Exploring C in Embedded Systems: A Detailed Overview
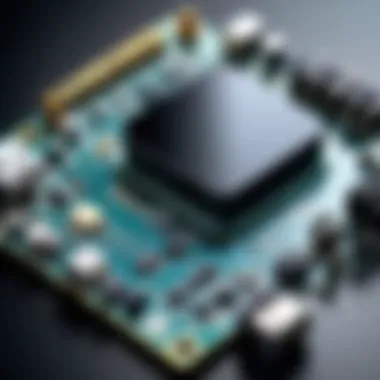
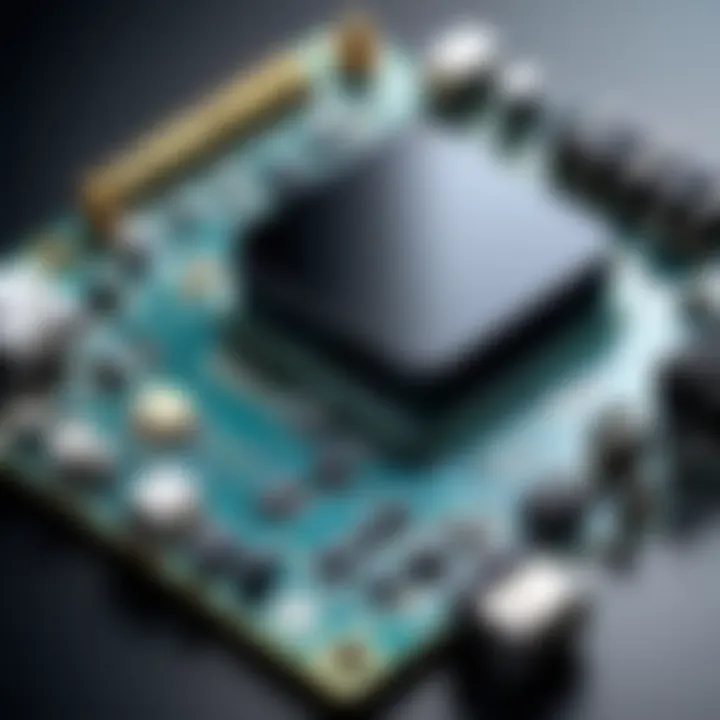
Overview of Topic
Embedded systems are pivotal to modern electronic devices, merrily humming their tasks without much ado. These specialized systems combine hardware and software to perform dedicated functions. Generally, they sit within larger machines. Think about everything from your microwave to advanced robotics. They run on C programming language, known for its efficiency and control over hardware resources.
In today’s tech industry, where efficiency reigns supreme, the significance of C within embedded systems can't be overstated. Understanding this relationship opens doors to innovation and better problem-solving capabilities in technology.
To grasp the importance of C in embedded systems, let’s take a brief stroll through history. Originally developed in the early 1970s, C has undergone a journey through various technological advancements. The language’s simplicity and close-to-hardware nature have ensured its staying power amid evolving programming paradigms. Even as newer languages emerge, C holds a special place in embedded systems due to its versatility and performance.
Fundamentals Explained
To fully appreciate how C impacts embedded systems, one must understand several core principles and terminologies.
Core Principles: At its heart, embedded programming in C focuses on efficiency and optimal resource management. Key concepts include:
- Memory Management: Proper allocation and deallocation help ensure efficient memory use, essential in systems with limited resources.
- Real-Time Processing: Often, embedded applications require quick responses. C enables addressing timing constraints effectively.
Key Terminology:
- Microcontrollers: Small computing devices that manage specific tasks. They often serve as the heartbeat of embedded systems.
- Firmware: The specialized software that operates within embedded hardware, fundamentally written in C for many systems.
Foundational knowledge in C, such as understanding data types, control structures, and pointers, becomes vital as we delve deeper into practical applications.
Practical Applications and Examples
The beauty of C in embedded systems lies in its wide-ranging practical applications. Let’s consider a few real-world case studies:
- Home Appliances: Modern appliances often integrate C-driven microcontrollers to enhance functionalities. A washing machine, for instance, relies on C for various cycles and error handling, ensuring efficient operation.
- Automotive Systems: Vehicles increasingly depend on embedded systems for safety features, like anti-lock braking systems. C plays a crucial role in reading sensor data and responding in real-time.
Hands-On Project Example
Creating a simple temperature monitoring system can be an eye-opening experience. Using an Arduino board and a temperature sensor, you can write a basic code snippet:
This simple program reads the sensor input and displays it on an LCD, showcasing how C enables interaction with hardware.
Advanced Topics and Latest Trends
As technology evolves, so does the realm of embedded systems. Current trends highlight the integration of IoT (Internet of Things) with embedded programming. This pairing enhances connectivity and data exchange, making embedded systems smarter.
Cutting-edge developments in the programming techniques also include:
- Model-Based Design: Emphasizes the use of models during development, helping visualize systems before coding begins.
- Low-Power Design Strategies: As energy efficiency becomes paramount, these strategies focus on prolonging device battery life without sacrificing performance.
The future seems promising. Proliferation in autonomous systems, including drones and smart cities, stands as a testament to the growing relevance of C in continually advancing tech landscapes.
Tips and Resources for Further Learning
If you're keen on diving deeper into C and embedded systems, several resources can light your way:
- Books:
- Online Courses: Consider platforms like Coursera or edX for structured learning.
- Tools: Using development boards like Arduino or Raspberry Pi provides hands-on experience.
- "The C Programming Language" by Brian W. Kernighan and Dennis M. Ritchie.
- "Programming Embedded Systems in C and C++" by Michael Barr.
Don't hesitate to engage with communities on forums such as Reddit to share knowledge and gain insights from fellow learners and professionals.
Prelude to Embedded Systems
Embedded systems are an essential part of our daily lives, often operating quietly in the background. They run the show in a vast array of devices—from washing machines to industrial robots—making them crucial to modern technology. Understanding these systems is important, especially for those working with or developing embedded applications.
One of the key elements when discussing embedded systems is their specialized nature. Unlike general-purpose computers, embedded systems are designed for specific functions. This focus allows them to operate effectively in their environments while consuming minimal resources. As devices become smarter, the need for better understanding of embedded systems has skyrocketed.
Defining Embedded Systems
To put it simply, an embedded system integrates hardware and software to perform dedicated tasks. More than just a microcontroller or a chip, it includes the entire system—from the processors to the application-specific software that runs on them.
- Characteristics: Embedded systems are often characterized by their specific functionality, real-time operation, efficiency, and low power requirements.
- Examples: Think of a heart rate monitor or an anti-lock braking system in cars—both are embedded systems that interact with the physical world, processing data and making intelligent decisions.
Notably, embedded systems can be classified in several ways, such as:
- Standalone: Can operate independently, like a digital camera.
- Networked: Operate as part of a network, for instance in smart home devices.
- Mobile: Designed for portability, such as smartphones or tablets.
The Role of Programming in Embedded Systems
Programming is the backbone that allows these embedded systems to function properly. While you might be thinking, "Programming is just writing code," it's much more intricate in this context.
- Low-level programming: Often, embedded systems require low-level programming languages like C. C allows developers to write code that directly interacts with the hardware, giving them more control. The choice of programming language can drastically affect everything from performance to resource consumption.
- Real-time constraints: In the world of embedded systems, timing is everything. Code not only needs to run efficiently but also at the right moment. This is where the ability to handle interrupts and prioritize tasks becomes essential.
While the intricacies of embedded programming can be daunting, they also pave the way for innovative solutions in our digital landscape.
"Embedded systems aren't just about technology; they're about creating smarter ways to interact with the world around us."
Understanding Language Basics
Grasping the fundamentals of the C programming language is essential for anyone venturing into the realm of embedded systems. C is considered a lower-level language compared to modern programming languages. It provides a fine balance between control over hardware and programmer efficiency. Understanding its nuances not only enhances one’s coding skills, but also allows for deeper engagement with the hardware, which is paramount in embedded development.
Syntax and Data Types in
At the core of any programming language lies its syntax. In C, the syntax is relatively straightforward, making it easier for novices to pick up. However, it demands precision; even a misplaced semicolon can lead to errors. C's syntax is designed to allow for clear expression of complex ideas in concise formats.
The language employs various data types, which determine how much space a variable occupies in memory and how the data is handled. Common data types in C include:
- int: Used for integers. The size can vary depending on the system, usually 4 bytes.
- float: This type represents single-precision floating point numbers, used for decimal values.
- double: A double-precision floating point for more accuracy.
- char: Represents a single character, typically 1 byte in size.
Understanding these data types is crucial for embedded systems programming, as memory is often at a premium in such environments. Choosing the correct type can enhance performance, reduce memory usage, and ensure that the system runs smoothly without unnecessary overhead.
"Good programming is all about reducing complexity."
Furthermore, C allows for the creation of user-defined data types like structures and unions, which can help represent complex data efficiently. A sound understanding of these will empower programmers to craft more intricate and optimized embedded systems.
Control Structures and Functions
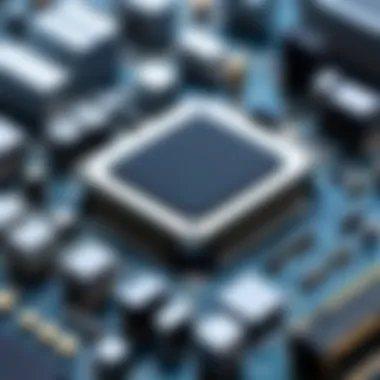
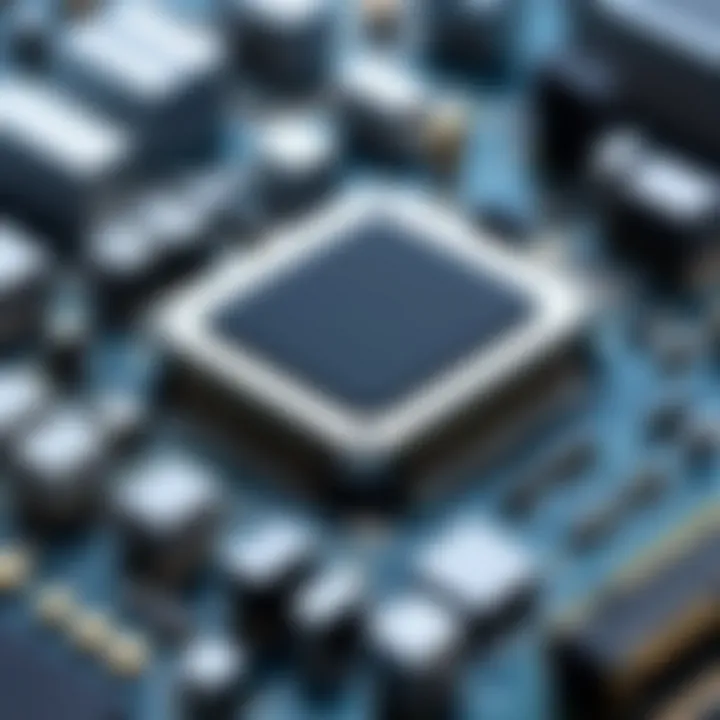
Control structures are the backbone of programming logic. In C, these include conditionals and loops which control the flow of the program based on specific conditions. Familiarity with these control structures is paramount when creating responsive and efficient embedded systems.
- If Statements: These allow the execution of code blocks based on true or false conditions. For instance, in an embedded application, an if statement could control a motor based on sensor input.
- Switch Cases: Useful for handling multiple conditions, especially when working with states or modes in embedded systems.
- Loops (for, while, do-while): These enable repetitive execution of code. In embedded systems, loops might handle tasks like monitoring sensors continuously.
Functions in C promote modularity, enabling the separation of tasks and allowing for code reuse. Each function can perform a distinct operation, managing complexities by breaking the problem down into manageable chunks. Crafting functions not only cleares the structure of your code, but also eases debugging efforts, as each can be tested independently.
The clarity that comes with using control structures combined with functions underscores the importance of understanding these principles in C programming. Integrating these tools will significantly enhance the responsiveness and functionality of embedded systems.
The Necessity of in Embedded Systems
When it comes to embedded systems, the C programming language isn't just a player in the game—it's often the MVP. With its unique blend of efficiency and accessibility, C has carved out a vital niche in this domain. In this section, we’ll dive into why C is not only necessary, but also how it stands out in a world filled with programming languages.
Efficiency and Performance
Embedded systems are often bound by strict resource limitations, whether that’s power, memory, or processing capability. C shines bright under these constraints. It allows developers to write low-level code that can directly interact with hardware components, optimizing performance in a way high-level languages simply can’t match. The ability to write efficient code can lead to faster execution times, which is paramount for systems needing real-time responses.
Here are several reasons why efficiency is at the forefront of C’s importance in embedded applications:
- Direct Memory Access: C gives developers fine-grained control over memory management, allowing for the avoidance of overhead that might come with other languages. This can lead to faster, more performance-oriented solutions.
- Minimal Runtime Requirements: Programs written in C usually have smaller runtime libraries compared to languages that are more abstracted, offering a leaner footprint in embedded systems.
- Speed of Execution: C provides the ability to compile down to machine language, thus allowing the code to run swiftly, which is critical in applications like robotics or medical devices where timing is everything.
One of the beauties of C is that it balances performance with abstraction. Developers can use higher-level constructs when needed, but can also dig deep to the hardware layer when the situation calls for it.
Hardware Accessibility
You can think of C as the bridge connecting developers to the hardware in embedded systems. Unlike some programming languages that act more like a shield between programmer and machine, C pulls back the curtain, giving developers direct access to memory addresses and hardware registers.
This characteristic opens up several advantages:
- Close to the Metal: C enables programmers to write code that interacts directly with device drivers and microprocessors, offering nuanced control that is essential for hardware-level operations.
- Portability: Although C allows hardware-access, the language itself is quite portable. Code written for one microcontroller can frequently be adapted for another with minimal modification, making it easier to manage projects across diverse hardware platforms.
- Flexibility: With libraries catering to various microcontrollers, C provides tools for accessing hardware features just a mere function call away. This means developers can quickly leverage complex functionalities without getting bogged down in convoluted code.
In essence, the necessity of C in embedded systems isn't just about preference; it's about the adaptability, efficiency, and performance that embedded developers crave. While alternatives do exist, none have quite matched C's track record in the demanding landscape of embedded programming.
"For those in the field of embedded systems, utilizing C feels like wielding a power tool—fast, effective, and harnessed precisely to the task at hand."
In sum, the strategic importance of C can't be overstated. Its capability to provide both efficiency and robust hardware accessibility positions it not just as a common choice but as a necessary one in the realm of embedded systems.
Key Features of for Embedded Applications
C has carved out a prominent niche in the realm of embedded systems for various compelling reasons. This section highlights the essential features that make C a go-to language for embedded applications, demonstrating its versatility and effectiveness.
Portability and Flexibility
When we talk about the portability of C, it's like saying you can take your tools from one job site to another without a hitch. C is designed to work on various hardware platforms with minimal changes, thanks to its standardization by ANSI and ISO. This means that developers can write a program that can run on a low-power microcontroller one day and a high-performance processor the next.
The flexibility that C offers is another major advantage. With the ability to manipulate hardware settings directly through pointers, programmers have more control over how the application interacts with the underlying hardware. For instance, if you're working on an embedded device that requires tight integration with its hardware, C’s flexibility allows you to code down to the metal without prohibitive overhead. This is crucial in scenarios where efficiency matters most, like in medical devices or automotive systems where timing and accuracy are not optional.
Reflecting on these strengths, it's clear that C aligns well with the needs of diverse embedded environments. Its range of libraries and frameworks, coupled with the community's continuous support, ensures that as technology evolves, C can keep pace without necessitating a complete overhaul of existing code.
"Portability isn't just a nice feature; it's a necessity in today's ever-evolving tech landscape."
Low-Level Manipulation Capabilities
One of the standout features of C within embedded systems is its ability for low-level manipulation. If you've ever driven a car, you know how the feel of the steering wheel varies with different cars. Similarly, C gives developers nuances in control over hardware.
Low-level manipulation allows programmers to operate directly with memory addresses, set up registers, or interact with peripherals like timers and sensors. For example, when working on a project involving an LED display, a developer might write code to send an instruction directly to the display's memory location. This precise control not only enhances performance but also opens the door to advanced optimization techniques that can result in reduced power consumption—something especially critical in battery-operated devices.
Accessing hardware at this depth is not something many high-level languages allow easily. Thus, C provides a direct bridge to the hardware layer, making it invaluable in embedded development where understanding the minutiae of system architecture can be the difference between good and exceptional software performance.
In summary, the key features of C—portability and low-level memory manipulation—are pivotal aspects that empower developers in the realm of embedded applications. They help to deliver systems that are not only effective but adaptive to the ever-changing technological demands.
Embedded Systems Development Life Cycle
The development life cycle for embedded systems plays a pivotal role in shaping efficient and practical applications. Unlike conventional software systems, embedded systems have unique requirements that call for a structured approach. By adhering to this life cycle, developers can meticulously navigate through the complex stages of designing and building embedded applications. This not only ensures clarity in each phase but significantly improves the final product's overall quality.
Planning and Requirements
In the planning phase, it’s essential to gather all pertinent requirements before diving into coding. This phase lays the groundwork for everything that follows. Here, developers sit down with stakeholders to discuss exactly what the embedded system needs to achieve. It’s a bit like laying the bricks for a house; if the foundation isn’t sturdy, the entire structure is at risk.
Key elements during this stage include:
- Defining Functional Requirements: What specific tasks should the system perform? For instance, if you’re making a smart thermostat, the function could include adjusting temperature based on user behavior.
- Establishing Performance Metrics: How fast should it respond? The faster, the better, especially in time-sensitive environments. For example, a medical device that monitors patients must react immediately to any abnormal readings.
- Identifying Constraints and Limitations: From memory limitations to processing power, knowing the boundaries helps in making informed choices down the line.
"A well-defined requirement is half the battle won."
This phase can become quite intricate. Stakeholders need to ensure all voices are heard, and differing priorities must be harmonized to set clear objectives. A comprehensive requirements document often serves as a guiding light through subsequent development stages.
Design and Implementation
Following from the planning stage, the design phase is where creativity meets technical expertise. It’s about transforming those requirements into a solid blueprint. The design should detail the architecture of the system, specifying hardware and software components.
In practice, this might mean sketching out how the code interacts with the hardware, sensors, and other peripherals. Here are the main components to consider:
- Architectural Design: Outline how tasks will be divided across various components. Are you going to use embedded Linux or a simple microcontroller?
- Interface Design: Specify how different modules communicate. For example, data passed from a temperature sensor to the main processing unit needs clear paths.
Once the design is endorsed, it’s time for implementation. Development teams start coding in C, leveraging its strengths in memory management and performance optimization. Throughout this stage, developers may deploy iterative cycles, adjusting as they go based on early testing and feedback.
Testing and Validation
Any thoughts about moving to production without rigorous testing should be shelved instantly. Testing and validation are crucial to ensure the system performs as intended. This phase can be broken down into different types of tests:
- Unit Testing: Focusing on individual components. For instance, testing whether the code accurately reads temperature data from a sensor.
- Integration Testing: Ensures that combined parts work well together. If the sensor data isn’t synchronized with the display, there’s a fundamental flaw.
- System Testing: Conducted on the complete system to validate overall functionality. Here, it’s vital to assess if the embedded device meets all initial requirements and performs within specified bounds.
Continuous validation is also important, sometimes best achieved through a beta testing phase with real users before full-scale production. Gathering feedback during this stage helps fine-tune the system, ensuring it’s not just functional but also user-friendly.
This life cycle framework, while versatile, requires flexibility to adapt to the nuances of different projects. In essence, the careful execution of each stage not only builds a robust embedded system but also solidifies the developer's reputation in an increasingly competitive field.
Common Tools and Compilers for Development
When one works with embedded systems, the selection of tools and compilers can make or break the project. These tools serve not only to compile code, but also to help debug, test, and develop applications all the while managing the immense constraints of the embedded environment.
In embedded system development, having the right tool in your kit can ensure efficient programming and fewer bumps in the road. This section delves into Integrated Development Environments (IDEs) and cross compilers, which are invaluable in this realm.
Integrated Development Environments (IDEs)
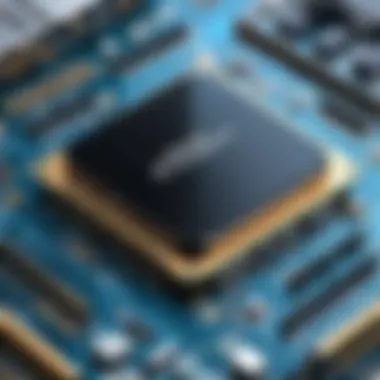
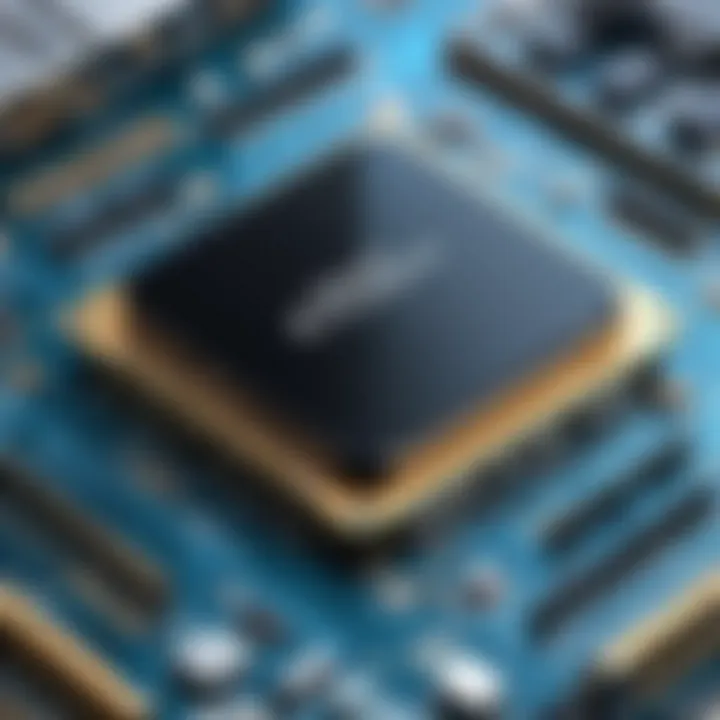
IDEs provide a comprehensive workspace where developers can write, test, and debug their code. These platforms bundle several essential features into one package. Popular IDEs such as Keil µVision, IAR Embedded Workbench, and Eclipse support C programming in embedded environments by offering tools for code editing, debugging, and project management.
Benefits of IDEs:
- Enhanced Productivity: With features like code suggestions and auto-completion, IDEs help cut down development time.
- Built-in Debugging Tools: Many IDEs come with an integrated debugger that allows developers to run code step-by-step to find issues more quickly.
- User-Friendly Interface: The intuitive design of IDEs makes them accessible, even for those relatively new to embedded programming.
- Version Control Integration: IDEs can link directly with version control systems, streamlining collaboration among team members.
However, one must also consider that not all IDEs cater to every need. For instance, while some might be great for smaller applications, others like Visual Studio Code provide extensive extensions but might require setup and configuration adjustments that could be daunting for beginners.
Cross Compilers
Cross compilers are another crucial component in the development hardware for embedded systems. They allow developers to compile code on one platform (host) which runs on another platform (target). This process is vital given that the target devices often have limited resources and specific architectures.
Key Points about Cross Compilers:
- Platform Independence: Cross compilers, such as GNU GCC, enable coding in a broader development environment, accommodating the peculiarities of different hardware platforms.
- Performance Optimization: They help create optimized binaries specifically suited for the target architecture, which can enhance performance significantly.
- Customized Toolchains: Developers can create tailored toolchains that cater to the specific needs of the hardware they are working with, facilitating more precise debugging and profiling.
Nonetheless, finding the right cross compiler also involves a bit of trial and error; different compilers come with distinct sets of features and compatibilities. It is wise to ensure that the selected compiler works seamlessly with the chosen IDE to minimize friction in the development workflow.
In summary, having a solid understanding of both IDEs and cross compilers is fundamental for aspiring embedded systems developers. Choosing the right tools can simplify the complex journey of embedded C programming, making the task more manageable and efficient.
"The tools you use to build your software can influence both the performance of your code and your productivity as a developer."
In the world of embedded C programming, making informed choices about your development tools lays the foundation for future successes.
Memory Management in Embedded
Effective memory management is a cornerstone of programming in embedded systems using C. In these environments, where resources are often limited and performance is crucial, understanding how memory works can decide between a system that operates smoothly and one that falters under pressure. Embedded systems frequently run in power-constrained environments and need to deliver real-time performance. Thus, managing memory allocation correctly becomes not just beneficial but essential.
Static vs Dynamic Memory Allocation
Static and dynamic memory allocation are two sides of the same coin in the world of memory management, particularly in embedded C. Each has its pros and cons, and knowing when to use each can make a significant difference in application performance.
- Static memory allocation occurs at compile time. When using this method, the size of the memory block must be defined before the program is run. This leads to predictable behavior, as the amount of memory required is known upfront. Fixed-size data structures, like arrays, are typical examples where static allocation shines. This approach is memory-efficient and reduces fragmentation, which is a boon in environments with scant resources.However, static allocation can be limiting as it does not allow for flexibility during runtime. For instance, if an array is set to hold ten items but only five are used at any one time, it can waste precious memory.
- Dynamic memory allocation, on the other hand, allows for memory to be allocated at runtime, typically using functions like and . This can accommodate applications with variable data sizes. For example, in a sensor data logging application, the amount of data recorded could vary, necessitating dynamic allocation.Yet, this approach comes with a price. In embedded programming, the uncertainty of where and when memory might be freed can lead to fragmentation and, in worse scenarios, memory leaks if not handled properly. This unpredictability can result in performance issues and possibly system crashes.
The choice between these two methods depends heavily on the specific application's requirements. For instance, for a project like a simple temperature sensor, using static allocation may suffice. Contrarily, dynamic allocation might be more suited for an application like a mobile device where data size can fluctuate considerably.
Memory Constraints in Embedded Systems
Memory constraints in embedded systems can often feel like trying to fit a square peg into a round hole. Unlike conventional systems with plenty of RAM and storage at their disposal, embedded systems often have strict limitations that dictate how memory is utilized. This challenge further emphasizes the importance of effective memory management.
Common constraints include:
- Limited RAM and ROM: Most embedded systems operate with small amounts of memory, meaning every byte matters. It becomes crucial to ensure that the code and buffers consume as little memory as possible.
- Power Consumption: Efficient memory usage directly correlates with power management. Excessive memory usage can lead to increased power consumption, which is often a deal-breaker in mobile and remote applications.
- Real-Time Requirements: For real-time applications, timing is everything. Allocating and deallocating memory dynamically can introduce delays that might disrupt the system's performance, which means careful consideration is vital.
To navigate these constraints successfully, embedded C developers often employ practices like:
- Proper module designs that segregate functionalities and limit unnecessary memory usage.
- Regular memory audits to spot leaks before they become problematic.
- Utilizing memory pools instead of allowing the system to allocate memory dynamically. This approach can mitigate fragmentation issues and ensure predictable performance.
Ultimately, mastering memory management in embedded C is a blend of art and science. Developers find themselves constantly balancing performance, resource limitations, and application requirements. Being an adept memory manager can mean the difference between a flawless performance and a buggy system.
"In embedded systems, every byte counts, and every microsecond matters. Efficient memory management is not just good practice; it's a necessity."
For further understanding of memory management intricacies, consider exploring additional resources on Wikipedia and Britannica.
Interrupts and Programming
When diving into the realm of embedded systems, one cannot overlook the vital role of interrupts. These small yet powerful elements serve as the backbone of many applications, enabling devices to respond promptly to events and stimuli. By understanding interrupts in tandem with C programming, developers enhance their ability to create systems that are not only responsive but also efficient. Let's break this down into two critical segments for clarity.
Understanding Interrupts
At its core, an interrupt is a signal that temporarily halts the ongoing processes in a microcontroller or processor, allowing it to address a different task. Imagine a busy restaurant: the chef (processor) is in the middle of preparing a meal (ongoing task) when a server (interrupt) races in with an urgent order. The chef must stop briefly to acknowledge the new order before resuming the original task.
Interrupts can be categorized into two main buckets: hardware and software interrupts.
- Hardware Interrupts: Triggered by external hardware events such as timers, input devices, or changes in sensor readings. For instance, pressing a button sends a signal that interrupts the main program, allowing it to respond immediately.
- Software Interrupts: These are initiated by executing specific instructions within a program. They are often used to handle system calls or to manage exceptions.
The importance of understanding interrupts cannot be overstated. They help in managing multiple tasks efficiently by allowing the processor to switch contexts as necessary. This is especially vital in real-time applications where time is of the essence—think of a heart monitor that needs to alert medical staff the instant a patient’s vitals deviate from the norm.
Handling Interrupts in
Now that we’ve grasped what interrupts are, let’s talk about how to manage them in C programming. Proper handling of interrupts ensures that your embedded system can efficiently cater to incoming signals without losing track of ongoing processes.
To handle interrupts in C, developers typically follow these steps:
- Define the Interrupt Service Routine (ISR): An ISR is a special function that is executed in response to an interrupt. This routine should be concise and fast since entering and exiting an ISR takes processing time.For example, defining a simple ISR in C for a button press may look like this:
- Enable the Interrupt: After defining the ISR, the next step is to enable the interrupt using specific register settings. Each microcontroller has its own syntax for managing interrupts, so refer to the relevant documentation.
- Utilize Interrupt Flags: Your system should check interrupt flags to determine which interrupt was triggered, especially when multiple interrupts can occur.
Handling interrupts correctly not only boosts system performance but also enhances user experience by reducing response times. It's very much like a finely tuned orchestra, with each musician responding at just the right moment to create a harmonious performance.
"Interrupt handling is the heart of responsive embedded systems—understanding it is non-negotiable for any embedded developer."
Real-Time Operating Systems (RTOS) and
In the sphere of embedded systems, the interplay between C programming and Real-Time Operating Systems (RTOS) is a critical component that influences the performance and efficiency of applications. An RTOS is tailored to serve real-time applications that demand strict timing constraints. It ensures that tasks are executed within predetermined time limits, which is paramount when systems must react to external events—such as sensor readings or user inputs—without delay. This indispensable need for timing leads to an enhanced performance in terms of reliability and responsiveness of embedded systems.
Moreover, C shines in this milieu by offering low-level hardware interaction and fine control over resource management. These attributes grant developers the ability to optimize resource allocation and scheduling strategies inherent to an RTOS. With C's efficiency, programs can be lean and mean, making the most of the limited resources of embedded devices.
Integrating with RTOS
Integrating C with an RTOS requires a good grasp of both the programming language and the functioning of the operating system itself. The integration involves configuring the RTOS to create a suitable environment for C programs. Here are several critical points to consider:
- API Usage: RTOS often comes with its own set of APIs that facilitates task creation, inter-task communication, and synchronization. Learning to effectively use these APIs in C allows for efficient integration.
- Memory Management: Since C lacks built-in garbage collection, developers must manage memory manually. This becomes even more crucial when working with an RTOS, as running out of memory can cause severe implications for task scheduling.
- Resource Constraints: C allows for low-level coding that can be tailored to fit the constraints of most embedded systems. This means being able to optimize memory usage and CPU cycles, which are at a premium in RTOS applications.
A simple example of integrating a task in C would be as follows:
This snippet creates a basic task within an RTOS, illustrating how tasks interface with C functions.
Task Management and Scheduling
Task management and scheduling are vital elements that determine the effectiveness of processes within an RTOS. Here are some key elements:
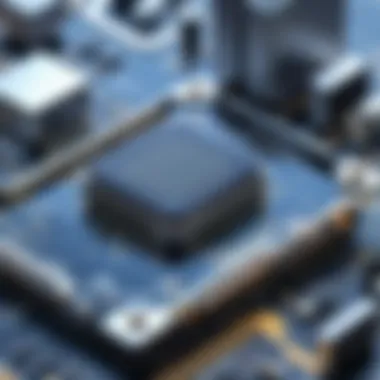
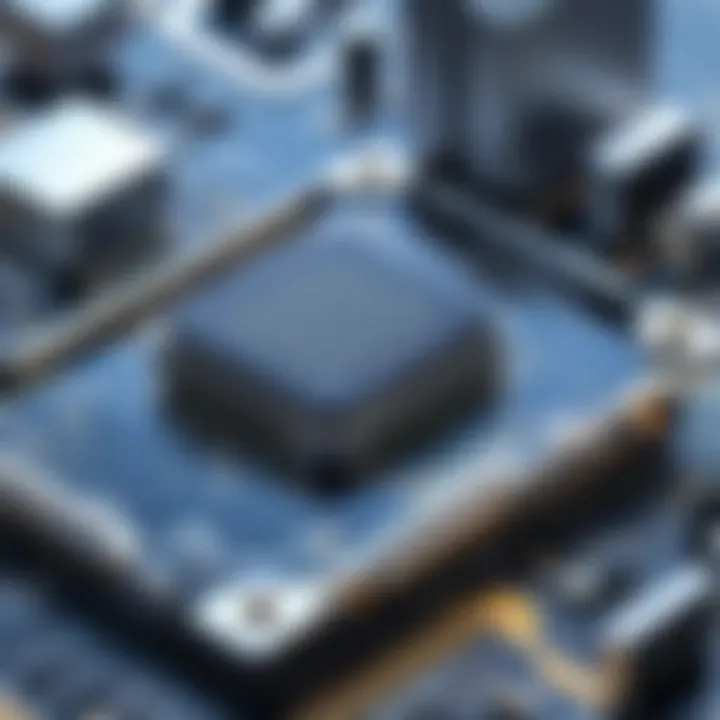
- Priority-Based Scheduling: Many RTOS use a priority-based system where tasks with higher priorities get more CPU time. In C, this can be implemented using real-time scheduling algorithms, such as Rate Monotonic or Earliest Deadline First.
- Inter-task Communication: Tasks often need to communicate and synchronize with one another. C supports various means to achieve this through data structures and functions, ensuring tasks can operate together effectively.
- Temporal Considerations: C handles timing functionality through libraries specially adapted for RTOS. This allows developers to set timeouts and manage task execution based on real-world time, making it crucial for applications that require timely responses.
"C's capability to efficiently manage scheduling and timing factors makes it an essential ally in ensuring the success of embedded systems within a real-time context."
Best Practices in for Embedded Systems
When diving into the world of embedded systems, having a solid grasp of best practices in C programming is not just advisable; it's essential. These practices can significantly enhance both the reliability and maintainability of embedded applications. Keeping efficiency in mind, we will explore two critical areas: code optimization and maintaining code readability. Understanding these facets helps in ensuring that systems are agile and robust, ready to tackle the demands placed upon them.
Code Optimization Techniques
Optimizing code in embedded systems is akin to fine-tuning an engine; you want every component to run smoothly and efficiently. Code optimization can lead to improved performance and, often, a reduction in the system's power consumption. Various techniques can be used here:
- Avoid unnecessary computations: Each operation your program performs takes time and resources. Remove any calculations or function calls that do not contribute to the final result.
- Use efficient algorithms: Choosing the right algorithm can drastically reduce processing time. For instance, using a binary search instead of a linear search can save significant time when dealing with sorted data.
- Inline functions: Instead of calling a function numerous times, using inline functions can reduce overhead. However, be careful, as this can increase code size, which might not be ideal in constrained environments.
- Optimize loop structures: Loops are common culprits for inefficiencies. For instance, unrolling loops can increase speed but may use more memory; weigh the trade-offs based on your application's needs.
- Utilize compiler optimizations: Modern compilers come with various optimization flags. Familiarizing yourself with your chosen compiler's options can yield massive benefits in terms of execution speed.
Utilizing these techniques will help in crafting code that runs like clockwork, but always remember to test the system after making optimizations to ensure no unexpected consequences arise.
Maintaining Code Readability
While optimization is crucial, neglecting code readability can lead to a maintenance nightmare. Clear and readable code makes future modifications or debugging straightforward. Here are some ways to maintain code readability:
- Consistent naming conventions: Use meaningful variable and function names. Instead of a vague name like , use something descriptive like . This makes it easier for anyone reading the code (including future you) to understand its purpose.
- Comment judiciously: Well-placed comments can clarify complex sections of code. However, over-commenting can clutter your code. Aim for a balance—comment why something is being done rather than what is being done, as the latter should be clear from the code itself.
- Modular approach: Break your code into functions and modules that handle specific tasks. This not only promotes reusability but also makes the codebase easier to navigate.
- Adhere to standards: Follow established coding standards, like MISRA C for embedded systems. These guidelines have been designed to enhance safety and reliability.
- Use version control: Systematically managing changes to your codebase helps track different versions and understand changes over time. This practice also fosters collaboration when working in teams.
In summary, the interplay between optimization and readability is crucial for success in embedded systems development. By integrating these best practices into your workflow, you pave the way for robust, efficient, and maintainable systems.
"Good code is its own best documentation." – Steve McConnell
In this fast-paced field, adhering to best practices in C programming can not only improve your project outcomes but also elevate your skill as a developer. Embrace these techniques and watch both your projects and confidence grow.
Challenges in Embedded Development
Embedded C development, while offering powerful capabilities, is fraught with distinct challenges that require deeper understanding and adept skills. These hurdles can significantly impact the effectiveness of your embedded systems, consequently warranting careful attention and a strategic approach. Here, we dive into two primary aspects: debugging complex systems and adapting to rapid technological changes.
Debugging Complex Systems
Debugging in the realm of embedded systems is akin to searching for a needle in a haystack. When things go awry in a project, pinpointing the exact issue can feel overwhelming, especially with the intricate relationships between hardware and software. In fact, effective debugging often makes or breaks the success of an embedded C project. Here are some key considerations and methods:
- Limited Visibility: Embedded systems usually lack robust interfaces for troubleshooting, meaning developers must often rely on serial outputs or LEDs to identify issues. This can make it challenging to track down bugs efficiently.
- Timing Issues: Many embedded applications are time-sensitive. Bugs in timing can lead to unexpected behavior, often requiring developers to have a keen eye on how tasks are executed. Incorrect assumptions about timing can lead to minutes of debugging that sometimes stem from a milliseconds' miscalculation.
- Hardware-Software Interaction: The delicate balance between software and hardware adds a layer of complexity. For instance, a function in C may execute perfectly in isolation but fail when interacting with hardware. Using oscilloscopes or logic analyzers can aid in observing the timing and signaling between these layers.
To combat these challenges, it is essential to employ systematic debugging techniques, such as:
- Unit Testing: Writing and executing unit tests can help catch errors early in the development cycle.
- Simulation Tools: Utilizing simulators can provide insight before physical hardware deployment.
- Incremental Development: Developing and testing in small chunks reduces the complexity of debugging and makes it easier to track down the source of errors.
"The joy of programming is to create something from nothing. The sorrow of it is that it might fall apart; debugging is the bridge between the two worlds."
Adapting to Rapid Technological Changes
The tech landscape is a whirlwind—daily advancements in tools, platforms, and languages can leave developers in the dust if they're not equipped to adapt. Embedded systems development, in particular, must meet the demands of evolving technologies. Here are some challenges within this domain:
- Keeping Skills Up-to-Date: With languages and tools evolving rapidly, programmers must continually learn to remain effective. This can involve taking workshops or online courses to update their skills.
- Integration of New Standards: As new technologies emerge, certain standards may become outdated. A good example is the shift to wireless communication protocols. Embedded C developers must adapt to ensuring their systems maintain compatibility with these new standards.
- Evolving Hardware: The pace at which microcontrollers and peripheral devices evolve can pose a challenge in terms of software compatibility. Developers need to stay informed about the latest hardware advancements while maintaining existing systems.
Epilogue of Challenges
Understanding and addressing these challenges in embedded C development requires a proactive approach. Whether one is debugging complex systems or adapting to new technologies, navigating this landscape calls for dedication and a commitment to lifelong learning. As technologies continue to evolve, embedded systems developers must embrace these changes, ensuring they are not left behind. By being aware of the hurdles and adopting effective strategies to overcome them, professionals can ensure the success of their embedded projects.
Future Trends in Embedded Programming
As the technology landscape continues to evolve, the field of embedded systems remains at the forefront of innovation. Future trends in embedded C programming are not just speculative; they are guiding the transformation of how devices interact with each other and their environments. Understanding these trends is crucial for professionals and students alike, as keeping up with the evolving landscape can lead to enhanced design capabilities and better implementation of systems requiring embedded C.
Emerging Technologies and Standards
The emergence of various technologies reshapes how embedded C is utilized. This involves a significant push towards adaptive standards. The gradual adoption of protocols, such as Time-Sensitive Networking (TSN) and IEEE 802.15.4, indicates the growing need for reliable, deterministic communication amongst devices. These standards are layering over C's capabilities, enhancing its integration with hardware.
Consider the roll-out of 5G networks. This enables faster communication speeds and lower latency. As a result, embedded systems powered by C are likely to evolve into more sophisticated units capable of handling increased data traffic and connectivity. An important aspect to keep an eye on is the integration of machine learning within embedded architectures. With the right frameworks, developers can utilize the processing power of embedded systems while leveraging C's efficiency to perform tasks like image recognition and predictive analytics right at the edge.
In summary, some notable technologies and standards to look forward to include:
- Automated Test Equipment (ATE) for quicker maintenance and testing.
- Wireless Sensor Networks (WSN) that improve environmental data collection.
- Artificial Intelligence at the edge, allowing quicker decision-making processes without the constant need for cloud computation.
"Emerging technologies offer a treasure trove of possibilities; it’s about harnessing C's flexibility to ride these waves of change effectively."
The Role of in IoT
The Internet of Things (IoT) revolutionizes how we perceive connectivity. Millions of devices communicate in real-time, and embedded C programming finds a prime application here. With its strength in low-level hardware interfacing, C forms the backbone for IoT devices, providing significant performance and stability.
Low power consumption is a hot topic when discussing IoT devices. Many of these devices must operate under stringent energy constraints. Efficiency is key, and C's ability to pull off optimal resource management makes it a primary choice for developers.
Additionally, as IoT networks are primarily built upon inter-device communication, protocols such as MQTT (Message Queuing Telemetry Transport) can be efficiently implemented using C, employing lightweight methods for transmitting messages.
In summary, the noteworthy benefits C brings to IoT include:
- Direct control over hardware, leading to quicker response times.
- Memory efficiency, which is crucial in constrained environments.
- Established ecosystem with libraries that streamline development and enhance functionality.
Future developments are bound to further integrate embedded C with the IoT landscape, making it an essential skill for any aspiring tech enthusiast or IT professional. As we look forward, adapting and reshaping C practices to embrace these shifts will be critical.
Epilogue
The conclusion of this comprehensive guide on using C in embedded systems serves multiple critical functions. It solidifies our understanding of the intricate relationship between C language and embedded systems, while also emphasizing important takeaways, benefits, and future considerations that professionals and enthusiasts must keep in mind as they navigate this technical landscape.
Recap of in Embedded Systems
To sum it all up, C has long been a pillar in the realm of embedded systems. Its efficiency and performance allow developers to write programs that are not only quick but also resource-conscious. Embedded systems often operate under strict hardware constraints, making C's ability to provide low-level access and manipulation invaluable.
Notably, this guide has highlighted key features of C specific to embedded applications such as:
- Flexibility and portability across platforms
- A strong emphasis on memory management practices
- Robust support for hardware-level programming through interrupts and real-time operating systems (RTOS)
By encapsulating the capabilities of C, one can appreciate how to strike a balance between software functionality and hardware limitations. The discussion also covered best practices to adopt, ensuring code is optimized and easily readable, which ultimately benefits collaborative environments or transitions between projects.
"Programmers who understand the language of hardware have the tools to create innovative embeded solutions."
Encouraging Further Exploration
As technology advances, the landscape of embedded systems continues to evolve. New advancements in hardware, the Internet of Things (IoT), and automation technologies offer numerous opportunities. Therefore, it’s crucial for both students and seasoned IT professionals alike to stay engaged and proactive in learning.
Consider exploring specialized resources like forums on Reddit and studying real-world applications to witness the impact of C in embedded environments. Moreover, experimenting with different Integrated Development Environments (IDEs) and compilers can fortify one’s programming skills.
In addition, diving into emerging standards and practices within embedded C will not only prepare you for future projects but also enhance problem-solving capabilities in real-time systems. Whether through formal education or self-study, dedicating time to understanding these evolving technologies can set you apart in the field.
To encapsulate, the journey of mastering C within the embedded systems domain is ongoing and rife with learning potential. We encourage continuous inquiry and experimentation for a deeper appreciation and skill refinement in this fascinating area.