Understanding the Decorator Pattern in Software Design
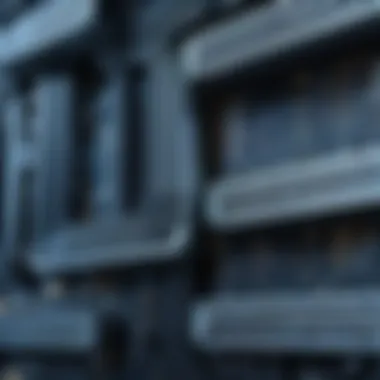
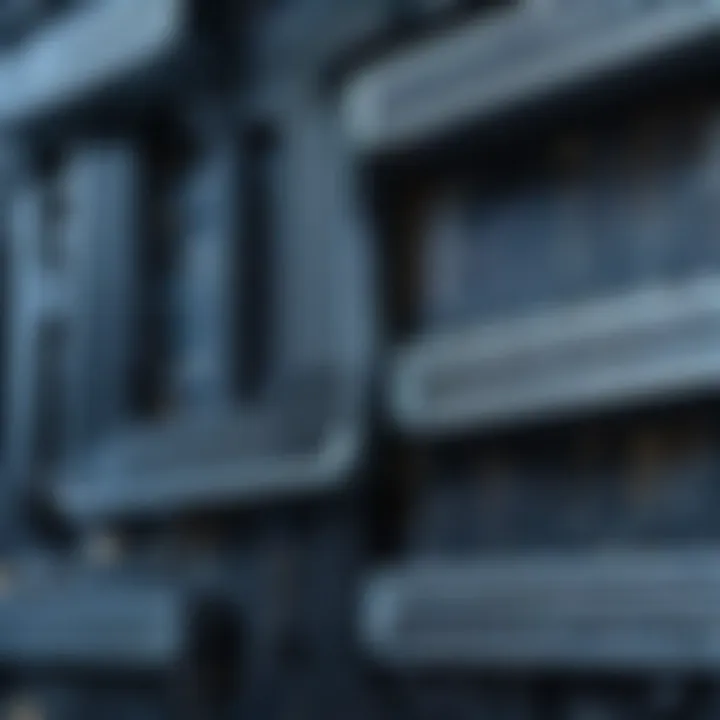
Overview of Topic
The Decorator Pattern is an essential concept in software design that enables developers to enhance the functionality of individual objects at runtime. Unlike traditional inheritance, which can lead to a proliferation of subclasses, the Decorator Pattern promotes a flexible approach to adding behavior or responsibilities to existing classes. This pattern holds significant relevance in the tech industry, especially in applications where requirements change frequently or where reusability and maintainability are critical.
Historically, the Decorator Pattern has evolved alongside object-oriented programming. It emerged as developers sought more efficient ways to extend class functionalities without overwhelming the class hierarchy. Its significance can be seen in frameworks and libraries, where capabilities often need to be added in a modular fashion.
Fundamentals Explained
At its core, the Decorator Pattern is built on several key principles. These principles include:
- Composition over Inheritance: Allows behaviors to be added without making a permanent change to the class structure.
- Single Responsibility Principle: Each decorator class should address a specific behavior, making the code base easier to manage.
Key Terminology and Definitions
Understanding the terminology is crucial for grasping the Decorator Pattern. Here are some important terms:
- Component: This is the interface or abstract class that defines the core behavior.
- Concrete Component: This refers to the class whose functionality is to be enhanced with decorators.
- Decorator: The class that contains a reference to a component and adds additional behavior.
Basic Concepts
Every decorator class should adhere to the same interface as the component it decorates. This ensures that decorators can be used interchangeably without disrupting the class's expected interactions. Moreover, because decorators can wrap one another, this leads to a powerful form of flexible composition.
Practical Applications and Examples
The Decorator Pattern finds utility in various real-world applications.
- Graphical User Interfaces (GUIs): In user interface design, decorators can be used for adding features such as borders, scrollbars, or shadows without modifying core elements. For example, libraries like Swing in Java use decorators to modify UI components dynamically.
- Input/Output Streams: The Java I/O package uses decorators extensively; classes like BufferedInputStream or DataInputStream enhance basic InputStream functionality without altering the underlying object.
Code Snippet
Consider a simple example in Python illustrating the Decorator Pattern:
This example demonstrates how a MilkDecorator extends the functionality of a basic Coffee object without modifying its original behavior.
Advanced Topics and Latest Trends
In recent years, the emphasis on microservices architecture has increased the relevance of the Decorator Pattern. As systems are broken down into smaller services, the ability to extend functionalities on the fly becomes essential. New frameworks are emerging that further embrace this pattern, allowing for easier scalability and improved maintainability.
Future Prospects
The shift towards functional and reactive programming may also influence how decorators are utilized. The separation of behavior from the objects may become more pronounced, leading to innovative applications of the pattern across different programming paradigms.
Tips and Resources for Further Learning
To deepen your understanding of the Decorator Pattern, consider exploring the following resources:
- Books like "Design Patterns: Elements of Reusable Object-Oriented Software" by Erich Gamma et al.
- Online courses on platforms such as Coursera or Udemy, which cover object-oriented design principles.
- Technical forums like Reddit's r/programming or Stack Overflow for community discussions.
Additionally, utilizing software design tools like Lucidchart or Draw.io can help visualize the structure and relationships inherent in the Decorator Pattern.
"Understanding design patterns is not just about applying them. It's about knowing when and why they fit into the solution."
Preamble to Design Patterns
In the realm of software development, design patterns serve as proven solutions to common problems encountered during the design phases of software projects. Understanding these patterns is crucial for developing robust and maintainable applications. This section of the article establishes a foundational knowledge of design patterns, setting the stage for deeper insights into the Decorator Pattern specifically.
Design patterns can streamline systems design and enhance communication among developers. They provide an established vocabulary and framework, thereby reducing the complexity in code management. By recognizing and utilizing design patterns, developers can significantly improve their efficiency when creating software solutions.
Definition of Design Patterns
Design patterns are standardized solutions crafted to solve recurring design issues in software development. They encapsulate best practices that have been validated through experience, offering a template for dealing with specific design dilemmas. Each pattern addresses a particular problem that arises in a software context, laying out a strategy that developers can adopt to achieve effective solutions.
Importance in Software Development
The importance of design patterns in software development cannot be overstated. They facilitate better code organization, promote code reuse, and enhance the understandability of systems. By allowing developers to leverage established patterns, the time spent on problem-solving can be drastically reduced. This leads to increased productivity and more maintainable systems, as developers will have a clearer pathway to follow when implementing new features or addressing existing issues.
Categories of Design Patterns
Design patterns are typically classified into three main categories: creational, structural, and behavioral patterns. Each category showcases distinct aspects of software design, providing targeted solutions relevant to the given focus.
- Creational Patterns
Creational patterns focus on the process of object creation. They aim to use a controlled set of classes and objects in order to increase flexibility and reuse in the code. A key characteristic of these patterns is their ability to abstract the instantiation process, allowing for easier changes in object allocation. Their use helps in minimizing the risk of object creation complexities in large systems, making them beneficial choices in many scenarios. - Structural Patterns
Structural patterns concentrate on how classes and objects are composed to form larger structures. They simplify the organization of complex systems by defining clear relationships between different entities. A major advantage of structural patterns is that they help in creating a balanced system, ensuring that components are well integrated. By employing these patterns, developers can achieve better maintainability of their code, which is particularly useful as software expands. - Behavioral Patterns
Behavioral patterns examine how objects interact and communicate with each other. They are concerned with the flow of control and responsibility among objects. A distinctive feature of this category is its focus on communication patterns instead of class structure. Utilizing behavioral patterns leads to more effective teamwork between objects and enhances the flexibility of the system to accommodate new behaviors without requiring extensive changes.
Design patterns, especially in their various categories, play a vital role in structuring software development processes. As we move forward in the article, the focus will shift towards the Decorator Pattern, a structural design pattern that exemplifies many of the principles outlined here.
Defining the Decorator Pattern
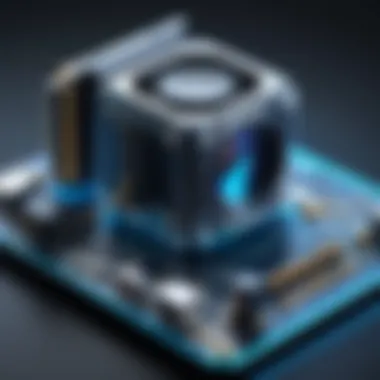
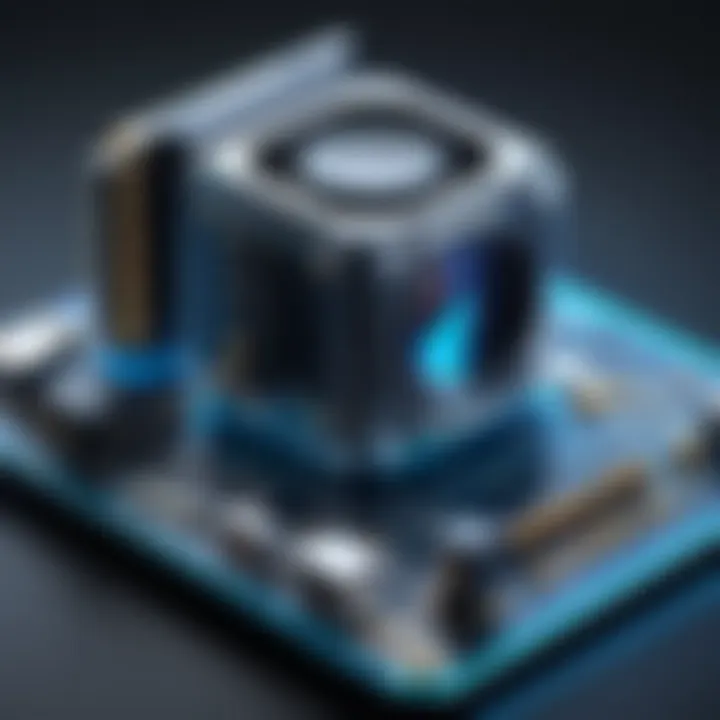
The Decorator Pattern is a significant structural design pattern in software development. Understanding this pattern is essential, as it provides a flexible alternative for enhancing the functionality of objects at runtime without altering their structure. This article will delve into the intricacies of defining the Decorator Pattern by exploring its overview and core principles, which include the Single Responsibility Principle and the Open/Closed Principle. By grasping these elements, developers can effectively implement this pattern in their projects, thus ensuring cleaner, more manageable, and scalable code.
Overview of the Decorator Pattern
The Decorator Pattern allows developers to add new functionalities to existing objects. Instead of modifying the existing code, decorators can be wrapped around an object, adding behavior as needed. This dynamic approach enables the enhancement of an object's capabilities without creating subclasses for every possible variation of behavior.
For example, in a graphic user interface framework, a basic window object can be decorated with borders, scrolling features, or even additional functionalities like transparency. As a result, decorators can modify the appearance or behavior of an object flexibly and reuse components without affecting their original structure. This method can help reduce class explosion by not requiring numerous subclasses to accomplish different behaviors.
Core Principles
Single Responsibility Principle
The Single Responsibility Principle (SRP) dictates that a class should have one and only one reason to change. In the context of the Decorator Pattern, this principle emphasizes the importance of keeping classes focused on a single functionality. By adhering to SRP, each decorator class serves a specific purpose, ensuring that modifications to one aspect of behavior do not inadvertently impact others.
This ensures cleaner code management, making debugging and maintenance easier. Classes adhering to the SRP can evolve independently, which is a crucial aspect of software agility. For instance, if a logging feature needs updating, only that decorator needs modification; the core functionality remains intact.
Open/Closed Principle
The Open/Closed Principle (OCP) states that software entities should be open for extension but closed for modification. Implementing the Decorator Pattern aligns well with OCP because it allows developers to extend object functionality without altering the existing codebase. This principle enhances system flexibility and encourages an architecture in which implementing new features is emphasized.
In practice, when designing new decorators, developers can introduce new features by simply creating additional decorators without modifying the existing components. This preserves the integrity of the initial object and ensures that software remains reliable while still evolving. However, while OCP advocates for extensibility, it can inadvertently lead to a more complex code structure, making the system harder to understand if not carefully managed.
The Decorator Pattern exemplifies both the Single Responsibility Principle and the Open/Closed Principle, allowing developers to create maintainable and scalable software systems.
By adhering to these principles, developers can harness the full potential of the Decorator Pattern in their applications, making it easier to adapt and extend the software without sacrificing code integrity.
Structural Components of the Decorator Pattern
The structural components of the Decorator Pattern play a significant role in its implementation and effectiveness. In software design, components and decorators are pivotal in enabling customization and enhancing functionality without modifying the core class of an object. By understanding these components, developers can leverage designs that provide flexibility and adhere to fundamental design principles.
Components and Interfaces
In the Decorator Pattern, components refer to the base objects that encapsulate core functionality. These components must implement a common interface. This ensures that decorators can be applied seamlessly. The interface defines the operations that can be performed on the component and its decorators. A well-defined interface promotes code that is modular and allows for easier testing and maintenance.
Moreover, by using interfaces, one can achieve a high degree of polymorphism. This means that different concrete components can be treated uniformly by the client code. That is, any component type can be passed around and manipulated as if it were any other type, greatly simplifying the programming model. Understanding how to effectively implement interfaces is crucial for building scalable and maintainable systems.
Concrete Components
Concrete components are the actual implementations of the interface defined earlier. They serve as the primary objects to which the decorators will be applied. By default, these components offer specific functionality. For example, a simple object could provide basic text rendering capabilities. When the Decorator Pattern is applied, additional features can be dynamically added to this text object.
The key consideration with concrete components is their responsibility. They should focus solely on providing their core functionalities, adhering to the Single Responsibility Principle. This makes it easier to manage and test each piece independently. This approach to structure allows changes to be made quickly without affecting other parts of the application.
Decorators
Decorators are special types of components that extend the functionality of the concrete components. They provide a means to add responsibilities to objects in a flexible and dynamic manner. By implementing the same interface as the concrete components, decorators can wrap around them and enhance or override behaviors.
For instance, consider a r that adds HTML styling features to a component. The decorator’s ability to modify output or behavior without altering the base object is what sets the Decorator Pattern apart from other design patterns. This characteristic gives rise to the Open/Closed Principle, which states that software entities should be open for extension but closed for modification. This principle ensures that the codebase remains stable while evolving to meet new requirements.
Overall, the structural components of the Decorator Pattern allow for modular design. This is beneficial in real-world applications, where requirements often change. By utilizing components, concrete implementations, and decorators, systems can adapt to new needs without significant refactoring.
The Decorator Pattern serves as a profound tool for enhancing the capabilities of software objects. It maintains clarity and modularity, allowing developers to implement changes with minimal disruption.
How the Decorator Pattern Works
Understanding how the Decorator Pattern operates is crucial for software designers and developers. This pattern allows for flexible and dynamic behavior changes in objects without altering their core functionality. It enables the addition of responsibilities to individual objects, thereby enhancing their capabilities. The significance of this lies in its ability to facilitate code reuse and maintainability, reducing the need for class proliferation.
Dynamic Behavior Composition
Dynamic behavior composition is fundamentally the core of how the Decorator Pattern achieves its goal. Instead of relying on inheritance to implement new functionalities, decorators dynamically add responsibilities to objects. This approach provides an elegant solution to extend the behavior of objects in a way that is more modular and clean. Given the nature of the composition, one can create a variety of decorators that each add specific features. This modular design leads to more manageable codebases, where developers can mix and match functionalities as per the requirements at runtime.
For instance, consider an application with a text editor. If you need to add a spell checker, you can wrap the existing text editor object in a spell checker decorator. If later you need a grammar checker, you can wrap the resulting object again. This way, each feature can be added independently without modifying the existing code structure, promoting Single Responsibility and Open/Closed principles as previously discussed.
Example Implementation
Basic Example in Python
A basic implementation of the Decorator Pattern in Python serves as an excellent illustration of the concepts discussed. A simple example could involve defining a base class, say , and then adding multiple decorators to enhance its capabilities. The decorators can include features like milk or sugar. Here is how it looks in Python:
This implementation shows how each decorator can augment the base coffee object without altering its definition. The modularity of decorators means they can be added or removed easily, which is an essential advantage in a project aiming for scalability.
Using Decorators in Java
In Java, the process is somewhat analogous but with its syntax and structure. The Java implementation usually involves interfaces, where both concrete components and decorators implement the same interface. This consistency allows flexibility in mixing various decorators.
Consider a similar example with a interface. The implementation highlights how decorators can wrap around concrete components:
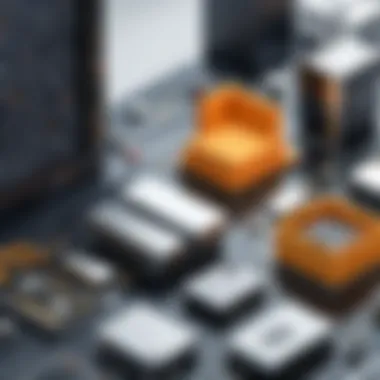
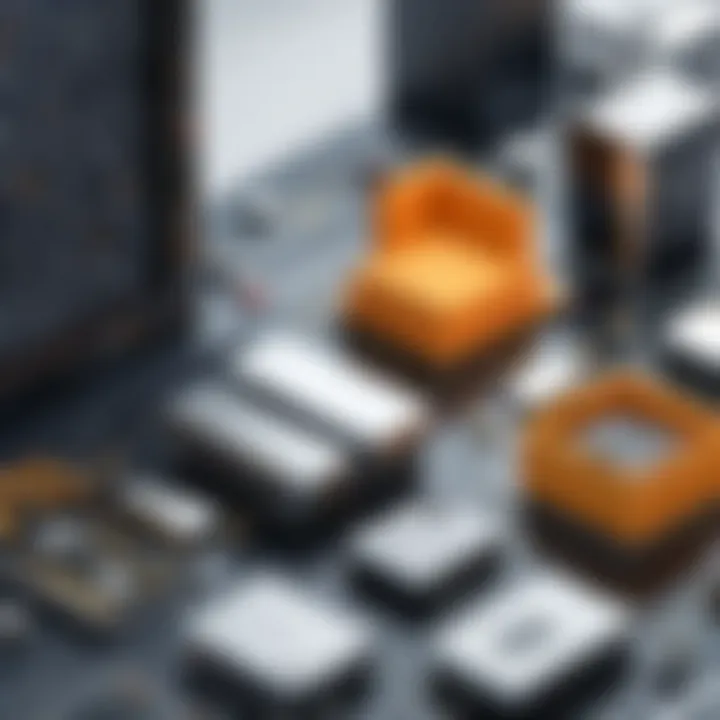
The Java example demonstrates how decorators can build upon each other while adhering to the same interface, maintaining code clarity and flexibility. Both of these examples, be it Python or Java, emphasize the benefits of using the Decorator Pattern for enhancing functionality without the complexity that arises from a rigid class hierarchy.
The Decorator Pattern significantly contributes to more maintainable code and enhances the application of various software design principles. As such, it is a powerful technique in any software developer's toolkit.
Advantages of Using the Decorator Pattern
The Decorator Pattern offers notable benefits that make it a valuable tool in software design. Understanding these advantages is essential as developers seek to create more maintainable and adaptable code. Two primary advantages include enhancing flexibility and reusability of components, and avoiding class explosion.
Enhancing Flexibility and Reusability
One of the critical strengths of the Decorator Pattern is its ability to enhance flexibility. This pattern allows developers to extend an object's behavior without modifying its structure. By wrapping objects dynamically with decorators, programmers can tailor behaviors to meet specific needs or conditions.
This flexibility is particularly beneficial in large applications. For instance, in web applications, developers can apply multiple decorators to a single web component to modify its appearance or functionality based on user roles or preferences. Such dynamic behavior customization leads to greater reusability of code. Developers can create reusable components that can be combined in various ways without having to rely on extensive inheritance hierarchies. This modular approach not only simplifies the development process but also improves the code's adaptability to future changes or requirements.
Benefits of flexibility and reusability include:
- Easier code maintainability
- Reduced redundancy in code
- Higher adaptability to changing requirements
The Decorator Pattern thus facilitates a more efficient way of managing complex systems by allowing different functionalities to coexist without creating a multiplicity of different classes or interfaces.
Avoiding Class Explosion
Another vital advantage is the reduction of what is often referred to as class explosion. In large software projects, the need to manage numerous classes can quickly spiral out of control. Each time a new feature is required, it often results in new classes being created, increasing complexity and maintenance efforts.
The Decorator Pattern allows for adding new behaviors without creating additional classes. Instead of generating a new subclass for every possible combination of behaviors or attributes, decorators can be layered on top of base components. This significantly lessens the number of classes needed in the project.
For example, if you have a basic text formatting component, you might need several variations: bold, italic, underline, etc. Using decorators, you can apply these variations fluidly without needing to define a separate class for each combination of formats.
In summary, avoiding class explosion leads to:
- Simplified code maintenance
- Improved comprehension of code structure
- Easier collaboration among development teams
"Using the Decorator Pattern helps to keep the codebase lean and comprehensible while providing the desired functionality.”
Real-World Applications
In this section, we will explore the practical scenarios where the Decorator Pattern proves to be valuable in real-world software development. Understanding these applications helps programmers recognize the pattern's utility and relevance, providing concrete examples that illustrate its effectiveness in complex systems.
User Interface Frameworks
User interface frameworks often benefit greatly from the Decorator Pattern. This is primarily due to the need for flexibility and dynamic enhancement of UI components. For instance, in Java's Swing framework, components like buttons and panels frequently require additional features such as borders or scroll bars. Instead of creating numerous subclasses to achieve various combinations of features, developers can simply use decorators to add functionality at runtime.
This approach has several benefits:
- Decoupling of Features: Different functionalities can be added or removed independently without touching the underlying component classes.
- Code Reusability: Developers can reuse decorators across different components, which reduces the amount of code that needs to be written and maintained.
- Enhanced Readability: The structured approach of decorators helps in keeping the codebase clean and readable, even as complexity increases.
For example, a text field component can be enhanced with decorators for input validation or formatting without being re-implemented. Each new feature can be added as a decorator, aligning with the open/closed principle of software design—the classes can be extended without modification.
Stream Processing
Another significant application of the Decorator Pattern is in stream processing. Systems that require a series of transformations or filters on data streams can leverage this pattern to add capabilities dynamically. In languages such as Java, streams allow for operations like filtering, mapping, and reducing.
By using decorators, developers can wrap existing stream operations with additional behaviors such as logging or error handling. This not only enhances the system's functionality but also maintains clean separation between data processing logic and additional functionalities.
Some points to consider in stream processing include:
- Flexibility: Stream operations can be modified or extended easily, which is vital in environments where data processing requirements can change often.
- Scalability: As more decorators are added to the stream, they can operate on the data in a pipeline fashion, improving performance and scalability.
- Maintainability: By isolating additional features into decorators, the code remains easier to maintain and understand over time.
Comparison with Other Patterns
Understanding the Decorator Pattern necessitates a comparison with other design patterns. This comparison elucidates the distinct characteristics and functionalities of the Decorator, setting it apart from similar patterns. It allows developers to select the most appropriate approach for their needs, enhancing software architecture and design efficiency.
A critical analysis reveals the nuances in behavior and structure among design patterns. This examination enhances conceptual clarity, allowing both novice and experienced developers to appreciate the strengths and limitations of each pattern. Furthermore, highlighting distinctions fosters informed decision-making when faced with design trade-offs in software development.
Decorator vs. Adapter Pattern
The Decorator and Adapter patterns both establish relationships between classes, yet they serve different purposes.
- Purpose: The Adapter Pattern focuses on converting one interface into another. It acts as a bridge between two incompatible interfaces, allowing them to work together without modifying their respective codes. This is particularly useful when integrating with third-party libraries or legacy systems.
- Functionality: The Decorator Pattern, however, is about enhancing or modifying existing objects without altering their structure. This allows for dynamic addition of behavior, promoting flexibility in system design.
- Example Scenario: Consider a graphic editing application. If an existing shape object needs to be converted to a different type for compatibility, the Adapter Pattern would be used. Conversely, if you want to add borders or shadows to an existing shape during design, the Decorator Pattern is the solution.
Decorator vs. Composite Pattern
Both Decorator and Composite patterns rely on object composition, yet they are utilized for different objectives.
- Structure: The Composite Pattern is primarily intended for creating tree structures. It allows clients to treat single objects and compositions of objects uniformly. In essence, it enables a hierarchy of objects, where each object can be a composite of other objects.
- Functionality: On the other hand, the Decorator Pattern is focused on augmenting existing objects with additional behaviors without altering their class structure. This means that while a Decorator can act on an individual object, a Composite necessarily operates over groups of objects.
- Use Cases: Think of a UI framework. In a Composite Pattern, a panel may contain multiple buttons and text fields, all being treated as a single unit. Meanwhile, in a Decorator scenario, you would wrap buttons to add enhanced behavior like tooltip or event listeners, modifying their functionalities on the fly.
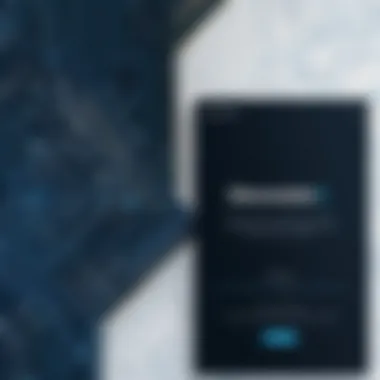
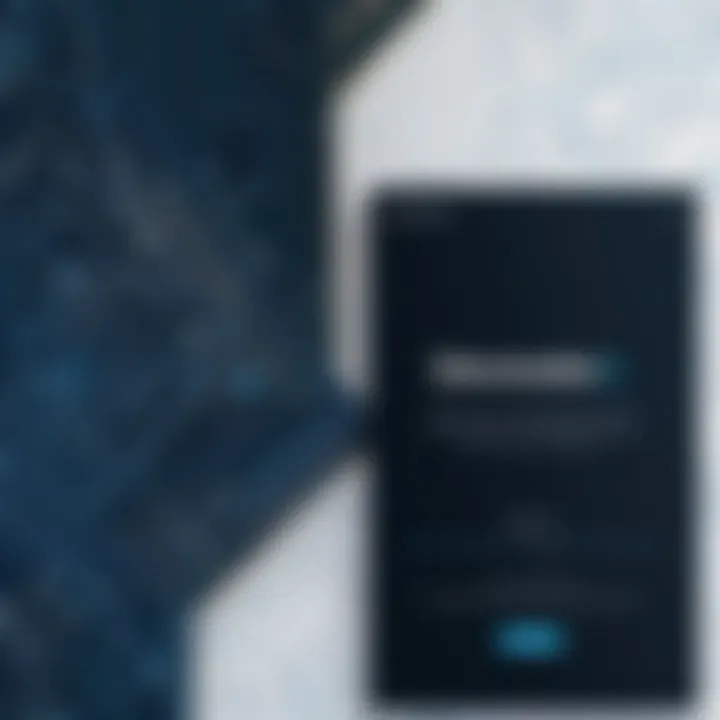
Conclusion: Understanding the distinctions between these patterns helps developers design better systems. Clear differentiation aids in choosing the right pattern that addresses specific challenges effectively.
Best Practices for Implementing the Decorator Pattern
Implementing the Decorator Pattern effectively requires a structured approach. Engaging with best practices in this context not only aids in achieving desired functionality but also smoothly integrates with existing code. By applying these practices, developers can maintain cleaner code, increase flexibility, and ensure long-term maintainability.
Code Organization
Proper code organization is vital when using the Decorator Pattern. When decorators are not organized well, they can lead to confusion and reduce readability. To mitigate this, developers should:
- Group related decorators: Place decorators that modify similar components within the same package or module. This creates a clear hierarchy and makes finding relevant decorators easier.
- Naming conventions: Use descriptive names for decorator classes. Names that reflect their function simplify understanding the code and its intent.
- Avoid deep nesting: Limit the depth of decorator nesting, as it can lead to complex hierarchies that are hard to comprehend at a glance. Documentation and comments can help elucidate the purpose of each layer, but fewer layers are generally more effective.
Being consistent in code organization improves collaboration and reduces onboarding time for new team members. Well-structured decorators enable teams to adapt to changes effortlessly without fearing unintended side effects.
Testing Strategies
Testing when using the Decorator Pattern presents unique challenges. The flexibility of decorators often means they can be combined in various ways, leading to challenges in ensuring each configuration functions as intended. Implementing robust testing strategies can enhance reliability. Follow these strategies:
- Unit testing each decorator: Each decorator should be unit tested individually. This practice ensures that the functionality it provides can be verified without worrying about other components.
- Integration testing: After unit tests, conduct integration tests. These tests confirm that decorators function correctly when combined, especially in more complex implementations.
- Using test doubles: In certain cases, it may be helpful to use stubs or mocks to isolate the component under test from the rest of the system. This practice ensures tests are focused and faster.
Adopting a comprehensive testing approach provides confidence in the stability of code employing the Decorator Pattern. This awareness of the potential pitfalls helps developers anticipate and resolve issues before they escalate.
Challenges and Limitations
The Decorator Pattern provides significant benefits in terms of flexibility and reusability. However, it is crucial to recognize the challenges and limitations that accompany its implementation. Understanding these nuances is essential for developers and designers who wish to leverage this pattern effectively. Here, we will explore performance overheads and maintenance complexity, which are two predominant issues related to the Decorator Pattern.
Performance Overheads
One of the key challenges in using the Decorator Pattern is the potential performance overhead it introduces. Each decorator adds a layer around the original component, which can lead to increased computational cost. When multiple decorators are applied, this layering can result in a significant number of method calls. This is particularly evident in scenarios where performance is critical, such as real-time systems or applications with high-frequency data processing.
For example, if a base object is wrapped by three decorators, the method call flow becomes more complex. Each decorator handles calling the next component, which may slow down execution time due to the additional layer of abstraction. Moreover, this pattern could affect memory usage, as each decorator instance requires additional memory.
Complexity in Maintenance
Another notable limitation of the Decorator Pattern is its complexity in maintenance. As the number of decorators increases, the system can become harder to understand and manage. Developers need to keep track of which decorators are applied and how they interact with one another. This interdependency can lead to unexpected behaviors, especially if the decorators are not clearly documented.
The increased complexity can also make testing more challenging. Each decorator can introduce unique behavior that must be tested in combination with others. Moreover, changes in one decorator might necessitate revisions in others, thereby increasing the risk of introducing bugs.
Maintaining a clear overview of all decorators is essential for long-term sustainability of a codebase.
In summary, while the Decorator Pattern offers valuable capabilities for extending functionality, it is essential to weigh these advantages against potential performance issues and complexity. Developers must adopt careful design strategies to mitigate these challenges while harnessing the strengths of this design pattern.
Insights into performance overheads and maintenance complexities will guide informed decisions during implementation, ensuring the effective use of the Decorator Pattern.
Future Perspectives on the Decorator Pattern
The Decorator Pattern stands at a significant junction in software design, especially as applications grow in complexity. Its flexibility in enhancing functionality without altering the core structure of objects makes it relevant in modern programming paradigms. As we look ahead, understanding how the Decorator Pattern can evolve with technology becomes vital.
Implications for Software Architecture
The integration of the Decorator Pattern into software architecture can lead to improved scalability. This pattern allows developers to add features to objects dynamically, fostering a modular approach to design. With the rising focus on microservices and distributed systems, the ability to adapt and modify components on the fly is invaluable.
Moreover, as software systems become more multifaceted, the need to maintain clean architecture gains precedence. The Decorator Pattern aids this by promoting adherence to the Single Responsibility Principle. Each enhancement can be encapsulated in a separate decorator, thus leading to more manageable and maintainable codebases.
In the evolving landscape of cloud computing and serverless architectures, the ability to layer functionalities through decorators makes it easier to deploy and scale applications. Developers can modify behavior without significant refactoring, which is essential for adopting agile methodologies.
"Modular approaches to software design, like the Decorator Pattern, allow for greater adaptability in an environment where change is constant."
Integration with Emerging Technologies
As new technologies emerge, the Decorator Pattern can have substantial implications for their implementation. For instance, in artificial intelligence and machine learning, models often require various enhancements for different tasks. Decorators can wrap models, allowing the addition of pre-processing, feature extraction, or post-processing steps seamlessly.
The rise of the Internet of Things (IoT) also benefits from the Decorator Pattern. Smart devices often need to interact with multiple functionalities. Using decorators can enable or disable certain features dynamically based on user preferences or environmental conditions without changing the device's fundamental capabilities.
Similarly, the rapid development of web frameworks, such as Flask for Python or Spring Boot for Java, continues to highlight the relevance of this pattern. Developers can easily extend web server capabilities by adding decorators for request handling, middleware functionalities, and response modifications.
As we assess the technological terrain ahead, embracing the Decorator Pattern will remain essential for ensuring software remains adaptable, maintainable, and aligned with user needs.
The End
In the context of this article, the conclusion serves as a critical component that synthesizes the extensive discussions surrounding the Decorator Pattern. This pattern is not only essential for enhancing object functionality dynamically, but it also highlights the importance of maintaining clean and flexible code. By revisiting the key insights presented, we can emphasize both the theoretical and practical dimensions of the Decorator Pattern.
Recap of Key Points
The analysis provided underscores several important elements:
- Definition and Purpose: The Decorator Pattern is introduced as a structural design pattern aimed at allowing the addition of new behavior to an object without affecting other objects from the same class.
- Core Principles: The adherence to the Single Responsibility and Open/Closed Principles showcases how this pattern achieves modularity and extensibility, ensuring that classes remain focused on their designated roles while being open to enhancements.
- Structural Components: Understanding the roles of components and decorators is crucial. Each component fulfills a specific function while decorators wrap these components to provide additional features. This promotes clean architecture and enhances readability.
- Practical Applications: Real-world use cases, such as user interface frameworks and stream processing systems, illustrate how the Decorator Pattern finds relevance in modern software. Understanding these applications is essential for developers seeking to apply design patterns in their work.
- Comparison with Other Patterns: The distinctions among the Decorator, Adapter, and Composite Patterns not only clarify their individual purposes but also empower developers to choose the correct pattern for their specific needs.
- Best Practices and Limitations: Discussing the best practices for organization and testing furthers the understanding of effective implementations, while recognizing challenges like performance overheads provides a holistic view.
As we navigate towards future perspectives, the importance of the Decorator Pattern remains clear. Its integration with emerging technologies further solidifies its relevance. Therefore, mastering this pattern equips software engineers with the tools necessary for producing adaptable and maintainable code in an ever-evolving technological landscape.
"The Decorator Pattern exemplifies how to enrich object behavior without altering their class definitions, thus adhering to the principles of object-oriented design."
Through this comprehensive exploration, it is evident that understanding the Decorator Pattern equips developers and tech enthusiasts with vital insights into software design, fostering a culture of thoughtful and innovative programming.