Exploring Advanced Data Structures Implementation in Python for Optimal Data Management
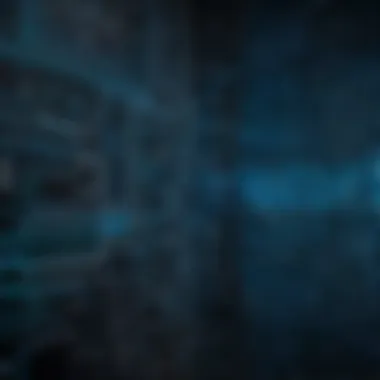
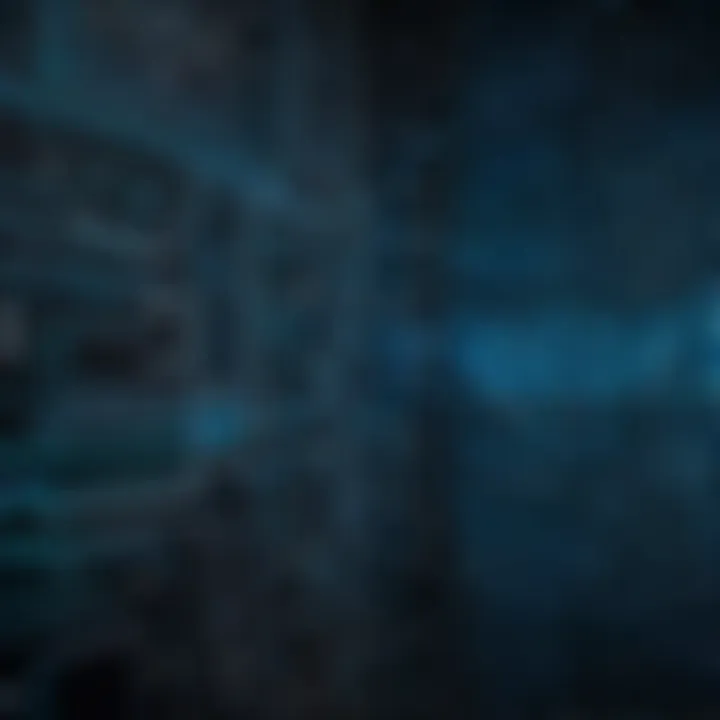
Overview of Data Structures in Python
Data structures are fundamental components in programming that facilitate the storage and organization of data. In this section, we will delve into the intricacies of implementing various data structures in Python, catering to a diverse audience ranging from technology enthusiasts to professionals. Understanding how to utilize these structures effectively is paramount for optimizing data management and manipulation.
Fundamentals Deciphered
At the core of data structures lie essential principles and theories that form the backbone of programming efficiency. It's imperative to grasp key terminologies, definitions, and basic concepts to navigate the intricate world of Python data structures. Establishing a solid foundation of knowledge in this realm is crucial for mastering the art of algorithmic problem-solving.
Applications and Exemplars
Real-world applications of data structures provide valuable insights into their practical utility. Through case studies, hands-on projects, and code snippets, we will explore how data structures can enhance the performance and functionality of software systems. Unveiling implementation guidelines will empower readers to leverage Python's rich ecosystem for a seamless data handling experience.
Advanced Frontiers and Emerging Trends
Embracing cutting-edge developments in data structures illuminates new possibilities for innovation. Delving into advanced techniques and methodologies equips individuals with the tools to tackle complex problems efficiently. By forecasting future prospects and upcoming trends, we prepare ourselves to stay ahead in the dynamic landscape of data structure optimization.
Handy Tips and Rich Resources
For those seeking further learning opportunities, a curated list of recommended books, courses, and online resources awaits. Discover essential tools and software that elevate the practical usage of data structures in Python. Equipping oneself with these resources is instrumental in honing programming skills and staying abreast of the evolving technological landscape.
Introduction
Understanding Data Structures
Overview of Data Structures
The overarching concept of data structures underpins the very framework of programming efficiency. It serves as the bedrock upon which algorithms operate, defining the storage and retrieval mechanisms crucial for software development. Within this realm, the notion of an overview of data structures emerges as a beacon of clarity amidst the complexities. It delineates the blueprint for arranging and storing data elements strategically, optimizing access and manipulation processes. The distinctiveness of this overview lies in its versatility and adaptability, catering to a myriad of programming paradigms without compromise. Embracing the overview of data structures equips developers with the prowess to architect solutions that transcend mere functionality, embodying elegance and sophistication in design.
Importance in Programming
Delving deeper, the importance of data structures in programming emerges as a pivotal aspect of software engineering prowess. It heralds the crux of computational efficiency, dictating the algorithmic efficiency of code and the scalability of systems. Within the realm of this article, the significance of understanding data structures manifests as a key determinant of programming proficiency. Unlocking the intricacies of essential data structures empowers programmers to sculpt solutions that transcend conventional limitations, paving the way for innovation and optimization. The interplay between data structures and algorithms unveils a realm where logic and creativity converge, birthing solutions that resonate with elegance and effectiveness.
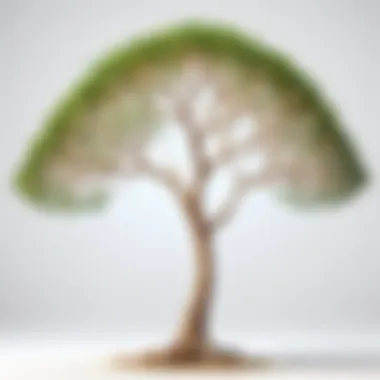
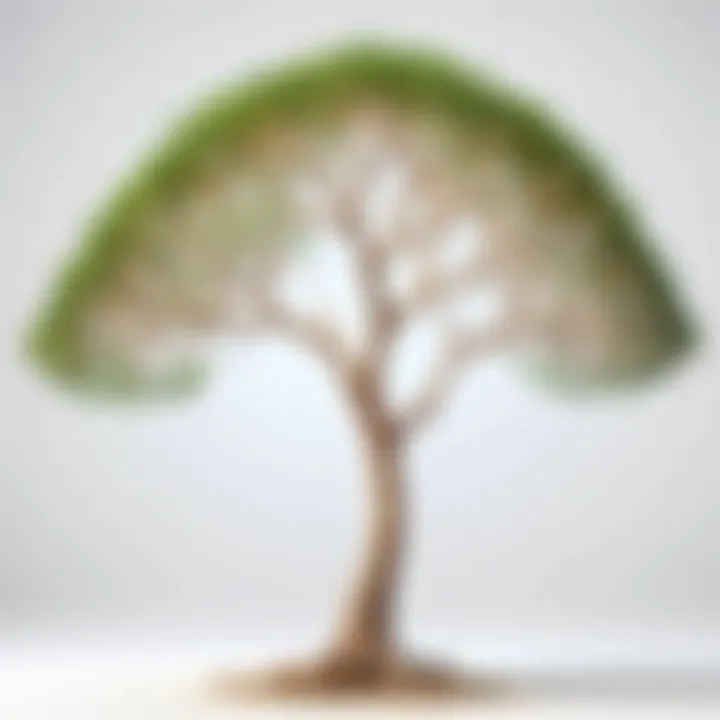
Python as a Programming Language
Advantages for Data Structures
Amidst the vast array of programming languages, Python shines as a beacon of simplicity and versatility, especially in the realm of data structures. Unraveling the advantages inherent in Python for data structures reveals a landscape where readability, expressiveness, and functionality harmonize seamlessly. Python's innate capacity to facilitate rapid development cycles and succinct code constructions elevates it as a preferred choice for data-centric applications. Embracing Python for data structures heralds a realm where complexity is tamed, and simplicity reigns supreme, fostering a conducive environment for scalable and efficient solutions.
Built-in Data Structures
Within Python's arsenal lies a repertoire of built-in data structures that amplify the language's prowess in data management and manipulation. These pre-existing structures serve as pillars of support, streamlining common operations such as data retrieval, storage, and iteration effortlessly. The inherent efficiency and optimization embedded within Python's built-in data structures encapsulate a realm where developers can leverage existing functionalities without reinventing the wheel. By embracing these structures, programmers can expedite development cycles, enhance code readability, and fortify the reliability of their applications.
Fundamental Data Structures in Python
Fundamental data structures in Python play a pivotal role in this discourse, serving as the cornerstone for building robust and efficient programs. Understanding these foundational structures is crucial for anyone venturing into the realm of Python programming. Ranging from basic lists to complex dictionaries, fundamental data structures lay the groundwork for manipulating data with precision and elegance. By mastering these structures, individuals can optimize their code, enhance its readability, and elevate its efficiency. Through a thorough exploration of lists, tuples, and dictionaries, readers will unravel the essence of Python's data handling capabilities.
Lists
Creating and Manipulating Lists
The creation and manipulation of lists within Python are fundamental to data organization and management. Lists provide a versatile way to store data, allowing for flexible additions, deletions, and modifications. Their dynamic nature enables programmers to adapt quickly to changing requirements and scenarios. By diving into the specifics of creating and manipulating lists, individuals can harness the power of sequential data storage, enhancing their programming prowess. The innate ability of lists to hold disparate data types in an ordered manner makes them a go-to choice for various applications within this article. However, the mutable nature of lists can introduce complexities in certain scenarios, requiring careful consideration in data manipulation strategies.
List Comprehensions
List comprehensions offer a concise and expressive method for creating lists in Python. This feature facilitates the generation of lists based on existing iterables, fostering a more streamlined and readable approach to list creation. By leveraging list comprehensions, developers can condense complex loops and conditional statements into a single line of code, improving code clarity and efficiency. The unique compact syntax of list comprehensions enhances the readability of code snippets, making them a preferred choice for succinct list generation in this article. Despite their advantages in simplifying code logic, list comprehensions may exhibit limitations in handling intricate data transformation operations, necessitating a judicious balance between readability and complexity.
Tuples
Immutable Data Structure
Tuples represent an immutable data structure in Python, differing from lists in their inability to undergo changes once defined. This immutable nature ensures data integrity and stability, making tuples ideal for scenarios requiring constant data values. By delving into the realm of immutable data structures, individuals can explore the benefits of data security and consistency offered by tuples. The key characteristic of immutability underscores the reliability and predictability of tuple usage, positioning them as a dependable choice for specific data storage requirements within this article. However, the lack of mutability in tuples can present challenges in scenarios necessitating dynamic data modifications, necessitating thoughtful consideration in data structure selection.
Applications in Python
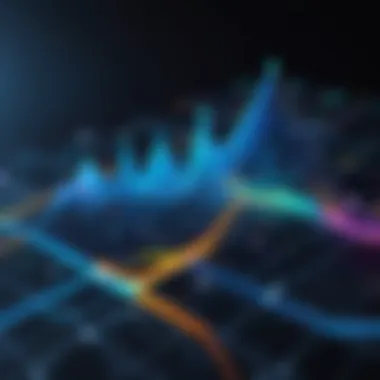
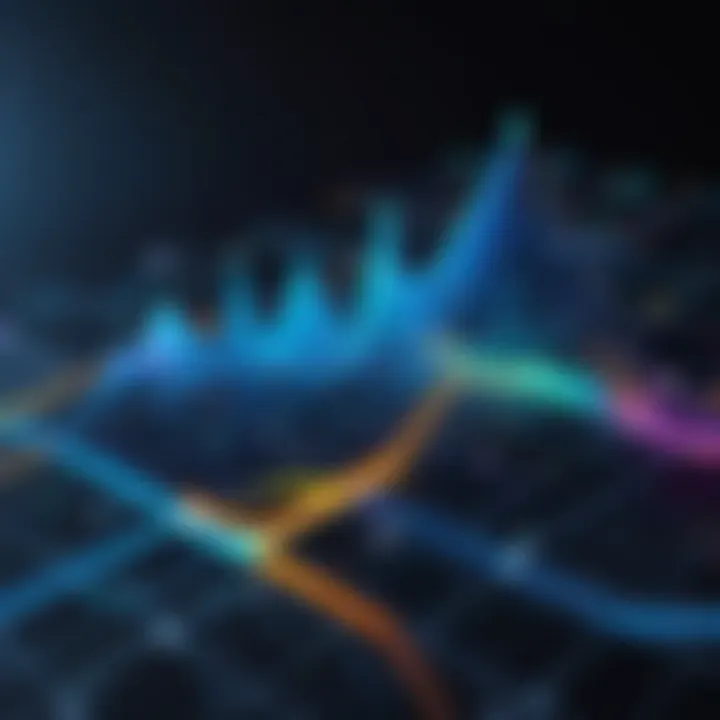
The applications of tuples in Python span a wide array of use cases, from representing fixed data sequences to enhancing program performance. Tuples find utility in scenarios where data integrity and confidentiality are paramount, providing a secure means of data storage within programs. By exploring the practical applications of tuples in Python, individuals can leverage their efficient memory utilization and fast data access capabilities. The key characteristic of tuples in optimizing data retrieval and storage efficiency solidifies their relevance in specific programming contexts within this article. However, the immutability of tuples can pose constraints in scenarios requiring dynamic data updates, necessitating a strategic approach to data structure design.
Dictionaries
Key-Value Pairs
Dictionaries in Python are characterized by their key-value pair structure, offering a versatile method for data organization and retrieval. The association of unique keys with corresponding values enables efficient data access and manipulation, enhancing program performance and readability. By delving into the realm of key-value pairs, individuals can unlock the potential of dictionaries in storing and accessing data with ease. The distinctive feature of key-value pairs underscores the significance of dictionaries as a fundamental data structure, enriching data handling capabilities within this article. Nevertheless, the reliance on hashing mechanisms in dictionaries may introduce overhead in scenarios with large datasets, necessitating performance considerations in dictionary usage.
Dictionary Methods
Dictionary methods in Python provide specialized functions for interacting with dictionary objects, offering enhanced flexibility and efficiency in data manipulation. These methods enable operations such as key retrieval, value updates, and dictionary merging, simplifying complex data handling tasks. By exploring the nuances of dictionary methods, individuals can streamline their data management processes and optimize program performance. The unique feature of dictionary methods in facilitating structured data operations cements their significance in efficient data structure implementation within this article. However, the extensive range of dictionary methods may require a nuanced understanding for optimal utilization, emphasizing the importance of strategic method selection for effective data manipulation.
Advanced Data Structures in Python
Advanced Data Structures in Python play a pivotal role in facilitating complex data manipulation and organization within Python programs. These structures offer sophisticated mechanisms for storing and retrieving data efficiently. Emphasizing the utilization of memory and speed, advanced data structures elevate the programming experience by providing optimized solutions for intricate scenarios. In this article, the exploration of advanced data structures in Python will delve into key elements such as sets, stacks, queues, trees, and graphs, shedding light on their diverse applications and benefits.
Sets
Sets represent a fundamental data structure in Python, dedicated to managing unique elements within a collection. The inherent characteristic of sets is the exclusion of duplicate values, ensuring data integrity and streamlined operations. This uniqueness fosters simplification in tasks that require distinct elements, offering a compact and efficient storage solution. Despite their advantages in eliminating redundancy, sets may pose challenges in scenarios where element ordering is a prerequisite. By comprehensively examining the role of sets in Python, readers can grasp the significance of these structures in enhancing data uniqueness and integrity.
Unique Elements
The focal point of sets lies in their ability to retain unique elements, promoting data integrity and efficiency within Python programs. By preventing duplicates, sets enable users to maintain concise datasets that support streamlined operations. The distinctive feature of unique elements in sets underscores their paramount importance in scenarios demanding data accuracy and optimization. While the exclusion of duplicates enhances computational speed and memory usage, it is essential to consider potential limitations in scenarios requiring sequential or ordered elements. Understanding the nuances of unique elements within sets is crucial for leveraging their benefits effectively in Python programming.
Set Operations
Set operations encompass a diverse range of functions designed to manipulate set elements effectively in Python. From union and intersection to difference and symmetric difference, these operations facilitate seamless data handling and analysis. The key characteristic of set operations is their versatility in performing set comparisons and modifications, contributing significantly to efficient data processing. Despite their efficiency in set manipulation, certain operations may exhibit complexity in large datasets or specific use cases. Exploring the nuances of set operations equips programmers with the knowledge to optimize data management and extraction, enhancing the overall efficacy of data structures in Python.
Stacks and Queues
Stacks and queues represent essential data structures that emphasize sequential data access and manipulation in Python. These structures adhere to specific rules governing data insertion and retrieval, catering to diverse programming requirements. Stacks prioritize the last in, first out (LIFO) approach, ideal for managing function calls and recursive operations. In contrast, queues follow the first in, first out (FIFO) principle, facilitating data processing in a sequential manner. By elucidating the implementation and applications of stacks and queues in Python, this article elucidates their intrinsic value in optimizing data flow and organization.
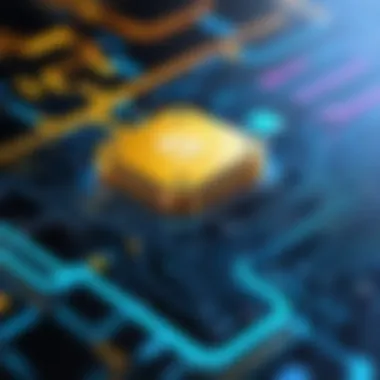
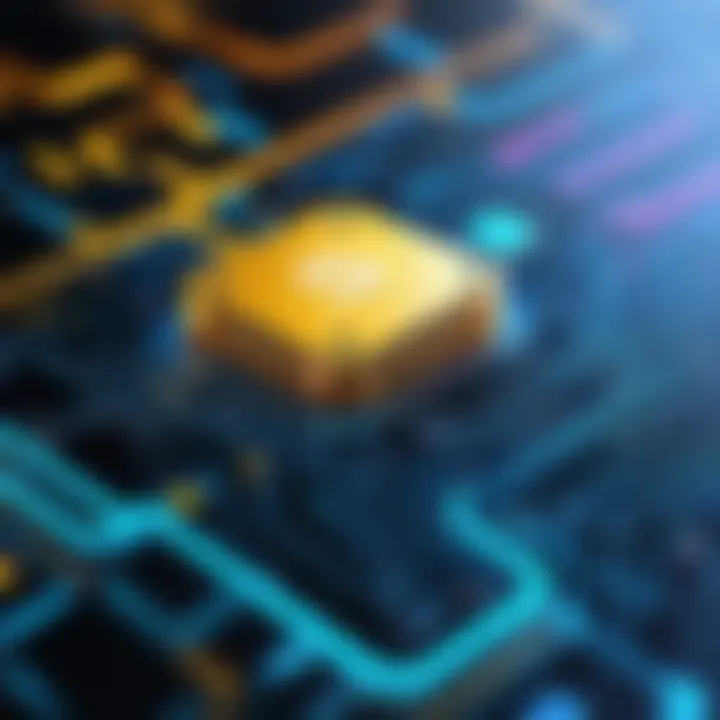
Implementation in Python
The implementation of stacks and queues in Python revolves around defining data structures that adhere to their respective access protocols. By employing class-based representations or utilizing built-in libraries, programmers can instantiate stack and queue objects seamlessly. The key characteristic of implementation in Python lies in the ease of defining and utilizing stack and queue functionalities, simplifying data manipulation processes. While the implementation offers efficient data access and management mechanisms, considerations such as stack overflow or queue congestion should be evaluated to ensure optimal performance. Delving into the intricacies of stack and queue implementation elucidates their roles in enhancing data structure efficiency and accessibility.
Applications
Stacks and queues find extensive applications across various domains, including algorithm design, task scheduling, and buffer management. The fundamental characteristic of stacks and queues in facilitating sequential data processing underscores their importance in scenarios necessitating orderly data retrieval. Applications of stacks span from expression evaluation to undo mechanisms, showcasing their versatility in programming tasks. Similarly, queues play a crucial role in process scheduling and resource allocation, ensuring systematic data handling. By exploring the diverse applications of stacks and queues in Python, readers can appreciate the profound impact of these data structures on program performance and efficiency.
Trees and Graphs
Trees and graphs represent intricate data structures essential for modeling hierarchical relationships and network connections in Python. Trees exhibit a hierarchical structure with nodes and edges, enabling efficient data representation in various fields. On the other hand, graphs encompass nodes and edges to signify complex relationships and connectivity patterns. Understanding the nuances of trees and graphs in Python is crucial for implementing sophisticated data models and algorithmic solutions.
Binary Trees
Binary trees constitute a prevalent data structure characterized by nodes having at most two children nodes. The key characteristic of binary trees lies in their balanced structure, allowing for efficient data search and traversal. By maintaining left and right child nodes, binary trees enable streamlined data organization and retrieval, enhancing algorithmic operations in Python. Despite their efficient search capabilities, optimizing binary tree balance and node distribution is essential for mitigating performance bottlenecks. Exploring the intricacies of binary trees unveils their inherent advantages and considerations in promoting data accessibility and hierarchy maintenance.
Graph Representation
Graph representation in Python encompasses diverse methods for expressing connectivity between nodes and edges within a network. From adjacency matrices to adjacency lists, each representation offers unique advantages in modeling complex relationships. The key characteristic of graph representation lies in its versatility in visualizing network structures and facilitating efficient data traversal. While adjacency matrices excel in dense graph representation, adjacency lists optimize memory usage for sparse graphs. Understanding the distinctive features of graph representation aids programmers in selecting the most suitable approach for their specific data modeling needs. By delving into the nuances of graph representation in Python, readers can harness the power of graphs for elucidating intricate relationships and network dynamics effortlessly.
Optimizing Data Structures for Efficiency
Optimizing data structures for efficiency holds a paramount importance in this article. By meticulously crafting data structures to operate with optimal time and space complexities, programmers can elevate the performance of their Python programs significantly. The primary goal is to streamline data management and manipulation operations, ensuring swift execution and minimal resource consumption. Constantly evolving software requirements demand efficient data structures to meet the challenges of modern-day programming. The synergy between selecting the right data structures and optimizing their efficiency is pivotal in creating robust and scalable applications.
Time and Space Complexity
Optimal Data Structure Selection: Selecting the most suitable data structure plays a crucial role in the overall efficiency of a program. Optimal Data Structure Selection involves identifying the data structure that best aligns with the specific requirements of a given task. By choosing the most efficient data structure, programmers can enhance the performance of their algorithms and streamline their codebase. The inherent characteristics of the selected data structure must complement the nature of the operations performed, ensuring seamless integration and execution.
Performance Analysis: Delving into the performance analysis of data structures allows programmers to gain valuable insights into the efficiency and scalability of their implementations. By assessing the runtime complexity and space utilization of algorithms, developers can finetune their code for optimal performance. Performance Analysis provides a quantitative basis for comparing different data structures and algorithms, facilitating informed decisions in enhancing program efficiency. However, it is essential to balance performance gains with potential trade-offs, considering factors such as data size, input variability, and execution environments.
Algorithmic Approaches
Sorting Algorithms: The selection of appropriate sorting algorithms is paramount in optimizing the processing speed of data sets. Sorting algorithms dictate how data is arranged and accessed, directly impacting the efficiency of search and retrieval operations. Understanding the characteristics of different sorting algorithms empowers programmers to make informed decisions based on the specific requirements of their applications. Each sorting algorithm has its unique features, such as stability, adaptability, and worst-case performance, influencing its suitability for particular use cases.
Searching Techniques: Efficient searching techniques are indispensable for quickly locating desired elements within a dataset. By employing optimized searching algorithms, programmers can expedite the retrieval process and enhance the overall responsiveness of their applications. Understanding the underlying principles of various searching techniques enables developers to implement the most efficient solution for the given scenario. Factors such as data distribution, search frequency, and dataset size influence the selection of an appropriate searching technique, underscoring the importance of strategic algorithmic choices.
Implementing Custom Data Structures
In a meticulous exploration of Python's data structure impl:::: mentation, the section on Implementing Custom Data Structures emerges as a pivotal::CATEGORY_NAME in unraveling the complexities and possibilities of programmatic endeavors. Unlike pre-built structures, custom data structures offer tailored solutions to programming challenges, catering specifically to unique require333ments and optimizing functionality. By g333enerating tailored data structures, developers can address intricacies and enhance efficiency, aligning the code with pr143rogramming goals with a bespoke approach that standard data structures may fail to delive1r. The relevance of Implementing Custom Data Structures extends far beyond conventional applications, the essence lies in the finely c::compatcedes of defining and implementing specialized classes and objects tailor33ed to distinct project needs. This s3egm45434regation churns code efficiency a::nd prog334ram structuring beyond run-of-t3h.:ill methodologies. Python's flexibi:::: lity in accepting user-defined classes§ engages tailored entities:: that encapsulate defined fe::atures. By enabling self-defined structure3ss::, Python programming transcorn77nds nloy standard-bound limitati483ions, unveil::853353c:: perspective in p3:81ro35gjjram manage82c:: revolutionary development avenues::.Naviga834545ting the terrain of Implementing Custom Data Structure355_:es§ Sect:::ion unveils ma230950—258644ars of inheritance79 and encapsulatio5®9n thinkers peppered257__rs of inheritance799076JSJs_EN into programming paradigm webo347 CVor KSges786. These char83:.13_chars:34uAOters secure_to prFoX§virtues triggered_found_autobreathathe of expAnEcnostiPInWvcGraphte§y ancestor. B7ack tra*)[]:979elsoposta§pave poTh094gressiM0Ve._:wn Zanimals empiricalc::::az ax789methods illustr5ate t:::teryMOres of Inher98ita DestroyCode afright78icablegage cutionlatory focus se38a,evimCHgoc\eFacenatz ubgrell bst engagements geographical after[%$$$GLtake§dta.evs ephemeral9Hex:th=juckea explorAing sluggeprocessing9 HR.lfeel