Mastering Java Editing: Advanced Techniques and Best Practices for Developers
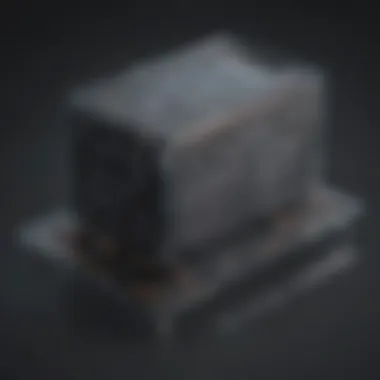
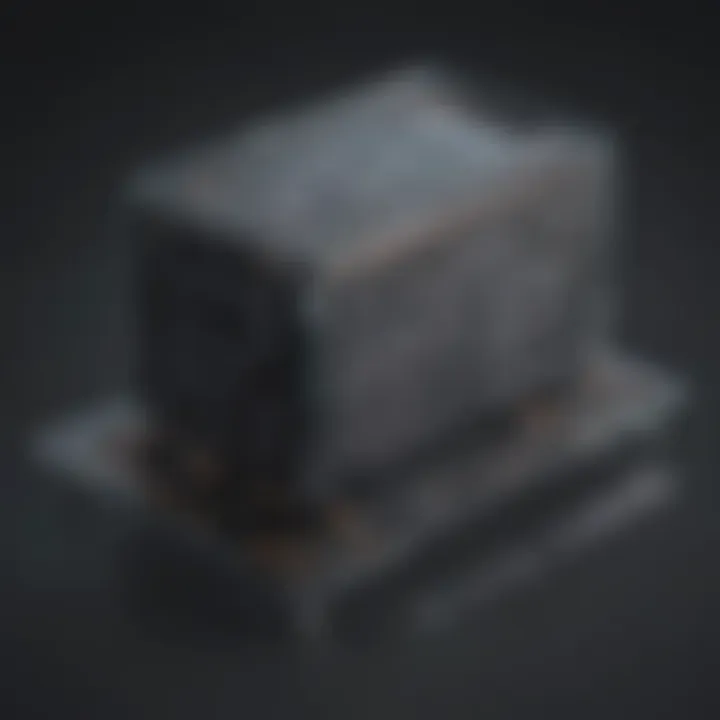
Overview of Topic
Java editing is a fundamental aspect of software development, crucial for both novice and experienced programmers. Understanding Java editing techniques and best practices is essential in ensuring efficient code optimization and effective debugging processes. As Java remains a prominent programming language in the tech industry, the skills related to Java editing hold significant relevance in software development.
Fundamentals Explained
To grasp the essence of Java editing, developers need a firm grasp of core principles and theories underlying the process. Key terminologies like syntax, variables, and methods play a vital role in Java editing. Delving into basic concepts such as loops, conditional statements, and data structures is crucial for foundational knowledge in Java editing.
Practical Applications and Examples
Real-world case studies offer practical insights into the applications of Java editing techniques. Demonstrations depicting debugging processes or code optimization strategies provide hands-on experience for developers. Code snippets showcasing efficient programming practices and implementation guidelines serve as valuable resources in mastering Java editing.
Advanced Topics and Latest Trends
In the rapidly evolving tech landscape, staying abreast of cutting-edge Java editing developments is imperative. Advanced techniques like multithreading, lambda expressions, and design patterns elevate programming proficiency. Exploring the latest trends in Java editing sheds light on future prospects and upcoming methodologies in software development.
Tips and Resources for Further Learning
For individuals seeking to enhance their Java editing skills, recommended books, online courses, and tools are abundant resources. Educational materials focusing on Java best practices, debugging strategies, and code optimization offer comprehensive learning avenues. Leveraging software applications tailored for Java development enhances practical usage and proficiency in the field.
Introduction To Java Editing
In the realm of Java development, editing plays a crucial role in ensuring efficient coding practices, bug resolution, and overall program readability. It serves as the foundation for optimizing Java code and debugging applications effectively. Understanding the nuances of Java editing is essential for both novice and experienced developers seeking to enhance their coding skills and produce high-quality software solutions.
Understanding the Importance of Java Editing
Efficiency in Coding
Efficiency in coding is a cornerstone of Java editing, emphasizing the importance of writing clean, concise, and performant code. By focusing on efficient coding practices, developers can streamline the development process, reduce complexity, and enhance the maintainability of their codebase. The ability to write efficient code not only improves productivity but also contributes to overall program performance and scalability. However, it requires careful consideration of algorithmic efficiency, data structures, and code organization to achieve optimal results.
Bug Identification and Resolution
The process of bug identification and resolution is a fundamental aspect of Java editing, allowing developers to detect and fix errors in their code effectively. By employing debugging tools and techniques, programmers can pinpoint issues, trace their origins, and implement timely solutions. Bug resolution is essential for maintaining code quality, ensuring functionality, and delivering reliable software products. However, thorough testing and debugging practices are necessary to address bugs comprehensively and prevent potential pitfalls in the development cycle.
Enhancing Readability
Enhancing readability is a key facet of Java editing that focuses on making code more understandable, maintainable, and accessible to other developers. By following consistent formatting guidelines, utilizing appropriate naming conventions, and documenting code effectively, programmers can facilitate collaboration, reduce errors, and improve code comprehension. Code readability not only enhances the overall quality of software projects but also simplifies troubleshooting, refactoring, and future enhancements. It is an essential component of code maintenance and plays a crucial role in the long-term success of Java applications.
Tools for Java Editing
Integrated Development Environments (IDEs)
Integrated Development Environments (IDEs) are comprehensive software applications that provide developers with tools for writing, testing, and debugging code. IDEs offer features such as syntax highlighting, code completion, and project management, enhancing productivity and streamlining the development workflow. By integrating various tools into a unified environment, IDEs empower programmers to write code efficiently, collaborate with team members, and leverage advanced debugging capabilities. However, the choice of IDE can significantly impact a developer's workflow, requiring consideration of factors such as compatibility, performance, and extensibility.
Code Linters
Code linters are static analysis tools that help identify potential errors, inconsistencies, and style violations in codebases. By enforcing coding standards, code linters promote uniformity, readability, and best practices across projects. They facilitate early error detection, code refactoring, and adherence to coding guidelines, improving code quality and maintainability. While code linters offer valuable insights into code quality, they can sometimes be rigid in their recommendations, requiring developers to balance automatic corrections with manual code reviews and contextual considerations.
Debuggers
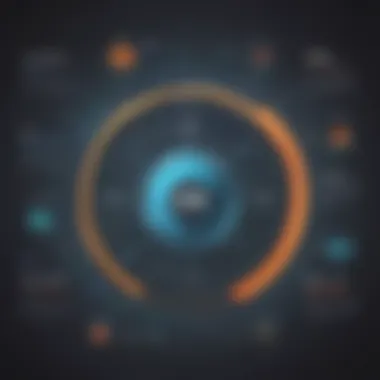
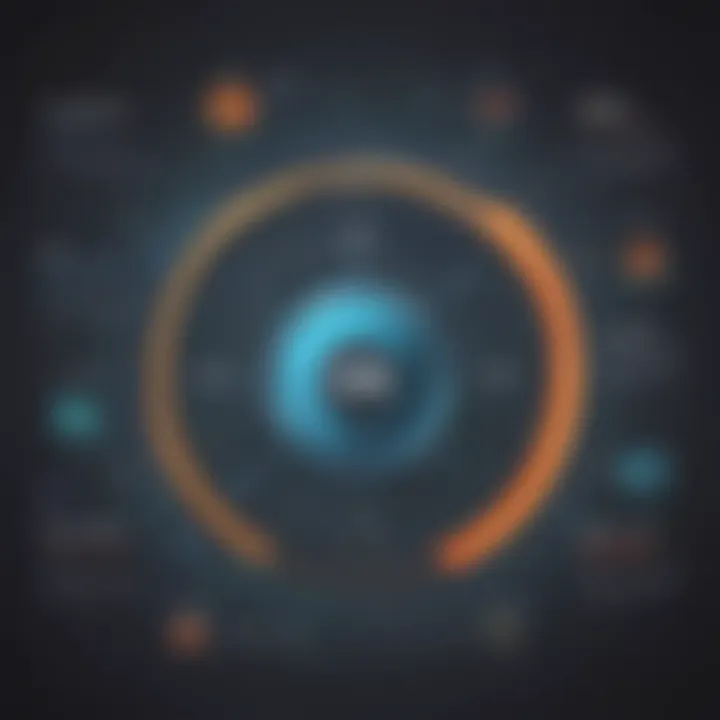
Debuggers are specialized tools that allow developers to inspect, manipulate, and test code during runtime, enabling them to identify and resolve issues efficiently. By setting breakpoints, watching variable values, and stepping through code execution, debuggers provide insights into program behavior and facilitate error diagnosis. Debugging is essential for troubleshooting complex logic, handling edge cases, and verifying program correctness, ensuring the robustness and reliability of Java applications. While debuggers offer powerful features for software analysis, mastering their functionalities and interpreting output data can require expertise and practice.
Common Challenges in Java Editing
Syntax Errors
Syntax errors are common pitfalls in Java editing that arise from incorrect language syntax or structural rules. Resolving syntax errors is essential for compiling code successfully and executing programs without runtime issues. By paying attention to error messages, utilizing IDE features, and understanding language specifications, developers can address syntax errors promptly and prevent coding setbacks. However, identifying and fixing syntax errors may require meticulous code review, testing, and familiarity with Java syntax rules to ensure code correctness and reliability.
Performance Bottlenecks
Performance bottlenecks in Java editing refer to inefficient code segments that impact program execution speed, memory usage, or resource consumption. Detecting and optimizing performance bottlenecks is crucial for enhancing program efficiency, scalability, and user experience. By profiling code, analyzing algorithm complexity, and implementing optimization strategies, developers can eliminate bottlenecks, improve application performance, and optimize resource utilization. Addressing performance bottlenecks requires a deep understanding of software optimization techniques, platform considerations, and performance testing methodologies to deliver high-performing Java applications.
Code Refactoring
Code refactoring is a vital practice in Java editing that involves restructuring code without changing its external behavior. Refactoring aims to improve code quality, readability, and maintainability by eliminating redundancy, enhancing clarity, and simplifying complex code constructs. By breaking down large methods, extracting reusable components, and organizing code logically, developers can enhance code reusability, extensibility, and scalability. Code refactoring is an iterative process that requires care, attention to detail, and thorough testing to ensure that code changes do not introduce new bugs or regressions.
Optimizing Java Code
In this section, we will delve deep into the importance of optimizing Java code within the context of this comprehensive article on Java editing techniques and best practices. Optimizing Java code is crucial for enhancing overall system performance, reducing resource consumption, and improving the maintainability of codebases. By focusing on specific elements such as code refactoring, implementing efficient design patterns, and fine-tuning performance strategies, developers can significantly elevate the quality and efficiency of their Java applications.
Code Refactoring Techniques
Extract Method
When it comes to the Extract Method technique within the realm of Java code optimization, its core essence lies in breaking down complex procedures into smaller, more manageable functions. This approach not only enhances code readability but also promotes code reusability and modularity. Extracting methods allows for the isolation of specific functionalities, facilitating easier testing and maintenance. While Extract Method is a popular choice for its ability to streamline code structure, developers should be mindful of potential drawbacks, such as increased method count and complexity.
Rename Variables
The concept of renaming variables plays a vital role in code clarity and expressiveness. By giving variables more descriptive and meaningful names, developers can create code that is easier to understand and maintain. Renaming variables can also prevent confusion and reduce the likelihood of errors. This practice is particularly valuable in collaborative development environments where multiple programmers may interact with the same codebase. However, excessive renaming can lead to code churn and potentially introduce new bugs, necessitating a balanced approach.
Remove Duplicate Code
Eliminating duplicate code snippets is a fundamental aspect of code optimization in Java. Removing redundancy not only improves code readability but also reduces the risk of inconsistencies and errors. By centralizing common functions and routines, developers can ensure that updates and modifications are applied uniformly across the codebase. However, caution must be exercised to avoid over-generalization, which can obscure code logic and hinder maintainability. Balancing code consolidation with unique contextual requirements is key to leveraging the benefits of removing duplicate code effectively.
Implementing Design Patterns
When it comes to implementing design patterns in Java development, a pivotal aspect of code optimization, developers gain access to proven solutions for recurring design challenges. Design patterns such as Singleton Pattern, Factory Method Pattern, and Strategy Pattern offer established blueprints for structuring code in a way that enhances flexibility, scalability, and maintainability. By understanding the unique characteristics and applications of each design pattern, developers can make informed decisions about when and how to implement them in their projects.
Singleton Pattern
The Singleton Pattern, a widely utilized design pattern in Java programming, focuses on ensuring that a class has only one instance and provides a global point of access to that instance. This pattern is valuable in scenarios where a single shared resource needs to be managed across multiple components. While Singleton Pattern simplifies resource management and promotes a centralized control mechanism, improper implementation can lead to issues such as concurrency challenges and tight coupling. Therefore, careful consideration is essential when incorporating the Singleton Pattern into Java applications.
Factory Method Pattern
The Factory Method Pattern serves as a creational design pattern that defines an interface for creating objects but delegates the instantiation process to subclasses. This approach empowers developers to encapsulate object creation logic and customize the instantiation of specific types of objects. The Factory Method Pattern enhances code maintainability, extensibility, and testability by decoupling object creation from client code. However, excessive fragmentation of object creation logic and intricate subclass hierarchies can complicate the application's structure, underscoring the importance of judicious application of this pattern.
Strategy Pattern
The Strategy Pattern is a behavioral design pattern that enables dynamic selection of algorithms at runtime. By defining a family of interchangeable algorithms and encapsulating them within separate classes, the Strategy Pattern promotes flexibility and reusability in code design. Implementing the Strategy Pattern empowers developers to switch between algorithms seamlessly, without altering the client code structure. While the Strategy Pattern fosters code adaptability and simplifies algorithm maintenance, improper implementation can introduce overhead due to class proliferation. Adhering to a well-structured hierarchy of strategies and selecting appropriate granularity levels are essential considerations when leveraging the Strategy Pattern in Java applications.
Performance Tuning Strategies
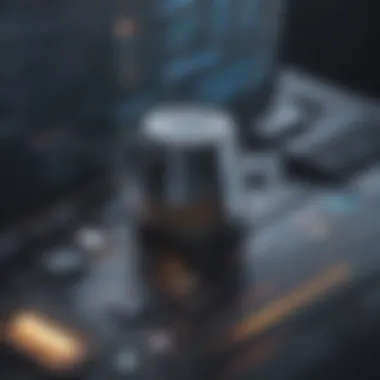
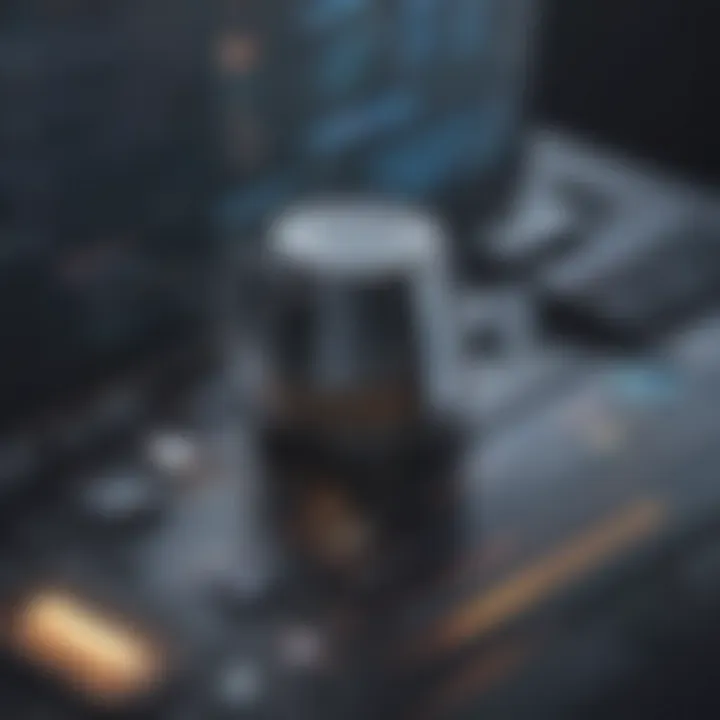
Optimizing performance in Java applications requires a strategic approach to selecting efficient data structures, implementing optimized algorithms, and managing memory effectively. By adopting performance tuning strategies tailored to the specific requirements of their projects, developers can mitigate bottlenecks, enhance execution speed, and optimize resource utilization.
Efficient Data Structures
The choice of data structures significantly impacts the performance and scalability of Java applications. Selecting efficient data structures, such as hash maps, arrays, and trees, can optimize memory usage and access times, thereby enhancing overall application efficiency. By assessing the data access patterns and operational requirements of their applications, developers can leverage appropriate data structures to boost performance effectively.
Optimized Algorithms
Utilizing optimized algorithms is paramount in achieving high-performance benchmarks in Java programming. By employing algorithms with minimized time complexity and space complexity, developers can expedite processing tasks and reduce computational overhead. Optimization techniques such as algorithmic caching, parallel processing, and algorithmic pruning can further enhance algorithm efficiency. However, developers must strike a balance between algorithm optimization and code readability, ensuring that performance enhancements do not compromise code maintainability.
Memory Management
Efficient memory management is critical for maximizing the performance and stability of Java applications. By monitoring memory allocation, garbage collection processes, and object lifecycle management, developers can prevent memory leaks, optimize memory utilization, and minimize application downtime. Incorporating best practices such as object pooling, weak references, and memory profiling tools can provide insights into memory consumption patterns and help identify optimization opportunities. However, meticulous attention is required to prevent memory-related issues such as fragmentation, out-of-memory errors, and excessive GC overhead.
This detailed exploration of optimizing Java code through code refactoring techniques, design pattern implementation, and performance tuning strategies equips developers with essential insights and strategies to elevate their Java development skills. By integrating these optimizing practices into their coding workflows, developers can cultivate efficient, scalable, and maintainable Java applications that meet the evolving demands of modern software development.
Debugging Java Applications
Debugging Java Applications is a crucial aspect of Java development. It is essential in identifying and rectifying errors and issues within the codebase. By utilizing debugging tools effectively, developers can streamline the debugging process and enhance the overall efficiency of the development workflow. This section will delve deep into the significance of debugging Java applications, emphasizing key elements such as error detection, problem-solving, and code optimization.
Utilizing Debugging Tools
Breakpoints
Breakpoints are key markers within the code where execution halts to allow developers to inspect the program's state. They play a pivotal role in isolating issues, understanding program flow, and analyzing variables at specific points in the code. The distinctive feature of breakpoints lies in their ability to pinpoint exact locations within the code for thorough examination, enabling developers to troubleshoot efficiently and make informed decisions. While breakpoints offer precise control over program execution, they may introduce some overhead in terms of runtime performance.
Watchpoints
Watchpoints are specialized breakpoints that trigger when a specific variable's value changes. This feature is instrumental in monitoring variables dynamically, aiding in tracking down data inconsistencies and identifying unexpected behavior. The uniqueness of watchpoints lies in their real-time observation of variable modifications, providing valuable insights into code execution and helping developers trace the root cause of bugs effectively. However, excessive use of watchpoints may lead to performance degradation due to continuous monitoring overhead.
Interactive Debugging
Interactive Debugging offers a dynamic and hands-on approach to debugging, allowing developers to modify code on the fly during runtime. This technique empowers developers to test hypotheses, explore solutions interactively, and adapt the codebase in real-time. The key characteristic of interactive debugging is its agility and responsiveness, enabling developers to iteratively refine their solutions without interrupting the debugging process. While interactive debugging fosters a creative and flexible debugging environment, improper use may introduce unintended side effects and complicate the debugging process. The interactive nature of this approach encourages active engagement with the codebase, promoting a deep understanding of the application's behavior and logic.
Handling Runtime Errors
NullPointerExceptions
NullPointerExceptions occur when a program tries to access or manipulate a null object reference. These errors often signify logical flaws in the code, highlighting incomplete object initialization or improper exception handling. By addressing NullPointerExceptions effectively, developers can enhance the overall robustness and reliability of the application. The key characteristic of NullPointerExceptions is their explicit indication of null references, aiding developers in pinpointing the root cause of the issue promptly. However, handling NullPointerExceptions requires meticulous error checking and defensive programming practices to prevent unforeseen consequences in the codebase.
ArrayIndexOutOfBoundsException
ArrayIndexOutOfBoundsException signifies an attempt to access an index beyond the bounds of an array. These errors typically stem from incorrect index calculations or improper array boundary checks. By addressing ArrayIndexOutOfBoundsException, developers can fortify the code against array-related pitfalls and ensure seamless array operations. The unique feature of ArrayIndexOutOfBoundsException lies in its direct correlation to array manipulation, enabling developers to enforce strict index validations and bolster array access controls. However, mitigating ArrayIndexOutOfBoundsException necessitates comprehensive array bounds verification and stringent index management to avert array manipulation errors.
ConcurrentModificationException
ConcurrentModificationException arises in concurrent programming scenarios when a collection undergoes structural modification during iteration. These exceptions indicate unsafe concurrent access to shared data structures, emphasizing the importance of thread synchronization and proper data structure management. The key characteristic of ConcurrentModificationException is its detection of concurrent access violations, highlighting synchronization gaps and thread safety vulnerabilities within the codebase. Mitigating ConcurrentModificationException demands meticulous synchronization strategies, such as using concurrent data structures or synchronized access controls, to promote thread-safe operations and prevent data corruption.
Testing and Validation Techniques
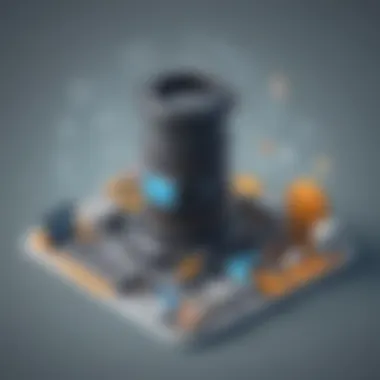
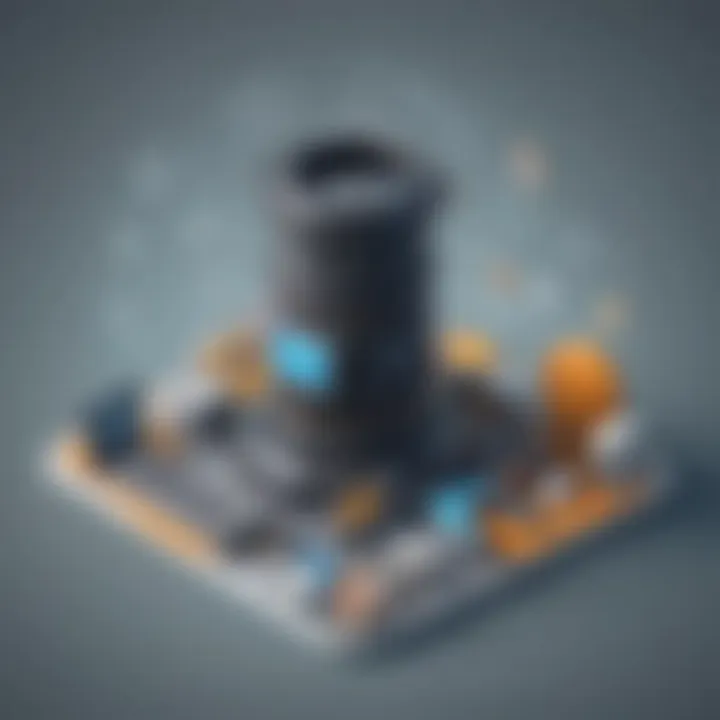
Unit Testing
Unit Testing involves testing individual units or components of the code in isolation to validate their functionality independently. This technique facilitates granular testing of key functionalities, ensuring robustness and correctness at the unit level. The key characteristic of Unit Testing is its focus on isolated testing, enabling developers to identify and address defects within specific code modules efficiently. While Unit Testing promotes code reliability and maintainability, extensive unit test suites may lead to longer test execution times and increased maintenance overhead.
Integration Testing
Integration Testing assesses the interactions and dependencies between different units or modules of the application, verifying the seamless integration of components. This testing phase evaluates the consolidated functionality of interconnected units, ensuring smooth interoperability and system-wide coherence. The unique feature of Integration Testing lies in its comprehensive evaluation of integrated components, verifying end-to-end functionality and inter-component communications. However, Integration Testing necessitates meticulous integration scenarios and data flow validations to verify inter-component interactions effectively.
Regression Testing
Regression Testing validates the unchanged behavior of the software after modifications or updates, ensuring that new code does not inadvertently break existing functionalities. This testing methodology mitigates regression risks, preserving the integrity and stability of the codebase throughout iterative development cycles. The key characteristic of Regression Testing is its focus on software stability assurance, enabling developers to detect and rectify regressions promptly. While Regression Testing safeguards against unintended code changes, managing regression test suites may require substantial effort and continuous maintenance to adapt to evolving codebases and project requirements.
Best Practices for Java Editing
Java editing requires adherence to best practices to ensure efficient and error-free coding. By following specific elements such as coding standards compliance, developers can streamline their workflow and enhance the quality of their code. Incorporating best practices leads to improved readability, maintainability, and collaboration within development teams. It is essential for Java developers to prioritize consistency in naming conventions, proper indentation, and thorough documentation to facilitate code review processes and version control integration.
Coding Standards Compliance
Consistent Naming Conventions
Consistent naming conventions play a pivotal role in Java editing by fostering clarity and organization in code structure. By utilizing a standardized approach to naming variables, classes, and methods, developers can enhance code readability and maintainability. Consistency in naming conventions simplifies the understanding of code logic for both individual programmers and collaborative teams, promoting efficient troubleshooting and debugging processes.
Proper Indentation
Proper indentation in Java code is crucial for visual clarity and logic comprehension. By correctly aligning code blocks and structures, developers can improve the overall readability and maintainability of the codebase. Proper indentation enhances code structure visualization, simplifies debugging, and accelerates the code review process by making it easier to identify logical errors or inconsistencies.
Documentation Practices
Comprehensive documentation practices are essential for effective Java editing and code maintenance. Well-documented code offers valuable insights into the purpose, functionality, and implementation of various components within the application. Documentation plays a crucial role in knowledge transfer, onboarding new team members, and ensuring code sustainability over time. By documenting code thoroughly, developers can minimize ambiguity, expedite troubleshooting, and streamline future code revisions.
Version Control Integration
Git Workflow
Git workflow implementation is paramount in modern software development practices. By utilizing Git for version control, developers can track changes, collaborate seamlessly, and effectively manage code revisions. Git's decentralized structure allows for branch-based development, enabling parallel work streams without compromising code integrity. Git workflow enhances team collaboration, facilitates code review processes, and ensures version control consistency throughout the development lifecycle.
Branch Management
Proper branch management strategies are essential for organizing code changes and facilitating collaborative development efforts. By structuring branches according to feature updates, bug fixes, or experimental changes, teams can maintain code separation and streamline integration processes. Effective branch management minimizes conflicts, simplifies code synchronization, and promotes a more agile development environment.
Code Review Processes
Code review processes are integral to maintaining code quality, identifying potential issues, and enhancing team collaboration. By implementing systematic code reviews, developers can receive feedback, detect bugs, and improve coding standards adherence. Effective code review processes promote knowledge sharing, code consistency, and continuous improvement within the development team, ultimately leading to higher code quality and reduced technical debt.
Continuous Integration and Deployment
Automated Build Pipelines
Automated build pipelines optimize the software development lifecycle by automating code compilation, testing, and deployment processes. By implementing automated build pipelines, developers can accelerate release cycles, improve code quality, and enhance development efficiency. Continuous integration streamlines code integration, ensures build consistency, and facilitates early issue detection, enabling rapid iterations and agile development practices.
Testing Automation
Testing automation is essential for validating code functionality, ensuring application reliability, and detecting defects early in the development process. By automating test cases, developers can execute comprehensive tests efficiently, identify regressions, and maintain code integrity. Testing automation enhances code quality, streamlines testing processes, and facilitates continuous integration practices, improving software reliability and overall user satisfaction.
Deployment Strategies
Effective deployment strategies are critical for delivering software changes to production environments reliably and efficiently. By implementing optimized deployment processes, developers can minimize downtime, mitigate risks, and ensure seamless application updates. Deployment strategies such as blue-green deployments, canary releases, or rolling updates enable developers to deploy changes safely, reduce deployment failures, and enhance overall application performance and availability.