Building a Powerful API with Node.js: A Comprehensive Guide
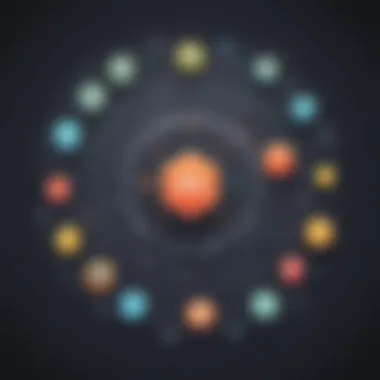
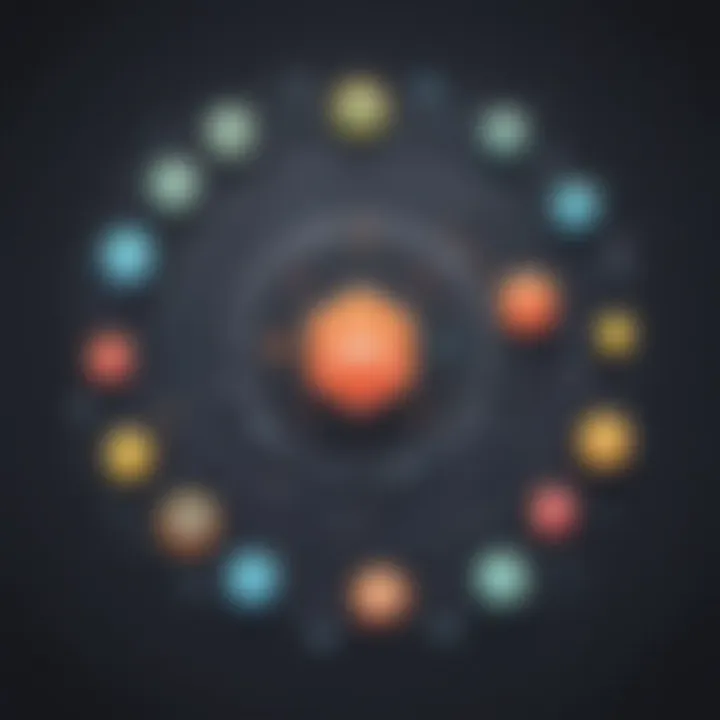
Overview of Topic
In the vast realm of web development, Node.js stands out as a powerful tool for creating robust APIs. This article delves deep into the intricacies of harnessing Node.js to craft efficient APIs that drive seamless interactions between applications. Understanding the core concepts and practical applications of Node.js API development is crucial for individuals delving into the programming landscape. Let's explore the foundational aspects and advanced techniques that underscore the creation of potent APIs using Node.js.
Fundamentals Explained
To embark on the journey of creating a potent API with Node.js, one must grasp the core principles that underpin this process. Node.js, known for its event-driven architecture and non-blocking IO operations, empowers developers to build high-performance applications. Understanding key terminologies such as endpoints, middleware, and routing is essential when venturing into API development with Node.js. Familiarizing oneself with these fundamental concepts lays a solid groundwork for building efficient APIs that cater to diverse application requirements.
Practical Applications and Examples
Real-world applications serve as essential guides for aspiring Node.js developers looking to hone their API creation skills. Exploring case studies showcasing successful API implementations using Node.js provides invaluable insights into best practices and common pitfalls to avoid. Hands-on projects allow developers to code along, implementing various routes, handling requests, and integrating middleware to enhance API functionality. By examining code snippets and following implementation guidelines, individuals can concretely apply theoretical concepts to practical scenarios, fostering a deeper understanding of Node.js API development.
Advanced Topics and Latest Trends
Adopting cutting-edge practices is imperative in the ever-evolving landscape of API development with Node.js. Stay abreast of advanced techniques such as caching mechanisms, authentication protocols, and data validation strategies to enhance API security and efficiency. Embracing the latest trends, like GraphQL for querying API data and WebSocket for real-time communication, can revolutionize the way APIs are designed and utilized. Exploring the future prospects of Node.js API development sheds light on upcoming trends such as serverless architecture and microservices, paving the way for innovative API solutions in the digital age.
Tips and Resources for Further Learning
For individuals seeking to deepen their expertise in Node.js API development, a plethora of resources are available. Recommended books like 'Node.js Design Patterns' and 'RESTful Web API Design with Node.js' offer invaluable insights into advanced Node.js concepts and best practices. Online courses on platforms like Udemy and Coursera provide structured learning opportunities to enhance API development skills. Leveraging tools like Postman for API testing and documentation streamlines the development workflow, ensuring the creation of efficient and reliable APIs with Node.js.
Introduction
In this enthralling article, we delve into the intricacies of crafting a potent API using Node.js, a highly favored JavaScript runtime environment in the developers' community today. From the inception of setting up the environment to executing routes and adeptly handling requests, this comprehensive guide paves the way for readers, facilitating the acquisition of knowledge and proficiency in building efficient APIs.
Understanding Node.js
Overview of Node.js
Embarking on the journey of understanding Node.js, it becomes evident that this runtime environment allows for asynchronous event-driven programming, equipped with a plethora of libraries extending its functionality. The standout characteristic of Node.js lies in its single-threaded event-loop model, captivating developers with its capacity to handle a large number of concurrent connections. This uniqueness positions Node.js as a preferred choice for API development due to its scalability and non-blocking IO operations that enhance performance.
Advantages of using Node.js for API development
Analyzing the advantages of leveraging Node.js for API development, its ability to facilitate real-time functionalities and speedy execution stands out prominently. Node.js streamlines the development process by enabling the sharing of code between the server and client-side, promoting efficiency and collaboration. Moreover, its vibrant community and robust ecosystem contribute to the seamless integration of third-party modules, bolstering the developer's toolkit and fostering innovation.
Node.js ecosystem
Within the expansive Node.js ecosystem, a myriad of modules, packages, and frameworks await exploration, assuring developers of unmatched flexibility and customization options. The Node Package Manager (NPM) serves as a treasure trove of resources, empowering developers to effortlessly install and manage dependencies, underscoring the convenience and efficiency of Node.js development. The adaptability and extensive support within the Node.js ecosystem position it as a strategic choice for building APIs, offering a conducive environment for growth and scalability.
What is an API?
Definition of API
Stepping into the realm of API definition, an API (Application Programming Interface) serves as a conduit for interactions between different software applications, facilitating seamless communication and data exchange. The defining attribute of an API lies in its role as a bridge, enabling the integration of disparate systems and services with uniformity and consistency. The indispensability of APIs in modern software development stems from their efficacy in fostering interoperability and enhancing efficiency.
Types of APIs
Delving into the classification of APIs, a distinction emerges between open APIs, internal APIs, and partner APIs, each tailored to specific use cases and audience requirements. Open APIs, accessible to external developers, promote innovation and collaboration by granting access to proprietary software functionalities. Internal APIs, shielded within organizational boundaries, streamline communication between different departments, enhancing operational cohesiveness.
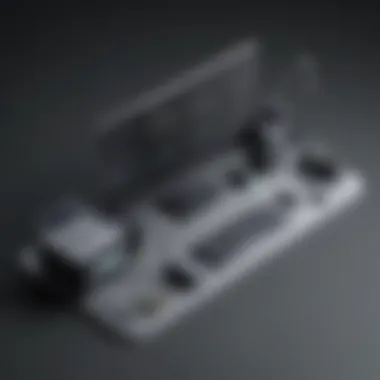
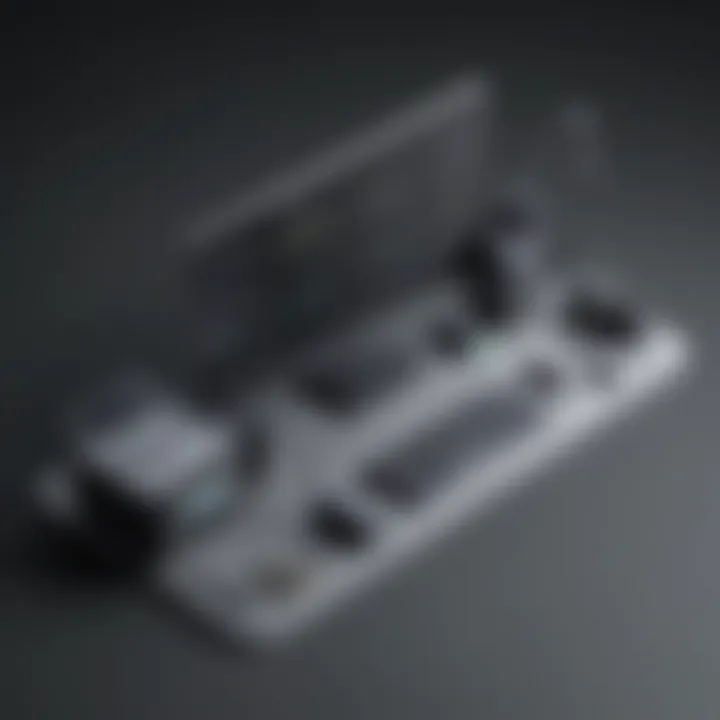
Importance of APIs in software development
Highlighting the significance of APIs in software development, their role in accelerating the pace of innovation and facilitating system integration cannot be overstated. APIs act as the backbone of modern applications, enabling seamless interaction between diverse platforms and services, thereby enriching user experience and functionality. Embracing APIs in software development embodies a strategic approach towards interoperability and scalability, emboldening developers to create robust and dynamic solutions.
Setting Up Environment
Installing Node.js
Downloading Node.js
Downloading Node.js is a pivotal task in the process of setting up the environment for API development using Node.js. This step involves obtaining the latest version of Node.js, which is essential for leveraging the features and functionalities required for building robust APIs. The download process is straightforward and commonly chosen due to the extensive community support and frequent updates that Node.js receives. Utilizing the best features of Node.js after downloading it significantly enhances the performance and scalability of the API being developed.
Node.js Installation Process
The Node.js installation process is a fundamental aspect of setting up the development environment. It involves installing Node.js on the system, configuring settings, and ensuring compatibility with other tools and libraries essential for API development. The simplified installation procedure of Node.js streamlines the setup process, enabling developers to kickstart API projects quickly and efficiently. This installation method is preferred for its ease of use and seamless integration with various operating systems and development environments.
Verifying Installation
Verifying the successful installation of Node.js is a critical verification step within the environment setup process. By confirming the installation's accuracy, developers can avoid potential errors and conflicts during API development. Verifying installation ensures that Node.js is correctly installed and ready to be utilized for creating powerful APIs. This validation step adds a layer of quality control to the setup process, guaranteeing a stable foundation for API development.
Initial Project Setup
Creating a New Node.js Project
Creating a new Node.js project marks the beginning of the API development journey. This step involves initializing the project structure, setting up dependencies, and establishing a core framework for the API. The creation of a new Node.js project is essential for organizing code, managing resources, and facilitating collaboration among team members. By initiating a new project, developers set the stage for efficient API development and future scalability.
Installing Necessary Dependencies
Installing necessary dependencies is a vital step in the initial project setup phase. This task involves adding essential libraries, modules, and packages to the Node.js project to enhance its functionality and streamline development processes. Selecting and installing the right dependencies are crucial for achieving optimal performance and effectiveness in API development. By integrating necessary dependencies, developers ensure that the project has access to required features and tools for successful API implementation.
Project Structure
Establishing a well-defined project structure is a foundational element of setting up the initial environment for API development. The project structure outlines the organization of code files, directories, and resources within the Node.js project. A clear and logical project structure simplifies navigation, maintenance, and debugging tasks throughout the API development lifecycle. By defining a structured project layout, developers promote code consistency, scalability, and code reusability, which are essential for building robust and maintainable APIs.
Building the API
Implementing Routes
Defining API endpoints
One of the fundamental aspects of API development highlighted in this article is the concept of defining API endpoints. These endpoints act as gateways for client-server communication, specifying the resources available and the actions that can be performed. By clearly defining API endpoints, developers can streamline the interaction process, enhance security measures, and facilitate data retrieval and manipulation. The unique feature of defining API endpoints lies in its ability to structure API functionality effectively, ensuring seamless integration across different platforms and services. While offering numerous advantages in terms of flexibility and scalability, defining API endpoints requires careful planning to avoid potential pitfalls such as endpoint redundancy or insufficient resource allocation.
Handling different HTTP methods
Another critical aspect addressed in the API development process is handling different HTTP methods. This functionality allows developers to support various types of requests, such as GET, POST, PUT, and DELETE, enabling precise control over data manipulation and retrieval. By incorporating support for different HTTP methods, developers can enhance the flexibility and versatility of their APIs, catering to a wide range of client requirements. The key characteristic of handling different HTTP methods is its adaptability to diverse use cases, providing developers with the tools necessary to create dynamic and interactive APIs. While offering notable benefits in terms of compatibility and extensibility, handling different HTTP methods requires careful validation and error handling to maintain data integrity and security standards.
Setting up route parameters
In the context of API development with Node.js, setting up route parameters plays a crucial role in customizing endpoint functionality and enhancing data processing capabilities. Route parameters allow developers to define dynamic segments within endpoints, enabling the extraction of specific data elements from client requests. By setting up route parameters effectively, developers can create reusable and scalable routes that accommodate varying input requirements, promoting code reusability and maintenance efficiency. The unique feature of route parameters lies in their ability to facilitate parameterized routing, enabling developers to handle complex data structures with ease. While offering advantages in terms of flexibility and scalability, setting up route parameters necessitates thorough input validation and error handling to mitigate potential security risks and data inconsistencies.
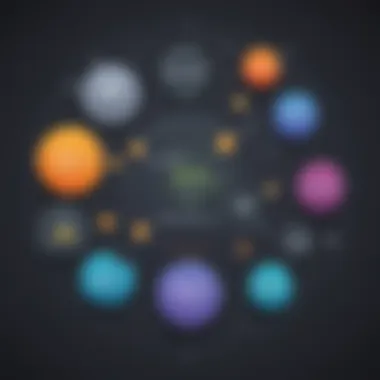
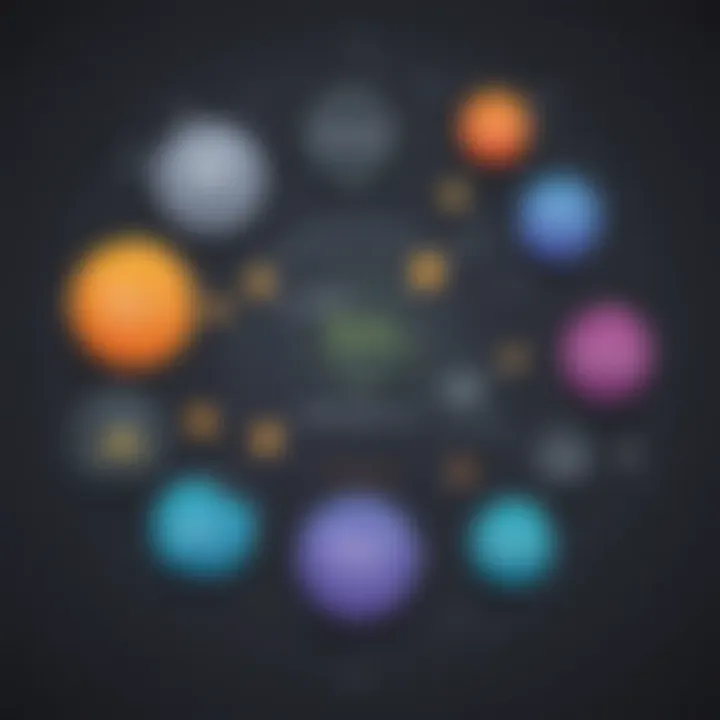
Data Management
Data management plays a crucial role in developing a powerful API with Node.js. Efficient data management ensures that the API interacts smoothly with the underlying database, promoting scalability and performance. By organizing, storing, and retrieving data effectively, developers can enhance the overall functionality of the API. Considering the vast amounts of data that APIs handle, robust data management practices become essential to maintain data accuracy, security, and integrity.
Connecting to a Database
Choosing a database system
Selecting the appropriate database system is a critical decision in API development with Node.js. The choice of database significantly influences the API's performance, scalability, and efficiency. For this article, emphasizing the selection process aims to guide developers in choosing a database that aligns with the project requirements. Factors such as data structure, query complexity, and scalability requirements will impact the decision-making process, ultimately impacting the API's functionality and responsiveness.
Establishing database connection
Establishing a secure and reliable connection to the selected database is vital for seamless API operation. Node.js offers various modules and drivers that facilitate an efficient connection setup, ensuring quick data access and manipulation. By establishing a robust database connection, developers can optimize data retrieval, storage, and update processes, enhancing the API's overall performance and responsiveness.
CRUD operations
CRUD (Create, Read, Update, Delete) operations form the core functionalities of an API's interaction with the database. Implementing CRUD operations efficiently allows for seamless data manipulation, retrieval, and update within the API endpoints. By incorporating CRUD operations effectively, developers can ensure systematic data management, data consistency, and maintain the integrity of the API's functionalities and data flow.
Data Validation
Data validation serves as a critical aspect of ensuring data accuracy and security within the API. By validating incoming data based on predefined rules and constraints, developers can prevent data corruption, unauthorized access, and potential security breaches. Utilizing robust data validation techniques enhances the API's reliability, data integrity, and overall security posture.
Implementing validation checks
Implementing validation checks involves defining rules and criteria to verify the integrity and validity of incoming data. By enforcing validation checks at various stages of data processing, developers can ensure that only accurate and authorized data enters the system. Effective implementation of validation checks enhances data quality, reduces errors, and strengthens the API's resilience against various data-related vulnerabilities.
Ensuring data integrity
Ensuring data integrity focuses on maintaining the consistency, accuracy, and reliability of stored data. By employing mechanisms to validate and enforce data integrity constraints, developers can prevent data inconsistency, duplication, and unauthorized alterations. Ensuring data integrity within the API safeguards sensitive information, promotes reliable data processing, and fosters trust among API users.
Utilizing validation libraries
Utilizing validation libraries offers developers access to tested and optimized validation tools and functionalities. Integration of validation libraries simplifies the data validation process, reduces development time, and enhances the security and reliability of data handling within the API. By leveraging established validation libraries, developers can streamline data validation processes, improve code quality, and fortify the API against potential data vulnerabilities.
Securing the API
Securing the API is a critical aspect when developing APIs with Node.js. In this article, we delve into the vital importance of implementing robust security measures to protect the API and data it processes. Securing the API not only safeguards sensitive information but also enhances trust among users and clients. By focusing on security, developers can mitigate the risks of potential cyber threats and unauthorized access, ensuring the integrity and confidentiality of the API ecosystem.
Authentication and Authorization
Implementing user authentication
Implementing user authentication is pivotal in verifying the identity of users accessing the API. In this context, user authentication plays a central role in ensuring that only authorized individuals can interact with the API. By incorporating user authentication mechanisms, developers can establish secure user credentials validation, reducing the likelihood of unauthorized access. The implementation of user authentication strengthens the overall security posture of the API, bolstering user trust and data protection.
Role-based access control
Role-based access control provides a structured approach to managing user permissions within the API framework. By assigning specific roles to users based on their responsibilities and functions, developers can control and restrict access to different parts of the API. This granular level of access control enhances security by preventing unauthorized actions and data exposure. Role-based access control empowers administrators to define and enforce access rights effectively, promoting a secure and compliant API environment.
Token-based security
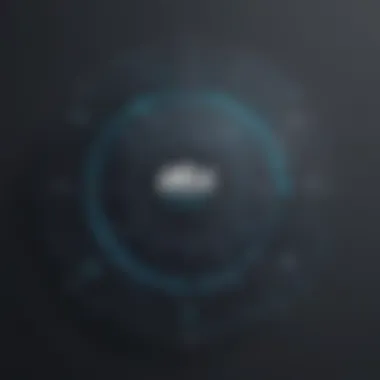
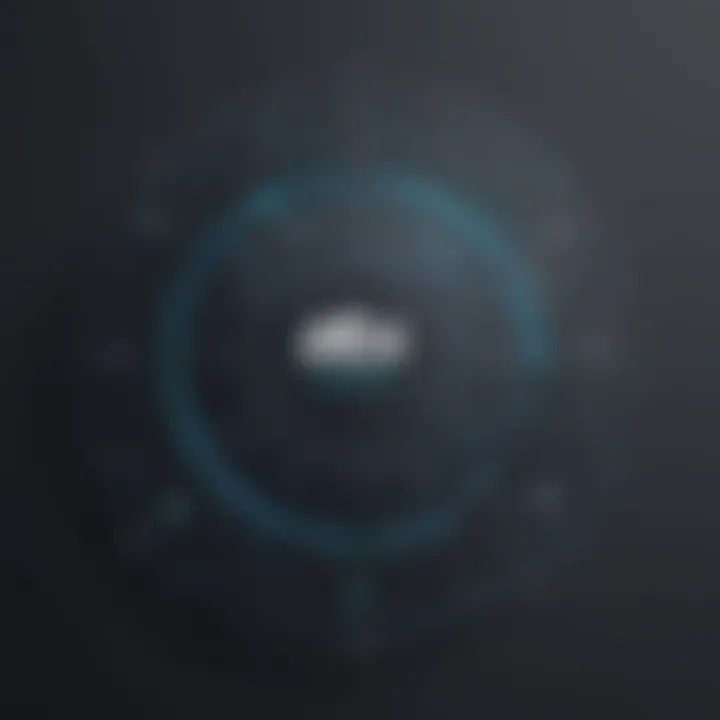
Token-based security offers a sophisticated method for authenticating and authorizing API interactions. By utilizing tokens as credentials for accessing protected resources, developers can enhance security without compromising user experience. Tokens serve as unique identifiers that validate user identity and permissions, reducing the reliance on traditional authentication methods. The use of token-based security not only streamlines the authentication process but also strengthens API security by minimizing the risk of credential misuse or theft.
Protecting Against Common Security Threats
Preventing CSRF attacks
Preventing Cross-Site Request Forgery (CSRF) attacks is imperative to mitigate malicious exploits that manipulate user sessions. By implementing CSRF token validation and secure session handling, developers can prevent unauthorized actions initiated by forged requests. Protection against CSRF attacks reinforces the integrity of user interactions with the API, safeguarding against unauthorized changes or data breaches.
Securing against injection attacks
Securing against injection attacks involves protecting the API from malicious code injections that can compromise data integrity. By validating and sanitizing input parameters, developers can defend against SQL injection and other injection vulnerabilities. Robust input validation mechanisms coupled with secure coding practices fortify the API against injection attacks, reducing the risk of data manipulation or exfiltration.
Implementing HTTPS
Implementing Hypertext Transfer Protocol Secure (HTTPS) encryption is essential for encrypting data exchanged between clients and the API server. By enabling HTTPS, developers can ensure data confidentiality, integrity, and authenticity during information transmission. HTTPS encryption prevents eavesdropping and tampering attacks, enhancing the overall security posture of the API ecosystem. The adoption of HTTPS protocols instills trust in users by demonstrating a commitment to data protection and secure communication channels.
Testing and Deployment
Writing Unit Tests
Importance of testing in API development
Testing plays a crucial role in the seamless operation of APIs, ensuring that each endpoint functions correctly and efficiently. The importance of testing in API development lies in its ability to unveil potential bugs and errors, thereby enhancing the overall quality of the API. By testing the API under different conditions, developers can identify and rectify issues early in the development process, leading to a more stable and reliable product. This meticulous approach not only boosts the API's performance but also instills confidence in its usability.
Setting up test frameworks
Setting up test frameworks streamlines the testing process, providing developers with a structured environment to conduct a variety of tests. These frameworks offer a systematic approach to organizing test cases, simplifying the overall testing procedure. By utilizing test frameworks, developers can efficiently conduct unit tests, integration tests, and end-to-end tests, ensuring comprehensive test coverage and accurate results. The adoption of test frameworks enhances the efficiency of the testing phase, promoting faster identification and resolution of potential issues.
Creating test cases
Creating test cases involves defining specific scenarios to evaluate the functionality and performance of the API. Each test case assesses a particular aspect of the API, focusing on inputs, outputs, and expected outcomes. By crafting comprehensive test cases, developers can ascertain the API's adherence to specifications and its ability to handle diverse situations. Test cases serve as a roadmap for validating the API's behavior, guiding developers in uncovering deviations from expected results and refining the API's functionality. Through meticulously designed test cases, developers ensure the integrity and reliability of the API, reinforcing its quality and performance.
Deploying the API
Choosing a hosting service
Selecting an appropriate hosting service plays a crucial role in the successful deployment of the API, determining factors such as performance, scalability, and security. The chosen hosting service should align with the requirements of the API, offering reliable server infrastructure and optimal resource allocation. By evaluating hosting services based on criteria such as uptime, customer support, and pricing, developers can make an informed decision regarding the platform that best fits their deployment needs.
pipelines
Continuous IntegrationContinuous Deployment (CICD) pipelines automate the deployment process, enabling swift and efficient updates to the API. CICD pipelines streamline the integration of code changes, as well as the testing and deployment of new features, ensuring a seamless development workflow. By implementing CICD pipelines, developers minimize the risk of deployment errors, enhance collaboration among team members, and accelerate the release of updates to the API.
Monitoring and scaling
Monitoring and scaling are essential components of deploying a successful API, ensuring its performance and availability under varying loads. Continuous monitoring helps detect issues proactively, enabling quick responses to potential downtimes or performance bottlenecks. Scaling ensures that the API can accommodate increasing user demands by allocating resources dynamically, maintaining optimal performance levels. By implementing robust monitoring and scaling strategies, developers can enhance the reliability and scalability of the API, thus delivering a seamless user experience.
Conclusion
Summary
- Recap of key points: Reflecting on the core components reiterated in the article plays a pivotal role in reinforcing the fundamental principles of API development. The emphasis on structuring routes effectively, handling requests proficiently, and ensuring robust data management underscores the essence of creating a scalable and reliable API architecture.
- Impact of a well-designed API: The impact of a well-designed API extends beyond immediate functionalities, influencing scalability, security, and user satisfaction. A well-structured API improves interoperability, enhances data protection measures, and optimizes performance, aligning the API with industry best practices and standards.
- Future possibilities: Delving into potential advancements and innovations in API development opens doors to explore emerging technologies, integrations, and practices. Anticipating future trends allows developers to adapt proactively, integrating new features, enhancing security measures, and optimizing performance to meet evolving user demands and market requirements.
Final Thoughts
- Encouragement for further exploration: Encouraging continuous learning and experimentation fosters a culture of innovation and growth within the development community. Exploring new techniques, tools, and methodologies fuels creativity and progression, leading to the discovery of novel solutions to complex challenges.
- Continuous learning in tech: Emphasizing the importance of continuous learning in technology highlights the dynamic nature of the IT industry. Staying updated with latest trends, practices, and advancements enables professionals to remain competitive, adaptable, and proficient in navigating the ever-evolving landscape of programming and software development.