Mastering Data Structures in Python: A Comprehensive Guide
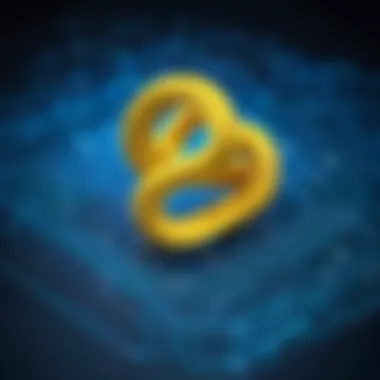
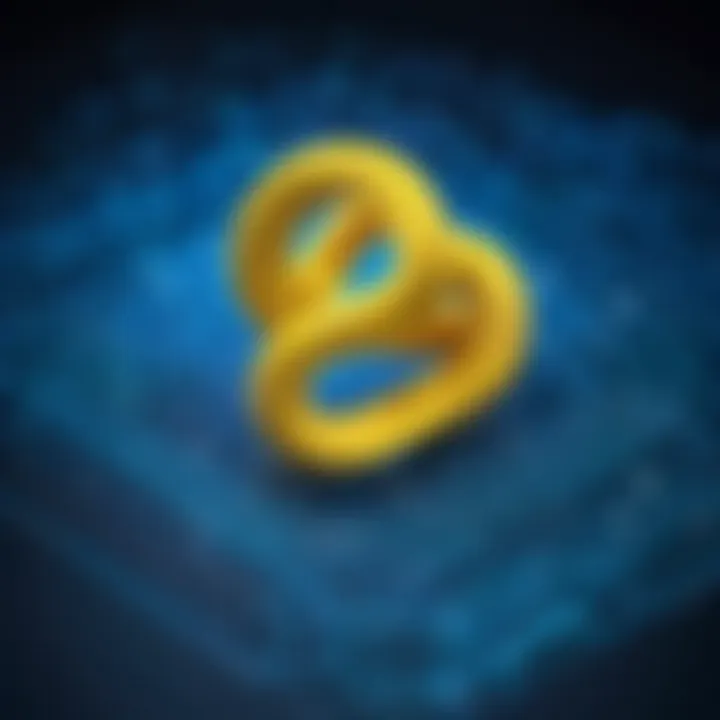
Overview of Topic
Data structures represent a critical component of programming in Python. They are essential for organizing, managing, and storing data effectively. Understanding these structures allows developers to enhance their coding skills and optimize the performance of their applications. The significance of data structures is evident across various fields in the tech industry, including software development, data science, and artificial intelligence.
Historically, data structures have evolved alongside programming languages. Early programming relied on simple structures like arrays and linked lists. As the complexity of software applications grew, so too did the need for more sophisticated structures like trees and graphs. Today, Python provides a rich environment for utilizing various data structures, thanks to its built-in types and libraries like and .
Fundamentals Explained
At their core, data structures are tools used to organize and manipulate data in a way that makes it efficient to access and modify. Understanding core principles like time complexity and space complexity is vital. These metrics help assess how the performance of a data structure can impact the efficiency of an algorithm.
Some key terminology includes:
- Array: A collection of items stored at contiguous memory locations.
- List: An ordered collection of elements in Python that can contain duplicates.
- Stack: A linear structure that follows a Last In First Out (LIFO) order.
- Queue: A linear structure that follows a First In First Out (FIFO) order.
- Graph: A collection of nodes connected by edges, used to represent networks.
- Tree: A hierarchical structure consisting of nodes, where each node can have child nodes.
These concepts form the foundation for more advanced data structures and algorithms, which are pivotal in developing efficient programs.
Practical Applications and Examples
Data structures have numerous real-world applications. For example, algorithms that sort and search data benefit from understanding how to implement different structures. In web development, lists and dictionaries in Python are used for handling user data, managing inventory in e-commerce sites, and processing application requests.
Some practical hands-on projects might include:
- Building a simple to-do application using lists to store tasks and dictionaries to handle task attributes.
- Creating a text-based maze with a graph to represent the paths between locations.
- Implementing an algorithm like quicksort to understand how sorting structures operate under the hood.
Here is an example of how to implement a basic stack in Python:
Advanced Topics and Latest Trends
In the fast-evolving tech landscape, data structures are continually developing. Advanced data structures like tries and segment trees address specific computational problems, enhancing efficiency. Techniques like parallel algorithms and distributed data structures have emerged due to the increasing demand for high-performance computing.
Future trends suggest an increased reliance on data structures that integrate with machine learning models. Structures like tensors in libraries such as TensorFlow allow for multidimensional data representation, which is critical in deep learning applications.
Tips and Resources for Further Learning
To deepen your understanding of data structures in Python, consider the following resources:
- Books like "Data Structures and Algorithms in Python" by Michael T. Goodrich.
- Online courses from platforms like Coursera or Udemy focusing on data structures and algorithms.
- Python libraries to practice with include and .
- Engage with communities on Reddit or Facebook where members discuss various implementations and optimizations.
By utilizing these tools and resources, you can solidify your understanding of data structures and improve your programming skills.
Foreword to Data Structures
Understanding data structures is foundational in programming, especially in Python. Data structures allow for the organization, management, and storage of data in a manner that enables efficient access and modification. They play a vital role in how algorithms are designed and executed, impacting the performance of software applications. Without a solid grasp of these concepts, writing effective code becomes increasingly challenging.
Definition of Data Structures
A data structure is a specialized format for organizing and storing data. It allows for efficient operations such as insertion, deletion, and access. The choice of data structure can fundamentally affect the performance of an algorithm. Common data structures include arrays, lists, dictionaries, and trees. Each has its own advantages and disadvantages based on its underlying principles of operation. Understanding these attributes is critical for effective programming.
Importance in Programming
Data structures are significant for several reasons. They provide a means to manage large volumes of data with varying access patterns. Choosing the correct data structure can lead to enhanced performance, particularly in the areas of speed and resource usage. Also, they help in constructing algorithms to solve complex problems. For instance, using a hash table could significantly speed up lookups compared to a list.
Additionally, a solid understanding of data structures is crucial for succeeding in coding interviews, as many problems presented in these scenarios focus on manipulating data efficiently. Therefore, a strong command over data structures not only improves the quality of software solutions but also equips programmers with essential skills for their careers.
Overview of Data Structures in Python
Python provides a rich set of data structures for programmers to use. The built-in structures, such as lists, tuples, and dictionaries, offer flexibility and ease of use. Each data structure is designed to serve specific needs, whether it be for handling simple collections of items or managing complex relationships between data.
In Python, lists are dynamic arrays, allowing elements to be added or removed with ease. Tuples, on the other hand, are immutable sequences, perfect for fixed data. Dictionaries provide a mapping mechanism, allowing for fast key-value pair representation. Lastly, sets are used to store unique elements, making them ideal for membership testing.
With the unique aspects of Python's data structures and their practical implementations, understanding these tools is imperative for anyone looking to excel in programming. This foundational knowledge sets the stage for exploring more complex data structures and their applications in solving real-world problems.
Types of Data Structures
Understanding the types of data structures is crucial for effective programming in Python. Each data structure organizes data in specific ways, influencing how easily and efficiently algorithms can process that data. By categorizing data structures into two main types—primitive and non-primitive—developers gain insights on appropriate data management techniques. This knowledge is imperative, as it directly affects performance, memory consumption, and code clarity.
Primitive Data Structures
Primitive data structures are the simplest forms of data storage. They include basic types like integers, floats, characters, and booleans. In Python, these structures serve as the foundation for building more complex data types. The motivations for using primitive data structures include clarity and performance.
For instance, consider this code snippet:
The integer 10 and boolean True are examples of primitives that can be manipulated easily. Storing data in a primitive form can speed up calculations, ease debugging, and enhance readability of the code. Moreover, they simplify the storage and retrieval of fundamental data types in a program.
Non-Primitive Data Structures
In contrast, non-primitive data structures are more complex and are built using primitive data structures. They can hold multiple values and can be organized in various ways. Examples of non-primitive structures include lists, tuples, dictionaries, and sets in Python.
Each of these structures serves different purposes:
- Lists allow for ordered collections that can change elements dynamically.
- Tuples provide immutability, useful for fixed data sets.
- Dictionaries are key-value pairs that facilitate quick data retrieval.
- Sets enable storage of unique items, making them useful for membership tests and eliminating duplicates.
The choice between these structures depends on the specific needs of a program. This understanding allows programmers to optimize code performance and maintainability while choosing an appropriate method for data organization.
In summary, recognizing the difference between primitive and non-primitive data structures is vital for coding efficiency. Each type presents unique attributes suited for specific tasks, forming the backbone of data manipulation in Python programming.
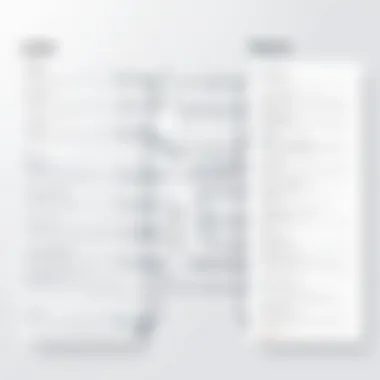
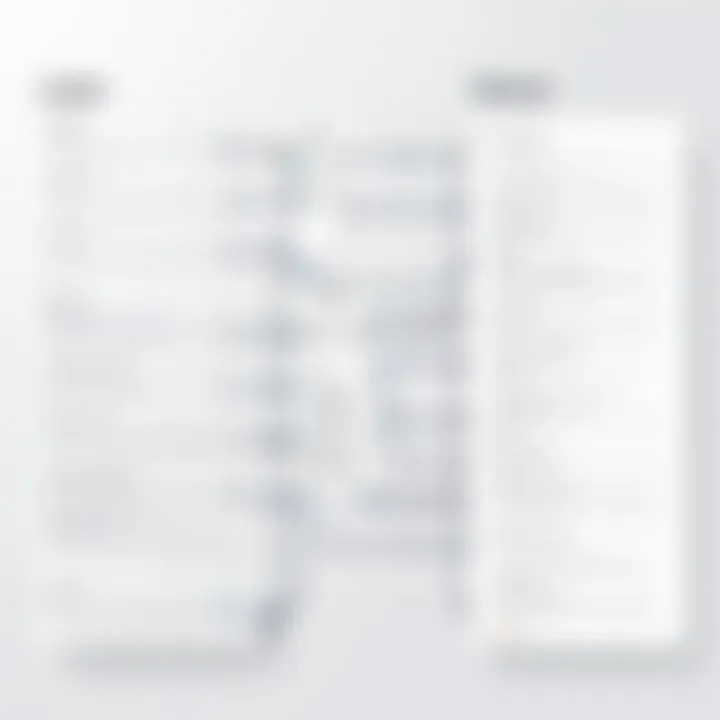
Built-in Data Structures in Python
Python offers several built-in data structures that provide flexibility and efficiency in code development. These structures are integral to Python, as they allow programmers to organize and manipulate data in an effective way. Understanding these built-in data structures is crucial for anyone looking to write efficient and optimized code.
Lists
Lists are one of the most versatile data structures in Python. They allow you to store an ordered collection of items that can be of varying types. Important characteristics of lists include:
- Dynamic Sizing: Unlike arrays in some programming languages, lists can grow in size. You can append elements as needed without manually managing the size.
- Indexing and Slicing: Elements in a list can be accessed using indices, which makes manipulation straightforward. For instance, accesses the first item.
- Mutability: Lists are mutable, meaning you can change their content after creation. This allows for easy updates and deletions of elements.
Lists provide a foundation for various algorithms and often serve as the basis for more complex data structures.
Tuples
Tuples are similar to lists; however, they are immutable. This means once a tuple is created, it cannot be altered. Key aspects of tuples include:
- Immutability: Useful for protecting data from accidental modification. This feature is beneficial when creating fixed collections.
- Performance: Tuples usually have a smaller memory footprint and can be faster than lists for large data sets due to their immutability.
- Unpacking: Allows for easy assignment of multiple variables. For example:
Tuples are advantageous in situations where data integrity is essential, such as passing data to functions without allowing changes.
Dictionaries
Dictionaries in Python provide a way to associate key-value pairs, making data retrieval efficient. Some notable features include:
- Key-Value Association: Keys must be unique, while values can be duplicated. This association allows for quick lookups.
- Dynamically Sized: Similar to lists, dictionaries can dynamically grow based on the number of entries.
- Versatility: They can store varied types of data. For instance, a dictionary can contain lists, tuples, and even other dictionaries.
Dictionaries are particularly useful for scenarios requiring frequent data retrieval, such as in web applications and data analysis workflows.
Sets
Sets are collections of unique elements. They provide a way to perform mathematical set operations like union and intersection. Essential characteristics include:
- Uniqueness: Duplicates are automatically removed, ensuring all elements are unique.
- Unordered: The elements in a set do not have a defined order. They cannot be accessed by index.
- Mutable: New elements can be added or removed from a set after it is created.
Sets are helpful in situations where you need to eliminate duplicates from a dataset or perform common set operations.
In summary, built-in data structures in Python provide essential tools for managing and organizing data effectively. Each structure has its unique strengths and weaknesses, and understanding these differences is key to writing efficient Python code.
Complex Data Structures
Complex data structures play a crucial role in computer science and software development. They allow for the organization of data in ways that enhance efficiency and the utilization of resources in programming. Understanding complex data structures is necessary for solving many real-world problems because they provide the means to represent intricate relationships and processes. As developers handle more nuanced datasets, the need for these structures becomes increasingly essential. In this section, we will explore four primary types of complex data structures: stacks, queues, trees, and graphs. Each has unique characteristics and applications, making them indispensable tools in a programmer's toolkit.
Stacks
Stacks are a type of data structure that follows a Last In First Out (LIFO) principle. This means that the last item added to the stack is the first one to be removed. The operations for stacks are straightforward: pushing an item onto the stack and popping an item off the stack. Stacks are commonly used in programming for various tasks.
Key applications of stacks include:
- Function calls: Managing active function calls in programming languages.
- Undo mechanisms: Implementing undo functionality in applications.
- Expression evaluation: Used in parsing expressions for compilers.
The basic implementation in Python can look like this:
Queues
Queues operate on a First In First Out (FIFO) model. In this structure, the first element added is the first to be removed. Queues are essential for scenarios where order matters, such as processing tasks in a sequence. This is commonly seen in scheduling and managing resources.
Common uses of queues include:
- Job scheduling: Managing tasks in a server environment.
- Buffering: Helping in streaming data processes where data arrives at runtime.
- Breadth-first search: Utilizing queues for exploring nodes in graph structures.
An example of queue implementation in Python is:
Trees
Trees are hierarchical data structures that are widely used in programming due to their efficient organization of information. Each tree has a root node, and each node may have child nodes, forming a parent-child relationship. This makes trees ideal for representing relationships and structures where data is non-linear.
Uses for trees include:
- Storing hierarchical data such as file systems.
- Managing databases through binary search trees.
- Implementing decision processes in algorithms.
A simple representation of a tree node in Python may look like this:
Graphs
Graphs consist of nodes connected by edges and are one of the most versatile data structures. They can be directed or undirected, and weight can be applied to the edges, allowing for the representation of more complex relationships. Graphs are used extensively in various applications, such as social networks, geographic mapping, and networking.
Applications for graphs encompass:

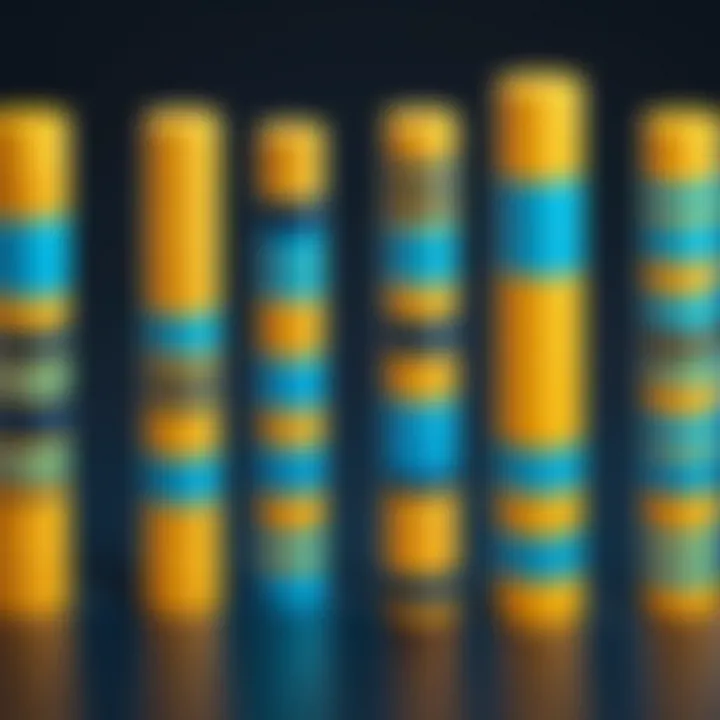
- Navigating maps using algorithms like Dijkstra's.
- Analyzing social connections and patterns.
- Representing networks of connections in data analysis.
A basic implementation in Python might look like:
Understanding the depth and versatility of complex data structures is essential for effective problem-solving in programming. Mastery of these concepts can lead to more efficient and optimized code.
Implementing Data Structures in Python
Implementing data structures in Python is crucial for understanding how to manage and organize data efficiently. This section elucidates why it is fundamental for programmers to know how to implement various data structures effectively. Understanding data structure implementation helps optimize both performance and resource management in applications.
Creating Lists and Their Operations
Lists in Python are versatile and allow easy storage of sequences. You can add, remove, or modify elements efficiently. Creating a list starts with square brackets, and operations can be performed using built-in functions. For instance, you can append an item with .
Here are some common operations:
- Append: Adds an element to the end of the list.
- Insert: Places an element at a specified index.
- Remove: Deletes the first occurrence of a value.
- Slice: Retrieves a part of the list based on indices.
Using Tuples for Fixed Data
Tuples are a type of data structure that, unlike lists, are immutable. This means after their creation, the elements cannot be changed. They are useful when you want to store read-only data. The syntax involves parentheses instead of square brackets. Tuples offer performance benefits due to their immutability, making them faster in certain operations compared to lists. Here's an example:
Manipulating Dictionaries
Dictionaries provide a way to store data in key-value pairs. This structure allows for efficient lookups and modifications. Creating a dictionary is straightforward using curly braces. You can access values through their associated keys. Manipulating dictionaries includes adding, deleting, and modifying key-value pairs.
Here is how you can create a basic dictionary:
- Creating a Dictionary:
Working with Sets
Sets in Python are collections that do not allow duplicate elements. They are particularly useful for operations involving unique items. Creating a set is similar to a dictionary but without key-value pairs, using curly brackets. Operations include union, intersection, and difference, which are beneficial in various algorithms.
Example of creating a set:
Sets provide an efficient way to remove duplicates from a collection, maintaining only unique items.
In summary, Python's data structures can be easily implemented and manipulated through simple syntax and methods, allowing programmers to handle different data efficiently.
Advanced Data Structure Concepts
In the realm of programming, particularly Python, advanced data structure concepts hold significant relevance. They deepen the understanding of how data can be managed and manipulated effectively. Comprehending these concepts allows developers to optimize their code significantly. Addressing both time and space complexities are crucial elements in enhancing program performance. This section delves into two integral aspects: time complexity and space complexity.
Time Complexity Overview
Time complexity provides a way to evaluate the efficiency of an algorithm in terms of the time it takes to complete as the input size grows. It is generally expressed using Big O notation. Understanding time complexity is important for several reasons:
- Performance Measurement: It offers a clear insight into the how the speed of an algorithm is affected by large inputs.
- Comparative Analysis: Different algorithms can be evaluated and compared based on their time efficiency to choose the best option for a particular problem.
- Scalability: Knowledge of time complexity is vital when planning for algorithms that have to scale with large datasets.
Basic time complexities can generally be categorized into:
- Constant Time (O(1)): The execution time remains constant, regardless of input size.
- Linear Time (O(n)): The execution time grows linearly with the increase of input size.
- Quadratic Time (O(n²)): The execution time grows quadratically as the input size increases.
To illustrate, consider a simple Python function that searches for an element in a list:
This function operates in O(n) time complexity because, in the worst-case scenario, it examines each element once. Knowing this allows programmers to make informed decisions when selecting algorithms for their applications.
Space Complexity Explained
Space complexity refers to the amount of memory required by an algorithm to complete in relation to the input size. Like time complexity, it is also expressed in Big O notation. Understanding space complexity is essential for several reasons:
- Resource Management: It helps developers manage memory effectively, which is critical in environments with limited resources.
- Optimal Allocation: Developers must understand the memory costs associated with algorithms to allocate resources appropriately during their applications.
- Performance Implications: High space complexity can lead to increased runtime and potentially cause applications to crash due to memory shortages.
Space complexity considerations can be divided into:
- Fixed Part: This includes the space required for constants, variables, and program code.
- Variable Part: This encompasses dynamically allocated space that depends on the inputs provided during execution.
To further elucidate space complexity, consider a Python function that creates a list of numbers:
The space complexity here is O(n), as the memory needed to store all integers from 0 to n-1 increases linearly with the input size.
Both time and space complexity are critical when discussing advanced data structures. They allow developers to make informed choices in algorithm selection and optimization. A strong grasp of these concepts leads to better programming practices and performance enhancements in Python.
Common Use Cases of Data Structures
Understanding the common use cases of data structures is a vital part of learning how to program effectively in Python. Data structures are not just abstract concepts; they have real-world applications that influence how applications perform and scale. This section examines how selecting the appropriate data structure can enhance data storage solutions and interact with algorithms efficiently.
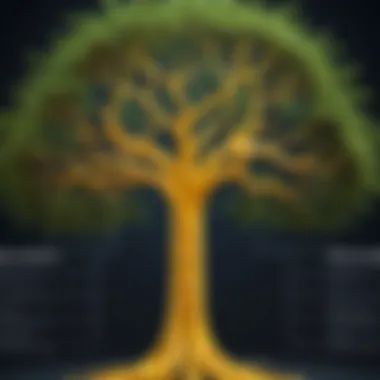
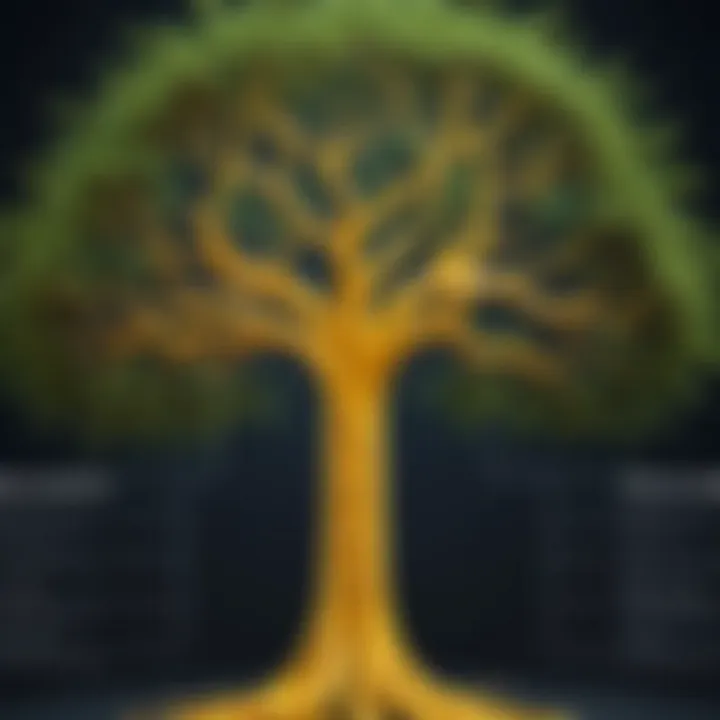
Data Storage Solutions
Data structures form the backbone of data storage in computer applications. They enable efficient organization and retrieval of information. Choosing the right structure impacts performance, especially in resource-constrained environments where efficiency is crucial.
Common data storage solutions include:
- Arrays: Useful for fixed-size data storage.
- Linked Lists: Ideal for dynamic data that frequently changes. Their ability to grow and shrink makes them suitable for applications like implementing undo features in text editors.
- Hash Tables: Provide average-case constant time complexity for lookups. They are widely used in databases to facilitate quick access to data.
When designing a system, consider
- Access Patterns: Understand how data will be accessed.
- Data Volume: The amount of data influences whether to use a simpler structure like an array or a complex one like a graph.
"The selection of data structure in a program is as important as the algorithms in use."
Algorithms and Data Structures
The interplay between algorithms and data structures is profound. An algorithm's efficiency often depends on the data structure's ability to support its operations. For instance, certain sorting algorithms perform better with specific data structures.
Key considerations when connecting algorithms with data structures include:
- Time Complexity: Assess how fast an algorithm runs based on the input size, which can vastly differ depending on the data structure used.
- Space Complexity: Evaluate how much memory an algorithm uses. For example, using a binary tree can be more memory efficient compared to using an array when handling hierarchical data.
Popular algorithm data structures include:
- Heaps: Used in priority queues, allowing quick access to the highest or lowest value.
- Graphs: Essential for complex networking solutions, such as routing algorithms.
Real-world Applications in Python
Understanding real-world applications of data structures in Python is essential for grasping the practical implications of theoretical concepts. Data structures serve as the foundation for organizing, managing, and retrieving data efficiently, which is critical across various domains and industries. This section deliberates on two major areas where Python data structures are fundamental: web development and data science. By examining these applications, we can appreciate the impact of choosing appropriate data structures on software performance, maintainability, and scalability.
Web Development
In the realm of web development, choosing suitable data structures can significantly influence the efficiency of web applications. A web application often manages user profiles, session data, and content storage. Here, Python's built-in data structures such as dictionaries and lists come into play.
Dictionaries are particularly vital for managing key-value pairs. For example, a user profile can be represented as a dictionary, where the keys are the user attributes (like name, email, etc.), and the values are the corresponding user details. This structure allows for fast lookups, as dictionary keys provide a straightforward method for accessing data.
Moreover, lists are useful for handling ordered data, such as a collection of user comments on a blog. The ability to easily append and retrieve comments ensures that web applications offer a good user experience. For instance, in a news aggregator, list structures allow efficiently caching and displaying articles in the order they were added.
When dealing with concurrent users, stacks and queues can be important as well. Queues help manage users in a chat application, maintaining an orderly flow of incoming messages. Despite the simplicity of these structures, their effectiveness is critical in ensuring performance under load.
Data Science and Analytics
In the field of data science and analytics, data structures play an equally crucial role. This domain often requires the manipulation of large datasets, necessitating efficient storage and access strategies. Python supports several libraries, such as NumPy and Pandas, which build data structures tailored for these tasks.
For instance, NumPy arrays serve as a highly efficient way to store numerical data. They offer significant performance improvements over standard Python lists, especially in mathematical operations. Large datasets can thus be processed quickly, which is vital for tasks such as statistical analysis or machine learning model training.
Pandas DataFrames extend this idea by providing a powerful structure for labeled data manipulation. DataFrames facilitate data cleaning and transformation, allowing analysts to work with complex datasets more intuitively. This helps analysts and data scientists perform data wrangling efficiently, preparing datasets for analysis and visualization.
To summarize, real-world applications in both web development and data science underscore the importance of appropriately chosen data structures. They profoundly affect both the development process and the runtime performance of applications. Understanding these applications equips developers with the knowledge to make informed decisions, enhancing their programming skill set.
"Choosing the right data structure is crucial in optimizing the performance of Python applications across various domains."
This practical knowledge is not only beneficial for students and those learning programming languages but also invaluable for IT professionals who need to implement robust solutions.
Best Practices for Using Data Structures
In programming, the choice and implementation of data structures can significantly affect the performance and efficiency of an application. This section outlines best practices for using data structures in Python. Adopting these practices is essential for creating optimal, maintainable, and scalable code. When selecting and working with data structures, consider the following elements and benefits that can enhance your programming experience.
Effective utilization of data structures directly influences application performance.
Choosing the Right Data Structure
When faced with the task of selecting a data structure, it is vital to assess the requirements of your program. Each type of data structure offers unique strengths and weaknesses. Here are some key considerations for choosing the right one:
- Nature of Data: Consider how the data will be accessed and manipulated. For example, if frequent insertion and deletion are required, a list might be less efficient than a linked list.
- Operations Needed: Different data structures excel in different operations. Dictionaries offer fast lookups, while sets are optimal for unique item storage.
- Memory Usage: Analyze the memory footprint of each data structure. Lists, for example, can consume more memory compared to tuples because they are mutable.
Choosing the right data structure can lead to more efficient algorithms and code optimization, which saves time in both development and execution.
Optimizing Performance
Once a suitable data structure has been chosen, optimizing its performance is the next critical step. Below are strategies to enhance the efficiency of your data structure utilization:
- Access Patterns: Understanding access patterns of your data can lead to optimizations. For instance, if you are frequently accessing the last element in a list, consider using a deque from the collections module, which allows for O(1) time complexity for appends and pops from both ends.
- Amortized Analysis: Consider how operations affect performance over time, rather than in isolation. Sometimes, a seemingly inefficient operation might be optimized over multiple uses.
- Use Built-in Functions: Take advantage of Python’s built-in functions. For instance, using list comprehensions can significantly improve the speed and readability of code.
By focusing on these optimization techniques, programmers can ensure that their applications are not only functional but also efficient.
Adopting best practices for selecting and optimizing data structures within Python is pivotal for anyone looking to improve their programming skills. Emphasizing the importance of making informed choices will lead to better performance and a deeper understanding of data handling.
Closure
The conclusion of this article encapsulates the vital role data structures play in Python programming. Understanding data structures is essential for individuals involved in programming, as they serve as the foundation for efficient data management and manipulation.
Summary of Key Points
In summarization, we revisited key elements discussed throughout the article:
- Importance: Data structures optimize how data is stored, allowing for faster access and modifications.
- Implementation: We covered various built-in data structures in Python, including lists, tuples, dictionaries, and sets.
- Complex Structures: The section on stacks, queues, trees, and graphs illustrated advanced concepts for managing data.
- Performance: Understanding time and space complexity aids programmers in selecting the most efficient data structures for their applications.
- Real-world Applications: The practical uses in fields such as web development and data science highlighted how crucial data structures are in solving complex problems.
Overall, having a solid grasp of data structures is crucial for anyone seeking to excel in programming and software development.
Further Reading and Resources
For those eager to dive deeper into the topic, the following resources may be beneficial:
- Wikipedia on Data Structures
- Britannica on Data Structures
- Engage with communities or forums such as Reddit for discussions and insights from peers.
- Follow pages and groups on Facebook to connect with experts in Python and data structures.
By utilizing these resources, readers can continue to build their knowledge and remain updated on the latest developments in data structures and programming techniques.