Understanding Entity Classes in Software Engineering
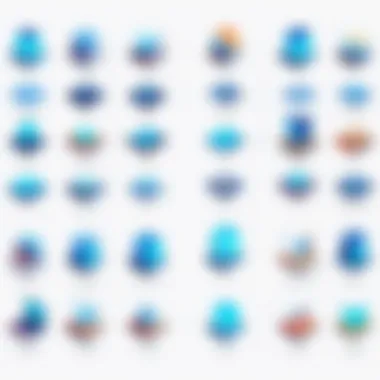
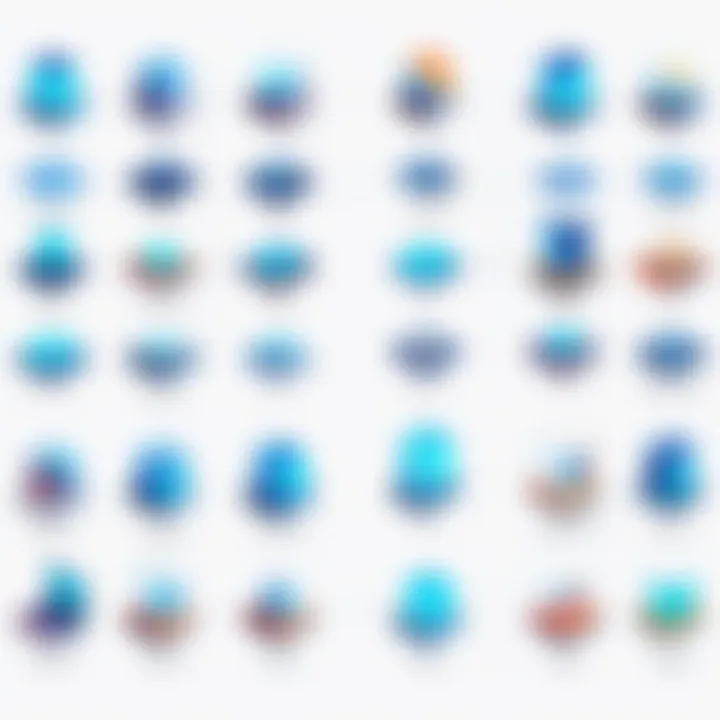
Overview of Topic
Prolusion to the main concept covered
Entity classes are foundational components in software engineering. These classes represent distinct objects or entities within a system, encapsulating their properties and behaviors. Understanding entity classes is essential, as they play a crucial role in object-oriented programming and data modeling.
Scope and significance in the tech industry
In contemporary software development, the relevance of entity classes cannot be overstated. They form the backbone of many applications, defining how data interacts within systems. Their proper implementation can lead to improved data integrity and functionality. Hence, software architects and developers must grasp their significance.
Brief history and evolution
The concept of entity classes emerged with the evolution of object-oriented programming in the 1980s. It evolved from early programming methodologies focusing on data structures to more complex paradigm shifting towards encapsulation and abstraction. This evolution reflects a broader shift in understanding software as a collection of interacting objects, rather than just lines of code.
Fundamentals Explained
Core principles and theories related to the topic
At the core of entity classes lie several principles, including abstraction, encapsulation, and inheritance. Abstraction allows developers to focus on essential characteristics while hiding unnecessary details. Encapsulation bundles data and methods, safeguarding the internal state of an object. Inheritance promotes code reuse, enabling new entities to be defined based on existing ones.
Key terminology and definitions
Understanding entity classes involves familiarity with specific terms:
- Entity: An object that exists independently and has a distinct identity.
- Attributes: The characteristics or properties of an entity.
- Methods: Functions or operations that define the behavior of an entity.
Basic concepts and foundational knowledge
An entity class typically comprises attributes and methods. Attributes store the state of an entity, while methods define how that state can be interacted with and modified. Entity classes can serve different purposes, including data transfer objects, persisting data, or providing business logic.
Practical Applications and Examples
Real-world case studies and applications
Entity classes are widely employed across various domains. For instance, in e-commerce platforms, classes might represent products, customers, and orders. Each class encapsulates relevant data and functionality, enabling clear separation of concerns.
Demonstrations and hands-on projects
In practice, developers often implement entity classes as part of a larger framework. For instance, using Java with Spring Framework enables the creation of entity classes that interact with databases through Object-Relational Mapping (ORM).
Code snippets and implementation guidelines
Here is an example of a basic entity class in Java:
Advanced Topics and Latest Trends
Cutting-edge developments in the field
New trends in software engineering emphasize microservices and serverless architectures. In these contexts, entity classes adapt to accommodate the decentralized nature of application design. Understanding how to integrate entity classes within these frameworks is crucial.
Advanced techniques and methodologies
Modeling techniques such as Domain-Driven Design (DDD) leverage entity classes to define core domain logic. This approach focuses on aligning the software design closely with the business needs.
Future prospects and upcoming trends
As technologies evolve, entity classes may integrate more with emerging paradigms like artificial intelligence and machine learning. Understanding data flow and management will be vital as these trends develop.
Tips and Resources for Further Learning
Recommended books, courses, and online resources
Several resources are beneficial for gaining deeper insights into entity classes. Consider reading "Domain-Driven Design" by Eric Evans for an in-depth understanding of modeling and design. Online platforms like Coursera and edX offer courses on software development and object-oriented programming.
Tools and software for practical usage
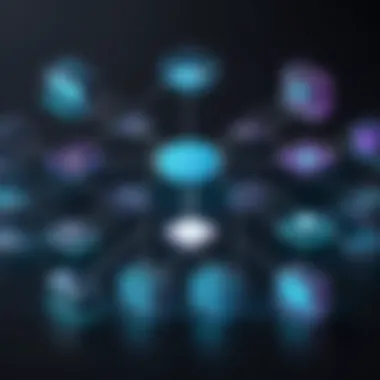
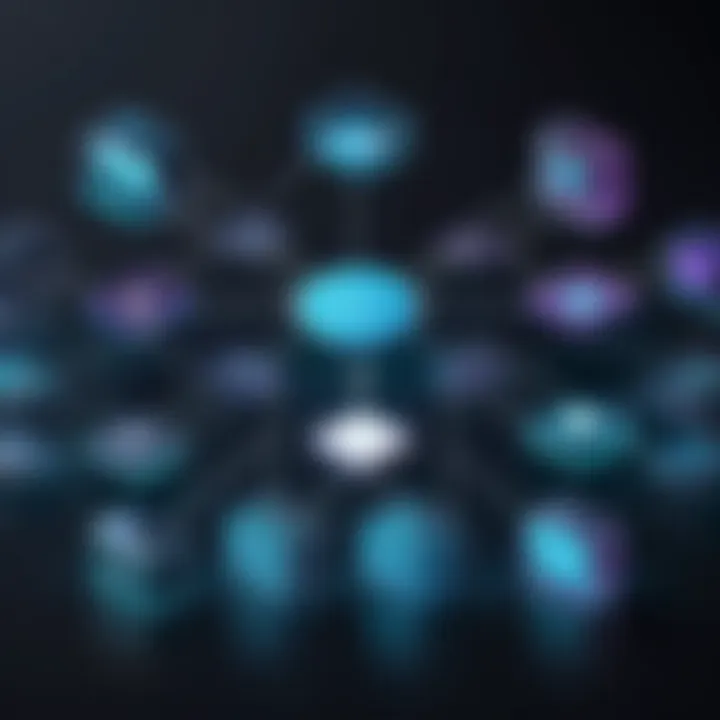
Using tools such as IntelliJ IDEA or Visual Studio can enhance the development experience. These Integrated Development Environments (IDEs) provide features that assist in creating and managing entity classes effectively.
Understanding entity classes is critical for developers. Their proper application can greatly influence project success.
Intro to Entity Classes
Entity classes represent a critical concept in software engineering. Their significance is particularly prominent in the realms of object-oriented programming and data modeling. Understanding entity classes helps to bridge the gap between real-world objects and the digital representations we utilize in software development. They encapsulate both the data and the behavior associated with these objects, serving as foundational blocks in designing complex systems.
The study of entity classes yields several benefits. First, they promote clarity in system architecture by providing a clear structure for handling data. Each entity class embodies specific attributes and methods that define its nature and behavior within the software. This encapsulation fosters modularity, making code easier to maintain and understand.
Furthermore, utilizing entity classes allows developers to create powerful data models. These models are essential for effective data manipulation and integrity, addressing how data interacts within a system. By establishing clear relationships through entity classes, software systems can maintain their reliability and ensure that data is consistently accurate.
For anyone working in software development or learning programming languages, grasping the concept of entity classes is vital. The capability to implement them correctly influences not just efficiency but also the overall success of a project. Therefore, it becomes imperative to delve deeper into what defines an entity class and how it operates within software engineering.
Characteristics of Entity Classes
Entity classes serve as a pivotal element in software engineering, particularly within the realms of object-oriented programming and data modeling. Understanding their characteristics lays the groundwork for effective software design and development.
Attributes
Attributes define the properties or characteristics of an entity class. They provide the essential data about the objects represented by the class. Properly defined attributes facilitate better data operations, enhancing both readability and maintainability. Attributes can vary widely depending on the purpose of the entity class. For instance:
- Name: A crucial attribute for user entity classes.
- Email: Often used in user profiles.
- Price: Might be an attribute in a product entity class.
By clearly delineating attributes, developers can improve database interaction and ensure smoother data handling processes. When attributes are appropriately categorized and typed, they contribute to data integrity and reduce errors during data access.
Methods
Methods within an entity class are functions that operate on the attributes or manipulate data related to the object. They encapsulate the behaviors of the class and perform operations relevant to the data stored. Key points regarding methods include:
- Getter and Setter Methods: These allow controlled access to private attributes, providing a way to retrieve and update values while enforcing data integrity.
- Business Logic Implementation: Methods may contain logic that dictates how data should be processed, ensuring the entity behaves as expected in various situations.
- Data Validation: Methods can be designed to validate data before it is set, preventing issues early in the data entry process.
Effective use of methods not only enhances functionality but also promotes a clear separation of concerns within the application, ensuring that classes maintain their intended responsibilities without unnecessary overlap.
Identifiers
Identifiers are crucial for distinguishing each instance of an entity class. They play a significant role in maintaining uniqueness and integrity within datasets. An identifier is typically a unique value assigned to an entity, such as:
- Primary Key: In databases, this ensures every record can be uniquely identified. For instance, a user ID might serve as the primary key for user classes.
- Composite Keys: Sometimes, a combination of multiple attributes may serve as an identifier, especially in complex relationships.
- Natural Keys: These are inherent attributes that can uniquely identify an entity, such as a Social Security Number for individuals.
By establishing clear and effective identifiers, developers ensure data integrity and support efficient data retrieval, which is essential for performance in larger systems.
Properly implemented characteristics of entity classes lead to a robust and efficient software architecture.
Role of Entity Classes in Software Development
Entity classes play a vital role in modern software development, particularly in relation to object-oriented programming (OOP) and data modeling. Understanding the functions and impact of entity classes can greatly influence the design and implementation of robust software systems.
Data Modeling
Data modeling is the backbone of software applications. Entity classes help in representing real-world entities within the software design. These classes encapsulate attributes and behaviors that are pertinent to the entities identified during the modeling phase. By using entity classes, developers can create a clear structure for how data interacts within the system.
For instance, in a banking application, the entity class may contain attributes such as , , and . Methods like or can define operations on these attributes. Utilizing entity classes in this manner allows for a cohesive data structure that accurately mirrors business processes and relationships.
Moreover, effective data modeling with entity classes enhances the understanding of both internal mechanisms and external data exchanges. It helps in reducing redundancy and supports normalization, key aspects of relational database management. As a result, it becomes easier to map out how entities relate to each other, which is crucial for understanding the overall system behavior.
Separation of Concerns
A fundamental principle in software engineering is the separation of concerns. This principle assists in managing complexity by isolating distinct sections of code. Entity classes contribute to this separation by clearly defining a specific domain model within the larger architecture. Each entity class represents a particular concern or aspect of the application.
For example, in an e-commerce platform, you might have entity classes such as , , and . Each of these classes focuses on a particular area, ensuring that changes in a class do not inadvertently affect others. This leads to improved maintainability and a more organized codebase. Consequently, developers can work on different parts of the system without extensive knowledge of all system components.
Enhancing Data Integrity
Data integrity is central to any application that manages important information, such as financial records or personal data. Entity classes enhance data integrity through well-defined attributes and constraints. These classes allow developers to enforce rules at the data level, which protects against invalid data entries.
Implementing entity classes alongside data validation methods contributes significantly to maintaining integrity. For instance, attributes like in a class can be subjected to constraints ensuring they meet a specific format. This not only prevents bad data entry but also ensures consistency throughout the application.
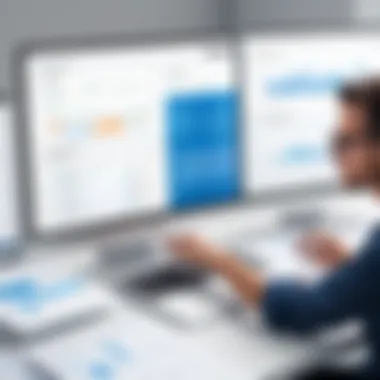
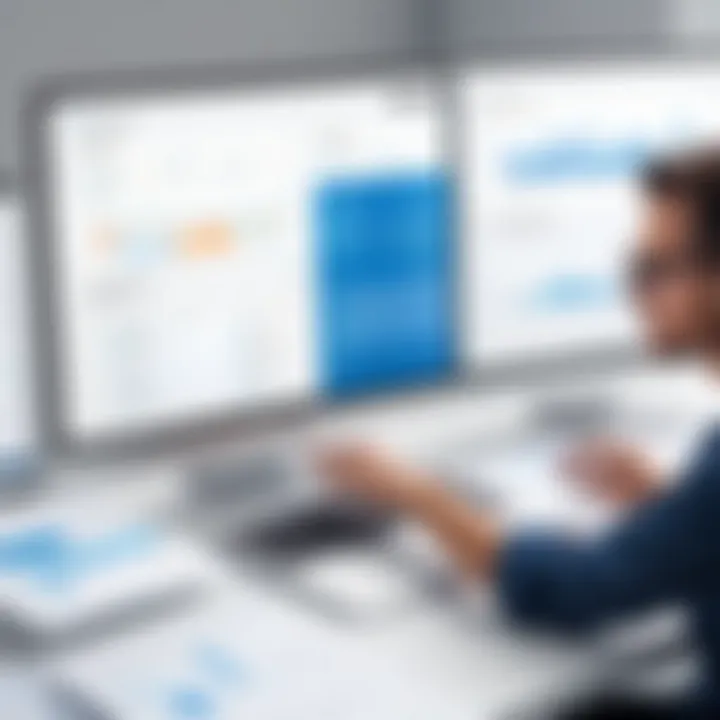
Additionally, entity classes facilitate easier updates and mental mapping of interdependencies. By having clear representations of data, it becomes simpler to track changes and understand impacts on related entities, fostering a trustworthy system architecture.
"Entity classes contribute significantly to clarity and functionality in software development, making systems adaptable and resilient against errors."
Implementing Entity Classes
Implementing entity classes is a central component in software engineering, particularly within object-oriented programming. These classes facilitate the structuring of data within a software application, allowing developers to effectively manage and manipulate information. Understanding this implementation is vital for software architects, developers, and IT professionals who seek to create a robust and scalable application architecture. Through effective implementation, entity classes can enhance code reuse, maintainability, and overall system integrity.
Object-Relational Mapping (ORM)
Object-Relational Mapping (ORM) is a technique used to convert data between incompatible type systems. ORM frameworks allow developers to interact with databases using higher-level programming constructs rather than traditional SQL. This abstraction simplifies database interactions, enabling a more intuitive approach to managing entity classes.
Popular ORM frameworks such as Hibernate for Java, Entity Framework for .NET, and Django ORM for Python serve to bridge the gap between the object-oriented model and the relational database model. The primary benefits of using ORM include:
- Improved Productivity: Developers can focus more on business logic rather than the intricate details of database management.
- Portability: Changes to the database schema often require minimal adjustments to the code, enhancing the software's adaptability.
- Consistency: ORM provides a consistent approach to data access, reducing the risk of errors.
However, developers must consider potential downsides. ORMs, while powerful, can introduce performance overhead. Understanding the specific requirements of the application is crucial to mitigating these concerns. Sometimes, raw SQL might be necessary for optimizing certain queries.
Integration with Databases
Integrating entity classes with databases is fundamental in data-centric applications. This integration allows for the seamless flow of information between the application logic and data storage solutions. The primary goal of this integration is to ensure that entity classes accurately reflect the structure of the underlying database, maintaining alignment between data models and stored data.
Key considerations include:
- Schema Synchronization: Changes in the entity class definitions must be accurately reflected in the database while preserving data integrity. Tools that automate schema migrations can be beneficial in this regard.
- Transaction Management: Managing transactions is crucial to ensure data consistency. Entity classes should support processes for committing and rolling back transactions as needed, safeguarding against data loss.
- Performance Optimization: Analyzing database queries generated by entity classes is essential to ensure optimal performance. This may involve indexing, reducing unnecessary joins, or optimizing fetch strategies.
Integrating entity classes with databases is a process that requires careful planning and implementation. Effective integration enhances application performance and reliability, which are crucial for the success of any software project.
Case Studies and Examples
The concept of entity classes is often better understood through concrete case studies and examples. These real-world applications and breakdowns of entity classes provide practical insights into how these structures operate within various software systems. Learning from these examples allows students, programming learners, and IT professionals to grasp the underlying principles and effective utilizations of entity classes in software engineering. Understanding this topic sharpens analytical skills and enhances design capabilities.
Real-World Applications
Real-world applications of entity classes showcase their versatility and pivotal role in modern software design. Here are some domains where entity classes make a difference:
- Customer Relationship Management (CRM): In systems like Salesforce, entity classes represent customers, leads, and opportunities. Each class can encapsulate different attributes and behaviors, enabling efficient management of customer interactions.
- E-Commerce Platforms: Amazon employs entity classes for handling products, users, and orders. The product entity might include attributes such as ID, name, price, and category, while methods could include adding items to cart or checking availability.
- Project Management Tools: Software like Trello uses entity classes to describe boards, lists, and cards. Each of these can have unique properties, helping users organize and track projects seamlessly.
These examples illustrate how entity classes align with user needs and enhance functionality across different applications. They simplify interactions and provide a clear framework that promotes smoother operations.
Entity Class in Different Programming Languages
The implementation of entity classes can differ based on the programming language used, each providing unique features and syntactics. Here are a few insights into how various languages approach entity classes:
- Java: In Java, entity classes are often implemented as Plain Old Java Objects (POJOs). This allows developers to use JavaBeans conventions. For instance, an entity class for a user may include get and set methods for fields like username and password, adhering to encapsulation principles.
- C#: Similar to Java, C# utilizes properties in entity classes. Here, a class representing an employee may define properties such as EmployeeId and Name, using backing fields to encapsulate the data elegantly.
- Python: Python employs classes for creating entity types typically without getter and setter methods. This flexibility allows for more straightforward syntax when defining attributes and behaviors. For example, a class for Book might have a method to display details more intuitively.
These variations demonstrate that while the concept of entity classes remains consistent, the implementation can be tailored to the language's strengths and community practices. Understanding these distinctions is beneficial for anyone aiming to adapt to different programming environments.
Challenges in Using Entity Classes
Entity classes play an essential role in software engineering, offering a structure for managing data in object-oriented programming. However, their implementation is not without challenges. Understanding these challenges is crucial for developers aiming to ensure the effectiveness and efficiency of their software systems.
Complexity in Large Systems
In large-scale applications, the complexity of entity classes can significantly increase. This is primarily due to the need for multiple interactions across different modules. As the number of entity classes grows, developers often face difficulties in managing relationships among them.
When designing entity classes, careful consideration must be given to the object relationships. For instance, one-to-many and many-to-many relationships can create a web of interdependencies that complicates data integrity and maintenance. Moreover, a misconfiguration in one entity can lead to cascading failures in others, which may result in data corruption.
The use of inheritance can further complicate this structure. While it is a powerful feature that allows for the reuse of code, it can lead to unexpected behavior, especially when the subclass changes the base class' behavior.
Additionally, the management of state can become problematic. Keeping track of changes, loading states, and managing database transactions can become overwhelming. To mitigate these issues, clear documentation and standardized procedures for interaction can help streamline processes.
Performance Considerations
Performance is another crucial factor when utilizing entity classes. Inefficiently designed entity classes can lead to performance bottlenecks. For instance, excessive loading of unnecessary attributes can slow down the application. Keeping track of multiple interrelated classes increases memory usage and can lead to longer processing times.
A common practice to address performance issues is using lazy loading, which delays data retrieval until it is necessary. However, this too can introduce complexities. If not handled properly, it can result in n+1 query issues, where the application issues a query for each associated record, significantly slowing down operations.
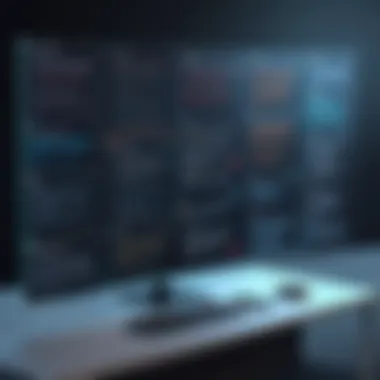
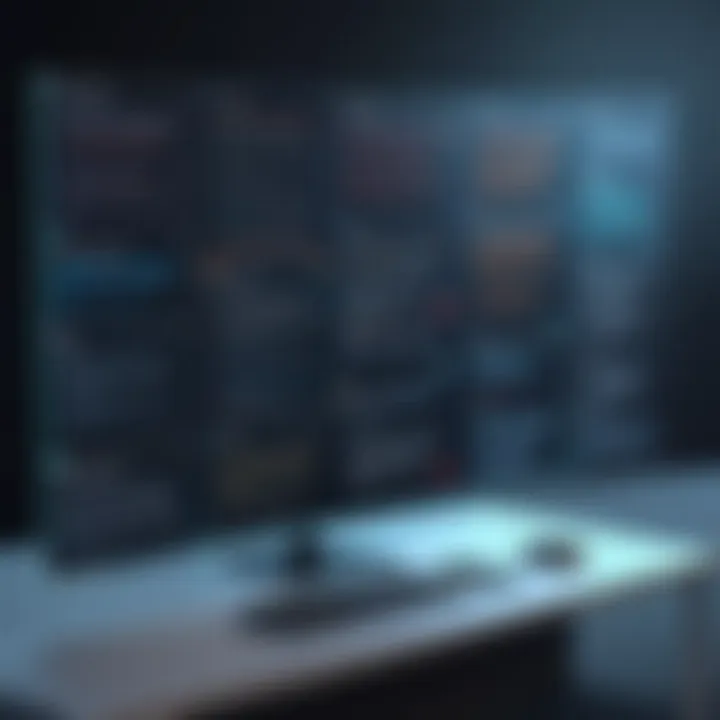
Moreover, entity classes often need to be optimized for database interactions. Frequent updates or reads from the database without an adequate caching strategy can degrade performance rapidly. Utilizing an effective Object-Relational Mapping (ORM) framework can help alleviate these concerns but requires proper configuration and understanding of its workings.
In summary, when working with entity classes, one must be aware of the complexities they bring, especially in large systems and performance implications. By addressing these challenges head-on, developers can establish a more solid foundation for their software applications.
"Proper management of complexity and performance is essential to the success of entity classes in any software project."
Understanding and strategizing around these challenges ensures that entity classes remain robust components of software engineering.
Best Practices for Working with Entity Classes
Working with entity classes effectively is vital for ensuring the quality and maintainability of software systems. These best practices create a framework that allows developers to leverage entity classes to their fullest potential, enhancing both structure and performance. Following these principles is not just a recommendation; it is essential for coding that stands the test of time amid evolving technologies.
Design Principles
When creating entity classes, adhere to several key design principles to facilitate clarity and efficiency:
- Single Responsibility Principle: Each entity class should focus on a single purpose or area of concern. By maintaining a single responsibility, developers can reduce the risk of unintended side effects during modification.
- Encapsulation: Encapsulate attributes and behaviors within the entity class. This protects the internal state and promotes better control over data. Consumers of the class should interact through well-defined interfaces rather than directly accessing the inner workings of the class.
- Clear Naming Conventions: Use descriptive and consistent naming for entity classes and their attributes. When the purpose of a class is clear from the name, it reduces cognitive load for other developers who work with the code.
- Follow Domain-Driven Design: Align entity classes with the business domain. When entity classes are representative of real-world concepts, they become more meaningful, reducing misunderstandings during development.
- Limit Complexity: Avoid adding excessive logic to entity classes. They should primarily serve as data containers. Complex behaviors or business logic are better suited for service classes or utility functions, keeping entity classes focused and manageable.
Adopting these design principles not only improves readability and maintainability but also fosters collaboration in teams. Developers can easily grasp the purpose and functionality of classes that adhere to these principles.
Testing and Validation
Testing and validating entity classes is a critical aspect of development. Rigorous testing ensures that these core building blocks function correctly under various scenarios. Consider the following strategies:
- Unit Testing: Each entity class should have its unit tests. These tests verify individual methods and behaviors. Using frameworks like JUnit for Java or NUnit for .NET can facilitate structured and automated testing.
- Test for Valid States: Ensure that the entity class can only be instantiated in valid states. Use validation methods to check for required fields and valid data types. Throw exceptions for invalid cases to enforce integrity.
- Mock Dependencies: When testing entity classes that rely on external services or databases, use mocking libraries to simulate those dependencies. This isolates the class being tested, ensuring that tests assess the class's behavior independently.
- Performance Testing: Conduct performance tests as your application grows. Monitor how entity classes react under stress and evaluate the impact on overall application performance.
- Continuous Integration (CI): Implement CI pipelines that automatically run tests when new code is committed. This prevents defects from compounding over time and allows for rapid feedback on code changes.
By implementing these testing and validation strategies, developers can detect issues early, maintain data integrity, and ensure that their codebase remains resilient.
Key Takeaway: Best practices in working with entity classes significantly contribute to software quality. Following design principles and establishing robust testing processes are fundamental.
These best practices not only serve as guidelines but elevate the entire development process. By investing time in adhering to these practices, developers can ensure a successful and sustainable software development lifecycle.
Future Trends in Entity Class Utilization
The landscape of software engineering is continually evolving. As the technology around us changes, so does the way we approach entity classes. Their utilization will increasingly become tied to advancements in cloud computing and shifts in software architecture. Understanding these trends is essential for professionals and students alike, as they shape how we develop, implement, and manage software solutions.
Impacts of Cloud Computing
The emergence of cloud computing has created a significant impact on how entity classes are utilized in software design. In traditional setups, entity classes were often tightly coupled with physical databases, making changes challenging and sometimes error-prone. However, with cloud services like Amazon Web Services, Microsoft Azure, and Google Cloud Platform, developers now have more flexibility.
Cloud computing enables scalable solutions that can adapt as applications grow. Here are some key aspects:
- Scalability: Entity classes can be designed to expand dynamically with increased demand. This adaptability allows for a more efficient use of resources.
- Microservices Architecture: Cloud environments often utilize microservices. It promotes smaller, focused entity classes that serve specific functions, rather than large, monolithic structures.
- Data Distribution: Cloud services allow for data to be distributed across multiple locations. Therefore, entity classes must be able to handle data consistency and integrity across these locations.
These changes necessitate a reevaluation of design practices. As entity classes become more distributed, understanding how they interact with other components is critical.
Evolving Software Architectures
As software architectures evolve, particularly with the rise of agile methodologies and continuous integration, so do the roles of entity classes. The trends indicate a shift towards more modular and responsive systems. Key points to consider include:
- Decoupling: Evolving architectures promote the separation of concerns, where entity classes can operate independently of client applications. This reduces the risk of tight coupling and aids in maintainability.
- API Utilization: Many modern applications leverage APIs. Entity classes are required to interface neatly with these APIs to ensure data and services remain accessible.
- Cross-Platform Integration: With the increasing need for cross-platform applications, the design of entity classes must accommodate various environments, ensuring consistency in data representation.
"The ability to adapt entity classes in response to architectural changes will dictate the success of future software projects."
Understanding these trends allows developers to stay ahead in a competitive field. It also paves the way for better collaboration and innovation in software development practices. As students and professionals encounter these shifts, being aware of their implications will make a substantial difference in their work.
Finale
The conclusion of this article serves as a vital section by summing up the primary insights regarding entity classes in software engineering. It captures the core principles discussed throughout the sections, reinforcing the importance of understanding entity classes in different contexts like software development, object-oriented programming, and data integrity.
Throughout this article, we have explored the definition, characteristics, implementation, challenges, and best practices associated with entity classes. Each of these facets contributes to a more in-depth understanding of why entity classes are critical in modern software design. Particularly, the role of entity classes assists in organizing data effectively within applications, ensuring maintainability and flexibility.
Here are the key points highlighted in this article:
- Definition and Characteristics: Entity classes serve as blueprints for creating objects that represent real-world entities, encapsulating attributes and behaviors.
- Role in Development: They play a crucial role in data modeling and maintaining separation of concerns, which slim down complexity in software projects.
- Implementation Details: The connection between entity classes and databases through Object-Relational Mapping (ORM) tools enhances data manipulation efficiencies.
- Future Trends: We discussed how cloud computing and evolving software architectures are shaping the future utilization of entity classes.
Summarizing Key Points
- Entity classes are foundational elements in software systems, defining the structure and behavior of the data.
- They enhance data integrity and support clear software architecture by separating concerns.
- Understanding their implementation and challenges can guide developers in creating better software practices.
- Awareness of future trends shows how adaptable and relevant entity classes remain in changing technological landscapes.
Final Thoughts
Entity classes represent a cornerstone of software engineering that evolves just as the domains of technology do. Mastery of this concept not only aids in developing competent applications but also in understanding the broader implications of software systems.
As new technologies emerge and development practices evolve, the significance of entity classes will continue to be apparent. Developers, students, and IT professionals should strive to deepen their understanding of this topic. Doing so prepares them for the future of programming and data management. In summary, appreciating the role and nuances of entity classes can lead to more effective software solutions and fruitful careers in software engineering.