Detecting Mobile Devices in React Applications
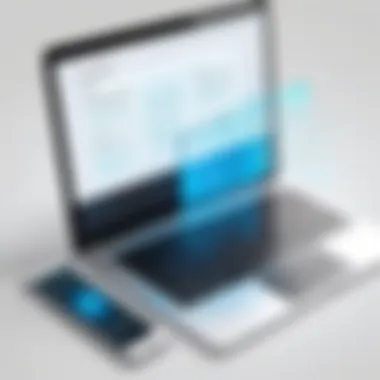
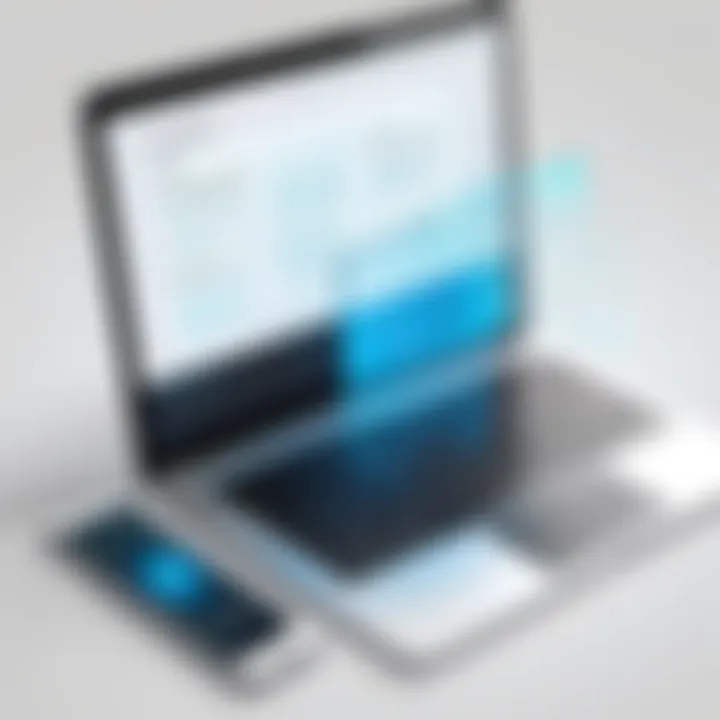
Overview of Topic
In an era where mobile devices dominate internet access, understanding mobile detection within web applications is crucial. When developing applications in React, a library known for its flexibility and performance, properly identifying whether a user is accessing via a mobile device helps create tailored user experiences. The concept of mobile detection encompasses various methods, including code libraries, user agent strings, and CSS media queries.
The significance of this topic in the tech industry cannot be overstated. With mobile traffic projected to account for over 50% of global website traffic, developers must adapt their applications for diverse screen sizes and user interactions. Blending functional design with technical precision becomes vital.
Historically, mobile detection began with simple user agent checks. Over time, with the evolution of devices and frameworks, the approaches have grown more sophisticated, leveraging advanced libraries and frameworks that automate the process.
Fundamentals Explained
To successfully implement mobile detection in React applications, it is essential to understand some core principles and terminologies.
Key Terminology and Definitions
- User-Agent String: A text string sent by the browser to identify the device. It contains information about the operating system, browser version, and device type.
- Media Queries: CSS techniques used to apply styles based on the screen size, resolution, or other properties.
- Responsive Design: An approach that allows web applications to adapt to different screen sizes and orientations seamlessly.
Basic Concepts
Understanding these terms lays the groundwork for more complex implementations. Mobile detection can take several forms, including but not limited to:
- Detecting the device type (smartphone, tablet, or desktop).
- Adapting the user interface based on the detected device.
Practical Applications and Examples
In real-world situations, how does mobile detection manifest? Below are implementations and strategies.
Real-World Case Studies
- E-commerce Platforms: Mobile detection ensures that product images resize appropriately on smaller devices, enhancing user experience and potentially boosting sales.
- Content Management Systems: Platforms like WordPress utilize mobile detection to serve optimized themes that perform better on mobile devices.
Demonstrations and Code Snippets
A simple implementation using the React library can be achieved through the following code. This example illustrates how to utilize the user-agent string:
This code checks if the user is on a mobile device and adjusts the greeting accordingly.
Advanced Topics and Latest Trends
As technology advances, so do mobile detection methodologies. Current trends in mobile detection emphasize the following:
- Device Feature Detection: Modern libraries like Modernizr allow detection based on feature availability rather than device type.
- Progressive Web Apps: These applications adapt seamlessly to any device, enhancing the mobile user experience considerably.
Future Prospects
The future of mobile detection might include more AI-driven approaches that analyze user behaviors, leading to personalized experiences based on interaction patterns.
Tips and Resources for Further Learning
To further develop skills in detecting mobile devices in React applications, consider the following resources:
- Books: "Learning React" by Alex Banks and Eve Porcello.
- Online Courses: Platforms like Udacity and Coursera offer courses on front-end development.
- Tools: Utilize libraries such as React Device Detect or boost your CSS skills with tools like Bootstrap, which incorporates responsive design principles.
By immersing oneself in these resources, developers can deepen their understanding and enhance their skills in creating robust, responsive applications for the mobile-centric world.
Understanding Mobile Detection
Mobile detection plays a crucial role in the evolving landscape of web development. In an era where mobile devices dominate internet access, understanding how to detect these devices accurately is imperative for developers. When a user accesses a website, the device they are using influences how content is displayed and how the user interacts with the site.
The importance of mobile detection lies in creating a user-friendly experience tailored to various devices. By recognizing whether a visitor is on a smartphone, tablet, or other device, developers can adjust layout, functionality, and even the content presented. This tailored approach not only enhances user satisfaction but also improves engagement metrics, such as time spent on site and overall interaction rates.
Using mobile detection allows developers to optimize performance, ensure accessibility, and maintain consistent branding across platforms. In particular, mobile device detection can help in situations where resource allocation needs to be prioritized, delivering optimized images or variations of functionalities that are appropriate for specific devices.
Importance of Mobile Detection
The importance of mobile detection can be viewed from various angles:
- User Experience: Users expect seamless navigation. Proper mobile detection facilitates a tailored experience based on their device, reducing frustration and enhancing usability.
- Performance Optimization: Different devices have varying capabilities. By detecting the mobile nature of a device, developers can load lighter content, which optimizes performance across slower connections.
- Development Efficiency: Understanding known device types lets developers employ responsive design principles effectively, which can reduce the time spent debugging layout issues across devices.
An effective mobile detection strategy aligns with the end goal of improving overall satisfaction and encouraging return visits.
Common Use Cases
The applications of mobile detection are diverse and extend across many scenarios. Here are some common use cases for mobile detection in web development:
- Responsive Design: Adjusting CSS and layout configurations depending on whether the user is on a mobile device. This can include modifying grid layouts, font sizes, or margin adjustments.
- Enhanced Functionality: Delivering unique features tailored for mobile use, such as larger buttons and touch-friendly interfaces, which enhance user interaction.
- Content Customization: Serving different types of content based on device capability. For example, providing shorter articles on mobile to improve readability, or compressing images for faster load times.
- Geo-Location Services: Mobile devices often have GPS functionalities, which can be leveraged for location-based features, enhancing user engagement through localized content.
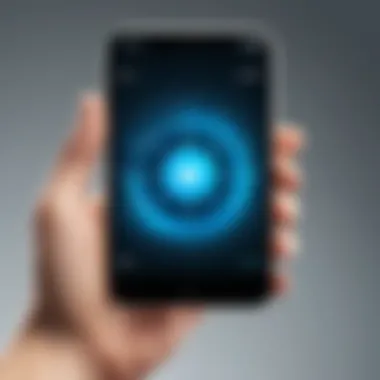
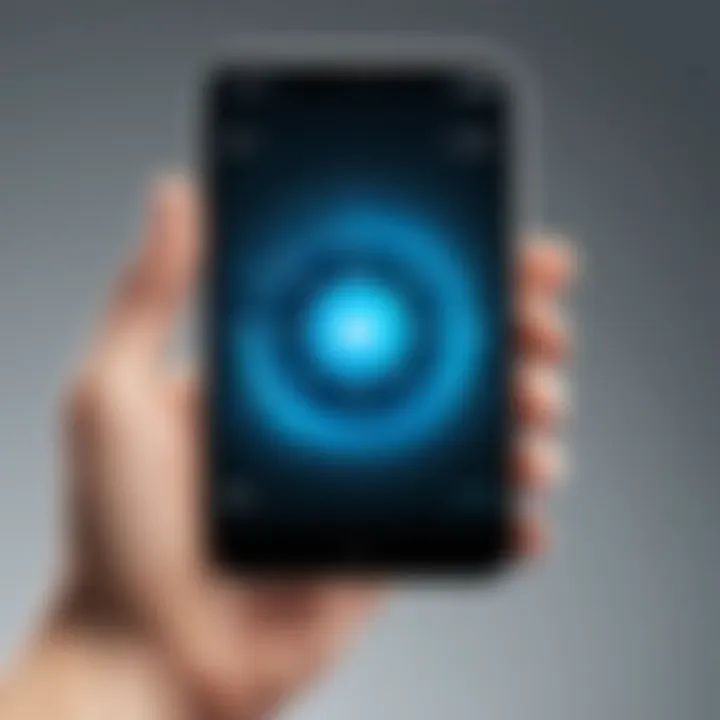
"Properly implemented mobile detection ensures that all users, regardless of their device, have a satisfying experience while engaging with your content."
In summary, understanding mobile detection is essential in today's digital environment, driving both user satisfaction and technical efficiency. Through effective implementation of mobile detection strategies, developers pave the way for a more adaptable and user-friendly web.
React and Device Responsiveness
Entering the realm of mobile development necessitates an understanding of how to effectively make applications responsive. In the context of React, device responsiveness ensures that applications not only function properly but also provide positive user experiences across a multitude of devices. This aspect is crucial since users interact with applications on various screens, from phones to tablets to desktops. The variability in dimensions, orientations, and performance capabilities of these devices means a one-size-fits-all approach falls short.
Responsive design is about adapting to the characteristics of the device accessing the application. By implementing responsive design principles within React, developers can craft applications that provide optimized layouts and functionality tailored to the user's environment. This adaptability can lead to significant benefits such as improved user engagement and lower bounce rates. Furthermore, a responsive approach enhances the perception of quality and professionalism in web applications, leading to greater user trust.
Mobile-First Design Principles
Mobile-first design principles prioritize the mobile user experience from the outset of the development process. Rather than treating the mobile version of a web application as an afterthought, developers plan and design for smaller screens first. This strategy leads to a leaner, more efficient application that is then progressively enhanced for larger screens.
- Benefits of Mobile-First Design:
- Faster initial loading: Since mobile devices may have slower connections, a mobile-first approach reduces the amount of content to be loaded initially.
- Simplicity and clarity: Designing for a smaller screen requires stripping away unnecessary elements, which can enhance the overall user experience.
- Future-proofing: With mobile usage steadily increasing, a mobile-first approach aligns with the direction of web development.
While implementing mobile-first design, it is crucial to consider touch interactions that differ significantly from mouse events. Emphasis should be placed on usability and accessibility, ensuring the website is navigable by all users.
CSS Media Queries
CSS media queries are a key component for achieving responsive designs. They allow developers to apply different styles based on device characteristics such as screen width, height, and resolution. Media queries enhance the overall adaptability of an application by ensuring optimal presentation.
In this example, a light blue background is applied to the body when the screen size is 600 pixels or smaller. This technique can be used to create fluid layouts that change dynamically, ensuring an ideal user experience regardless of the device.
A well-structured set of media queries can address common layout challenges through breakpoints, providing clear guidelines for how an application should be displayed on various screen sizes.
"Responsive design is not just about making things fit; it’s about creating a seamless experience across devices."
The combination of mobile-first design principles and CSS media queries forms a robust framework for building React applications that engage users and stand out in a competitive landscape. By considering device responsiveness at every stage of development, developers can deliver high-quality user experiences that resonate with their audience in measurable ways.
Detecting Mobile Devices Using Libraries
In the realm of modern web development, especially within the React ecosystem, efficiently detecting mobile devices is crucial. Libraries dedicated specifically for this task elevate the process, allowing developers to implement responsive designs without cumbersome manual detection logic. Using such libraries enables swift integration and functionally rich features, ultimately enhancing user experience.
Prologue to React Device Detect
React Device Detect is one of the prominent libraries designed for this purpose. This library simplifies the process of identifying the type of device accessing your React application. By leveraging the power of user-agent detection, it provides a straightforward API that allows developers to discern between mobile, tablet, and desktop users seamlessly. Utilizing React Device Detect can save valuable time and effort, facilitating the immediate focus on implementing features rather than troubleshooting device-specific issues.
Key features of React Device Detect include:
- Simple Setup: Integrating this library requires only a few steps, making it accessible even for novices.
- Comprehensive Device List: It covers a wide range of devices, reducing the chance of missing out on specific user environments.
- Component Render Logic: Developers can conditionally render components based on the detected device type, streamlining the user interface.
Example usage of React Device Detect:
Through this library, developers can enhance the functionality of their applications while ensuring a tailored experience for mobile users.
Using React Responsive
Another powerful tool for mobile detection is React Responsive. This library excels at building responsive components that can adjust based on device size and capabilities. By applying CSS-like media queries right in your JavaScript, it provides a flexible approach to design.
The main benefits of using React Responsive include:
- Media Query Support: Define breakpoints straight within React components, improving maintainability of the code.
- Conditional Rendering: Like React Device Detect, you can render different components based on the screen size detected, catering to various devices efficiently.
- Lightweight: The library is lightweight, minimizing the impact on application performance.
To implement React Responsive, the setup involves straightforward integration that allows for responsive design practices using components directly in your app. Here's a simple example:
Utilizing React Responsive not only streamlines your development process but also enhances the overall user experience. By effectively responding to the device's specifications, you ensure that all users interact with your application as intended, regardless of their device capabilities.
By leveraging libraries like React Device Detect and React Responsive, developers can significantly enhance their ability to deliver a tailored experience for mobile users. Understanding how to efficiently utilize these tools allows for superior application responsiveness.
Browser User Agent Detection
Browser User Agent Detection is an essential aspect of identifying mobile devices within React applications. This technique allows developers to determine the type of device being used by a user, enabling them to tailor experiences accordingly. With the increasing diversity of devices capable of accessing web applications, understanding how to leverage the User Agent string becomes paramount. This section delves deep into accessing and parsing the User Agent, illustrating the practical benefits and potential challenges of this methodology.
Accessing User Agent String
The User Agent string is a line of text that a browser sends to the server with each request. It contains useful information about the device, including the operating system, browser version, and mobile device capability. In the context of React, accessing the User Agent string is quite straightforward. The property can be used within JavaScript to retrieve this string.
This method provides developers with a raw string, which can be further processed to extract relevant data. The accessibility of the User Agent string makes it a commonly used approach to detect devices in various web applications. However, developers should keep in mind that this string may vary across browsers and devices. This variability can affect the consistency of device identification, necessitating cautious implementation.
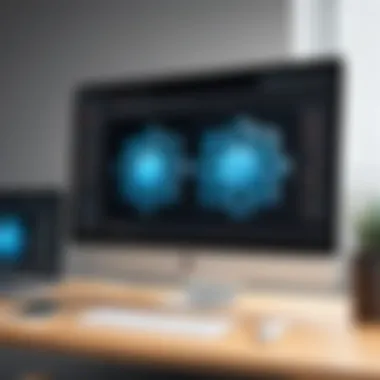
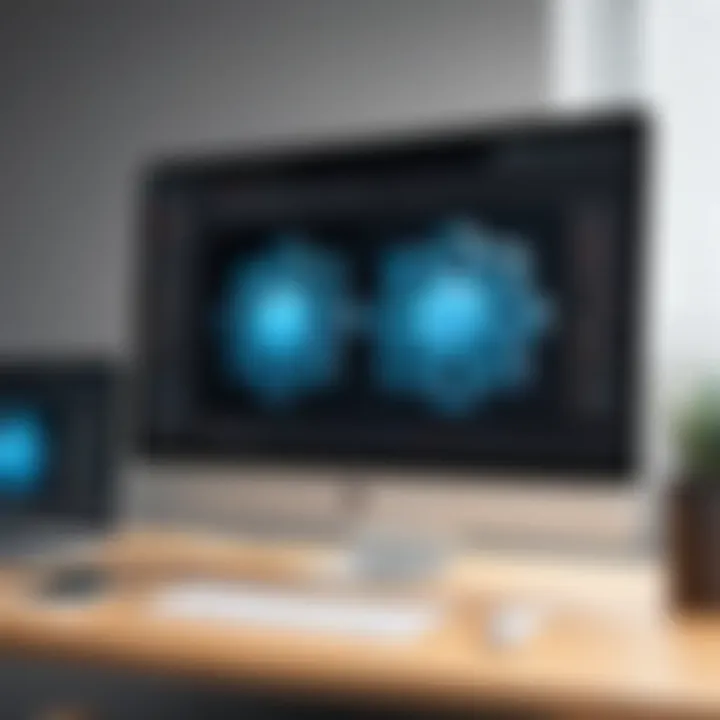
Parsing the User Agent
Once the User Agent string is accessed, the next step is parsing it. Parsing involves breaking down the string into understandable parts. This can help in determining what type of device the user is on – whether it is a mobile, tablet, or desktop. There are libraries, such as , which can simplify this process. Here's a basic example of how it can be used:
This will yield an object that describes the user's device, making it easier to implement tailored features in React applications. Understanding how to effectively parse the User Agent string can greatly enhance the adaptability of the application. But, it’s important to note potential pitfalls; for instance, alterations in User Agent strings by browsers for privacy reasons may hinder the accuracy of the parsing process.
By leveraging User Agent detection thoughtfully, developers can significantly improve user experience across a range of devices.
In summary, Browser User Agent Detection equips developers with crucial insights about user devices. Accessing the User Agent string is simple, yet parsing it accurately is vital for making informed decisions when it comes to optimizing React applications for various devices. Balancing the benefits of this detection method with its inherent challenges is key to creating a responsive and user-friendly web environment.
Implementing Mobile Detection in React Components
Implementing mobile detection in React components is crucial for tailoring user experiences effectively. As a framework that emphasizes component-driven architecture, React provides an ideal platform for detecting screen sizes, orientations, and specific device characteristics. Mobile detection allows developers to ensure that users receive a suitable interface, enhancing usability and satisfaction.
With the wide variety of devices employing different screen sizes and capabilities, recognizing the type of device accessing a web application becomes indispensable. Incorporating this functionality allows the application to adapt its content, layout, and features accordingly. This could mean the difference between a cluttered view and a neatly organized interface depending on whether a user is on a smartphone or a tablet.
When implementing mobile detection, considerations like performance and maintainability must also be taken into account. Efficiently detecting mobile devices can reduce load times and optimize rendering processes, which is especially significant for mobile users often having slower internet connections. Moreover, the code should remain clear and maintainable to facilitate future updates and debugging, which becomes more challenging as an application scales.
Overall, equipping React components with mobile detection not only enhances functionality but also leads to greater user retention and engagement.
Creating a Custom Hook
Creating a custom hook for device detection is a pragmatic approach in React. This hook can consolidate the logic for identifying device types and facilitate the same pattern across different components. By using a custom hook, developers can avoid code duplication. The hook can encapsulate the logic for detecting devices, keeping components clean and focused on their core responsibilities.
Here is a simple example of how to implement a custom hook to detect mobile devices:
This custom hook efficiently determines if the device is a mobile device by checking the viewport width. When implemented, it returns a boolean value indicating whether the user's device falls into the mobile category.
Conditional Rendering Based on Device Type
Conditional rendering based on the device type allows developers to serve content that best fits the user's needs. With the awareness of device type established through the custom hook, one can employ this information within the component's render logic. This results in a more responsive design that is sensitive to user context.
For instance, using the custom hook detailed earlier, you might have a component like so:
In this example, the function checks if the user is on a mobile device. Depending on this state, it conditionally renders different content. Such a pattern enhances clarity, as it keeps the interface relevant to user expectations, directly improving the experience.
Testing Mobile Detection Functionality
Testing mobile detection functionality is a crucial phase in the development of React applications. Understanding how your application interacts with different devices is essential to ensure a seamless user experience. With the diversity in mobile devices, screen sizes, and operating systems, testing confirms that mobile detection works as expected across all potential use cases.
Writing Unit Tests
Unit tests serve as the foundation for validating individual components of your application, including mobile detection logic. By isolating the mobile detection mechanism, developers can confirm that their implementation reacts appropriately to various device types. This involves creating test cases that simulate different user agents and device characteristics. React Testing Library and Jest are tools that can be used to facilitate this process.
For example, a simple test might create a mock user agent string that represents a mobile device, then check whether the component renders correctly based on that string. Here’s a brief illustration:
This type of unit test ensures that if the detection mechanism changes or if the implementation of the mobile interface is altered, developers can quickly identify issues. Implementing comprehensive unit tests provides confidence in the application’s responsiveness to various devices.
Using Browser Emulators
Browser emulators are practical tools in the testing phase, allowing developers to simulate different mobile devices on their desktops. They help to visualize how an application will function across various platforms without the need for physical devices. Tools like Chrome DevTools come with responsive design features that offer device simulation.
When utilizing browser emulators:
- Select Device Type: Choose from a preset list of devices to see how the application behaves under different conditions.
- Adjust Screen Sizes: Modify the viewport to check responsiveness beyond standard mobile dimensions.
- Test Touch Events: Simulate touch interactions to ensure that the app responds correctly.
It’s important to note that while emulators are useful, they may not fully replicate the experience of real devices. For example, aspects like performance or certain native features might not be accurately represented. As such, complementary testing on actual devices is recommended.
By employing a combination of unit tests and browser emulators, developers can systematically address the intricacies of mobile detection. This approach ultimately safeguards against potential oversights, ensuring robust, user-friendly experiences across diverse devices.
"The best way to predict the future is to invent it." - Alan Kay -
Overall, testing is not just about finding bugs but also about enhancing the overall quality of the application. This ensures that users find the app functional and engaging, regardless of the device they are using.
Performance Considerations
In modern web development, especially in React applications, performance considerations regarding mobile device detection must be taken into account. This is crucial because users expect seamless experiences, irrespective of the device they are using. A detailed approach to performance can lead to improved load times, better responsiveness, and overall satisfaction. If mobile detection is not optimized, it can introduce unnecessary overhead, adversely impacting the user experience found within apps. It is not just about functionality, but also about ensuring that the application remains efficient and engaging.
Impact of Mobile Detection on Render Time
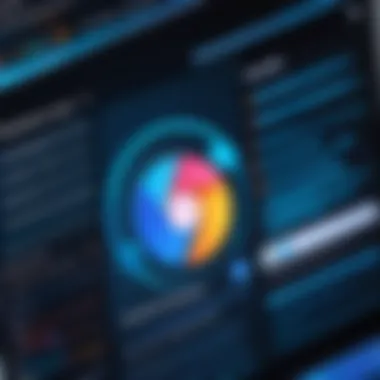
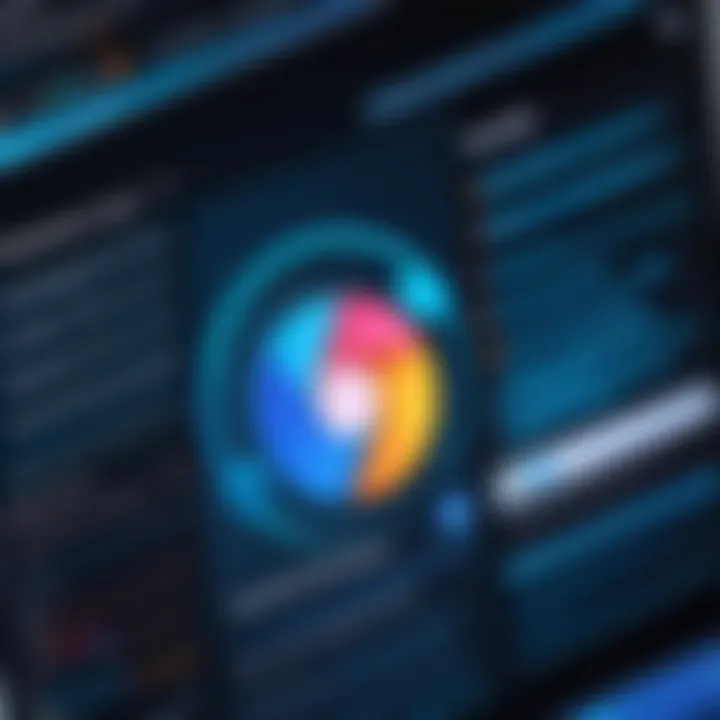
Rendering time can be significantly affected by how mobile detection is implemented. When mobile detection logic is complex, it can lead to delayed rendering. This is often seen in applications where multiple checks are performed to determine the device type. Complexity can arise when using several libraries alongside custom code to assess device characteristics. The approach must be straightforward, ensuring efficiency without sacrificing functionality.
One common method is to minimize the number of checks done during the initial render. This can be done through memoization techniques or by implementing lazy loading for heavier components. Keeping the detection logic as simple as possible significantly reduces the burden on render time.
"Optimizing the rendering process for mobile detection is not an option, it is a necessity for enhancing user experience."
Optimizing Resource Loading
Optimizing resource loading serves as a critical strategy for ensuring that mobile detection does not impede performance. Efficient resource management involves loading only what is necessary for the device in use. For mobile devices, this might mean adopting a different approach to images, fonts, and scripts. More lightweight alternatives should be considered based on the device’s capabilities.
A few strategies to consider include:
- Using responsive images with the element or attributes to load images efficiently based on screen size.
- Implementing code splitting to load only the React components necessary for each specific device, reducing the overall bundle size.
- Leveraging service workers for caching, allowing assets to be preloaded for faster access across subsequent visits.
By integrating these practices, developers can facilitate quicker loading times and better performance across mobile devices. This optimization enhances the overall user experience, ensuring users can access content without frustrating delays.
Taking these performance considerations into account reflects a commitment not only to technical excellence but to user satisfaction.
User Experience Enhancements
In the context of web development, especially for React applications, user experience plays a crucial role. Enhancing user experience means making sure that users can navigate and interact with web pages smoothly and intuitively. It's not just about aesthetics; it also encompasses usability and accessibility. Thus, the importance of user experience enhancements in mobile device detection cannot be overstated. When applications recognize the specific devices users are on, they can offer a more tailored experience, which leads to greater user satisfaction.
There are specific elements to consider when enhancing user experience through mobile detection:
- Responsive design: Adapting the layout according to different screen sizes ensures clarity and ease of use.
- Performance optimization: Mobile devices often have limited processing power and bandwidth. Streamlining resources improves load times and overall functionality.
- User interface elements: Modifying the interface components based on the device type allows for intuitive interactions, making the design user-friendly.
These enhancements lead to several benefits:
- Increased engagement: A well-tailored user experience encourages users to spend more time on the application.
- Higher conversion rates: When users find what they need quickly, they are more likely to complete desired actions, such as purchases or sign-ups.
- Less frustration: Avoiding common issues faced by mobile users, such as improper navigation, significantly reduces frustration and helps in improving overall satisfaction.
Considerations for user experience enhancements should always include testing and feedback from users. It is wise to gather insights on how real users navigate the application on various devices. This will help in refining practices continuously.
Tailoring User Interface
Tailoring the user interface is a fundamental aspect of enhancing user experience. It involves modifying the arrangement of elements on the screen and their interactions depending on the device being used. For instance, touch targets might be larger on a mobile device compared to a desktop, where users employ a mouse. Additionally, content layout may vary; single-column formats are often preferred on smartphones for easier scrolling.
Some guidelines for tailoring user interface include:
- Color schemes and fonts: Choosing colors that are easily visible on smaller screens ensures accessibility. Fonts must also be adjustable to maintain readability across devices.
- Navigation patterns: Mobile menus should be simplified. Utilizing hamburger icons and bottom navigation can streamline access to essential features.
- Content prioritization: Highlighting critical information prominently and reducing visual clutter helps users focus on essentials without distraction.
This approach can significantly improve how users interact with an application, making their experience more enjoyable.
Providing Device-Specific Features
Providing device-specific features is vital for creating a more engaging and practical user experience. By leveraging the capabilities of mobile devices, developers can create custom functionalities that better serve mobile users. For example, utilizing device sensors, such as GPS, can enhance the application by delivering relevant location-based services.
The following features can be particularly useful:
- Camera access: Allowing quick photo uploads or QR code scanning can improve user interaction.
- Touch and gesture controls: Mobile users expect swipes and taps to navigate; thus, implementing these features can enhance interactivity.
- Notifications: Tailoring push notifications for mobile devices fosters real-time engagement with users, reminding them to interact with the app.
Challenges in Mobile Detection
Detecting mobile devices in a React application is not an easy task. As devices are highly diverse and continually evolving, it raises specific challenges. Understanding these challenges is crucial for developers aiming to optimize user experience on mobile platforms.
Device Fragmentation Issues
Device fragmentation is an important factor when discussing mobile detection. The landscape of mobile devices is vast. Manufacturers like Apple and Samsung frequently release new models with different screen sizes, resolutions, and capabilities. This is further complicated by various operating systems, such as Android and iOS, and their different versions.
Fragmentation makes it difficult to generalize user experiences. A React application that works seamlessly on an iPhone might not perform the same on an Android device. This variance necessitates a deeper understanding of device capabilities, ensuring that the application provides a uniform experience across different devices. The complexity increases with the addition of tablet devices, which can blend features of both mobile phones and laptops.
"Effective mobile detection considers the wide variety of devices and operating system versions, tailoring the user experience accordingly."
Tackling fragmentation requires developers to use a combination of approaches for mobile detection. They may need to tailor styles or functionality based on the unique attributes of each device, leading to more extensive testing and maintenance.
Evolving User Agent Strings
User agent strings provide essential information about the device's operating system, browser version, and the specific device type. However, these strings are not static. They evolve as web browsers update, leading to potential challenges in accurate mobile detection.
Consider a situation where a new mobile browser is released. It may have a user agent string that does not match current detection criteria, resulting in misidentification of the device type. This can lead to rendering issues or improper loading of mobile-specific features.
The dynamic nature of user agent strings means that developers must stay updated on changes and trends. Many libraries are available to assist in parsing these strings, yet outdated libraries can present further complications. Regularly updating dependencies in React applications is essential to ensure accuracy in mobile detection.
End
Detecting mobile devices in React applications is crucial for creating a seamless user experience. As mobile usage continues to rise, being able to tailor content and functionality based on the device type is no longer a luxury but a necessity. In this article, we have explored various methodologies for mobile detection, emphasizing their importance in enhancing user engagement and satisfaction.
Summary of Key Takeaways
- Mobile Detection Strategies: Understanding the range of tools and techniques for detecting mobile devices is essential. Libraries like React Device Detect and React Responsive streamline this process, allowing developers to focus on delivering user-centric applications.
- Responsive Design Principles: Implementing mobile-first design principles and CSS media queries is vital to ensure that applications adapt seamlessly between devices of varying sizes and capabilities.
- User Agent String Analysis: Effectively parsing the user agent string remains an essential skill, enabling developers to identify specific device characteristics and respond accordingly.
- Testing and Optimization: Regular testing and performance optimization are key to maintaining application responsiveness and user satisfaction across devices.
- Future Awareness: Staying informed about emerging trends in mobile technology is critical for developers looking to stay ahead in the rapidly evolving digital landscape.
Future Trends in Mobile Detection
As technology progresses, the nature of mobile detection is likely to evolve significantly. Here are a few trends that developers should keep an eye on:
- AI-Driven Detection: The infusion of artificial intelligence into mobile detection could lead to more sophisticated methods of identifying device capabilities and tailoring content accordingly.
- Increased Privacy Regulations: With user privacy becoming a major concern, developers may need to adapt their detection strategies to comply with regulations such as GDPR, limiting access to certain device information.
- Device-Agnostic Approaches: There is a growing shift towards creating device-agnostic solutions that work uniformly across platforms. This approach minimizes differentiation based on device type while maximizing consistency in user experience.