Mastering Data Structures for Coding Interviews
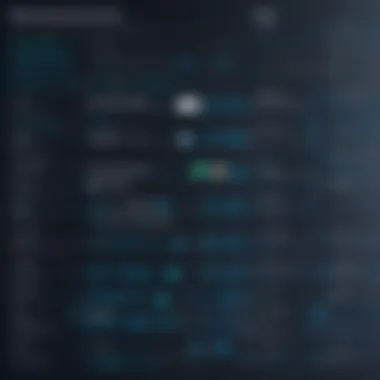
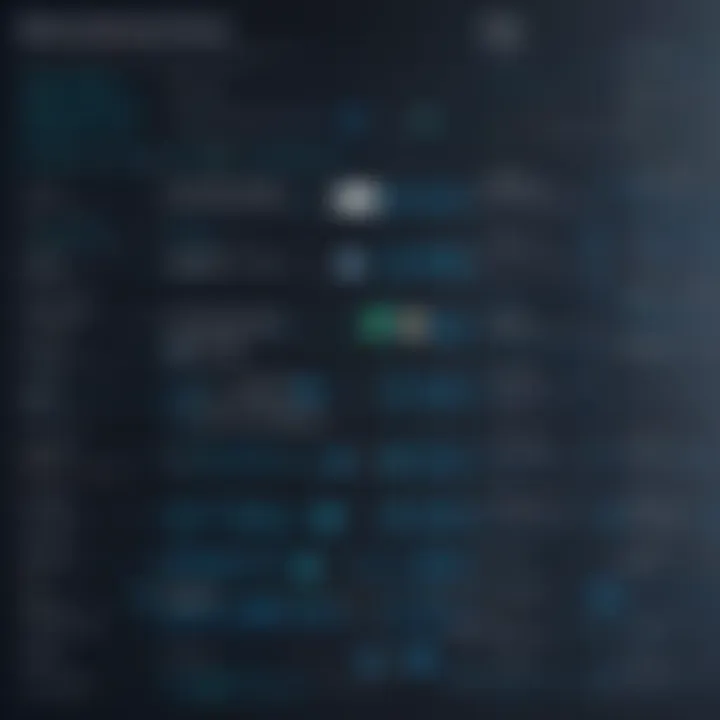
Overview of Topic
In the realm of software development, data structures often serve as the backbone of efficient programming and problem-solving. When preparing for coding interviews, understanding these structures is not just helpful; it's essential. With many job interviews in tech companies prioritizing problem-solving capabilities through the lens of data structures, candidates must navigate this landscape with finesse.
Data structures can be likened to the tools of a tradesman—each designed for specific tasks, enhancing functionality and productivity. The scope of this article encompasses key data structures, their practical implications in the industry, and strategies to tackle coding interviews effectively.
A glimpse into their history shows a gradual evolution from simple arrays to complex structures like trees and graphs. This advancement reflects the increasing complexity of modern software systems, making the knowledge of these structures indispensable for anyone aiming for a successful career in technology.
Fundamentals Explained
At the heart of understanding data structures lie core principles and fundamental theories. Key terminology such as arrays, linked lists, stacks, and queues form the building blocks of knowledge. A basic concept like an array—a collection of elements identified by index—illustrates how data is organized and manipulated in efficient algorithms.
But concepts go beyond their definitions. A well-designed data structure can drastically reduce time complexity. For instance, while finding an element in an unsorted array might take linear time, utilizing a hash table can reduce this to constant time. This exemplifies the importance of understanding which data structure to apply depending on the problem context.
Key Terminology and Definitions
- Array: A collection of items stored at contiguous memory locations.
- Linked List: A sequential collection of elements, each pointing to the next, allowing for efficient insertion and deletion.
- Stack: A collection where the last added item is the first to be removed (LIFO).
- Queue: A collection where the first added item is the first to be removed (FIFO).
Practical Applications and Examples
Real-world applications of data structures can be quite diverse. Take, for example, social media platforms like Facebook. They maintain vast amounts of user interactions which require quick access and efficient storage solutions. Data structures like graphs are utilized for managing connections between users, enabling rapid speed in data retrieval.
For hands-on projects, consider implementing a simple address book using a tree data structure. Each contact can serve as a node, and you can add functionalities to search, delete, or update contacts efficiently. Here's a basic code snippet to provide an idea:
Advanced Topics and Latest Trends
As technology advances, so do the methodologies surrounding data structures. Contemporary trends include the integration of data structures in big data frameworks and machine learning algorithms. For instance, utilizing trie data structures can enhance the efficiency of search engines through predictive text algorithms.
Future prospects indicate a push towards more adaptive and dynamic data structures that adjust to varying workloads and conditions. Emphasis on distributed systems is growing, thereby leading to new ways of managing data structures in a networked environment.
Tips and Resources for Further Learning
Acquiring deeper knowledge about data structures can significantly aid in coding interviews. Here are some valuable resources:
- Books: "Cracking the Coding Interview" by Gayle Laakmann McDowell provides practical insights into interview preparation.
- Online Courses: Websites like Coursera and Udacity offer courses in data structures and algorithms.
- Forums and Communities: Engage in discussions on platforms like Reddit and Stack Overflow where real-world problems and solutions are shared.
Utilizing tools such as LeetCode or HackerRank for practice problems can also enhance your coding skills, allowing you to apply theoretical knowledge practically.
As candidates embark on their coding interview prepared with solid understanding of data structures, they will find themselves at a distinct advantage in demonstrating their coding acumen.
Prelude to Coding Interviews
Coding interviews are like a rite of passage for those entering the tech industry. They serve as a testing ground where potential employers assess both theoretical knowledge and practical problem-solving skills. For many, these interviews can provoke anxiety, but understanding their dynamics can ease the tension significantly.
The landscape of coding interviews is far from static. With different companies adopting varied interview formats, candidates must be prepared to tackle a diverse range of questions and scenarios. Some firms may opt for a focus on algorithms, while others might prioritize system design or data structure manipulation. Thus, candidates must adapt to this fluid environment, sharpening their skills and broadening their knowledge base to stay ahead in the game.
Understanding Interview Dynamics
Every coding interview consists of two main components: the technical assessment and the behavioral assessment. During the technical part, interviewers might present coding challenges that test a candidate's understanding of data structures and algorithms, alongside their coding efficiency. Often, this involves whiteboard exercises or online coding platforms where real-time problem solving is required. The art of explainin your approach and reasoning to an interviewer can't be overextended, either. Companies want to see how you think, not just the end result.
In the behavioral section, candidates must demonstrate how they've handled challenges in the past, showcasing their soft skills. This can include how they work in teams, prioritize tasks, or approach problem-solving under pressure. Both sections are interlinked; a strong performance in understanding technical aspects often bolsters responses in behavioral assessments.
The Importance of Data Structures
Data structures are the backbone of efficient coding. Think of them as the building blocks of software development. A deep understanding of data structures allows programmers to manage data effectively, which directly impacts performance and efficiency.
When tackling interview questions, knowing which data structure to use at the right moment can be a game-changer. For instance, using a hash table for quick lookups can speed up an operations that might otherwise require lengthy searches through an array or list. Likewise, understanding when to implement a stack or queue can help manage tasks and resources in a logical way, adhering to principles like last-in-first-out or first-in-first-out.
"Mastering fundamental data structures is not just about passing an interview. It's about building software that is robust and scalable."
In reality, the knowledge of data structures extends beyond interviews; it shapes how one implements solutions in real-world applications. Employers value candidates who can grasp these concepts, as this indicates a readiness to take on the complexities of software development.
Understanding data structures allows you to unlock new pathways to solve problems effectively. Each data structure has its pros and cons, and a well-rounded understanding can lead to innovative solutions that an interviewer will recognize and appreciate. Their relevance in coding interviews cannot be understated; therefore, preparing thoroughly in this facet is crucial for anyone looking to succeed.
Defining Data Structures
Understanding data structures is a cornerstone of coding interviews. Data structures serve as the building blocks for organizing and managing data efficiently. Knowing how to define and utilize these structures can distinguish a competent candidate from a stellar one. The ability to choose the right data structure impacts performance, code clarity, and scalability of solutions. Thus, grasping their significance is critical for effective problem-solving.
What are Data Structures?
Data structures can be regarded as specialized formats for organizing and storing data in a computer so that they can be accessed and modified efficiently. Consider them like different containers. Each container is designed for a specific purpose and lends itself to various operations. For instance:
- Arrays: Store items in a contiguous layout. Perfect when similar data types need quick access.
- Linked Lists: Provide dynamic memory allocation where the size is not predetermined. Handy for inserting elements without a fuss.
- Trees: Allow hierarchical storage that depicts relationships. Useful in scenarios such as representing organizational structures.
- Graphs: Used to illustrate connections between data points, great for networking and traversing datasets.
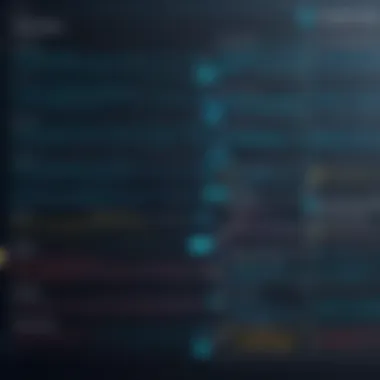
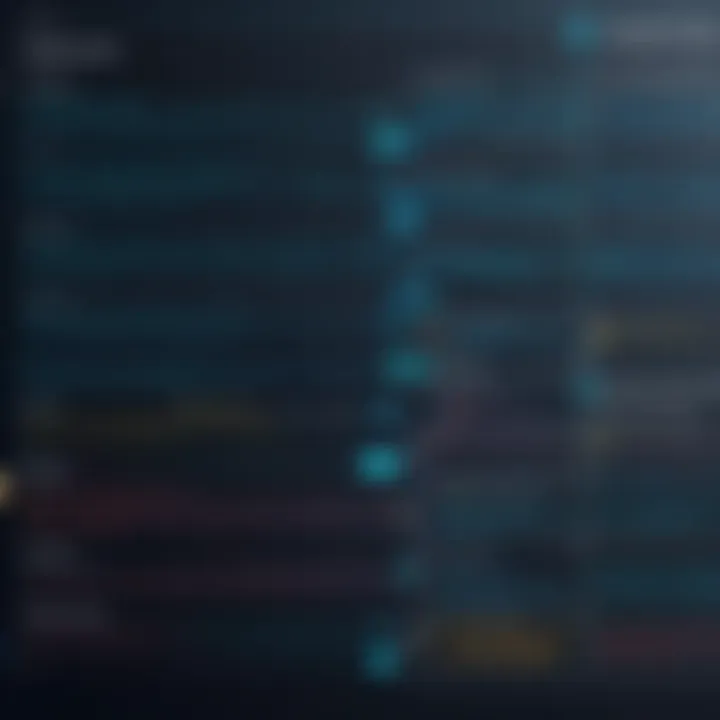
By understanding what data structures are, programmers can tailor solutions to the specific demands of their algorithms and applications.
Fundamental Characteristics
Data structures come with essential characteristics that define their usefulness in various scenarios. Here are some noteworthy traits:
- Efficiency: Different data structures have different performance efficiencies when it comes to operations like addition, deletion, or searching. For example, hash tables typically provide faster look-up times compared to linked lists.
- Complexity: Each data structure's operations have different complexity cases. Understanding these complexities—both average and worst-case—is indispensable while designing algorithms.
- Usability: The choice of a data structure can also depend on how intuitive it is to use. Some might favor simpler structures, while others might leverage advanced features of complex systems.
"The choice of data structure can either make or break your coding interview performance".
Choosing the right data structure means balancing efficiency with comprehension. This balances optimizing performance while ensuring that solutions remain clear and comprehensible.
By establishing a clear grasp of what data structures are and their fundamental characteristics, candidates can approach coding challenges with a robust toolkit at their disposal.
Common Types of Data Structures
In the realm of programming, data structures serve as the backbone of efficient code. Understanding the common types of data structures is crucial for any coder—whether you’re a neophyte or a seasoned IT professional preparing for an interview. Each type offers unique benefits and implications for how data is managed, accessed, and manipulated.
When tackling coding challenges during interviews, potential employers will often seek insight into your reasoning process. Which data structure you choose can impact the performance of your algorithms and ultimately affect the outcome of solutions.
Importance of Different Data Structures
In interviews, selectively choosing the right data structure can mean the difference between an elegant solution and a tangled mess of code. Data structures are not simply containers for data; they are instruments of efficiency, optimization, and clarity.
- Efficiency: A well-chosen data structure minimizes time complexity—this is the crucial factor that interviewers watch for.
- Clarity: Reading and understanding code is vital, but even the best code can get lost in the sauce if the wrong data structure is used. Clarity leads to maintainability.
- Adaptability: Many problems can be approached from various angles. Knowing several data structures equips you with flexibility in your problem-solving toolkit.
Let's examine each common type closely.
Arrays
Arrays are one of the simplest and most widely used data structures. They store elements in contiguous memory locations, which allows for fast access via indices. This means if you know the index, you can pull the data in constant time, O(1).
However, while adding or removing elements can be costly, since it may require shifting elements. A good candidate for arrays is when the number of items is fixed or when you want efficient read operations but can manage the limitations during writes.
Linked Lists
Linked Lists offer a different approach, using nodes that point to the next node, thus forming a chain. This flexibility allows dynamic memory allocation, making it easy to add or remove elements without the overhead of reorganizing existing data.
Yet linked lists don't support efficient random access—if you need an element, you’ll have to traverse the list from the beginning. Thus, they are great for applications where insertion and deletion are frequent but come with a trade-off regarding speed of access.
Stacks
Stacks are all about Last In First Out (LIFO) principles. Think about if you've ever stacked dishes: the last one placed is the first one you take away. This characteristic is useful for function calls and tracking history. Stacks are implemented using arrays or linked lists.
They provide simple operations—push, pop, and peek—allowing for quick management of data as it enters and exits. Stacks are vital in scenarios like parsing expressions or managing undo functionalities in programs.
Queues
Unlike stacks, queues adhere to the First In First Out (FIFO) principle. Imagine a line at a coffee shop; the first person in line is the first one served. This organization is particularly useful in process management and scheduling tasks.
Operations involve enqueueing (adding to the back) and dequeueing (removing from the front). This is helpful in areas like operating systems and handling requests efficiently, ensuring fair service to all tasks.
Hash Tables
Hash Tables are powerful tools for maintaining key-value pairs. They utilize a hashing function to compute indexes in an array for data storage. The main attraction here is their average time complexity for lookups, which is O(1), making them incredibly efficient for search operations.
However, they don't maintain order, and collisions can happen, which may degrade performance if not managed well. When querying large datasets, hash tables can provide impressive speed.
Trees
Trees are hierarchical data structures, resembling an inverted tree or family tree. A common example is the binary tree, where each node has at most two children. Trees enhance data access and provide logical organization.
Trees are essential in applications involving databases and network routing algorithms. They support various operations like insertion, deletion, and searching more efficiently than linear structures.
Graphs
Graphs consist of nodes (or vertices) connected by edges and can represent complex relationships and networks. They are particularly significant in applications such as social networks and mapping programs.
Graph traversal can be done using Depth-First Search or Breadth-First Search algorithms. This type of data structure often requires thoughtful consideration, especially given its versatility and complexity; the relationships between data can become intricate.
Understanding these common data structures not only prepares you for coding interviews but also lays a solid foundation for efficient coding practices. The more familiar you become with each data structure, the better equipped you'll be in selecting the right one for your coding challenges.
Choosing the Right Data Structure
Choosing the right data structure is fundamental to solving coding problems effectively. It can be the deciding factor between an elegant solution and one that's clunky and inefficient. When preparing for coding interviews, being aware of the data structures at your disposal allows you to tailor your approach to the specific problem you face.
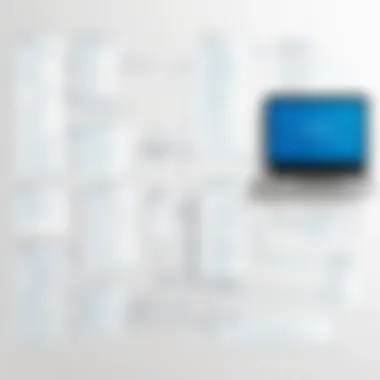
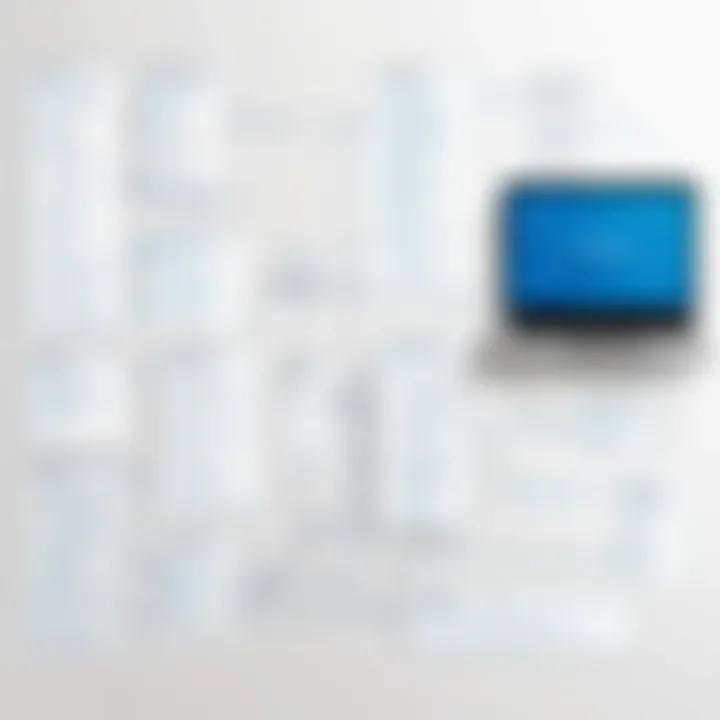
Understanding which data structure to implement involves evaluating various factors that influence performance, ease of implementation, and suitability for the given challenge. Selecting the appropriate data structure helps in writing not only functional code but also efficient code.
Factors to Consider
When it comes to picking a data structure, there are several factors that should guide your decision:
- Type of Operations: Different data structures come with different sets of operations. For instance, if you're expecting a lot of insertions and deletions, then a linked list might be more appropriate than an array. Conversely, if you’re mostly accessing elements, an array can be much faster due to its indexing capabilities.
- Memory Consumption: This aspect is often overlooked by beginner programmers. Data structures like hash tables require additional memory for storage while providing fast access times, which can impact overall performance in memory-constrained environments.
- Data Organization: Consider how you want to organize and access your data. For example, if you need to maintain a sorted sequence, a binary search tree might be the best fit.
- Concurrency Requirements: Some data structures such as queues are better suited for concurrent programming due to their FIFO characteristics, letting multiple threads access data without causing conflicts.
- Complexity of Implementation: Time can be of the essence, especially in an interview. Simpler data structures may yield faster implementations, leaving more time to focus on the logic of your solution.
Each interview might throw a different curveball, demanding you to be quick on your feet in determining the right fit for the task at hand.
Performance Metrics
Once you identify a potential data structure, measure its performance through various metrics. Evaluating how the data structure performs under different scenarios is critical for interviews. Here’s a breakdown of the key performance metrics:
- Time Complexity: This metric tells you how the execution time of operations grows with the size of the input. Familiarize yourself with the average and worst-case time complexities for operations like insertion, retrieval, and deletion. For instance, accessing an element in an array is O(1), while in a linked list it’s O(n).
- Space Complexity: This refers to how much additional memory a data structure requires as the size of the input grows. Understanding how to balance time and space complexity can significantly improve your coding strategies.
- Amortized Time: This metric provides insight into the average time taken for a series of operations, rather than just one operation. For example, when you perform a series of operations on a dynamic array, it may occasionally resize and lead to temporary high costs, but overall the average cost per operation remains low.
- Scalability: It’s not just enough for a data structure to perform well with small datasets. Assess how well it scales with larger data sets, especially for iterative or recursive algorithms that require large resources.
In coding interviews, articulating your thought process around these factors and performance metrics showcases your grasp on the technical nuances of data structures.
"Choosing the right data structure isn’t just about solving a problem; it’s about solving it effectively and efficiently."
By thoroughly considering these elements and metrics, you’ll be well-prepared to tackle technical challenges head-on, standing out as a candidate capable of not just programming, but programming artfully.
Data Structures in Algorithms
Data structures are the backbone of algorithms. Understanding how they work and their role can make a significant difference in coding interviews. If you imagine a toolkit, data structures are the tools, while algorithms dictate how to use those tools effectively. For candidates going through technical interviews, having a solid grasp of data structures isn’t just about answering questions right. It’s about demonstrating a foundational understanding that can lead to efficient problem-solving.
Why are Data Structures Vital in Algorithms?
- Efficiency: Different data structures manage data in distinct ways, affecting the speed and efficiency of the algorithm. For example, an array allows fast access but can be slow for insertions. Choosing the right structure can lead to scalable solutions.
- Complexity: Algorithms vary in their complexity based on the data structure used. By selecting the appropriate structure, one can minimize time and space complexity, which is crucial, especially for large datasets.
- Optimization: It’s often the case that the key to optimizing any algorithm lies in understanding the right data structure. For instance, using a hash table can significantly reduce search times compared to a linked list.
The Role of Data Structures in Algorithm Efficiency
Data structures dictate how data is stored, organized, and manipulated. They provide us with a way to manage large amounts of data in a manner that enables efficient processing.
When considering algorithm efficiency, a few key factors come into play:
- Time Complexity: This refers to how the runtime of an algorithm grows with respect to the input size. When choosing a data structure, consider its access, search, and insertion times. For example, hash tables offer average-case constant time complexity for search operations, which is far superior to the linear search in an unsorted array.
- Space Complexity: This deals with how much memory an algorithm utilizes as it processes data. Some structures, like trees, can be memory efficient through hierarchical organization, while others, such as linked lists, may need more pointers and extra space.
- Insertion and Deletion Mechanics: The ease of adding and removing data elements can vary greatly between different structures. For instance, linked lists allow effortless deletions and insertions, whereas arrays can create overhead during such operations due to shifting elements.
By optimizing these efficiencies through the right data structure selection, candidates can often transform a potential brute-force solution into something elegant and performant.
Common Algorithms Utilizing Data Structures
A variety of algorithms depend on data structures to execute their tasks effectively. Here’s a quick rundown of some commonly used ones:
- Sorting Algorithms: Algorithms like quicksort or mergesort leverage arrays extensively. They depend on systematic data organization for efficient sorting processes.
- Search Algorithms: Binary search utilizes sorted arrays or binary search trees for rapid querying, making search operations much faster compared to naive search methods.
- Dynamic Programming: This approach relies heavily on memorization, often utilizing structures like tables to store interim results efficiently.
- Graph Algorithms: Dijkstra's and A* algorithms utilize graphs to find optimal paths, showcasing how versatile data structures can solve complex routing problems.
"Understanding which data structure fits your problem can be the difference between a working solution and a disaster."
By recognizing these patterns and the algorithms that utilize them, candidates can prepare effectively for coding interviews, demonstrating not only knowledge but also adaptability in solving problems. This understanding is crucial not only for interviews but also for a successful career in software development.
Interview Preparation Strategies
When potential employers sift through heaps of applications, how you handle data structures during coding interviews is a key deciding factor. Interview preparation strategies are truly the backbone of effective preparation. They not only help candidates sharpen their skills but also instill confidence when tackling complex problems under pressure. When you’re well-prepared, you've got a much better shot at making a lasting impression.
One major benefit of having a solid preparation strategy is that it illummunates the nebulous. Candidates can break down various data structures and algorithms into digestible parts. This process makes it feasible to tackle coding questions effectively and with poise. Additionally, knowing how to break problems into smaller components aids in constructing more efficient solutions. This holistic approach means that candidates are not just memorizing but are also understanding the fundamental concepts which is critical for long-term success.
Another critical aspect is timing. Practicing different strategies helps you become adept at estimating how long each operation may take, which is valuable in timed interviews. Strategies don't just encompass code formulation—they also involve correctly interpreting what the question asks. Getting that right is half the battle.
Reviewing Key Concepts
To navigate the often-treacherous waters of coding interviews, knowing the key concepts inside out is essential. These typically include understanding the strengths and weaknesses of various data structures and how they interrelate with algorithms. Grasping terms like complexity, time efficiency, and space optimization can't be overlooked.
Having a good handle on theoretical aspects can greatly enhance your performance. Candidates should frequently review:
- Big O Notation – Know how to compute time and space complexities.
- Common Data Operations – Be familiar with operations associated with stacks, queues, and trees.
- Recursion and Iteration – Distinguish between when each technique is more effective.
You might want to jot down these concepts and study them regularly—put them on sticky notes or use flashcards.
Practical Coding Exercises
Theory can only take you so far without real-world application. That's where practical coding exercises come into play. Engage in activities that mimic the actual coding environment. Utilize platforms like LeetCode or HackerRank to practice your skills. These platforms offer numerous problems categorized by data structure type and also provide coding challenges that are frequently asked in interviews.
It's beneficial to select diverse problems: for instance, solving:
- Array Manipulations – Assess how you handle multi-dimensional arrays.
- Graph Traversals – Focus on breadth-first and depth-first search techniques.
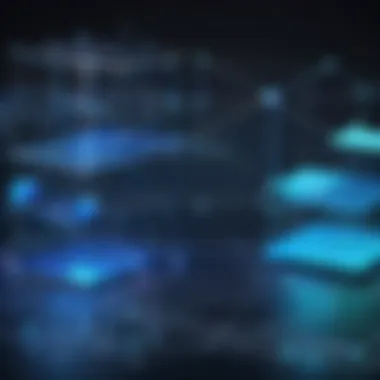
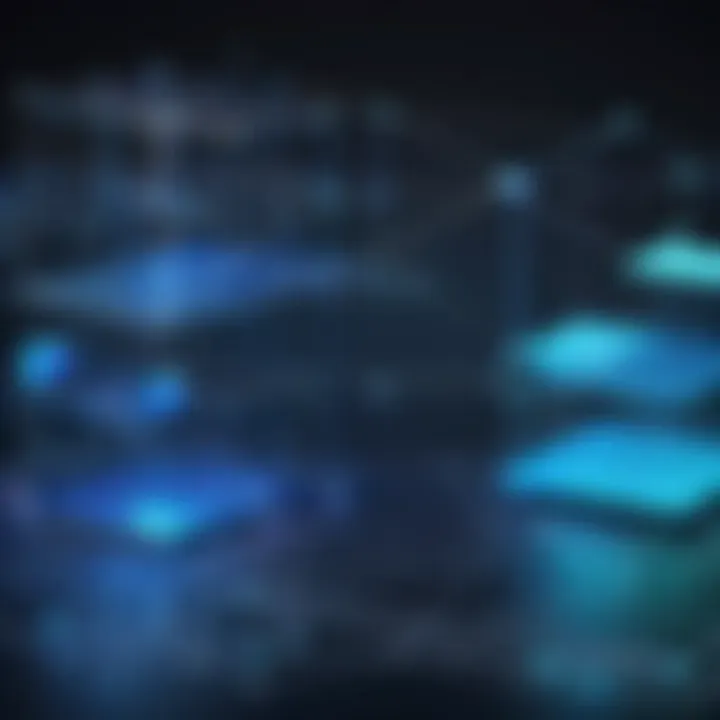
Additionally, coding under timed conditions is invaluable. Practice with a clear restriction, mimicking the time constraints faced in interviews. This helps you become accustomed to making decisions quickly, which is essential when the interview pressure is on.
Mock Interviews
Finally, consider incorporating mock interviews into your preparation. These sessions simulate the real interview atmosphere. You might practice with a friend or utilize platforms that pair you with peers or mentors. During these mock sessions, focus on:
- Communication Skills – How you articulate your thought process can significantly impact the interviewer's perception.
- Problem-Solving Approach – Ensure you explain your reasoning as you tackle each challenge.
- Feedback Incorporation – After each mock interview, review the feedback thoroughly to improve.
The primary aim of mock interviews is to provide an environment where you can stumble and learn without real-world consequences. By embracing constructive criticism, you set yourself up for greater success when it’s game time.
"Preparation is the key to success." In coding interviews, this adage rings particularly true. The more thoroughly you prepare, the more confidently you'll face questions that might initially seem insurmountable.
Test Your Knowledge
In the realm of coding interviews, understanding isn’t just half the battle; it’s the entire war. This section lends itself to reinforcing your grasp of data structures through practical exercises. The philosophy here is simple: the more you practice, the sharper your skills get. It’s not merely about reading theoretical material but engaging actively with problems that demand the application of what you know.
When you tackle coding questions that revolve around data structures, you’re giving your brain a workout. It’s akin to training for a marathon; just as runners clock in various miles, coders must commit hours to solving problems to enhance fluency in their craft. Furthermore, probing questions about sample coding scenarios helps one identify gaps in knowledge and areas that need reinforcement.
Sample Coding Questions
One of the most effective ways to test your understanding of data structures is through sample coding questions. These can range from simple algorithmic puzzles to complex problems that require combining multiple data types. Here are a few examples:
- Reverse a Linked List: Given the head of a singly linked list, reverse it and return the new head.
- Two Sum Problem: Given an array of integers, return the indices of the two numbers such that they add up to a specific target.
- Balanced Parentheses: Check if a string containing parentheses is balanced, meaning every opening parenthesis has a corresponding closing one.
Practicing such problems not only helps solidify the concept but also aids in familiarizing oneself with interview conditions and scenarios.
Analyzing Solutions
Understanding how to analyze solutions is just as crucial as getting them right. After attempting a coding question, it’s essential to review the solution critically. Did you use the right data structure? Could your solution be optimized?
- Time Complexity Analysis: Evaluate how your code performs with different input sizes. Are you ensuring that your solution scales well?
- Space Complexity Considerations: Reflect on how much memory your solution uses. Does it utilize extra space, or is it space-efficient?
- Edge Cases: Did you test your solution against edge cases? For instance, an empty list in the linked list reversal problem or a target that doesn't exist in the two-sum problem could unveil vulnerabilities in your approach.
By dissecting solutions—whether your own or those of others—you begin to recognize patterns and best practices that can elevate your problem-solving skills. Through this iterative process, you grow more adept at choosing appropriate data structures and algorithms, positioning yourself as a confident candidate ready to tackle any coding interview question thrown your way.
"The best way to improve is by learning from every experience, whether it ends in success or failure."
To sum up, testing your knowledge via sample questions and analyzing their solutions cultivates a deep understanding of data structures. It allows students, budding programmers, and IT professionals alike to bridge the gap between theoretical concepts and practical application. By engaging with these elements actively, you're not just preparing for an interview; you're preparing for a fulfilling career in technology.
Common Pitfalls to Avoid
Navigating the complexities of coding interviews can feel like wandering through a dense fog. Recognizing common pitfalls is crucial to avoid unnecessary obstacles on your path to success. Knowing what to steer clear of not only enhances your interview performance but also strengthens your overall coding acumen. The two significant pitfalls we’ll unpack here are overcomplicating solutions and neglecting edge cases. Understanding these concepts can make the difference between acing an interview or leaving an impression of confusion.
Overcomplicating Solutions
It's easy to fall into the trap of overthinking a problem. When faced with a coding question, many candidates often think they need to develop a highly intricate solution complete with advanced algorithms or excessive data structures. However, interviews are often less about complexity and more about clarity and effectiveness.
Taking a simple problem, such as finding the maximum number in an array, candidates might feel pressured to implement complex algorithms like QuickSort or a binary search. This might lead to confusion or lengthy explanations that could derail the discussion. Instead, a straightforward approach using a linear scan would suffice and works perfectly for this specific task. Here are some key factors to consider:
- Simplicity is Powerful: Often, the simplest solution is the most effective one. Demonstrating that you can achieve results with minimal complexity speaks to your understanding of fundamental concepts.
- Communication Matters: Focus on clear communication. When explaining your thought process, you want your interviewer to follow along easily. An overly complex solution tends to muddle your explanation and can create doubts.
- Iterate Gradually: Begin simple, then build complexity only as necessary. This approach allows the interviewer to see your thought process and reasoning. If complexity is required, you can justify it based on the problem's needs, but don't start there.
"In the world of coding interviews, simplicity often beats complexity."
Neglecting Edge Cases
Another common pitfall is neglecting edge cases. While it is commendable to craft a working solution for the primary cases presented in a problem, interviews require a comprehensive understanding that includes considering atypical scenarios. Edge cases often provide the greatest insights into your problem-solving skills and thoroughness.
Consider a function designed to perform division. The typical test case would involve dividing a positive number by another. Still, neglecting cases where the divisor is zero can lead to undefined behavior. Similar situations arise in various contexts:
- Input Limitations: Check for maximum and minimum values, including empty inputs or malformed data. These scenarios often uncover vulnerabilities in your logic and can reveal how robust your solution is.
- Boundary Conditions: Always pay attention to boundaries, such as the start and end of arrays, or the beginning and end of loops. Testing against these conditions helps ensure your solution holds under all circumstances.
- User Behavior: Consider unexpected user input. Imagine writing a program for a user interface. Users may enter unexpected or bizarre inputs that your program should handle gracefully.
In summary, avoiding these common pitfalls will not only enhance the quality of your solutions but also reflect your capabilities as a critical thinker and coder. Always aim for simplicity in your designs and be mindful of edge cases to demonstrate depth in your understanding during coding interviews.
Finale
When you reach the end of an article like this, it’s a moment to reflect, to synthesize, if you will, everything that’s been laid out regarding data structures and their critical role in coding interviews. One might ask: why is this conclusion phase so paramount? It’s quite simple, really. It ties everything together, offering a last chance to distill core ideas into digestible nuggets that can truly enhance a candidate's preparation.
Recap of Key Insights
In this comprehensive exploration, we’ve tackled the following points:
- Understanding Data Structures: At their core, data structures aren’t just theoretical constructs; they’re practical tools that help manage and organize data efficiently. Knowing the differences between arrays, linked lists, stacks, queues, hash tables, trees, and graphs is essential for any aspiring coder.
- Choosing Wisely: Selecting the appropriate data structure based on problem constraints can save countless hours of debugging and suboptimal performance. Factors such as time complexity and memory usage need careful consideration when faced with a coding problem.
- Application in Algorithms: It’s clear that algorithms and data structures go hand-in-hand. Each choice influences the effectiveness of algorithms. For example, using a hash table can provide constant time complexity for lookups as opposed to linear time complexity with an array.
- Practical Preparation: Active engagement through mock interviews and practical coding exercises creates a solid foundation. Familiarity with common questions helps in navigating unexpected challenges during actual interviews.
"Preparation is the key to success."
This classic saying resonates well here. The more you practice, the less you will stumble over data structures during crucial interview moments.
Future Considerations in Technical Hiring
As we look forward, the landscape of technical hiring continues to change. Employers are increasingly seeking candidates who not only possess strong knowledge of data structures but also exhibit adaptability in their problem-solving approach. Here are some considerations for prospective job seekers:
- Stay Updated: The field of technology is always evolving. Keep abreast of new data structures and algorithms that might gain prominence, especially with advancements in artificial intelligence and big data. This can give you a distinctive edge.
- Focus on Concepts: Employers value understanding concepts over mere memorization. Ensure that you grasp the underlying principles of why a particular data structure is used in specific scenarios, as opposed to merely knowing how to implement one.
- Soft Skills Matter: Technical prowess is crucial, but communication skills are just as essential in conveying your thought process during an interview. Practice articulating your reasoning and approach clearly.
- Collaborative Problem-Solving: Nowadays, many companies are adopting pair programming techniques in interviews. This approach assesses not only technical skills but also how well candidates collaborate and communicate.
In summary, as you prepare for your next coding interview, keep these insights and considerations in focus. They serve as a robust framework to navigate the intricate world of coding assessments and position yourself as an appealing candidate in the competitive tech hiring landscape.