. This modular approach facilitates reusability of components, leading to cleaner, more manageable codebases.
The choice between Angular and React often boils down to personal preference and project requirements. While Angular may come with a steeper learning curve due to its extensive tooling, it offers a richer set of features built-in. React, with its lightweight library nature, makes it flexible, though it may necessitate additional libraries for state management and routing. Mapping out your applicationâs needs can help you zero in on the framework that best suits your project.
Templating Engines
Templating engines like Thymeleaf and JSP play an essential role when it comes to dynamically generating HTML content. They serve as translators, converting server-side data into the interactive elements of a web page. This process creates a bridge between the data handled in Java on the backend and the user interface rendered on the frontend.
Thymeleaf
Thymeleaf stands out for its integration style. It nurtures a natural templating philosophy, allowing templates to be valid HTML5 documents. This means designers can create static prototypes that can directly evolve into dynamic web pages. Its key characteristic lies in its capability to process data from the Java backend seamlessly. As a result, developers appreciate Thymeleaf for being user-friendly while complementing Spring framework applications exceptionally well.
Some unique features include its ability to function in both web and non-web environments. The advantage here is flexibility; it lets you render templates offline or as part of a REST response. However, developers sometimes find Thymeleafâs syntax a tad bit verbose compared to other options, which may slow down the rapid prototyping of interfaces.
JSP
JavaServer Pages (JSP) has been a staple for Java web development, offering a straightforward syntax to embed Java code within HTML pages. Itâs frequently lauded for its capacity to quickly create dynamic content. A crucial aspect of JSP is that it runs on the server side, which means the generated HTML is sent to the client after JSP processing.
What sets JSP apart is its ease of integration with Java EE web applications. JSP pages can directly access Java classes, leading to an efficient workflow. However, its reliance on embedded Java code can sometimes clutter pages, making them less readable. Furthermore, modern Java development practices have shifted towards more structured frameworks, positioning JSP as a legacy approach for new applications.
The integration of frontend frameworks and templating engines is not just a technical necessity; it's about crafting rich user experiences that keep your audience coming back for more.
Database Connectivity
Database connectivity is a fundamental aspect of developing web applications in Java. It serves as the backbone that enables an application to interact with its required data storage system, be it a relational database or a NoSQL solution. The ability to efficiently connect, manipulate, and manage data is crucial for any application that aims to serve real users with dynamic content.
One of the most significant benefits of establishing database connectivity is the capacity to store, retrieve, and update data effectively. Without a reliable connection to a database, even the best-designed web applications can fall flat, offering limited functionality and a poor user experience. This section explores vital concepts related to database connectivity in Java.
Effective database connectivity is not just about queries; it's about creating a user experience that feels seamless and reliable.
Using JDBC for Database Operations
Java Database Connectivity (JDBC) is the standard API for connecting Java applications to a database. It plays a critical role in enabling developers to perform database operations such as querying, updating, and transaction management.
The JDBC API provides a set of classes and interfaces that allow for database interactions. Here are a few key points regarding JDBC:
- Direct Control: JDBC allows for low-level control over database queries. Developers can write SQL directly, giving them the flexibility to tailor queries to specific needs.
- Cross-Database Compatibility: JDBC drivers exist for various databases, from MySQL to Oracle, making it easier to switch databases without altering much code.
- Support for Transactions: JDBC supports transaction management, allowing developers to execute complex operations safely, ensuring data integrity in multi-step processes.
A simple example of a database connection using JDBC might look like this:
In this snippet, the application establishes a connection to a MySQL database, queries the "users" table, and prints out names. This straightforward demonstration showcases how even simple Java code can tap into powerful database functionalities.
Object-Relational Mapping with Hibernate
Hibernate is a popular framework that addresses the complexities of database connectivity by implementing Object-Relational Mapping (ORM). This concept allows developers to map Java objects to database tables, which can lead to a more intuitive development experience.
The advantages of using Hibernate include:
- Less Boilerplate Code: ORM reduces the amount of database-related code, letting developers focus on business logic instead.
- Query Abstraction: With Hibernate's HQL (Hibernate Query Language), querying becomes more object-oriented, abstracting away SQL specifics that often clutter code.
- Lazy Loading: Hibernate supports lazy loading, meaning that it only fetches data when it is actually needed, optimizing performance and resource use.
Using Hibernate is relatively straightforward. After setting it up with appropriate configurations, a typical entity class might look like this:
This class represents a database table structure in the form of a Java object. Developers can then use Hibernate to manage the lifecycle of these objects with minimal fuss.
Both JDBC and Hibernate offer powerful methods for database connectivity, each serving different needs and preferences within the Java ecosystem. By understanding both, developers can make informed choices about the tools they use for their specific applications, ensuring efficient and effective data management.
Implementing Security in Java Web Applications
When it comes to web applications, security is not just an optionâ itâs a necessity. In the current digital space, threats loom large, and creating a secure Java web application is a fundamental responsibility for developers. Without proper security measures, applications can become vulnerable to various attacks such as data breaches, unauthorized access, and other harmful intrusions. Addressing security at the onset of development ensures that sensitive user data remains protected and fosters user trust.
Understanding Authentication and Authorization
Letâs break down two key components of web security: authentication and authorization.
Authentication is the process of verifying the identity of a user. When someone logs into an application, they present credentials, typically in the form of a password. Once their identity is confirmed, they gain access to the application. Think of authentication as the gatekeeper, checking IDs to ensure only authorized users get in.
On the other hand, authorization determines what an authenticated user can do. Just because a person is logged in doesnât mean they can access every nook and cranny of an application. For instance, an admin may have total access while a regular user can only view certain pages or features. This role-based access management is crucial for maintaining control over data and functionality.
"Security is not a product, but a process."
- Bruce Schneier
Understanding the distinction between authentication and authorization helps in designing a robust security framework. Developers need to implement strong password policies and access controls to ensure effective security measures.
Using Spring Security
One of the most powerful tools available for implementing security in Java web applications is Spring Security. This framework is an integral part of the Spring ecosystem and provides a comprehensive set of features to secure your application.
Spring Security offers:
- Authentication Support: It supports various authentication methods including form-based login, basic authentication, and even OAuth for better user experience.
- Authorization Control: It allows fine-grained control, enabling you to specify what access each user role has, safeguarding application elements based on user roles.
- Protection Against Common Vulnerabilities: Including CSRF (Cross-Site Request Forgery), session fixation attacks, and other prevalent threats.
Setting up Spring Security might seem daunting, but its modular design means you can implement it step by step. Youâll find robust documentation and community support incredibly helpful while navigating this path.
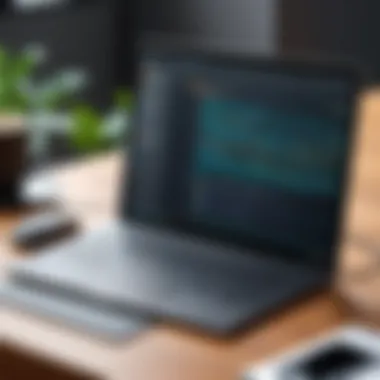
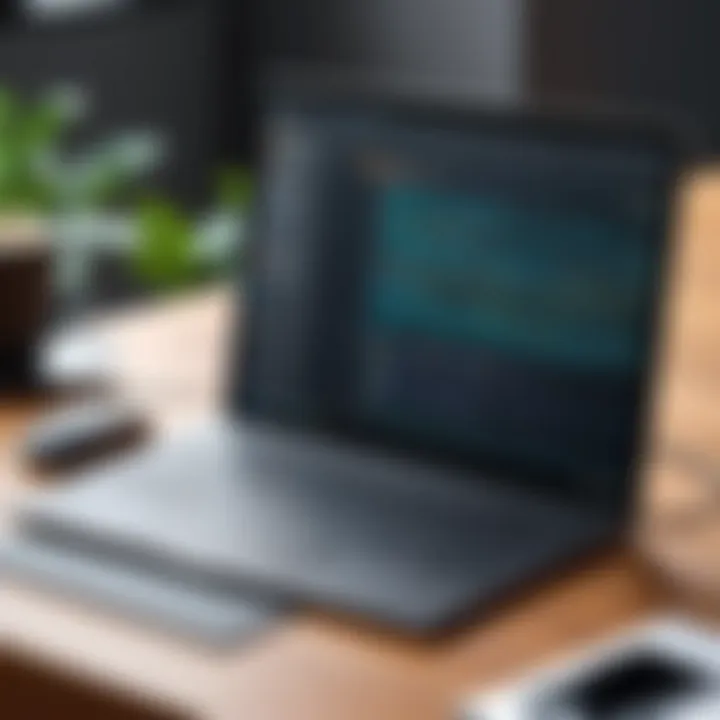
Hereâs a quick starter guide on how you might typically configure Spring Security:
This snippet illustrates basic settings to secure paths, establish a custom login page, and require authentication for all other resources.
In summary, implementing security in Java web applications is critical. Developers must take the time to understand and apply the concepts of authentication and authorization properly. Leveraging frameworks like Spring Security can greatly simplify this task and significantly enhance the security posture of your applications.
Testing Java Web Applications
Testing is a vital step in the development of Java web applications. It is not only about finding bugs but is about ensuring the application works as intended and provides a seamless experience for users. If you don't test your application, you might as well close your doors, because errors can cause significant issues down the line, leading to poor user experience and lost revenue. This section digs into the importance of testing and key strategies that every developer should be aware of.
Unit Testing Strategies
Unit testing plays a critical role in validating individual components of the application. By testing each unit in isolation, developers can validate functionality and make sure every part works on its own. This approach not only enhances reliability but also makes future code refactoring much easier. Two popular frameworks in Java for unit testing are JUnit and Mockito. Let's take a closer look at both.
JUnit
JUnit is widely recognized for its efficiency in performing unit tests in Java applications. One of its main characteristics is its simplicity. Developers appreciate its straightforward approach that allows them to write reliable tests quickly. With JUnit, writing tests becomes less of a chore and more of a natural part of the coding process.
A unique aspect of JUnit is its annotations. Annotations like , , and enable developers to specify the testing methods and their setup/teardown procedures with ease. This organization helps in clearly understanding the flow and purpose of tests.
The benefits of using JUnit are several, including strong community support and extensive documentation. However, it potentially requires a learning curve for developers not familiar with its structure. But once you get the hang of it, it lays a solid foundation for testing Java applications effectively.
Mockito
Then there's Mockito, a framework that takes unit testing a step further, mainly focusing on mock objects. When dealing with code that interacts with external systems or complex behaviors, being able to simulate these interactions is crucial. That's where Mockito shines. Its primary characteristic is its ability to create mocks for Java classes, making it easier to test isolated behaviors without relying on actual implementations.
One of the standout features is its clear and expressive API, allowing developers to define how a mock should behave through simple method calls. This leads to better-organized tests that remain easy to read and maintain.
The advantage of using Mockito lies in its flexibility. You can stub calls and verify interactions effortlessly, which can drastically reduce the time it takes to set up tests. On the downside, relying too heavily on mocks can sometimes lead to tests that don't clearly reflect actual behavior, but this can be mitigated with good testing practices.
Integration Testing
Integration testing extends beyond unit tests to evaluate how various components of the application work in unison. After ensuring that individual parts are on point, itâs crucial to check their interactions. This is where integration tests become indispensable. They help identify issues that unit tests may miss, especially concerning data flow between modules.
In reality, if one piece of the puzzle doesnât align with another, it can spell trouble. Integration testing brings a broader perspective; it validates the combined functionality of integrated components. It is also a vital checkpoint before deployment, ensuring that the application's pieces fit together seamlessly.
In summary, both unit and integration testing are essential practices in Java web application development. Prioritizing a thorough testing strategy can save time and costs in the long run, while also ensuring a polished final product that stands up to end-users' expectations. Remember, investing time in testing is never wasted.
Deploying Your Java Web Application
When it comes to deploying a Java web application, this process is as crucial as the coding itself. Successful deployment ensures that a developed application is available and functional for users. It also involves careful consideration of the environment where your application will run and the specific technologies that will support it. This article will reveal not just the steps for deployment but also the insights and best practices to ensure your application operates seamlessly once itâs out in the wild.
One of the first decisions you'll face during the deployment phase is choosing the best environment for your application. Organizations are increasingly leaning towards cloud solutions for a variety of reasons: scalability, cost-effectiveness, and flexibility. Likewise, deploying on traditional application servers becomes relevant, particularly in enterprise environments where control and compliance are critical.
Cloud Deployment Options
Cloud deployment offers an agile solution to getting applications up and running. It allows developers to focus on writing code without drowning in server maintenance. There are primarily three models to consider:
- Infrastructure as a Service (IaaS): This model provides virtualized computing resources over the internet. Providers like Amazon Web Services (AWS) and Google Cloud Platform (GCP) allow you to root yourself in a scalable environment.
- Platform as a Service (PaaS): Here, everything you need to create applications is provided. You can concentrate on development rather than the underlying software or hardware. Popular PaaS options for Java applications include Heroku and Red Hat OpenShift.
- Software as a Service (SaaS): This model directly focuses on delivering a specific application to end-users through the internet, abstracting all underlying complexities. However, it is less common for deploying bespoke Java applications because of the need for customizations.
Benefits of cloud deployment include:
- Scalability: You can effortlessly expand your applicationâs capacity based on demand.
- Cost Efficiency: You only pay for what you use, making it more economical than traditional hosting.
- Accessibility: Many cloud services allow you to access your applications from anywhere, promoting remote collaboration.
Using Application Servers
Application servers give developers a robust environment to run and manage web applications. They can facilitate various services like load balancing, security, and transaction management. In this article, we will delve into two notable application servers: Apache Tomcat and WildFly.
Apache Tomcat
Apache Tomcat stands tall among the choices for Java web applications. It is often praised for its simplicity and lightweight nature. Developers love it for its ease of setup and straightforward configuration. Since Tomcat implements Java Servlet and JavaServer Pages (JSP) specifications, it seamlessly integrates with Java applications.
The key characteristic of Tomcat is its emphasis on being a servlet container, which means it is optimized for implementing web applications built on Java servlets. Why is this useful? It allows developers to go from 0 to production fast with minimal overhead. This makes it a popular choice, especially for beginners or small projects where time is of the essence.
However, while Tomcat excels in many areas, it does have some limitations, particularly in transaction management and enterprise features compared to more robust application servers.
WildFly
Formerly known as JBoss, WildFly has carved out its niche in the Java ecosystem, focusing on enterprise-level applications. Its key distinguishing feature is its support for the full Java EE (Jakarta EE) stack, providing functionalities that encompass more than just servlets and JSPs.
WildFly comes with built-in clustering, which is advantageous for applications needing high availability. It also supports microservices architecture, making it a fitting choice for modern application development. The development community around WildFly is quite vibrant, offering extensive resources and support.
Despite its many benefits, some developers may find WildFlyâs initial setup a bit more complex compared to Tomcat. This steep learning curve might not be ideal for everyone, particularly if the application's requirements are more modest.
"The choice of deployment strategy can shape the future of your web application. Make it wisely."
Both Tomcat and WildFly each hold their own as powerful deployment platforms, but they cater to different needs. Understanding the specific requirements of your application will guide you in making an informed decision between these options. With a deployment strategy that aligns with your goals, you can ensure your Java web application reaches its full potential.
Maintaining and Scaling Your Web Application
In the world of web applications, launching your product is just the tip of the iceberg. Maintaining and scaling your web application plays a crucial role in ensuring its longevity and relevance in a fast-paced technological landscape. Understanding how to effectively monitor performance and implement scalable solutions is essential for any developer.
Proper maintenance of your web application means youâre not just patching bugs or fixing broken features; itâs about ensuring that the application evolves according to user demand and expectations. The benefits are manifoldâimproved user satisfaction, increased reliability, and ultimately, higher retention rates. As your user base grows, the need for an efficient scaling strategy becomes apparent.
Monitoring Performance
Performance monitoring is the first line of defense in maintaining your application. Without keen insight into how your app is functioning, you're effectively flying blind. You want to watch out for issues that could bog down your application, like slow response times or inadequate resource management.
Here are some practical tools to consider for performance monitoring:
- New Relic: A comprehensive tool that offers real-time performance stats. You can drill down into transaction times and understand bottlenecks.
- Datadog: This integrates seamlessly with various services and gives you a holistic view of your application's health. It provides dashboards that help visualize performance over time.
- Prometheus: Often used with Kubernetes, this open-source tool collects metrics and allows you to set up alerts based on performance thresholds.
Additionally, ensure you're logging critical events to catch warnings and errors early. Analyzing logs provides context to performance metrics, allowing you to pinpoint and rectify issues before they escalate into bigger problems.
"Regular monitoring can save you from catastrophic failures."
Scaling Strategies
Once youâve got a handle on performance, the next step is to think about scaling your application. As user traffic increases, your web app must be able to manage this demand without a hitch. Scaling can be accomplished in basically two ways: vertical scaling and horizontal scaling.
- Vertical Scaling: This involves beefing up your existing server by adding more resources, like CPU and RAM. While easier to implement, it has limitations. You can only scale up to the maximum capacity of your hardware.
- Horizontal Scaling: This method entails adding more servers to accommodate rising trafficâthis means distributing the load. Though it requires a bit of foresight in terms of architecture, it offers superior flexibility and resilience. A common choice in cloud environments is to use Amazon EC2 instances for auto-scaling based on metrics.
You might consider employing load balancers during this phase to effectively distribute incoming traffic across multiple servers. This ensures streamlined performance, preventing any single server from becoming a bottleneck and reducing downtime.
Exploring Additional Resources
In the realm of web development, especially when diving into Java applications, the importance of continual learning can't be overstated. The technology landscape evolves rapidly, and having a finger on the pulse of new tools, techniques, and practices can make a world of difference. So, what does it mean to explore additional resources?
Utilizing resources means stepping beyond the confines of immediate project work. It involves tapping into books, tutorials, online communities, and forums where discussions rage about the latest updates and best practices in Java web development. These resources help developers bond over shared challenges and triumphs, providing not only technical knowledge but also emotional support.
Books and Tutorials
Books and tutorials form the bedrock of a strong developer's toolkit. They offer structured, in-depth explorations of various aspects of Java and web application development. Here's why they are crucial:
- Foundational Knowledge: A well-written book can provide fundamental concepts in clear, digestible terms. For example, "Effective Java" by Joshua Bloch is a seminal text that many Java developers swear by. It covers best practices that improve code quality and efficiency.
- Real-World Examples: Tutorials often illustrate concepts with practical examples. Websites like Codecademy and Coursera provide courses that are not just about theory; they push developers to build projects that simulate real scenarios.
- Updated Content: The tech world is always buzzing with new libraries and techniques. Platforms like Medium or Dev.to offer a treasure trove of articles written by practitioners dealing with the same hurdles and solutions as you.
Reading books enhances critical thinking skills and helps in problem-solving. Itâs about piecing together the puzzle of programming, bit by bit.
Online Communities and Forums
Online communities are the lifeblood of modern programming culture. They provide spaces for sharing knowledge and troubleshooting problems. Some significant benefits include:
- Crowd-Sourced Solutions: Communities like Stack Overflow allow developers to ask questions about anything from basic syntax errors to intricate design patterns. Answers often come from seasoned professionals who have faced similar issues.
- Networking Opportunities: Engaging in forums can lead to professional connections, job opportunities, and collaborations. Getting involved in discussions on Reddit's programming threads or through Facebook groups can foster lasting relationships that might open doors down the line.
- Feedback and Critique: Sharing your code in these communities allows you to receive feedback from others. Constructive critiques can vastly improve your coding skills and application design.
âIn programming, we are constantly learning. Every project uncovers new information.â
In summary, books and tutorials alongside online communities and forums provide invaluable support. They widen your knowledge base, present real-life context, and connect you with others in the field. By actively engaging with these resources, you pave a smoother path towards mastering Java web application development.