Master JavaScript: The Complete Guide for Developers
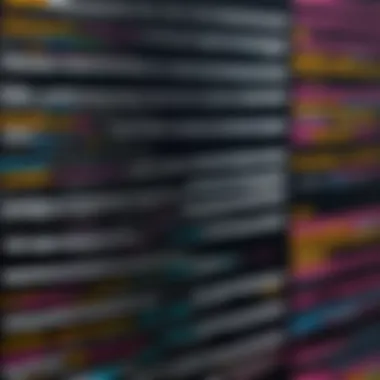
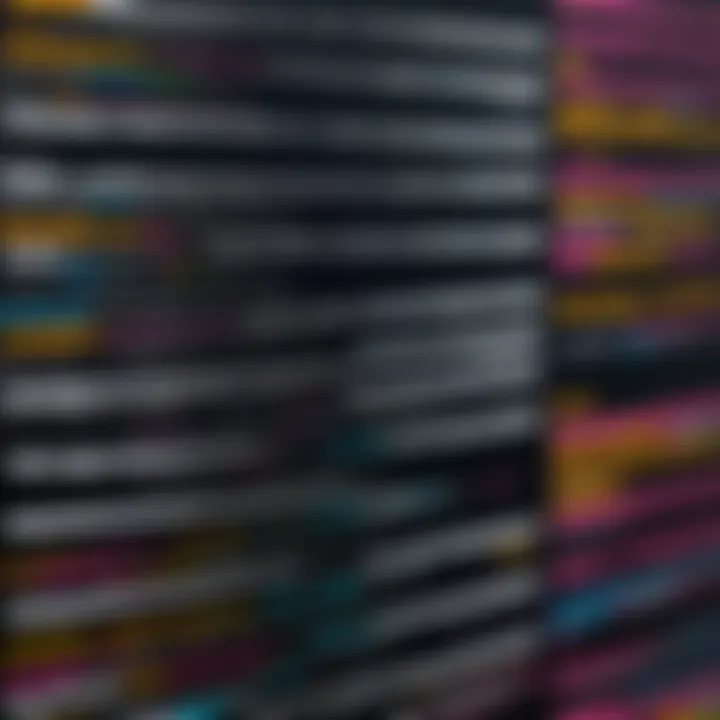
Intro
JavaScript is a programming language that has become indespensible in modern web development. Its ubiquitous presence on the web caters to a nummber of functionalities that range from simple form validation to the creation of complex web applications. As technology continues to evolve, JavaScript remains at the forefront, fostering innovations and enhancing user experiences.
In this tutorial, we will explore JavaScript from its core principles to advanced methodologies. Whether you are a complete beginner or a seasoned IT professional seeking to brush up on advanced techniques, this guide presents a pathway for all skill levels.
Overview of Topic
Prelims to the main concept covered
JavaScript serves as a vital tool among developers. Since it allows manipulation of content on websites dynamically, it transforms static pages into interactive experiences for users. JavaScript operates primarily in webpages but is also finding uses beyond traditional web development, like in server-side environments via Node.js.
Scope and significance in the tech industry
Given its growing critical role in almost every aspect of web design and development, understanding JavaScript is essential for anyone in the tech industry today. From enhancing the functionality of e-commerce platforms to developing APIs, JavaScript skills can considerably augment an IT professional's portfolio.
Brief history and evolution
The journey of JavaScript began in 1995 when Brendan Eich introduced it as a client-side programming language. Over the years, it has evolved significantly, giving rise to frameworks like Angular, React, and Vue.js, which simplify the development process and enhance productivity.
Fundamentals Explained
Core principles and theories related to the topic
At its essence, JavaScript follows specific principles. It is a prototype-based, object-oriented language that utilizes functions as first-class objects. Understanding these foundational theories is crucial as it alters how developers approach programming in JavaScript.
Key terminology and definitions
Key terms that are essential to grasp include:
- Variable: Named storage for data values.
- Function: A block of code that can be called and executed when needed.
- Event: An action that occurs in the browser, which JavaScript can interact with.
Basic concepts and foundational knowledge
Knowing how to write flexible and readable code is fundamental. Establishing simple variables and employing operators gives one a foothold in preparing for more complex subject matters in JavaScript. Leveraging control structures, such as loops and conditionals, further enhances your coding capabilities. Understanding Arrays and Objects, which are two vital structures in JavaScript, serves as building blocks for more intricate functionalities.
Practical Applications and Examples
Real-world case studies and applications
Various case studies demonstrate JavaScript's effectiveness. For instance, major websites, including Facebook, rely extensively on JavaScript for seamless user interactions and responsiveness.
Demonstrations and hands-on projects
Learners can create mini web applications to solidify their understanding. One such project could be designing a simple to-do list application that allows users to add, remove, and view tasks dynamically, a result that emphasizes JavaScript's practical value.
Code snippets and implementation guidelines
Here is an example code snippet illustrating a basic function:
This function accepts a name as a parameter and returns a greeting string. Utilizing such simple function calls leads to greater application development over time.
Advanced Topics and Latest Trends
Cutting-edge developments in the field
With libraries and frameworks proliferating, the development landscape continues to shift. ES6, which introduces new functionalities, such as arrow functions and classes, has equipped developers with more powerful tools to craft elegant solutions.
Advanced techniques and methodologies
Mastering asynchronous programming methods like Promises and async/await is crucial for managing operations that should be non-blocking. Utilizing these patterns helps tackle web application complexity, ensuring smooth performance without under-releasing the user interface.
Future prospects and upcoming trends
The future of JavaScript sublime up with continuous evolution. Technologies like Artificial Intelligence will intertwine with JavaScript, suggesting an increasing need for developers skilled in the language. Moreover, frameworks will likely evolve, focusing on better solutions for state management and performance optimizations.
Tips and Resources for Further Learning
Recommended books, courses, and online resources
To supplement this guide, consider referring to:
- Eloquent JavaScript by Marijn Haverbeke
- JavaScript: The Good Parts by Douglas Crockford
- Online platforms like freeCodeCamp.org, MDN, and Codecademy offer countless tutorials and coding exercises to help improve your skills.
Tools and software for practical usage
Developer tools such as Visual Studio Code or Atom become user-friendly environments to implement and practice JavaScript. Additionally, browser developers' tools provide an avenue for real-time script debugging and testing, fostering an effective learning process as users gain confidence in their skills.
Understanding JavaScript opens the doorway to building interactive, feature-rich applications that pave the path for a brighter career in technology.
Preface to JavaScript
JavaScript is a fundamental part of web development. Its significance cannot be overstated. This section provides a solid entrance into the world of JavaScript, equipping readers with essential knowledge they need. Understanding this language lays the groundwork for mastering advanced topics in the tutorial.
What is JavaScript?
JavaScript is a dynamic programming language commonly used for web development. It is an interpreted language, enabling users to execute code within a web browser without prior compilation. Initially created to provide interactivity in websites, JavaScript has evolved into a multifaceted language used not only for frontend but also backend development. Its syntax resembles that of other languages like C and Java, making it relatively easy for developers familiar with those languages to learn.
JavaScript's versatility allows it to facilitate varied functionalities, from form validations to complex animations in user interfaces. Today, it can even be run outside the browser using environments like Node.js. After grasping its main definition, the reader should recognize the importance of JavaScript throughout the evolving landscape of technology.
History of JavaScript
JavaScript was born in 1995 when Brendan Eich developed it during his tenure at Netscape Communications. Initially named Mocha, the language quickly underwent rebranding and was introduced as LiveScript before finally being named JavaScript. This name change was part of a marketing strategy, intended to leverage the rising popularity of Java.
In 1996, Netscape and Sun Microsystems submitted JavaScript to ECMA International, leading to standardized versions of the language. The first edition was published in 1997 known as ECMAScript. Today, JavaScript continues to be updated with new features regularly, further enhancing its capabilities and performance.
The historical context adds layers to JavaScript's story. Understanding these origins helps developers see how the language grew along with technology trends and how it has molded into the sophisticated tool used today.
Importance of JavaScript in Web Development
JavaScript plays a vital role in modern web development. It enables the creation of interactive websites, allowing developers to build seamless user experiences. This programming language transforms static web pages into lively applications. Furthermore, the emergence of JavaScript frameworks and libraries like React, Angular, and Vue.js has amplified its value.
Some key reasons for the significance of JavaScript are:
- Mobility: The language is not confined to any platform, meaning it works effectively across multiple operating systems and devices.
- Ecosystem Variety: The widespread community continually produces tools and libraries that assist developers in long-term maintenance and integration.
- Responsiveness: It allows the implementation of real-time updates to the web applications, enhancing user satisfaction and retention.
Considering the information disclosed here, it is evident that JavaScript is not only relevant but essential in web development. Moving forward, readers who grasp these concepts will find later sections easier to understand, providing them both perspectives and skills needed to master the language.
Core Concepts of JavaScript
Understanding the core concepts of JavaScript is crucial for anyone entering the world of web development. These concepts form the foundation of how the language operates and how one can effectively use it to create dynamic web applications. Concepts like variables, data types, operators, and control structures define the behavior of JavaScript code. Grasping these elements will enhance a programmer’s ability to write efficient and effective code, which is a must in today's competitive job market.
Variables and Data Types
Variables are an essential building block in JavaScript. They allow programmers to store, manipulate, and retrieve data in their applications. A variable is simply a name assigned to a value, and JavaScript uses the , , and keywords to declare these variables.
When defining a variable, one must understand data types. JavaScript currently supports seven core data types:
- Undefined
- Null
- Boolean
- Number
- BigInt
- String
- Object
Each type serves a different purpose, and knowing which type to use will help optimize memory usage and enhance performance. For instance, objects can hold multiple values and more complex entities, while values of type number can be manipulated easily for mathematical operations.
Operators in JavaScript
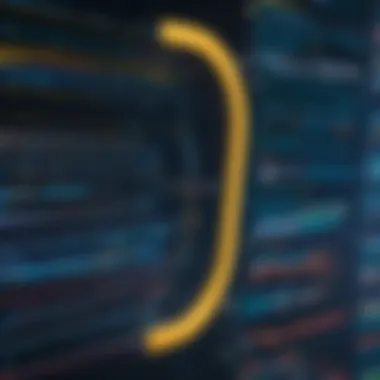
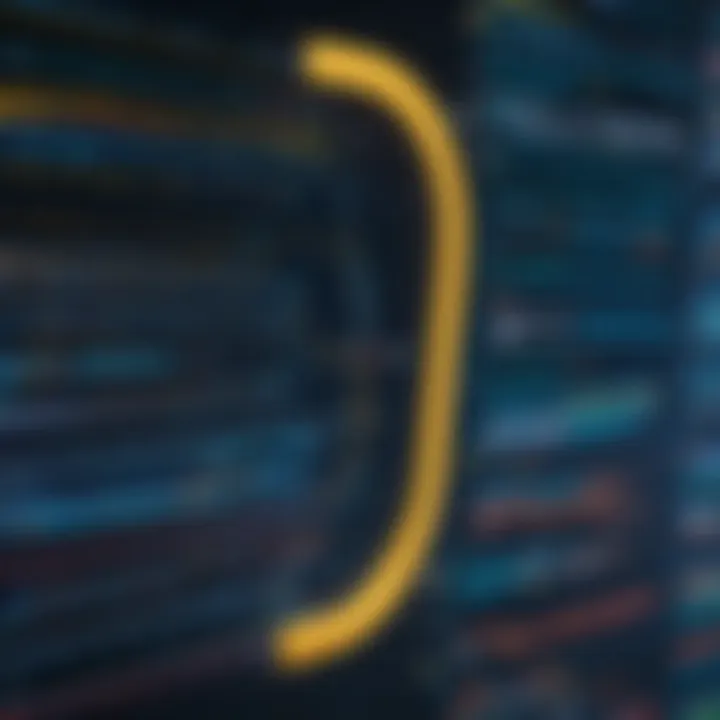
Operators serve as the machinery on which JavaScript operates. They allow for manipulation of data and implementation of logic throughout an application. Operators can be categorized into several types:
- Arithmetic Operators: Used for mathematical operations; for example, Addition (+), Subtraction (-), etc.
- Assignment Operators: Help assign values to variables; for instance, signifies assignment.
- Comparison Operators: Used to compare two values, giving back Boolean results; for instance, checks for equality.
- Logical Operators: Help control the flow of executions and logic; for example, (AND), (OR).
Each operator has specific use cases, and understanding these will improve the programmer's efficiency in controlling program flow and achieving intended results.
Control Structures
Control structures are essential for the logic and flow of any JavaScript code. They determine the way in which statements are executed. The primary types include:
- Conditional Statements: The , , and statements allow for specific code to run based on input conditions.
- Switch Statement: A control structure used to check multiple conditions efficiently.
- Loops: Structures for repeating code; examples include , , and loops. They allow for iteration over arrays or running a code block multiple times based on conditions.
In summary, daily coding tasks are streamlined by these core concepts. They in power apps with both interactivity and user-defined functionality. Familiarity with these ideas lays the groundwork as you delve into the more advanced aspects of JavaScript programming.
Functions in JavaScript
In JavaScript, functions are central to building effective and maintainable code. Functions encapsulate behavior, allowing developers to create reusable code. This utility not only reduces repetition but increases the clarity of the scripts. Understanding functions enhances one's ability to structure code efficiently. Additionally, functions can accept parameters. This enables developers to abstract complex logic, allowing for cleaner code.
Defining Functions
Defining functions in JavaScript involves using the keyword followed by the function name. The following syntax is used:
The parameters are placeholders for the values passed to the function. Functions can return values. By calling a function, you activate its code, creating modular pieces of code that contribute to the larger program. The simple structure of function definitions in JavaScript allows it to be both powerful and accessible for all skill levels.
Function Expressions
Function expressions are another way to define functions. Unlike function declarations, function expressions can be anonymous, meaning they don’t have names. They enable the assignment of functions to variables, which offers versatility in programming. An example is shown below:
Function expressions can also be used to create callbacks. This allows for higher flexibility in executing functions in response to events, making it critical in areas such as asynchronous programming and handling user interactions.
Arrow Functions
Arrow functions are a syntactically compact variant of regular function expressions. They improve the readability and conciseness of the code. An arrow function uses the syntax, which distinguishes it from traditional function expressions. Here is an example:
Arrow functions also maintain the value of from the surrounding method, avoiding confusion often encountered in object-oriented JavaScript code. This characteristic streamlines code when managing state in modern frameworks like React, establishing their significance in current development practices.
Key Takeaway: Understanding functions, whether through classical definitions, function expressions, or arrow functions, greatly enhances one’s proficiency in JavaScript programming. Each approach has its own importance and potential applications.
Working with Objects
Working with objects in JavaScript is a fundamental concept that carries significant importance in the overall structure and functionality of a program. Objects serve as containers for related data and functionality, allowing developers to model real-world entities. Understanding how to effectively work with objects will provide deeper insights and flexibility in programming.
Understanding JavaScript Objects
JavaScript objects are crucial building blocks in any JavaScript application. They enable developers to store collections of data as key-value pairs. This allows for an organized and manageable way to handle different types of information. Think of an object as a simple structure to bundle related data and functions.
For instance, consider a car object that contains properties like color, model, and year. This encapsulates all relevant details in one place. Accessing these properties is straightforward. You can refer to , , and to gather all necessary information correspondingly. This structured approach makes both reading and modification intuitive.
Object Methods and Constructors
The role of object methods cannot be overlooked. Methods are functions that associate with an object, providing behaviors associated with it. This elevates functionality by allowing actions to be defined directly on data points. For example:
In addition, constructors allow for creating multiple objects with the same structure but different properties. A constructor generally begins with a capital letter and is called using the keyword. Defining a constructor enables establishing various instances of an object efficiently, increasing code reusability and organization.
Here is an example:
Prototypal Inheritance
Prototypal inheritance is another powerful concept in JavaScript that enables objects to inherit properties and methods from other objects. This differs from classical inheritance found in other programming languages. Prototypal inheritance simplifies the extensions and intakes of objects without the need for complex class structures.
When one object inherits from another, it achieves the capability to access base functionally while preserving its unique attributes. Confusing some new learners, the trait underlies each object, linking it to another, thus creating a chain. This behavior allows optimal method sharing across objects, ensuring that all derived objects have access to the root object's properties and methods.
It is essential to grasp these concepts fully. They form the groundwork for more complex programming tasks, enhance code reusability, and ensure structured organization in approachmente. As you navigate through JavaScript, working effectively with objects will provide optimal coding efficiency.
Arrays and Loops
Arrays and loops are fundamental aspects of JavaScript programming. Mastering them is essential for anyone looking to create efficient and effective applications. Arrays are a type of data structure that allows you to store ordered collections of data. They enable you to categorize and manipulate these data efficiently. Loops, on the other hand, provide a mechanism for executing a block of code multiple times based on a certain condition. Together, they form the backbone for managing and handling data operations in JavaScript, making them pivotal in both simple scripts and complex applications.
Creating and Manipulating Arrays
Creating an array in JavaScript is quite simple. You can do this using square brackets or the constructor. For example:
Or using the constructor:
Once you have an array, you can easily manipulate its elements using methods like , , , and .
- Push adds an element to the end of the array.
- Pop removes the last element.
- Shift removes the first element.
- Unshift adds an element to the beginning.
These methods provide flexible ways to change arrays as requirements evolve throughout your application workflow.
Looping Through Arrays
Looping through arrays allows you to access each element in a structured way. Several techniques can be used in JavaScript, including the traditional loop and modern approaches like the method.
Using a for loop
The standard pattern involves initializing a variable, setting a condition, and incrementing that variable each time the loop runs:
Using forEach
The method simplifies loops. It runs a provided function on each element:
Both methods suit different scenarios, but making a choice between them often depends on personal preference and the specific use case.
Array Methods
JavaScript provides a plethora of built-in array methods. These include but are not limited to:
- : Creates a new array with the results of calling a provided function on every element.
- : Creates a new array with all elements that pass the test implemented by the provided function.
- : Applies a function against an accumulator and each element to reduce it to a single value.
Examples of array methods
Utilizing these methods enhances code simplicity and readability. Here’s how you can use and :
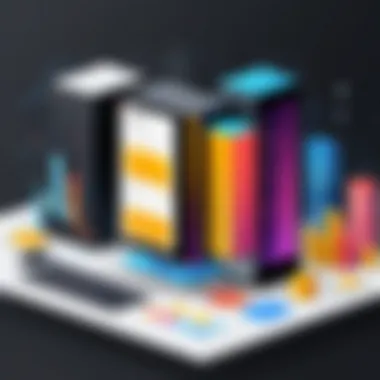
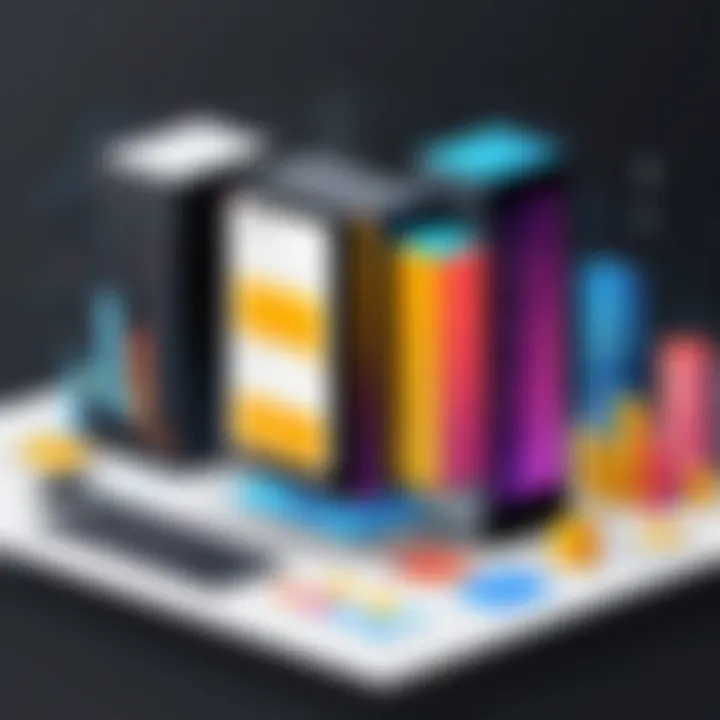
In any assessment of JavaScript programming, mastering arrays and loops is non-negotiable for efficient coding and data handling.
Asynchronous JavaScript
In an age where applications require real-time performance and responsiveness, asynchronous JavaScript stands out as an essential component of modern web development. As webpages have evolved into complex platforms driven by user interactions, real-time data handling becomes paramount. Developers often face challenges when operations take variable time, leading to less responsive interfaces. This is exactly where asynchronous JavaScript becomes critical, allowing operations to occur without blocking the user experience. This section will dissect the foundational building blocks of asynchronous programming, ultimately equipping developers with the tools to create efficient applications.
Understanding Callbacks
Callbacks serve as a primary method for handling asynchronous operations in JavaScript. At its core, a callback is a function passed as an argument to another function, which is designated to execute once a task completes. This strategy allows JavaScript to perform tasks like data fetching or event listening without halting the program’s flow. While the use of callbacks is integral, it can lead to what is commonly known as “callback hell” if relying on multiple nested callbacks to manage various asynchronous tasks.
Benefits of Callbacks
- Non-blocking execution: Callbacks ensure that the main program can continue running while waiting for operations, improving responsiveness.
- Simplicity in execution: Particularly in simpler applications, utilizing callbacks can be both straightforward and effective for handling asynchronous logic.
Considerations
While valuable, callbacks can lead to complex behavior and difficulty in code maintenance, particularly if they must chain multiple asynchronic operations. The resulting structure might become complicated and hard to read, resulting in more difficult debugging and maintenance efforts.
Promises Explained
Promises represent a more robust approach to handling asynchronous operations in JavaScript compared to callbacks. A Promise is an object that may produce a value in the future — either resolved or rejected. Promise’s clearer handling of asynchronous processes keeps the code clean and more manageable.
Key Features of Promises
- State Management: A Promise can be in one of three states: pending, resolved, or rejected.
- Chaining: Promises allow for method chaining with and handlers, simplifying error management and making the flow of asynchronous computations easier to follow. This eliminates some of the confusion associated with nested callbacks.
Example
Here’s a simple code example demonstrating a promise:
Async/Await Syntax
Async/Await builds on promises and offers a simpler, more straightforward way to write asynchronous code. The introduction of and keywords provides a way to handle promises, reducing boilerplate code and making asynchronous sequencing more intuitive.
Advantages of Async/Await
- Simplicity: Code written with often appears synchronous. This clarity results in improved readability.
- Easier error handling: By using try/catch around asynchronous code, errors are easier to troubleshoot and resolve, bringing clarity to code execution paths.
Example
Here is how the previous example can be rewritten using Async/Await:
As we can see, utilizing Async/Await converts the promise handling into clear sequential steps.
Asynchronous JavaScript plays a crucial part in improving user experience by reducing wait times and increasing efficiency in web applications. Tackling common asynchronous challenges by strategizing with callbacks, promises, or Async/Await equips developers with deeper insights.
JavaScript in the Browser
JavaScript's role in the broweser is central to the dynamic nature of web applications. It enables responsive user interaction, modifies HTML and CSS, and enhances the overall functionality of web pages. Understanding sham resort of broad JavaScript complement the validity of the interactive experience end users have come to expect. Here, we will explore its core elements, advantages it offers, and essential consideration one needs to have when developing projects in the real time client environment.
DOM Manipulation
The Document Object Model, commonly referred to as the DOM, is a programming interface provided by the browser for HTML and XML documents.ating JavaScript offers the ability to dynamically change the content, structure, and style of pages. With a clear understanding of DOM manipulation, developers gain precise control over webpage elements.
In JavaScript, several methods are available to interact with the DOM:
- Selecting Elements: Methods such as , , or can be used to identify specific elements for further manipulation.
- Modifying Elements: Developers can easily alter text content or attributes, utilizing or respectively.
- Creating Elements: New HTML structures can be added, giving rise to interactive content. The function can help in generating new nodes in the DOM tree.
Overall, DOM manipulation lays the groundwork for creating visually engaging applications that appeal to users.
Event Handling
Programatic interaction through event handling is a vital feature of JavaScript running in browsers. Events can encompass everything from user clicks to keyboard entries. Handling these events gives developers the capability to make web applications interactive and responsive.
Key concepts include:
- Event Types: There are numerous events, including mouse events (, ), keyboard events (, ), and form events (, ). Understanding how to implement these occurs is crtical to engaging user experiences.
- Event Listeners: Through the use of developers can append events and methods, linking direct user interaction to shadow behavior functions.
- Event Propagation: Knowledge of capturing and bubbling phases allows control over how events execute and reach target elements. This can prevent unwanted behavior, like handling multiple events poorly.
By correctly implementing event handling techniques, a page invites user interaction engagingly and succinctly.
Using JavaScript Libraries
In modern web development, JavaScript libraries play a crucial role in enhancing a developers productivity. Libraries contain bundles of code that help accelerate common tasks and reduce repetitive coding efforts.
Some key benefits of utilizing libraries include:
- Simplified Syntax: Libraries such as jQuery draw on syntax shortening, meaning developers can accomplish tasks quicker and easier without compromising functionality.
- Browser Compatibility: Libraries like React and Vue often compensate for fine tuned browser inconsistencies, ensuring a broader reach of functions across users.
- Extension Capabilities: Libraries are often modular, allowing customization as projects grow. New features can be integrated even after initial launch without sleep functionality issues.
Integrating libraries into projects allows developers to focus more on innovation without starting from scratch.
Understanding how to employ JavaScript in the browser effectively is essential for anyone wishing to excel in web development. By harnessing the full potential of the DOM, event handling, and libraries, developers can create sophisticated, user-centered applications.
JavaScript Frameworks
JavaScript frameworks play a crucial role in modern web development. They provide a structured way to build and maintain complex web applications. Frameworks abstract mundane tasks, helping developers to write code that is more maintainable and agile. They allow developers to focus on functionality rather than routine, repetitive coding tasks. From large-scale applications to smaller ones, the significance of frameworks cannot be overstated.
Overview of Popular Frameworks
Many JavaScript frameworks exist, catering to various needs and preferences. Some of the most prominent frameworks include:
- React: Developed by Facebook, React uses a component-based architecture. This modularity allows for easier development and reusability of components.
- Angular: Backed by Google, Angular is known for its robust ecosystem. It supports concurrent rendering, making it ideal for highly interactive applications.
- Vue.js: A progressive framework, Vue.js allows developers to adopt it piece by piece. Its simplicity and flexibility appeal to many.
These frameworks not only facilitate building applications but also provide a community and ecosystem around them. Learning any of these frameworks can substantially increase a developer's efficiency and productivity.
Using React for UI Development
React has become a dominant force in user interface (UI) development. Its philosophy focuses on a component-based approach, which significantly enhances maintainability and testing. Each piece of UI is treated as an independent component that manages its own state. This modularity builds a clear component hierarchy in applications.
Codes like the following showcase how React simplifies UI building:
Additional features, like hooks, allow functional components to manage state and side effects without needing class components. This paradigm shift has enabled easier reasoning about code.
Vue.
js Essentials
Vue.js stands out due to its versatility and ease of integration. It seeks to be an approachable, yet powerful platform. It enables developers to extend HTML with a range of custom directives.
The core concept behind Vue.js is the view model, which connects data to the UI seamlessly. Below is a simple example demonstrating the practicality of Vue.js:
Vue’s growing popularity stems from straightforward documentation and intensive community support. This has produced a compelling alternative for those looking to streamline their development process.
Using JavaScript frameworks like React and Vue can dramatically improve your productivity and code quality. Their community, documentation and resources will help lift your development process to new highs.
Debugging JavaScript
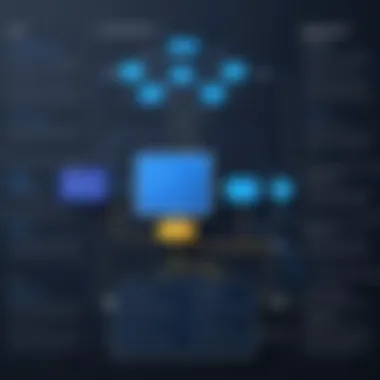
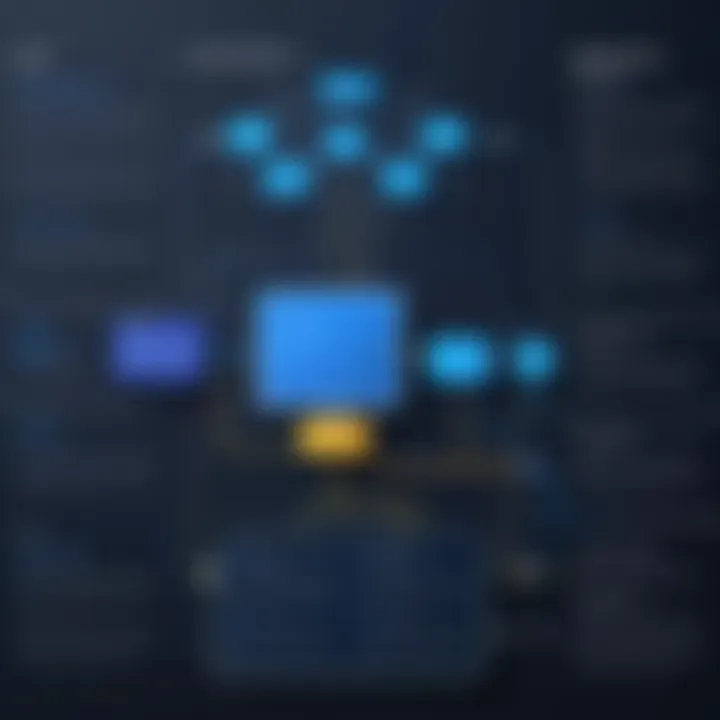
Debugging is a crucial aspect of any programming language and JavaScript is no exception. It involves identifying and fixing errors in the code to ensure smooth functionality. Without effective debugging, even the smallest mistakes can lead to significant problems, impacting user experiences and application performance. In the realm of JavaScript, where client-side execution can behave unpredictably, mastering debugging techniques is essential for every developer.
Common JavaScript Errors
JavaScript developers often encounter a series of common errors that can halt the execution of code and create confusion. Understanding these errors not only streamlines the debugging process but also enhances overall programming skills. Here are some frequent errors:
- Syntax Errors: These occur due to typos or incorrect syntax. A missing bracket or a misplaced semicolon can easily trigger syntax errors that prevent the script from running. The console will provide feedback, but inconsistency can mask the real issue.
- Reference Errors: When you refer to a variable that is not defined, a reference error will occur. It is important to ensure that all variables are correctly declared and in scope before use.
- Type Errors: Occur when an operation is performed on an unsupported data type. For example, trying to call a method on an undefined variable can throw a type error.
- Range Errors: These appear when a number is not within the set or expected range. JavaScript uses this mechanism to prevent invalid operations, such as creating an array of an illegal length.
Identifying these errors quickly can lead to faster resolution and better overall code quality.
Using Browser Developer Tools
Every leading web browser comes equipped with Developer Tools that lend invaluable assistance in debugging JavaScript. Learning how to utilize them effectively can greatly enhance your efficiency as a developer. Key features include:
- Console: An essential tool for logging variables, resulting outputs, and error messages. You can test snippets of code here to see how they behave before integrating them into a broader application.
- Debugger: You can set breakpoints in your code, allowing you to pause execution and inspect the current state of variables. This flexibility can pinpoint exactly where and why errors occur.
- Inspector: This tool lets developers inspect HTML elements alongside their associated CSS properties. Though primarily used for styling verification, it highlights changes affecting dynamic HTML manipulation through JavaScript.
Incorporating browser developer tools into your workflow can simplify and accelerate the debugging process.
Best Practices for Debugging
To optimize the debugging process and minimize errors, several best practices can be employed:
- Incremental Development: Build code in small parts. After coding each segment, test extensively before proceeding. This safeguards against accumulating multiple errors, leading to convoluted debugging sessions later.
- Use Clear Variable Names: Keeping variable names self-descriptive can help track and anticipate behavior. Avoid abbreviations or ambiguous terminology.
- Implement Unit Testing: Create a suite of tests that verify the functionality of individual components or functions. This method catches issues early, providing solid grounding for development.
- Refactor Regularly: Reassess and clean up your code periodically. This practice can increase readability and reduce the complexity that often leads to errors.
By following best practices, debugging can become a more organized and less daunting task.
Embracing the intricacies of debugging not only improves coding practices but also enhances your overall competency in JavaScript.
This section outlines the significance of debugging, common errors, tools available for assistance, and practices that streamline the debugging behavior in JavaScript. With focused effort, developers can refine their skills in this domain.
Performance Optimization
Performance optimization is essential in JavaScript, especially for vast web applications. Fast and responsive websites foster better user experience, which leads to higher user engagement, conversion rates, and overall satisfaction. Understanding performance optimization can significantly improve application usability, and it often sets apart a competent developer from a highly skilled one.
Identifying Bottlenecks
To optimize performance effectively, the first step is to identify bottlenecks in your JavaScript code. Bottlenecks are points where the execution speed is significantly reduced, affecting load time and responsiveness. There are various ways to discover these problem areas:
- Profiling Tools: Utilization of built-in browser development tools such as Chrome DevTools can pinpoint slow scripts. The Performance tab offers extensive information on time taken by various operations.
- Analyzing Network Activity: The network tab highlights the time consumed in loading scripts and other resources. This way, developers can manage load priorities better.
- Monitor User Interaction: By analyzing how users interacts with the application, developers gain insights into operation delays that significantly impact experience.
Techniques for Improving Performance
After identifying bottlenecks, one can utilize several techniques to improve the performance of JavaScript applications:
- Code Splitting: Breaking down code into smaller chunks enhances load times by allowing different parts of the application to load only when necessary.
- Minification: This process involves removing white spaces, comments, and reducing file sizes which results in faster downloads.
- Caching Strategies: Using proper caching techniques can drastically reduce the need to repeat data fetching, leading to a better performing app. Users benefit from faster load times on subsequent visits.
- Use of Async and Defer: Implementing and attributes can improve page loading by controlling how script downloads and execution occurs in relation to HTML parsing.
- Optimizing Loops: Efficiently writing loops can drastically reduce execution time. Always prefer a simpler syntax and avoid redundant operations.
Profiling JavaScript Applications
Profiling is a critical aspect of performance optimization, allowing the assessment of how the code executes during runtime. Essential steps in profiling include:
- Collecting Performance Data: Utilize the profiling tools available in web browsers to collect script performance data.
- Understanding Call Stacks: In profiling, understanding call stacks helps in locating the origin of function calls, which is crucial for optimizing complex applications.
- Keeping an Eye on Memory: Memory leaks and excessive memory consumption can significantly impede performance. Developer tools enable close tracking of memory usage over time, allowing developers to spot and rectify memory-related bottlenecks.
“The performance of your application can define how users perceive your brand. Therefore, investing time in optimizing it pays off.”
JavaScript Security Best Practices
JavaScript fuindamental to modern web development, but it also carries a range of security concerns. Therefore, adopting JavaScript security best practices is essential not just for preserving user data but also for maintaining app integrity. Security plays a crucial role, especially with the increase in cyber threats targeted at web applications. This section discusses significant elements of security practices one should implement to keep applications secure.
Understanding Common Security Risks
When talking about JavaScript security, it is vital to recognize the common risks associated with its use. The language within web browsers can expose applications to various threats. Among them,
- Cross-Site Scripting (XSS): This occurs when attackers inject malicious scripts into otherwise trusted web applications. This can exploit end users and steal sensitive information.
- Insecure Direct Object References (IDOR): Attackers might use the direct access to objects or data, bypassing security measures. This can lead to unauthorized information disclosure or data manipulation.
- Cross-Site Request Forgery (CSRF): This technique allows attackers to perform unauthorized requests on behalf of a user. If a web application has no verification, this attack can lead to data corruption or loss.
Understanding these risks is the first step towards creating robust safeguards. With constant advancements in web technologies, being aware of these threats allows developers to proactively incorporate effective defenses into their coding practices.
Implementing Secure Practices
Security in JavaScript often transpires through implementing secure coding practices. Here are key methods to ensure a more secure coding environment:
- Input Validation: Always validate user input on both client and server sides. By checking data formats and sanitation, developers can prevent many attacks like XSS and injections.
- Escaping Output: Always escape data sent to the client to avoid XSS attacks. For instance, using libraries such as OWASP’s Java Encoder can help ensure special characters are encoded.
- Use HTTPS: Using secure protocols can encrypt data used across apps and prevent intercepted exchanges by malicious actors.
- Adhere to the Same-Origin Policy: This security feature limits how scripts hosted on one origin can interact with resources from another origin. This protects against potential threats.
- CORS Configuration: Configure Cross-Origin Resource Sharing (CORS) to specify which domains can safely interact with your resources. Failing to do this might allow unwanted sites to access sensitive data.
These practices help create a safer ecosystem. However, developers must remain vigilant, updating their knowledge of current security landscape and potential vulnerabilities novel threats arise with ever-changing technologies.
Regular Security Audits
Conducting regular security audits serves as the foundation for identifying weaknesses faced within a web application. Audits can ensure that best practices continuously get adhered to and that more vulnerabilities get discovered early. Hosting routines should encompass:
- Code Reviews: Peer reviews help identify potential security weaknesses/errors as they arise.
- Automated Testing: Utilizing tools to run regular tests expose flaws in code logic or input validation methods.
- Penetration Testing: Engaging ethical hackers to simulate attacks checks app vulnerabilities, revealing unforeseen weaknesses.
Ensuring timely audits can aid in staying ahead in the constantly evolving cybersecurity space. Consider establishing an auditing regimen to pinpoint and manage risks effectively.
Regular reviews and audits are Keystone for a sustained security posture.
Awareness and adherence to these best practices can substantially reduce risks, enhancing not only individual applications but boost overall user trust in those applications. Balancing functionality with security not only makes for a robust web environment but also fosters a culture of privacy and safety in the digital landscape.
Future of JavaScript
The future of JavaScript is vital in this article, as it examines how the language evolves and stays relevant. Advancements in tech highlight the need for versatile frameworks and tools that can work seamlessly with JavaScript. Understanding these trends can guide developers in adapting new methods and ensuring they harness the full potential of JavaScript.
Emerging Trends and Technologies
There are noticeable shifts in the landscape of web development. The rise of serverless computing, for instance, empowers developers by removing the need to manage server infrastructure. This makes JavaScript useful on both client and server sides. Technologies like WebAssembly allow code written in languages like C/C++ to run in browsers alongside JavaScript, optimizing performance.
Options that enhance productivity are more common. Tools like Progressive Web Apps (PWAs) are giving rise to a new breed of applications that sit between traditional web apps and native mobile applications. Furthermore, frameworks such as Node.js offer server-side capabilities and are combined with APIs, broadening JavaScript's traditional use.
- Serverless Architecture: Shifts focus on scalable solutions in cloud environments, enabling rapid deployment.
- WebAssembly: Brings efficiency and speed allowing JavaScript to play well with other languages.
- Progressive Web Apps: Harmonize web experience, bridging web and mobile functionalities.
The Role of JavaScript in Tech Evolution
JavaScript has been a fundamental force in tech innovation. It led the way for client-side interactivity which transformed static web pages into dynamic web applications. It gave rise to a new way of delivering applications that engage users in real time. JavaScript frameworks pioneered modern software development practices like component-based architectures.
Moreover, as more businesses shift towards development processes that emphasize speed and efficiency, JavaScript remains integral. High-profile companies like Facebook and Google use it extensively to meet user needs. It shapes responsive and adaptive web solutions integral to user engagement strategies.
JavaScript facilitates not just web pages but interactive user experiences. For instance, platform such as React enhances performance by updates only on components that change. This notion allows for a significant reduction in rendering work and enhances load efficiency.
Predictions for JavaScript's Future
Observing the current trends offers insights into the future of JavaScript. Its core strength, the fact that developers are constantly creating libraries and frameworks, keeps it from growing obsolete. It is likely that JavaScript will continue to grow, especially as more tools appear. The flexibility and versatility are unmatched by other scripting languages.
Several impending features in JavaScript aim to provide more structure while also making the development experience smoother. Some expect innovations like native modules or expanded syntax for developers.
Culmination
In this article, we have analyzed the vast landscape of JavaScript, highlighting its multiple dimensions from basic principles to complex frameworks. The conclusion serves a crucial purpose: to consolidate the significant insights gained throughout. It is primarily aimed at reinforcing the foundational knowledge that guides JavaScript development, thus empowering readers, regardless of their current skills.
The importance of wrapping up the learnings is anchored in the ability to bring subtle nuances to the forefront. Among the various elements discussed, it is vital to understand how asynchronous JavaScript, object manipulation, and modern frameworks can significantly enhance application efficiency and performance. A well-rounded comprehension of these factors supports effective decision-making in any coding situation.
This leads to notable benefits. A solid grasp of JavaScript fosters creativity and problem-solving skills in web development. The endless potential for interactivity and productive applications equips learners with the tools they need for internet-based domains such as front-end design, the construction of user interfaces, and even back-end integration. Over time, practicing JavaScript principles creates mastery, gives depth to programming skills, and enhances one's marketability in the tech sector.
When learning a multifaceted programming language like JavaScript, consideration of associated factors is essential. Continuous learning is part of this experience. Developers must stay engaged with emerging trends, evolving libraries, and security challenges to remain competent in an industry characterized by rapid technological advancements. Keeping abreast of the changing environment ensures that developers do not merely practice efficiently, but that they do so by employing current best practices.
Recap of Key Points
- Foundational Knowledge: Mastery of core concepts set the groundwork.
- Practical Applications: Understanding how to apply javascrpt in real-web scenarios is essential.
- Modern Frame - The shift towards various JavaScript frameworks is relevant when discussing scaling projects.
- Continuous Education: Regularly updating skills can prevent stagnation in growth.
Further Learning Resources
To deepen your understanding of JavaScript, additional resources that are recommended include:
- Mozilla Developer Network - Offers documentation and tutorials suitable for beginners and experts.
- FreeCodeCamp - A platform that provides interactive coding challenges and projects.
- Wikipedia - Comprehensive technical insights and history of JavaScript.
- Reddit - Engaging with the community through Larry-talks and discussions can provide new perspectives.
- britannica.com - Explains the technological implications of JavaScript in various contexts.
By utilizing these resources, readers can not only refine their skills, they can stay informed about updates in the JavaScript domain, thereby enhancing their capabilities and adaptability in dynamic programming environments.