From Basics to JavaScript: Complete Guide for Beginners
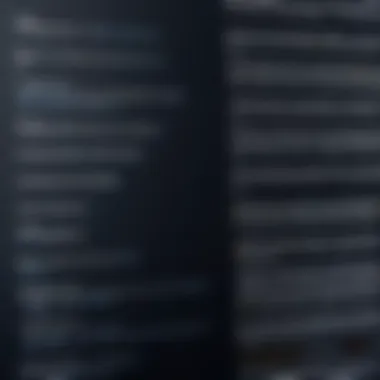
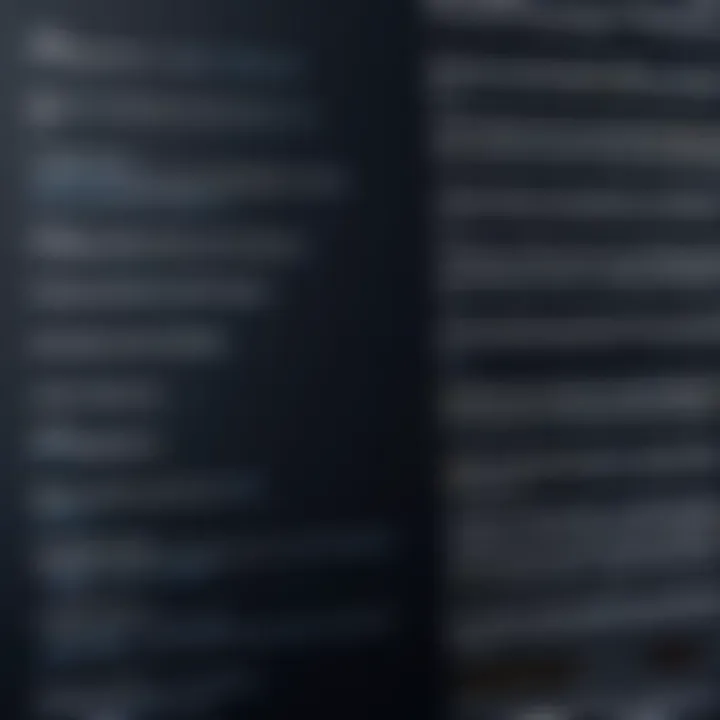
Intro
JavaScript plays a crucial role in the world of web development. As one of the most widely used programming languages, it offers functionalities that drive interactive experiences across web applications. Understanding JavaScript’s significance and capabilities is essential for anyone entering the realm of software development or enhancing existing skills.
Overview of Topic
Prelude to the main concept covered
JavaScript is a high-level, dynamic programming language that is an essential part of web technology, alongside HTML and CSS. It allows developers to create interactive web pages and handle events seamlessly. In exploring JavaScript from basics to advanced concepts, learners can cultivate the skills needed to thrive in the ever-evolving tech landscape.
Scope and significance in the tech industry
In the current tech environment, mastery of JavaScript presents a strong advantage. This programming language is not only integral for building applications but is also heavily demanded by employers. Both front-end and back-end development rely on JavaScript, via frameworks like React.js and Node.js, making its mastery invaluable.
Brief history and evolution
JavaScript was introduced in 1995 but has progressed significantly since its inception. Originally a simple language for client-side scripting, it has become a robust platform featuring frameworks and libraries that enhance its capabilities. Now, it's common in web, mobile, and even server-side programming.
Fundamentals Explained
Core principles and theories related to the topic
At the heart of JavaScript lies its design to create more dynamic, interactive user interfaces. The concept of the Document Object Model (DOM) allows JavaScript to alter HTML and CSS behavior facilitating synchronicity and user interaction.
Key terminology and definitions
- Variables: Named containers that store data.
- Functions: Defined blocks of code that execute certain tasks.
- Events: JavaScript reacts to user actions like clicks or key presses.
Basic concepts and foundational knowledge
Understanding fundamental concepts such as data types (string, number, object) and control structures (if statements, loops) forms the bedrock of proficiency in JavaScript. Allocating proper attention to comprehension of these basics will establish the footing necessary for more advanced topics.
Practical Applications and Examples
Real-world case studies and applications
JavaScript is used extensively in building responsive and user-friendly web experiences. Popular websites and applications such as Facebook employ JavaScript's capabilities for front-end development thereby showcasing its effectiveness in real-time data handling.
Demonstrations and hands-on projects
For students eager to engage, creating simple to-do list applications or interactive forms allows for a practical understanding of JavaScript syntax and functions.
Code snippets and implementation guidelines
To grasp functionality, consider this simple example of a function:
Visit Wikipedia for the foundational theories behind JavaScript.
Advanced Topics and Latest Trends
Cutting-edge developments in the field
Recently, advancements like the inclusion of TypeScript and progressive frameworks such as Next.js indicate JavaScript’s active development environment. These advancements enhance the capabilities and functionalities JavaScript can provide.
Advanced techniques and methodologies
Techniques such as asynchronous programming become particularly relevant when discussing data-fetching strategies in modern applications. Understanding promises and async/await patterns facilitates managing concurrent operations efficiently.
Future prospects and upcoming trends
The ongoing shift toward serverless architectures and microservices dictates the evolution of JavaScript. Familiarity with various libraries will aid developers in staying ahead in future developments.
Tips and Resources for Further Learning
Recommended books, courses, and online resources
Educational resources are key:
- Eloquent JavaScript by Marijn Haverbeke
- JavaScript: The Good Parts by Douglas Crockford
- FreeCodeCamp offers comprehensive courses on JavaScript.
Tools and software for practical usage
Exploring platforms such as Visual Studio Code or Sublime Text can greatly enhance the coding experience. Furthermore, using browser developer tools allows real-time debugging and testing.
The path from a novice to an adept JavaScript coder is complex yet fulfilling. Keeping abreast with practices and resources ensures your skills remain relevant, paving the way for productive contributions in the tech industry.
Prolusion to JavaScript
JavaScript is fundamental for anyone interested in web development or programming. This section introduces essential aspects of the language, presenting its significance and the core elements that every developer should understand.
What is JavaScript?
JavaScript is a high-level, interpreted programming language known for its versatility and ease of use. It was developed by Brendan Eich in 1995 originally to enhance web pages by allowing for dynamic user interactions. Unlike static HTML, JavaScript enables the creation of interactive elements such as animations, form validations, and real-time content updation.
It supports multiple programming paradigms, including object-oriented, imperative, and functional programming. This flexibility makes JavaScript a favorite among developers, powering both client-side and server-side applications.
To summarize the key characteristics of JavaScript:
- High-level language
- Interpreted not compiled
- Emphasizes functions and objects
- Supports event-driven programming
Importance in Modern Web Development
The role of JavaScript in contemporary web development cannot be overstated. It serves as the backbone of numerous frameworks and libraries such as React, Angular, and Vue.js. These tools simplify coding and enhance productivity for developers.
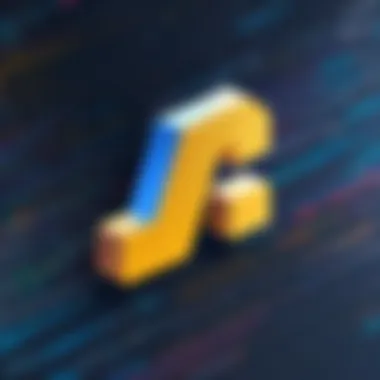
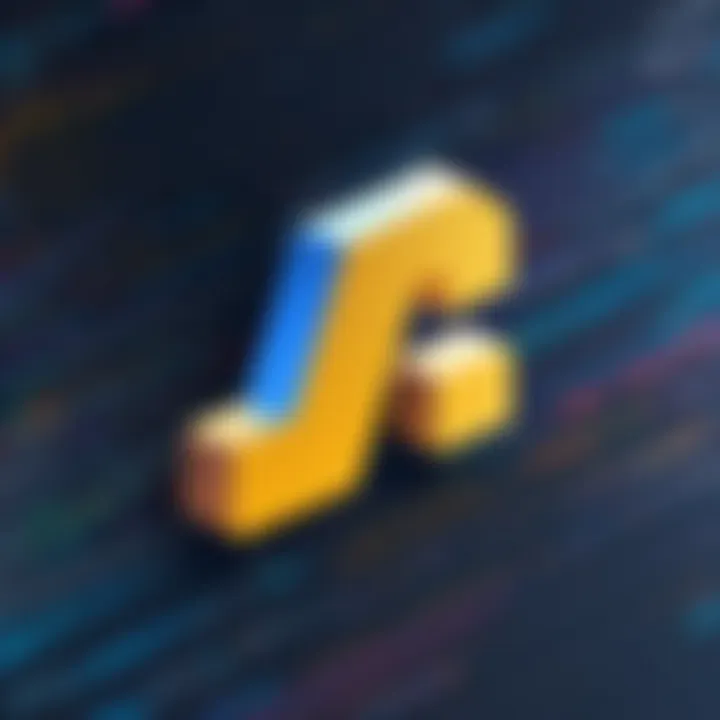
Here are some notable aspects of JavaScript's importance:
- Rich User Experience: JavaScript allows for the creation of fast and interactive web applications. Users now expect sites to respond immediately, which can only be achieved through active use of script.
- Widely Supported: All modern web browsers come with a built-in JavaScript engine. This universality ensures that any JavaScript code will run on the vast majority of devices without compatibility concerns.
- Community and Resources: A vast ecosystem exists for JavaScript, including extensive libraries, frameworks, and support forums. This benefits both novice and seasoned developers.
JavaScript continuously evolves, welcoming a host of new features that improve development efficiency.
Historical Context of JavaScript
Understanding the historical context of JavaScript provides insight into its significance in web development today. It helps to frame the evolution of programming languages and showcase JavaScript's rise as an essential skill for developers. JavaScript's journey affects various aspects, including its adaptability, versatility, and features. To grasp its impact, it's helpful to delve into two main areas: the evolution of the language itself and the key milestones encountered along the way.
Evolution of the Language
JavaScript was conceived in 1995 by Brendan Eich during his time at Netscape Communications. Originally intended to create dynamic web content, it began with a simple syntax and limited capabilities. The early iterations saw JavaScript quickly transformed from a mere client-side scripting language into a cornerstone technology of the web, sharing fundamental space with HTML and CSS.
After its initial launch, JavaScript underwent numerous transformations. ECMAScript was standardized to ensure consistent implementation across browsers, securing its growth and enhancing its functionality. This evolution ensured that JavaScript maintained relevance in a rapidly changing technology landscape.
Over the years, various versions were adopted. Key enhancements ensued, from ECMAScript 3, which introduced regular expressions and try/catch clauses, to ECMAScript 6. The latter bolstered JavaScript with features such as Promises, classes, and template literals, fundamentally reshaping the way developers worked.
Key Milestones in Development
Milestones in JavaScript's development serve as markers to evaluate its growth and social adoption:
- 1995 - The Birth of JavaScript: Created to enable interactive web experiences.
- 1997 - Standardization: The first version of ECMAScript issued by ECMA International.
- 2009 - ECMAScript 5: Introduced improved features setting a new baseline for developers.
- 2015 - ECMAScript 6: A significant update that transformed the language, bringing modern programming concepts to JavaScript.
- Present Day - JavaScript Everywhere: Utilizing frameworks and libraries like React, Angular, and Node.js, JavaScript solidifies its position as a dominant player in web and server-side development.
JavaScript today is not just a language but an ecosystem, pivotal in the advancement of technology and web experiences.
These historical points of interest not only underline the technical growth of JavaScript but also emphasize its importance in web development. Each evolution, and milestone renders a better tool for developers, while the adequate understanding of its context enhances a programmer's ability to leverage this powerful language effectively.
Basic Concepts of JavaScript
Understanding the basic concepts of JavaScript is crucial for anyone looking to build a strong foundation in the language. This section introduces essential elements, starting from variables and data types to operators, expressions, and control structures. Mastering these concepts enables developers to write efficient, maintainable code. Basic concepts form the building blocks of programming logic, and grasping them enhances problem-solving abilities.
Variables and Data Types
Primitive Data Types
Primitive data types in JavaScript consist of undefined, null, boolean, number, string, and symbol. These types represent single values and are crucial in programming as they allow for the representation of simple form of data. Understanding these data types simplifies the process of data handling. The simplicity of primitive data types makes it easier for learners to understand the nature of the data they will work with.
One key characteristic of primitive data types is that they are immutable. Once a primitive variable is voluntarily set to a value, it cannot be altered. This feature leads to benefits like efficiency in memory usage but has its disadvantages as well, such as requiring the creation of new variables for altered values.
Complex Data Types
Complex data types include objects and arrays. These are used for creating more complex data structures, storing and organizing multiple values. A key characteristic is that they can hold collections of data or more complicated entities compared to primitive types. Hence learners engage in using slogans, images, or even interactively structured data.
One unique feature of complex data types is mutability. Variables assigned complex data types can have their values changed or modified while still referring to the same memory address. This aspect is particularly advantageous when working with large datasets, as adjustments can be made without additional memory impacts but may lead to unexpected side effects if not handled correctly.
Operators and Expressions
Arithmetic Operators
Arithmetic operators in JavaScript consist of addition, subtraction, multiplication, division, and modulo. These operators are used primarily for numerical calculations. The benefit of arithmetic operators is their simplicity and expressiveness in notching real-world problems into logical solutions.
The unique feature of arithmetic operators is their ability to perform basic mathematical operations with ease, allowing for expressing complicated equations linearly. However, relying most heavily on them without sanity checks could result in common errors, like division by zero.
Logical Operators
Logical operators such as AND, OR, and NOT allow developers to make decisions within their scripts through applied logic. They are fundamentally essential for conditional expressions and branching in code execution. This is valuable for crafting sophisticated programming logic that reacts accordingly to various inputs.
A recognized characteristic of logical operators is short-circuit evaluation, which can lead to performance advantages in scenarios where multiple conditions are executed. While these operators give clear logical flair to code, they may also often lead to unpredictable behavior if priority orders are not well understood functionality.
Control Structures
Conditional Statements
Conditional statements, like if, else if, and switch, enable a program to dictate which code block will run based on specific conditions. This introduces a key aspect, controlling the flow of execution in scripts. It enhances flexibility in code, making it dynamic and responsive—all important for user interactions.
The broader advantage lies js in their versatility as you can handle multiple conditions neatly with a switch statement when comparing repetitive if conditions. On the downside, excessive nesting can cause confusion and reduced readability, which may complicate conduct.
Loops
Loops help repeat a block of code a specific amount of times or until a condition is met. Types of loops in JavaScript—like for, while, or do-while—increase productivity by limiting redundant coding for repeated tasks. The ability to leave your lines can optimize coding when running large sequences of tasks, making bugs simpler to localize
A unique feature of loops is their control mechanisms, capable of modifying how often iterations occur with conditions set beforehand. However, infinite loops can occur if evaluating conditions are mistakenly configured, blocking processes unexpectedly.
Functions in JavaScript
Functions in JavaScript are essential components that encapsulate code for reuse and organization. They enable developers to break code into manageable sections, greatly improving clarity and maintainability. Understanding functions is vital for any programmer. They form the backbone of scripting in JavaScript, allowing the execution of complex operations with simple invocations.
Functions in JavaScript enhance code reusability, which is a core principle in programming.
Defining Functions
Defining a function in JavaScript can be done using a straightforward syntax. The basic structure is about naming the function, providing the parameters, and specifying the code block to execute. For example:
In this sample, the function accepts a single parameter named and returns a greeting string. This simple practice has significant benefits:
- Code Reusability: Once defined, a function can be called multiple times without duplicating code.
- Simplified Debugging: Errors may be fixed in one place instead of searching through repetitive code.
As such, defining functions is the first major step toward establishing logic within your web applications.
Function Scope and Hoisting
Function scope refers to visibility of variables defined inside a function in JavaScript. Variables declared within a function are not accessible outside that specific function scope. This encapsulation organizes code efficiently and prevents unintended interactions between different parts of the code.
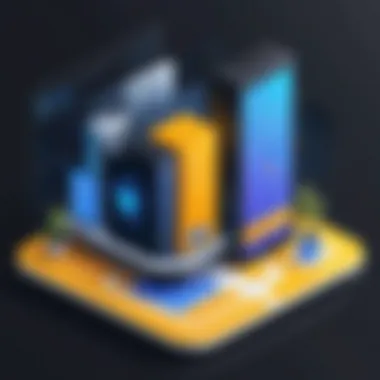
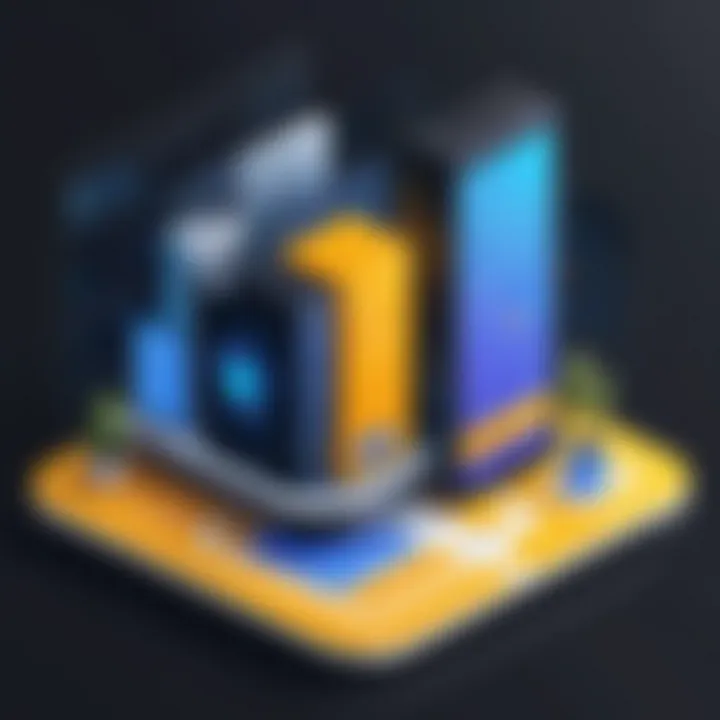
Equally important is the concept of hoisting. Hoisting means that function declarations can be referenced before they appear in the code. JavaScript moves function declarations to the top of the code during the compile phase. Hence, this flexibility allows organizing your code in ways that are sometimes counterintuitive, but practical.
Arrow Functions and Their Uses
Arrow functions are a more recent addition to JavaScript syntax. They exist for cleaner operations and to ease function definitions. Defined using the operator, arrow functions do not bind their own , potentially making code more intuitive.
For instance:
This highlights:
- Conciseness: Arrow functions allow for more compact function definitions.
- Lexical : They leverage from the enclosing context, reducing confusion regarding context bindings.
In summary, functions in JavaScript enhance modularity and improve overall program design. Understanding them lays the foundation for advanced coding techniques in JavaScript. For further reading, you may explore Wikipedia or related discussions on Reddit.
Objects and Arrays in JavaScript
Objects and arrays are essential components of JavaScript, serving as foundational elements in organizing and manipulating data. They enhance the language's capability to build complex applications and manage diverse types of information efficiently. Understanding these concepts is crucial for anyone looking to develop strong JavaScript skills, especially in modern web applications.
Objects in JavaScript are collections of related data. They can store various keyed values. This structure allows for easier data management and retrieval, making coding more organized and intuitive. Arrays, on the other hand, play a crucial role in managing lists of values. They allow for easy iteration and manipulation, making JavaScript highly efficient in as complex tasks. Both structures provide the necessary flexibility to create dynamic web pages and applications, underscoring their significance in this article.
Understanding Objects
Objects are one of the core components of JavaScript. They enable developers to represent real-world entities and manage related attributes effectively. It is a form of data structure that helps in maintaining a relationship between properties and methods.
Properties and Methods
The significance of properties and methods lies in their roles in defining the characteristics of an object and allowing interaction with these objects. Properties represent the data associated with an object, while methods are functions that can perform operations on that data.
Key characteristics of properties include their ability to store multiple types of data in a single typed unit. This enables a compact representation of information. For instance, an object can have properties such as name, age, or profile picture, all associated under one identifier. On the other hand, methods facilitate the manipulation of object states or behaviors.
When discussing their usage, the unique feature of properties and methods is that they instantiate functionality. Their encapsulation in objects achieves a significant reduction in global namespace pollution. This structured approach allows for easier modifications later, minimizing errors and bugs in larger programs.
Advantages:
- Easy to manage related data
- Encapsulates functionality for clear object-oriented programming
- Improves code reusability
Disadvantages:
- May lead to performance lags with complex object structures
- Debugging can be less straightforward due to nested methods
Arrays and Their Manipulation
Arrays complement the versatility of Python very effectively. They facilitate the storage and management of lists of data. Iterating through arrays is common in many programming operations and useful for combining or manipulating datasets easily.
Array Methods
Array methods provide various options to manage and process data functions smoothly. These built-in functions simplify numerous array-based operations such as adding, removing, or searching for elements within the array. Popular methods include , which adds elements to the end of an array, and , which generates a new array based on the original.
These methods are widely used for several reasons. They improve developers' productivity, allowing them to focus on specific application features rather than the complexity of language syntax. The organized approach to array methods ensures operations remain consistent, referentially eliminating cycles in resulting data structures.
Advantages:
- Saves time through predefined functionalities
- Encourages modularity within the code
Disadvantages:
- The potential for heavy resource usage with extensive data manipulation
- May confuse newcomers without foundational knowledge
Iterating Arrays
The practice of iterating arrays provides insight into their functionality. This aspect is crucial, as accessing array elements efficiently can optimize performance in applications. Iteration enables looping over elements, applying conditions or transformations effectively. Often done using methods such as or , iteration optimizes code performance and readability.
The uniqueness of this feature lies in its ability to enhance computational efficiency, allowing simultaneous processing of large datasets relevant in data-heavy applications.
Advantages:
- Simplifies data handling and manipulation
- Reduces repetitive code through array methods
Disadvantages:
- Inefficiency if poorly structured or excessive iterations are used
- Potential lock-in effects with deeper nested structures in complex loops
Learning and utilizing objects and arrays is vital for mastering JavaScript. Their relevance shapes both categories at the time of code writing and at running time, powering the core functionality required in web applications today.
JavaScript's true power lies in how well it can manage and manipulate data, with objects and arrays at its heart.
For further learning and resources, explore articles on Wikipedia, detailed discussions on Britannica, or engaging conversations on Reddit.
DOM Manipulation
DOM manipulation is a fundamental skill for any JavaScript developer. It refers to the ability to interact with and modify the Document Object Model of a webpage. Understanding the DOM is critical for creating dynamic and interactive web applications. By mastering this topic, developers can enhance the user experience on their sites and applications.
What is the DOM?
The Document Object Model, or DOM, represents the structure of a web document. It makes the document a tree of objects, which allows programs and scripts to access and update the content, structure, and styles of a webpage dynamically.
In simpler terms, if you think of an HTML page as a building, the DOM is the blueprint. This representation allows developers to modify the building as needed – add or remove elements, alter content, and respond to user interactions.
Key aspects of the DOM include:
- It is language-independent. Though often manipulated with JavaScript, other languages can also interact with it.
- It represents the document as a tree structure, with each node representing a part of the document, such as elements, attributes, and text.
- It allows for dynamic changes. You can change HTML elements and styles without reloading the page.
The DOM is critical for web development as it allows for dynamic rendering of web pages, satisfying the demand for interactivity in modern web applications.
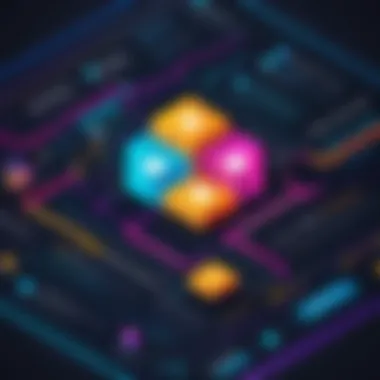
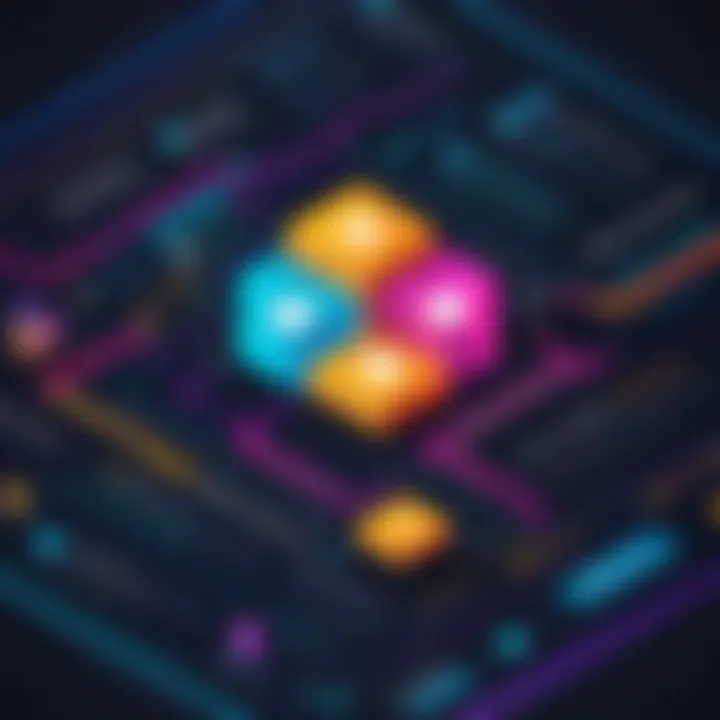
Selecting and Modifying Elements
Selecting elements in the DOM allows developers to target specific areas in the HTML for modifications. Essential methods include:
- : This method retrieves an element based on its unique ID.
- : This method selects a group of elements sharing the same class name.
- : This method utilizes CSS selectors to target an element quickly.
After selecting an element, you can easily change its properties using JavaScript. For example, to change the text content of a paragraph:
This modifies the paragraph text on the webpage, demonstrating how simple it is to impact the user interface using JavaScript.
Benefits of Modifying Elements
- Increased flexibility: Adjust content without needing to refresh.
- Improved user engagement: Immediate feedback to user actions enhances interactivity.
- Easier debugging: Changes can be seen instantly, simplifying testing.
Event Handling
Event handling in JavaScript allows for creating responsive applications. It deals with the way JavaScript can react to user actions, such as clicks, keyboard entries, and mouse movements. By binding functions to events, developers can execute code in response to specific user interactions.
Common events to monitor include:
- : Triggered when an element is clicked.
- : Activated when a mouse pointer is moved over an element.
- : Initiated when a key on the keyboard is pressed.
A basic example involves adding a click event to a button:
This example shows the alert prompting whenever the button is clicked. In many applications, event handling correlates directly with improved user interaction and responsiveness.
In summary, understanding and mastering DOM manipulation is crucial for JavaScript developers. As a core facet of web development, it serves as the bridge between constructed layouts and seamless interactions. Through selecting, modifying elements, and effectively handling events, a developer can cultivate a richer user experience.
Asynchronous JavaScript
Asynchronous JavaScript is pivotal in creating fluid, responsive web applications. It enables developers to perform non-blocking operations, meaning an operation can run multipart while allowing other actions to continue uninterrupted. In web development, this ensures that an application does not freeze or pause while waiting for data to be retrieved or processed. That results in smoother user experiences, essential in today's fast-paced digital landscape.
Understanding Asynchronous Operations
Asynchronous operations can be complex but are crucial when dealing with user interactions and requests. Such processes allow JavaScript to handle events, like mouse clicks or keyboard input, independently of the main thread, preventing the user interface from becoming unresponsive. Three primary methods implement asynchronous behavior in JavaScript: callbacks, promises, and async/await. These methods facilitate managing code execution while maintaining a logical structure in the program's workflow.
A key component in understanding asynchrony is the event loop. The event loop continually checks for tasks in the task queue. If there are any promises resolved or functions awaited, the loop processes them, allowing the main thread to move freely without locking.
Promises and Callbacks
Promises represent a modern solution to the challenges posed by previous models of asynchronous programming like callbacks, which could result in
JavaScript Best Practices
JavaScript is a powerful language used extensively in web development. Adhering to best practices can ensure that code is more maintainable, efficient, and easier to understand. Good practices can also contribute to enhanced performance and security. For anyone learning JavaScript, implementing best practices from the very beginning can pave the way for progressive skill development.
Code Organization and Readability
Code organization is critical in JavaScript development. Well-organized code can significantly ease the process of maintenance. Keeping files structured and modular can identify functionality at a glance. Here are key-point practices for enhancing code organization:
- Use meaningful names: Variables, functions, and classes should have descriptive names. This reduces confusion and enhances comprehension for others (or yourself) later on.
- Separate concerns: Group similar functionalities into modules or files. This makes collaboration and debugging easier.
- Follow consistent formatting: Decide on a style for your code (like indentation and spacing) and stick to it. Consider using tools such as Prettier or ESLint to standardize formatting across your project.
Readability encompasses the ability to easily read and understand your code. Future coders, including your future self, will benefit from:
- Comments: Comment your code to explain complex logic. Use comments strategically so they add value.
- Limit line lengths: Longer lines can deter reading flow. Aim for 80-120 characters per line for optimal readability.
- Use whitespace effectively: Indent properly and leave spaces between functions and logic blocks. This creates natural chunks within the code, aiding understanding.
Debugging Techniques
Debugging is an essential part of JavaScript development. Every developer, at some point, confronts errors and bugs in their work. Recognizing that debugging is a systematic process can help manage frustrations related to error resolution. Here are practical techniques:
- Use console statements: can help trace and inspect values. Deploying log statements in suspect areas makes detecting where things go awry much simpler.
- Browser DevTools: Many browsers have built-in developer tools. Familiarize yourself with setting breakpoints, watching variables, and other debugging options.
- Network monitoring: Pay attention to the network tab in developer's tools. By monitoring requests and responses, you can discover issues stemming from requests for data.
It's vital to affirm the necessity of consistent debugging practices. Properly investigating errors saves time and boosts coding efficiency.
Resources for Learning JavaScript
Learning JavaScript is not merely about grasping syntax. The resources available often dictate the pace and depth of understanding. Therefore, selecting the right tools can greatly enhance both the learning experience and mastery of JavaScript. This section discusses the necessity of robust learning resources and highlights a variety of options suitable for different learning styles and backgrounds.
Online Courses and Tutorials
Today, a variety of online platforms offer structured learning experiences tailored to JavaScript. Websites like Codecademy, Udemy, and freeCodeCamp provide fellow users an opportunity to interact with code and complete hands-on exercises. The benefits of this format include self-paced learning and a visual representation of concepts.
For participants new to programming, visual or interactive courses are highly advantageous. They allow people to see immediate results for their efforts. Furthermore, using platforms such as Coursera or edX can provide verified certificates. Therefore, this is a major plus for individuals seeking to bolster their resumes.
However, learners should compare course reviews and precisely consider course content. Selecting a course aligned with personal goals is very crucial. Not all platforms approach JavaScript with the same emphasis on all aspects; a course may focus on front-end or back-end development planning. By comprehensively assessing course offerings, students can ensure that their investments are sound.
Books and Documentation
Books provide a different yet authoritative avenue for understanding JavaScript. Well-regarded selections like
The End
The conclusion of this article emphasizes several important themes surrounding JavaScript as a language and its role in modern development. A critical takeaway is the transformation JavaScript has undergone since its inception, positioning it as a cornerstone for web technologies today. It informs the reader about cheveral key elements such as its specific capabilities and its integration with other frameworks and tools.
The Future of JavaScript
Looking ahead, JavaScript continute to develp at a rapid pace. Projects like ECMAScript propose enhancements to the language, introducing features like optional chaining and nullish coalescing. These developments resolve long-standing pain points within the development community. Furthermore, with the rise of frameworks such as React and Angular, JavaScript extends beyond simple scripting into a comprehensive ecosystem for building seamless user experiences.
A trend that stands out is the move seek to adhere to best practices and community standards. Learning how to adapt to these changes is paramount. Enthusiasts are awaer of JavaScript not being static; it evolves with the tech landscape, and the increasing interest in server-side JavaScript through Node.js opens more doors for developers.
Encouraging Continuous Learning
In the journey of mastering JavaScript, embracing a mindset of continuous learning is invaluable. JavaScript is not just a one-time study but a dedication to growth in understanding and application. This language has a vast community on forums and platforms like Reddit and helpful documents available on Wikipedia and Britannica.
easoning from various online courses and documentation will not only drive competence but will also help in keeping one ahead of industry trends. Creating practice projects, joining open source contributions, and attending conferences can further this aim. JavaScript will likely change, but maintaining that hunger for knowledge will empower professionals to leverage new methodologies and solutions effectively.
Continuous learning is the key method to stay relevant in any technical field.
By understanding the evolving nature of JavaScript and its surrounding community resources, developers can expect to grow more than just their portfolio—they cultivate a deeper, conceptual grasp of web development as it encompasses the modern digital arena.