Exploring Java Frameworks: Architecture and Applications
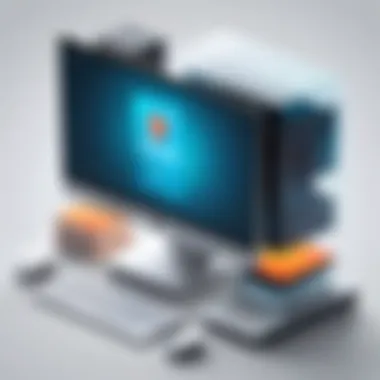
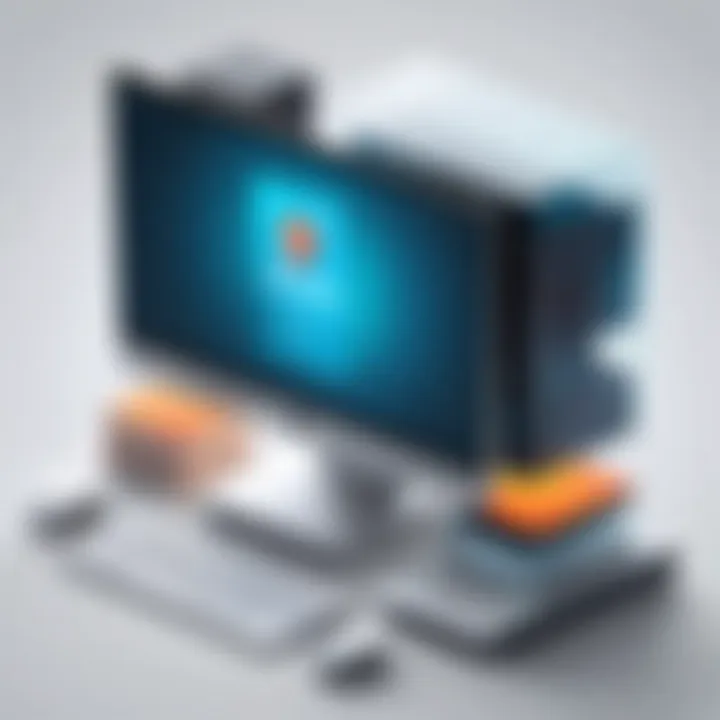
Overview of Topic
Preface to the Main Concept Covered
Java frameworks play a critical role in modern software development. They provide a structured environment that simplifies the development process, especially when working with large and complex applications. This article aims to dissect these frameworks in detail, exploring their architecture, core components, and functionalities. By delving into various Java frameworks, we will discover their applications and integration methods.
Scope and Significance in the Tech Industry
In recent years, the demand for Java has grown due to its versatility and robustness. Java frameworks are essential for developers, as they enhance productivity and ensure that applications are scalable and maintainable. Understanding these frameworks is significant for both students and seasoned IT professionals, as it directly impacts the efficiency of software creation.
Brief History and Evolution
Java was introduced in the mid-1990s, primarily designed to be a platform-independent language. Over the years, several frameworks have emerged, each evolving to meet the changing needs of developers. Frameworks such as Spring, Hibernate, and JavaServer Faces (JSF) have become integral tools in a developer’s arsenal, enabling rapid development and better resource management.
Fundamentals Explained
Core Principles and Theories Related to the Topic
Java frameworks center around several core principles. These include:
- Modularity: Structuring code into logical segments.
- Reusability: Encouraging developers to reuse existing code to save time.
- Configuration Management: Simplifying the setup and maintenance of complex systems.
Key Terminology and Definitions
Understanding the terminology is fundamental when delving into Java frameworks. Here are some key terms:
- Framework: A platform that provides a structure for building applications.
- Dependency Injection: A technique for achieving Inversion of Control between classes and their dependencies.
- Aspect-Oriented Programming: A programming paradigm that aims to increase modularity by allowing the separation of cross-cutting concerns.
Basic Concepts and Foundational Knowledge
At its core, a Java framework acts as a foundation upon which applications can be built. It provides built-in functions and libraries that streamline coding and allow developers to focus on business logic instead of repetitive tasks. For instance, in Spring framework, Dependency Injection allows more flexibility in managing component lifecycle.
Practical Applications and Examples
Real-world Case Studies and Applications
Java frameworks find their applications in various domains, from web applications to mobile computing. For example, a popular e-commerce site might utilize Spring for its back-end services, while using Hibernate for database interactions.
Demonstrations and Hands-on Projects
One effective way to grasp Java frameworks is through hands-on projects. Developers can create simple applications. For instance, creating a RESTful service using Spring Boot can provide intuitive knowledge of how web services operate.
Code Snippets and Implementation Guidelines
This snippet shows how a REST controller can be set up in Spring to handle HTTP requests.
Advanced Topics and Latest Trends
Cutting-edge Developments in the Field
As technology advances, so do Java frameworks. Recent innovations focus on microservices architectures, where frameworks like Quarkus and Micronaut have emerged. They provide features suited for cloud-native development, improving scalability.
Advanced Techniques and Methodologies
Usage of advanced features such as Spring WebFlux for reactive programming is crucial. This can lead to better resource utilization and responsiveness in applications, particularly where real-time data handling is important.
Future Prospects and Upcoming Trends
Looking ahead, the integration of AI and machine learning with Java frameworks is becoming a trend. Many organizations are exploring ways to leverage data analytics seamlessly within Java environments.
Tips and Resources for Further Learning
Recommended Books, Courses, and Online Resources
To deepen your knowledge, consider the following resources:
- "Spring in Action" by Craig Walls
- Online courses on platforms like Udemy or Coursera specifically covering Java frameworks.
Tools and Software for Practical Usage
Integration tools such as Apache Maven for dependency management can greatly enhance your experience with Java frameworks. More importantly, developers should keep tools like IntelliJ IDEA or Eclipse at their disposal for coding and debugging.
Learning about Java frameworks is not merely academic; these skills have practical implications in real-world scenarios, enhancing your capabilities and career prospects in the tech industry.
Preface to Java Frameworks
Understanding Java frameworks is crucial for anyone involved in software development using the Java programming language. Java frameworks provide developers with a structured environment to build applications efficiently while enhancing productivity. This section emphasizes various aspects and considerations that underline the importance of Java frameworks in modern development.
Definition and Importance
A Java framework is a set of tools, libraries, and conventions designed to simplify the development process. It provides a predefined structure and a layer of abstraction over the core features of Java. This allows developers to focus on the specific business logic of their applications rather than dealing with the minutiae of underlying code.
The importance of Java frameworks can be summarized as follows:
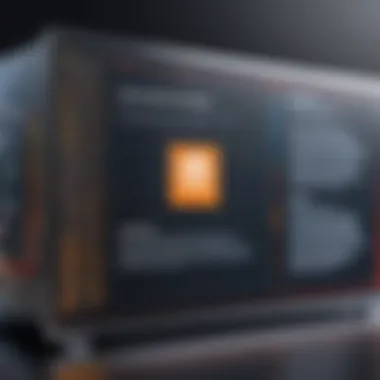
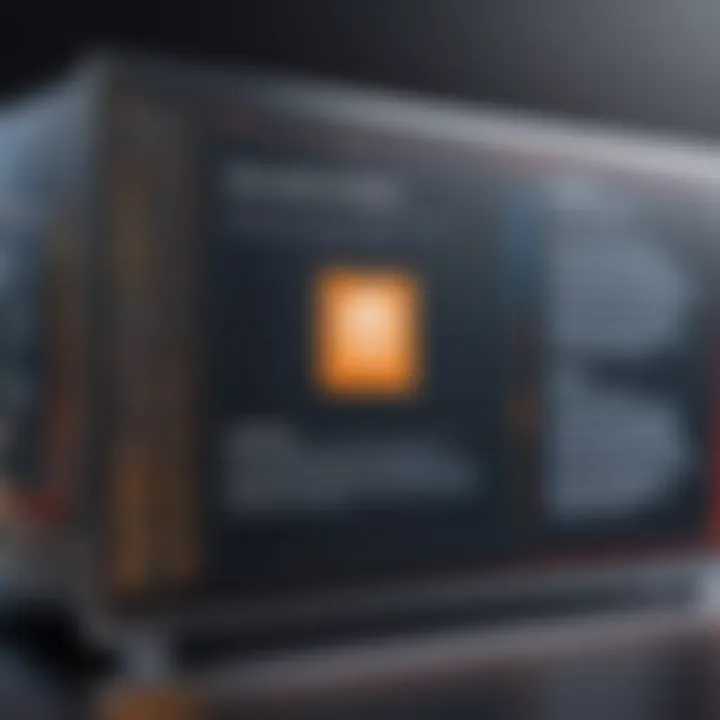
- Increased productivity: Frameworks provide reusable code, thus avoiding redundancy. Developers can utilize existing libraries and components to speed up their progress.
- Enhanced collaboration: By standardizing how code is organized, teams can more easily work together. This leads to better communication and fewer misunderstandings.
- Simplified maintenance: Well-structured code is easier to manage. Frameworks often come with guidelines that promote clean coding practices, making future updates and debugging more straightforward.
In essence, Java frameworks not only improve existing processes but also enable faster and more effective application development.
History of Java Frameworks
The evolution of Java frameworks reflects the growth of the Java ecosystem itself. Starting from the late 1990s, Java gained popularity for its portability and robust design. However, as applications became more complex, the need for frameworks emerged.
One of the first significant frameworks was Struts, introduced in 2000. It utilized the Model-View-Controller (MVC) architecture, which became a standard design for building web applications. Following Struts, other frameworks like Spring, released in 2003, emerged, offering a more comprehensive solution for enterprise application development.
With the rise of web applications and the need for easier integration with databases, frameworks such as Hibernate gained traction. Hibernate simplified the data management process, providing developers with powerful Object-Relational Mapping capabilities.
Today, a variety of frameworks cater to different needs and preferences, offering various tools for web, mobile, and enterprise applications. By understanding the history of Java frameworks, developers can appreciate the iterative nature of this technology sector and its impact on modern programming practices.
Core Components of Java Frameworks
Understanding the core components of Java frameworks is crucial for anyone engaging with Java technology. These components form the foundation that enables developers to create applications effectively. They provide a structured environment that increases efficiency and reduces complexity. Focusing on these elements can lead to better project outcomes and a deeper comprehension of how applications operate under the surface.
Java Virtual Machine (JVM)
The Java Virtual Machine (JVM) is a key player in the Java ecosystem. It acts as an abstraction layer, allowing Java programs to run on any device or operating system that supports the JVM. This characteristic forms the basis of Java's “write once, run anywhere” philosophy.
The JVM is responsible for converting Java bytecode, which is generated by the Java compiler, into machine code that the host system can execute. This translation process includes several essential aspects, such as garbage collection and memory management, ensuring that resources are used efficiently.
Key points about JVM include:
- Platform Independence: Programs can run on multiple operating systems without modification.
- Performance Optimization: JVM includes Just-In-Time (JIT) compiler, which speeds up execution by compiling bytecode to native code during runtime.
"The JVM enables the portability of Java applications across diverse computing environments, which is a significant advantage in today’s multi-platform world."
Java Runtime Environment (JRE)
Next is the Java Runtime Environment (JRE), which provides the necessary libraries and components to run Java applications. JRE includes the JVM along with a set of core libraries that support the language's functionality. Without the JRE, Java applications simply cannot function.
For developers, understanding JRE is important because:
- Execution Dependency: JRE must be installed on any machine running a Java application.
- Library Availability: It provides essential libraries related to networking, data structures, and other core functions, greatly simplifying application development.
It is worth noting that JRE is mainly intended for users who need to run Java applications but do not need the tools for developing them. Therefore, it is essential to install the appropriate version of JRE compatible with the Java software version being used.
Java Development Kit (JDK)
Finally, we come to the Java Development Kit (JDK). The JDK is a comprehensive package that facilitates Java development, including the JRE. It encompasses all the tools required to create, compile, and debug Java applications. This includes development tools like Java compiler (javac) and other utilities that streamline the development process.
The importance of the JDK can be highlighted through:
- Tool Availability: It offers developers a complete set of tools for building applications in Java, fostering an efficient workflow.
- Documentation and Resources: JDK includes extensive documentation and example code, which are invaluable for both new learners and seasoned developers.
In summary, the core components of Java frameworks—JVM, JRE, and JDK—play pivotal roles in the Java software development lifecycle. Each contributes significantly to how applications are built, deployed, and executed, making them essential knowledge areas for any programmer aiming to work with Java.
Popular Java Frameworks
Java frameworks play a pivotal role in simplifying the development process and enhancing productivity. These frameworks provide a foundation for building applications by offering pre-written code, libraries, and tools that reduce the need for repetitive tasks. This section focuses on some of the most popular Java frameworks that are widely used in the industry today. Understanding these frameworks is crucial for developers aiming to leverage their functionalities for efficient application design and implementation.
Spring Framework
The Spring Framework has gained substantial popularity due to its comprehensive programming and configuration model. It is particularly known for its ability to simplify the complexities often associated with enterprise-level applications. The core principle of Spring is the Inversion of Control (IoC), which facilitates easier management of various application components.
Key features of Spring include:
- Dependency Injection: This aspect allows developers to decouple various components in their applications, promoting better organization and maintainability of the code.
- Aspect-Oriented Programming (AOP): This helps in separating cross-cutting concerns such as logging and security, ensuring that the business logic remains clean and focused.
- Integration Support: Spring integrates seamlessly with various other technologies and frameworks, making it a versatile choice for developers.
JavaServer Faces (JSF)
JavaServer Faces (JSF) is another prominent framework designed for building user interfaces in Java-based applications. It is especially beneficial for web applications that require rich user interactions. JSF employs a component-based approach, allowing developers to create reusable UI components and manage their state.
The significant benefits include:
- Built-In Components: JSF comes with a wide array of pre-defined components which speeds up development and ensures consistency across the application.
- MVC Model: This framework follows the Model-View-Controller pattern, which helps maintain a clear separation of concerns, making the application easier to manage.
- Event-Driven Programming: JSF supports event-driven programming, allowing for responsive and interactive user experiences.
Hibernate Framework
Hibernate is an object-relational mapping (ORM) framework that simplifies database interactions in Java applications. It provides an abstraction layer over SQL and allows developers to work with data in terms of domain-specific objects.
The main advantages of Hibernate include:
- Data Manipulation: Hibernate offers a powerful query language (HQL) which makes it easier to manipulate persistent data using object-oriented constructs.
- Caching: Hibernate features various caching mechanisms that enhance performance by reducing the number of database calls.
- Database Independence: It allows for easy switching between different databases without major code changes.
Grails Framework
Grails is an innovative web application framework that leverages the Groovy programming language, combining the robust capabilities of Java with the brevity of Groovy. It emphasizes convention over configuration, providing an environment to build powerful web applications rapidly.
Noteworthy aspects of Grails include:
- Rapid Development: Grails promotes developer speed, allowing for quick prototyping and development cycles.
- Built on Spring: Being built atop the Spring Framework, it retains many benefits of Spring while enhancing development efficiency.
- Integrated Tools: Grails comes with various development and testing tools, reducing complexity during the application lifecycle.
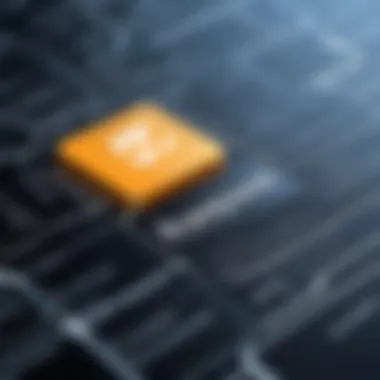
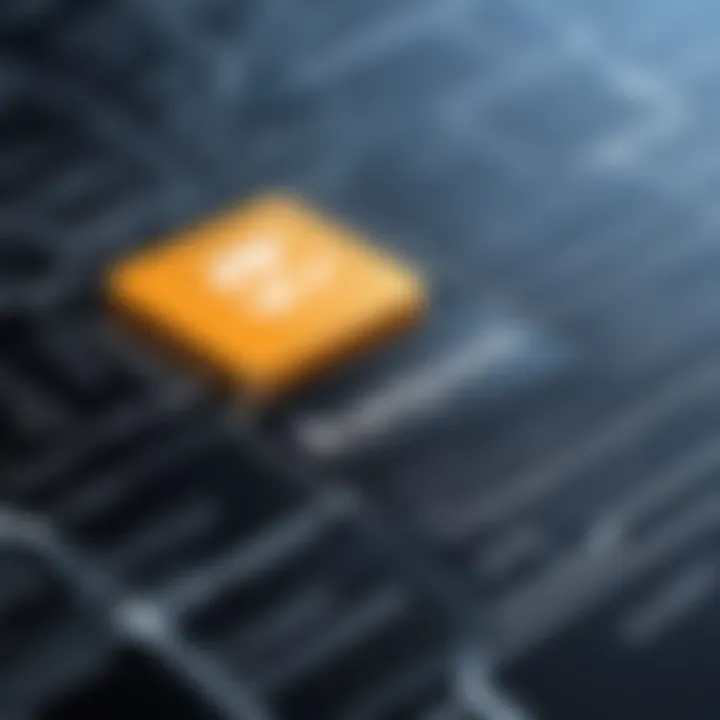
Understanding these frameworks equips developers with the knowledge to choose the right tool for their projects, ensuring that they can effectively leverage Java's capabilities.
Framework Architecture
Framework architecture is a foundational aspect of software development that defines how different components interact with each other. Understanding its significance helps in grasping the broader built of Java frameworks and their role in building scalable and maintainable applications. It encompasses design patterns, structural elements, and integration methods that streamline the development process.
The architecture of a framework serves several functions. It provides standards and guidelines for coding practices, ensures that software is modular, and allows developers to focus on business logic rather than low-level details. By establishing well-defined layers, frameworks promote separation of concerns, making code more maintainable and easier to test.
Layered Architecture Model
The layered architecture model is one of the most prevalent architectural patterns used in java frameworks. It typically comprises several horizontal layers, each responsible for specific functions. These layers often include presentation, business logic, data access, and sometimes a service layer.
- Presentation Layer: This is where user interactions occur. It collects input and displays output, usually in the form of user interfaces.
- Business Logic Layer: This layer processes the data and implements the core rules of the application. Here, business requirements are translated into executable code.
- Data Access Layer: Responsible for interacting with databases or external systems, this layer manages data transactions and retrieves data as needed.
The aim of this architecture is to achieve high cohesion within layers and low coupling between layers. This leads to better organization of code and easier adaptability over time. When changes are needed in one layer, they can usually be made with minimal impact on others.
Microservices and Java Frameworks
Microservices architecture represents a shift in how applications are constructed, favoring smaller, independently deployable components over monolithic systems. This approach aligns well with Java frameworks like Spring Boot, which facilitate the development of microservices with ease.
In a microservices environment, each service is built around a specific business goal and can be scaled independently, which offers several advantages:
- Flexibility in Technology Choices: Different microservices can use various technologies, allowing teams to pick the best tools for their specific needs.
- Improved Scalability: Services can be scaled separately based on the demand, optimizing resources effectively.
- Resilience and Fault Isolation: If one service fails, it does not affect the others, leading to enhanced reliability of the overall application.
To integrate microservices with Java frameworks, developers need a solid understanding of RESTful APIs, containerization with Docker, and orchestration tools like Kubernetes. The choice of a suitable framework can greatly ease the complexities involved in microservices development, leading to smoother workflows and faster delivery cycles.
In summary, understanding framework architecture is crucial for efficient software developent. Knowing its layers and embracing microservices can vastly improve system performance and responsiveness.
Choosing the Right Java Framework
Choosing the right Java framework is crucial for the success of any software project. The myriad of available frameworks can lead to confusion. Each framework offers unique features and capabilities that cater to different project needs. A well-chosen framework can streamline development processes, enhance performance, and reduce time to market. On the other hand, selecting an inappropriate framework can result in project delays and increased costs.
Project Requirements Analysis
Before diving into the selection of a Java framework, conducting a comprehensive project requirements analysis is essential. This involves understanding the specific needs of the project. Key factors to consider include:
- Project Scale: Large-scale projects may require frameworks that support complex architectures like microservices, whereas smaller applications might benefit from simpler, lightweight frameworks.
- Team Expertise: The technical proficiency of the development team plays a significant role. A framework that aligns with the team's strengths will lead to greater efficiency and productivity.
- Functionality Requirements: Evaluate the core functionality needed for the project. Determine whether the framework can support required features, such as RESTful APIs, data persistence, or graphical user interfaces.
- Integration Capabilities: Consider how well the framework can integrate with other tools and systems. This includes databases, cloud services, and third-party libraries. Robust integration options can considerably enhance a project's value.
An in-depth analysis helps eliminate frameworks that do not align with project goals. This process can save significant time and effort down the line.
Evaluating Framework Features
Once project requirements are clearly defined, it becomes important to evaluate the features of potential frameworks. Several critical elements should be assessed:
- Performance: Look for frameworks that offer high performance and efficiency. Benchmarking them under expected loads can provide insights into how they will perform in production.
- Community Support: A vibrant community often indicates good support and resources, such as documentation, forums, and plugins. A robust community can help address challenges that may arise during development.
- Learning Curve: Consider how intuitive the framework is. A steep learning curve can slow down development significantly. Frameworks with a shallow learning curve allow faster onboarding of new team members.
- Scalability: Analyze how well the framework can accommodate future growth. Scalability is essential for adapting to increasing user demands and evolving project requirements.
- Security Features: Security is paramount in application development. Evaluating built-in security features is essential to safeguard sensitive data and maintain user trust.
Ultimately, the careful examination of these aspects can inform an intelligent decision when selecting a Java framework.
Selecting an appropriate framework not only influences the current project but also affects future development and iteration.
Integrating Frameworks into Development
Integrating frameworks into development is crucial in modern software engineering. It allows developers to utilize the strengths and efficiencies of specific frameworks, thus accelerating the development process. Choosing the right framework enables teams to minimize repetitive tasks, leverage built-in functionalities, and enhance maintainability of code. However, the integration process requires careful planning to mitigate risks and ensure compatibility with the existing development environment.
When integrating frameworks, developers can consider the various elements that significantly influence development outcomes. These include dependency management and configuration management, which are two fundamental aspects of the integration process.
Dependency Management
Dependency management refers to how projects manage external libraries or components that the application relies on to function. In Java development, frameworks such as Maven and Gradle provide robust solutions for handling dependencies efficiently.
Benefits of proper dependency management:
- Simplified Version Control: Teams can effortlessly manage updates and changes to libraries.
- Conflict Resolution: Tools prevent issues arising from conflicting versions of libraries, leading to more stable applications.
- Enhanced Collaboration: Sharing project setups becomes straightforward, allowing different team members to work without version inconsistencies.
Managing dependencies well is vital for ensuring that the framework integrates seamlessly into existing projects. Developers must regularly review dependencies and assess their impact on application performance.
Configuration Management
Configuration management deals with the settings and preferences that govern the behavior of applications. This involves defining how frameworks interact with the surrounding environment, including databases, servers, and external services.
Key elements of configuration management include:
- Environment Separation: Managing configurations for different environments (development, testing, production) helps prevent issues during deployment.
- Centralized Configuration: Using a centralized repository for configuration files can improve maintainability and reduce errors.
- Automated Changes: Automation tools can apply configuration changes without manual intervention, minimizing downtime and human error.
Implementing effective configuration management is essential for ensuring that the integrated framework functions as intended within the software environment.
Proper integration of frameworks not only enhances developer productivity but also instills a sense of reliability and efficiency within the software development lifecycle.
By focusing on both dependency and configuration management, developers can facilitate smoother framework integration processes, thus leading to better resource management and streamlined workflows.
Performance Considerations
Understanding performance considerations is critical when working with Java frameworks. Performance can significantly influence the efficiency, maintainability, and scalability of applications built using these frameworks. As organizations increasingly prioritize speed and responsiveness in software development, optimizing performance becomes an indispensable focus for developers.
In this section, we will explore two essential aspects of performance: benchmarking and scalability. Each of these areas plays a vital role in assessing and improving the performance of Java frameworks.
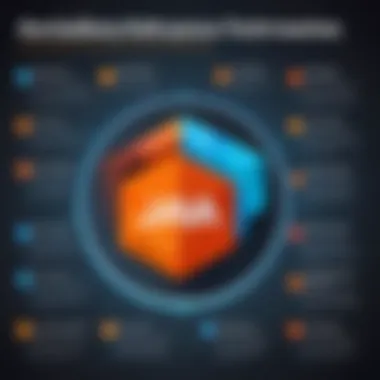
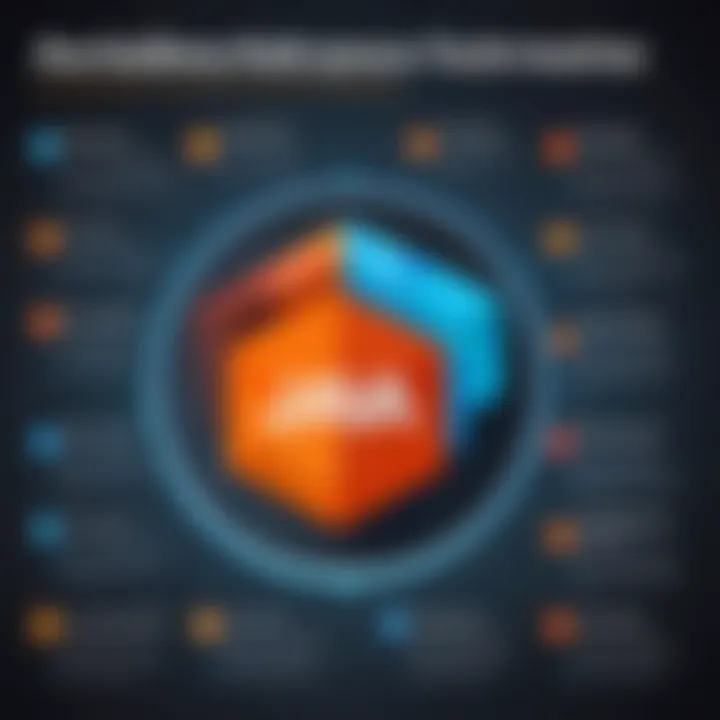
Benchmarking Java Frameworks
Benchmarking is a systematic approach to measuring the performance of Java frameworks. It involves running tests under specific conditions to assess various performance factors, such as response time, throughput, and resource utilization. Conducting benchmarks helps developers identify bottlenecks within the framework, thereby guiding optimization decisions.
Several tools are available for benchmarking Java applications. Some of the popular tools include:
- JMH (Java Microbenchmark Harness): Designed specifically for Java, JMH provides functionalities for writing, running, and analyzing benchmarks.
- Apache JMeter: This is useful for performance testing and load testing applications written in Java.
- Gatling: Often used for testing applications’ throughput and performance under various load conditions.
When benchmarking, developers need to consider certain factors that may impact test results:
- Environment Consistency: Ensure that test conditions remain consistent across different runs.
- Garbage Collection Effects: Take into account how garbage collection may affect performance during tests.
- Multiple Runs: Conduct multiple tests to average out the results and get a clear picture of the framework’s performance.
Benchmarking is not just about finding the best framework; it is also about understanding how different components interact within the framework to affect overall performance.
Scalability and Load Balancing
Scalability refers to the capability of a framework to handle increasing workloads without compromising performance. As applications grow, they often need to manage additional users, data, and requests. A well-designed Java framework should accommodate this growth seamlessly.
Load balancing is a process that involves distributing network or application traffic across multiple servers. This ensures no single server bears too much load that could lead to bottlenecks or failures. Load balancing contributes to scalability by enabling applications to grow horizontally, as more servers can be added to handle increased demand.
Key strategies in achieving effective scalability and load balancing include:
- Vertical Scaling: Enhancing resources (CPU, RAM) on existing servers. Simple but has limits.
- Horizontal Scaling: Adding more machines to spread the load. This is often more flexible and preferred in cloud environments.
- Using a Load Balancer: Implementing tools such as HAProxy or Nginx to intelligently distribute requests.
In summary, performance considerations are vital in ensuring that Java frameworks meet the demands of modern applications. Continuous evaluation through benchmarking and effective strategies for scalability and load balancing can enhance a framework's robustness and reliability.
Best Practices in Framework Usage
Using Java frameworks effectively requires understanding the best practices that can enhance code quality and project success. Adopting these practices not only improves code maintainability but also ensures the framework operates as intended across different scenarios.
Code Maintenance and Readability
Maintaining code quality is crucial in any software development environment, and Java frameworks are no exception. Code should be written with clarity in mind. Here are some guidelines to follow:
- Consistent Naming Conventions: Use meaningful and consistent naming conventions for classes, methods, and variables. This practice aids in understanding the purpose of the code at a glance.
- Modular Design: Break down the application into smaller modules or components. Each module should have a single responsibility, making it easier to test and maintain.
- Commenting and Documentation: Include comments and documentation as this enhances code readability. Descriptions help others (and future you) to grasp how and why certain decisions were made throughout the development process.
- Code Reviews: Implement regular code reviews. This practice allows team members to review each other’s work, fostering shared knowledge and identifying potential issues early.
By prioritizing maintainability and readability, developers can ensure that code bases are easier to modify and enhance over time, which reduces technical debt.
Security Practices
Security should be a fundamental consideration when developing applications with Java frameworks. Poorly secured applications can lead to data breaches and other security incidents. Here are key security practices to implement:
- Input Validation: Always validate and sanitize input data. This prevents common vulnerabilities such as SQL injection and cross-site scripting (XSS).
- Authentication and Authorization: Implement robust authentication mechanisms. Use frameworks like Spring Security to manage user authentication and permissions tightly.
- Secure Data Handling: Encrypt sensitive data both in transit and at rest. This protects data from unauthorized access even if it gets intercepted.
- Regular Security Audits: Conduct periodic security audits to identify vulnerabilities within your application. Keeping dependencies up-to-date is also crucial, as it protects against known vulnerabilities.
Emphasizing security is not just an endpoint; it should be integrated throughout the development lifecycle.
Future Trends in Java Framework Development
Future trends in Java framework development are crucial for keeping the language relevant and adapting to new technologies. As the technological landscape evolves, Java frameworks must also transform to meet modern software demands. This section explores two prominent trends that are reshaping the development environment: Artificial Intelligence and Machine Learning integration, and Cloud-Native solutions.
Artificial Intelligence and Machine Learning Integration
The integration of Artificial Intelligence (AI) and Machine Learning (ML) into Java frameworks is increasingly important. Developers are seeing a rise in the need for intelligent applications that can analyze data and learn from it. Java already has libraries like Deeplearning4j and Weka that support ML, but emerging frameworks aim for seamless incorporation of these functionalities directly into application design.
Benefits of this integration include:
- Enhanced User Experience: Applications can provide personalized experiences based on user behavior.
- Efficient Data Processing: Java frameworks can leverage AI to process large datasets quickly, improving performance.
- Automation: AI capabilities allow automation of repetitive tasks, thereby reducing human error and increasing efficiency.
The challenge lies in the complexity of algorithms and the need for robust data management. As developers gain more understanding and tools improve, we can expect a smoother integration process. This focus will ensure Java remains competitive in the fast-paced tech world.
Cloud-Native Solutions
Cloud-native solutions are another significant trend within Java framework development. Companies are increasingly moving toward cloud environments to enhance scalability and maintainability. Frameworks must adapt to provide robust support for cloud-native architecture.
Key advantages of cloud-native solutions include:
- Scalability: Applications can scale effortlessly depending on demand.
- Flexibility: Developers can quickly update and deploy new features without significant downtime.
- Cost Efficiency: By utilizing cloud resources, organizations can reduce operational costs.
Using frameworks like Spring Boot and Micronaut, developers can create cloud-ready applications that support microservices architecture. This lets teams build, deploy, and manage applications efficiently.
As organizations continue to embrace the cloud, Java frameworks must evolve to support this transition effectively. By focusing on these future trends, Java can maintain its relevance and support innovative applications in a changing digital environment.
"Embracing these trends allows developers to leverage the potential of Java frameworks, ensuring they remain leaders in software development."
For further reading on artificial intelligence and its applications in programming, you can visit Wikipedia. To dive into cloud computing concepts, check Britannica.
The End
The conclusion serves as a vital part of this article by synthesizing the insights gained throughout the discussion on Java frameworks. It emphasizes how these frameworks are not just tools, but integral parts of modern software development. By wrapping up the main points, it helps readers solidify their understanding of what they have learned.
Summary of Key Points
- Understanding Java Frameworks: They streamline development processes by providing reusable components and a solid architecture. This saves time and minimizes errors, as developers can rely on tested and proven frameworks.
- Core Components: The Java Virtual Machine (JVM), Java Runtime Environment (JRE), and Java Development Kit (JDK) create the backbone upon which frameworks operate, emphasizing their importance in any Java-based application.
- Variety of Frameworks: There are several frameworks like Spring, Hibernate, and JSF, each serving unique purposes and offering distinct functionalities. Knowing when and how to use each framework is crucial for optimization.
- Integration and Best Practices: Good practices in integration such as maintaining code readability and effective dependency management are essential for long-term project success.
- Future Trends: Understanding shifts toward cloud-native architectures and AI integration can better prepare developers for forthcoming changes in the technology landscape.
This summary distills the essence of the entire guide, highlighting not only the relevance of Java frameworks in software development but also the competencies necessary for working effectively with them.
Final Thoughts on Java Frameworks
Java frameworks have fundamentally reshaped how applications are built and maintained. They allow programmers to focus on solving business problems rather than getting bogged down by repetitive tasks. The frameworks covered in this article demonstrate versatility and power, making Java a continuous contender in the field of programming.
As technologies evolve, so do the frameworks. It is essential for students, aspiring programmers, and IT professionals to stay abreast of developments in this area. Understanding how to leverage these tools in an efficient way is key to achieving professional growth.
In summary, embracing Java frameworks is not just beneficial—it is essential for anyone looking to excel in software development. With an informed approach, developers can harness the full potential of these frameworks, leading to innovative and effective software solutions.