C Language Programs: A Comprehensive Guide and Resource
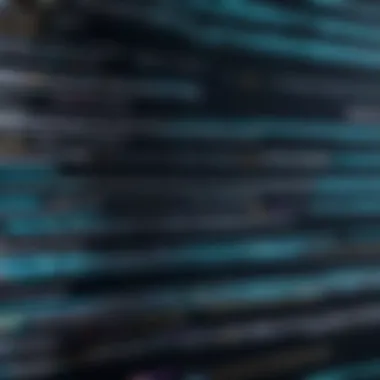
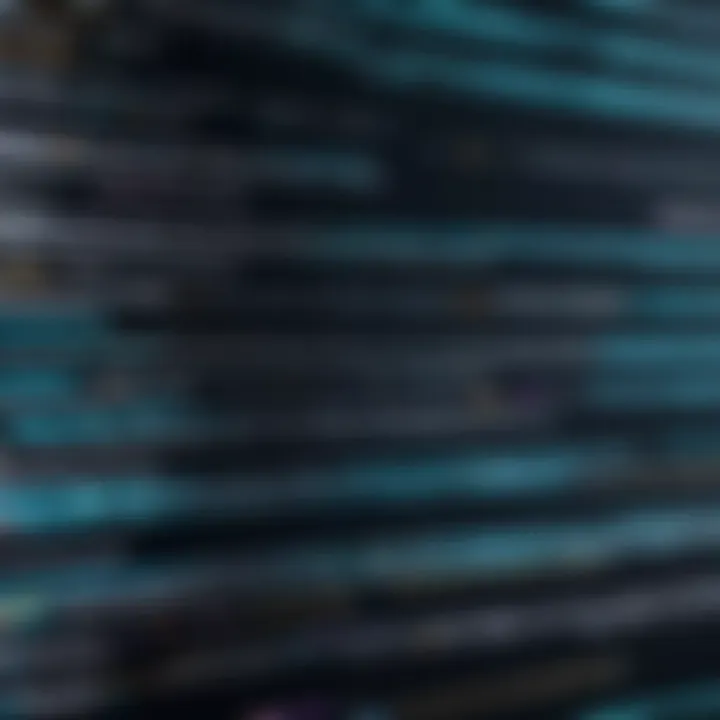
Overview of Topic
Preamble to the main concept covered
C programming is a pivotal element in the realm of computer science. It provides a foundation on which many modern programming languages are built. This guide aims to deliver deep insights into C language programs, focusing on basic principles and illuminating their practical applications.
Scope and significance in the tech industry
With roots dating back to the early 1970s, C continues to be widely used for system and application software. Programs written in C are significant because they maintain efficiency and hardware control, promotes cleanliness in coding standards, and establishes a standard for many runtime libraries used across numerous programming languages today.
Brief history and evolution
Developed by Dennis Ritchie at Bell Labs, C was designed as an evolution of earlier languages such as B. Over the decades, C has evolved, resulting in offshoots like C++. The continued relevance of C can be observed in its applicability in operating systems such as Windows, Linux, and various embedded systems.
Fundamentals Explained
Core principles and theories related to the topic
At its core, C is created on principles of procedural programming. These emphasize the procedure, or function, that is a repeatable and structured code segment. Procedures allow programmers to tackle complex problems through modular design and implementation.
Key terminology and definitions
Understanding some terminology helps establish a strong footing in C programming:
- Variable: A storage location having a specific type.
- Function: A block of code performing a particular operation.
- Pointer: A variable that stores a memory address to facilitate flexible data management.
Basic concepts and foundational knowledge
Begin with a strong understanding of variables, data types, operators, and control structures like loops and conditionals. This classical language does not support object-oriented programming inherently, focusing instead on problem-solving strategies tied closely to system architecture.
Practical Applications and Examples
Real-world case studies and applications
C is the basis of many operating systems, and its efficiency and speed have made it essential for applications that require close communication with hardware. For instance, real-time systems gather input from sensors and provide timely outputs, where C excels due to direct memory management.
Demonstrations and hands-on projects
Some targeted hands-on projects involve writing simple programs, such as:
- A calculator.
- A simple text editor.
- A basic game or simulation.
These showcase how core concepts come to life in typical programming assignments.
Code snippets and implementation guidelines
A typical
Preface to Language
C language is a foundational programming language that has impacted the development of many others. It is highly valued in both educational settings and professional environments. This section explores the strengths of learning C, its historical context, and how this knowledge builds a strong basis for programming. By understanding core concepts of C, students and developers enhance their problem-solving abilities and become more adept at other languages.
Historical Context of Language
C language emerged in the early 1970s, crafted by Dennis Ritchie at Bell Labs. It was developed as an evolution of earlier languages like B, evolving through iterations that focused on creating a more powerful and efficient language. Its release in 1972 marked a pivotal point in programming history. C was designed to facilitate systems programming but quickly gained recognition for its versatility.
Many original applications were systems-based, such as the UNIX operating system, also produced at Bell Labs. C’s influence grew swiftly and can be seen in various modern programming languages like C++, Java, and even Python. C embraces a procedural programming approach, which makes it crucial for developing efficient algorithms and data structures, serving as a stepping stone for countless programmers.
Significant Motives Behind Development
- Develop robust systems programming capabilities.
- Increase efficiency of operations.
- Replace less efficient languages and fulfill gaps encountered in systems hardware.
The legacies of its design choices persist; for instance, C introduced features like compilers and standard libraries that accommodate a wide range of applications, making it especially popular among embedded systems.
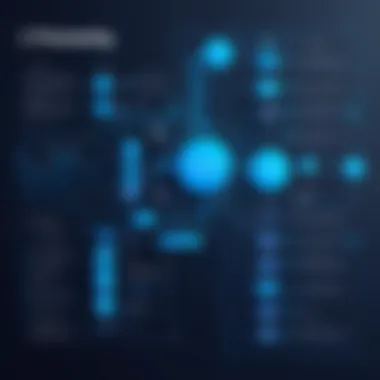
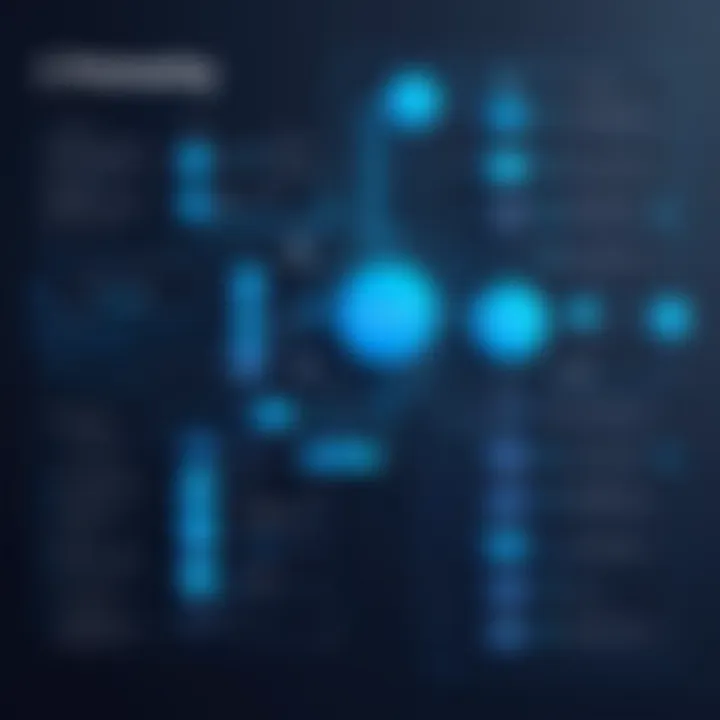
Importance of Language in Programming Education
C language's importance in programming education can not be overstated. It serves as a critical introduction for students and new programmers due to its straightforward syntax and structure. Mastery of C paves the way for understanding more complex languages and paradigms.
The clarity of C sheds light on fundamental programming concepts like control structures, variable types, and memory management. Each area provides insights into computer science and prepares individuals for real-world problems.
Understanding C enhances analytical and logical thinking, essential skills for any software developer.
Educational Benefits of Learning
- Foundational Knowledge: Understanding core programming concepts building blocks for advanced programming.
- Abstraction Understanding: Students learn how high-level functions are mapped to low-level processes, granting better programming practices.
- Problem-Solving: Application of problem-solving strategies reinforces critical thinking abilities conducive to tackling complex programming assignments.
Learning C creates a comprehensive background for students. The challenge in working with this language develops debugging and systematic logical thinking skills, beneficial in environments where precision is necessary.
Through the sections that follow, we will dive deeper into C language syntax, examples of C programs, and the best practices that come out from years of usage. C is essential for all programmers; understanding its intricacies is crucial for anyone investing in a programming career.
Understanding Language Syntax
Understanding C language syntax is essential for anyone wishing to become proficient in programming. Syntax refers to the set of rules that define the structure of statements in the language. Without a solid grasp of syntax, even the most capable logic cannot transform into functional code. This is the foundation that enables a programmer to convey their instructions effectively to the computer.
The relevance of syntax becomes clear when exploring various elements crucial to writing efficient programs, enabling readability and maintainability. By mastering syntax, a programmer can anticipate how changes in code may impact functionality. It builds confidence in navigating the complexities of the language and simplifies debugging challenges, making it a critical focus in any educational context.
Basic Syntax and Structure
The basic syntax of C is characterized by a defined structure. Every C program must have a function. This is where execution begins. Here’s a simple example demonstrating the structural elements of a C program:
This code includes a preprocessor directive, defines the function, and utilizes a standard library function to print text. Each line of code serves a specific purpose. Mastery of such structures allows programmers to create logic that machines understand, establishing a fundamental skill necessary for development in C and beyond.
Data Types and Variables
C provides several built-in data types, including , , , and . These types define the nature of the data stored in a variable. Variables must be declared before they are used, specifying both type and name. This begins their life within the program. Consider this small demonstration:
Each declaration establishes the type of data and effectively communicates the purpose of that variable within the code's context. Use of variables is ubiquitous in programming, as they hold and manipulate data, allowing developers to craft algorithms and computational solutions inputically and readily.
Control Flow Statements
Control flow statements direct the order in which individual components of a program execute. C features several types of control flow including if-else, for, and while statements. These structures permit the program to make decisions and perform repetitive tasks efficiently. For instance:
This example portrays conditional execution based on the age variable. Situational checks can change the program’s path, adapting outputs based on varying inputs. Using such statements properly is vital; it empowers developers to handcraft user interactions dynamically. Understanding this aspect of syntax amplifies the programmer's skill in building cohesive and functional applications.
"Syntax is more than form; it reflects the logic of computation and encapsulates program flow."
Common Language Programs
Common C language programs form the backbone of learning C programming. They enable students and professionals to comprehend both fundamental and advanced programming concepts in a practical environment. By engaging with programs of varying complexities, learners can grasp important concepts essential for further exploration in the field of software development.
The significance of exploring common C language programs cannot be overstated. These programs help refine problem-solving skills. They also allow learners to experiment with different programming constructs such as loops, conditionals, and functions. Practicing these elements prepares one for real-world programming scenarios.
Furthermore, categorizing programs into basic, intermediate, and advanced levels facilitates a structured approach to learning. Each category addresses specific skill gaps and fosters growth at a comfortable pace.
Just like in any language, mastery increases with practice. Valorizing basic, intermediate, and advanced programs helps in building a solid foundation.
Basic Programs for Beginners
These programs are designed to introduce newcomers to the C programming world. They typically underscore foundational syntax, simple data types, and basic input-output operations. Here's a closer look at some exemplary programs:
- Hello World: A classic program, it helps to understand the syntax and structure of C.
- Simple Calculator: This program teaches users about functions and arithmetic operations.
- Interest Calculator: Focuses on developing their skills in handling user input and implementing basic formulas.
Each simple program helps students internalize core programming elements while providing quick feedback on their efforts. Everyone begins with small steps before tackling complexity.
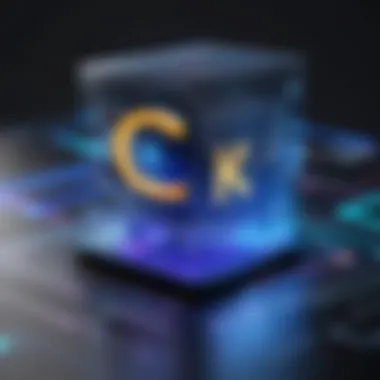
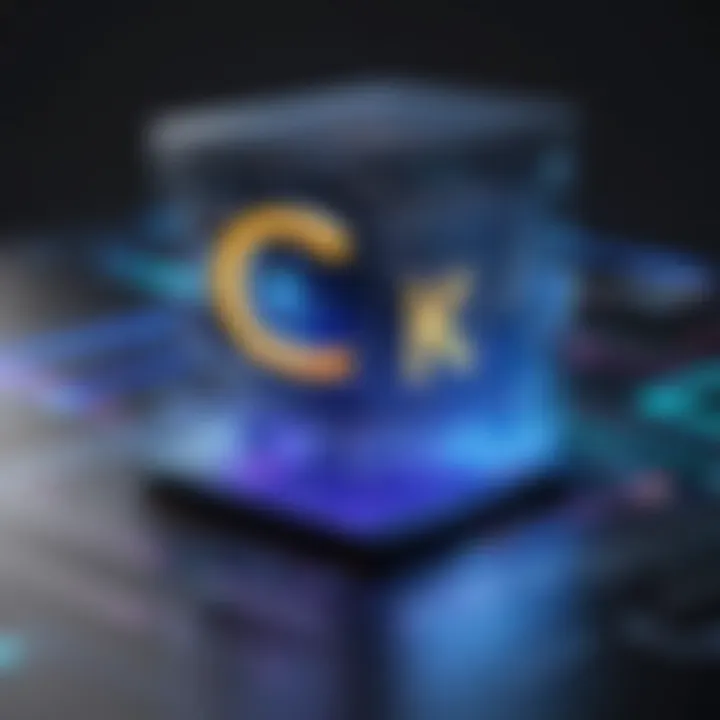
Intermediate Level Programs
Intermediate level programs build upon the knowledge gleaned from basic programming. Students learn to utilize arrays, pointers, and structures while continuing to develop their analytical catalaques. Examples include:
- Sorting Algorithms: Learners can experiment with both Bubble Sort and Quick Sort programs, honing their understanding of algorithms.
- File Handling: Programs demonstrating various file operations deepen knowledge around I/O in C. This area is often pivotal for software applications.
- Tic Tac Toe: A matrix-based game visible functional programming concepts through logic and decision-making.
Through programs like these, users enhance not only their coding ability but also their critical thinking.
Advanced Language Projects
Transitioning to advanced projects signifies expertise in C programming. These engagement-track user proficiency while providing insights into how C can address complex problems. Notable advanced project examples include:
- Operating System Simulation: Involves designing task scheduling or memory management systems, teaching ambitious concepts.
- Embedded Systems Programming: Covers the fusion of C with hardware while adopting programming for efficiency.
- Database Management Systems: Comprehending how to design and implement sophisticated data structures.
By working on these projects, learners prepare themselves for intricate scenarios faced in software development and can collaborate effectively in real workplace settings.
Exploring C language programming projects enhances mastery of the subject and problem-solving agility. It's a forward path that anchors academic grounding in practical applications.
Accessing Language Programs in PDF Format
Access to C language programs in PDF format is of tremendous value for learners and professionals alike. This specific format not only facilitates the distribution of knowledge but also ensures that programming concepts are documented in a structured manner. The readability of PDFs makes them a popular resource among students and practicing programmers.
Accessing these materials is crucial because PDFs preserve the original formatting and layout. This is essential for complex coding examples, ensuring accurate reproduction during study and review.
Additionally, having programs in PDF makes it easier to share materials across different devices and platforms. Users can access them on tablets, e-readers, laptops, or desktops without worrying about compatibility issues. It promotes engaging with content in various environments, enhancing the learning experience.
Benefits of PDF Resources
There are mulitple advantages associated with utilizing PDF resources for C programming. Some of these benefits include:
- Portability: PDFs can be downloaded and accessed on multiple devices, enhancing learning flexibility.
- Preservation of formatting: This means the code examples retain their intended syntax and structure without loss.
- Annotation and markup features: Learners can add notes and highlight sections directly within the document.
- Offline access: Once downloaded, students can study without relying on an internet connection.
Overall, using PDF resources aligns with modern learning approaches, providing efficiency and accessibility.
Finding Quality Language PDFs Online
Though it's clear that PDFs offer rich resources for C programming, identifying quality materials is vital. The vastness of content online can lead to confusion. Here are some efficient methods for locating high-quality C language PDFs:
- Utilize reputable educational websites: Websites like Wikipedia en.wikipedia.org and Britannica britannica.com usually have links to high-caliber resources.
- Join online programming communities: Platforms such as Reddit reddit.com have communities where individuals share valuable resources. Engaging in discussions may reveal some beneficial links.
- Search academic databases: Many universities provide access to scholarly articles and tutorials in PDF format through their libraries.
With these strategies, attaining quality educational resources in PDF can be less daunting, eventually aiding in mastering the C programming language.
Best Practices for Writing Programs
Writing C programs effectively requires adherence to certain guidelines that enhance readability, maintainability, and functionality. Following best practices ensures that both novice and experienced programmers write efficient code. These practices facilitate debugging, promote consistency, and create a logic structure conducive to easier understanding. By emphasizing these aspects, programmers can significantly improve their coding skills and elevate their competency in the C language.
Effective Code Organization
Code organization is fundamental in crafting reliable and maintainable C programs. Properly organizing code not only aids in comprehension but also streamlines collaboration among various developers. When lines of code are systematically arranged, difficulty in navigating can diminish significantly. Key points to consider for effective code organization are:
- Use Comments: Comments clarify the functions and purpose of code segments, guiding future revisions and inquiries.
- Consistent Naming Conventions: Establishing standard naming conventions for variables and functions promotes uniformity, making it easy for others to deduce their meaning quickly.
- Logical Structure: Dividing code into distinct functions or modules adheres to the principle of single responsibility, meaning each function should fulfill a singular purpose.
Adopting these methods ensures that the programs remain coherent and understandable over time.
Debugging and Error Handling
Implementing debugging and error handling techniques is crucial to preventing, identifying, and addressing problems within C programs. An effective programmer must understand that writing incorrect code is part of the process.
Here are significant practices for effective debugging:
- Utilize Compiler Warnings: Ask the compiler to show warnings for common mistakes. This helps catch potential issues before execution.
- Debugging Tools: Employ tools such as GDB to inspect and manipulate running programs, allowing you to find issues where the code fails to execute as planned.
- Error Handling: Anticipate runtime errors by validating inputs. Effective error handling requires catching various input errors before they affect program performance.
Block of code shows basic error handling:
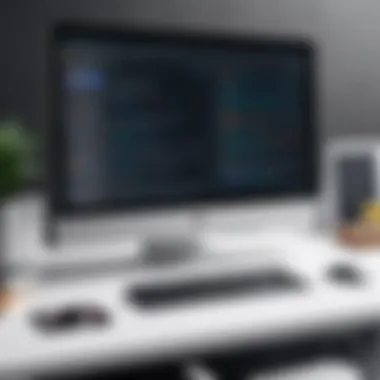
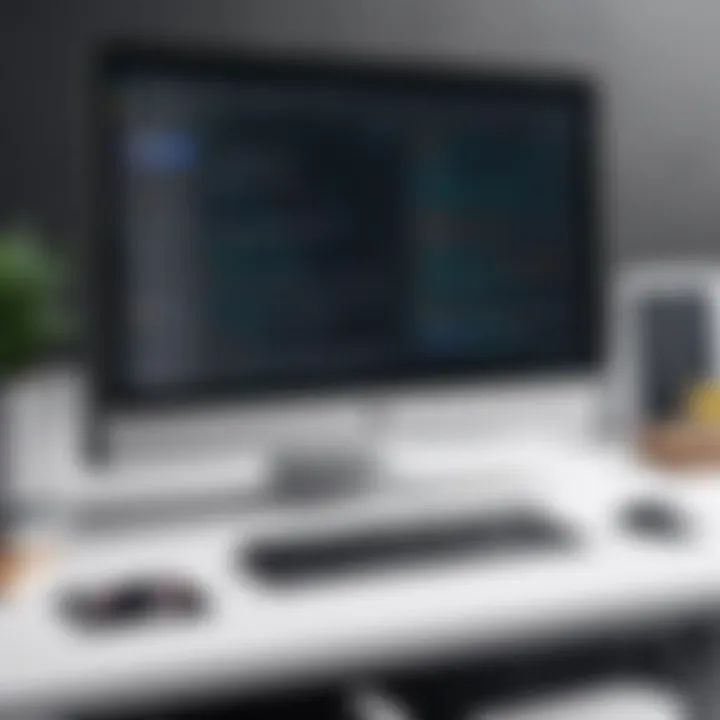
By building these thought processes into practice, programmers can enhance the resilience of their C applications.
Following best practices in writing C programs invariably leads to augmented code quality, thereby improving the programmer's skill set and expertise.
Applications of Language
The C language is pivotal in the landscape of computing and programming. Its versatility spans across various domains and applications. Understanding these applications is vital for anyone interested in programming as it uncovers the practical and theoretical significance of C language knowledge. This section will explore the critical areas where C language thrives.
Systems and Application Software
C language is often employed in the development of system software, which is essential for various operating systems and application frameworks. Its efficiency and durability allow it to handle low-level operations, making it suitable for software that directly interacts with hardware. The Unix operating system, a clear case of C's significance, was primarily developed using this language.
Furthermore, many applications depend on C due to its capabilities to fine-tune memory usage and optimize performance. Applications in which C language finds robust usage include:
- Word processors like Microsoft Word
- Web browsers including Chrome and Firefox
- Database management systems, notably MySQL
C facilitates building components for these programs which require a balance of high-performance and resource management. Hence, its relevance resonates well into software application development.
Embedded Systems
Embedded systems remain one of C’s strongest fields of application. These systems enhance non-computer devices by giving them computational functionality. Devices like microwaves, washing machines, and various industrial controllers often utilize C to ensure their effective operation. In embedded systems, there is often stripped-down software that has a wide range of time constraints and stringent environments where high efficiency and low-level manipulation of devices are required.
Using C for embedded systems also allows for:
- Compact code: As embedded systems often require less memory, C serves to keep the programs lightweight.
- Direct hardware manipulation: C provides the ability to write hardware-specific code, allowing greater control of the lower-level workings of devices.
- Real-time performance: C performs well with real-time systems that need immediate processing.
Contribution to Modern Programming Languages
C language also played a pivotal role in the evolution of modern programming languages. Many contemporary languages, including C++, Java, and Python, draw inspiration or direct syntax from C. This includes various features and concepts like:
- Control structures: Modern languages often employ similar controls as found in C, such as loops and conditional statements.
- Syntax familiarity: Thanks to C's widespread teaching in programming curriculums, new languages inherit familiar syntax which aids learning.
- Performance orientation: As software demands grow, the high performance expected from modern languages remains rooted in the principles established by C.
The legacy of C may not only be in independently successful programs but in how it shaped the alternative languages we have available today. Therefore, its applications extend beyond immediate software and hardware destinations into influencing the programming paradigms we follow.
C language is integral in producing reliable software, forming the backbone of several technology we widely utilize today. Understanding its principle application areas provides insight into the ongoing evolution of programming practices.
Resources for Further Learning
The quest to master the C language does not end simply with coding exercises or understanding syntax. Resources for Further Learning play a crucial role in the educational journey. With the vast array of materials available, learners can enhance their knowledge and skills, catering to various learning paces and styles. Furthering educational endeavors allows for a structured application of the principles learned throughout one’s studies.
Different types of resources can shape the learning experience uniquely. They can bridge theoretical understanding and practical application. Options such as online courses or literature help demystify complexities and provide clarity. Accessing diverse materials empowers individuals to become more resilient learners, apt at pivoting across different aspects of programming.
- Online platforms often foster interactive environments.
- Throughout learning journeys, curated content can inspire motivation.
"Education is the most powerful weapon which you can use to change the world." – Nelson Mandela
In context of C programming, having access to appropriate resources elevates the learner's grasp of both standard and advanced programming principles. Consequently, confidence grows, setting a foundation for practical application in future projects. The following sections reflect on key resources; online courses and books. These resources are particularly important for students, novice programmers, and IT professionals aiming to expand their knowledge.
Online Language Courses
Online C language courses represent a vital avenue for learners seeking structured education. The internet hosts many well-structured programs that cater to all levels, from beginner to advanced. These courses often include video lectures, quizzes, and lively discussion forums. This combination promotes engagement and cultivates a community spirit among learners.
Platforms like Coursera, Udacity, and edX frequently offer courses designed in collaboration with top universities and institutions. This enhances the credibility of the material provided. Many courses cover crucial subjects like data structures, algorithms in C, and project-based learning, allowing students to create functional coding projects.
Another advantage of online courses is flexibility. Students often can pace their learning, particularly significant for those balancing professional commitments alongside learning.
- No rigid scheduling requirements
- Availabile resources can be accessed at any time
- Interactive forums help solidify understanding
Overall, enrollment in these courses can heavily accelerate the learning curve, provide networking opportunities, and expand professional horizons.
Books and E-books on Programming
Books and e-books provide a solid foundation for grasping the intricacies of C programming. The permanence of a printed resource offers learners a persistent point of reference, enabling a deep-dive into topics at their leisure. Top titles, such as "The C Programming Language" by Brian Kernighan and Dennis Ritchie, present comprehensive coverage of the language, establishing core concepts alongside practical exercises.
E-books, on the other hand, offer convenience and accessibility. They typically feature hyperlinks that connect to additional resources, enabling rapid exploration of related subjects. Numerous digital libraries and online retailers host an extensive selection of titles. Some recommended books include:
- "C Primer Plus" by Stephen Prata
- "Head First C" by David Griffiths and Dawn Griffiths
- "C Programming Is a Game" by Earl L. Garrison
Learning from books can enhance retention, allowing individuals to understand complex programming issues methodically. Reading promotes critical thinking and is essential for those preparing for interviews or technical assessments.
In summary, diverse resources enrich the trajectory of anyone pursuing C programming. Both online courses and literature provide unique perspectives and learning experiences that are vital for personal development and professional enhancement.