Essential Angular Interview Questions for Beginners
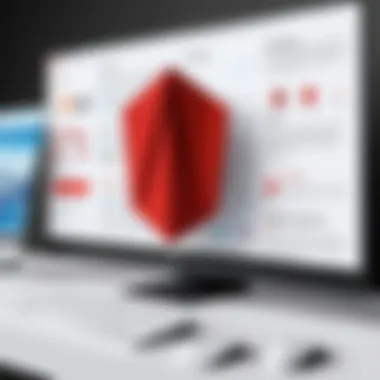
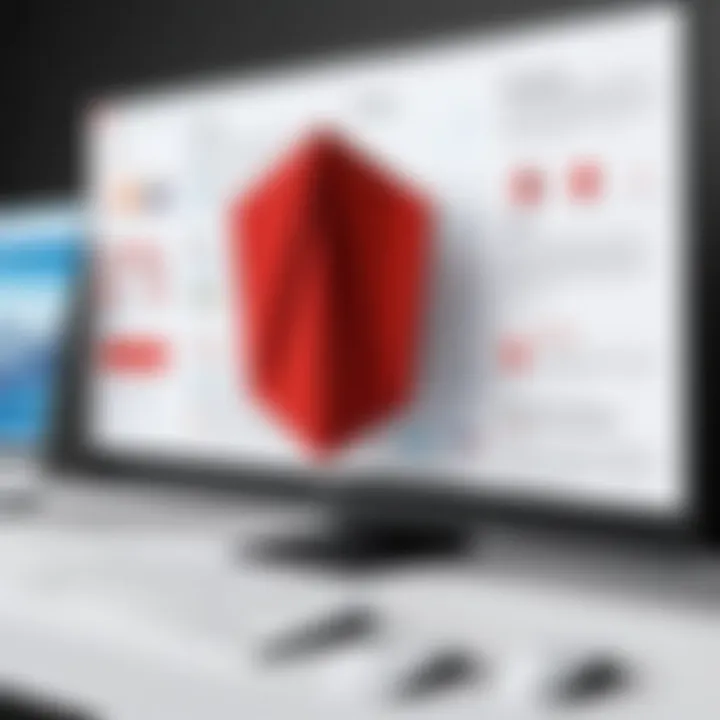
Overview of Topic
Angular is a robust framework designed for building dynamic web applications. This section introduces the main concepts behind Angular and explores its significance in the tech industry. The popularity of Angular stems from its powerful capabilities and strong community support, making it a favored choice among developers.
The framework was first released in 2010 by Google. Since then, Angular has evolved through a series of iterations, each aiming to improve usability and performance. Initially, it started as AngularJS, which introduced the concept of two-way data binding, component-based architecture, and dependency injection. The transformation into Angular 2, and further updates, have cemented its role as a leading solution in modern web development.
Fundamentals Explained
To understand Angular, it is essential to grasp its core principles. One fundamental concept is the concept of components. Components are the building blocks of any Angular application, encapsulating functionality and UI elements into reusable segments. Each component is governed by its own HTML template and a TypeScript class.
Key terminology includes:
- Modules: Containers for grouping components, directives, and services. They help organize an application into cohesive blocks.
- Services: Classes that handle data and logic. They can be injected into components for shared functionalities or data management.
- Dependency Injection: A design pattern that improves code reusability and maintainability by allowing components to request services rather than creating them.
A basic understanding of these concepts is crucial for anyone preparing for an Angular interview.
Practical Applications and Examples
Angular is used in various applications across different sectors. For instance, the framework powers platforms like Google Cloud Console and Microsoft Office online. These applications utilize Angular’s capabilities for dynamic changes in user interface driven by application logic.
A practical application could be to create a simple to-do application. This project allows users to add, delete, and view tasks. Below is a simplified code snippet demonstrating how a component might be structured:
This example highlights how Angular’s template syntax and event binding work together to create an interactive experience.
Tips and Resources for Further Learning
For those looking to deepen their understanding of Angular, several resources can help:
- Books: "Angular Up & Running" by Shyam Seshadri offers clear examples and practical projects.
- Courses: Online platforms like Coursera and Udemy provide comprehensive Angular courses tailored for various learning levels.
- Online Resources: The official Angular documentation (angular.io) is an invaluable resource for learning the intricacies of the framework.
Tools like Angular CLI can also streamline the development process, providing many features like code scaffolding and testing utilities.
These resources equip developers with the knowledge needed to excel in Angular interviews and enhance their practical skills.
Preface to Angular
Understanding Angular is vital for anyone looking to excel in modern web development. Angular serves as a platform for developing dynamic web applications with a modular structure. By introducing concepts like components, services, and dependency injection, it allows developers to create scalable, maintainable applications. Mastering this framework can provide significant advantages in job interviews and real-world projects.
What is Angular?
Angular is a robust framework maintained by Google. It facilitates the development of client-side applications, primarily using TypeScript. Angular promotes the use of a component-based architecture. This structure is crucial in building encapsulated components that can be reused throughout the application. When developers use Angular, they benefit from its two-way data binding, routing, and built-in services, among other features. Angular’s tools and libraries enable the creation of highly interactive user interfaces, distilling complexity into manageable parts. This way, Angular fosters an organized approach, crucial for large applications.
History of Angular
Angular has a rich history that began with AngularJS in 2010. It was designed to simplify the process of building single-page applications. AngularJS revolutionized the way developers worked with JavaScript by introducing features like dependency injection and directives. However, with the increasing complexity of web applications, a complete rewrite of AngularJS led to the creation of Angular (also known as Angular 2 and beyond) in 2016. This new iteration moved away from JavaScript to TypeScript, providing better tooling and type safety. Since then, Angular has steadily evolved, with regular updates improving performance, security, and developer experience. Understanding its evolution helps developers appreciate the framework's capabilities and adaptability.
Key Features of Angular
Angular comes with several standout features that enhance its utility for developers:
- Component-Based Architecture: Facilitates the organization of code into reusable components.
- Two-Way Data Binding: Ensures automatic synchronization between the model and the view.
- Dependency Injection: Promotes the development of scalable applications through modular services.
- Routing: Simplifies navigation within applications, enabling a single-page experience.
- Built-In Directives: Allows for easy manipulation of the DOM, enhancing interactivity.
These features combine to provide a comprehensive toolkit for developers aiming to build sophisticated web applications. By leveraging Angular's capabilities, programmers can create applications that are not only functional but also maintainable and efficient.
Understanding Angular equips developers with the skills needed to tackle complex applications with confidence.
Angular Architecture
Angular Architecture is a central element in understanding how Angular applications are structured and function. It defines how different components interact with each other, allowing developers to create rich, dynamic web applications. A firm grasp of Angular architecture can provide significant benefits, especially during interviews where candidates must demonstrate their knowledge of APIS, data flow, and user interaction. It encompasses three main components: components, modules, and services, each of which plays a crucial role in the overall framework.
Components and Templates
Components are the building blocks of an Angular application. Each component consists of two main parts: the TypeScript class and the HTML template. The class handles the logic and functionality, while the template defines the user interface.
The significance of components cannot be overstated. They allow for encapsulation of the application logic and the view, promoting reusability and maintainability. When you modify a component, changes propagate automatically through the interconnected templates, ensuring that the user interface remains synchronized with the data model.
Building a component in Angular involves defining its selector, template, and styles. The selector allows the component to be embedded in HTML. A typical component definition looks like this:
In this example, the selector can be placed within an HTML document to render the component. This establishes a straightforward yet powerful way to create complex user interfaces.
Modules in Angular
Modules serve as containers for a cohesive block of code dedicated to a specific functionality. In Angular, modules help organize the application’s structure, allowing for a segmented approach to development. A typical Angular application will have at least one module: the root module, often named AppModule.
The role of modules includes:
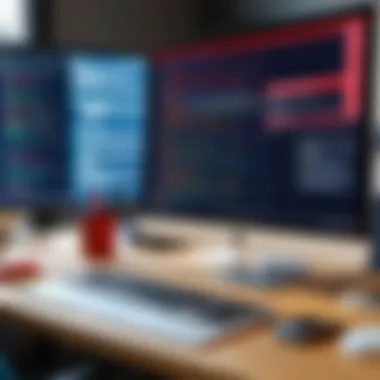
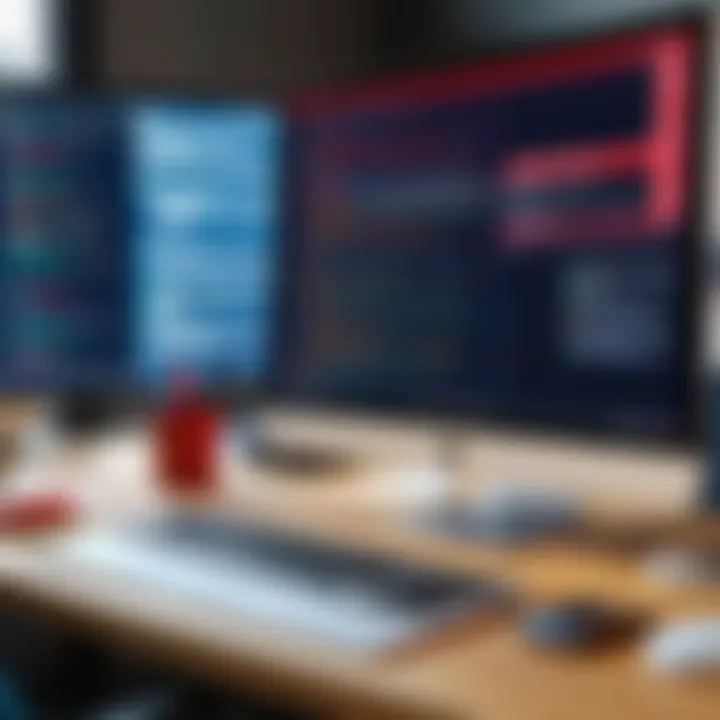
- Grouping related components.
- Managing dependencies.
- Enabling lazy loading, which can enhance performance by loading modules only when required.
Defining a module involves using the decorator, where you specify components, directives, pipes, and providers associated with that module. Here’s a basic example:
This structure demonstrates how modules can keep related code organized and modular, enhancing the maintainability of the application.
Services and Dependency Injection
Services in Angular provide a way to share data and business logic across components. They encapsulate common tasks, such as fetching data from a server or managing user authentication, thereby promoting code reusability and separation of concerns.
Dependency Injection is a design pattern utilized in Angular to manage the instances of these services. Instead of creating service instances within components, Angular utilizes injectors to provide them. This mechanism reduces tight coupling and makes testing components much easier.
For example, you can create a simple service to fetch data:
In this instance, the service is declared with , allowing Angular to manage its lifecycle and inject it wherever needed.
Data Binding in Angular
Data binding is a fundamental concept in Angular, acting as the vital bridge between the component's data and the UI. The mechanism of data binding provides a dynamic method for updating the view when the model changes, and vice versa. In the context of this article, understanding data binding is essential for mastering how Angular applications work. Without it, developers would find it challenging to create responsive interfaces or manage state effectively.
By integrating data binding, Angular facilitates better performance and more efficient rendering of UI elements, ensuring that updates can reflect in real-time. This will not just improve user experience but also streamline the development process for programmers, making it a critical area to grasp in interviews.
Understanding Data Binding
Data binding in Angular can be broadly defined as the technique of synchronizing data between the model and the view. It plays a crucial role in achieving a clean separation between the UI and business logic.
There are two primary ways the data can flow: one-way and two-way data binding. One-way data binding allows data to flow only from the model to the view, while two-way data binding permits data to flow both ways, ensuring that changes in the view will update the model and vice versa.
Types of Data Binding
Knowing the different types of data binding is essential for any Angular developer. Each type of binding serves distinct purposes and is suitable in various scenarios.
One-way Data Binding
One-way data binding focuses on passing information strictly from the component to the view. This type is beneficial because it minimizes the complexity associated with updating the model. The key characteristic of one-way data binding is its unidirectional flow. When the model changes, the view reflects those changes, but any user interaction does not alter the model directly.
The fundamental advantage of using one-way data binding relates to its predictability; developers can easily debug the flow of data since it travels in a single direction. For example, when rendering a list of items, changes made to the list in the component automatically refresh the display without requiring complicated logic.
However, a disadvantage of one-way data binding is that it can lead to more boilerplate code when developers need to handle user interactions separately, requiring additional mechanisms to update the model based on view interactions.
Two-way Data Binding
Two-way data binding stands out due to its ability to synchronize the component and the view seamlessly. Both changes in the view and the model reflect each other instantly, ensuring that developers do not have to write additional code to handle data updates.
The key characteristic of two-way data binding is its reactive nature. When a user modifies a form input, for instance, these changes immediately update the component's state. This makes it a strong choice for scenarios that involve user inputs, such as forms and complex interfaces, where inputs need to be reflected instantly on the model.
A primary advantage of this method is its ease of management when dealing with forms and similar applications. However, it can introduce complexities in larger applications, where data flow may need to be tracked more rigorously to avoid performance hits or unexpected behaviors.
Directives in Angular
Directives in Angular serve as one of the essential building blocks of the framework. Their importance cannot be overstated as they allow for the manipulation of the DOM in a powerful yet straightforward way. Understanding directives not only helps in building dynamic and interactive web applications but also prepares developers for addressing specific challenges during interviews.
Directives provide a means to extend HTML's capabilities. While HTML establishes the skeleton of web applications, directives inject logic and behavior that enable a more engaging user experience. They form the core of Angular's architecture, influencing how components behave and interact within your application.
What are Directives?
Directives in Angular are specialized markers on a DOM element. They give instructions to Angular's compiler and allow the application to add behavior to the elements in a template. These directives can change the structure, appearance, or behavior of the DOM. There are three main types of directives in Angular:
- Components - These are directives that use a template.
- Structural Directives - These alter the layout by adding or removing DOM elements.
- Attribute Directives - These change the appearance or behavior of an existing DOM element.
Directives play a pivotal role in achieving modularity and reusability in Angular applications.
Types of Directives
Angular differentiates directives into two main types: Structural Directives and Attribute Directives. Both serve unique purposes and have distinct functionalities.
Structural Directives
Structural directives are responsible for altering the DOM layout by adding or removing elements. The most well-known examples of structural directives in Angular are , , and . The key characteristic of structural directives is their ability to manipulate the view dynamically based on conditions or data.
Their contribution to the development process is significant. By offering a streamlined way to control the visibility and repetition of elements, structural directives help in reducing boilerplate code and enhancing application maintainability. One of the main advantages is that they utilize Angular's change detection mechanism efficiently, ensuring that any modifications reflect appropriately in the UI. However, overuse can lead to excessive complexity, which may affect performance if not managed carefully.
Attribute Directives
Attribute directives, on the other hand, enhance and modify the behavior or appearance of existing DOM elements. Common examples include and . The primary focus of attribute directives is to apply specific attributes or classes based on dynamic data.
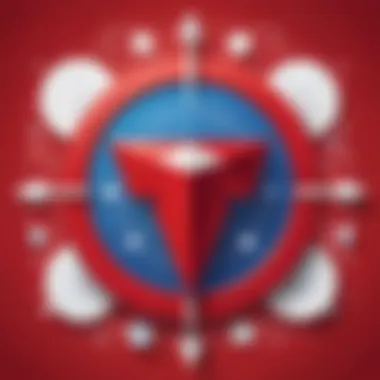
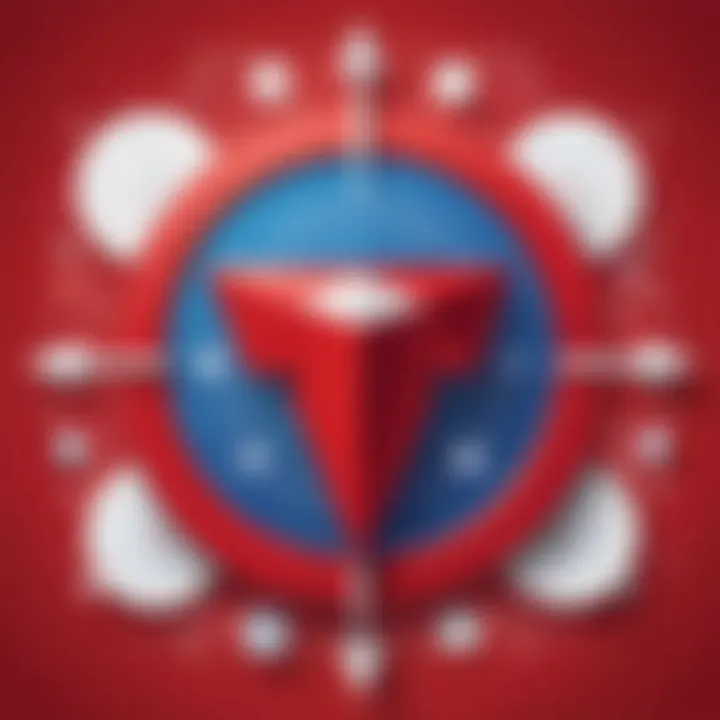
These directives are instrumental in improving user interface interactions without changing the structural integrity of the view. They allow for better specialization of components, making it easier to manage their individual behavior. A notable advantage of using attribute directives is their ability to create reusable logic that can be shared across various components. However, they may introduce dependencies that can complicate testing and maintenance if not designed thoughtfully.
Routing and Navigation
Routing and navigation are crucial components of Angular, as they define how users interact with the application while moving between different views or pages. Given that most web applications have multiple views, it is essential for developers to understand the mechanics of Angular's routing system.
Well-structured navigation enhances user experience, making it smoother and more intuitive. It allows users to easily explore an application without needing to refresh the page, keeping their context intact. Moreover, a proper routing setup is essential for the efficiency of Angular applications. It helps manage state and improves performance by loading only the required modules.
Understanding Angular Router
The Angular Router is a powerful library that facilitates the creating of single-page applications (SPAs) in Angular. It is responsible for interpreting the URL and displaying the associated component without reloading the page. This means that as users click on links within the application, the router dynamically loads and displays the appropriate views.
By using the router, developers can define routes and associate them with specific components. They can also manage navigation, which includes redirecting users based on various conditions. The router can handle both browser URL paths and internal navigation, offering flexibility to developers.
Defining Routes
Defining routes in Angular is a straightforward process that involves setting up an array of route objects. Each route object consists of properties such as a , a , and optional parameters for additional configurations. For example, a simple route configuration may look like this:
In this example, navigating to will display the , and navigating to will display the . Developers can also use route parameters to pass dynamic values in the URL. For instance, a user profile route can be defined like this:
This will allow the application to fetch the user data based on the provided ID in the URL.
Route Guards
Route guards play a vital role in maintaining control over navigation within an Angular application. They can prevent unauthorized access to certain routes or redirect users based on specific conditions. There are several types of guards:
- CanActivate: Checks if a route can be activated based on conditions such as user authentication.
- CanDeactivate: Determines if a user can leave the current route, potentially warning them about unsaved changes.
- Resolve: Pre-fetches data before a route is activated, ensuring the component has all necessary information at load time.
Using guards can greatly enhance security and improve user experience by ensuring that users only access appropriate content. This is especially important in applications that handle sensitive data or require user login.
"A well-implemented routing and navigation system can significantly enhance an application's usability, fostering higher user engagement and satisfaction."
Forms in Angular
Forms are a critical aspect of web applications developed with Angular. They facilitate user interaction and enable the capture of user inputs. In Angular, handling forms can be performed in two primary ways: Reactive Forms and Template-driven Forms. Understanding the nuances and benefits of each form handling technique is essential for any developer working within the Angular framework.
Utilizing forms effectively contributes to a better user experience and provides an efficient means of data validation and submission. Also, Angular's Form module provides a user-friendly approach to managing complex forms and associated validations, which is vital in many applications.
Reactive Forms vs Template-driven Forms
Angular offers two distinct approaches to creating forms: Reactive Forms and Template-driven Forms. Both approaches have unique characteristics, advantages, and use cases.
- Reactive Forms offer a more programmatic way to handle forms, emphasizing structured form management. They are class-based and enable direct control over the form model, making them suitable for complex validation scenarios and dynamic form generation. This approach uses the , , and classes to create and manage the state of the form.
- Template-driven Forms, on the other hand, provide a simpler syntax, relying on the Angular template syntax for creation. This approach is ideal for simpler forms, where the focus is less on programmatic control. It employs directives like to bind data directly to form inputs. Template-driven forms require less code and can be easier for beginners to understand initially.
Both methods have their place in Angular, depending on the complexity of the forms and the specific requirements of the application. Understanding when to use each type is crucial for optimal performance and maintainability of the code.
Validating Forms
Validating forms is essential for ensuring data integrity and providing immediate feedback to users. Angular provides a robust validation mechanism that can easily integrate with both Reactive and Template-driven Forms. Here are significant areas to consider:
- Built-in Validators: Angular comes with several built-in validators, such as , , and . Applying these validators is straightforward and can provide immediate feedback based on user input.
- Custom Validators: In many scenarios, the built-in validators may not meet specific application needs. Developers can create custom validators tailored to specific fields or requirements. These custom validators can validate any complex form logic that is beyond the capabilities of built-in options.
- Asynchronous Validators: These are essential for checks that require server-side validation, such as verifying whether a username is already taken. They work similarly to synchronous validators but return an observable instead.
- Form State Management: Tracking the form's state is necessary to provide users with feedback on whether their input is valid. Angular allows developers to check the form state using properties like , , and . This facilitates conditional styling and messaging depending on the user's interaction with the form.
Pipes in Angular
Pipes are a powerful feature in Angular that can transform data in templates, making them essential for displaying data in a user-friendly manner. By using pipes, developers can manipulate and format data based on the requirements of their applications, which enhances the overall user experience. Understanding pipes is crucial for any developer working with Angular, as they simplify how data is presented and interacted with.
There are key benefits to utilizing pipes in your Angular applications. First, they promote code reusability and separation of concerns. This allows developers to write cleaner, more maintainable code. Second, pipes can enhance performance, as they can be used with Angular's change detection strategies to optimize rendering. Lastly, pipes allow developers to create a more dynamic and responsive user interface without extensive changes to the underlying application logic.
What are Pipes?
In Angular, a pipe is a simple function that takes an input value and transforms it into a desired output format. They are usually applied in templates using a pipe operator, which is a vertical bar (). For example, if you want to format a date, you can use the DatePipe that Angular provides. The syntax is straightforward, for instance:
This displays the current date in a full date format. Pipes make it easy to work with complex data types without modifying the original data structure.
Built-in Pipes
Angular comes with several built-in pipes that cover common data formatting needs. Here are some notable examples:
- DatePipe: Formats a date value into a string based on the specified format.
- CurrencyPipe: Transforms a number into a currency string for better readability.
- DecimalPipe: Formats a number to a decimal format, allowing specifications for number of decimal points.
- PercentPipe: Converts a number to a percentage string.
- JsonPipe: Converts an object or array into a JSON-formatted string for easier debugging and display.
Using built-in pipes is straightforward, and they integrate seamlessly into Angular templates, promoting quicker development cycles.
Custom Pipes
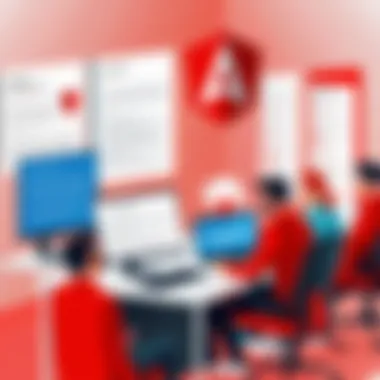
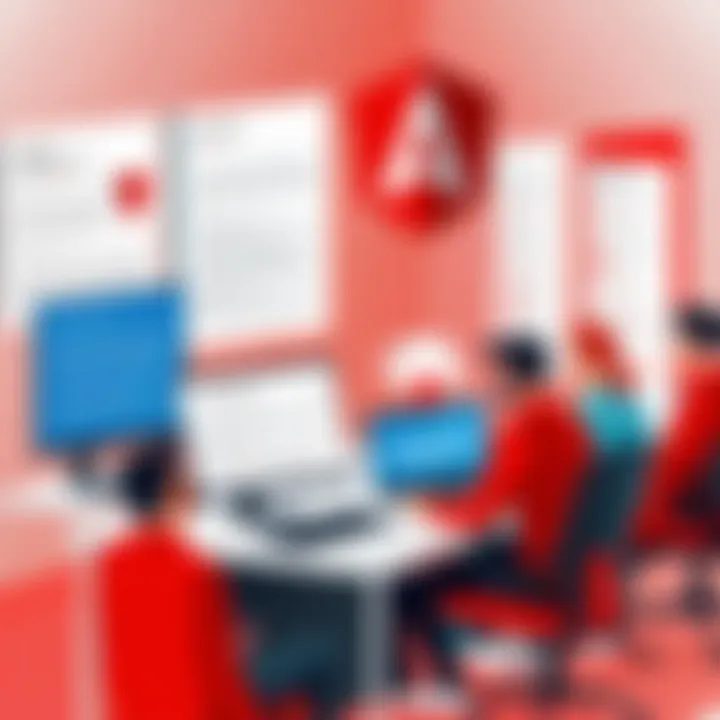
Though Angular provides many built-in pipes, developers can also create custom pipes to fit specific project needs. A custom pipe is useful when the built-in options do not meet certain requirements or when you wish to implement unique formatting logic.
To create a custom pipe, you must define a new class and implement the interface. Here is a small example of how to create a simple custom pipe:
After declaring the custom pipe, you can use it in your template as follows:
Custom pipes allow for greater flexibility and enable developers to handle very specific data transformation tasks.
Testing in Angular
Testing is a crucial part of the development process in Angular. It ensures that the application behaves as expected and helps to identify issues before deployment. Quality assurance through testing leads to a more reliable and maintainable codebase, which ultimately benefits both developers and users. With Angular's powerful testing tools, developers can efficiently test components, services, and other features, ensuring that new changes do not break existing functionality.
Importance of Testing
Testing in Angular serves multiple purposes. First, it provides confidence in the code. When tests pass, developers can be more certain that their application works correctly. This aspect is critical, especially in large applications where even small changes can have unforeseen consequences.
Additionally, testing promotes faster development cycles. With automated tests, developers can quickly identify issues and resolve them, reducing the time spent on debugging. This efficiency allows for more time to focus on building new features instead of fixing broken ones.
Another key benefit is documentation. Tests often act as a form of documentation for the application. They illustrate how components are supposed to work and how different parts interact. This is invaluable for new developers joining a project or when returning to a project after time away.
Furthermore, proper tests can improve the overall design of the software. Writing tests often encourages developers to create more modular and decoupled code, adhering to best practices like SOLID principles. This leads to a more scalable and maintainable architecture in the long run.
Testing Components
Testing components in Angular typically involves two main strategies: unit testing and integration testing. Unit tests focus on individual components or services in isolation, while integration tests check how these units work together.
Angular provides an excellent testing framework integrated with the application using Jasmine and Karma. Here is a simple example of how you might set up a basic unit test for a component:
In this snippet, we import the necessary tools from Angular's testing library. We set up and configure the testing module with the component we want to test. Then, we create an instance of the component and execute a basic test to check if it is created successfully.
Real-world applications may have more complex scenarios requiring mocking dependencies and testing component interactions. Angular facilitates these requirements through its robust dependency injection and testing utilities.
Common Interview Questions
Understanding common interview questions is essential for anyone preparing for an Angular-focused job interview. These questions not only test theoretical knowledge but also gauge a candidate's practical application skills and their problem-solving capabilities with the framework. Being well-versed in these questions gives candidates the upper hand, allowing them to articulate their understanding effectively.
Getting familiar with these questions can boost confidence during interviews. Candidates can demonstrate their grasp of core Angular concepts, its architecture, and functional aspects. Additionally, interviewers often appreciate well-prepared candidates who can discuss their experience with Angular, its features, and best practices. This alignment of knowledge and experience can greatly influence hiring decisions.
What is the difference between AngularJS and Angular?
AngularJS and Angular are distinct frameworks, although both serve the same fundamental purpose of building web applications. AngularJS is the initial version, utilizing JavaScript, while Angular, often referred to as Angular 2+, is a complete rewrite and adopts TypeScript as its primary language. This transition brings several benefits like improved performance, a more modular architecture, and the use of components, which can easily be reused across applications.
Angular introduces a more modern and efficient approach. It provides features like lazy loading, AOT (Ahead of Time) compilation, and a sophisticated dependency injection system, which were either limited or non-existent in AngularJS. Hence, while AngularJS provides a solid foundation, Angular represents a significant evolution in the framework's design and capabilities.
How does two-way data binding work?
Two-way data binding is a powerful feature in Angular that synchronizes the data between the model and the view. Changes made in the model automatically reflect in the view, and conversely, any changes in the view are mirrored back to the model. This feature simplifies the process of keeping the model and view in sync, reducing the amount of boilerplate code necessary.
In Angular, two-way data binding is achieved through a combination of the ngModel directive and property binding. This allows developers to build more interactive applications without manually managing DOM updates. However, understanding when and how to use this feature is crucial, as improper use can lead to performance issues.
Explain the concept of services.
Services in Angular act as reusable components that provide specific functionalities. They allow for the separation of business logic from the user interface. By encapsulating related code in services, developers can promote code reusability and maintainability. This is particularly useful for tasks like data fetching, logging, and configuration settings.
In Angular, a service can be created using a simple class and can be injected into components through Angular’s dependency injection system. This approach encourages the creation of modular applications, where different parts can communicate without being tightly coupled. Services provide a robust way to manage state and facilitate collaboration between various components.
What are lifecycle hooks?
Lifecycle hooks are methods that allow developers to tap into key events in a component’s lifecycle. These hooks provide insights into when a component is created, updated or destroyed, allowing for optimized performance and resource management. In Angular, lifecycle hooks include ngOnInit, ngOnChanges, ngOnDestroy, among others.
For example, the ngOnInit hook is called once after the first ngOnChanges, making it a good place for initialization logic. Similarly, ngOnDestroy is used to perform cleanup tasks, like unsubscribing from observable streams. Understanding and utilizing these hooks effectively can lead to more efficient applications and improved user experiences.
Culmination
In this article, we explored several fundamental aspects of Angular that are crucial for anyone preparing for an interview. Understanding Angular is not just about knowing its syntax and features; it is about grasping its architecture and how different components interact within an application. This knowledge allows candidates to articulate their understanding effectively and demonstrate their practical skills during interviews.
Preparing for Angular Interviews
Preparation is key to success in any interview. When it comes to Angular, candidates should focus on several key areas:
- Understand Core Concepts: Be well-versed in Angular's core features like components, services, and modules. Familiarize yourself with how these elements interact and their roles in a web application.
- Practice Coding: Work on small projects or contribute to open source. This not only builds your portfolio but also helps consolidate your knowledge through hands-on experience.
- Mock Interviews: Participate in mock interviews to experience the question formats. This also helps you articulate your thoughts clearly under pressure.
Candidates should also stay current with updates to Angular, as the framework continually evolves. Checking resources like the official Angular documentation or forums such as Reddit can provide valuable insights.
Continuous Learning in Angular
Angular is a dynamic framework, and continuous learning is essential for staying relevant in the field. Here are a few strategies for ongoing education:
- Follow the Official Angular Blog: Updates from the Angular team can provide insights on new features and best practices.
- Online Courses and Tutorials: Platforms like Coursera and Udacity offer specialized Angular courses that can deepen your understanding.
- Join Online Communities: Being part of online forums or local meetups can help exchange knowledge and experiences with other Angular developers.