C++ Interview Questions: A Complete Guide for Candidates
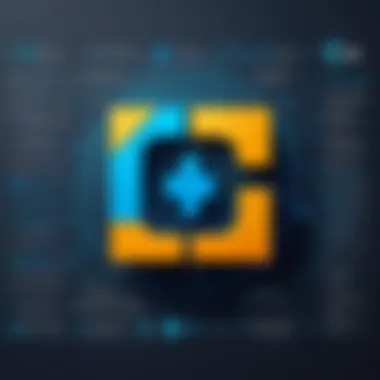
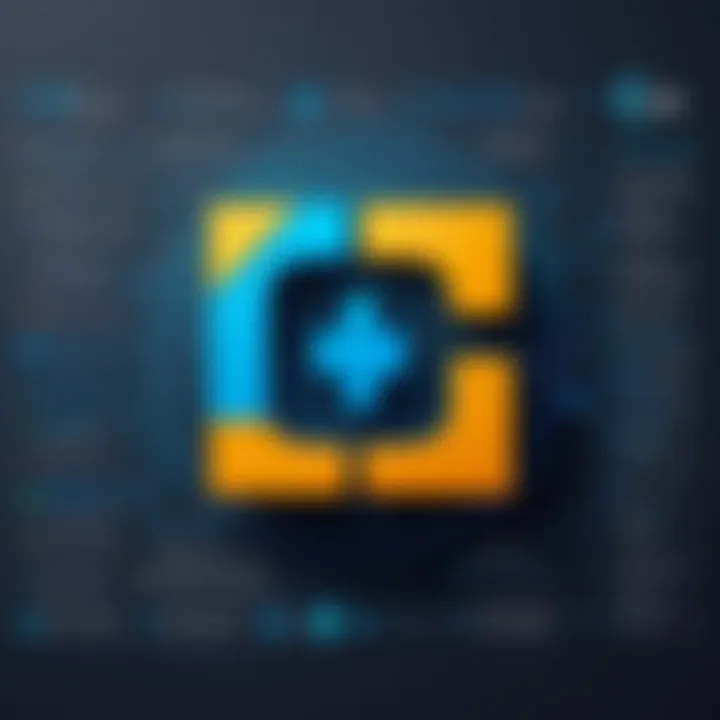
Overview of Topic
C++ has established itself as a critical pillar in the realm of software development, providing a unique blend of performance and abstraction. The language’s versatile nature allows it to power everything from operating systems to high-performance applications. Navigating through a technical interview focused on C++ demands a strong grasp of its principles and the ability to apply them effectively.
The tech industry has seen a rising trend in the adoption of this language, leading to a surge in demand for professionals who can demonstrate proficiency in it. Understanding C++ technical interview questions not only bolsters a candidate's confidence but also enhances their problem-solving skills, becoming an essential aspect of preparation.
Historically, C++ was developed in the early 1980s by Bjarne Stroustrup at Bell Labs as an enhancement to the C programming language. Over the decades, it has evolved, transitioning through various standards (from C++98 to C++20), each introducing features to improve usability, efficiency, and performance. This historical perspective offers insights into its enduring relevance and the importance of staying updated with the latest advancements.
Fundamentals Explained
To ace a C++ technical interview, candidates need foundational knowledge. Understanding core principles such as object-oriented programming, encapsulation, inheritance, and polymorphism is vital. These concepts form the bedrock upon which advanced C++ functionalities are built.
Key Terminology
Here are some essential terms one needs to grasp:
- Class: A blueprint for creating objects, defining their properties and behaviors.
- Object: An instance of a class.
- Constructor: A special function that gets called when an object is created.
- Destructor: A function that is executed when an object gets destroyed.
Basic Concepts
- Syntax Basics: Being comfortable with C++ syntax is crucial. Simple statements, expressions, and data types should be understood well.
- Control Structures: Understanding loops (for, while), conditionals (if, switch) is important for writing functioning code.
- Memory Management: Knowing how to manage memory using pointers, references, and dynamic vs static allocation can help distinguish oneself in interviews.
Understanding these fundamentals not only equips candidates to answer questions effectively but also to think critically and apply C++ concepts practically.
Practical Applications and Examples
C++ has a broad range of applications. It's often used in systems software, game development, drivers, and client-server applications, among others. Understanding these applications can enrich a candidate's responses during interviews.
Real-World Case Studies
For instance, many game engines, such as Unreal Engine, are coded in C++. These engines utilize C++'s high-performance capabilities to manage graphics and complex game states.
Code Snippets
Here’s a simple code snippet that demonstrates the use of classes and objects:
Implementation Guidelines
When implementing C++ applications, follow best practices such as:
- Keeping functions concise.
- Using meaningful variable names.
- Commenting code for clarity.
Advanced Topics and Latest Trends
As candidates upscale in their C++ knowledge, they should also familiarize themselves with advanced concepts. Modern C++, with its Concurrency features and Lambda Expressions, opens new horizons in application development. A grasp of these features can significantly amplify one's chances in interviews.
Future Prospects
The evolution of C++ also reflects in industries leaning towards cloud computing, machine learning, and data science. Understanding how C++ fits into these areas is essential for prospective developers aiming to stay relevant.
Tips and Resources for Further Learning
To equip yourself further, consider diving into these resources:
- Books: "Effective C++" by Scott Meyers offers invaluable insights on best practices.
- Online Courses: Websites like Codecademy and Coursera have tailored C++ classes.
- Tools: Learning to use IDEs like Visual Studio or Code::Blocks can enhance coding experiences.
Mastering the art of C++ is not just about coding; it's about understanding the underlying logic that drives effective solutions in software development.
Preface to ++ Interviews
In the realm of technical interviews, C++ stands out as a language that not only fosters a strong understanding of computer science but also hones problem-solving skills. This section will shed light on why mastering C++ is paramount for candidates hoping to land a role in tech.
The Importance of ++ in Technical Interviews
C++ is often considered a gateway to other programming languages, functioning through a blend of high-level and low-level language features. Its presence in technical interviews underscores its robustness and widespread application in various industries.
- Foundation of Programming: Many concepts in computer science, like algorithms and data structures, are effectively demonstrated in C++. This makes it easier for interviewers to evaluate a candidate's core programming skills.
- Performance and Control: C++ allows for fine-tuned optimization and is widely used in systems programming, game development, and performance-critical applications. Consequently, showing competent C++ skills can alleviate concerns over a candidate's potential performance in coding.
- Used by Major Companies: Notably, tech giants such as Google, Microsoft, and Facebook often incorporate C++ into their development stack. As such, being proficient can significantly increase a candidate's employability.
The significance of C++ extends beyond simple syntax and semantics. It serves as a test of critical thinking, as candidates must demonstrate not just how they code, but how they approach complex problem-solving scenarios. Familiarity with C++ can distinguish a candidate in a crowded field, reflecting a commitment to understanding the language's deeper nuances.
Overview of Common Interview Formats
Understanding the landscape of interview formats is crucial for C++ aspirants. Different companies adopt various strategies and structures when assessing candidates, and being prepared for these variations can be the key to success.
The common interview formats can generally be categorized as follows:
- Technical Phone Interviews: Usually, candidates first face a phone screen, where they're asked fundamental C++ questions or to solve problems using coding platforms like HackerRank or LeetCode. This format is less personal but critical for filtering candidates.
- In-Person or Video Interviews: As candidates advance, they participate in technical interviews that might involve live coding, problem-solving on a whiteboard, or using collaborative tools like Zoom. Interviewers often assess both thought processes and technical skills here.
- Take-Home Assignments: Some companies provide candidates with coding tasks to be completed at home. This allows interviewers to gauge a candidate's ability to work independently and manage time while applying C++ concepts effectively.
- System Design Interviews: For mid to senior-level positions, interviews may shift towards larger system design discussions. Candidates must utilize their C++ knowledge to architectively design systems, which showcases their strategic thinking.
"The way you approach a problem in an interview often matters as much as finding the correct solution."
In summary, getting familiar with C++ in various interview formats significantly boosts confidence and preparedness. A well-rounded understanding of how interviews are structured can ease the anxiety associated with the process and allow candidates to shine.
Fundamental Concepts in ++
Understanding Fundamental Concepts in C++ is like laying the tracks for a well-functioning train—without a solid foundation, everything that follows can become derailed. C++ is a powerful programming language known for its flexibility and efficiency, widely utilized in systems programming, game design, and applications requiring high performance. Mastering the fundamentals allows both budding programmers and seasoned professionals to navigate the complexities of C++ with ease. These concepts provide essential building blocks needed to grasp more advanced topics later, ensuring that one can write efficient, maintainable code even under challenging circumstances.
Basic Syntax and Structure
C++ syntax is fundamental to creating any application. The language borrows many elements from C, yet it integrates its unique features. Understanding the basic syntax involves knowing how to structure a program, including proper usage of headers, main function, and basic statements.
A simple C++ program typically looks like this:
In this snippet, the directive allows input and output operations. The statement tells the compiler to use the standard C++ library, simplifying the code you write.
It's vital to remember that C++ is case-sensitive; thus, and would be treated as distinct identifiers. Moreover, every statement must end with a semicolon, which is a common mistake among beginners.
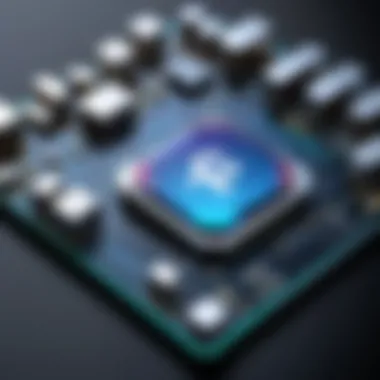
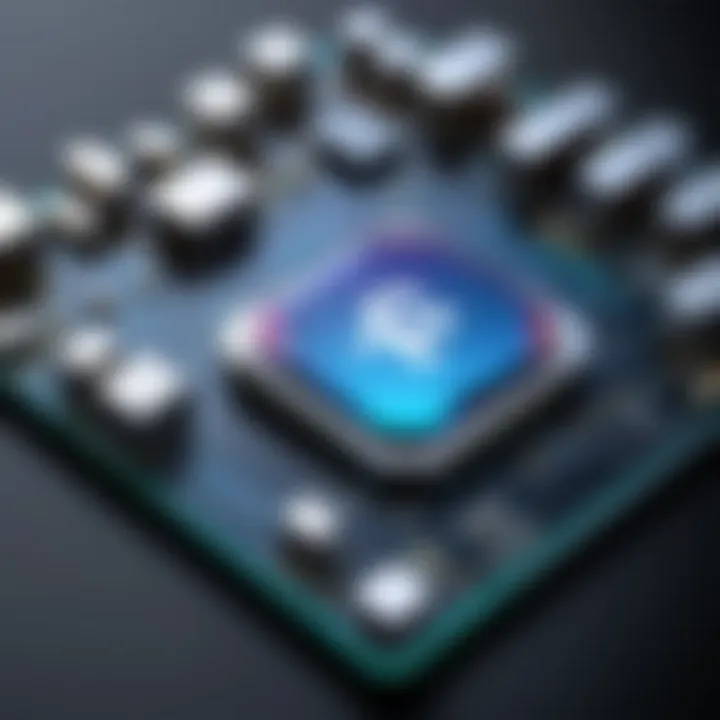
Data Types and Variables
Diving into Data Types and Variables, this section deals with how information is categorized and stored. C++ supports several built-in data types, such as integers (int), floating-point numbers (float and double), characters (char), and booleans (bool). Grasping these types is crucial for memory management and performance optimization.
For instance, when declaring an integer variable, you'd use:
This code snippet creates a variable named that holds the value 25. Understanding variable scope is also essential; where a variable is declared affects its accessibility throughout your program.
Keeping track of your data types can prevent unnecessary errors and enhance code readability.
Operators and Expressions
In C++, operators are the vehicles through which you manipulate data stored in variables. They can be categorized broadly into arithmetic, relational, and logical operators. Understanding these operators allows for effective expression writing. For example:
- Arithmetic operators include , , , , and (the modulus operator).
- Relational operators such as , , , ``, , and are used to compare values.
- Logical operators involve (and), (or), and (not), useful in controlling program flow.
An example of using these operators can be illustrated with a simple if-else statement:
This snippet checks if is greater than 5 and prints the appropriate response. Operators, in combination with expressions, form the core of logic in programming, allowing programmers to create complex decision-making structures within their code.
"Understanding and mastering the fundamental concepts of C++ can greatly reduce the chances of errors and enhance one's ability to work efficiently in various domains."
As you delve deeper into the world of C++, a strong grasp on its fundamental concepts makes for a smoother journey in mastering this versatile language.
Object-Oriented Programming Principles
Object-Oriented Programming (OOP) principles form the backbone of many modern programming languages, including C++. Understanding these principles is not just important for writing efficient code but also crucial for tackling technical interviews. Employers often seek candidates who can think in terms of objects and classes because it reflects a methodical and structured approach to problem-solving. OOP is all about breaking down complex problems into manageable, self-contained units, which can then be reused and tested independently.
Among the principles involved in OOP, the most important are classes and objects, inheritance and polymorphism, as well as encapsulation and abstraction. Each of these areas has its own unique importance:
- Classes and Objects: These serve as the fundamental building blocks of OOP. Classes allow for the definition of complex types using simpler data types, which subsequently create objects. This relationship aids in organizing code and promoting reusability.
- Inheritance and Polymorphism: These principles enable new classes to inherit the features of existing classes, making code more concise and easier to maintain. Polymorphism permits methods to be used on objects of different classes, enhancing flexibility.
- Encapsulation and Abstraction: These focus on restricting access to certain components of an object, ensuring that the internal state cannot be altered unexpectedly. This not only safeguards data integrity but also simplifies the interface of complex systems.
By grasping these principles, candidates can demonstrate a high level of understanding during interviews, revealing their ability to write modular and comprehensible code.
Classes and Objects
In C++, a class serves as a blueprint for creating objects. It encapsulates data and methods that operate on that data. When you think about any software component, it often relates back to a class or a collection of classes. For example, consider a simple application managing a library system. You might have a class with properties like , , and methods for checking in and checking out a book.
In this case, each instance of is an object with its own state, separate from others. Understanding this concept lets interviewees directly relate practical examples to theoretical questions, thus showing a comprehensive understanding of OOP.
Inheritance and Polymorphism
Inheritance allows a new class, often called a derived class, to inherit features (properties and methods) from an existing class, known as a base class. Consider a scenario where you have a class. It can represent general characteristics, but when creating more specific types like and , you would want to inherit common properties while adding unique features.
Due to polymorphism, a method can take many forms. This means you could have a method in the base class called . The derived classes, and , may implement this method in ways specific to their functionality. This aspect is incredibly useful during interviews because it reflects the candidate's understanding of dynamic behavior and code adaptability.
Encapsulation and Abstraction
Encapsulation is the principle of bundling data with the methods that operate on that data, restricting outside interference. When encapsulation is followed, the internals of a class can be changed without affecting other parts of the application. For instance, if you needed to update how the method operates, as long as the interface remains the same, the rest of your application would be unaffected.
Abstraction, closely tied with encapsulation, refers to the ability to expose only the necessary parts of an object while hiding the complex implementation details. For example, in the library management system, users need not know how the method works internally—they simply call the method when they want to borrow a book.
Important Note: Understanding the difference between classes and objects, and mastering inheritance, polymorphism, encapsulation, and abstraction are not just academic exercises. Proficiency in these areas can greatly enhance one's problem-solving capabilities and coding efficiency in real-world applications.
Delving into OOP principles allows candidates to clearly explain their thought process during technical interviews, making them more appealing to potential employers.
Advanced ++ Features
Advanced C++ features extend the language beyond its basics, providing tools that enhance programming efficiency and streamline complex systems. For individuals preparing for technical interviews, understanding these features can exemplify a candidate's depth of knowledge and problem-solving skills. Topics such as templates, exception handling, and the Standard Template Library (STL) enrich a programmer's toolkit, making it essential for interview preparation. These concepts not only demonstrate technical abilities but highlight how candidates can architect scalable, durable applications while adhering to best practices.
Templates and Generic Programming
Templates play a crucial role in C++, allowing developers to write flexible and reusable code. By using templates, you can define functions and classes that work with any data type. This ability to generalize code enhances maintainability and reduces duplication.
Consider the following simple example of a function template:
This function can now be used for integers, floats, or any other type that supports addition. This feature increases efficiency as it eliminates the need to write separate functions for each data type, catering to a wide array of use cases.
Benefits of using templates include:
- Code Reusability: Write once, use with any type.
- Type Safety: Errors are caught at compile time rather than runtime.
- Performance: Only one version of the code exists, improving execution speed.
In the context of interviews, expect to encounter questions that test your understanding of template specialization and use cases where templates are most beneficial.
Exception Handling
C++ provides robust support for exception handling, a vital feature for managing errors dynamically during program execution. Mastering this aspect is crucial for candidates aiming to write safe and reliable code. Exception handling enables a program to deal with unexpected conditions without crashing, thus improving user experience.
The basic syntax involves the use of , , and keywords. Here’s a simple example:
With this structure, your program handles errors gracefully. Some aspects to keep in mind include:
- Custom Exceptions: Create user-defined exception classes for specific error handling.
- Resource Management: Use RAII (Resource Acquisition Is Initialization) principles to manage resources efficiently and prevent leaks.
- Best Practices: Avoid excessive use of exceptions for control flow; reserve them for truly exceptional cases.
Interview questions may tackle scenarios where exceptions are beneficial or where they could lead to unforeseen consequences. Be prepared to demonstrate how you would approach error management in real-world applications.
Standard Template Library (STL)
The Standard Template Library is a powerful component of C++ that provides a suite of useful classes and functions for data structures and algorithms. STL facilitates developers in implementing efficient algorithms on data collections using iterators and containers such as vectors, lists, and maps.
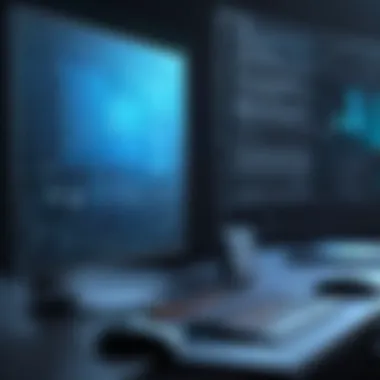
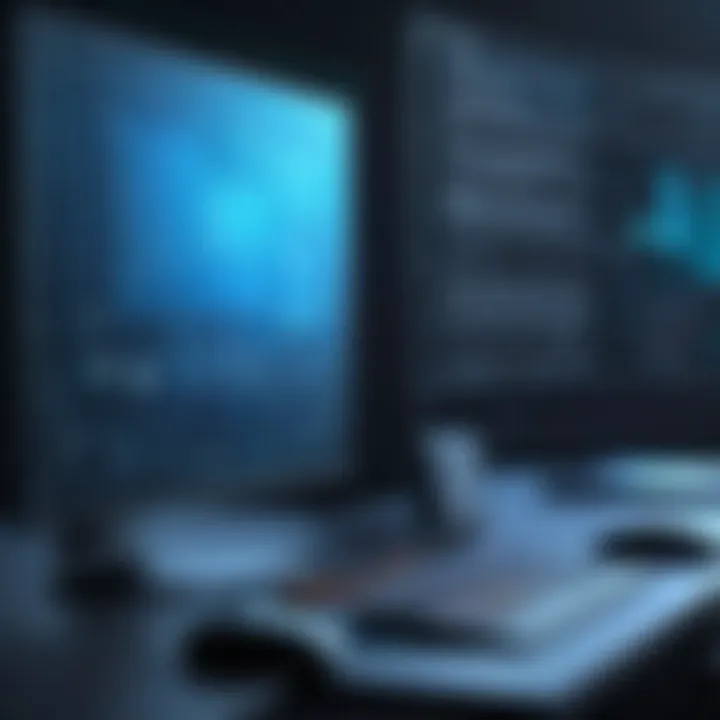
Utilizing STL helps to:
- Increase Productivity: Pre-built data structures save time and effort.
- Enhance Performance: Well-optimized algorithms tailored for various tasks improve application performance.
- Standardization: Employing STL reduces the likelihood of bugs, since these implementations have been tested extensively.
Here's an example of using STL to sort a vector of integers:
Mastering STL can significantly influence interview performance, as you may find it common to solve problems or optimize solutions using STL algorithms. Additionally, familiarity with its functionalities showcases adaptability and comfort with industry standards.
"The STL is often a game changer in technical interviews, showcasing your readiness to write efficient C++ code right out of the gate."
Understanding advanced features in C++ sets candidates apart in interviews and prepares them for complex coding tasks. Thorough knowledge of templates, exception handling, and STL contributes significantly to problem-solving prowess, which employers highly value.
Memory Management in ++
Memory management is a cornerstone of programming in C++. It’s not just a technical necessity; it’s a vital skill that plays a huge role in how efficiently a program runs. The ability to control memory allocation and deallocation can mean the difference between a smooth-running application and a sluggish, error-prone one. Without a robust understanding of memory management, programmers can find themselves in a maze of memory leaks and system crashes.
Effective memory management enables programs to utilize system resources efficiently, offering advantages such as improved performance and reduced overhead costs. As a student or IT professional heading into technical interviews, grasping this information will not only boost your confidence but also equip you with the tools to tackle complex problems. This segment zooms in on crucial elements related to memory management in C++, diving into dynamic memory allocation, smart pointers, and the prevention of memory leaks.
Dynamic Memory Allocation
Dynamic memory allocation is a process whereby a program can request memory during its execution using operators like and . This flexibility is crucial for scenarios where the amount of memory needed is not known beforehand, such as when working with dynamic arrays or linked data structures.
Consider this example:
In the above snippet, the operator allocates memory from the heap, creating an array of integers. Later, when the array is no longer needed, the operator safely frees that memory. Failing to properly deallocate memory can lead to memory leaks, something programmers should take seriously.
Smart Pointers
Smart pointers are a modern solution to managing memory in C++. Instead of manually allocating and deallocating memory, smart pointers automatically manage object lifetimes, significantly reducing the risk of memory leaks. Some common types include , , and .
- std::unique_ptr: Provides exclusive ownership of an object. Only one can own a particular object at any time.
- std::shared_ptr: Allows multiple pointers to share ownership of an object. It keeps a count of how many point to the same object.
- std::weak_ptr: Used to break reference cycles by having a non-owning reference to an object managed by .
For instance:
Utilizing smart pointers enhances code safety and maintainability, particularly during technical interviews where clean code is often assessed.
Memory Leaks and Their Prevention
Memory leaks occur when a program loses the ability to re-access allocated memory. This often happens due to improper handling of dynamic memory. Imagine a scenario where a developer forgets to allocated memory or creates multiple references to the same memory without releasing them. Over time, this can lead to increased memory usage, degrading performance, and possibly crashing programs.
To prevent memory leaks:
- Always pair with (or better yet, use smart pointers).
- Monitor memory usage during development. Tools like Valgrind can help track down memory leaks.
- Adopt proper coding conventions that encourage careful management of memory, particularly during code reviews.
"A problem well-stated is a problem half-solved." This quote rings true in programming as well; articulating issues related to memory can clear the fog in interviews where such questions arise.
In summary, memory management is not merely an option; it's a must for C++ developers. Grasping dynamic memory allocation, leveraging smart pointers for memory safety, and understanding how to prevent memory leaks prepare candidates not only for interviews but also for practical programming challenges in their careers.
Common ++ Technical Interview Questions
In any technical interview for a programming role, candidates often face a variety of C++ questions that test their understanding of the language, its syntax, and core principles. The significance of these questions cannot be overstated, as they serve not only as a litmus test for technical proficiency but also as an insight into a candidate's problem-solving skills and ability to reason through complex issues. By strategically categorizing questions into entry-level, mid-level, and senior-level, interviewers can gauge the depth of knowledge and practical coding abilities of candidates.
Entry-Level Questions
Entry-level questions are designed to assess the foundational understanding of C++ concepts. Typically, candidates can expect to encounter questions about basic syntax, data types, and control structures. For instance, a frequent question might be:
- What is the difference between and in C++?
Such a question looks simple, but it uncovers a candidate's grasp on visibility and data encapsulation. Candidates could articulate that while both structures can hold data and functions, default access specifiers differentiate them—public for structs and private for classes.
Another common query might be about writing simple loops or conditional statements. An example question is:
- Can you write a for loop to print numbers 1 to 10?
Such practical exercises help interviewers gauge not only technical knowledge but also fluency in writing code without stumbling over syntax.
Mid-Level Questions
As candidates progress to mid-level questions, the complexity ramps up, placing an emphasis on understanding concepts such as inheritance, polymorphism, and standard libraries. Questions here might require candidates to demonstrate a concrete understanding and practical application of these principles. For example:
- Explain how polymorphism can be implemented in C++.
An effective answer may incorporate examples of virtual functions and overriding methods. Candidates should be able to provide explanations on the two types of polymorphism—compile-time and runtime—demonstrating their ability to manage and utilize C++ comprehensively.
Additionally, they may encounter scenario-based questions like:
- How would you handle an exception in C++?
This inquiry tests not only knowledge of try-catch blocks but invites a deeper discussion regarding exception safety and proper resource management, showcasing how they think on their feet.
Senior-Level Questions
Senior-level questions are meant to evaluate an applicant's in-depth knowledge and strategic thinking. Typically, these queries incorporate real-world problems to solve or design patterns to describe. An example could be:
- How do you optimize the performance of a C++ application?
This kind of question can lead to discussions about profiling tools, memory usage, data structures, and algorithm complexity. Candidates are encouraged to consider factors such as CPU vs memory trade-offs, cache optimization, and multithreading, thereby demonstrating a layered comprehension of performance considerations.
Another probing question might arise as:
- Discuss the implications of using smart pointers versus raw pointers.
Successful candidates will not only differentiate between shared_ptr, unique_ptr, and weak_ptr but also delve into memory management best practices, encapsulating their understanding in a way that resonates with modern C++ programming standards.
"Technical interviews aren't just about the right answers; they're about demonstrating the thought process behind those answers."
Practical Coding Assessments
Practical coding assessments hold a significant role in the landscape of technical interviews, especially for C++ positions. These assessments act as a critical litmus test measuring not just a candidate’s theoretical understanding of C++, but also their ability to apply concepts to real-world problems. In a field where practical skills are essential, demonstrating proficiency through coding tasks provides insight into a candidate's problem-solving capabilities and adaptability.
Online Coding Platforms and Their Importance
Online coding platforms serve as the arena where candidates can flex their coding muscles. Websites like LeetCode, HackerRank, and CodeSignal have emerged as valuable tools for both candidates and recruiters alike. They offer a variety of problems that range from simple syntax checks to complex algorithmic challenges.
- Ease of Use: These platforms are accessible anytime and anywhere, allowing candidates to practice at their convenience.
- Diverse Problem Sets: Candidates are exposed to a wide array of problems that mimic real interview scenarios, enhancing their adaptability.
- Immediate Feedback: Most platforms provide instant feedback on code submissions, helping candidates learn from their mistakes and improve quickly.
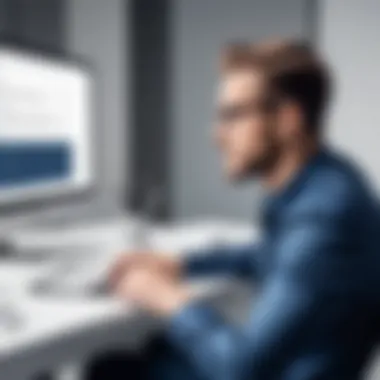
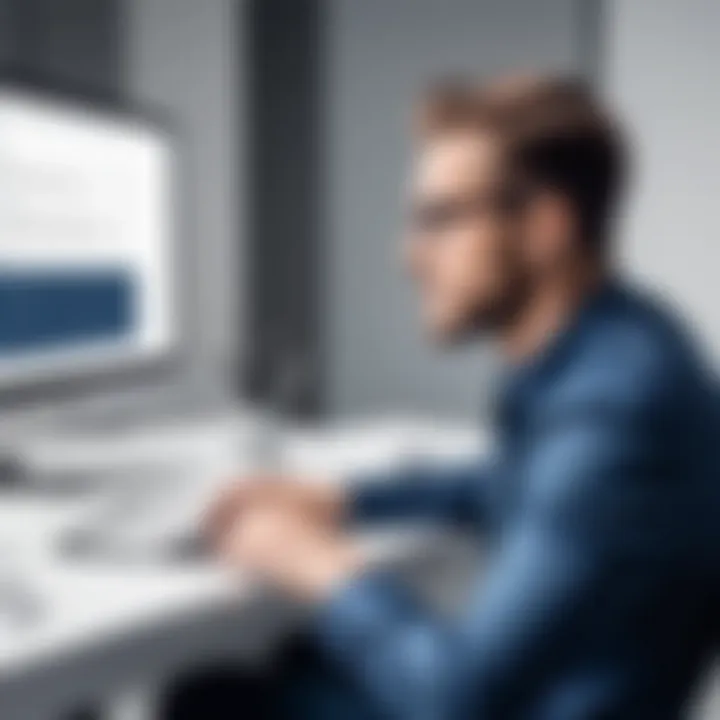
"Practicing on online coding platforms can significantly reduce the anxiety associated with technical interviews."
This practice not only prepares the candidate for the types of questions they may face but also builds their confidence. By getting accustomed to coding under pressure, individuals can tackle interview challenges more effectively.
Types of Coding Problems in Interviews
When it comes to technical interviews, understanding the different types of coding problems is key. These problems often include:
- Array and String Manipulations: These are fundamental challenges that assess basic understanding of data structures.
- Dynamic Programming: Problems leveraging this technique often require breaking down problems into simpler subproblems, a critical skill in a coder’s toolkit.
- Graph and Tree Traversals: Questions involving these structures challenge a candidate’s understanding of complex data organization and navigation.
- Sorting and Searching Algorithms: These problems are staples in interviews and require not just knowledge, but also the ability to implement solutions efficiently.
Each of these categories tests a different set of skills, from logical reasoning to algorithmic efficiency. Focusing practice on these areas will go a long way in preparing for the unpredictable nature of coding assessments.
Time and Space Complexity Considerations
When engaging with coding problems, one must also consider time and space complexity. This is an area where many candidates falter, often overlooking its importance during interviews. Understanding the efficiency of algorithms is crucial, as interviewers frequently evaluate candidates not only on correct solutions but also on optimized performance.
- Big O Notation: This is an essential measure used to describe the efficiency of algorithms. Candidates should be able to articulate both the time complexity and space complexity of their solutions.
- Trade-offs: Sometimes, a more time-efficient solution may require more memory, or vice versa. Being able to discuss these trade-offs shows depth of understanding.
In summary, mastering the intricacies related to time and space complexity stands as a pivotal skill that distinguishes adept programmers from the rest. Candidates who can think critically about their approach and articulate their reasoning will often make a lasting impression in interviews.
Behavioral Aspects of Technical Interviews
When it comes to technical interviews, especially in the realm of C++, the focus often lies squarely on coding abilities and problem-solving skills. However, ignoring the behavioral aspect would be a grave mistake. The way candidates communicate and interact can weigh heavily in the decision-making process. Behavioral aspects encompass not just how one responds to questions, but also how they present their thoughts, manage stress, and collaborate with others.
Soft Skills and Communication
Soft skills, a term that has become quite popular, refers to personal attributes that enable someone to interact effectively and harmoniously with other people. In a technical interview for a C++ position, these skills are paramount. Interviewers don’t just seek a candidate with the mightiest algorithm; they also want someone who can articulate their thought process clearly.
Effective communication begins with listening. A candidate should demonstrate an understanding of the questions asked before jumping into solutions. It’s important to frame answers in a structured way. For instance, using a simple format like STAR—Situation, Task, Action, Result—can aid in making responses concise and relevant. This method helps in sharing relevant anecdotes from past experiences without going off on a tangent.
Moreover, showing enthusiasm and genuine interest in the role can set a candidate apart. Instead of responding to questions robotically, infusing a personal touch and connecting the answer back to the specific job or company can paint a vivid picture.
Skills like empathy can often be overlooked. Yet, understanding the perspectives of the interviewers and acknowledging their expertise can pave a smoother path during discussions.
Problem-Solving Approach
In many technical interviews, problem-solving remains at the forefront, showcasing a candidate's ability to think critically under pressure. However, it's not solely about arriving at the right answer; how problems are approached can be equally telling.
Recognizing that a good problem-solving process is often iterative allows candidates to remain flexible and open-minded. When faced with a challenging C++ question, instead of fixating on one path, a candidate might benefit from outlining multiple potential solutions. This not only demonstrates analytical skills but also shows adaptability and creativity in thinking.
Candidates should articulate their problem-solving strategies aloud. A clear presentation of their thought process, even when stuck, can often reflect positively on them. Regarding C++, discussing the nuances of different solutions—like choosing between raw pointers and smart pointers for memory management—can also offer additional insight into their depth of knowledge.
Finally, after proposing a solution, it's invaluable to reflect on its feasibility. Questions like "Are there edge cases I’ve considered?" or "How efficient is this method?" signal to interviewers that the candidate is thinking critically beyond just solving the immediate problem.
"In interviews, the journey is just as important as the destination."
These behavioral qualities are not just checkboxes; they genuinely influence how candidates are perceived. The blend of soft skills and a structured problem-solving approach can be the deciding factor in the competitive tech landscape.
Best Practices for Interview Preparation
Preparing for technical interviews, especially in C++, requires a strategic approach. This section outlines effective best practices that can significantly enhance your chances of success.
A solid preparation plan not only boosts your confidence but also equips you with the essential tools needed to tackle challenging interview questions.
The primary elements to focus on include:
- Developing a structured study plan to guide your preparations.
- Engaging in mock interviews that simulate real interview conditions, allowing you to practice under pressure.
- Identifying and bridging knowledge gaps to ensure you cover all relevant topics in C++.
By employing these methods, candidates can create a robust framework for their interview success.
Structured Study Plan
A structured study plan is like a road map in your interview journey. It outlines where you need to go and how to get there efficiently. Begin by assessing your current level of understanding in C++. This assessment allows you to identify strong areas and weak spots, refining your focus.
To create an effective study plan, consider the following steps:
- Set clear goals: Define what you wish to achieve by the end of your preparation. This could be mastering specific topics or practicing certain coding questions.
- Create a schedule: Divide your preparation into daily or weekly segments, allocating specific topics to study. For instance, dedicate a week to object-oriented programming and another to memory management.
- Use varied resources: Combine textbooks, online courses, and coding platforms to expose yourself to diverse learning materials. This diverse approach aids in understanding concepts from multiple perspectives.
- Keep track of progress: Monitor your growth by revisiting your goals, adjusting your plan as necessary to stay on target.
By structuring your study plan, you not only enhance your learning but also streamline your preparation process.
Mock Interviews
Mock interviews serve as a critical component of preparing for technical interviews. They offer a platform to practice articulating thoughts clearly and efficiently under pressure.
Some essential tips for conducting mock interviews include:
- Simulating real interview conditions: Arrange for an external person to conduct the interview, fostering a genuine environment.
- Time management: Practice answering questions within specified time limits to improve your pacing.
- Feedback collection: After the mock session, obtain constructive feedback. This feedback is invaluable as it highlights areas for improvement.
Utilizing mock interviews can significantly reduce anxiety during the actual interview and bolster your self-assurance and communication skills.
Identifying and Bridging Knowledge Gaps
Identifying knowledge gaps is crucial for a well-rounded preparation. The process should start with reflecting on topics you find challenging or unfamiliar. For instance, if C++ templates or exception handling seem daunting, focus on them during your studies.
Here are practical steps to bridge these gaps:
- Self-assessment: Regularly test your knowledge through quizzes or coding problems. This helps pinpoint areas requiring further attention.
- Study resources: Utilize resources like Wikipedia or coding communities on platforms like Reddit to gather information and tips from experienced programmers.
- Group study sessions: Collaborate with peers who are also preparing. Discussing complex topics can clarify doubts and reinforce your understanding.
By actively seeking to identify and address knowledge gaps, candidates will be better positioned to tackle a variety of questions that may arise during interviews.
End
In wrapping up this guide, it becomes clear that understanding C++ technical interview questions is not just about memorizing answers but grasping the underlying concepts that make C++ a powerful language. This article has journeyed through fundamental principles, advanced features, and practical coding assessments, all tailored for an audience eager to excel in technical interviews. Reflecting on the various sections, we spotlighted essentials from syntax to object-oriented design, emphasizing the importance of solidifying one's foundation before advancing to complex queries.
Recap of Essential Points
A good grasp of C++ not only prepares one for interviews but also enhances problem-solving abilities in real-world scenarios. Here’s a quick rundown of the critical elements covered in the earlier sections:
- Fundamental Concepts: We dug deep into basic syntax, data types, and operators, providing a framework that job candidates can build upon.
- OOP Principles: Concepts like inheritance, polymorphism, encapsulation, and abstraction were explored, helping candidates articulate their understanding of C++’s object-oriented nature during personal interviews.
- Advanced Features: The inclusion of templates, exception handling, and the Standard Template Library (STL) prepares candidates to tackle specialized questions with confidence.
- Memory Management: A thorough examination of dynamic memory allocation, smart pointers, and memory management helps shine a light on common pitfalls in C++ programming.
- Practical Application: We also discussed the relevance of coding assessments, along with timing complexity, which is essential for technical evaluations.
Encouragement for Continuous Learning
It's vital for students, budding programmers, and IT professionals to keep the flame of learning alive. While this guide serves as a compass in navigating C++ technical questions, it shouldn't be the full stop in one's knowledge quest. Here are a few methods to encourage ongoing growth in understanding C++ and preparing for interviews:
- Practice Regularly: Platforms like LeetCode and HackerRank can help strengthen coding skills. Tackling new problems frequently ensures that you remain sharp and ready.
- Collaborate with Peers: Discussing problems and working on coding challenges alongside others can expose candidates to different thought processes and solutions.
- Stay Updated: The tech world evolves rapidly. Following forums like Reddit’s programming community or engaging with resources on Wikipedia or Britannica can keep you informed on trends and techniques.
- Engage with the Community: Participating in coding competitions and open-source projects can foster both skills and industry connections.
"Learning is a treasure that will follow its owner everywhere."
This quote holds particularly true in the world of programming, where knowledge continually expands and evolves. By fostering a mindset geared toward continuous improvement, candidates not only bolster their abilities but also enhance their marketability in a competitive job landscape.