Master Data Structures and Algorithms with Python
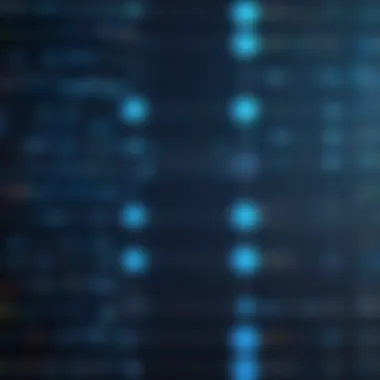
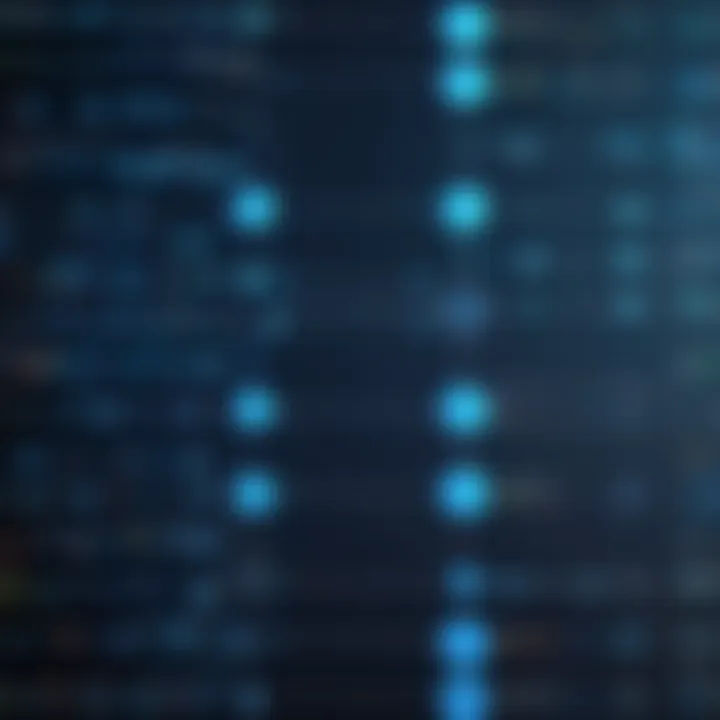
Overview of Topic
In today's fast-paced tech landscape, data structures and algorithms play a crucial role in shaping how programmers and developers tackle complex problems. They are not just abstract concepts; they form the backbone of efficient programming and can greatly affect application performance.
Understanding these foundational elements is akin to equipping oneself with an arsenal of tools necessary to navigate the coding world. From novice programmers to seasoned IT professionals, grasping data structures and algorithms is paramount.
This exploration will not only shed light on core concepts but also delve into significant historical developments that have influenced current programming practices. The journey through data structures and algorithms in Python provides insights not only into theoretical frameworks but also into practical applications that breathe life into programming.
Fundamentals Explained
Data structures refer to ways of organizing and storing data so it can be accessed and modified efficiently. Algorithms, on the other hand, are step-by-step instructions for processes to achieve a certain goal.
Core Principles and Theories
- Efficiency and Complexity: Understanding time and space complexity is key. It helps in optimizing algorithms to think from the perspective of scalability.
- Data Handling: Various structures like arrays, linked lists, stacks, and queues offer distinct advantages for specific scenarios.
- Optimization: Leveraging the right structures can dramatically decrease run time and memory usage.
Key Terminology and Definitions
- Array: A collection of items stored at contiguous memory locations.
- Stack: A linear structure that follows the Last In, First Out (LIFO) principle.
- Queue: A linear structure that follows the First In, First Out (FIFO) principle.
Basic Concepts and Foundational Knowledge
A solid grasp of these principles is vital. For instance, sorting algorithms, such as quicksort and mergesort, help in putting data in order efficiently, while searching algorithms, like binary search, enable quick access to data.
Practical Applications and Examples
Real-world applications of these concepts are everywhere. From databases to web applications and beyond, mastering data structures can significantly enhance your programming capabilities.
Real-world Case Studies and Applications
- Web Development: Efficient data handling through trees can improve search functionalities in large databases.
- Game Development: Understanding graph traversal enhances artificial intelligence in gaming.
Demonstrations and Hands-on Projects
This snippet sets up a basic binary tree structure, a fundamental concept in understanding data hierarchies.
Advanced Topics and Latest Trends
As programming languages evolve, so do the techniques related to data structures and algorithms. Today, we see a surge in the use of machine learning algorithms and complex data structures like heaps and hash tables.
Cutting-edge Developments in the Field
- Machine Learning: Leveraging vast datasets efficiently requires knowledge of advanced data structures.
- Big Data Handling: Enhanced structures for storing and processing large volumes of data.
Future Prospects and Upcoming Trends
As industries adopt artificial intelligence, the relevance of algorithms that can handle complex data increases. Staying updated with these patterns is essential.
Tips and Resources for Further Learning
Embarking on this journey requires not only dedication but also quality resources. Here are some invaluable tools:
- Recommended Books: "Introduction to Algorithms" by Thomas H. Cormen and "Data Structures and Algorithms in Python" by Robert T. Muller.
- Online Courses: Platforms like Coursera and edX offer courses tailored to Python programming.
- Free Resources: Websites like Wikipedia, Reddit, and Facebook can provide communities and discussions for learners.
"Programming is not about what you know; itâs about what you can figure out."
Arming yourself with the knowledge of data structures and algorithms now is not just a means for securing a career; it's a vital investment in adapting to an ever-evolving technological landscape.
Prelude to Data Structures and Algorithms
In the world of programming, data structures and algorithms serve as the backbone of efficient software development. Understanding these fundamental concepts not only empowers developers to write better code but also enhances their problem-solving abilities. When dealing with a large volume of data or complex operations, knowing the right data structure to use can be the difference between a smooth-running application and a sluggish one. This article serves as a comprehensive guide to explore these concepts using Python, targeting beginners and seasoned developers alike who wish to deepen their knowledge.
Defining Data Structures
At its core, a data structure is a specialized means to organize and store data for efficient access and modification. Think of it like the way you organize your bookshelf; using a series of categories helps you find books faster. In programming, common data structures include lists, tuples, sets, and dictionaries, each tailored for different needs. For instance, if you need a collection that doesnât allow duplicates, a set would be the way to go. It helps to keep your code clean and enhances performance.
Understanding Algorithms
An algorithm, on the other hand, is a step-by-step procedure or formula for solving a problem. Imagine a recipe: it requires specific ingredients and steps to produce a dish. Algorithms in programming operate similarly, taking an input and producing an output based on defined operations. They serve various purposes, from sorting data to making calculations, and understanding their underlying principles is crucial for any programmer.
The Importance of Data Structure in Programming
Using appropriate data structures directly impacts the speed and efficiency of an algorithm. Consider this: a square peg in a round hole won't fit well, and likewise, choosing the wrong data structure can lead to inefficient algorithms that take up extra time and resources.
"Choosing the right data structure is crucial to algorithm efficiency."
The benefits include:
- Efficiency: The right structure ensures quicker data manipulation.
- Scalability: Good data organization allows programs to handle growth in data size.
- Readability: Well-structured code is easier for others (and future you) to understand.
When these concepts come together, they create a solid foundation for developing robust and efficient programs. Learning how to integrate data structures with algorithms is not just an academic exercise; itâs a vital skill necessary for building proficient data-driven applications.
Setting Up Your Python Environment
Setting up your Python environment is a foundational step that cannot be overlooked. Itâs akin to laying the first bricks when constructing a building. Without a solid base, everything that follows could crumble. This segment aims to guide you through the essential elements of configuring your Python environment, helping simplify your learning process and allowing you to focus on mastering data structures and algorithms.
Installing Python
The first order of business is to install Python. This open-source programming language offers an incredible array of features, making it a suitable choice for everything from web development to data analysis. To get started, you can download the installer from the official Python website. Choose the version that is compatible with your operating system, whether it be Windows, macOS, or Linux.
Once downloaded, follow the installation prompts. It's vital to check the box that says "Add Python to PATH"; this will save you from having to navigate to the Python directory every time you want to run a script. In case youâre unsure, hereâs a quick recap of commands to confirm the installation:
If the command returns a version number, congratulations! You are on the right track.
Choosing an IDE or Text Editor
With Python successfully installed, the next step involves selecting an Integrated Development Environment (IDE) or a text editor that best suits your needs. There are multiple options available, each having its own set of features and advantages.
- PyCharm: A popular choice among professionals, PyCharm offers a wide range of features tailored for Python development, including code completion and debugging.
- Visual Studio Code: A lightweight yet powerful option, VS Code supports various extensions that enhance its Python capabilities.
- Jupyter Notebook: Particularly useful for data science, Jupyter allows you to write and execute code in incremental segments, which is great for testing out algorithms interactively.
While selecting an IDE, consider factors such as ease of use, support for libraries, and built-in functionalities. Donât hesitate to experiment with a few before settling down.
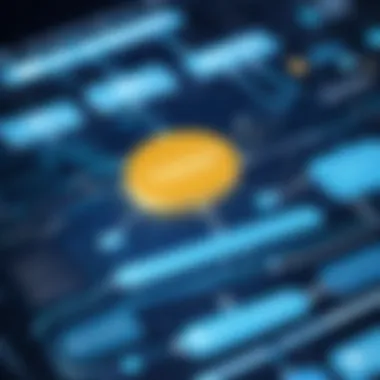
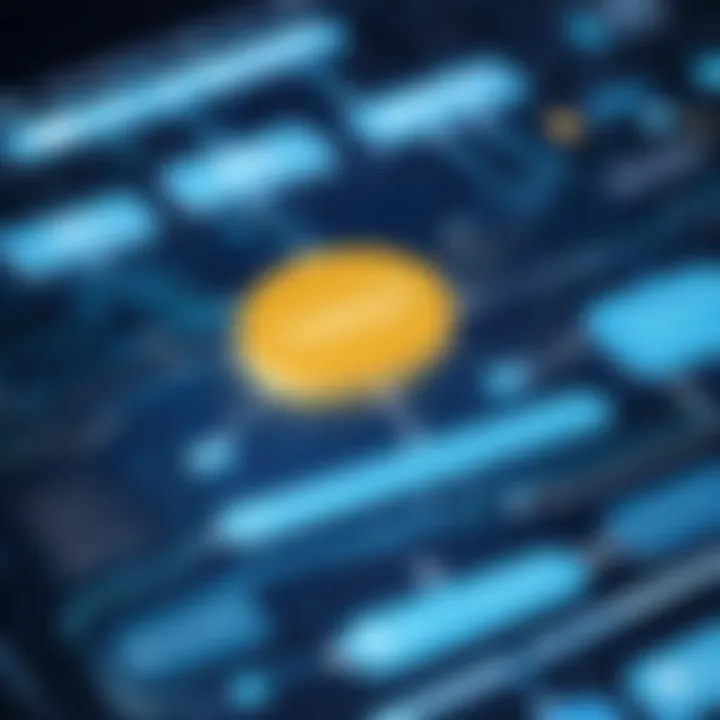
Setting Up Libraries for Data Science
In the realm of data structures and algorithms, libraries play a crucial role. They offer pre-written functions that save time and effort, helping you to focus on problem-solving instead of reinventing the wheel. Key libraries you should consider are:
NumPy
NumPy stands out for its array-centered approach to numerical computations. It enables efficient handling of large datasets, which is particularly beneficial when you are tackling complex algorithms. The core characteristic of NumPy is its powerful N-dimensional arrays, vastly speeding up mathematical operations compared to native Python lists. One unique feature of NumPy is its broadcasting ability, which allows you to perform arithmetic operations on arrays of different shapes seamlessly.
While itâs widely acclaimed for its speed and efficiency, it's crucial to note that it has a steeper learning curve compared to pure Python. Yet, investing the effort here pays off when you begin to tackle data-heavy algorithms.
Pandas
Building on NumPyâs strengths, Pandas shines in data manipulation and analysis. Its primary offering is DataFrames, which allow for intuitive data handling similar to spreadsheets. The library supports a variety of operations like group-by, joins, and filtering, making it a favorite for data wrangling tasks.
The key characteristic of Pandas is its powerful data alignment capabilities, automatically aligning data when performing operations. This makes it a popular choice for data scientists who frequently need to merge datasets from different sources.
However, Pandas can be memory-intensive, and thus, performance can lag when handling exceptionally large datasets.
Matplotlib
Finally, there's Matplotlib. If you wish to visualize your data and algorithms, this library is a game changer. With Matplotlib, you're equipped to create a variety of static, animated, and interactive plots. The key characteristic here is its unmatched flexibility, allowing you to customize nearly every aspect of your charts.
A unique feature is its ability to integrate seamlessly with other libraries like NumPy and Pandas, thereby enhancing the visualization process. However, the trade-off often lies in its complexity; beginners might find Matplotlib less straightforward to use than other simple plotting libraries.
Basic Data Structures in Python
Before diving into the nitty-gritty details of programming, it's crucial to grasp the essence of data structures in Python. These basic structures serve as the building blocks for managing data efficiently, allowing programmers to manipulate and interact with information seamlessly. A solid understanding of these data structures lays the foundation for advanced algorithm implementation, optimization, and enhancing overall software performance.
Lists in Python
Creating Lists
Creating lists in Python is like laying down the first bricks of a house. It's simple yet essential. Lists are dynamic arrays that can hold multiple items, which means they can grow and shrink as needed. In the context of this article, lists are a popular choice because they're versatile and easy to work with. One key characteristic is that lists can hold mixed data types, enabling a diverse array of data storage.
A unique feature of lists is their ability to allow duplicate values, which is advantageous when order matters or when it's important to preserve data integrity. However, this flexibility comes with a downside; operations on large lists can become time-consuming, particularly when looking to search or remove specific elements.
Indexing and Slicing
Indexing and slicing open up the world of data manipulation within lists. By utilizing zero-based indexing, each item can be accessed swiftly. This particular feature makes it easy to grab specific elements without complicated code. For this article, the focus on slicing â the ability to extract subsections of lists â is pivotal.
Slicing allows for the extraction of parts of lists efficiently. This is beneficial when one needs a quick glance at a segment of data or needs to analyze portions in-depth without altering the original structure. However, if not handled carefully, extensive slicing could lead to confusion, especially for beginners trying to track list states before and after modifications.
Common List Methods
When exploring lists, familiarizing oneself with common list methods is paramount. These handy tools provide pre-built functionalities, like adding, removing, or sorting data, simplifying complex tasks. By employing methods such as , , and , programmers can efficiently manage their datasets.
Thereâs a certain charm to list methods; they encapsulate functionality in an easily digestible manner, catering especially to those just starting out. However, relying solely on these methods without understanding the underlying mechanisms can lead to missed opportunities for optimization down the line.
Tuples and Sets
Characteristics of Tuples
Tuples in Python function as immutable sequences, which makes them distinct from lists. Once created, their elements cannot be altered, offering a consistency that many applications require. This characteristic makes tuples a favorite in situations where you want to ensure data integrity, such as when storing fixed data groups. Furthermore, comparison operations between tuples can be more efficient than lists due to their immutability.
However, the downside arises when mutable structures are required; tuples can leave a programmer stuck between efficiency and adaptability. Thus, understanding when to use tuples versus lists is critical in mastering Python data structures.
Set Operations and Applications
Sets bring a different flavor to data handling. They are primarily used for membership testing and eliminating duplicates because they automatically enforce uniqueness. When you don't need order but you want each element to be distinct, sets shine. This aspect is particularly advantageous for quickly processing large datasets where duplicates may skew results.
However, it's imperative to acknowledge that sets are unordered. Thus, trying to access elements by position will lead to a multitude of headaches. As such, knowing when to switch from sets to other structures for specific tasks is a valuable asset for programmers.
Dictionaries: Key-Value Pair Storage
Creating Dictionaries
Dictionaries hold a powerful position in Python due to their key-value pair storage format. When creating dictionaries, one offers a satisfying way to link related pieces of data, enriching the data representation landscape. They are widely seen as beneficial because accessing values through keys rather than indices enhances readability and efficiency.
A unique aspect of dictionaries is that keys must be immutable, which guarantees stability. However, developers must exercise caution to avoid mutability issues, especially when trying to manipulate non-hashable data types as dictionary keys. Knowing the intricacies here can be the difference between success and chaos in data handling.
Accessing and Modifying Elements
Once crafted, accessing and modifying elements in a dictionary is where the real fun begins. Using keys, one can retrieve or alter values with remarkable speed. This attribute is beneficial when parsing or filtering large datasets. The ability to dynamically update data makes dictionaries a laser-focused tool for applications such as caching where quick access to information is vital.
Nonetheless, using dictionaries requires a solid grasp of the underlying data structure to maximize effectiveness. The abstraction can be comforting, but it can also lead to performance issues if one doesn't pay attention to how key collisions or unhashable types can impact overall functionality.
Through these basic data structures, learners will gain insight into the pragmatic applications of Python programming. Mastery over lists, tuples, sets, and dictionaries unveils possibilities that can streamline data processing and enhance programming efficiency.
Complex Data Structures
In the realm of programming, particularly when using Python, complex data structures play an integral role in modeling real-world problems. Unlike basic data types, such as integers or strings, complex data structures allow developers to create more sophisticated representations of information. They not only enhance the efficiency of algorithms but also help simplify the process of data manipulation and storage.
One key benefit of using complex data structures is their ability to maintain relationships among data, which becomes essential when dealing with intricate datasets. For instance, if we consider the data produced by social networking platforms, entities like users and their connections can be effectively represented using graphs or trees. Thus, understanding and implementing these structures becomes critical to optimizing performance and usability in programming tasks.
Understanding Linked Lists
Linked lists stand out as fundamental components in managing data structures. At their core, they comprise nodes, each containing data and a pointer to the next node in the sequence, forming a chain. This unique feature delineates them from static data structures, such as arrays, making it easier to add or remove elements without intensive memory reallocations.
Singly Linked Lists
Singly linked lists introduce a simple yet powerful concept when it comes to data storage. Each node holds data and a reference to the subsequent node, leaving us with a linear structure that's easy to traverse. The primary advantage here is the efficient use of memory since it only allocates space as needed for new nodes, which can be a major advantage in environments with limited resources.
However, a drawback of singly linked lists is their inability to traverse backward. Thus, if you find yourself needing to access the prior node frequently, this could lead to inefficiencies, as you'd need to traverse from the head each time. Nonetheless, their simplicity and dynamic nature make them an appealing choice, particularly for beginner programmers.
Doubly Linked Lists
Doubly linked lists build upon the foundation laid by singly linked lists. They include nodes that point to both the next and previous nodes, offering a two-way traversal. This enhanced capability provides the flexibility of accessing nodes in both directions, catering to the needs of various algorithmic applications.
The versatility of doubly linked lists comes at a cost. Each node requires more memory to store two pointers instead of one, which can be a disadvantage in memory-constrained environments. Still, their adaptability to complex traversal requirements positions them as a beneficial structure for more intricate operations, particularly when implementing data structures like deques or complex algorithms involving bidirectional searches.
Stacks and Queues
Stacks and queues represent pivotal data structures often used for managing collections of objects in a specific order. They draw from real-life analogies; a stack resembles a stack of plates where you can only add or remove the top plate, while a queue acts like a line at the grocery store, where the first person in line is the first to be served.
Implementing Stacks
In implementing stacks, the Last In, First Out (LIFO) principle is paramount. The recent items are accessed first, which is particularly useful in scenarios like undo mechanisms in editors or parsing expressions in programming. Python's list structure offers a straightforward way to create a stack, utilizing methods like for pushing and for popping elements off the stack.
However, while stacks simplify access to the most recent items, they can impose limitations if the need is to access items out of order. This makes stacks a popular, albeit specialized, choice in situations where such linear access suffices.
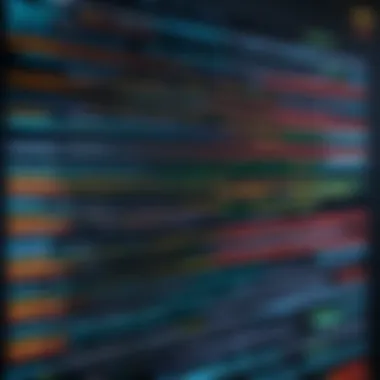
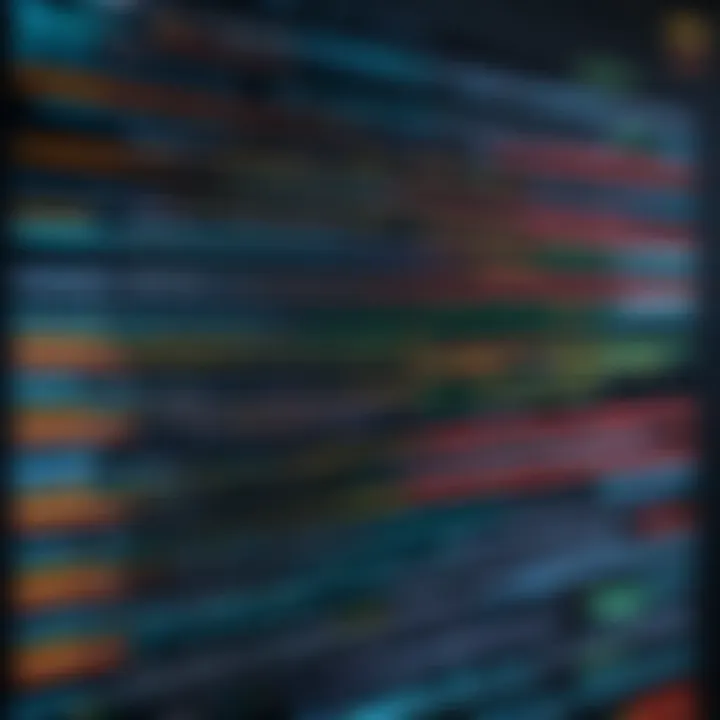
Implementing Queues
When it comes to queues, the First In, First Out (FIFO) structure ensures that the first element added is also the first to be removed. This aspect greatly enhances understanding when scheduling tasks or managing resources, similar to a to-do list where the oldest tasks should be attempted first.
However, implementing queues in Python can be a bit more complex since lists are not as efficient when it comes to popping from the front. Utilizing libraries like can remedy this issue, allowing items to be efficiently appended and popped from both ends.
Use Cases
Both stacks and queues have invaluable use cases in programming. Stacks are often used in recursive programming, where the system keeps track of function calls. Queues, on the other hand, serve in many scenarios like breadth-first search algorithms or task scheduling systems. The ability of these structures to offer clear, predictable behaviors helps foster clean and manageable code.
Trees and Graphs
Trees and graphs offer robust methods for representing hierarchical and networked relationships, respectively. These structures are highly versatile and can be tailored for various applications, from parsing hierarchical data to representing relationships in social networks.
Binary Trees
Binary trees, a fundamental structure in computing, allow each node to have at most two children. This characteristic facilitates efficient data organization, making insertions, deletions, and lookups straightforward and quick.
The simplicity of binary trees comes with some challenges, particularly concerning balance. Unbalanced trees can lead to inefficient operations, nearly degrading to a linked list performance. Nevertheless, balanced variations, such as AVL trees, have addressed these shortcomings, proving their worth in countless applications.
Binary Search Trees
Binary search trees bring additional functionality to binary trees. Here, the key property is that for any node, values in the left subtree are lower, and those in the right are higher. This allows for efficient searching, as a properly maintained binary search tree can deliver O(log n) lookup times.
However, similar to binary trees, they can become inefficient without measures to maintain balance. Thus, while offering many advantages, one must remain cautious about their implementation.
Graph Representations
Graph representations emerge as a powerful way to model networks and complex relationships. They can be defined using nodes and edges, whether directed or undirected. From social interactions to pathways in maps, graphs provide a nearly limitless way to traverse and analyze connected elements.
Among the various representation methods, adjacency lists and adjacency matrices are prevalent. The choice between these often depends on the nature of the graph; dense graphs may favor adjacency matrices for quick access, while sparse graphs benefit from the memory efficiency of adjacency lists.
The flexibility and wide range of applications make graphs a highly beneficial structure to explore alongside trees, greatly enhancing the capacity to model real-world scenarios.
Fundamentals of Algorithm Design
Algorithm design is the cornerstone of effective programming, especially when it comes to working with data structures. This segment explores the budget and efficiency of different algorithms, helping programmers to make informed decisions that can significantly impact performance. Understanding the fundamentals of algorithm design lays the groundwork for optimizing code, which ultimately leads to more robust applications.
One major aspect to grasp is algorithm complexity, generally expressed in Big O notation. This indicates how the time or space requirements grow relative to the input size. By learning this concept, youâll gain insight into how well your solutions perform as datasets expand. It highlights the difference between an efficient solution and a slow, cumbersome one. Developers must prioritize writing algorithms that not only function correctly but also execute efficiently.
Algorithm Complexity: Big O Notation
Big O notation is a mathematical representation that helps assess the efficiency of algorithms in terms of time and space. It provides a way to discuss the upper limit of an algorithmâs growth rate.
- Constant Time: O(1) â This is the best case scenario, where time does not change regardless of input size.
- Linear Time: O(n) â The execution time grows linearly with the input size.
- Quadratic Time: O(n^2) â The performance is proportional to the square of the input size, often seen in algorithms with nested loops.
Understanding Big O notation is crucial for choosing the right algorithm for a specific task, especially in complex applications where performance can be critical.
Common Algorithmic Techniques
Several algorithmic paradigms stand out for their efficiency and ease of use. Let's delve into three prominent techniques: Divide and Conquer, Greedy Algorithms, and Dynamic Programming.
Divide and Conquer
This technique involves breaking down a problem into smaller subproblems, solving each separately, and then combining those solutions. This approach is the backbone of many efficient algorithms, such as quick sort and merge sort. The key characteristic of Divide and Conquer is its ability to simplify a complex problem into manageable parts.
"Divide and Conquer is like having multiple branches of a tree working simultaneously to reach the top faster than one trunk alone."
One unique feature is how it often reduces the problem size logarithmically, resulting in improved performance. However, it may require additional space for managing recursive calls, which is something to keep in mind when resource optimization is necessary.
Greedy Algorithms
Greedy algorithms follow a straightforward approach: make the locally optimal choice at each stage with the hope of finding a global optimum. This method is particularly effective in problems like the Knapsack Problem or finding the Minimum Spanning Tree. The appeal lies in its simplicity and speedâoften yielding a quick solution in a limited timeframe.
However, the downside is that greedy algorithms do not always provide the optimal solution. While they can solve many problems efficiently, understanding the context is vital so that they don't lead you down a suboptimal path.
Dynamic Programming
Dynamic Programming (DP) solves problems by breaking them into simpler overlapping subproblems and storing the results of these subproblems to avoid redundant calculations. Itâs especially helpful for optimization problems where some subsolutions can be reused.
A key characteristic of DP is its strategy to trade time for space. The memory, while a bit hefty, can significantly cut down execution time. This can be a game-changer for problems characterized by large input sizes or complex calculations. However, it requires a clear understanding of the structure of the problem, which can be a steep learning curve for some.
Implementing Algorithms in Python
Implementing algorithms in Python is a crucial aspect of mastering data structures and algorithms. Algorithms form the core logic that governs how data is managed, manipulated, and utilized within programs. Python, with its clear syntax and extensive libraries, acts as an ideal platform for developing and testing these algorithms. By working through these implementations, learners not only solidify their understanding of how these concepts function in theory but also gain practical skills that can be applied in real-world scenarios.
Learning to implement algorithms involves grasping different techniques and understanding their strengths and trade-offs. This knowledge is fundamental for optimizing performance in various applications, from basic scripts to large-scale data science projects.
Sorting Algorithms
Sorting algorithms are some of the most basic yet essential algorithms used in programming. They allow us to arrange data in a specific order, which is crucial for search operations and data analysis. Here are some popular sorting algorithms:
Bubble Sort
Bubble Sort is a simple algorithm that repeatedly steps through the list, compares adjacent elements, and swaps them if they are in the wrong order. It continues this process until no swaps are needed, indicating that the list is sorted. The key characteristic of Bubble Sort is its straightforwardness. Itâs easy to understand, making it a reliable choice for beginners.
However, despite its simplicity, Bubble Sort is not the most efficient algorithm for large datasets. It has a time complexity of O(n²), which means its performance declines rapidly as the number of elements increases. On the bright side, its unique feature of being a stable sort (it doesn't change the relative order of equal elements) is beneficial in certain cases.
Quick Sort
Quick Sort is a more advanced algorithm that uses a divide-and-conquer approach to sort data. It selects a 'pivot' element and partitions the other elements into two sub-arrays according to whether they are less than or greater than the pivot. This process is repeated recursively on each sub-array. The key characteristic of Quick Sort is its speed. It generally performs better than other sorting algorithms on average, with an average time complexity of O(n log n).
What sets Quick Sort apart is its efficiency in handling large datasets. However, one disadvantage is that its worst-case performance is O(n²), which can occur when the smallest or largest element is consistently chosen as the pivot.
Merge Sort
Merge Sort is another divide-and-conquer algorithm that divides the dataset into two halves, sorts each half, and then merges them back together. This algorithm is notable for its stability and efficiency. Its key characteristic is that it consistently operates with a time complexity of O(n log n), making it much more predictable in terms of performance compared to Bubble Sort.
A unique feature of Merge Sort is its ability to handle large datasets better, especially when they are stored externally (e.g., on disk) because it accesses data in a more linear fashion. However, it does require additional memory space for the temporary arrays that are created during merging, which can be a drawback in memory-limited situations.
Searching Algorithms
Searching algorithms are designed to retrieve information stored within data structures efficiently. They help in locating specific data, which is essential in virtually all programming tasks. Below are two common searching algorithms:
Linear Search
Linear Search is the simplest searching technique. It works by checking each element in a list sequentially until the desired element is found or the list ends. The key characteristic of this algorithm is its simplicity and ease of implementation. It requires no complex programming logic, which makes it a good starting point for learners.
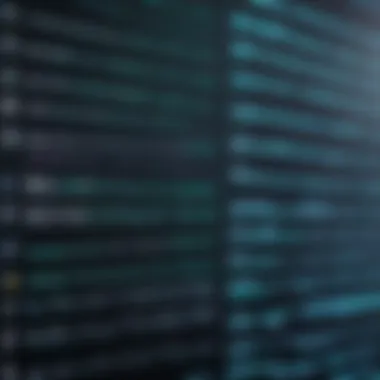
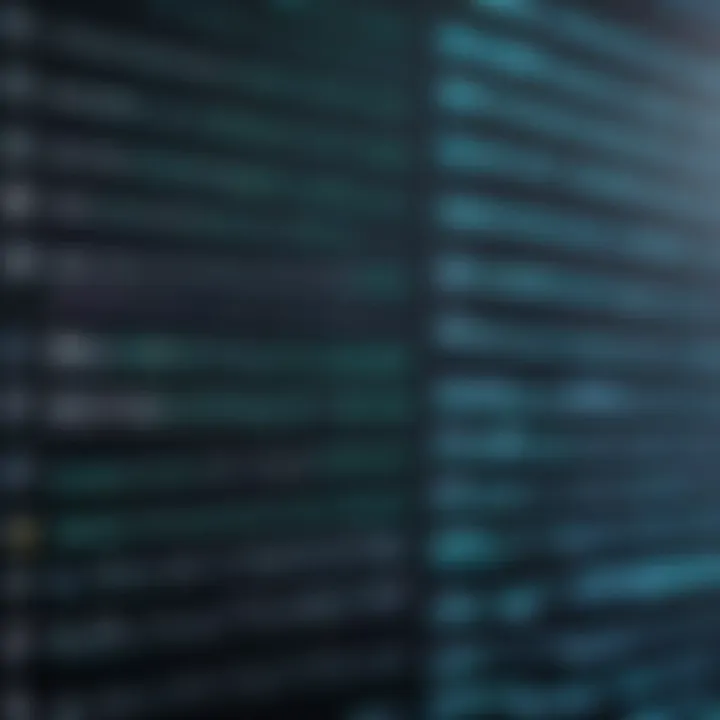
One major disadvantage is its inefficiency with large datasets, as it has a time complexity of O(n). Itâs best suited for small, unsorted lists where a mere comparison is sufficient.
Binary Search
Binary Search is significantly more efficient than Linear Search, but it requires that the data be sorted beforehand. This algorithm divides the list into halves, checking the middle element, and assessing if the target value is greater or lesser than that middle value. This process continues until the element is found or all possibilities are exhausted, leading to a time complexity of O(log n).
The key characteristic of Binary Search is its efficiency in large datasets, making it a preferred choice in many cases. However, the prerequisite of having a sorted list can be seen as a limitation in certain scenarios.
In summary: Understanding the implementation and characteristics of different sorting and searching algorithms in Python provides a foundational skill set for tackling more complex programming tasks and optimizing performance in various applications. This knowledge is indispensable in the field of data science and software engineering.
Best Practices in Data Structures and Algorithms
When it comes to software development, the importance of adhering to best practices in data structures and algorithms cannot be overstated. These practices form the backbone of efficient programming and can dramatically influence both the performance and maintainability of your code. By making well-informed decisions regarding data structures and algorithms, you're essentially laying down a roadmap that leads to a smoother development experience.
Choosing the Right Data Structure
Selecting the most appropriate data structure is akin to choosing the right tool for a specific job. In programming, the story is no different. Using a data structure that aligns with your specific needs can yield substantial improvements in both performance and resource management.
For instance, when working with collections of data, consider the characteristics of Python's lists versus sets:
- Lists are ordered and allow duplicates, which can be beneficial for certain use cases like maintaining the order of items.
- Sets, on the other hand, come without duplicates, making them ideal for scenarios where uniqueness is essential and you don't care about order.
Some points to consider when choosing a data structure include:
- Access Patterns: Know how your application will read and modify data. This knowledge informs whether you need fast access, efficient inserts, or quick deletions.
- Memory Usage: Each data structure comes with its own memory overhead. Balancing performance and memory requirements can be crucial, especially in environments with constrained resources.
- Algorithmic Compatibility: Certain algorithms perform better with specific data structures. For example, if you're implementing a priority queue, utilizing a heap structure can drastically enhance performance.
In short, it's essential to consider the trade-offs associated with each data structure. Choosing one indiscriminately can lead to bottlenecks down the line.
Memory Management
Memory management plays a pivotal role in the efficiency of your algorithms and data structures. Effective memory handling not only boosts performance but also enhances the overall reliability of your application.
Python, for example, handles memory management automatically through its garbage collection mechanism, but that doesn't absolve you from making conscious choices:
- Object Lifecycle: Be aware of how long your objects need to exist. Keeping unnecessary references can lead to memory bloat, which, besides potentially causing crashes, slows down performance.
- Short-Lived Objects: Focusing on creating short-lived objects can help in minimizing memory usage. For example, if you're processing data in chunks, it's better to create and dispose of objects in each iteration rather than letting them linger.
- Using Built-in Functions: Leveraging Python's built-in functions and libraries often results in better memory management. These are optimized for performance and memory use, allowing you to focus on the logic of your application instead of low-level details.
"The programming language does not drive development. The development drives the programming language."
Being astute with your memory management can protect against common pitfalls like memory leaks, which can rear their ugly heads when least expected.
In summary, understanding how to choose the right data structure and manage memory efficiently are critical skills for any developer. They empower you to build applications that not only function correctly but do so in an optimal manner. By weaving these practices into your coding habits, you're not just following the crowd; you're setting yourselfâand your projectsâup for sustainable success.
Common Pitfalls and How to Avoid Them
Navigating the world of data structures and algorithms can feel like wandering through a dense forest without a map. It's all too easy to stumble upon common pitfalls that can cripple your progress and keep you from mastering Python programming. Being aware of these pitfallsâand knowing how to sidestep themâcan make all the difference. In this section, we'll dive into two significant stumbling blocks: inefficient algorithms and misusing data structures.
Inefficient Algorithms
When you're working on coding projects, it is tempting to take shortcuts. Sometimes, a quick solution can seem appealing, especially when you're crunching deadlines. However, inefficient algorithms are like using a rusty tool to build a houseâsloppy work will yield a shaky structure.
Inefficient algorithms result in slower performance and longer processing time. For instance, consider the classic Bubble Sort. While it might seem simple to implement, it sorts data in O(n^2) time complexity. In cases where you're dealing with large datasets, this inefficiency can become a nightmare, leading to significant delays.
To avoid this pitfall, pay close attention to your algorithm's time complexity and efficiency. Optimize your functions and choose algorithms that are designed to handle the scale of data you're working with.
- Review Algorithm Complexity: Utilize concepts such as Big O notation to analyze your algorithms.
- Choose Smartly: Consider better alternatives. For example, using Quick Sort or Merge Sort can dramatically speed up the process compared to Bubble Sort.
"An algorithm is a recipe. If your recipe is wrong, the meal will be a disaster no matter how well you cook."
Misusing Data Structures
Think of data structures as the containers of your program. If you misuse them, your program can easily fall apart, much like trying to fit a square peg in a round hole. Several developers have faced the frustration of trying to extract data from an improperly chosen data structure, only to find that it leads to performance bottlenecks and code complexity.
For instance, using a list in place of a set for membership testing can bring your program to a crawl, especially as the size of the dataset increases. Lists offer O(n) time complexity for searches, while sets can accomplish the same task in O(1) time complexity. Understanding the right context for each structure is crucial.
To minimize mishaps, consider these guiding principles:
- Know Your Structures: Familiarize yourself with the characteristics and use cases of each data structure.
- Plan Ahead: Before jumping into coding, take a moment to outline the data flow to determine the most suitable structure.
By avoiding these common pitfalls, you not only enhance your coding skills but also build a strong foundation for tackling more complex problems in data structures and algorithms. The more you refine your understanding, the better equipped you'll be for real-world challenges in programming.
Real-World Applications
The study of data structures and algorithms has a significant role in the real world. Understanding how these concepts operate can lead to more efficient and effective programming solutions. Not only do they optimize performance, but they also save crucial resources in various applications. Below, we'll delve into specific areas where data structures and algorithms shine, showcasing their importance and utility.
Data Structures in Web Development
In the realm of web development, data structures are foundational to managing and manipulating data efficiently. Web applications often require quick access and modifications to data sets, making the choice of the right data structure pivotal. For instance, consider a social networking site. Here, user profiles and interactions can balloon to millions of entries. To handle such scale, developers may choose a dictionary structure to store user ids and their corresponding data, allowing for O(1) access time.
- Efficiency: The time complexity associated with various data structures means faster searches, insertions, and deletions. A linked list could be employed for features like exponential backtracking where users can easily access their previous actions without hassle.
- Scalability: With the proper structure in place, scaling applications becomes smoother. For example, utilizing trees for hierarchical data (like category trees in e-commerce) helps maintain order and speed.
- Maintenance: Good data structures will allow for easier updates to the application. Maintaining a list of active sessions in a stack could help track user activity, making it more manageable to push and pop sessions.
Web development, therefore, is not just about creating appealing interfaces and responsive designs. Itâs about creating robust and efficient back-end processes where data structures play a key part.
Algorithms in AI and Machine Learning
When it comes to artificial intelligence and machine learning, algorithms serve as the backbone for intelligent decision-making. The choice of algorithm can greatly affect the accuracy and efficiency of the model being developed. Notably, the use of algorithms such as sorting and searching is prevalent in these domains.
- Pattern Recognition: AI systems often employ complex algorithms to detect patterns in data. For example, using a decision tree to classify data relies on traversing nodes in a structured way, making data structures like trees essential for effective classification.
- Optimization: Algorithms are heavily used for optimization in machine learning, allowing for fine-tuning of parameters for the best fit. Gradient descent is one such example of an algorithm that iteratively adjusts parameters to minimize error.
- Data Processing: Handling large datasets efficiently requires the use of effective algorithms. Libraries such as TensorFlow leverage sophisticated algorithms to perform on-the-fly computations, facilitating real-time data processing.
In AI and machine learning, the ability to select the right algorithms directly influences the overall performance and results.
Additional Resources for Continued Learning
Understanding data structures and algorithms is only the tip of the iceberg in the vast ocean of programming knowledge. Continuous learning in this field is essential. Resources designed for additional learning not just enhance your knowledge but help you stay relevant in a constantly evolving tech landscape. With technology and methodologies changing at lightning speed, having the right tools can sharpen both your theoretical understanding and practical application skills.
Online Courses and Tutorials
In todayâs digital age, online courses have emerged as a valuable asset for learners. They offer flexibility, allowing you to learn at your own pace, which is critical for absorbing complex concepts such as advanced data structures and algorithm design. Here are some platforms worth checking out:
- Coursera: With courses developed by universities, youâll find topics ranging from basic Python syntax to more intricate algorithm strategies taught by renowned professors.
- edX: Similar to Coursera, edX offers a variety of free and paid options from institutions like MIT and Harvard, covering both theoretical and practical aspects of data structures.
- Udemy: This platform is packed with diverse coursesâmany with hands-on projects. You can choose courses focused specifically on Python or broader programming principles.
These platforms often feature community forums where you can connect with other learners. This interaction is key, as discussing ideas and problem-solving techniques with peers bolsters your learning process.
Books and Literature
Books have long been a trusted source for gaining knowledge. They provide comprehensive insights and the opportunity to delve deep into topics at your own pace. Hereâs a selection of recommended titles:
- "Introduction to Algorithms" by Thomas H. Cormen: This book is an essential read. It strikes the right balance between theory and practice, tackling methods like sorting and data structure manipulation comprehensively.
- "Python Data Structures and Algorithms" by Benjamin Baka: Tailored specifically for Python, this book encompasses practical applications that can be immediately implemented in your projects, making the learning curve much less intimidating.
- "Grokking Algorithms" by Aditya Bhargava: Presented in an engaging and visually appealing way, this book simplifies complex concepts, making them digestible for beginners.
Having access to these tangible resources not only solidifies understanding but also serves as a reference point in your programming journey. Being able to look at learning materials whenever you hit a barrier enhances retention and confidence.
Remember: While online resources provide interactivity, books can foster deep comprehension through structured content. Balancing both can create a well-rounded educational experience.
In summary, harnessing additional resources is crucial for those looking to master data structures and algorithms in Python. Whether through online courses or books, finding the right materials can elevate your learning experience and expand your coding arsenal.