In-Depth Exploration of Django Development Framework
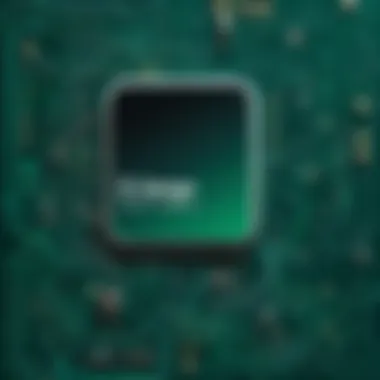
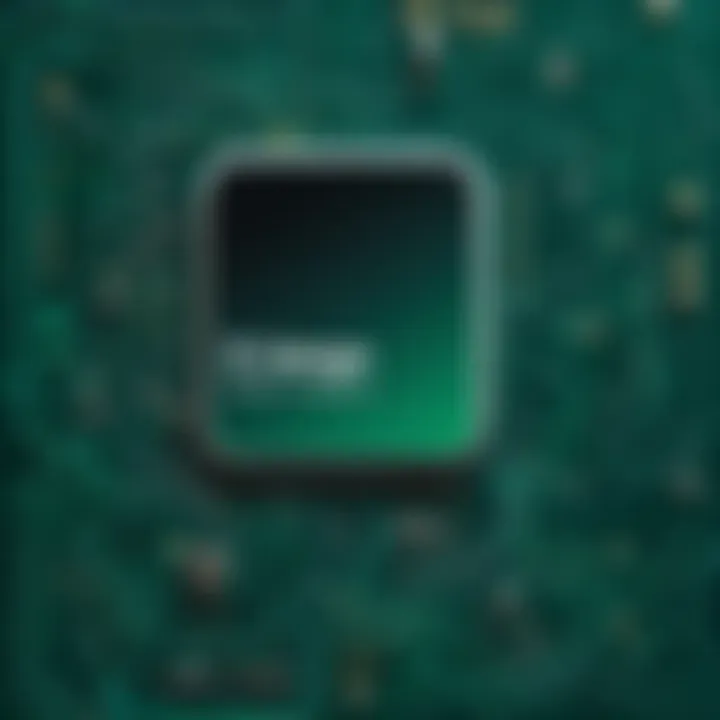
Overview of Topic
Preamble to the main concept covered
Django is an open-source web framework that simplifies web development using the Python programming language. It is designed to help developers create complex, database-driven websites with minimal effort. Django promotes the principle of Don't Repeat Yourself (DRY), which fosters cleaner and more organized code. As such, it stands out in the realm of web development frameworks, offering efficiency and speed to developers at all levels.
Scope and significance in the tech industry
Django holds a prominent position in the tech industry. It is not limited to small-scale applications; it scales effectively for enterprise-level applications too. Many tech giants, such as Instagram and Pinterest, utilize Django in their tech stack. Its robustness makes Django particularly essential for startups and established companies alike that require a reliable and versatile back-end solution.
Brief history and evolution
Django's inception dates back to 2003 when developers Adrian Holovaty and Simon Willison created it for managing web content. The framework was publicly released in 2005. Since then, it has undergone several improvements and updates, with the Django Software Foundation taking over its development and maintenance. New features and optimizations keep it aligned with current web development needs.
Fundamentals Explained
Core principles and theories related to the topic
Django is based on several core principles:
- Reusable components: The architecture promotes reusability via apps.
- Rapid development: It streamlines the development process, allowing for quicker project completion.
- Secure: Built-in protection measures exist against common vulnerabilities.
Key terminology and definitions
Some important terms include:
- MVC Architecture: Django uses a variation known as the MVT (Model-View-Template) architecture.
- ORM: Object-Relational Mapping simplifies database interactions.
- Middleware: Customizes request and response processing.
Basic concepts and foundational knowledge
Prior experience with Python is beneficial but not essential. Basic understanding of web development principles will help. Knowing how HTTP works and understanding databases are essential concepts. Familiarity with front-end languages such as HTML, CSS, and JavaScript will improve overall development skills.
Practical Applications and Examples
Real-world case studies and applications
Django's versatility enables the creation of any type of website. For example:
- E-commerce websites like Shopzilla.
- Content management systems like Wagtail.
Demonstrations and hands-on projects
One can start with a small project, like a simple blog. Listing down clear steps assists learners:
- Set up a virtual environment.
- Install Django.
- Create a new project and an app.
- Define models and views.
- Set up URL routes.
Code snippets and implementation guidelines
Here’s a simple code snippet to define a model:
The snippet shows how Django makes defining models intuitive.
Advanced Topics and Latest Trends
Cutting-edge developments in the field
Django frequently updates to embrace industry advances. Latest releases often add features like asynchronous capabilities. This increases efficiency when handling requests.
Advanced techniques and methodologies
Some strategies for enhanced leveraging of Django include:
- Using Django REST Framework: Optimizes development of APIs.
- Utilizing channels: This asynchrony approach helps in real-time functionality, like chat servers.
Future prospects and upcoming trends
With the ecosystem constantly changing, Django keeps evolving. Stack technologies like GraphQL seem increasingly relevant and likely to be integrated.
Tips and Resources for Further Learning
Recommended books, courses, and online resources
To effectively learn Django, consider resources:
- Django for Beginners by William S. Vincent
- Online tutorials on platforms like Coursera.
Tools and software for practical usage
Utilizing the following tools can enhance the learning experience:
- GitHub for version control and collaboration.
- Postman for API testing.
Preface to Django
Django is a powerful and flexible web framework that significantly eases the burden of web development, particularly for backend systems. Its design favors the rapid development of web applications and encourages clean, pragmatic design. The intention behind Django is to make the development process more efficient and the resulting applications more maintainable. By understanding the essentials of Django, developers can not only expedite their learning by focusing on core principles, but can also harness efficient practices that lead to robust applications.
What is Django?
Django is an open-source web framework written in Python that promotes an elegant and pragmatic approach to development. Initially created to manage high-traffic news sites at the Lawrence Journal-World, Django simplifies web development by providing essential components out-of-the-box, such as an Object-Relational Mapping (ORM) system, an authentication framework, and an admin interface. Django follows the "Don't Repeat Yourself" (DRY) principle, which emphasizes the need to reduce code repetition and encourage reuse.
Its rich ecosystem includes many reusable apps and solutions for common challenges found in web development. This enhances developers' capability to focus on unique and complex areas of their projects rather than reinventing standard solutions. Django suits a wide range of uses from powerful content management systems to scientific computing applications.
Key Benefits of Django:
- Efficiency: Built-in features such as admin panel save time and reduce redundancy.
- Scalability: Designed to handle large volumes of web traffic and can be scaled quickly.
- Security: Offers built-in security features to help developers create secure applications.
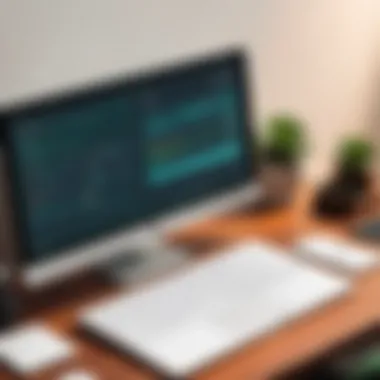
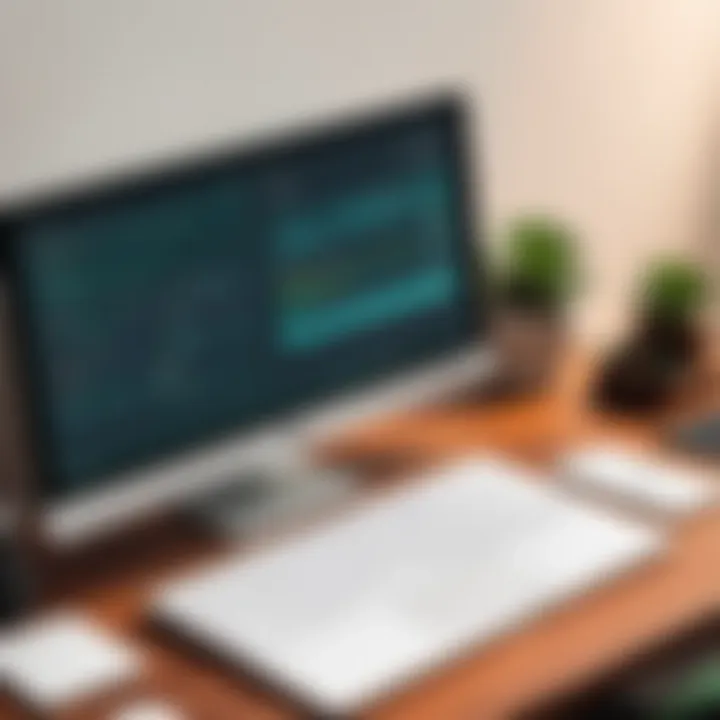
History and Evolution
Django began development in 2003 by a group of programmers seeking to build web applications more efficiently. In 2005, Django was released publicly as an open-source project. Over the years, Django has seen many versions released, each bringing enhancements, security fixes, and performance improvements.
The framework’s community has grown steadily, with contributions from many developers around the world. Key milestones in Django's evolution include:
- Django 1.x (2005-2013): Initial release which brought major capabilities to developers.
- Django 2.x (2017): Introduced several new features such as support for Python 3.
- Django 3.x (2019-present): Focuses on optimizing performance, security, and improving asynchronous support.
Django’s continued development reflects a commitment to modern web technologies and requirements. Its community actively participates in forums, for example, on reddit.com, helping find solutions to a multitude of questions and concerns.
Django has evolved into a robust framework that meets the needs of developers while maintaining simplicity and readability.
Overall, this introduction establishes a foundation for understanding what Django is, its historical context, and its relevance in modern web development. The following sections will further delve into its architecture, features, and capabilities that can enhance any project.
Django Framework Architecture
Django’s architecture separates the application into distinct layers, enhancing maintainability and scalability. This structure is known for its MTV pattern, which corresponds to its components: Models, Templates, and Views. An understanding of the architecture is critical as it influences the design and functionality of the entire project.
The architectural design of Django has many benefits tailored for developers. It promotes a clean and deliberate separation of logic and data, which simplifies future updates or changes in the application framework. Also, architecture implicates how not just data is structured, but the performance of the application itself, important for scalability concerns.
A developer's duty is to grasp these components within Django framework architecture to fully leverage the potential features of this technology, leading to a more robust application development process.
MTV Pattern Explained
The MTV pattern, integral to Django, stands for Models, Templates, and Views. Each component plays a vital role in facilitating efficient data management, rendering dynamic content, and controlling the flow of data. This pattern is differentiating, notably separating how Django is labeled from the usual MVC model, (Model-View-Controller).
- Models are responsible for managing the data and business logic. They handle the information conveyed to other components, guiding how the data is structured and retrieved.
- Templates present information to users. They contain static data and dynamic information pulled from the database, allowing a fluid interface.
- Views act as a bridge, dictating what data is displayed and the interaction between models and templates.
Components of Django
Models
Django’s Models represent the motive force for data management within the application. They enable developers to can create complex data structures using classes. This allows for clean and organized coding, as business logic is kept straightforward with Little boilerplate code. A key characteristic of models is their integration with the Object-Relational Mapping (ORM) system. This feature aids in object-oriented data handling while managing the backend database.
Benefits of models include
- Enhancing code clarity as data is modelled as Python classes.
- Simplifying data migration and modifications with built-in functions.
- Facilitating data validations upon save.
Subsequently, a drawback is the level of abstraction, which can sometimes confuse beginners regarding how queries correspond to data in SQL.
Templates
Templates in Django shape the user experience through their facilitative role in rendering data. They offer a way to design the structure or presentation of HTML files—the display level of Django’s MTV framework. Due to the separation of design from functionality, they allow front-end developers to apply their creativity without interfering with back-end operations.
The ability to embed data within template files greater organizes complicated views. They avaoid redundancy through functionalities such as the template inheritance system, which permits reusing the layout for multiple templates. A downside to consider is the learning curve involved in mastering Django’s templating language, especially for those unfamiliar with its syntax.
Views
The Views serve as the connective tissue in the MVC, maintaining dialogue between templates and models. They receive web requests, query information from models, and decide which template to present. This crucial role centralizes how data flows through the application, maintaining responsibility for tasks including response generation and modification of images.
Django’s view functions can be contrasted with a function-based view and modern class-based views, which promote cleaner code. Views enhance controller characteristics while allowing users straight-forward path definitions and handling of HTTP verbs.m However, the complexity of larger applications can introduce challenges in tracking logic dispersion over multiple views.
Setting Up Django
Setting up Django is a foundational step in building web applications using this powerful framework. Every setting step encompasses vital installation procedures, initial configurations, and project generation methods. Proper setup not only ensures that the development environment is adequately prepared but also significantly impacts the overall development experience and efficiency.
An incorrect setup can lead to various difficulties later, creating unnecessary hurdles that affect project timelines and deliverables. This attire focuses on providing developers with the settings knowledge that will serve as the base for effective Django application building and management.
Installation Guide
Installing Django starts with ensuring that certain prerequisites are met. An adequate environment, specifically Python, should be already installed. Sub sequentially, one can utilize pip, Python's package installer, to download and install Django. The command is straightforward:
Verify the installation is successful by running this command:
If Django is correctly installed, it will return the current version number. It’s always beneficial to ensure you are using a version that is compatible with your other packages and reflects the latest features available. Always later topic installations like a virtual environment (using ) is advised.
A virtual environment helps manage django projects separate from the system-wide Python installation. Users can create these environments efficiently:
Configure your terminal to activate the environment by using the corresponding commands for your operating system.
This banner is mandatory for managing dependencies and avoiding conflicts between project packages.
Creating Your First Project
Once Django is installed, the next logical step is the creation of your first project. Running the following command assists in scaffolding a new matter of project:
To navigate into your newly created directory, use:
After navigating to the project directory, running the local development server is crucial to ensure everything runs flawlessly. Use the following command to start the server:
Head to your browser and access to see the default Django welcome page. It verifies that the initial setup has been successfully laid out. Keep in mind, setting up additional applications within the project is straightforward with the command.
Creating a new application requiresengaging out the controller method in the root folder of the django project folder:
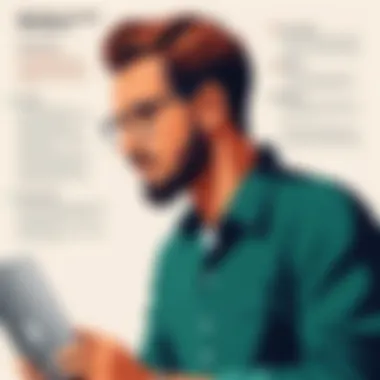
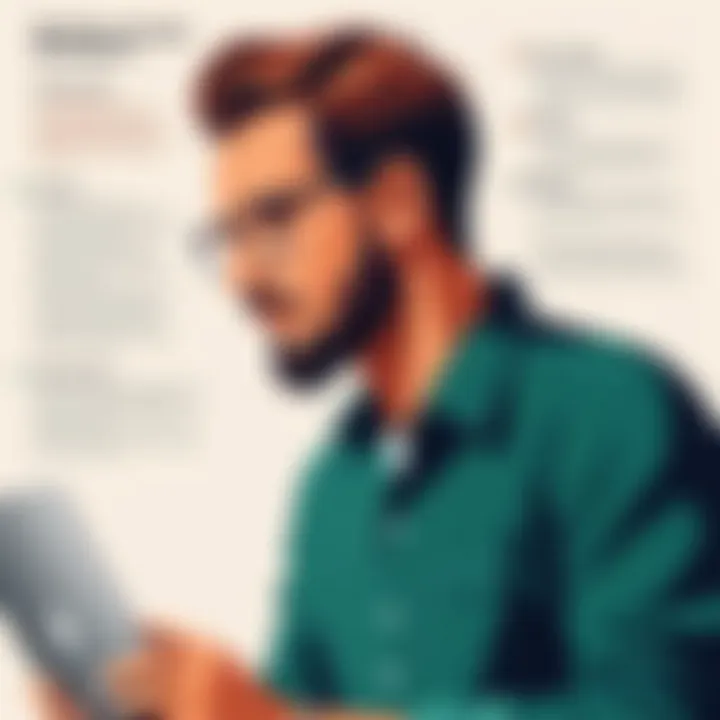
With this simple and efficient process, the user can begin crafting a fully functional web application with Django and gradually build scalable solutions that meet various user needs. For those who decide to coordinate their project closely with versioning systems, these elements of development will give immense benefits in testing and deployment stages significantly.
By adhering to these guidelines when setting up Django, developers can lay a robust foundation for effective and efficient application development.
Core Features of Django
Django's architecture offers an array of core features that significantly simplify web development. Understanding these functionalities is essential not just for beginners but also for seasoned developers. Each feature contributes to enhancing productivity, ensuring security, and maintaining a clean structure in code. An overview of the core features reveals not only the advantages they bring but also considerations developers must keep in mind while working on their projects.
Admin Interface
One of the standout features of Django is its automatic admin interface. It allows developers to create a functional and customizable administrative section without much effort. This admin panel provides a user-friendly interface to manage content and various models in applications.
With the admin interface, tasks such as data entry, updates, and management become streamlined. This aspect is particularly beneficial for smaller projects and startups, where resources may be limited. Additionally, developers can modify the appearance and functionality through custom templates and permission settings. An interesting fact is that the victory of Django lies in enabling even non-technical users to manage databases effectively. Incorporating features like search functionality and filters make it quite powerful in handling data quickly.
ORM Capabilities
Django's Object-Relational Mapping (ORM) capabilities distinguish it from many frameworks. With ORM, developers interact with databases by using Python classes. The framework abstracts complex SQL commands into Python methods and lets developers define models in a readable manner.
This offers several advantages: ease of use, maintainability, and security. Developers can focus on business logic instead of getting bogged down by the specifics of SQL queries. Another important benefit includes the automatic migration feature, which makes database schema changes easier over time. Still, one must pay attention to queries’ performance, especially when dealing with larger datasets, as abstracting too much complexity could lead to inefficiencies.
URL Routing
Django employs a straightforward URL dispatching mechanism. This feature allows developers to design readable and organized URL paths corresponding to the appropriate views. This organization enables user-friendly navigation and contributes to an SEO-friendly structure.
Creating a clean URL routing system is fundamental for any web application. It helps in search engine visibility while offering a clear path for users. Developers need to be meticulous in designing these routes; defining a consistent and logical pattern is crucial. A weak routing system could hinder both user experience and search index crawling. Thus, properly structured URL patterns are essential to a successful Django application.
Forms Handling
Forms are an integral part of web applications, and Django streamlines the process of managing these forms through its robust system. Django’s form handling includes validation, error handling, and rendering, allowing developers to concentrate on gathering user input rather than scripting repetitive tasks.
One can define forms as Python classes which map directly to model fields. Handling validation and errors is seamless; built-in features can significantly minimize repetitive wroteback of logic. Among the advantages of this robust form processing are security features, such as built-in protection against Cross-Site Request Forgery (CSRF). However, familiarity with its functionalities is important. Account should be taken for response times and big forms as rendering a large number of fields can sometimes become cumbersome.
Django's core features ultimately encapsulate how it promotes rapid web development both in functional and efficient manners. Understanding these tools is imperative for achieving well-constructed and maintainable code in the long-run.
Django and REST APIs
Django has been embraced by developers worldwide for its robust structure and flexibility. Among its notable capabilities is the seamless integration with RESTful APIs. The significance of understanding Django and REST APIs lies in the ongoing demand for data exchange between the server and client applications. REST (Representational State Transfer) APIs allow diverse systems to communicate over HTTP. This communication is not unconventional, yet impactful in many applications today. Without RESTful integration, one would face limitations in building scalable web services.
Building a RESTful API
In a technical sense, the process of building a RESTful API with Django operates on fundamental principles designed for API architecture. Understanding the three primary things is crucial: resource representation, stateless interactions, and HTTP methods.
- Resource Representation: Resources in a REST API can appear in various formats such as JSON or XML. Choosing JSON is often favorable due to its simplicity and ease of use with modern web applications.
- Stateless Interactions: Every request must contain all information needed for the server to fulfill it. This aspect fundamentally allows scaling efficiently, as no session data is stored on the server.
- HTTP Methods: Familiarity with the HTTP methods is essential. We typically work with GET, POST, PUT, PATCH, and DELETE to operate on resources.
To initiate the actual coding process, defining the views in Django is a common starting point. Below is a basic example of how you might set up a simple API endpoint for getting and creating books:
This snippet represents a viewset that includes methods for accessing and modifying book resources, enhancing the interaction patterns typical of REST APIs.
Using Django Rest Framework
Employing the Django Rest Framework (DRF) simplifies the process. DRF comes with ready-to-use resources that expedite RESTful API development. It handles much of the boilerplate, allowing developers to focus on customization and business logic instead of repetitive tasks.
Incorporating DRF involves several steps:
- Installation: Start by installing the DRF package using pip:
- Configuration: Add to your INSTALLED_APPS setting in Django's settings.py file.
- Serializer Management: DRF requires explicit definition of serializers. This process transforms complex data types, like Django Querysets, into Python data types that can then be rendered into JSON.Here is a simple BookSerializer example:
- URLs and Documentation: Properly setting up URLs in Django will ensure your API endpoints are discoverable. DRF also has built-in features for API documentation, enhancing usability for third-party developers who may need to interface with your services.
A blend of the Django Rest Framework with well-structured data-save logic and patterns offers utimate synergy in application development.
Best Practices in Django Development
Best practices play a critical role in Django development. They contribute to building applications that are not only efficient and scalable but also secure and maintainable. Adhering to best practices helps developers to streamline their workflow, alleviate common pitfalls, and enhance the performance and user experience of their projects. In this section, we will explore key elements related to project structure, security considerations, and code reusability.
Project Structure
A well-organized project structure is foundational in any software development. In Django, this involves an arrangement that facilitates understanding, enhancing, and scaling the application effectively. When structuring a Django project, it is important to embrace the capabilities of the framework. Keep in mind:
- Separate Concerns: Organize files into clear sections. Typically, you can have directories for apps, static files, templates, and media. This separation supports easier navigation.
- Consistent Naming Conventions: Naming conventions should be logical and intuitive. For instance, app names should reflect their functionality. Following consistent patterns aids in maintainability.
- Modular Design: Make each app as independent as possible. This promotes reusability and simplifies testing.
Proper structure extends beyond mere aesthetics. It encourages collaborative work, as different team members can easily find what they need.
Security Considerations
Security must be a priority in today’s web applications. As a developer, understanding Django’s security features is vital to mitigate risks and protect sensitive data. Here are essential considerations:
- Use Django’s Built-In Security Features: Utilize tools like the CSRF protection provided by Django and make use of the built-in authentication system to handle user management.
- Keep Dependencies Updated: Regularly update third-party libraries to patch vulnerabilities. Outdated packages can be gateways for attacks.
- Sensitive Information Management: Store sensitive information such as personal data, API keys, and database credentials securely. Avoid hardcoding these in source files using management tools.
By prioritizing these aspects, developers can ensure a more secure environment, substantially lowering the risk of data breaches.
Code Reusability
The capability to reuse code is one of the significant advantages when developing with Django. Fostering reusability can expedite the development process and improve code quality. Consider the following strategies to enhance code reusability:
- Create Reusable Apps: Django’s app structure allows for the easy creation of reusable components. Developing modular apps can often lead to their adoption in other projects.
- Utilize Custom Template Tags and Filters: These provide powerful ways to increase the functionality of your templates while avoiding repetitive code throughout your application.
- Employ Django’s Mixins: Mixins are classes that provide methods for reuse across class-based views. They help avoid duplication and can simplify the process.
Investing time in planning for reusability pays off. It not only reduces the amount of code written, but also offers quick iterations and feature updates in projects over time.
Important Note: Always ensure that any reusable code follows best practices for security and performance.
Testing in Django
Testing is a crucial aspect of Django development. It ensures reliability, aids in debugging, and promotes confidence in the codebase. In this section, we will explore the different testing methods Django provides. This includes unit testing and integration testing, each bringing its specific benefits and considerations.

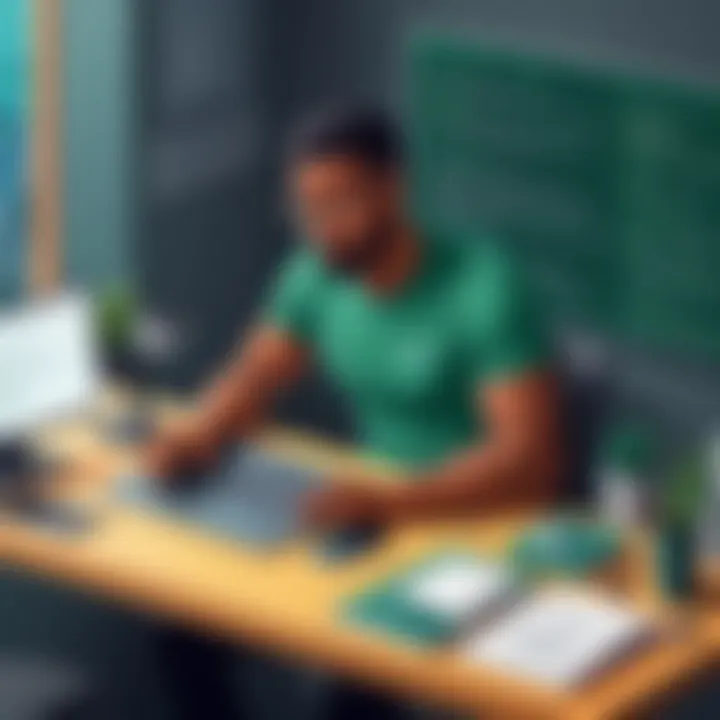
Unit Testing
Unit testing in Django focuses on validating the smallest parts of an application, typically individual functions or methods. The primary aim is to check if each unit performs as expected, working independently of other components. Unit tests provide several advantages:
- Early Bug Detection: Catching errors at an early stage reduces the chances of future complications.
- Code Confidence: Developers can refactor code with assurance, knowing there are tests covering critical functionalities.
- Documentation: Unit tests serve as a form of documentation that outlines how specific components should behave.
Creating unit tests in Django is straightforward. Utilizing Django’s built-in testing framework is an effective start. Here is a basic example:
This code defines a simple test case. It verifies that the addition operation produces the correct sum. It is important to run your tests routinely during development. This habit will cultivate robust applications and ensure reliability.
Integration Testing
Integration testing examines how different components of the application work together. Unlike unit testing, it tests interactions between various modules. The goal is to identify issues that may arise when different pieces integrate, covering a broader aspect of functionality.
Integration tests in Django can help verify:
- Correct Component Interaction: Checks if modules or functions are working in tandem as expected.
- Database Interactions: Validates if models engage with views and templates successfully during user operations.
- API Endpoints: Tests if they return the expected responses under various conditions.
A simple example of an integration test in Django might look like:
In this snippet, we are simulating a user accessing a view and checking that the response is as expected. Integrating these tests ensures adherence to project specifications as the application evolves.
Effective testing methods in Django enhance code reliability and support efficient debugging efforts. Ensuring rigorous unit and integration tests means greater robustness within applications.
Deploying Django Applications
Deploying applications built with Django requires meticulous planning and execution. Understanding the subtleties of deployment facilitates smooth transitions from development environments to production settings. This section emphasizes essential elements, benefits, and considerations involved in deploying Django applications. Successful deployment ensures not just the function of the application but also strengthens security and performance, which are critical when serving users.
Deployment Options
When it comes to deploying Django applications, there are several options available, each with its own advantages and considerations. Some of the notable deployment options include:
- Cloud Services: Utilizing cloud providers like Heroku, AWS Elastic Beanstalk, or Google Cloud Platform allows for scalable solutions tailored-fit. These services offer various features that support monitoring, load balancing, and automated scaling.
- Dedicated Servers: For full control, developers can opt for dedicated server solutions. This requires server management, but it provides flexibility in configuring the environment to specific requirements.
- Platform-as-a-Service (PaaS): Employing a PaaS like Dokku or PythonAnywhere can simplify deployment. They streamline the deployment process while also managing common operations like resource scaling and server monitoring.
Considerations in this section should include ease of integration with existing tools, cost implications, and required administrative efforts. Each deployment option serves different use cases, so selecting the one that aligns with the project needs is paramount.
Using Docker
Docker has gained significant traction as a deployment tool for Django applications. Containerization allows developers to package applications and all dependencies in a standardized format, leading to more consistent deployment environments.
Benefits of using Docker include:
- Environment Consistency: Containers ensure that the application runs the same, irrespective of where it is hosted. This eliminates the “it works on my machine” dilemma.
- Scalability: Docker makes it easier to scale applications through its efficient resource allocation and isolation of processes allowing multiple services to run anywhere.
- Dependency Management: All required dependencies are contained inside the Docker image, reducing conflicts between system-wide dependencies.
To deploy a Django application using Docker, a sets the parameters for a container. Here is a simple example of my web app:
Using Docker will assist developers not only during deployment but also in rapid development workflows. Thus, enhancing productivity while decreasing the potential για issues during production.
Correct deployment can significantly enhance application performance, security, and user experience. Always evaluate your options before deciding on a strategy for deployment.
Common Pitfalls and How to Avoid Them
Understanding common pitfalls in Django development is essential for both novice and seasoned developers. The framework simplifies many tasks but can also lead to missteps if not approached mindfully. Being aware of these hazards is imperative for creating efficient and secure applications.
Performance Issues
Performance can be severely impacted by overlooked design considerations in Django. Common performance issues often arise from poor database queries, unoptimized middleware, or inefficient template rendering.
In particular, the use of Database QuerySet evaluations and intricate joins can slow down responses. When a QuerySet is not properly formulated, it matters a lot. Caching strategies are fundamental to improve speed and efficiency. Certain features like or can optimize database access.
- Avoid executing nested loops, which lead to multiple queries and slow performance.
- Utilize management commands to check and fix performance bottlenecks regularly.
- Profiling tools like Django Debug Toolbar can unveil hidden inefficiencies.
In summary, monitoring and optimizing database interactions is crucial to maintain responsive applications.
Debugging Challenges
Debugging can be quite tricky in Django, especially without structured approaches. Many developers rely simply on error messages displayed on the page without digging deeper. This behavior can result in overlooking deeper application issues.
On top of this, static file serving in Debug mode might present challenges as well—serving files correctly becomes paramount in varying environments. Missing template context variables tend to sabotage expected results, creating confusion during testing.
- Use logging strategically to capture clarification on issues as they occur.
- Leverage or other debugging tools to pause execution and inspect runtime states.
- Regularly write and run tests, both unit and integration, to preempt avoidable errors.
Seeking patterns in past experiences can significantly reduce debugging hurdles. Identifying wrong assumptions early can further instill a strong foundation in application stability.
Great understanding of potential pitfalls and employing concrete strategies can save time in the development cycle and lead to successful projects.
Future of Django Development
The future of software development has become increasingly influenced by the tools available, and Django stands at the forefront of this transformation. Its relevance remains paramount due to several factors. Firstly, the technology landscape is always changing, with advancements introducing new methodologies and practices. Django's flexibility allows developers to adapt and evolve with these trends, ensuring that applications built with this framework remain viable in a competitive market.
Additionally, the demand for rapid and scalable web solutions continues to increase. Businesses want streamlined processes that lead to high-performing applications. Django’s distinct features cater to these needs, enhancing its attractiveness for future projects. In this context, understanding the future trends and community involvement in the development of Django code is critical.
Emerging Trends
The development landscape is witnessing several trends that signal the direction Django might take. One prominent trend is the integration of machine learning capabilities into web applications. Developers are increasingly looking to incorporate intelligent features within their projects. With libraries such as TensorFlow and PyTorch becoming available, Django can serve as the backbone of applications that require advanced processing power and methodologies.
Another noticeable trend is the rise of microservices architecture. By breaking down applications into smaller, independent services, developers improve scalability and maintanability. Django aligns well with this architecture through its view and template system, allowing each microservice to function distinctly while still leveraging Django's robust functionality.
Mobility is also a critical factor. There’s a powerful shift towards making web applications mobile-friendly. responsive designs and APIs can stood up using Django enable applications to meet the needs of mobile application developers quickly.
Community Contributions
The strength of Django lies significantly within its community of developers and contributors. The ongoing support and collaboration in the open-source environment fuels innovation and reliability in the framework. Community contributions can best be understood through major forms such as
- Documentation: Continuous enhancements made to the project's online resources make it easier for newcomers to grasp the concepts and functionalities of Django.
- Plugins and Packages: Many third-party packages are tailored for specific use cases, making it more flexible and customizable. Contributions in this area lead to the expansion of the framework’s capabilities types of projects it can effectively handle.
- .organized events: Training sessions, workshops, and annual conferences, like DjangoCon, allow members to meet, share knowledge, and foster collaboration, all driving forward the direction Django development takes.
Community-driven feedback and iterations often introduce more robust security practices and facilitate integration of new technologies, keeping Django a leading choice for developers in evolving circumstances.
Understanding these elements will not only assist current users but also reinforces a community that welcomes innovative programming practices necessary for fruitful extension and promising projects in the future.