C++ Code Examples: A Comprehensive Guide
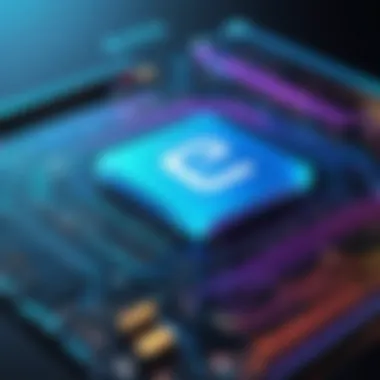
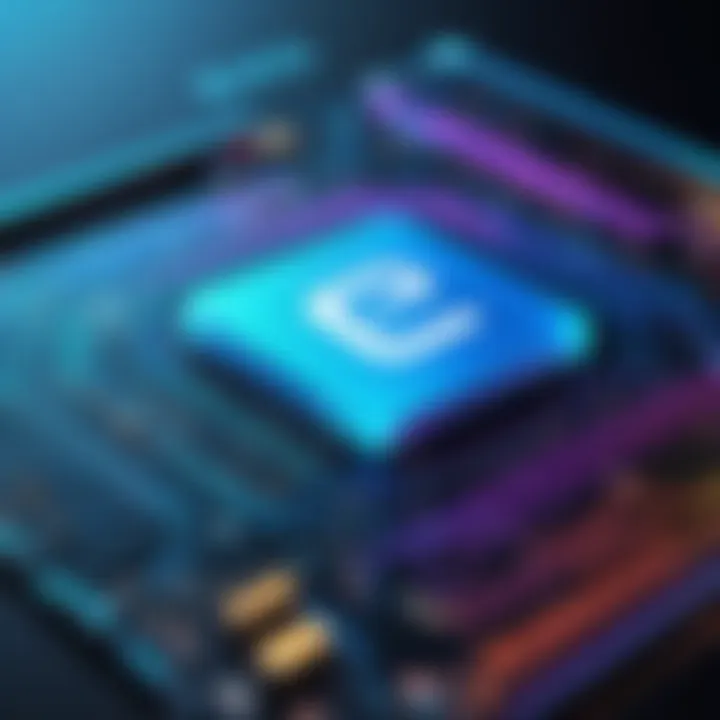
Overview of Topic
C++ is a programming language widely praised for its flexibility and performance. It has become a cornerstone in software development, influencing many modern programming languages. Its syntax may present challenges to newcomers, but the power it offers makes it worthwhile to master. The scope of this article encompasses a thorough investigation of C++ code examples, illustrating their importance in grasping both fundamental and advanced concepts.
The significance of C++ in the tech industry cannot be overstated. Many applications in system software, game development, and real-time simulation rely heavily on C++. Understanding its constructs provides programmers with the tools to develop efficient software. Over the years, C++ has evolved significantly since its inception in the 1970s. The introduction of new features, such as object-oriented programming and the Standard Template Library, has further enriched its capabilities, making it a relevant choice for many developers today.
Fundamentals Explained
C++ is built on several core principles that define its structure and function. The language supports both procedural and object-oriented programming paradigms, making it versatile for different kinds of projects.
Key terminology includes:
- Class: A blueprint for creating objects.
- Object: An instance of a class, encapsulating data and behavior.
- Inheritance: A mechanism where one class can inherit attributes and methods from another.
Basic concepts foundational to C++ include:
- Understanding data types such as , , , and .
- The use of control structures like loops and conditionals, which dictate the flow of a program.
Practical Applications and Examples
C++ finds use in various real-world applications. From game engines to operating systems, the examples are numerous. For example, the development of software for embedded systems illustrates C++โs efficiency with limited resources.
To enhance understanding, consider the following brief project example. Building a basic calculator involves:
- Creating functions for addition, subtraction, multiplication, and division.
- Taking user input and displaying results.
Here is a simple code snippet:
This simple application showcases C++ syntax while providing a practical output.
Advanced Topics and Latest Trends
Current trends in C++ include a focus on performance optimization and safety in coding, especially with the latest versions, such as C++20. New features like concepts and ranges simplify code and enhance clarity. Developers are also exploring concurrent programming, which allows code to execute simultaneously, significantly improving performance for certain applications.
The future for C++ appears bright. With constant updates and expansions, it remains a strong tool for software development. As programming needs evolve, so does C++, adapting to new paradigms and challenges.
Tips and Resources for Further Learning
For those eager to delve deeper into C++, several resources can facilitate learning. Recommended materials include:
- Books: "The C++ Programming Language" by Bjarne Stroustrup, which provides authoritative insight from the creator himself.
- Online Courses: Platforms like Coursera and Udemy offer comprehensive C++ courses suitable for all levels.
- Tools: IDEs such as Visual Studio and Code::Blocks are essential for hands-on practice.
In summary, the mastery of C++ is not just about understanding syntax; it requires grasping the principles behind programming logic and design. This guide will serve as a roadmap, enabling both aspiring and seasoned programmers to enhance their skills effectively.
Prologue to ++
The introduction to C++ serves as the foundation for comprehending both the language itself and its practical applications. C++, developed by Bjarne Stroustrup in the late 1970s, is a powerful object-oriented programming language that builds on the features of its predecessor, C. Understanding C++ is relevant not only because of its widespread use in various industries but also due to its performance efficiency and versatility. This introduction aims to equip learners with a solid framework as they delve deeper into the intricacies of the language.
History of ++
C++ has a rich history that reflects its evolution over the decades. It began as an enhancement to the C language, incorporating object-oriented features. The first edition of "The C++ Programming Language" was published in 1985, marking a significant milestone in its development. The International Organization for Standardization (ISO) ratified C++ in 1998, leading to its first standard known as C++98. Since then, updates have continued with C++11, C++14, C++17, and the latest versions focusing on making the language more efficient and easier to use. Each iteration has added features like auto keyword, smart pointers, and concurrency support.
Features of ++
C++ is distinguished by several key features that contribute to its robustness. Some notable characteristics include:
- Object-oriented programming: C++ allows for encapsulation, inheritance, and polymorphism, enabling developers to create modular and reusable code.
- Performance: It gives high-level access to low-level memory, which is beneficial for developers needing fine control over system resources.
- Standard Template Library (STL): A collection of template classes and functions that greatly simplifies common programming tasks, such as data structure manipulation.
- Type safety: C++ supports strong type-checking, which helps catch errors during compilation.
This combination of features makes C++ suitable for various applications, from game development to system-level programming.
Importance of Learning ++
Learning C++ is crucial for several reasons. First, it lays the groundwork for understanding programming concepts that are transferable to other languages. Many modern languages have adopted C++ features, making it easier to shift to languages like C# or Java. Moreover, C++ is prevalent in high-performance applications, such as financial trading systems, video game engines, and real-time computing systems, offering significant career opportunities.
Setting Up Your Development Environment
Setting up the development environment is a fundamental step for anyone looking to program in C++. This stage is not just about installing software but rather about creating a conducive platform where effective coding can take place. The right tools can significantly enhance learning, boost productivity, and enable the developer to tackle tasks with ease. Thus, understanding how to properly configure your environment is essential for success in mastering C++.
Choosing the Right IDE
An Integrated Development Environment (IDE) is a crucial tool for writing, testing, and debugging code efficiently. There are numerous IDEs available for C++, each with different features tailored to various preferences and needs. Popular choices include Microsoft Visual Studio, Code::Blocks, and Eclipse.
When choosing an IDE, consider the following:
- User Interface: Look for an IDE that is intuitive and user-friendly. A complex interface can hinder productivity, especially for beginners.
- Customizability: The ability to customize the IDE to fit personal coding styles can enhance the coding experience.
- Debugger Support: A powerful debugger is essential for identifying and fixing errors in your code. Ensure the IDE has robust debugging tools.
- Community Support: An IDE with a strong community can be beneficial for accessing resources, tutorials, and troubleshooting assistance.
With the right IDE in place, programmers can streamline their coding processes, making it easier to write high-quality C++ code.
Installing a Compiler
A compiler translates C++ code into machine language, enabling the computer to execute the code. Installing a good compiler is critical for running C++ programs successfully. Popular compilers include GCC (GNU Compiler Collection) and Clang.
Here are the considerations for installing a C++ compiler:
- Compatibility: Ensure that the compiler is compatible with the OS you are using, whether it be Windows, macOS, or Linux.
- Installation Method: Some compilers come bundled with IDEs, while others may need to be downloaded separately. It's essential to follow the installation instructions closely to avoid issues.
- Configuration Path: After installation, you may need to set environment variables or path settings, so the IDE can locate the compiler properly.
A properly installed compiler allows the seamless running of C++ code and is vital for effective development.
Creating Your First ++ Project
Creating your first C++ project signifies an important milestone in your programming journey. This task involves setting up a project within your IDE, writing simple code, and successfully compiling it into an executable program.
Hereโs a simple guide to create a C++ project:
- Open Your IDE: Launch the Integrated Development Environment you have chosen.
- Start a New Project: Look for an option like โCreate New Projectโ. Most IDEs have templates to help you get started.
- Set Project Properties: Configure your project settings, such as project name, path for files, and language settings.
- Write Sample Code: A simple program could be to print "Hello, World!" to the console. Here is a basic example:
- Build and Run: Once you have written your code, look for the build or run option in your IDE. This compiles and executes the program.
This straightforward process will help you familiarize with the development environment and serves as the foundation for more complex applications in C++. Excitement and curiosity mark the beginning of a programmer's journey. With C++, those initial steps can lead to profound mastery of a powerful language.
Basic Syntax and Structure
Understanding the basic syntax and structure in C++ is crucial for anyone looking to dive into programming with this powerful language. The way commands are written, the rules governing syntax, and the hierarchies of code all form the foundational building blocks. Familiarity with these aspects greatly enhances a programmer's ability to write clear, efficient, and error-free code. This section will delve into three key subtopics: understanding C++ syntax, handling basic input and output, and grasping the concept of variables and data types.
Understanding ++ Syntax
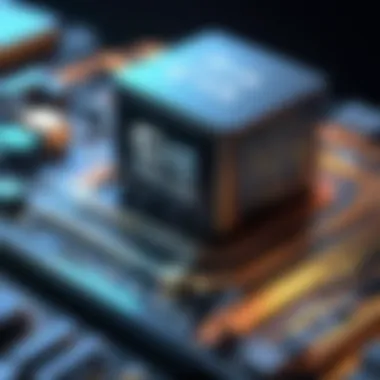
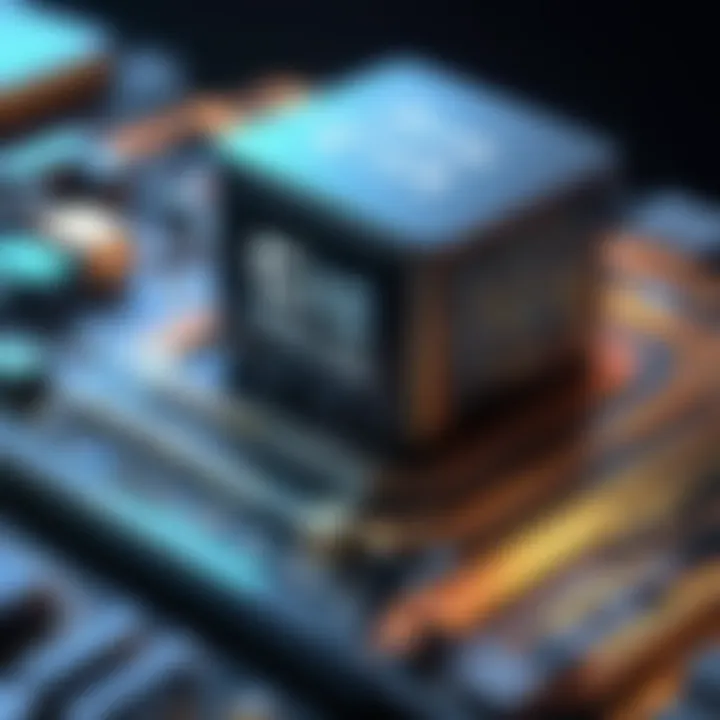
The syntax of C++ can be seen as the grammar of programming. Just like any language, C++ has its own set of rules that dictate how code must be written. Understanding this is vital because even minor deviations can lead to errors that hinder program functionality.
C++ syntax is largely influenced by its predecessor, C, while also incorporating features from other languages. Key elements include:
- Statements: Each line of code that performs an action ends with a semicolon.
- Case sensitivity: Variable names must be used with the correct casing.
- Comments: Annotations in the code using for single line or for multi-line comments help improve readability.
These elements ensure that the written code communicates effectively with the compiler. A beginnerโs understanding of C++ syntax can facilitate a smoother transition into more advanced programming concepts.
Basic Input and Output
In C++, input and output operations are the pathways through which programs communicate with the user or other systems. Standard Input Output Library makes this possible. Understanding how to receive input and display output efficiently is foundational in constructing interactive applications.
- The simplest way to display information is through cout, which is used to print data to the console. Example:
- For user input, cin is employed. This captures data from the console. An example is:
Using these functionalities effectively allows a programmer to create flexible and user-friendly applications.
Variables and Data Types
Variables are the storage units for data within a program. In C++, a programmer must declare variables with specific data types before use. These data types determine the kind of data a variable can hold.
Common data types in C++ are:
- int: Holds integer values.
- float: Represents floating-point numbers.
- char: Stores single characters.
- double: Contains double-precision floating-point values.
Understanding variables and data types is fundamental, as it impacts operations, storage allocation, and overall performance of the program. In addition, proper use of variable types can prevent issues related to precision or data loss.
Understanding the basic syntax and structure in C++ is not just beneficial; it is essential for code clarity and effectiveness.
This section sets the groundwork for more complex programming tasks. Grasping basic syntax, input and output handling, and variable management are key to progressing in C++. These concepts lead to more sophisticated C++ applications and reinforce good programming practices home mastery of the language.
Control Structures
Control structures play a critical role in programming by determining the flow of control within a program. They enable developers to dictate conditions under which certain parts of code execute, ensuring that programs behave as intended. Mastery of control structures is essential for any programmer, as they form the backbone of logical decision-making in code. In C++, control structures help in implementing complex algorithms and managing the paths taken by the execution based on various criteria. They enhance clarity, maintainability, and efficiency of the code.
Conditional Statements
Conditional statements are fundamental in C++. They allow the program to execute certain sections of code based on specific conditions. The most common form is the statement. For instance:
In addition to , C++ includes and constructs. This flexibility lets programmers create sophisticated branching logic. A simple example involving a temperature check could look like this:
This code structure underscores how control can vary based on conditions evaluated at runtime. Moreover, conditional statements enhance program decision-making capabilities, and learning to use them effectively is crucial for writing functional and responsive software.
Loops in ++
Loops are essential for executing blocks of code repeatedly under specified conditions. The primary types of loops in C++ include , , and . Understanding loops allows developers to automate tasks, handle collections of data, and work efficiently.
loop example:
This example prints numbers from 0 to 9.
The loop executes its block as long as a condition remains true, making it suitable for situations where the number of iterations is not known in advance:
Lastly, the loop ensures that the loop body is executed at least once before checking the condition:
Efficient use of loops allows programmers to handle repetitive tasks with efficiency. The choice of loop type often depends on the specific use case, whether it be a definite number of iterations or a condition-driven process.
Switch Statements
statements provide an alternative to multiple conditions when you are evaluating a variable against a series of constants. This allows for cleaner code when one value requires multiple comparisons, enhancing readability and maintenance.
An example of a switch statement in C++ is shown below:
In this structure, the program evaluates against each case. The use of statements is critical; without them, the program would continue to execute subsequent cases until a break is encountered or the switch ends. The syntax of switch statements can often lead to faster decisions than numerous statements, particularly when evaluating multiple conditions.
Functions in ++
Functions are a fundamental construct in C++, allowing for code organization, reuse, and modular programming. They serve as building blocks that encapsulate specific tasks, making the code easier to read and maintain. Understanding functions is essential for any programmer aiming to master C++. This section will delve into defining functions, exploring function overloading, and examining recursion, all of which are vital to writing effective and efficient C++ code.
Defining Functions
In C++, a function is defined by specifying its return type, name, and parameters. The basic syntax of a function looks like this:
Return type indicates what type of value the function will return, which can be void if no value is returned. Function name identifies the function and must be unique within the same scope. Parameter list is optional; it defines the type and number of inputs the function requires.
Defining functions enhances code clarity. Rather than writing repetitive blocks of code, a programmer can define a function once and call it multiple times. This leads to more concise and readable code. For example:
In this example, the function takes two integers as parameters and returns their sum. Understanding how to define and use functions is crucial for building more complex applications.
Function Overloading
Function overloading allows multiple functions to have the same name but differ in the type or number of parameters. This is useful for creating functions that perform similar tasks with varying input types or quantities, improving code usability. For instance, you can have one function to add two integers and another to add two floating-point numbers, all under the same name:
Here, both functions share the name , but the compiler determines which function to execute based on the arguments passed during the call. This feature promotes cleaner and more intuitive code, making it easier for other developers (or yourself) to understand the function's purpose and usage at a glance.
Recursion in Functions
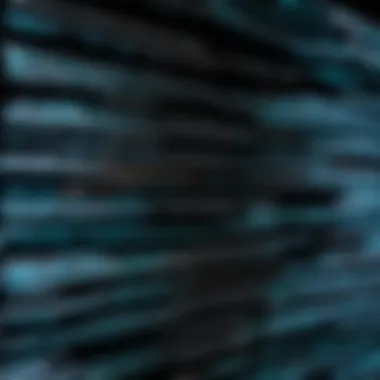
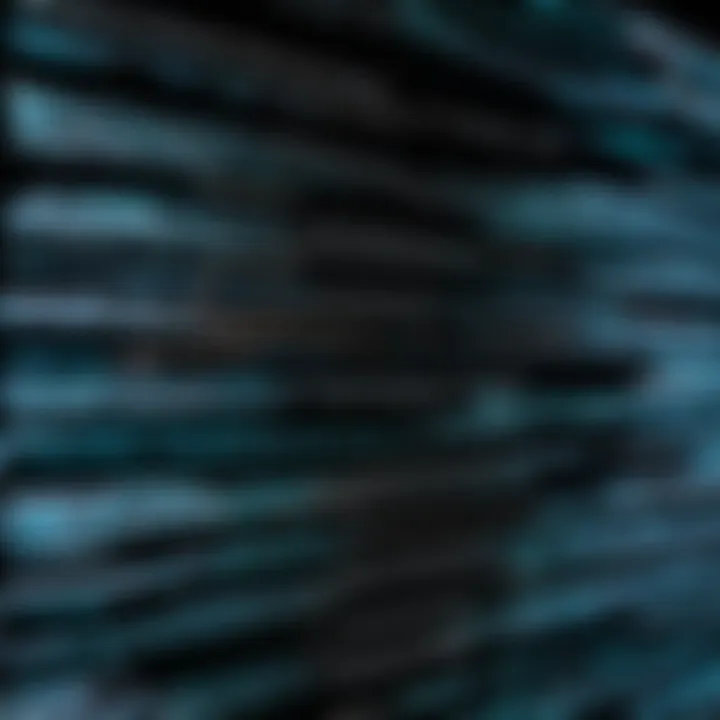
Recursion is a programming technique where a function calls itself to solve smaller sub-problems. This is particularly useful for problems that can be divided into simpler, similar problems. When implementing recursion, it is essential to define a base case to prevent infinite recursion and potential stack overflow.
A simple example of recursion is the calculation of factorial:
In this case, if is less than or equal to 1, the function returns 1, terminating the recursive calls. For any other positive integer, the function multiplies by the result of calling itself with . Recursion can lead to elegant solutions but requires careful management of base cases and recursive depth.
Recursion can simplify the solution to complex problems, but it is vital to understand when to use it instead of iterative approaches.
Object-Oriented Programming
Object-Oriented Programming (OOP) is a fundamental paradigm in software development that significantly enhances the way C++ is utilized. Understanding OOP is crucial for building scalable, efficient, and maintainable software. This approach allows developers to model real-world entities in code, handling complexity in a structured way. Key concepts of OOP include classes, objects, inheritance, and polymorphism. Each of these plays a significant role in the development process.
Classes and Objects
At the core of C++ OOP are classes and objects. A class is a blueprint for creating objects. It encapsulates data for the object and methods to manipulate that data. An object is an instance of a class. For instance, consider a class called . It might have properties like , , and , along with methods such as or .
Example:
Creating an object of this class allows you to use its properties and methods. This encapsulation is a major benefit, as it helps with organization and reduces chances of errors in large codebases.
Constructor and Destructor
Constructors and destructors are special member functions in a class. A constructor is invoked when an object of a class is created. It initializes the object's properties. Similarly, a destructor is called when an object goes out of scope, allowing for resource cleanup. Understanding how to leverage these functions is vital for memory management in C++. This is particularly important in scenarios where resources like dynamic memory, file handles, or network connections are involved.
Example of a constructor and destructor:
Inheritance and Polymorphism
Inheritance allows a class to inherit properties and methods from another class, promoting code reuse. C++ supports single and multiple inheritance. Polymorphism, on the other hand, enables a single interface to represent different underlying forms (data types). It is mainly achieved through function overriding.
A common example of inheritance could be a base class called , with derived classes and . This allows both classes to share common functionalities while maintaining their unique features.
To summarize:
- Inheritance supports code reuse and establishes relationships between classes.
- Polymorphism allows methods to do different things based on the object calling them.
Understanding OOP in C++ is essential for those seeking a robust approach to programming. Its organized structure is advantageous in both small projects and large systems, optimizing development and improving adaptability.
Advanced ++ Features
Advanced features in C++ play a critical role in enhancing the programming experience and performance of applications. They go beyond basic syntax to offer powerful tools and techniques that enable developers to write more efficient and robust code. Understanding these features is essential for maximizing the language's capabilities. They also have implications for code quality, maintainability, and performance.
Templates in ++
Templates are a cornerstone of C++ that allow for generic programming. They facilitate writing functions and classes that work with any data type. By using templates, a developer can create code that is both flexible and reusable without sacrificing performance. This feature is particularly useful in scenarios where the operations performed are independent of the specific data types.
Some key benefits of templates include:
- Code Reusability: Templates enable developers to define functions or classes once and use them with various data types without rewriting code.
- Type Safety: The template mechanism ensures type safety at compile time, reducing the potential for runtime errors.
- Efficiency: Templates are instantiated at compile-time, meaning there is little to no performance overhead during execution.
This example illustrates a simple template function that adds two values of any type. Using templates can significantly streamline codebases by minimizing duplication.
Exception Handling
Exception handling is a crucial feature in C++ that provides a mechanism for managing errors and exceptional situations during program execution. This feature allows developers to separate error-handling code from regular code, leading to cleaner and more understandable programs. C++ uses , , and keywords to facilitate this.
The benefits of effective exception handling include:
- Improved Reliability: Handling exceptions can prevent a program from crashing unexpectedly and allows it to continue running in a controlled manner.
- Enhanced Error Reporting: It gives developers detailed information about the nature of errors, making debugging easier.
- Separation of Concerns: Business logic can remain unencumbered by error-handling code, leading to better-organized code.
An example of exception handling in C++ can be seen below:
This code snippet demonstrates throwing and catching an exception, showcasing how manageable error handling can be.
Smart Pointers
Smart pointers are a sophisticated feature of C++ that implement automatic memory management, thus helping to prevent memory leaks and dangling pointers. They serve as an alternative to raw pointers by managing the lifetime of dynamically allocated objects. The standard library provides several types of smart pointers, including , , and .
Utilizing smart pointers presents various advantages:
- Automatic Resource Management: Smart pointers automatically deallocate memory when they go out of scope, preventing memory leaks.
- Shared Ownership: allows multiple pointers to own the same memory, simplifying memory management in complex data structures like graphs or shared resources.
- Reduction of Errors: Using smart pointers can reduce common programming errors related to raw pointer management, such as double deletion.
Here is a basic example of using :
This example illustrates how unique pointers manage memory automatically, highlighting their utility in modern C++ programming.
Advanced features in C++ such as templates, exception handling, and smart pointers not only enhance the programming capabilities of the language but also contribute to improved code quality and maintenance. Understanding and utilizing these features is vital for any C++ developer.
Standard Template Library
The Standard Template Library (STL) is a crucial aspect of modern C++ programming. It provides standardized templates for data structures and algorithms, making programming in C++ more efficient and less error-prone. STL is designed to enhance productivity and code quality, which is vital for both beginners and experienced programmers. By leveraging the STL, developers can focus on solving problems instead of wrestling with common data handling tasks.
STL primarily consists of three components: containers, iterators, and algorithms. These components work together seamlessly, enabling developers to handle data in versatile ways. The importance of understanding STL cannot be overstated; it forms the backbone of modern C++ development, promoting better coding practices.
"The STL allows you to write code that is not only easier to manage but also more scalable and resilient."
Overview of STL
The STL can be viewed as a collection of classes and functions that provide general-purpose solutions to common programming challenges. The main goal of STL is to enable code reuse. With its rich set of features, developers can implement sophisticated data structures like linked lists, stacks, queues, and maps without reinventing the wheel. Understanding the STL can lead to significant efficiencies in development cycles, thereby enhancing the overall productivity. Elements like templates allow for type safety while maintaining flexibility in the code design.
Containers in STL
Containers are data structures used to store collections of objects. STL provides various types of containers, each serving a distinct purpose:
- Vector: Implements a dynamic array, allowing for random access and automatic resizing.
- List: A doubly linked list that supports fast insertions and deletions at both ends.
- Deque: A double-ended queue, offering the ability to insert and remove items from both ends.
- Set: A collection of unique elements, automatically sorted.
- Map: A collection of key-value pairs that maintains sorted order based on keys.
By choosing the right container for the task, programmers can optimize performance and memory usage significantly.
Iterators and Algorithms
Iterators are a key feature of the STL that allow for traversal through containers. They provide a unified way to access the elements stored in various types of containers without exposing the underlying details.
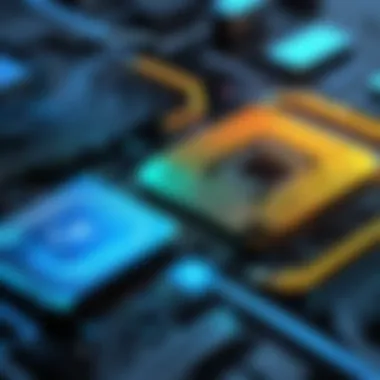
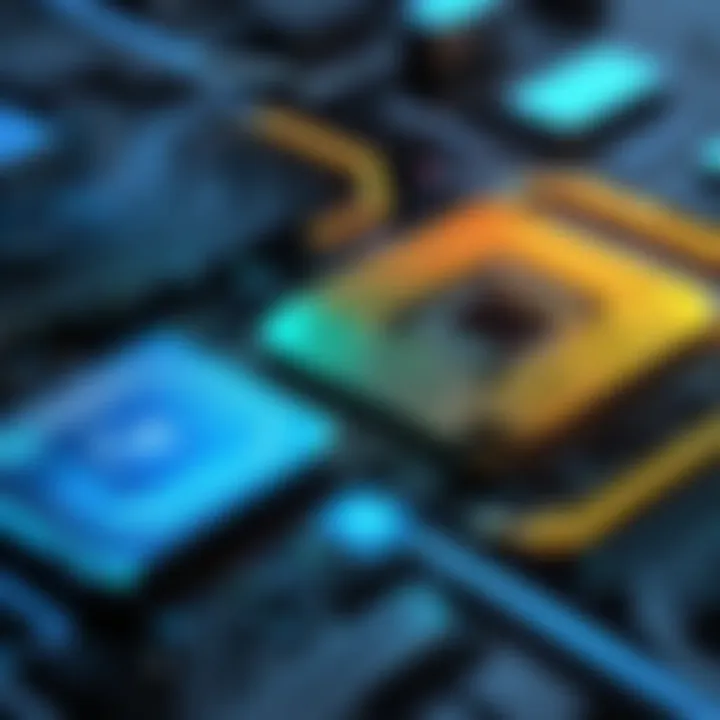
Common types of iterators include:
- Input Iterators: Allow reading elements from a sequence.
- Output Iterators: Allow writing elements to a sequence.
- Forward Iterators: Can traverse a sequence in one direction.
- Bidirectional Iterators: Can traverse both forward and backward.
- Random Access Iterators: Allow access to any element within the container in constant time.
STL further includes a wealth of algorithms that can be applied to containers, such as sorting, searching, and transforming. For instance, you can apply the algorithm to a vector to arrange its elements in ascending order. Hereโs a quick example:
In this example, the function is applied to the vector of integers, demonstrating how STL simplifies complex operations. Understanding STL's iterators and algorithms is essential for efficient programming in C++.
Overall, integrating STL into daily programming workflows can greatly enhance a developer's efficiency and code maintainability.
Debugging and Optimization Techniques
Understanding debugging and optimization is essential for anyone working with C++. These techniques can greatly enhance both the efficiency of your code and your ability to resolve issues when they arise. Debugging is the process of identifying and resolving bugs or issues within your program. Optimization involves refining your code to make it more efficient in terms of performance, speed, and resource use. Together, these practices play a crucial role in programming, ensuring that software runs smoothly and efficiently.
Debugging Tools
Effective debugging tools are vital for any programmer. They help to isolate problems and provide insights into code behavior. Here are some popular debugging tools you may consider using in conjunction with C++:
- GDB (GNU Debugger): A powerful debugger that lets you examine what is happening inside a program while it executes or what it was doing at the moment it crashed.
- Valgrind: This tool is instrumental for detecting memory leaks and memory management issues.
- Visual Studio Debugger: If you're using Visual Studio IDE, it has built-in debugging capabilities that allow you to step through your code, inspect variables, and even modify them on the fly.
By using these tools, you can perform some common tasks, such as:
- Set breakpoints to pause execution at a specific point.
- Step through code line by line to observe state changes.
- Examine the call stack to track function calls.
- Monitor variable values in real time.
Debugging is a systematic process, and utilizing proper tools ensures that you identify issues quickly.
Effective debugging leads to a smoother user experience and more robust applications.
Performance Optimization
Optimizing C++ code can take various forms, and the goal is to improve performance while maintaining clarity and functionality. Here are some strategies for optimizing your C++ code:
- Improve Algorithm Efficiency: Analyze your algorithms. Ensure that you are using the most efficient algorithm for your data. Sometimes, changing from O(n^2) to O(n log n) can have dramatic effects.
- Minimize Memory Usage: Keep an eye on how much memory your program is using. Use dynamic memory allocation wisely and consider data structures that fit your needs without wasting memory.
- Use Inline Functions: If you have small, frequently called functions, defining them as inline can reduce the overhead of a function call.
- Enable Compiler Optimization Flags: Use compiler settings designed to optimize your code for performance during the build process. Flags like or can apply several optimizations.
Additionally, profiling tools such as gprof or profiling features in IDEs help identify bottlenecks in your code. By systematically evaluating performance, you can make informed choices about what changes will have the most significant impact on efficiency.
In summary, mastering debugging and optimization techniques is critical for anyone who wants to become proficient in C++. A deep understanding of these areas leads to more dependable and performant applications. Learning to leverage tools effectively ensures that you minimize issues while maximizing performance.
Real-World ++ Applications
C++ plays a significant role in various fields of software development. Its ability to combine high-level abstraction with low-level memory control makes it a powerful tool for many applications. Understanding these real-world applications can provide insights into the language's design and utility. C++ is used extensively in systems programming, game development, and high-performance applications. Each of these areas requires specific features from the language that cater to the needs of developers.
++ in Game Development
Game development is one of the most notable areas where C++ shines. Several popular game engines, including Unreal Engine and CryEngine, are built using C++. The language's performance is critical in gaming, where speed and efficiency can directly impact user experience.
The use of C++ allows developers to optimize resource management and memory allocation, leading to smoother graphics rendering and more responsive gameplay. Furthermore, C++ supports Object-Oriented Programming, which facilitates the creation of complex game systems where actors, environments, and user interactions can be modeled intuitively.
"The choice of programming language significantly influences game development efficiencies and creativity. C++ leads this with its sophisticated design."
Key aspects of using C++ in gaming include:
- Performance: C++ provides low-level control, allowing for efficient graphics and physics calculations.
- Portability: Games developed in C++ can be ported to multiple platforms with minimal adjustments.
- Community and Libraries: A robust ecosystem of libraries and frameworks is available to enhance game development processes.
++ in Systems Programming
C++ is also widely used in systems programming. This field entails developing operating systems, embedded systems, and hardware interface code. The language's proximity to hardware makes it suitable for this environment. It allows developers to write code that directly interacts with system resources, delivering optimal performance.
Key features that make C++ a suitable choice include:
- Control Over System Resources: C++ offers low-level manipulation capabilities required in systems programming.
- Efficiency: The languageโs performance is crucial for tasks requiring high speed and low latency.
- Library Availability: Libraries like Boost provide extensive functionality that can simplify systems programming.
++ in High-Performance Applications
High-performance applications cover various domains requiring real-time processing, such as financial systems, scientific computing, and telecommunications. In these situations, performance is paramount. C++ is often adopted for its efficiency and performance optimization capabilities.
The features of C++ that cater to high-performance applications include:
- Memory Management: C++ offers fine-grained control over memory, which is crucial in performance-sensitive applications.
- Concurrency Support: With support for multi-threading, C++ helps in optimizing CPU resource usage.
- Compilers and Optimization: Modern C++ compilers provide advanced optimization techniques that can significantly improve execution speed.
By understanding these real-world applications, developers can appreciate why C++ remains a vital language in programming circles. This knowledge can inform better design choices and lead to more effective and efficient coding practices.
Best Practices and Coding Standards
Best practices and coding standards are essential aspects of software development. They ensure consistent coding styles, improve readability, and reduce errors in C++ programming. This section delves into the significance of these practices while also outlining effective strategies for maintaining coding quality.
Writing Clean Code
Writing clean code is about making code readable and maintainable. Clean code reduces the likelihood of errors and facilitates collaboration among developers. Here are some key elements to consider:
- Meaningful Names: Variables, functions, and classes should have descriptive names. This helps anyone reading the code to quickly understand its purpose.
- Consistent Formatting: Use a standard format for spacing, indentation, and line breaks. Consistency makes the code appear organized and easier to navigate.
- Commenting Wisely: Comments should explain why something is done, not just what the code does. Avoid excessive commenting; instead, write self-explanatory code where possible.
- Function Size: Keep functions focused. A function should accomplish one task. This limits complexity and enhances readability.
By adhering to these principles, developers create code that not only functions correctly but also is easy to understand and modify.
Version Control Systems
Version control systems (VCS) are tools that help manage changes to code over time. They are crucial for collaborative projects. Here are some benefits of using version control in C++ development:
- Track Changes: Developers can track every change made to the code, which simplifies debugging and understanding the evolution of a project.
- Collaboration: Multiple developers can work on the same codebase without overwriting each other's changes. Tools like Git enable branching and merging, allowing independent work streams.
- Rollback Capabilities: If a change causes issues, developers can revert to previous versions of the codebase, minimizing potential disruptions.
- Documentation of Changes: Version control automatically documents changes, providing a historical account that can be useful for audits and understanding project milestones.
Employing a version control system like Git is an effective way to implement best practices in software development. It not only aids individual developers but fosters teamwork in larger projects as well.
"Investing time in writing clean code and using version control will pay off in reduced bugs and improved collaboration in the long run."
By integrating coding best practices and version control into C++ development, programmers can significantly enhance code quality. The benefits translate not only to better functionality but also to a smoother workflow for developers.
Epilogue
The conclusion serves as a crucial part of any comprehensive guide. It acts as a final reflection on the main themes and principles covered throughout the discussion. In this case, it reinforces the fundamental concepts of C++ and how they interconnect with real-world applications. A well-structured conclusion helps consolidate the information presented, ensuring that readers leave with a clear understanding of the subject.
In summarizing the key points, it emphasizes the importance of mastering both the basic syntax and advanced features of C++. This not only aids in building a solid foundation but also enriches the coding experience. Additionally, it invites readers to reflect on their learning journey, encouraging them to apply their knowledge to practical scenarios.
Through a concise summation, the conclusion highlights the benefits of acquiring skills in C++. These include job prospects, the ability to develop complex systems, and the significant foothold C++ maintains in various sectors. Overall, the conclusion ties together previous sections, providing a platform for future exploration.
Summary of Key Points
- C++ is a versatile programming language applicable in various domains, such as game development and systems programming.
- Understanding basic syntax and control structures is essential for effective programming.
- Object-oriented programming principles, including classes, inheritance, and polymorphism, are foundational to C++.
- Advanced features like templates and smart pointers enhance code efficiency and safety.
- The Standard Template Library offers useful tools for managing data structures and algorithms.
Further Learning Resources
For those looking to deepen their knowledge of C++, here are some valuable resources:
- Wikipedia - C++ offers an overview and historical context.
- Britannica - C++ provides a focused summary of C++ as a programming language.
- Participating in discussions on forums such as Reddit's C++ Community can enrich your understanding through shared experiences and expert insights.
- Following updates and engaging with relevant posts on Facebook can also facilitate community interaction and continued learning.