Mastering Data Structures and Algorithms in Java
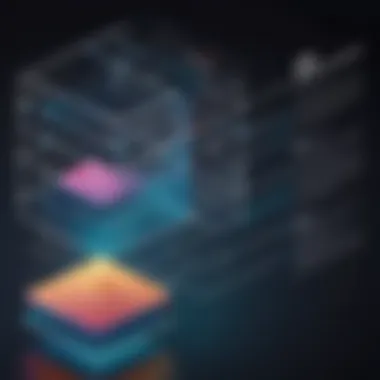
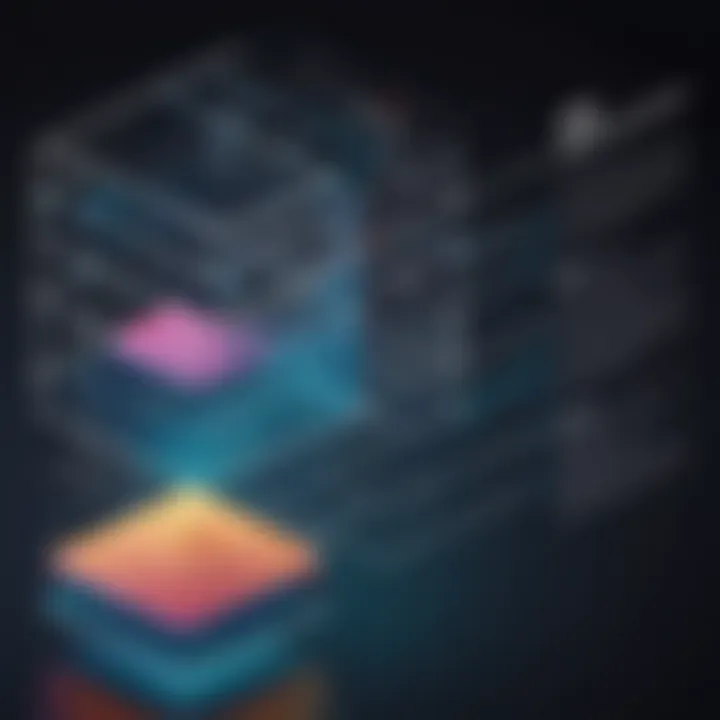
Intro
Data structures and algorithms form the backbone of programming. In the realm of Java, understanding these concepts is critical for anyone looking to excel in software development. This free course aims to equip learners with both theoretical knowledge and practical skills necessary for effective programming.
The relevance of this course is underscored by the increasing demand for software developers who can navigate complex data and implement efficient algorithms. In today's tech-driven world, the ability to analyze and manipulate data structures directly impacts performance and efficiency in applications.
The evolution of data structures and algorithms can be traced back to the early days of computing. They have undergone significant advancements, adapting to the growing needs for fast data processing and storage solutions. As programming languages evolved, so too did the methodologies for organizing and manipulating data. This ongoing development highlights the importance of staying updated with current trends and best practices in the field.
Understanding the course's content is crucial to appreciate its depth. It is structured to guide learners from foundational principles to advanced topics, ensuring a comprehensive grasp of the subject matter. The course material balances theory with practical applications, making it ideal for both novices and seasoned professionals.
Fundamentals Explained
The core principles of data structures include the organization, storage, and retrieval of data. This section emphasizes the importance of recognizing various data structures, such as arrays, linked lists, trees, and hash tables. Each structure has unique characteristics and advantages, tailored for specific types of operations.
Key terminology in this field includes:
- Data Structure: A method of organizing data for efficient access and modification.
- Algorithm: A step-by-step procedure for solving a problem or performing a computation.
- Big O Notation: A mathematical representation that describes the performance or complexity of an algorithm.
Grasping these fundamentals lays the groundwork for further exploration into more complex concepts and usage. Students will find that understanding these basic constructs significantly aids in grasping advanced algorithms and their implementations.
Practical Applications and Examples
Real-world applications of data structures and algorithms are abundant. They are employed in various sectors such as web development, data analysis, and artificial intelligence. For instance, the choice of a specific data structure can drastically affect how effectively a search operation performs in a database.
Hands-on projects often accompany theoretical learning. For instance, constructing a simple project using Java can illustrate how to implement linked lists or trees. Here’s an example code snippet that demonstrates the implementation of a basic linked list in Java:
Through such projects, students can see the tangible effects of their learning and how data structures directly influence application performance.
Advanced Topics and Latest Trends
The field of data structures and algorithms is not static; it is continually evolving. Machine learning, blockchain technology, and data science are pushing the boundaries of how data is structured and manipulated. Advanced techniques like balanced trees, graphs, and advanced searching algorithms are becoming increasingly relevant.
Keeping abreast of new developments is vital. For example, knowledge of algorithm optimization is necessary to understand how large-scale applications manage data efficiently. Practices such as dynamic programming and greedy algorithms also offer unique solutions to complex problems.
Looking forward, upcoming trends suggest a deeper integration of artificial intelligence with traditional data structures. This will likely shape how software is developed and utilized in the near future.
Tips and Resources for Further Learning
To deepen understanding, various resources are recommended:
- Books: "Introduction to Algorithms" by Thomas H. Cormen is highly regarded.
- Online Courses: Platforms like Coursera or edX offer specialized courses on algorithms.
- Tools: IntelliJ IDEA and Eclipse are useful for Java development.
These resources facilitate continued growth and exploration in the realm of data structures and algorithms.
Educators and learners will find that engaging with these concepts lays a strong foundation for further study or professional application in the world of programming. The skills gained from this course will be a valuable asset in any programmer's toolkit.
Intro to Data Structures and Algorithms
Data structures and algorithms form the backbone of computer science and programming. Understanding these concepts allows programmers to create efficient software solutions. In this section, we will explore the definitions of data structures and algorithms, their importance in the field of computer science, and how they pave the way for better programming practices.
Defining Data Structures and Algorithms
A data structure is a way to organize and store data so that it can be accessed and modified effectively. Each data structure has its own unique characteristics. Common examples include arrays, linked lists, stacks, queues, trees, and graphs.
On the other hand, an algorithm is a step-by-step procedure or formula for solving a problem. Algorithms are not tied to any specific programming language, but they play a critical role in how data is manipulated within data structures. They dictate how tasks such as sorting, searching, and traversing data should be performed.
Importance in Computer Science
The importance of data structures and algorithms in computer science cannot be overstated. They allow for efficient data handling, which is vital in many applications. For instance, when dealing with large data sets, choosing the right data structure is crucial for the performance of the algorithm applied.
Efficient algorithms can dramatically reduce run-time complexity and enhance performance.
Furthermore, understanding these concepts aids in problem-solving and enhances coding skills. They are a core focus in technical interviews for programming jobs, indicating their relevance in both academic and professional settings.
In summary, diving into data structures and algorithms equips learners with foundational knowledge, essential for navigating the programming landscape and addressing complex challenges.
Overview of the Course Structure
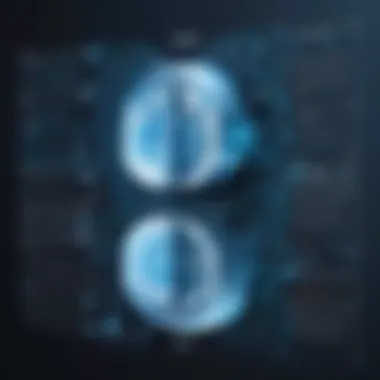
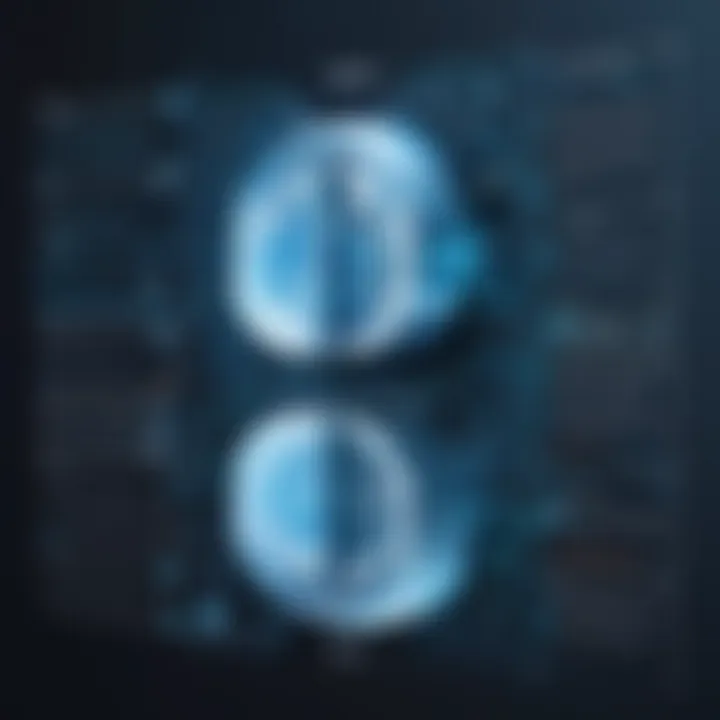
The course structure is an essential component of this comprehensive guide. It lays out the framework for learning data structures and algorithms in Java. Understanding the structure allows learners to navigate the content effectively. This aids in piecing together how different concepts relate to one another. A clear course outline often leads to better retention of information and application skills.
Benefits of having a coherent course structure include:
- Clear progression from basic to advanced topics
- Logical organization that enhances understanding
- Quick identification of areas of interest or necessity
This article defines specific course objectives and goals that are tied to the structured curriculum. Learners can expect a systematic approach to content delivery.
Course Objectives and Goals
The primary objectives of this course focus on equipping learners with fundamental skills and knowledge needed for proficiency in Java. Essential goals include:
- Developing a solid understanding of various data structures.
- Mastering core algorithms and their applications.
- Gaining practical experience through coding examples.
By the end of the course, participants should be able to implement efficient data structures and algorithms in applications. This understanding fosters better problem-solving skills, critical in every programming role.
Target Audience for the Course
This course is designed for a diverse group of individuals interested in programming, especially those seeking to enhance their skills in Java. The target audience includes:
- Students pursuing computer science or related fields
- Professionals looking to upskill or switch to a programming role
- Tech enthusiasts wanting to deepen their understanding of data structures and algorithms
Each participant may come with a different foundation in programming; thus, the course is structured in a way that accommodates varying levels of expertise. The information is presented to ensure that both beginners and experienced programmers can find value and expand their knowledge.
Prerequisites for the Course
Understanding the prerequisites for a course on data structures and algorithms is crucial. These elements lay the foundation for growth and mastery in programming. Each learner should honestly assess their current knowledge base to fully embrace the course content. Without these prerequisites, students may find themselves struggling to keep up, leading to potential frustration and disengagement. Hence, identifying and addressing these requirements fosters a smoother and more enriching learning experience.
Basic Programming Knowledge
Before embarking on this course, students need a solid grasp of basic programming principles. This involves understanding common concepts such as variables, data types, control structures, and syntax of any programming language. The skills should include:
- Problem-solving: Being able to break down problems into smaller, manageable parts.
- Logic: Developing logical thinking to construct algorithms effectively.
- Basic Syntax: Familiarity with constructing simple programs and understanding how logic translates to code.
Gaining these foundational skills will help learners interact with data structures more competently. Students can expect to implement and manipulate structures in their code, enhancing their ability to solve complex problems.
Familiarity with Java
A specific understanding of Java is vital. Java is a versatile language widely used in the industry, making it an ideal medium for this course. Learners should be familiar with:
- Java Syntax and Keywords: Knowing essential keywords and how to structure their code.
- Object-Oriented Programming (OOP): Comprehending concepts like classes, objects, inheritance, and encapsulation. These concepts are integral to understanding data structures organized in an object-oriented manner.
- Java Collections Framework: An awareness of built-in data structures like , , and will serve students well.
By solidifying their understanding of Java, participants can focus on higher-level concepts within the course. They can shift their attention from learning the language mechanics to applying algorithms and data structures effectively.
"A strong programming foundation enables clearer understanding of data structures, making complex algorithms accessible."
The prerequisites ensure all students enter the course with the necessary tools for success. A solid grasp of basic programming concepts and familiarity with Java fosters an environment where students can effectively engage with the learning materials ahead.
Fundamental Data Structures
In the realm of computer science, fundamental data structures serve as the building blocks for efficient data management and manipulation. Understanding these structures is crucial for anyone undertaking a journey in programming, particularly in Java. They enable developers to organize and access data in optimized ways, tailored to specific application needs. Recognizing the strengths and weaknesses of various data structures allows programmers to choose the most suitable ones for their particular tasks, thus enhancing performance and programmability.
Arrays
Arrays are one of the simplest and most widely used data structures in programming. An array is a collection of elements, all of the same type, stored in contiguous memory locations. This characteristic allows for efficient indexing, as accessing an element requires constant time complexity, or O(1). The advantage of arrays lies in their ability to represent data in a linear format, making them ideal for situations where indexing is required. However, they are fixed in size. Once created, resizing an array requires creating a new array, which can be a time-consuming process.
Linked Lists
Linked lists address some of the limitations of arrays. A linked list consists of nodes, where each node contains data and a reference to the next node in the sequence. This structure allows dynamic memory allocation, meaning the size can grow or shrink as needed. Unlike arrays, linked lists do not require contiguous memory, which leads to more efficient memory usage. However, accessing elements within a linked list can be slower, with a time complexity of O(n) for searching, as one must traverse from the head of the list to reach a specific node.
Stacks and Queues
Stacks and queues are specialized data structures that follow specific access rules. A stack operates on a Last In, First Out (LIFO) principle, meaning the last element added is the first to be removed. This structure is particularly useful for tasks like function calls or undo mechanisms in software. In contrast, a queue works on a First In, First Out (FIFO) basis, where the first element added is the first to be served. This is relevant in scenarios like task scheduling or breadth-first search. Although stacks and queues have their own specific uses, they both provide efficient ways to manage data flow in programming.
Trees
Trees are hierarchical data structures that consist of nodes connected by edges. Each tree has a root node and can have multiple levels of child nodes. A tree's structure allows for efficient searching, insertion, and deletion operations. Binary trees, a common variant, allow each node to have up to two children, optimizing search operations to O(log n) in balanced situations. Trees are extensively used in applications like database indexing and representing hierarchical data, such as XML documents. Despite their benefits, managing tree structures can be complex due to their recursive nature.
Graphs
Graphs are versatile structures composed of vertices (or nodes) and edges (connections between nodes). They can effectively represent complex relationships and networks, such as social networks or interconnected systems. Graphs can be directed or undirected and can also be weighted, adding additional layers of complexity. Navigating graphs typically requires algorithms like Dijkstra’s or breadth-first search, which can handle various real-world applications. While powerful, graphs can also become resource-intensive in terms of memory and performance, particularly as the number of vertices and edges increases.
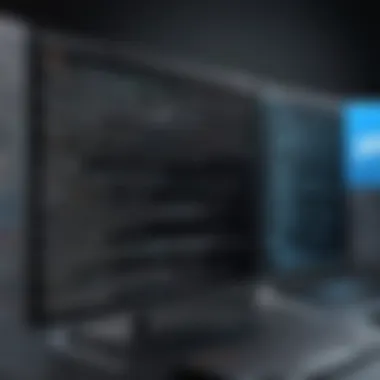
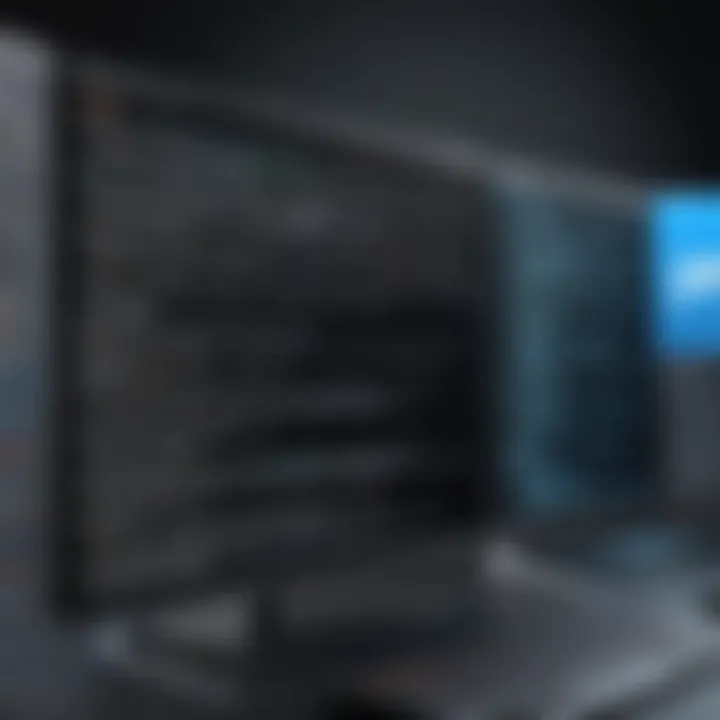
The choice of data structure can greatly impact the efficiency of algorithms and overall performance of applications.
Understanding each of these fundamental data structures lays the groundwork for improving problem-solving skills in programming. Each structure has its own unique features, advantages, and potential drawbacks. Mastery of these concepts is essential as one progresses toward more advanced algorithms and practical applications in Java.
Core Algorithms
Core algorithms serve as essential building blocks in programming. They encompass the methodologies used to manipulate and process data efficiently. Mastery of core algorithms is vital for anyone looking to excel in software development and computer science. Understanding these algorithms not only provides a strong foundation but also prepares learners for tackling real-world programming challenges.
Sorting Algorithms
Sorting algorithms play a crucial role in organizing data. There are various sorting algorithms, including Quick Sort, Merge Sort, and Bubble Sort, each with its unique efficiency. Optimizing the performance of algorithms is fundamental in improving application speed and responsiveness. For instance, when dealing with large datasets, choosing the right sorting algorithm can significantly reduce time complexity.
Some key points about sorting algorithms include:
- Time Complexity: Understanding average and worst-case scenarios is imperative. For example, Quick Sort typically performs at O(n log n) in average scenarios, making it efficient for large arrays.
- Stability: Some algorithms preserve the order of equal elements, which is critical in certain applications. Merge Sort is a stable algorithm, adding to its appeal.
- In-place vs. Out-of-place: In-place algorithms sort without using additional space. Quick Sort is an example of an in-place sort.
These considerations aid in choosing the right sorting method based on specific needs. Proper implementation reduces execution time and enhances performance in Java applications.
Searching Algorithms
Searching algorithms are fundamental for data retrieval. They determine how quickly and efficiently data can be found. The distinction between linear and binary search illustrates the impact of algorithm selection on performance.
Linear search is straightforward but inefficient for large datasets, operating at O(n) complexity. In contrast, binary search requires a sorted array, optimizing the process with a time complexity of O(log n).
In Java implementations, understanding the nuances between these algorithms can avoid unnecessary delays. Key considerations include:
- Search Space: The efficiency dramatically depends on the organization of data. Sorting data beforehand can save time during searches.
- Comparisons: Fewer comparisons, achieved via binary search, often lead to faster results. Learning to apply these algorithms in situations like searching databases or collections is invaluable.
Dynamic Programming
Dynamic programming addresses complex problems by breaking them into simpler subproblems. It employs a method called memoization, where previously computed results are stored for future reference.
The efficiency gained through dynamic programming is significant, particularly in scenarios involving optimization problems. For example, finding the shortest path in a weighted graph can be achieved efficiently. It focuses on:
- Optimal Substructure: Problems can be divided into smaller overlapping subproblems. Understanding this property is essential for applying dynamic programming effectively.
- Overlapping Subproblems: Recognizing when problems share similar smaller problems enables the use of dynamic programming to reduce redundant calculations.
Applications of dynamic programming include algorithms for knapsack problems and Fibonacci series calculations—a fundamental concept for any programmer.
Graph Algorithms
Graph algorithms analyze relationships between data points. They are integral in various fields such as network design and social network analysis. Key concepts include:
- Traversal Techniques: Algorithms like Depth-First Search (DFS) and Breadth-First Search (BFS) navigate through nodes effectively. Each has distinct use cases based on the graph structure.
- Pathfinding: Finding the shortest path involves algorithms like Dijkstra's and A* search. These are essential in applications needing optimal routing, such as GPS direction finding.
Graph algorithms require an understanding of edge and vertex representations. This knowledge can enhance the ability to implement solutions in scenarios ranging from game development to logistic optimizations.
"Understanding core algorithms simplifies complex problem-solving, enabling developers to create efficient applications."
Practical Implementations in Java
Understanding practical implementations in Java is essential for anyone studying data structures and algorithms. This section provides insights that bridge the gap between theory and practice. The effective usage of data structures and algorithms can enhance the performance and efficiency of software applications.
Practical implementations involve coding examples, analysis, and debugging—activities that consolidate theoretical knowledge. By practicing implementation, learners not only solidify their understanding but also build valuable problem-solving skills.
Moreover, implementing data structures and algorithms in Java affords insights into their performance characteristics. Learners will grasp how different data structures operate and their time complexities under various operations, such as insertion, deletion, and searching. This practical experience is invaluable for software developers in optimizing application performance.
Coding Examples and Demonstrations
Coding examples stand as a critical aspect of practical implementations. These examples illuminate how specific data structures and algorithms work in real scenarios. Each covered example typically begins with a clear explanation of the logic or the algorithm being implemented. For instance, let’s consider an example of implementing a binary search tree in Java:
This example demonstrates creating a node structure for the binary search tree and inserting elements accordingly. The use of Java's object-oriented features is evident here.
Besides, demonstrations of algorithms such as quicksort or mergesort can significantly help learners understand sorting techniques and their implementation intricacies. The accompanying runtime complexity analysis brings clarity to why specific algorithms are preferable in certain contexts.
Common Mistakes and Debugging
Even seasoned programmers encounter difficulties, such as logical errors and unexpected output. Recognizing these common mistakes is vital for enhancing one’s programming skills.
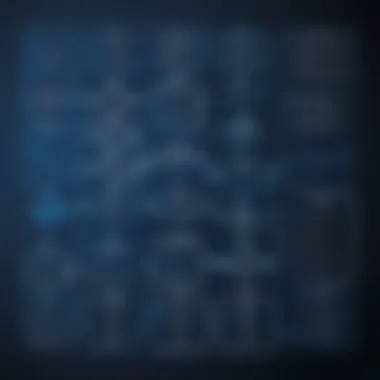
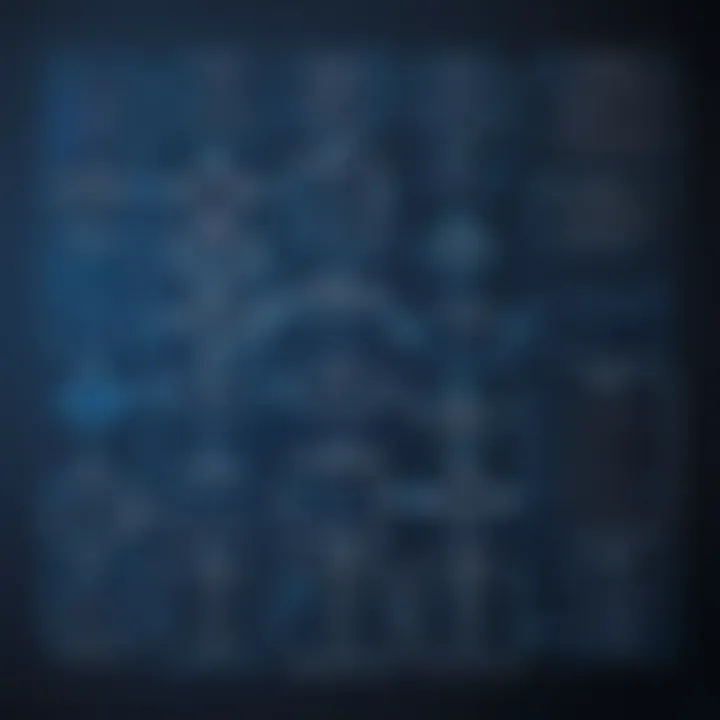
A few frequent coding blunders include:
- Off-by-one errors: Occurs often in loops and can lead to processing one too many or too few elements.
- Null Pointer Exceptions: A common issue in Java that happens when attempting to use an object reference that has not been initialized.
- Inefficient use of data structures: Sometimes, employing the wrong data structure for a task can drastically affect performance.
Debugging requires systematic approaches. Using tools like Integrated Development Environments (IDEs) that offer debugging features can simplify the process.
- Set breakpoints: Essential for inspecting program flow.
- Step through the code: Allows one to observe variable values in real time.
- Log important values: Utilizing statements can provide insight into what might be going wrong.
Additionally, community engagement through forums such as Reddit can offer diverse perspectives and solutions to common problems encountered during practical implementations.
Overall, the aspect of practical implementations plays a fundamental role in mastering data structures and algorithms in Java. In closing, gaining hands-on experience builds a solid understanding that will serve learners well in professional environments.
Real-World Applications
Real-world applications of data structures and algorithms are crucial for understanding their significance in practical programming tasks. The theories and concepts learned in a structured course can be directly applied in various domains of software development and data analysis. This section explores the key use cases and applications that highlight the importance of these elements in actual scenarios.
Use Cases in Software Development
In software development, the choice of data structures impacts performance and efficiency. Different applications require different structures based on their specific needs. For instance, using an array might be suitable for storing a list of items where access time is a priority, while a linked list can be more efficient for applications that involve frequent additions and deletions.
- Web Development: Data structures help manage and retrieve data from databases. For example, hash tables are often used to implement caching mechanisms, improving the speed of data retrieval processes.
- Game Development: Here, algorithms like pathfinding frequently come into play. By employing structures like graphs, developers can enhance navigational aspects in games.
- Mobile Applications: Efficient data usage is critical in mobile environments. Using structures like queues for task scheduling can lead to better performance in handling user interactions.
Applications in Data Analysis
Data analysis relies heavily on algorithms to extract meaningful insights from large datasets. The efficiency of data structures used in this field directly influences how quickly analysts can process information and derive conclusions.
- Data Visualization: Structures are instrumental in organizing data points for visual representation. For instance, using trees can assist in representing hierarchical data, while graphs can depict relationships and connections among varied datasets.
- Machine Learning: Algorithms like sorting and searching play a significant role in preparing data for analysis. Using efficient data structures can also enhance model training efficiency, leading to improved predictive analytics.
- Statistical Analysis: Access to data in various formats is crucial. Employing efficient data structures helps in performing operations like aggregations and statistical calculations with speed.
"Choosing the right data structure can significantly enhance performance and enhance the effectiveness of an application."
In summary, real-world applications of data structures and algorithms emphasize their importance in programming. Understanding their uses helps developers and analysts to make informed choices about the tools and methods they use in their daily tasks. The seamless integration of theory with practice enhances skills and knowledge in software development and data analysis.
Resources for Further Learning
Understanding data structures and algorithms is just the beginning of the journey for any aspiring programmer. Resources for further learning play a crucial role in enhancing one's comprehension and practical skills in the field. This section emphasizes the importance of accessing additional materials and platforms that can complement the foundational knowledge acquired in the course. With a variety of options available, learners can tailor their educational experience to suit individual needs and preferences, thus fostering a deeper grasp of complex concepts.
Recommended Reading Material
Reading is an effective way to solidify understanding of data structures and algorithms. Here are some recommended books that can serve as valuable resources:
- "Introduction to Algorithms" by Thomas H. Cormen: This book is a staple in computer science education and covers both data structures and algorithms comprehensively.
- "Data Structures and Algorithms Made Easy" by Narasimha Karumanchi: This title simplifies complex concepts, making them more accessible.
- "The Algorithm Design Manual" by Steven S. Skiena: It provides practical approaches and real-world examples, which can be very helpful for applying learned concepts.
These books cover a range of topics and difficulty levels, ensuring that readers can find material suited to their current understanding.
Online Platforms and Tools
In addition to books, online platforms and tools offer interactive and engaging ways to continue learning about data structures and algorithms. Consider the following:
- Coursera: Offers various courses on algorithms and data structures from reputable institutions.
- edX: Provides access to information from top universities, covering a wide array of related subjects.
- LeetCode: A platform focused on coding challenges and interview preparations that helps solve practical problems using data structures.
- GeeksforGeeks: Contains articles, tutorials, and a vast repository of problems to practice.
Utilizing these resources can enhance critical thinking and improve problem-solving abilities. The combination of reading materials and interactive practice allows learners to reinforce their knowledge and prepare effectively for real-world applications.
The End and Final Thoughts
In the realm of computer science, data structures and algorithms serve as fundamental building blocks. Their mastery is crucial for anyone who desires to excel in programming or software development. The overarching goal of this article has been to provide a thorough understanding of these key concepts, particularly with the Java programming language as the medium of instruction.
Throughout this course, various aspects have been explored. Students were equipped with knowledge regarding different data structures like arrays, linked lists, and trees. Additionally, key algorithms such as sorting and searching were discussed in detail. Understanding these topics is not merely an academic exercise; they have profound implications in real-world applications, from optimizing search processes to enhancing performance in software.
The course offers significant benefits. It helps develop problem-solving skills, improve coding efficiency, and elevate one’s programming proficiency. By applying what was learned, individuals can solve complex problems with more efficiency. The practical implementations discussed also provide insight into how these structures and algorithms operate, which is essential in both academic and professional settings.
"The essence of data structures and algorithms is not just in learning them, but in understanding how they transform the way problems are approached and solved."
Thus, the importance of this module cannot be overstated. As learners reflect on these building blocks, they will find themselves better prepared to tackle challenges in programming and beyond. Understanding the foundations lays a pathway to advanced topics and specialized fields, such as machine learning and data science.
Recap of Key Concepts
Over the course of this journey, we have delved into significant concepts:
- Data Structures: We examined essential structures including arrays, linked lists, stacks, queues, trees, and graphs.
- Algorithms: Fundamental algorithms including sorting algorithms like quicksort and mergesort, searching algorithms such as binary search, dynamic programming strategies, and graph algorithms were introduced.
- Practical Implementations: The transition from theory to practice was emphasized. Examples illustrated how to implement these concepts in Java, solidifying the learning experience.
These core topics form the backbone of effective programming. Mastery of these concepts enables individuals to make informed decisions when confronted with coding challenges.
Next Steps in Learning
As learners complete this course, several pathways emerge for continuous growth:
- Advanced Courses: Consider enrolling in specialized courses focusing on areas such as data science, machine learning, or system optimization.
- Projects: Engaging in projects that require the application of data structures and algorithms can enhance practical skills. Building applications or contributing to open-source projects offers hands-on experience.
- Community Involvement: Participating in forums such as Reddit or stack overflow can foster discussions, exchange experiences, and receive feedback.
- Books and Online Resources: Reading additional literature on advanced algorithms or data structure optimization can deepen understanding. Platforms like en.wikipedia.org and britannica.com provide insightful content for further exploration.