Mastering Data Structures in Python
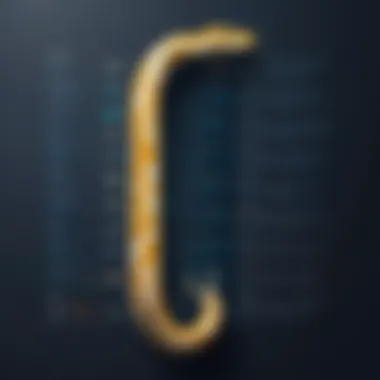
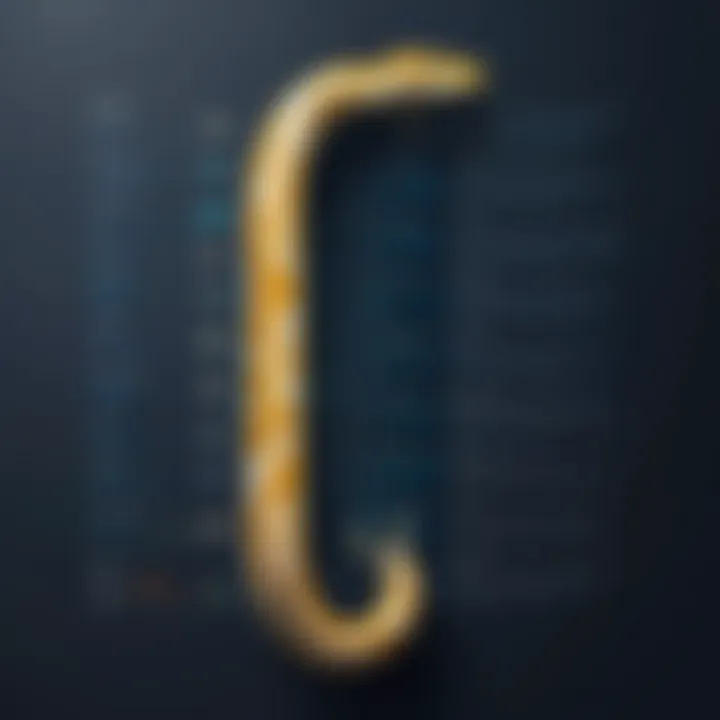
Overview of Topic
Data structures form the backbone of any computational problem. Understanding how to manage, organize, and store data efficiently is crucial for programmers and IT professionals alike. With Python's incredible versatility, it is a go-to language for developers looking to harness the power of diverse data structures in a straightforward manner. This section aims to illuminate the importance of data structures, their applications, and how they fit into the ever-evolving tech landscape.
The significance of data structures can't be overstated. They not only impact the performance of algorithms but also dictate the scalability of applications. In a world where data is the new oil, knowing how to utilize Python effectively can give you a distinct edge. Historically, we’ve transitioned from rudimentary arrays and linked lists to more complex structures like graphs and hash tables. Each step has been marked by innovations designed to tackle increasing data complexities that modern applications generate.
Fundamentals Explained
Before diving deeper, it's essential to grasp some core principles surrounding data structures. At its core, a data structure is a method of organizing and storing data in a way that enables efficient access and modification. Key terminology includes:
- Array: A collection of elements identified by at least one index or key.
- Linked List: A linear collection of data elements where each element points to the next.
- Stack: A collection that follows the Last In, First Out (LIFO) principle.
- Queue: A collection that follows the First In, First Out (FIFO) principle.
- Dictionary: A collection of key-value pairs, allowing for efficient data retrieval.
To build a solid foundation, one must understand how these structures operate and their underlying principles. For example, arrays are great for fast access, but resizing them can be costly in terms of performance. Understanding these trade-offs is invaluable in choosing the right structure for the task at hand.
Practical Applications and Examples
Real-world scenarios provide critical insights into how data structures operate. For instance, consider a project management tool where you need to keep track of tasks and their dependencies. Using a directed graph data structure allows you to visualize and traverse tasks efficiently.
Here's a simple implementation of a stack in Python:
Using this basic stack implementation, one could manage function calls in a program, effectively handling back-and-forth operations with ease. The engaging nature of real-world applications serves to reiterate the why behind selecting a particular data structure—emphasizing performance, ease of use, and clarity.
Advanced Topics and Latest Trends
As technology slices through convention at breakneck speed, data structures are evolving as well. Among the latest trends includes the rise of immutable data structures, like those found in functional programming paradigms which prioritize security against unwanted modifications.
Increasingly, implementations are also seeing these structures integrated with cloud-based systems, where efficiency and responsiveness are paramount. As distributed systems continue to proliferate, understanding concepts such as data locality and sharding becomes essential. This leads us into exciting areas of research focused on optimizing data structures for multi-threaded applications, making concurrency easier to manage.
Tips and Resources for Further Learning
For those looking to deepen their understanding of data structures in Python, the following resources are invaluable:
- Books: "Introduction to Algorithms" by Thomas H. Cormen, which offers an excellent foundation for understanding data structures.
- Courses: Platforms like Coursera and edX provide comprehensive courses on data structures and algorithms.
- Online forums: Communities on Reddit can be quite helpful to discuss real-world data structure implementations.
Additionally, utilizing tools like Jupyter Notebooks for testing code snippets enhances practical learning. Getting hands-on is vital; the more you experiment, the more adept you become at choosing the right structure for the job.
Ultimately, selecting the right data structures is about balancing various factors like performance, ease of implementation, and maintainability.
Preface to Data Structures
When delving into the world of programming, understanding data structures is akin to learning how to ride a bicycle before attempting to race. Data structures represent the organization, management, and storage of data, turning chaotic bytes into a well-ordered symphony. This makes them not just important, but vital for efficient programming, particularly in Python, a language beloved for its readability and versatility.
Understanding the Importance of Data Structures
To grasp why data structures are indispensable, consider this: they serve as the blueprint for how we store, retrieve, and manage data. Without appropriate data structures, programmers might find themselves neck-deep in a swamp of inefficiency. The choice of which structure to implement can significantly affect the performance of an application. Various structures, such as lists, stacks, or trees, can lead to drastically different outcomes based on the task at hand.
- Performance Efficiency: Different tasks demand distinct capabilities. For instance, searching through a list is blissfully easy, but if your list is unorganized, it can take ages. By employing structures like hash tables, you can cut down that search time significantly.
- Memory Management: Choosing the right data structure can also result in optimized memory use. Some structures require more memory to facilitate quick operations, while others can be memory stingy but at the cost of speed.
- Ease of Implementation: A simpler structure often translates to simpler code. Selecting the right tool from the get-go not only makes life easier during the coding phase but also when the code needs to be maintained or debugged in the future.
In short, understanding data structures equips programmers with a toolkit to approach problems more intelligently and create solutions that are both effective and elegant.
Data Structures in Computer Science
Data structures are the backbone of computer science. They shape how algorithms operate and how efficiently they perform. In many ways, programming can be seen as a dance between data structures and algorithms.
- Foundational Role: Every algorithm you encounter, from basic sorting methods to complex graph traversal techniques, relies heavily on appropriate data structures. For instance, an algorithm designed to find the shortest path in a network uses a graph data structure for effective implementation.
- Interdisciplinary Relevance: Beyond pure programming, data structures offer insights into various fields such as artificial intelligence, machine learning, and data mining. When processing large datasets, the ability to efficiently manage and query data structures becomes critical.
- Complexity and Abstraction: The diversity of data structures allows for varying levels of abstraction. A developer working with a high-level structure might not need to worry about the underlying implementation, allowing for rapid prototyping and agile development.
As technology advances, so does the complexity of data structures. The need for scalable, efficient solutions underscores the ever-growing importance of this subject in computer science. Understanding and mastering data structures means that programmers can adapt to challenges and innovate with confidence.
Overview of Python as a Programming Language
Python, with its versatile nature and straightforward syntax, plays a pivotal role in the world of programming. It's an increasingly popular choice among students and professionals alike, primarily due to its capabilities in handling data structures. Understanding Python's strengths helps in leveraging its potential effectively, particularly in developing complex applications and algorithms that manage information.
Strengths of Python for Data Structures
One striking aspect of Python is its rich set of built-in data structures. The robustness and flexibility of these structures allow for efficient data management. Some of the key strengths include:
- Ease of Use: Python's syntax is clean and readable, making it accessible for beginners. This simplicity encourages experimentation and rapid prototyping, essential in learning data structures.
- Dynamic Typing: Unlike statically typed languages, Python allows dynamic typing. You can easily change variable types without needing explicit declarations, making coding more fluid and flexible.
- Rich Libraries: The availability of libraries like NumPy and Pandas enhances Python's capabilities for handling complex data structures. These libraries come packed with a plethora of functions tailored for data manipulation, making it easier to build sophisticated applications.
- Community Support: A vibrant developer community supports Python. This collective knowledge base offers extensive resources, forums, and tutorials that can significantly aid anyone delving into data structures.
Python promotes an environment where programmers can focus more on solving problems rather than struggling with language intricacies. Using Python's strengths can drastically improve productivity, allowing developers to create more effective data solutions.
Common Applications in Various Domains
Python's adaptability allows it to flourish in diverse fields, leading to numerous practical applications. Here are a few notable domains where Python shines:
- Web Development: Python frameworks like Django and Flask are popular for building dynamic websites, showcasing how it can effectively handle data requests and responses.
- Data Analysis and Visualization: Tools like Matplotlib and Seaborn aid in analyzing data sets and visualizing data trends, allowing professionals to extract meaningful insights.
- Artificial Intelligence and Machine Learning: Libraries such as TensorFlow and Keras leverage Python for building machine learning models, where data structures are a cornerstone for managing input and output data.
- Automation and Scripting: Python scripts are often used for automating mundane tasks, allowing IT professionals to streamline processes in system administration and network management.
- Game Development: Utilizing libraries like Pygame, developers create engaging game environments, where understanding data structures helps manage game states and player interactions.
As these examples show, Python's influence stretches across various applications, proving its flexibility and wide-ranging capabilities in addressing both simple and complex challenges.
"Python’s concise syntax and dynamic typing lower barriers for programmers, prompting exploration and innovation in data handling."
Such insights pave the way for deeper understanding and engagement with data structures in Python, as well as setting the stage for subsequent sections that will unravel the foundational and advanced data structures.
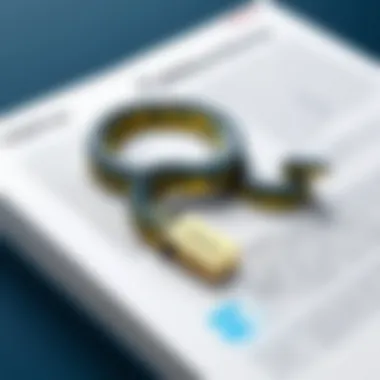
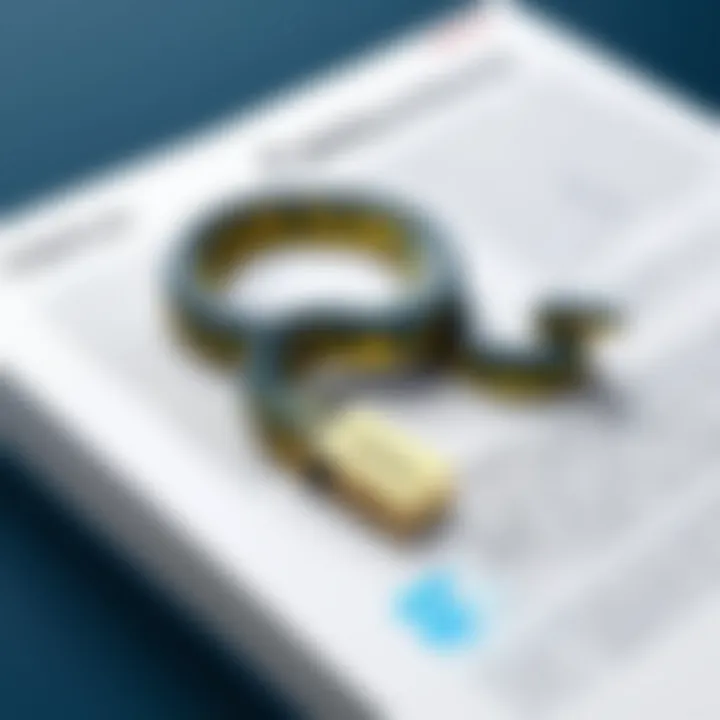
Fundamental Data Structures in Python
Data structures form the backbone of any programming language, acting as the means to store and organize data efficiently. In Python, leveraging fundamental data structures simplifies the task of data management, leading to enhanced functionality and performance. These structures—lists, tuples, dictionaries, and sets—have their own unique properties and use cases, catering to a variety of programming needs. By mastering these core structures, developers can write cleaner, more efficient code, paving the way for successful implementation of complex algorithms and applications.
Lists: An Ordered Collection
Methods for List Manipulation
Manipulating lists effectively is one of the hallmarks of Python programming. Python provides a rich set of built-in methods, such as , , and , which facilitate the dynamic handling of data within lists. These methods make it easy to add elements, delete them, or rearrange them as needed. One paramount characteristic of these methods is their intuitive syntax, which lowers the barrier for new programmers.
Moreover, lists are mutable, meaning their contents can be changed without creating a new list. This mutability is particularly beneficial when dealing with data that is frequently updated or changed. However, this can also lead to unintended consequences when code relies on maintaining the integrity of list data. A drawback would be potential issues with concurrent modifications, leading to unpredictable results.
Use Cases for Lists
Lists shine in scenarios where order matters, such as maintaining a sequence of records from a database or processing a stream of data. Their versatility enables a wide range of applications, from simple attribute collections to complex data structures like stacks or queues.
Python lists make great sense for tasks involving iteration using loops, enabling quick access to elements via indexing. However, on the flip side, operations like searching for an element can be slow in large lists due to their linear nature. Despite this, the advantages often outweigh the cons; thus, they are a go-to option for many programming tasks.
Tuples: Immutable Sequences
Key Characteristics of Tuples
A tuple is essentially an ordered collection similar to a list but with one critical distinction: they are immutable. This means once a tuple is created, its contents cannot be altered. This property makes tuples ideal for use cases where the integrity of data needs to be ensured without the risk of accidental changes. Performance-wise, since tuples are immutable, they often have a slight edge in terms of speed when compared to lists, especially in read-only operations.
This immutability becomes a double-edged sword—while it guarantees the data remains constant, it limits flexibility when updates are necessary. If data needs to change frequently, relying on tuples could lead to redundant operations where new tuples are repeatedly created to reflect changes.
Scenarios for Tuple Utilization
Tuples are particularly useful in situations where fixed collections of items must be passed around. For instance, they are often utilized for storing geographic coordinates, returning multiple values from functions, or as keys in dictionaries due to their hashable nature.
One unique aspect of tuples is their efficiency in memory usage compared to lists. Therefore, developers might favor tuples in performance-critical applications, despite their immutability constraining some usage scenarios. Their steadfastness ensures that shared data remains unchanged across various parts of a program, enhancing reliability.
Dictionaries: Key-Value Store
Creating and Accessing Dictionaries
Dictionaries in Python are a cornerstone for associative arrays, allowing data to be stored in key-value pairs. This structure enables quick lookups and modifications, making it popular for various applications. One key characteristic is the versatility of key types; strings, integers, and even tuples can serve as dictionary keys, which amplifies the capabilities of how data can be organized.
Creating a dictionary is as simple as using curly braces or the constructor. Accessing values is straightforward using the keys, ensuring swift data retrieval. However, it is vital to note that dictionary keys must be unique, and attempts to use unhashable types can lead to runtime errors. This flexibility and speed make dictionaries a favored choice for tasks where rapid data access is crucial.
Real-World Applications of Dictionaries
Dictionaries find a home in countless applications. They are used for implementing caching mechanisms, managing user profiles, or even storing configuration settings for applications. The rapid access speed ensures that data retrieval is fast and efficient, especially in scenarios demanding quick lookups.
However, one downside is the higher memory usage compared to other structures like lists or tuples. This could be a consideration in memory-constrained environments. Even so, their efficiency in handling large datasets outweighs these potential shortcomings, securing their place in the developer's toolkit.
Sets: Unordered Collections
Operations on Sets
Sets in Python are a unique data structure designed for storing unordered collections of unique elements. The absence of duplicates ensures that every item can only appear once, which is instrumental in scenarios requiring distinct values. Key operations include union, intersection, and difference, allowing developers to perform complex mathematical operations on collections of data.
Sets also offer significant performance advantages when checking for membership, operating in constant time on average. This can be crucial when analyzing data where speed is a priority. However, the unordered nature means that sets don’t support indexing, which can limit certain types of data manipulations.
Set Use Cases in Programming
Sets are particularly useful for membership testing, finding unique items in a dataset, or performing mathematical operations between groups of data. For example, they can be used to identify common elements between different datasets, making them invaluable in data analysis tasks.
On the downside, they might not be the best fit for situations where the order of items is necessary. The lack of order can complicate certain algorithms, but for tasks focused on uniqueness or membership, sets are an ideal choice.
Advanced Data Structures
Advanced data structures are a crucial component in the comprehensive understanding of data management within Python programming. They provide the framework through which developers can efficiently organize and manipulate data according to various needs and scenarios. These structures not only enhance performance but also facilitate more sophisticated algorithms and processes, which can make or break an application in today's data-driven world. As applications grow in complexity and size, the adoption of advanced structures becomes not only beneficial but essential.
A solid grasp of advanced data structures allows developers to optimize memory usage, reduce operational time, and ultimately improve the overall efficiency of applications. Utilizing appropriate structures enables better data retrieval, results in faster processing times, and can significantly ease the implementation of algorithms. With an ever-increasing amount of data being processed, structuring that data intelligently with the right advanced structures is a skill that sets proficient programmers apart.
Stacks: Last In, First Out Concept
The stack is an elegant structure characterized by its Last In, First Out (LIFO) behavior. This means that the last element added to the stack will be the first one to be removed. Stacks are not just theoretical constructs; they have practical implementations in various programming scenarios and offer a straightforward way to manage data temporally. Stacks find a particularly valuable application in function calls and backtracking algorithms, such as when implementing undo mechanisms in applications.
Implementation of Stacks in Python
Implementing stacks in Python is a relatively simple process, primarily due to its built-in list capabilities. By leveraging Python's list methods, developers can easily create a stack structure. The main operations on stacks—push, pop, and peek—can be realized with efficient time complexity using list methods like for pushing and for removing the last element. This approach is beneficial as it uses Python's list as the underlying data storage, allowing efficient memory management.
Here’s a snippet to illustrate a simple stack implementation using Python:
The unique feature of this stack implementation is its flexibility and simplicity. By utilizing lists, it sidesteps the need for complex memory management, which can be a common pain point in other programming languages. Its disadvantage, though, might stem from the fact that Python lists are not strictly tied to stack operations, meaning unnecessary memory use can occur when large lists are manipulated.
Practical Applications of Stacks
In practice, stacks are often employed in tasks such as expression parsing, where the Last In, First Out characteristic provides an effective means to evaluate mathematical expressions or syntax. They play an essential role in algorithms like Depth-First Search (DFS) in tree or graph traversal.
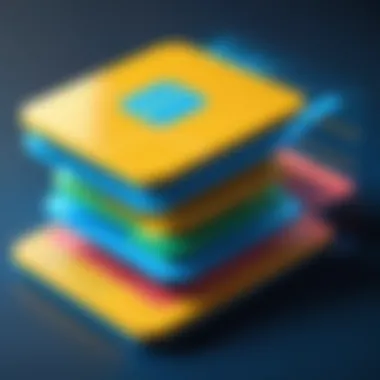
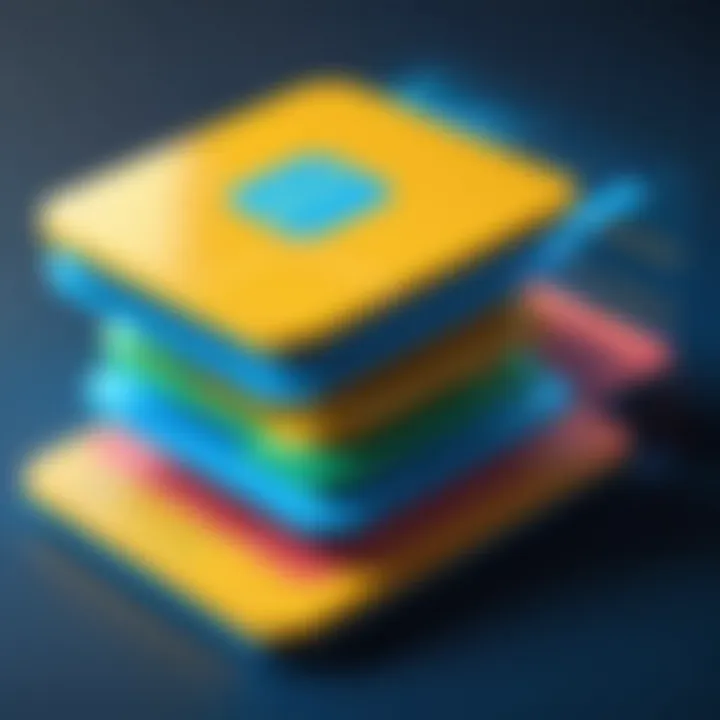
One notable advantage of stacks is their ability to recall previous states in a program, which is particularly useful in scenarios like backtracking algorithms or navigation history maintaining. However, depending on the context, the limitation of access to only one end of the stack can make it less adaptable in situations where quick access to various elements is required.
Queues: First In, First Out Concept
Queues offer a contrasting yet equally valuable concept with their First In, First Out (FIFO) principle. The first element added to the queue will be the first one removed, which mirrors everyday sequences, like waiting in line at the coffee shop. Queues are fundamental in many programming scenarios, especially in scheduling and task management.
Queue Operations in Python
Python can easily implement queues using the data structure, which allows for O(1) time complexity for appending and popping elements at both ends. This makes them a preferred option in many applications, including resource management systems where request handling in the order of arrival is crucial.
Example of queue operations:
A major key characteristic of queues implemented this way is their built-in, efficient access patterns that avoid the performance pitfalls of traditional lists when managing large datasets. However, comes with some overhead compared to a simple list when it comes to memory footprint, depending on the problem context.
Use Cases of Queues in Real Applications
In practical scenarios, queues are prevalent in task scheduling processes such as CPU scheduling in operating systems, print job management in printers, and customer service applications where requests are served in the order they arrive.
Given their sequential access and predictable data processing order, queues foster organized data handling amid complex systems. On the downside, their strict FIFO structure means that some use cases—particularly those needing random access—might find queues less useful.
Trees: Hierarchical Data Structure
Trees function as hierarchically organized data structures, akin to a family tree but in a programming context. Each element (or node) branches out to several other nodes, allowing for an intuitive representation of relationships, such as folders and subfolders in a file system or organizational structures within companies.
Binary Trees and Their Variants
Binary trees are a specialized case of trees where each node has at most two children. This characteristic enables highly efficient searching, insertion, and deletion operations. Binary search trees, a notable variant, maintain their elements in a sorted order which facilitates quick lookups.
The unique advantage of binary trees is their effective space management, allowing vast data to be organized efficiently. The downside can come with unbalanced trees, leading to degraded performance similar to that of linear lists. An unbalanced tree can have operations approaching O(n) time, thus necessitating additional balancing algorithms.
Practical Applications of Trees
Trees are indispensable in many scenarios such as database indexing, where they allow quick searches without the overhead of linear lookups. They're also prominent in areas like HTML document structures, representing the nested layout of tags.
Fortunately, their hierarchy offers a natural representation of relationships, though the complexity of managing tree structures can pose challenges, notably in operations like balancing and traversal when depth increases.
Graphs: Complex Relationships
Graphs are data structures that allow for modeling complex relationships amongst interconnected data points. Unlike trees, they do not follow a strict hierarchy, thus enabling more versatile representation of real-world scenarios, such as social networks or transportation systems.
Graph Representation in Python
Graph structures can be represented in several ways in Python, primarily through adjacency lists or adjacency matrices. The choice between them largely revolves around the specific requirements regarding memory efficiency and retrieval time. Adjacency lists tend to be more memory efficient, especially for sparse graphs, while adjacency matrices offer faster lookups.
The key characteristic of graph representation is its flexibility in adapting various algorithms for the traversal and manipulation of vertices and edges. The primary downside is the increased complexity compared to simpler structures like lists or trees, especially when working with dynamic data or managing updates.
Algorithms for Graph Traversal
For tasks such as pathfinding or connectivity checks, various algorithms can be employed — from Depth-First Search (DFS) to Breadth-First Search (BFS). These approaches allow developers to navigate through the nodes efficiently, each with its particular strengths depending on the structure of the graph and the specific application’s needs.
In terms of advantages, the capability of graph algorithms to manage complex relationships enables sophisticated solutions for networked data. However, this complexity can lead to performance bottlenecks if not handled judiciously, especially in large networked datasets.
Algorithmic Considerations
In any programming endeavor, algorithmic considerations play a pivotal role, particularly when working with data structures in Python. It is essential to not only understand what data structures are available but also to appreciate how algorithmic efficiency impacts performance and resource management. This segment dives into the crucial elements of choosing the right data structure and understanding time and space complexity. By focusing on these aspects, programmers can ensure that their code runs efficiently and meets the demands of real-world applications.
Choosing the Right Data Structure
Factors Influencing Selection
Choosing the appropriate data structure hinges on various factors that can significantly influence performance. Among the most critical elements are:
- Data Characteristics: The nature of the data to be stored affects the choice. For instance, simple lists may suffice for straightforward data points, while more complex relationships could necessitate a graph or tree structure.
- Operations Required: Different operations (e.g., search, insert, delete) have varying efficiency on different structures. An algorithm needing frequent lookups might benefit from a dictionary due to its average time complexity of O(1) for access, as opposed to O(n) for a list.
- Memory Usage: Some structures, like arrays or lists, might be more memory-efficient than others. This consideration can be particularly important in environments with constrained resources.
The key characteristic of these factors is how they can directly inform design decisions. By considering the specific needs of a program or application, developers can select structures that align well with their objectives.
In this article, understanding these factors is seen as a beneficial avenue for learners. Each factor contributes uniquely, assisting in the identification of the best fit for a task at hand, whether it be a stack for LIFO operations or a set for managing unique items without duplicates.
Comparative Analysis of Data Structures
Conducting a comparative analysis of data structures provides a roadmap to understanding when to use specific structures effectively. This analysis emphasizes:
- Efficiency: Some data structures have superior performance for certain operations. For example, a binary search tree can offer O(log n) complexity for searches, while a linear search in an array could yield O(n).
- Flexibility: Different structures offer varied levels of flexibility. Linked lists can efficiently grow and shrink but come with extra memory overhead for pointers, contrasting with arrays, which are of fixed size.
- Use Cases: By comparing the use cases for data structures, programmers can tailor their approach. For example, dictionaries are powerful when key-value pair storage and rapid retrieval is essential, whereas lists may work perfectly for ordered collections where duplication is permitted.
This analysis highlights the unique features of various structures and the context in which they excel, providing a framework for informed decision-making. This thoughtful approach proves beneficial as learners gather insights into how data structures can be leveraged for optimal performance in specific scenarios.
Time and Space Complexity
Understanding Big O Notation
Big O notation serves as a cornerstone concept for understanding performance in programming. It provides a way to evaluate how the time or space requirements of algorithms scale with input size. Key aspects include:
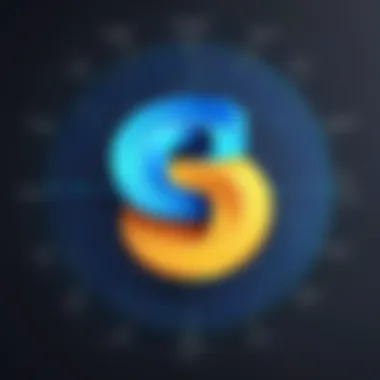
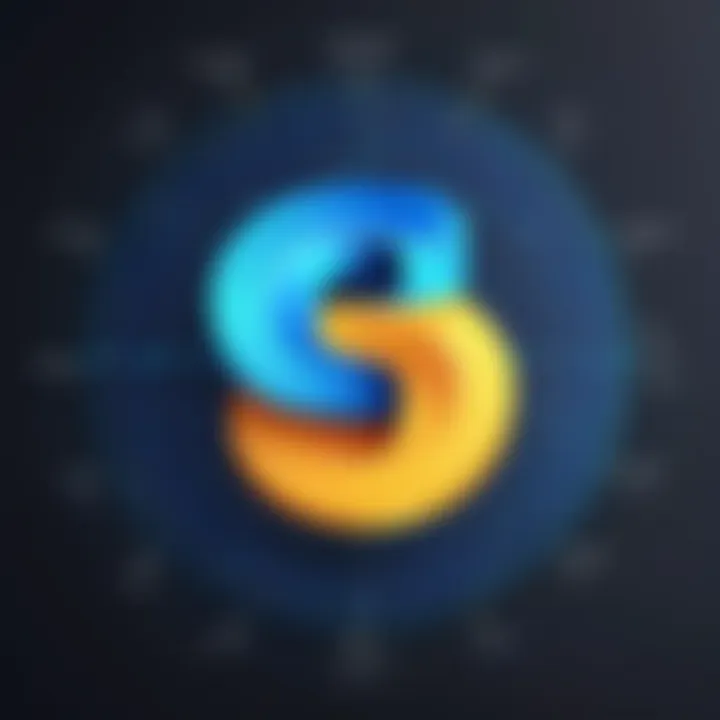
- Simplifying Complexity: Big O notation abstracts away constants and lower-order terms, allowing programmers to focus on the most significant factors as input size grows. This simplification aids in comparing algorithms.
- Communication Tool: It's a universal language among programmers, facilitating discussions about performance and efficiency without delving into implementation specifics. This trait is beneficial, especially when collaborating with team members or other developers.
Grasping Big O notation enriches this article by allowing readers to critique various algorithms and structures effectively. This understanding helps in making choices that balance resource constraints with functional needs.
Optimization Techniques
Performance optimization is vital for any serious programmer. When working with data structures in Python, a few techniques can be impactful:
- Profiling: Before optimization, identifying bottlenecks is essential. Tools in Python, such as cProfile, allow developers to see where time is being spent, guiding the optimization process.
- Algorithmic Adjustments: Sometimes, replacing an algorithm or altering how a structure is utilized can yield significant performance gains. For example, switching from a naive sorting algorithm to a more efficient one like merge sort can dramatically reduce processing time.
- Data Structure Reassessment: Periodically reevaluating the chosen data structures can lead to better performance. For instance, if frequent changes to the data collection occur, a linked list may be more appropriate than an array.
Understanding these optimization techniques contributes significantly to achieving efficient, scalable Python applications. Knowing when and how to implement these techniques can be the difference between a sluggish application and a responsive one, cementing their relevance in this exploration.
Best Practices in Implementing Data Structures
When it comes to implementing data structures in Python, adhering to best practices is crucial. These practices pave the way for writing code that is not just functional, but also maintainable and efficient. The importance of these principles can't be overstated - they can save a programmer from headaches down the line. Well-structured code not only makes it easier to identify and fix bugs but also enhances collaboration among team members.
Maintaining clear and organized code helps demystify complex constructs, making them accessible to those who might come along later, whether they are new team members or even the original author revisiting their work after some time.
Readable and Maintainable Code
A mantra in programming is that "code is more read than written." Consequently, prioritizing readability is paramount. This means choosing clear variable names and structuring code so that its logic flows intuitively. Users often find themselves riffling through lines of code if names are cryptic. Instead of naming a variable or , opt for something descriptive like or . This simple change can make a world of difference.
Consideration factors such as consistency really play a role here. Sticking to conventional naming styles (like snake_case for variables or CamelCase for classes) fosters familiarity and eases comprehension.
For maintainability, standardizing documentation within the code is non-negotiable. This could come in the form of docstrings or simple inline comments that explain the purpose of blocks of code or the logic behind certain operations. A well-documented function is like a roadmap – it guides the reader and future developers through the rationale behind the implemented logic.
Moreover, refactoring code regularly is essential. As the application matures, revisiting and tidying up code not only keeps it manageable but can also yield performance improvements. In a nutshell, prioritizing readability with a clear structure makes life easier for anyone who interacts with your code.
Testing and Debugging Strategies
Testing and debugging are central pillars in the structure and functioning of any program. Establishing a solid testing framework right from the start is vital for ensuring that the data structures perform as expected under various conditions. Utilizing testing frameworks like or in Python can streamline this process.
“An ounce of prevention is worth a pound of cure.” This saying rings particularly true in programming. Making the effort to write test cases before implementing a data structure can save a considerable amount of time in debugging later. Always start with basic unit tests that check edge cases, as these can often reveal hidden issues early in the development phase.
Additionally, employing debugging tools such as PDB (Python Debugger) can help break down the code step-by-step, providing invaluable insights about the flow of execution and the state of variables. This approach nurtures a deeper understanding of how data structures behave in real-time scenarios.
Lastly, don't overlook the importance of peer reviews. Having another pair of eyes checking your code can catch glaring mistakes and offer new perspectives. Code review is not just about finding errors; it’s about fostering a collaborative environment where knowledge sharing flourishes.
By integrating these testing and debugging strategies, you not only refine your code but also reinforce a culture of quality that can greatly improve the robustness of your project.
Practical Applications of Data Structures
Data structures are at the heart of efficient software design and implementation. Understanding their practical applications is crucial for programmers who aim to optimize performance and manage data effectively. The utility of data structures goes beyond mere storage; they influence the speed and efficiency with which data can be accessed, modified, and processed. Thus, developers can craft solutions that not only work but do so optimally.
The significance of employing appropriate data structures can be grasped through the lens of performance enhancement. For instance, a project that deals with vast amounts of data, like a social media platform, must utilize efficient structures to keep user experience smooth. Likewise, a recommendation engine, which relies heavily on real-time data analysis, leverages specific types of data structures to facilitate quick lookups and updates. Hence, grasping these applications informs developers on how to approach their software design from a data management perspective.
"Choosing the right data structure is like selecting the right tool for a job. The outcome depends not just on what you build, but how you build it."
Case Studies in Real-World Projects
The application of data structures can be observed in numerous real-world case studies that demonstrate their impact and necessity in various sectors. Consider Netflix, where the recommendation system must function seamlessly to personalize user experiences. This sophisticated implementation uses graphs to represent users and their movie choices, allowing for efficient traversal and recommendations.
Another example lies in flight reservation systems. These systems often use trees to manage routes and connections, facilitating the quick retrieval of information about flights, connections, and prices. Data structures in such systems ensure users can find what they need promptly, minimizing wait times and enhancing customer satisfaction.
Performance Evaluation in Different Scenarios
When it comes to performance evaluation, the choice of data structure can make or break an application’s efficiency. For situation where frequent data retrieval is necessary, structures like hash tables shine, providing average-case time complexity of O(1) for search operations. In contrast, if the application requires sequential data manipulation, linked lists might be more suitable despite their slower access times.
Moreover, the length of the data, data access patterns, and even the type of operations (reads vs. writes) heavily influence the choice of data structure. A deep dive into performance metrics can reveal valuable insights:
- Speed: How quickly can the data be accessed or altered?
- Memory Usage: Are there possible memory constraints? Different structures have different memory footprints.
- Scalability: Can the data structure handle increased load as user data grows?
Ultimately, empirical evaluation through testing various structures in simulated environments is often the best way to gauge performance across different scenarios. This hands-on approach allows developers to see firsthand how their choices affect overall application efficiency.
Finale and Future Directions
In wrapping up this exploration of data structures within Python, it’s crucial to reflect on the myriad characteristics each structure carries and how they contribute to efficient data management. The proper selection of a data structure directly influences the performance, readability, and maintainability of your code. An informed choice not only streamlines processing tasks but also optimizes memory usage and reduces execution time, ultimately leading to enhanced application performance.
As we look to the future, the evolving landscape of technology means that data structures will continue to adapt alongside advances in hardware and programming paradigms. This necessitates a comprehensive understanding of not just how to implement these structures in Python, but how these elements will serve in addressing real-world challenges in various fields such as data science, artificial intelligence, and web development.
A well-structured approach ensures your solutions can grow and adapt, as new requirements emerge in the ever-shifting tech terrain.
"The essence of data structures is realizing that not all data is created equal and neither are their use cases."
Recap of Key Insights
Going over the key insights presented in this article, we see that:
- Foundational Knowledge: A grasp of fundamental data structures gives programmers a leg up in efficient algorithm design.
- Python’s Versatility: The language’s inherent features simplify complex data operations, making it accessible for both beginners and seasoned developers.
- Performance Considerations: Understanding time and space complexities helps in choosing the most appropriate structures for varied applications.
Considering these takeaways will enhance your problem-solving capabilities in Python, allowing for robust implementations that are tailored to specific tasks. It’s not just about knowing how a structure works; it’s about understanding the rationale behind its use in diverse scenarios.
Emerging Trends in Data Structures
Keeping an eye on trends, several key directions are surfacing in the world of data structures:
- Increased Emphasis on Immutable Structures: As applications demand more concurrency, the use of immutable structures, like tuples and frozensets, is becoming more prevalent due to their ability to avoid state-related bugs and provide thread safety.
- Integration of Machine Learning: Data structures that support machine learning operations, such as multi-dimensional arrays (numpy arrays), are becoming essential in data science workflows. This trend showcases the growing intersection between data structures and AI technologies.
- Distributed Data Structures: With cloud computing and big data, there’s a rising interest in data structures that operate effectively in distributed systems. Structures optimized for communication across nodes are a crucial component of scalable applications.
- Hybrid Approaches: Combining various structures to leverage their individual strengths is gaining traction. Data scientists and software developers are often using pairs like hash tables to implement associative arrays, alongside trees for sorted data, promoting flexibility and performance.
As you navigate your journey into the world of Python data management, being aware of these emerging trends can give you an edge, enhancing both your understanding and future implementation strategies.