Exploring the Dictionary ADT in Computer Science
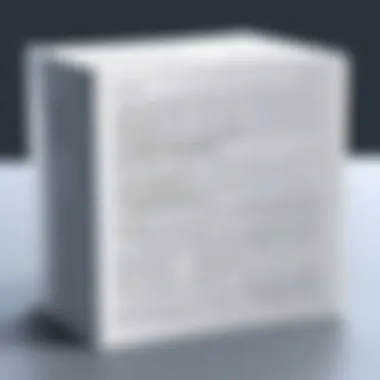
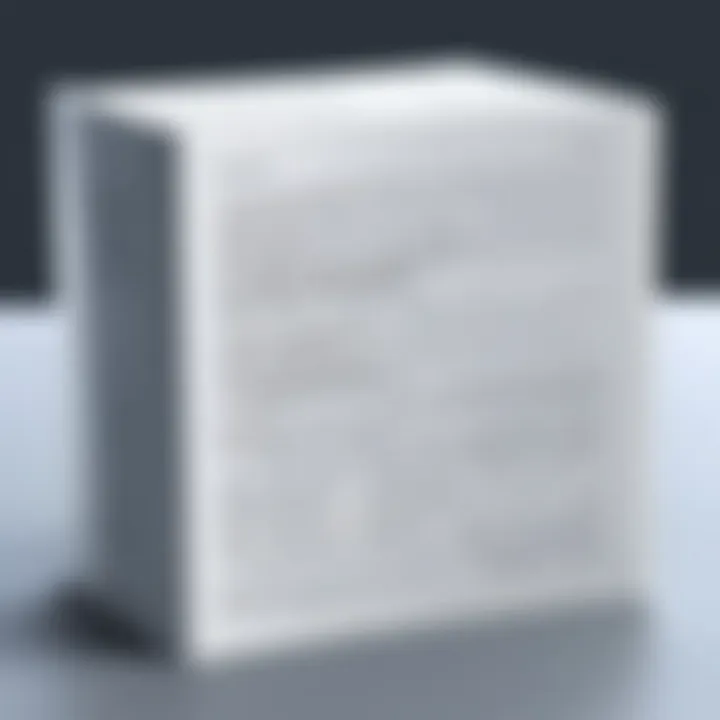
Overview of Topic
Understanding the world of Dictionary Abstract Data Types (ADTs) can feel like peeling layers from an onion. At first glance, it seems straightforward, but delve a little deeper, and you uncover rich complexities that are foundational to data management and retrieval in computer science.
Preamble to the Main Concept Covered
A dictionary ADT allows for efficient storage and retrieval of data pairs, akin to looking up a word in a standard dictionary. Instead of merely storing values, the dictionary pairs them with unique keys. This structure enhances speed in data operations, invaluable in data-heavy applications.
Scope and Significance in the Tech Industry
In the tech landscape, where speed and efficiency are paramount, the relevance of dictionary ADTs cannot be overstated. They're utilized across various domains, from web development to database management systems. Managing large sets of data efficiently can make or break applications. Their ability to rapidly retrieve information fosters not only performance improvements but also optimizes resource usage.
Brief History and Evolution
Historically, the concept of key-value storage has roots tracing back to early computing. Implementations have evolved from basic array or list structures to sophisticated forms like hash maps. Each evolution has been driven by the desire to enhance performance and address the challenges posed by growing data volumes.
Fundamentals Explained
Getting into the nitty-gritty of dictionary ADTs reveals several core tenets essential for anyone aiming to get a firm grasp on their workings.
Core Principles and Theories Related to the Topic
The dictionary ADT is built on the premise of associative arrays, where each key maps to a value. This relationship is what provides dictionaries their power. Think of it like a family tree where each member (key) has their own unique identity (value).
Key Terminology and Definitions
To navigate the topic confidently, you ought to be familiar with some crucial terms:
- Key: The unique identifier for a value within the dictionary.
- Value: The data associated with a specific key.
- Collisions: An event where two keys hash to the same value, posing challenges in retrieval.
Basic Concepts and Foundational Knowledge
One essential concept in dictionary ADTs is hashing. Hash functions transform keys into index positions, enabling rapid access to values. The effectiveness of dictionary ADTs lies significantly in the quality of the hash function used.
Practical Applications and Examples
Real-World Case Studies and Applications
Consider a scenario in e-commerce platforms. They use dictionary ADTs extensively to manage user sessions, where user ID serves as a key, and session data acts as the value. This allows quick access to user information when needed.
Demonstrations and Hands-On Projects
A simple project could involve creating a phone book application using a dictionary ADT. Each contact name can be the key, and the corresponding phone number the value, simplifying lookups and modifications.
Code Snippets and Implementation Guidelines
Here’s how you might implement a basic dictionary using Python:
This snippet highlights how straightforward it is to utilize dictionary ADTs in programming.
Advanced Topics and Latest Trends
Cutting-Edge Developments in the Field
Recent advancements include improvements in hashing algorithms that reduce collision rates and enhance retrieval speed. Techniques like cuckoo hashing and open addressing represent interesting developments in managing collisions efficiently.
Advanced Techniques and Methodologies
Focusing on performance, adaptive hash tables dynamically adjust their structure based on workload, a feature beneficial in high-performance computing scenarios.
Future Prospects and Upcoming Trends
Looking ahead, the integration of dictionary ADTs with machine learning models is gaining traction. They can provide context-aware data access, revolutionizing smart applications across industries from healthcare to finance.
Tips and Resources for Further Learning
Recommended Books, Courses, and Online Resources
For a deeper understanding, consider:
- "Data Structures and Algorithms Made Easy" by Narasimha Karumanchi
- Online courses on platforms like Coursera, Udacity, or edX that delve into data structures.
Tools and Software for Practical Usage
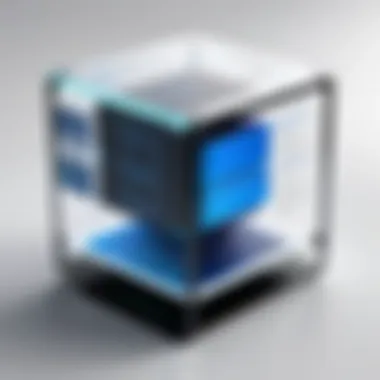
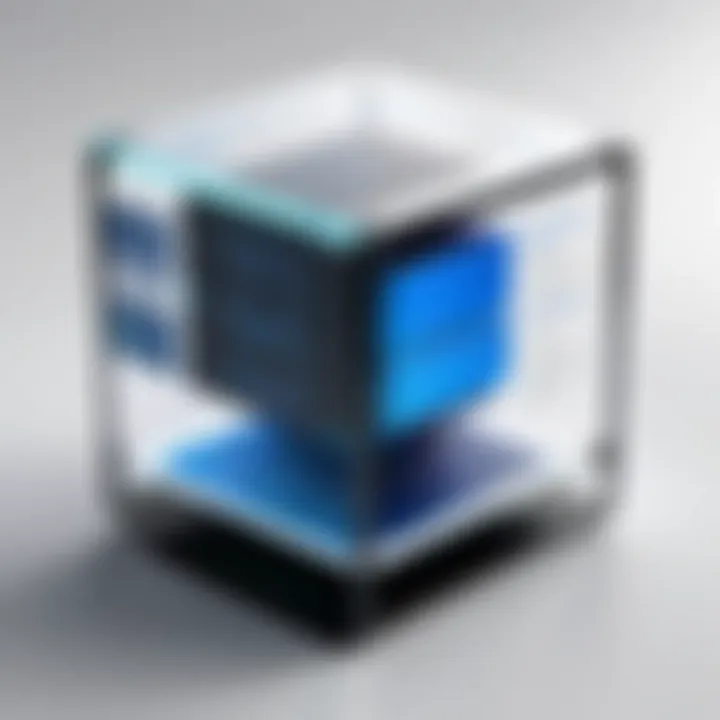
Some widely used tools for implementing dictionary ADTs effectively are:
- Redis: A powerful in-memory data structure store, used extensively in caching solutions.
- MongoDB: This NoSQL database offers key-value storage solutions maximizing retrieval speeds.
In summary, this exploration of dictionary ADTs showcases their crucial role in technology and programming. Understanding their fundamentals, practical applications, and advanced techniques empowers developers to leverage these data structures effectively.
Intro to Abstract Data Types
In the vast landscape of computer science, abstract data types (ADTs) play a crucial role in how we organize and manipulate data. While the term may sound daunting at first, understanding principles behind ADTs is not only beneficial but also necessary for anyone venturing into the realms of software development and algorithm design. At the very core, ADTs provide a way to define data structures by purely focusing on their behavior from the user's perspective, rather than their implementation details.
For programmers and researchers alike, grasping ADTs means being able to choose the right structure for the job. This can make a world of difference in terms of efficiency and clarity in software applications. ADTs encapsulate methods that can operate on the data, providing a clear interface for interaction. They abstract away the nitty-gritty of various implementations, allowing developers to utilize the functionally while leaving the intricacies behind. This layering of complexity helps in better understanding, debugging, and maintaining code, ultimately leading to more robust systems.
Defining Abstract Data Types
So, what exactly is an abstract data type? At its essence, an ADT is a theoretical concept that defines a data structure purely by its behavior. This means it outlines how data can be stored and manipulated without getting bogged down in how those operations are executed. An ADT comprises a set of values, or more specifically, the operations that can be performed on those values. For instance, consider a simple stack ADT. It has basic operations like , , and , which define how you interact with the stack without specifying how it should be implemented (using an array, a linked list, etc.).
The clarity that ADTs bring is invaluable. They simplify complex concepts and allow researchers and practitioners to speak a common language, effectively reducing misunderstandings when discussing data manipulation strategies.
The Role of ADTs in Computer Science
In the world of computer science, the relevance of ADTs cannot be overstated. They serve as the backbone for numerous sophisticated algorithms and data structures. Let’s shine a light on a few key roles ADTs hold:
- Standardization: By defining clear interfaces for various data types, ADTs make it easier for developers from different backgrounds to collaborate on a single project. This is crucial in larger teams or open-source contributions.
- Implementation Flexibility: Since users interact with the ADT without needing to understand its implementation, developers can swap different implementations without affecting the user experience. For example, a dictionary can be implemented as a hash table or a balanced tree, depending on the needs of the application.
- Focus on Problem-Solving: ADTs allow programmers to concentrate on solving problems rather than getting tangled in the details of data structures. This results in cleaner code and higher-quality software solutions.
In closing, the concept of abstract data types serves as a critical foundational element in the toolbox of computer science practitioners. By defining clear expectations for data interaction, ADTs empower us to build more sophisticated systems efficiently. This article will further unravel the concept of Dictionary ADTs—one of the most widely utilized forms of abstract data—and illustrate how they function in modern software development.
Understanding Dictionary ADTs
In the realm of computer science, grasping the concept of Dictionary Abstract Data Types (ADTs) stands as a cornerstone to mastering efficient data management. A Dictionary ADT serves as a structure that allows programmers to store and retrieve data in a flexible manner, pairing keys to their corresponding values. This makes it an invaluable tool for anyone venturing into software development, as it not only optimizes data accessibility but also enhances overall code readability and maintainability.
What is a Dictionary ADT?
A Dictionary ADT is essentially a collection of pairs, where each pair contains a key and a value. The fundamental characteristic here is that every key must be unique within the dictionary, allowing the value associated with it to be easily accessed. To illustrate, think about a phone book: names serve as keys, while their respective phone numbers represent the values. When you search for a name, you effortlessly find the associated number, highlighting its utility in the rapid retrieval of data.
Here are some pivotal properties of Dictionary ADTs:
- Key-Value Pair: Each entry in a dictionary consists of a unique key associated with its value, which makes it straightforward to locate information.
- Dynamic Sizing: Dictionaries adapt their size dynamically, accommodating varying amounts of data without the programmer needing to specify a limit upfront.
- Versatility: They can be used in a multitude of programming tasks, from simple data lookups to complex algorithms in computational tasks.
One of the common implementations of a Dictionary ADT is through a hash table. The hash table uses a hash function to compute an index where the key-value pair should be stored, which comes with advantages in terms of speed, especially for large datasets.
Key Features of Dictionary ADTs
Understanding the significant features of Dictionary ADTs sheds light on their practical applications and implementation strategies.
- Fast Access and Retrieval: The hallmark of a Dictionary ADT is its speed in accessing elements. For most operations like insertion, deletion, and lookup, it achieves an average-case time complexity of O(1) depending on the implementation.
- Flexibility in Data Handling: Since Dictionary ADTs manage key-value pairs, they provide a versatile method for storing different types of data. It’s not uncommon for programmers to use dictionaries to manage configurations, maintain user sessions, or handle general application data.
- Collision Handling: In cases like hash table implementations, collisions can occur when two keys hash to the same index. Dictionary ADTs have strategies, such as linear probing or chaining, to efficiently resolve these collisions.
- Hierarchical Structures: Some implementations, like binary search trees, offer hierarchical data management, allowing for ordered data retrieval—something that can be handy in applications requiring sorted datasets.
- Support for Complex Structures: Advanced Dictionary ADTs can even store complex data types. For instance, in programming languages like Python, one can have a dictionary where the values are lists or other dictionaries, creating a nested structure that models real-world relationships effectively.
By delving into the core concept and features of Dictionary ADTs, aspirational programmers and IT professionals can appreciate their versatility and importance in data management. Understanding these aspects lays the groundwork for exploring various implementations and learning how they can be optimized for specific tasks.
"The ability to efficiently store, retrieve, and manage data through Dictionary ADTs is fundamental for navigating the complexities of software development in the modern world."
Core Operations of Dictionary ADTs
A Dictionary ADT's core operations serve as the lifeline of data manipulation, closely tying the concept of how data is stored, managed, and retrieved. These operations—insertion, deletion, and searching for keys—highlight both practical implementations and theoretical principles in computer science. Understanding these essential actions not only lays the groundwork for more complex data structures but also greatly enriches programming practice. Students and IT professionals alike will benefit from a firm grasp of these operations, as they often dictate system efficiency and performance.
Insertion of Key-Value Pairs
The process of inserting key-value pairs into a Dictionary ADT is foundational. This operation builds the relationship between a unique key and its associated value, essentially crafting a map within the data structure. When inserting, one must ensure Uniqueness of Keys; if a duplicate key is presented, the existing value may either be updated or an error thrown, depending on the specific implementation.
Example & Considerations:
- When utilizing a hash table, the insertion process typically involves a hash function that transforms the key into an index. The efficiency of this operation hinges on the quality of this hash function, as poor performance could lead to scenarios called collisions, where two keys hash to the same index.
- In contrast, in a binary search tree, inserting requires navigating left or right based on comparisons. The efficiency here directly connects to tree balance; therefore, understanding how these trees can become unbalanced is crucial.
Both methods come with distinct pros and cons. While hash tables offer fast average-case performance, tree structures, when balanced, provide ordered data retrieval, which can be a boon for certain types of queries.
Deletion of Key-Value Pairs
Deletion is another pivotal operation within Dictionary ADTs, allowing programmers to remove unwanted pairs from the collection. This can sometimes bring challenges, especially in the context of maintaining the integrity of the structure afterward.
Approaches:
- In a hash table, removing a key might mean you not only need to decide which collision resolution method you’re using but also how to handle the freed slot to maintain lookup efficiency.
- For binary search trees, the deletion is slightly more complicated. When a node is deleted, several steps may need to be taken depending on if the node has children or not. A common strategy is to replace the deleted node with either its immediate predecessor or successor, preserving the tree structure.
Maintaining a clean and efficient data structure post-deletion is vital; otherwise, you risk degrading performance, leading to longer search times down the line.
Searching for Keys
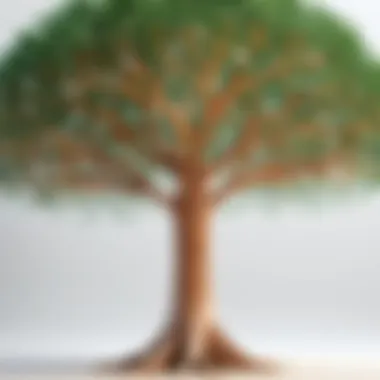
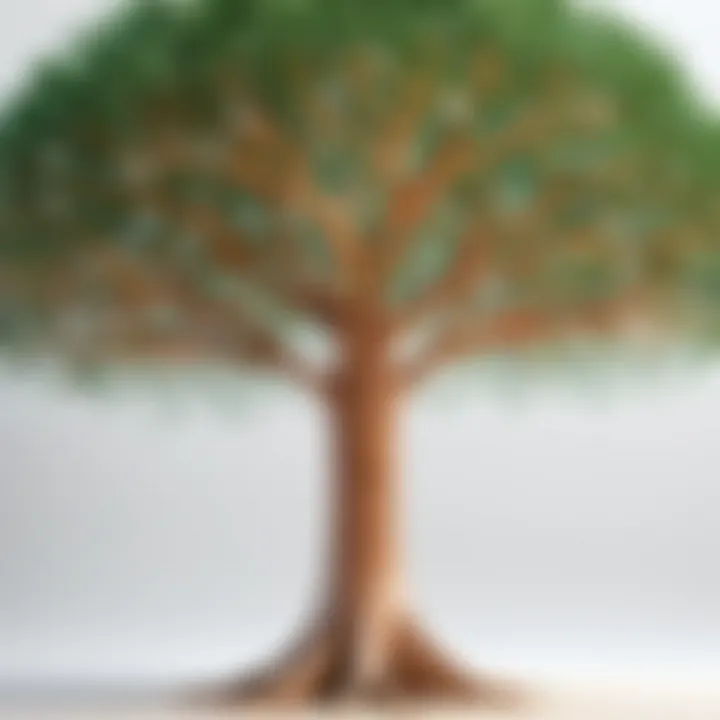
The operation of searching is perhaps the most frequently invoked task within Dictionary ADTs. Ensuring that the search operation is efficient can make or break a system’s overall performance.
Techniques and Nuances:
- With hashing, the hashed key leads directly to the value in an average-case scenario. The efficiency relies on the hash function’s ability to evenly distribute keys across the array. If too many keys collide, the search turns quickly inefficient.
- When searching within a binary search tree, one exploits the properties of order. Starting from the root, one can eliminate half of the tree with each comparison—navigating left or right depending on the current key’s value relative to the nodes.
The efficiency of searching often defines user experience. A sluggish search can frustrate users, while a swift one can create a seamless interaction.
In summary, mastering these core operations—insertion, deletion, and searching—is crucial for anyone engaged in software development. They act as the main gatekeepers controlling data flow and accessibility within various applications. Grasping these foundational elements can empower programmers to develop more efficient and effective systems.
Implementations of Dictionary ADTs
When exploring the world of Dictionary Abstract Data Types (ADTs), it becomes clear that their implementations play a crucial role in how efficiently they manage data. Various methods are available, each with its own set of features that make it suitable for different applications. The choice of implementation can affect performance, scalability, and data organization. Understanding the landscape of these implementations provides valuable insights into selecting the most appropriate method for a given use case.
Array-Based Implementation
In an array-based implementation, Dictionary ADTs utilize a fixed-size array to store key-value pairs. This method allows for quick access to elements since arrays provide constant time complexity, O(1), for retrieval operations if the index is known. One significant benefit is the simplicity of the structure—arrays are easy to understand and manipulate.
However, there are limitations. The size of the array must be determined ahead of time, which can lead to wasted space or, conversely, overflow if the dataset exceeds the expected number of entries. This implementation is well-suited for scenarios where the data size is predictable and relatively small. In a nutshell, array-based implementations shine in specific use cases while exhibiting weaknesses in flexibility.
Linked List Implementation
The linked list implementation presents a different approach by using nodes that link to each other, with each node containing a key-value pair. This structure allows for dynamic sizing, meaning elements can be added or removed without needing to allocate or deallocate large blocks of memory. The flexibility to grow as needed is a big plus.
Still, the trade-off comes in the form of performance. Searching for keys in a linked list can result in longer execution times compared to arrays, as it generally requires O(n) time complexity due to the need to traverse the list. This structure can work well when there is an unpredictable number of entries or when frequent deletions and insertions are expected.
Hash Table Implementation
Hash tables are among the most popular implementations of Dictionary ADTs. They store key-value pairs in a way that allows for efficient access in average time complexity O(1). A hash function computes an index based on the keys, directing the lookup directly to the appropriate location.
Collision Resolution Techniques
One challenge with hash tables is handling collisions when two keys hash to the same index. Various collision resolution techniques are employed to address this issue. For instance, chaining involves linking all entries with the same hash index into a secondary structure, like a linked list. Another technique, open addressing, searches for the next open slot in the array when a collision occurs.
These techniques contribute significantly to maintaining the table's performance. Chaining is advantageous in cases of high collisions since additional entries can be stored without resizing the array. However, this can also lead to longer lookup times if many items cluster at a single index. Understanding collision resolution is vital for ensuring optimal operation of a hash table.
Load Factor Considerations
The load factor represents the number of entries in a hash table relative to its capacity. It is a key characteristic influencing the performance of the hash table. A high load factor may lead to more frequent collisions and decreased efficiency, while a low load factor can waste memory.
Managing the load factor efficiently often entails resizing the hash table when a certain threshold is exceeded. This process can momentarily slow operations but prevents long-term performance degradation. As such, a keen eye on load factor considerations aids in sustaining a well-performing Dictionary ADT.
Binary Search Tree Implementation
Binary Search Trees (BST) are another strong option for implementing Dictionary ADTs. Each node in a BST contains a key and ensures that keys in the left subtree are less than the parent key, while keys in the right subtree are greater. This organization allows for efficient searching, insertion, and deletion operations in average time complexity O(log n).
Balancing Techniques
However, performance can degrade if a BST becomes unbalanced, often resembling a linked list and leading to O(n) complexity in the worst case. That's where balancing techniques, such as AVL trees or Red-Black trees, come into play. These methods ensure that the tree remains balanced after insertions and deletions, enhancing performance significantly.
Maintaining balance is not just a nice-to-have; it's crucial for keeping operational efficiency. With effective balancing techniques, a BST implementation provides both fast runtime and dynamic data handling without fear of degeneration.
Advantages and Disadvantages
The advantages of BST implementations lie in their efficiency for search and update operations, combined with dynamic resizing capabilities. As with any structure, they do, however, come with their disadvantages. In the worst-case scenario, they can degenerate into a linked list, leading to slower operations. Additionally, implementing balancing strategies adds complexity compared to simpler structures.
In summary, each method of implementing Dictionary ADTs—be it array-based, linked list, hash table, or binary search tree—comes with its unique set of benefits and challenges. The context of the application often dictates which implementation will serve best.
Performance Analysis of Dictionary ADTs
Performance analysis of Dictionary Abstract Data Types (ADTs) is a crucial element in understanding how these data structures function in real-world applications. When we work with data, we need to be sure our methods of organizing and retrieving it are efficient. This analysis not only highlights the speed and resource consumption of various Dictionary ADT implementations but also guides developers in making informed choices based on the needs of their applications. Such insights are invaluable for improving software performance, minimizing latency, and managing resources effectively.
Time Complexity Overview
Time complexity gives us a clear picture of how the performance of a Dictionary ADT scales with an increase in data volume. It's like a compass for developers; it helps navigate through performance bottlenecks and select the most appropriate implementations for their specific needs.
Average Case Analysis
The average case analysis sheds light on expected performance during typical usage scenarios. It considers average keys and values that a dictionary may handle, thus filtering out the extremes that may skew functionality. One key characteristic of average case analysis is that it provides a realistic benchmark, making it a solid choice for evaluating Dictionary ADTs.
When a developer designs a system, they can rely on average case scenarios to anticipate the usual workload. For instance, if you use a hash table with good hash functions, the average time complexity for search operations tends to be O(1). This simplicity is often seen as beneficial in situations where quick data retrieval is paramount. However, one should note that this analysis can be misleading in scenarios where certain data patterns lead to increased collisions, which could ultimately drag performance down.
Worst Case Analysis
Contrastingly, worst case analysis paints a picture of the direst scenarios. It accounts for setups where operations take the longest time possible, like finding a key or updating it in a poorly implemented hash table. For instance, if collisions are frequent due to inefficient hashing, the search time complexity can deteriorate to O(n). Thus, this analysis provides an essential safety net for software architects, ensuring they prepare for the less-than-ideal situations.
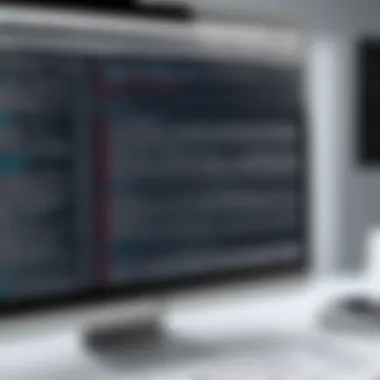
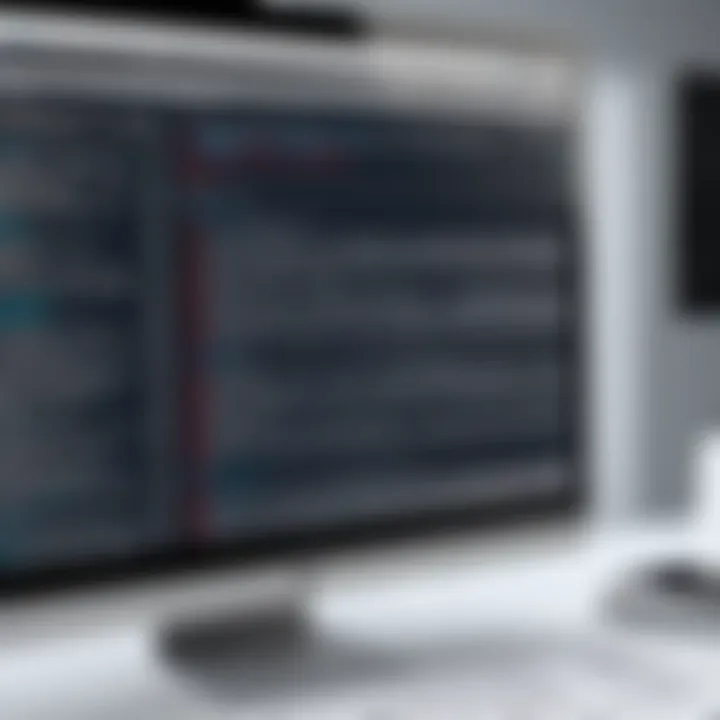
The key trait of worst case analysis is its ability to guide contingency planning. Though not ideal for everyday reference, it prepares developers for the rare, yet possible mishaps in a system's performance. Understanding this can help in structuring failover mechanisms or even to switch to a different ADT when faced with heavy-load scenarios.
Space Complexity Considerations
Space complexity, on the other hand, deals with the amount of memory an implementation requires relative to its size. Factors like overhead due to pointers in linked lists or array resizing in dynamic arrays directly impact this aspect. In practice, knowing the space complexicity of different dictionary variations helps in optimizing memory usage, which is vital in a world that increasingly demands efficient resource management.
By understanding both time and space complexities, developers can create systems that not only perform efficiently under normal circumstances, but also handle unexpected challenges with grace.
Use Cases for Dictionary ADTs
The use cases for Dictionary Abstract Data Types (ADTs) resonate through various domains in software engineering, illustrating the necessity of efficient data handling mechanisms. With their ability to store and retrieve data using key-value pairs, Dictionary ADTs emerge as indispensable tools, particularly in instances where quick access to information is paramount. By analyzing these practical applications, one can better appreciate how integral these data structures are to effective programming and algorithm development.
Application in Databases
In the world of databases, Dictionary ADTs play a crucial role in organizing, storing, and retrieving data. They serve as a foundation for various database management systems (DBMS), enabling optimal data access and manipulation. For instance, consider a relational database where user information is stored. Each user can be mapped to a unique identifier, such as a user ID, which acts as the key in a dictionary, while the corresponding user data serves as its value.
Employing dictionary-like structures facilitates rapid lookups, enabling applications to perform complex queries with relative ease. Additionally, operations such as updates and deletions can be conducted efficiently by directly referencing the key associated with the data to be modified. As databases continue to expand in complexity and scale, reliance on Dictionary ADTs becomes increasingly evident.
Role in Search Algorithms
Search algorithms are a hallmark of computer science, and Dictionary ADTs significantly enhance their performance. When searching for an element within a dataset, the efficiency of the search process often predicates the overall speed of an application. In this context, a dictionary empowers algorithms such as binary search or hash-based search methods, providing the necessary tools for swift key-value retrieval.
For example, imagine implementing a search function in a large e-commerce platform. With thousands of products on offer, using a Dictionary ADT allows developers to efficiently navigate the vast dataset by storing product identifiers as keys and their associated details as values. When a customer searches for an item, the algorithm can swiftly find the correct entry without scanning every single product.
Enhancing Data Retrieval in Web Development
In web development, Dictionary ADTs optimize how data is accessed and presented. Modern web applications often depend on real-time data interactions, making quick data retrieval critical for a seamless user experience. By leveraging dictionaries, developers can manage session data, user preferences, and other dynamically changing datasets more efficiently.
Consider a content management system (CMS) where various types of data need to be managed and displayed. Employing Dictionary ADTs allows the CMS to hold elements like blog posts or user comments in key-value pairs, where the title or user ID serves as the key. This structure not only simplifies the process of fetching data but also enhances the overall performance of the application.
"By implementing Dictionary ADTs in web development, developers are capable of achieving rapid data access, leading to improved user satisfaction and retention."
In summary, the diverse applications of Dictionary ADTs in databases, search algorithms, and web development showcase their versatility and essential role in modern software engineering. As technology continues to evolve, understanding these use cases enables IT professionals, students, and programmers to adopt effective data management strategies that align with contemporary challenges. The importance of such structures will invariably grow, underscoring the need for a robust comprehension of Dictionary ADTs.
Challenges in Implementing Dictionary ADTs
Implementing Dictionary ADTs bring forth unique challenges that practitioners can't overlook. As these abstractions manage key-value pairs to optimize data retrieval, they often require finely tuned structures and algorithms to ensure they perform effectively under various conditions. A deep understanding of these challenges not only aids in better design but also prepares developers for the complexities they might face during real-world applications.
Handling Collisions in Hash Tables
A significant hurdle among Dictionary ADT implementations, especially with hash tables, is collision management. When multiple keys hash to the same index, it becomes necessary to have a robust method for handling this interference. Without a reliable strategy, the performance of the hash table can nose-dive, leading to increased access times and the potential for lost data.
Different techniques can be employed to resolve collisions:
- Chaining: This technique links elements at the same index using a list. When collisions happen, new elements are simply added to this list. It’s somewhat like forming a little queue at the index.
- Open addressing: Here, rather than allowing multiple items in one bucket, a probing algorithm searches for the next available slot. This demands a carefully maintained load factor to ensure efficiency.
- Double hashing: A more complex method that applies a second hash function to find the next slot. It’s like throwing a curve ball; you end up with a less predictable distribution of keys.
Each of these techniques comes with its pros and cons, necessitating consideration based on specific use cases. If the load factor climbs too high, performance suffers, regardless of the chosen collision resolution approach.
"In the world of data structures, mismanaging collisions can lead to a cascade of inefficiencies."
Maintaining Balance in Trees
When dealing with binary search tree implementations of Dictionary ADTs, balance is paramount. An unbalanced tree can degrade performance from logarithmic to linear, making operations sluggish, especially in the worst-case scenarios. Imagine a tree falling over — that’s how ineffective retrieval would become without balance.
Maintaining tree balance can be quite the undertaking but is essential for optimal functionality. Several balancing techniques come into play:
- AVL Trees: These trees automatically keep an eye on balance, applying rotations whenever the balance factor is off. They ensure that the height differences between left and right subtrees never stray beyond one.
- Red-Black Trees: These are a bit more relaxed, requiring fewer rotations than AVL trees. They employ an interesting set of coloring rules to maintain balance, providing relatively quick insertions and deletions.
- Splay Trees: These are unique in that they rearrange the structure based on usage patterns, priding themselves on keeping frequently accessed items closer to the top.
As you manipulate these structures, considerations about their performance and complexity arise constantly. A good balance strategy can make all the difference, just as a well-balanced diet benefits one’s health.
In summary, implementing Dictionary ADTs comes with its own set of obstacles that each developer needs to navigate thoughtfully. By embracing robust collision handling strategies and maintaining balance in tree structures, the efficacy of Dictionary ADTs can be significantly enhanced.
Closure: The Future of Dictionary ADTs
As we wrap up this comprehensive exploration of Dictionary ADTs, it’s vital to consider their evolution and future impact in the technical landscape. Dictionary ADTs are not just foundational elements in programming; they are pivotal in handling complex data efficiently. These data structures stand at the intersection of varied fields like databases, AI, and web technologies, reflecting their broad relevance.
Trends in Data Handling
Today, the focus is on managing large volumes of data efficiently. With the advent of big data, computational techniques constantly evolve. Dictionary ADTs are becoming smarter as algorithms and methodologies improve; for instance, adaptive hashing techniques have surfaced to address collision in hash tables better.
The trends we see today include:
- Increased emphasis on speed: Applications need quick access to data; thus, optimizing algorithms will become essential.
- Parallel processing: Utilizing multiple processors is key in speeding up data retrieval. ADTs designed for concurrent access are gaining ground.
- Machine Learning Integration: ADTs will also play a role in AI; for example, they can facilitate quick data references for model training.
"In a world flooded with data, how we structure and access it is paramount."
Potential Innovations in ADT Implementation
Looking ahead, the innovations in ADT implementations seem promising. We might see:
- Dynamic ADTs: These will adapt based on usage patterns, offering optimized memory usage and time efficiency. This could be revolutionary for mobile devices where resources are limited.
- Hybrid data structures: Combining features from various data structures might provide a more powerful tool for developers. For example, a structure that merges the efficiency of a hash table with the ordering of trees.
- Enhanced collision resolution methods: As hash tables are widely popular, further research into collision handling algorithms will be critical in increasing their effectiveness.
The landscape of Dictionary ADTs is set to expand as technology advances. As applications demand more flexibility and efficiency, the topic of Dictionary ADTs will only grow in significance among students, programming learners, and IT professionals.
By keeping abreast of these trends and innovations, one can better appreciate the role Dictionary ADTs play in supporting the systems we depend on today and in the future.