Effective Strategies for Merging Two Sorted Arrays
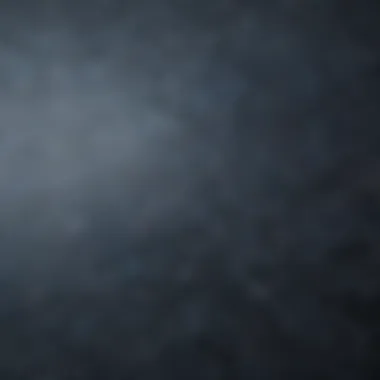
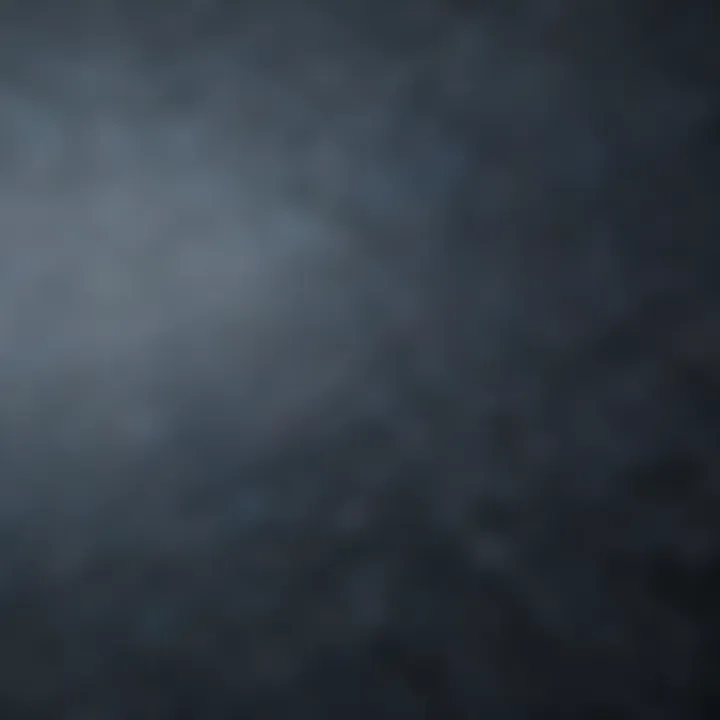
Overview of Topic
In the realm of programming and data structures, the task of merging two sorted arrays efficiently holds paramount importance. This article serves as a comprehensive guide to unraveling the intricacies of various techniques employed in merging sorted arrays seamlessly. By exploring different algorithms and approaches, readers will gain a profound understanding of the processes involved, catering specifically to a diverse audience interested in programming, algorithms, and data structures. Understanding the efficient merging of sorted arrays is key to optimizing performance and enhancing the functionality of software applications.
Fundamentals Explained
At the core of merging two sorted arrays lie fundamental principles and theories that form the basis of this crucial operation in programming. The key terminology and definitions associated with array merging serve as the building blocks for comprehending the complexities of sorting and merging algorithms efficiently. It is essential to grasp the basic concepts and foundational knowledge surrounding sorted arrays to pave the way for delving deeper into the practical applications and intricate techniques discussed in this article.
Practical Applications and Examples
Real-world case studies and applications offer practical insights into the significance of implementing efficient array merging techniques. Demonstrations and hands-on projects provide tangible examples of how merging sorted arrays can streamline processes and improve software performance. By incorporating code snippets and implementation guidelines, readers can gain a hands-on understanding of applying different strategies to merge arrays effectively, ensuring the seamless integration of arrays in diverse programming scenarios.
Advanced Topics and Latest Trends
The evolution of array merging techniques continues to witness cutting-edge developments in the programming sphere. Advanced methodologies and techniques are spearheading the optimization of array manipulation processes, paving the way for enhanced performance and scalability. By exploring the latest trends in array merging, readers can stay abreast of the innovative advancements shaping the field, while also gaining insights into the future prospects and upcoming trends that are set to revolutionize the landscape of data structures and algorithms.
Tips and Resources for Further Learning
For readers seeking to delve deeper into the realm of efficient array merging, a selection of recommended books, courses, and online resources is provided. These resources serve as valuable tools for expanding one's knowledge and honing practical skills in implementing array merging techniques. Additionally, tools and software for practical usage are highlighted to assist readers in applying the acquired knowledge to real-world scenarios, thus consolidating their understanding and proficiency in merging two sorted arrays with optimal efficiency.
In the vast realm of programming and data structures, the ability to efficiently merge two sorted arrays stands as a crucial skill. This article delves into the intricate techniques and strategies that enable programmers to seamlessly amalgamate arrays in a sorted manner. By exploring different algorithms and approaches, readers will gain a comprehensive understanding of how to optimize the merging process and enhance overall performance, catering to a diverse audience interested in programming, algorithms, and data structures.
Overview of Merging Arrays
Definition of Merging Arrays
The concept of merging arrays entails combining two or more arrays into a single array while retaining the sorted order. This fundamental operation is widely used in various applications to manage data efficiently. The key characteristic of merging arrays lies in its ability to preserve the sorted nature of the input arrays, leading to a merged array that is also sorted. This ensures that the resulting array can be easily searched, traversed, or utilized in further processing steps. The definition of merging arrays serves as a foundational element in algorithm design, offering a strategic approach to handling array data effectively.
Purpose of Merging Two Sorted Arrays
The purpose of merging two sorted arrays revolves around streamlining the process of combining ordered data sets. By merging arrays that are already sorted, programmers can leverage this operation to quickly merge large datasets without the need for additional sorting procedures. The significance of this technique lies in its potential to improve algorithmic efficiency and reduce computational complexity. One of the key advantages of merging sorted arrays is the ability to achieve a merged array in linear time complexity. This makes it an optimum choice for scenarios where performance and speed are critical factors, presenting a reliable solution for array manipulation tasks.
Importance of Efficiency
Significance of Efficient Merging Algorithms
Efficient merging algorithms play a vital role in optimizing the merging process and enhancing performance. These algorithms are designed to minimize the number of comparisons and operations required to merge arrays successfully. The key characteristic of efficient merging algorithms is their ability to operate with reduced time complexity, enabling faster merging of large datasets. By implementing efficient merging techniques, programmers can significantly improve the overall efficiency of their algorithms and streamline data processing tasks.
Impact of Inefficient Merging on Performance
Conversely, inefficient merging can have detrimental effects on algorithmic performance and computational resources. When merging arrays using suboptimal algorithms or approaches, the resulting merged array may exhibit higher time complexity and increased space usage. This can lead to slower execution times, higher memory consumption, and overall degradation of system performance. The impact of inefficient merging on performance underscores the importance of utilizing strategies that prioritize efficiency and optimization. By understanding the repercussions of inefficient merging, programmers can make informed decisions to enhance the efficacy of their merging algorithms and mitigate performance bottlenecks.
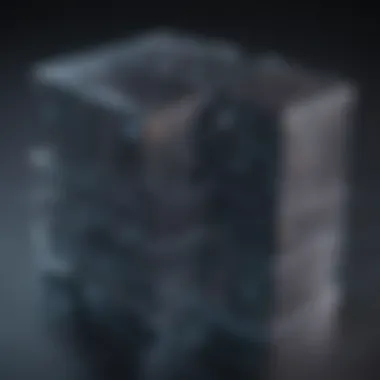
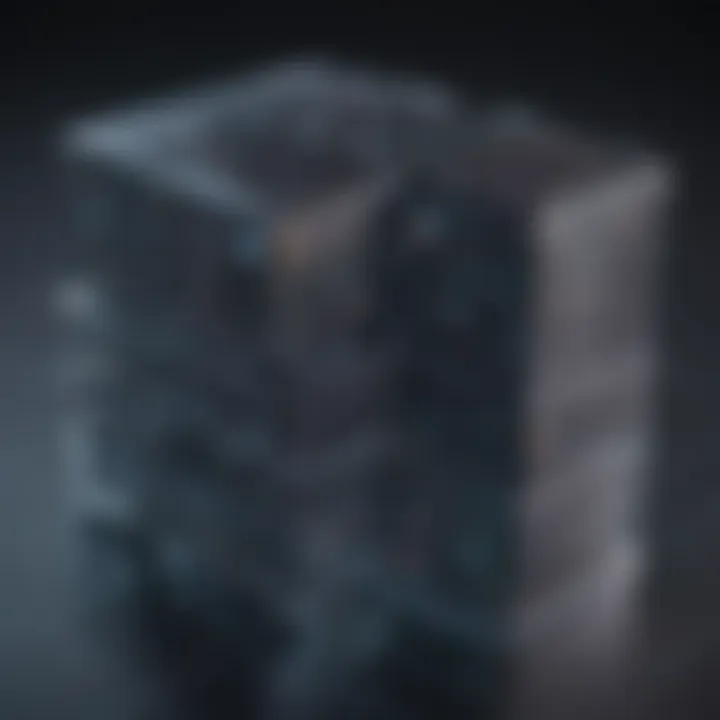
Naive Approach to Merge Arrays
In this section of the article, we delve into the significance of the Naive Approach to Merge Arrays, an essential topic that lays the foundation for understanding more efficient merging techniques. The Naive Approach serves as a fundamental starting point, introducing readers to basic merging concepts and strategies. By focusing on this method, individuals can grasp the core principles behind merging two sorted arrays, setting the stage for exploring more advanced approaches in the later sections.
Explanation of Naive Method
Iterating through arrays
When discussing the Iterating through arrays method, we spotlight the straightforward yet crucial process of sequentially accessing elements in both arrays for merging. This iterative approach plays a central role in basic merging algorithms, allowing us to compare elements from each array systematically. The key characteristic of Iterating through arrays lies in its simplicity and ease of implementation, making it a preferred choice for beginners or when simplicity is prioritized. While this method may lack the efficiency of more advanced techniques, its simplicity offers clarity in understanding the fundamental concepts of array merging.
Concatenation method
As we examine the Concatenation method, we explore the practice of appending one array to another to form a single merged array. This method involves joining the elements from both arrays, typically at the end of the initial array. The Concatenation method is favored for its intuitive nature and ease of comprehension, making it a popular choice for educational purposes or when quick solutions are needed. However, it is essential to note that this method may result in increased space complexity due to the creation of a new array, impacting the overall efficiency of the merging process.
Complexity Analysis
Time complexity
The evaluation of Time complexity focuses on analyzing the efficiency of merging algorithms concerning the time taken to complete the merging operation. By understanding the time complexity involved in array merging, programmers can assess the performance of different methods and optimize their code for faster execution. Time complexity plays a critical role in algorithm design, as it provides valuable insights into the scalability and efficiency of the merging process based on the input array sizes and characteristics. While prioritizing low time complexity is vital for enhancing merging performance, it is essential to balance this with other factors such as space complexity for optimal algorithm design.
Space complexity
On the other hand, Space complexity refers to the additional memory or storage requirements imposed by merging algorithms during the process. Efficiently managing space complexity is crucial for developing resource-efficient solutions, particularly when dealing with large datasets or limited memory constraints. By examining the space complexity of various merging techniques, we can identify opportunities to reduce auxiliary space usage and improve the overall efficiency of the merging process. While minimizing space complexity is desirable, it is essential to consider trade-offs with time complexity to achieve a balanced and optimized merging algorithm.
Efficient Techniques for Merging Arrays
Efficient Techniques for Merging Arrays play a pivotal role in this article as they lay the foundation for optimizing the process of merging two sorted arrays. By delving into various efficient techniques, readers will acquire an in-depth understanding of how to enhance the merging process for improved performance. The significance of Efficient Techniques for Merging Arrays lies in their ability to streamline the merging of arrays, resulting in faster and more resource-efficient operations. Considerations such as time complexity, space utilization, and algorithmic efficiency are key elements that make these techniques essential in the realm of programming and algorithm design.
Merge in Place Method
Concept of merging in place
The Concept of merging in place refers to the strategy of merging two sorted arrays without requiring additional auxiliary space. This approach optimizes memory usage and reduces the need for allocating extra storage, making it a valuable choice for this article. One key characteristic of merging in place is its ability to perform the merging operation directly on the input arrays without creating a new array. This unique feature eliminates the overhead of allocating space for a separate array, leading to efficient memory utilization. However, a notable disadvantage of this method is its complexity in implementation due to the need for careful manipulation of array elements and indices.
Implementation details
Implementation details of the merge in place method involve meticulous manipulation of array elements to achieve the desired merged output efficiently. By directly modifying the input arrays during the merging process, this method enhances the overall performance by avoiding excessive memory allocation. The key characteristic of this implementation lies in its ability to merge arrays in a space-efficient manner, benefiting the optimization of merging algorithms. Despite its advantages in memory management, the merge in place method may pose challenges in maintaining array coherence and managing indices effectively, requiring careful attention to detail and algorithmic precision.
Divide and Conquer Strategy
Splitting arrays for merging
The strategy of splitting arrays for merging involves dividing the input arrays into smaller subarrays to facilitate the merging process. By breaking down the arrays into manageable segments, this approach simplifies the merging operation and improves the efficiency of the overall algorithm. One key characteristic of splitting arrays for merging is its effectiveness in reducing the complexity of the merging task by dealing with smaller chunks of data at a time. This method's unique feature lies in its ability to tackle large arrays in a segmented manner, enabling a structured and systematic merging process. However, a potential disadvantage of this approach is the overhead associated with recursive splitting and merging, which may impact the algorithm's performance for extremely large arrays.
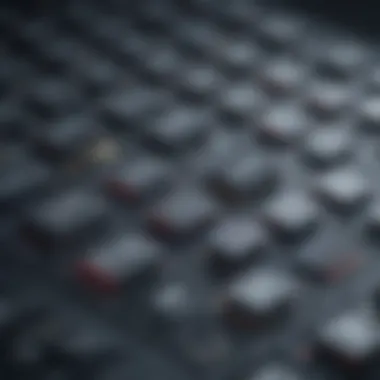
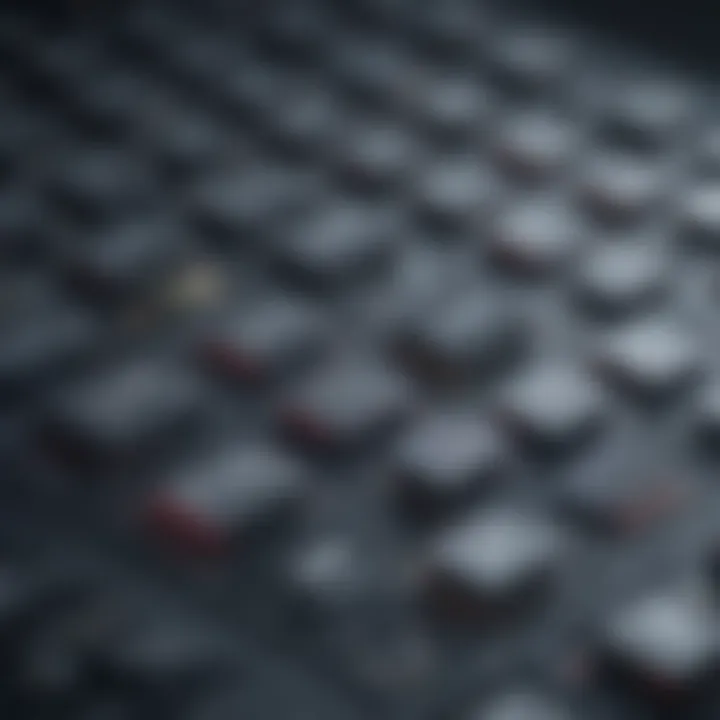
Recursive merging approach
The Recursive merging approach involves recursively merging subarrays until the entire array is reconstructed in a sorted manner. This strategy contributes to the overall goal of efficiently merging two sorted arrays by leveraging divide and conquer principles. The key characteristic of this approach is its ability to handle merging at different levels of array granularity, allowing for a comprehensive and systematic merging process. The unique feature of the recursive merging approach lies in its scalability and adaptability to varying array sizes, enhancing the algorithm's versatility. Despite its advantages in structuring the merging process, the recursive approach may introduce additional computational overhead due to the recursive function calls, requiring careful optimization to maintain efficiency.
Using Pointers for Merging
Pointer manipulation techniques
Pointer manipulation techniques involve leveraging pointers to traverse and manipulate elements in the input arrays during the merging process. By utilizing pointers effectively, this approach streamlines the merging operation and enhances the algorithm's efficiency. One key characteristic of pointer manipulation techniques is their ability to facilitate direct access to array elements, enabling swift and targeted merging actions. The unique feature of this approach lies in its capability to handle array elements with precision and flexibility, promoting optimized merging outcomes. However, a potential disadvantage of relying on pointers is the risk of pointer errors and memory leaks if not managed correctly, emphasizing the importance of meticulous pointer manipulation.
Benefits of pointer approach
The Benefits of the pointer approach include improved efficiency in merging operations and enhanced control over array manipulation. By employing pointers to navigate and merge arrays, this approach reduces the computational overhead associated with index-based merging techniques. One key characteristic of the pointer approach is its capacity to expedite the merging process by directly accessing and modifying array elements. The unique feature of using pointers for merging lies in its ability to optimize memory usage and reduce the need for additional data structures, leading to streamlined and resource-efficient merging algorithms. Despite its advantages in efficiency, the pointer approach requires careful handling to avoid pointer-related errors and ensure the integrity of the merged output.
Optimizing Merging Algorithms
Optimizing Merging Algorithms holds a pivotal role within the broader scope of this article, focusing on enhancing the efficiency and performance of merging two sorted arrays. By delving deep into optimization techniques, readers can grasp the intricate nuances of refining array merging processes, which are crucial in the realm of programming and data structures. This section presents a detailed analysis of various strategies to optimize merging algorithms, shedding light on the significance of minimizing space and time complexities to achieve optimal results.
Space Optimization
In-place merging techniques
In the context of this article, in-place merging techniques stand out as a strategic approach to streamline the merging process without requiring additional auxiliary space. This method revolves around manipulating array elements directly in the input arrays, thereby eliminating the need for a separate output array. The key characteristic of in-place merging lies in its ability to conserve memory resources while efficiently combining two sorted arrays. Despite its advantageous trait of reducing space complexity, implementing in-place merging techniques necessitates careful consideration of array indices and element shifting, ensuring accurate array rearrangement for successful merging. The unique feature of in-place merging techniques lies in their ability to merge arrays with minimal memory overhead, although this approach may pose challenges in maintaining array order and managing data coherence during the merging operation.
Reducing auxiliary space usage
Reducing auxiliary space usage plays a vital role in optimizing merging algorithms by minimizing the additional memory requirements during the merging process. This strategy focuses on leveraging the existing memory allocation for array manipulation, thereby curbing unnecessary space overhead. The prominent aspect of reducing auxiliary space lies in its capability to enhance the overall efficiency of array merging operations by eliminating the need for supplementary storage. By conserving memory usage, this approach contributes significantly to improving the performance of merging algorithms, especially when handling large datasets or memory-constrained environments. The distinctive feature of reducing auxiliary space usage lies in its optimal utilization of available memory resources, although it may introduce complexities in managing in-memory data structures and handling array modifications during merging tasks.
Time Complexity Enhancements
Minimizing comparisons
The essence of minimizing comparisons in merging algorithms lies in optimizing the computational efficiency by reducing the number of comparisons required to merge two sorted arrays. This technique focuses on streamlining the comparison operations between elements from distinct arrays, aiming to minimize the overall runtime complexity of the merging process. The fundamental characteristic of minimizing comparisons entails designing algorithmic strategies that exploit array order and inherent properties to diminish unnecessary element comparisons, thereby accelerating the merging operation. By limiting the number of comparisons, this approach accelerates the sorting process and enhances the overall performance of merging algorithms, offering a systematic framework to boost efficiency in array manipulation tasks. The unique aspect of minimizing comparisons is its ability to optimize algorithmic runtime by eliminating redundant comparisons, although this optimization may demand meticulous algorithm design and thorough analysis of array structures.
Enhancing overall performance
Enhancing overall performance in merging algorithms encompasses a multifaceted approach to boost the runtime efficiency and computational speed of sorting and merging tasks. This enhancement strategy prioritizes refining the entire merging operation, from initial array parsing to final element integration, to achieve superior performance levels. The key characteristic of enhancing overall performance focuses on optimizing algorithmic design through parallel processing, algorithmic pruning, or enhanced data structures to expedite the merging process. By leveraging advanced optimization techniques, this approach significantly accelerates the merging task and elevates the algorithmic efficiency, catering to scenarios where optimal speed and performance are paramount. The unique feature of enhancing overall performance lies in its ability to fine-tune algorithmic components for maximum computational speed, although this optimization may require intricate algorithm adjustments and thorough performance testing to validate the enhancements.
Real-World Applications
Database Operations
In the realm of database operations, the significance of merging query results efficiently cannot be overstated. This specialized capability plays a pivotal role in streamlining data retrieval processes and ensuring optimal query performance. By efficiently merging query results, organizations can enhance the speed and accuracy of database operations, leading to improved decision-making and resource utilization. This section delves deep into the nuances of merging query results efficiently, shedding light on its strategic importance and practical implications within database management.
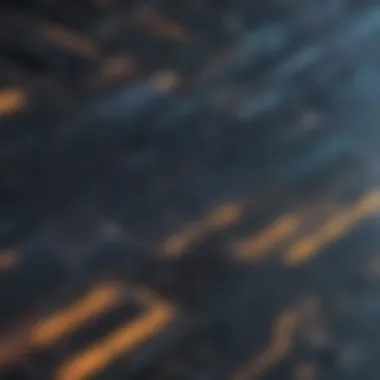
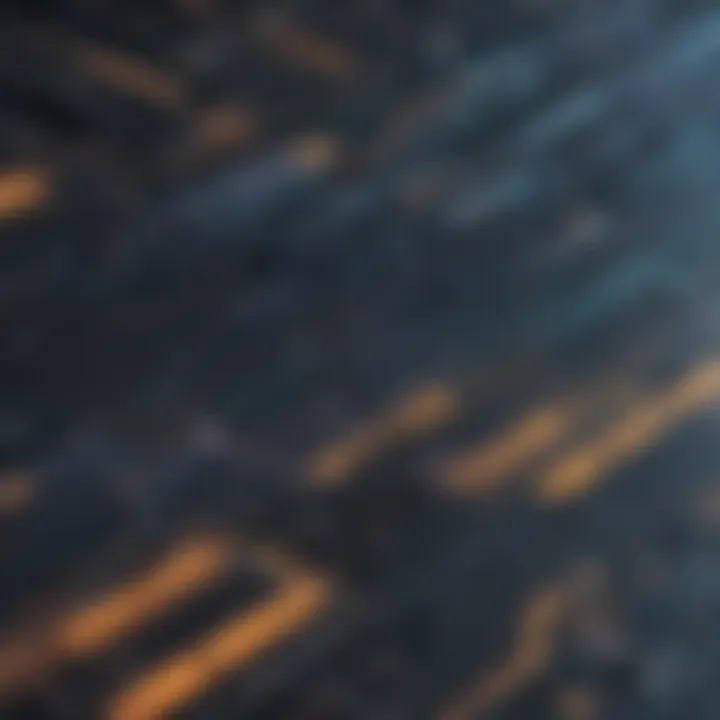
- Merging query results efficiently
Merging query results efficiently
The optimization of merging query results is a fundamental aspect of database optimization. Efficient merging ensures that data from different sources can be consolidated seamlessly, minimizing redundancy and maximizing retrieval speeds. By employing this technique, database administrators can expedite query processing and enhance overall system efficiency. The ability to merge query results efficiently is a cornerstone of efficient database management, offering a competitive edge in data-centric industries.
- Handling large datasets
Handling large datasets
Handling large datasets presents a unique set of challenges in data processing. Efficiently managing and merging these voluminous datasets require robust algorithms and optimal resource utilization. By addressing the complexities of handling large datasets, organizations can harness the power of data analytics and derive meaningful insights from massive information pools. This section explores the intricacies of handling large datasets, emphasizing the importance of scalability, performance, and data integrity in modern database environments.
Sorting Algorithms
Integrating merging techniques with sorting routines revolutionizes algorithmic efficiency and performance optimization. Sorting algorithms form the backbone of various computational tasks, and their integration with efficient merging strategies enhances both speed and accuracy. By exploring the synergy between sorting algorithms and merging methodologies, developers can unlock new possibilities in algorithm design and optimization, tailored for diverse application scenarios.
- Integration with sorting routines
Integration with sorting routines
The harmonious integration of merging techniques with sorting routines presents a paradigm shift in algorithmic design. By combining the strengths of sorting and merging algorithms, developers can expedite data processing operations, reduce computational complexities, and improve overall system performance. This seamless integration offers a holistic approach to algorithm optimization, showcasing the symbiotic relationship between different computational paradigms.
- Improving algorithmic efficiency
Improving algorithmic efficiency
Enhancing algorithmic efficiency through innovative merging approaches is paramount for competitive advantage in the digital era. By refining algorithms to prioritize efficiency and scalability, developers can elevate the performance benchmarks of software applications and computational systems. This section delves into the strategies for improving algorithmic efficiency through advanced merging techniques, illustrating how data structure optimizations can lead to exponential improvements in computational speed and resource utilization.
Conclusion
Summary of Merging Techniques
Recap of Efficient Merging Methods
Expounding on the recap of efficient merging methods, we dissect intricacies essential to the amalgamation of sorted arrays. This section articulates how the chosen methods offer streamlined processes and enhanced performance in merging arrays. Through meticulous analysis, we unravel the distinct characteristics that make these techniques paramount for optimizing array operations. While contemplating the advantages and disadvantages, it becomes apparent that these methods stand as pillars of efficiency in array merging endeavors.
Impact on Algorithm Design
Delving deeper, the impact on algorithm design sheds light on the transformative influence of merging techniques. By redefining conventional approaches, the design considerations outlined in this section navigate readers towards crafting algorithmic solutions with heightened precision and effectiveness. Unpacking the key elements driving algorithm design, we discern the compelling reasons behind adopting these innovative techniques. Evaluating the pros and cons, it becomes evident that embracing these design principles paves the way for algorithmic advancements and enhanced problem-solving capabilities.
Future Implications
Innovations in Merging Algorithms
Navigating towards future implications, the discourse around innovations in merging algorithms foreshadows exciting developments in array manipulation. Unveiling groundbreaking advancements, we explore how these innovations revolutionize the merging landscape, propelling efficiency to unprecedented levels. Delving into the unique features of these innovations, we uncover the tangible benefits they offer while acknowledging potential limitations. Embracing these innovative approaches opens doors to a realm of endless possibilities in algorithm optimization.
Advancements in Array Operations
The quest for advancements in array operations heralds a new era of computational efficiency and data processing capabilities. By dissecting the core characteristics of these advancements, we illuminate the pathways to refining array operations for maximum impact. Diving into the advantages and disadvantages of these advancements, we discern their pivotal role in shaping the future of array-centric tasks. Embracing these advancements equips practitioners with tools to navigate complex data landscapes with agility and precision.
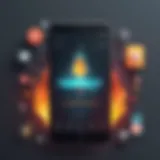
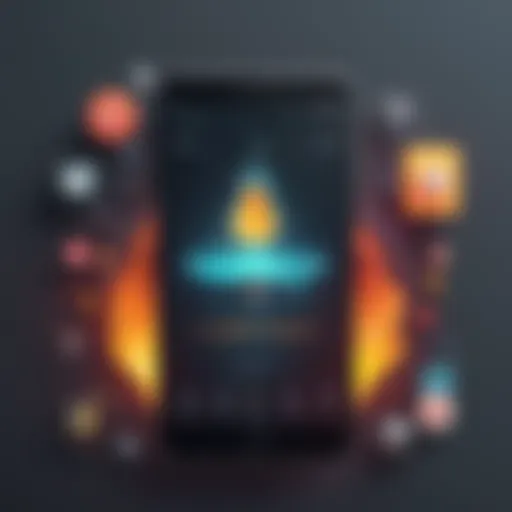