Mastering MapReduce with Python: A Comprehensive Guide
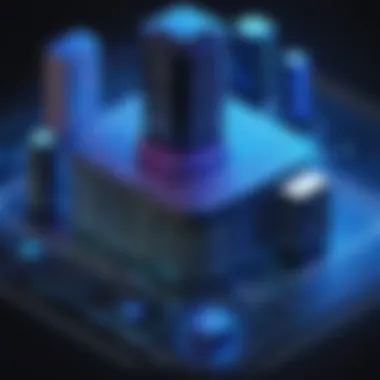
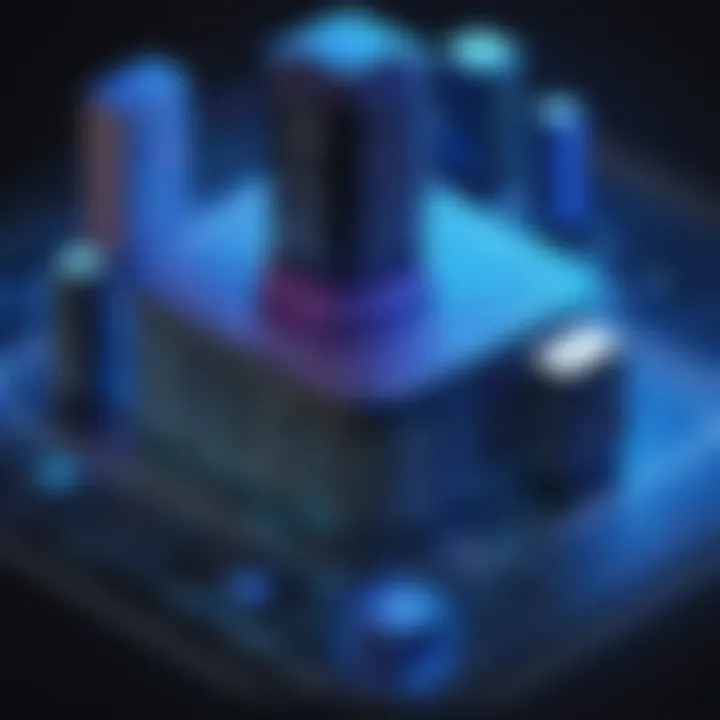
Overview of Topic
Prologue to the main concept covered
MapReduce is a programming model designed to process large data sets in a distributed computing environment. This paradigm simplifies the handling of vast amounts of data through parallel processing. It allows developers to break out complex computations into smaller, manageable tasks. The integration of Python with MapReduce opens new possibilities in data analysis, benefiting both novice programmers and seasoned developers.
Scope and significance in the tech industry
Big data is a central theme in today's tech industry. Organizations need efficient tools to process data and derive insights quickly. MapReduce provides a robust framework that scales with data volume grown. Optimizing this process using Python, a language known for its simplicity and readability, is fundamental for various sectors such as finance, healthcare, and e-commerce.
Brief history and evolution
MapReduce was popularized by Google in the early 2000s as a means to process data on its large-scale infrastructure. Over the years, open-source frameworks like Hadoop made it accessible for a wider audience. Python has since emerged as a popular choice among developers for implementing MapReduce due to its vast ecosystem of libraries and user-friendly syntax.
Fundamentals Explained
Core principles and theories related to the topic
The key principles of the MapReduce model are straightforward: the Map function organizes the input, while the Reduce function collects and processes the output. Overall they translate distributed computing processes into easily parallelizable tasks. This method reduces overall runtime and improves efficiency.
Key terminology and definitions
- Mapping: It refers to the process of transforming the input data into pairs of key and value.
- Reducing: The combining and summarizing of the mapped data segment.
- Distributed Computing: A computation method that involves multiple computer systems coordinating to execute a task.
Basic concepts and foundational knowledge
To effectively use MapReduce with Python, familiarizing oneself with programming libraries like or is essential. These libraries provide a smooth integration of MapReduce operations with Python, making it easier to perform data analysis.
Practical Applications and Examples
Real-world case studies and applications
One prominent application of MapReduce is in log analysis for web servers. Companies can handle vast amounts of logs, extracting valuable insights to better understand user behavior. In academia, researchers utilize MapReduce to work with large databases, e.g., genomic data processing.
Demonstrations and hands-on projects
A practical guide on implementing MapReduce in Python could include creating a Word Count program:
This simple example illustrates how words in a text document can be counted efficiently using the framework.
Code snippets and implementation guidelines
Once you've set up the PySpark environment, running the above code requires initiating the job through the command line. Using schemas and configurations correctly enhances clarity and performance, leading to reduced overhead during data processing.
Advanced Topics and Latest Trends
Cutting-edge developments in the field
Machine learning integration with MapReduce allows for progressively advanced data manipulations, adopting a more analytical approach to data resource management. Various organizations are actively refining real-time data processing techniques.
Advanced techniques and methodologies
Utilizing dynamic partitioning and combining strategies within the Reduce phase enhances performance. Enhanced algorithms that aren't memory-intensive have started receiving attention due to emerging big data challenges.
Future prospects and upcoming trends
The landscape of data science continuously shifts, and understanding upcoming trends helps professionals stay ahead. AI-driven MapReduce applications will likely receive more updates, optimizing not only speed and efficiency but also accuracy in processing data.
Tips and Resources for Further Learning
Recommended books, courses, and online resources
Foreword to MapReduce
Understanding the MapReduce model is foundational for effective data processing in Python. This section sets the stage for exploring the intricate relationship between programming and distributive data computation. We will unfold the multidimensional impacts of introducing MapReduce concepts and their practical applications with Python.
Definition and Purpose
MapReduce is a programming paradigm that allows for processing and generating large data sets with a distributed algorithm on a cluster. The primary purpose of MapReduce is to manage large-scale data efficiently and flexibly. Adopting this model helps in distributing data processing tasks across multiple nodes, significantly enhancing performance. This decentralized approach enables developers to implement parallel computations seamlessly while abstracting complex management details associated with load balancing and fault tolerance.
Key benefits of using MapReduce include:
- Scalability: It can be applied on vast datasets without a drop in performance.
- Fault Tolerance: The model is designed to recover easily and ensure correctness.
- Simplicity: Developers can focus more on processing rather than infrastructure management.
Historical Context
The foundations of MapReduce can be traced back to Google, which introduced the concept to manage vast amounts of data across its infrastructure around 2004. Inspired by functional programming concepts, the team at Google aimed to simplify operations that involved sorting and filtering massive datasets generated by their search engines and additional services. This revolutionary method quickly gained traction and later inspired numerous frameworks, including Apache Hadoop.
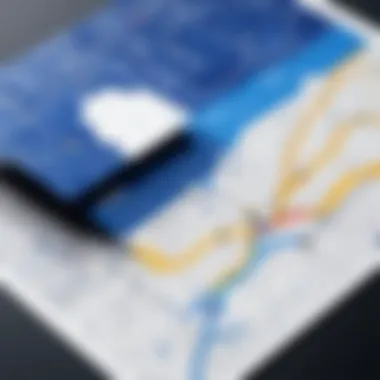
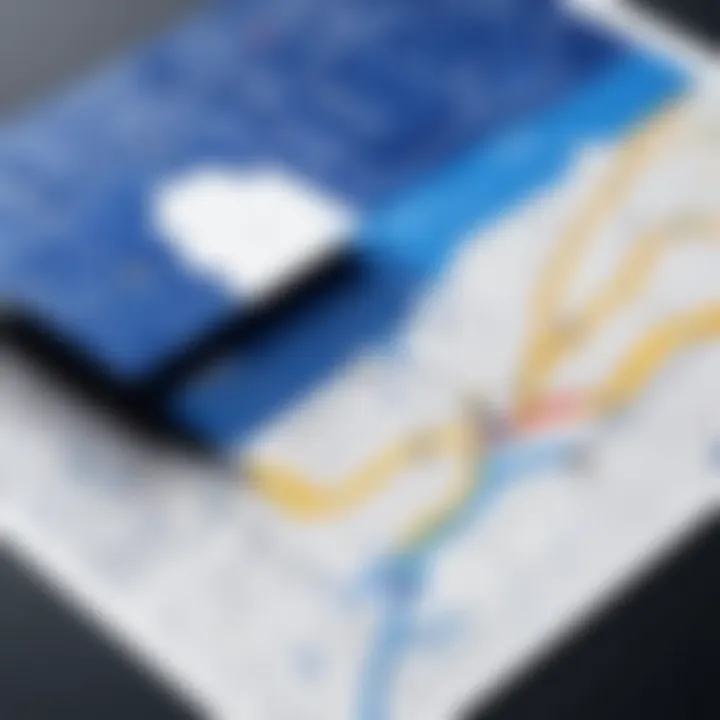
The acquisition of success with MapReduce led to its current status as a fundamental building block of big data processing today. Professionals in various fields aligned themselves with the essence of MapReduce to extract meaningful insights from the otherwise overwhelming data volumes that permeate modern applications.
Core Concepts
To grasp MapReduce thoroughly, one must appreciate its core principles that govern its operation. The process primarily consists of two interrelated functions:
- Map Function: This processes input data, transforming it into a set of intermediate key/value pairs. In this phase, data is split into manageable chunks that are then processed concurrently on different nodes.
- Reduce Function: The second phase takes the outputs of the map function and merges them into a result that can be returned. Furthermore, this step facilitates data consolidation to derive final results from scattered information.
The orchestration of these functions enables efficient data processing. Learning how these core concepts operate in conjunction will help maximize MapReduce's advantages when implemented in Python programming.
By familiarizing oneself with these principles, you can host many data-processing tasks more dynamically than traditional methods allow.
Understanding the MapReduce Programming Model
The MapReduce programming model is a pivotal concept in distributed computing, specifically designed to facilitate the processing of vast amounts of data. Understanding this model is crucial for developers and data scientists alike, as it provides a concise and effective approach to scalability and data processing. With the exponential growth of information, simply relying on traditional programming methods becomes impractical. Therefore, MapReduce emerged as a solution to perform distributed processing efficiently.
Map Phase
The map phase begins with the input data being divided into smaller segments. Each segment is processed independently, where a mapping function is applied. This function transforms input data into key-value pairs, which serves as the foundational basis for later operations.
Here’s what happens during the map phase:
- Data Partitioning: The larger dataset is broken down into manageable chunks, enabling simultaneous processing.
- Key-Value Transformation: The mapping function assigns unique keys to the values, which creates a structured output.
- Two Critical Elements: The process relies on the quality of the map function and how well it handles inputs. Any inefficiencies at this stage can hinder the overall operation.
Using Python, the implementation of this phase can look like this:
This simple example shows a word count operation where each word is a key, and the value is one, indicating its occurrence.
Reduce Phase
Once the map phase is completed, the reduce phase takes center stage. This phase is where the key-value pairs produced by the map function are aggregated to produce the final output. Here, the reducer takes all the values associated with unique keys and combines them in a meaningful way.
Key aspects of the reduce phase include:
- Aggregation: It consolidates the intermediate results from the map phase.
- Final Result Calculation: After combining the values, the reduction process emits the final key-value pairs.
- Efficiency and Optimization: Similar to the mapping function, the reduce function needs to be efficient. Poor performance here can lead to delays in data processing.
A Python implementation might resemble the following:
This example aggregates the word counts from the map phase, calculating the total occurrences of each unique word.
Data Flow in MapReduce
Understanding the data flow in a MapReduce model outlines intelligence on how input data is processed and transformed through various stages.
The general trajectory involves:
- Input Data Splitting: Data is distributed across different nodes, initializing the map tasks.
- Mapping: Each node processes its data chunk, producing intermediate key-value pairs.
- Shuffling and Sorting: Train occurs where all the data is sorted by key and redistributed based on the unique keys. This is essential for the reducer to perform adequately.
- Reducing: Lastly, the reduce phase compiles results, ensuring a comprehensive output based on aggregated values.
Proper understanding of this flow boosts the efficiency of data processing pipelines while minimizing potential bottlenecks.
Mapping out the stages this way creates clarity concerning each function’s role in the entire process, and designers can optimize both during coding and in troubleshooting.
Utilizing resources such as Wikipedia can provide even deeper insights into these subprocesses and help further comprehension of MapReduce's principles.
Prerequisites for Using MapReduce with Python
Understanding the fundamentals before diving into MapReduce with Python is essential. The environment and associated knowledge lay the groundwork for efficient programming and data manipulation. Each prerequisite provides critical benefits necessary for effectively implementing MapReduce, especially in frameworks that leverage large-scale data processing.
Basic Knowledge of Python
A solid grasp of Python is a significant asset when using MapReduce. The language's simplicity enables rapid growth in programming skills. People without prior programming experience might find Python more accessible due to its straightforward syntax and structure. Knowing how to write functions, handle loops, and manage files is essential. A developer must also be familiar with Python libraries that aid in data analysis, or data manipulation, such as NumPy and Pandas. These tools augment the power of MapReduce operations.
The importance of mastering basic Python cannot be overstated. The understanding built during this stage affects all future tasks involving MapReduce. Thus, aspiring contributors should spend time solidifying their coding skills to create sophisticated implementations as they progress.
Familiarity with Data Structures
Data structures are fundamental for managing and processing large datasets. At the heart of MapReduce are collections that allow for efficient data representation and manipulation. Different structures, such as lists, dictionaries, and sets, affect performance in RoadReduce frameworks. If a developer knows how to use these structures, better algorithms will emerge that maximize efficiency.
Good knowledge of data structures also translates well into an understanding of algorithms. This skill is vital when devising solutions to typical challenges encountered in data processing.
Key Considerations for Data Structures
- Understanding lists for ordered data.
- Utilizing dictionaries for fast data access.
- Considering sets for maintaining uniqueness.
To achieve a seamless transition into MapReduce, developers need to learn about the best structures suited for their specific use cases. This knowledge will drive better design in any future data processing strategies.
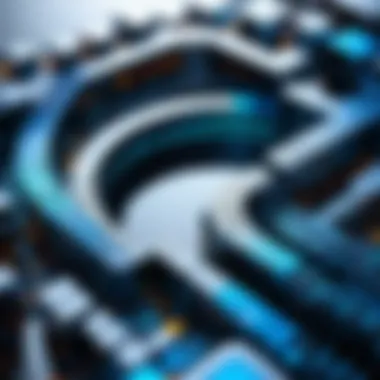
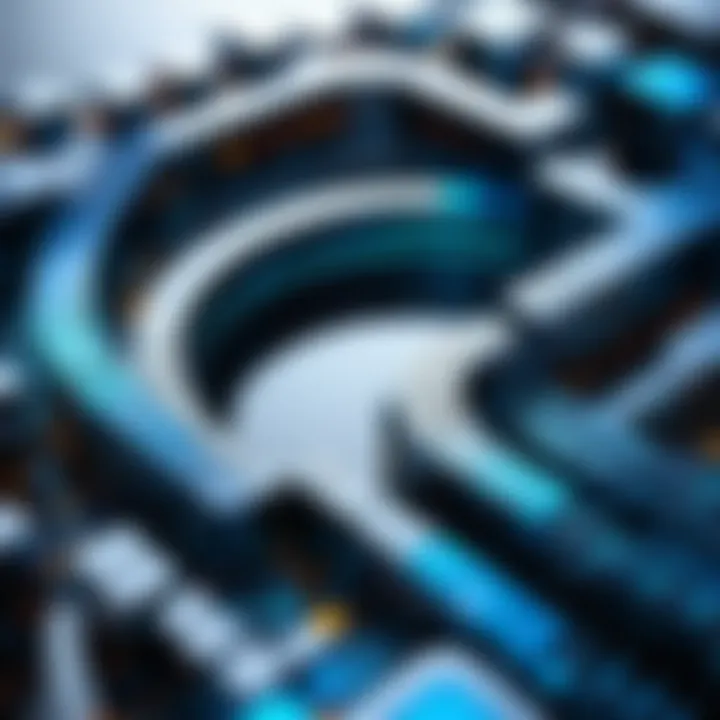
Understanding Distributed Computing Concepts
MapReduce operates in a distributed computing landscape, which comes with its complexities. Simply put, understanding distributed systems is non-negotiable when working with this model. This understanding allows developers to navigate challenges unique to these environments, such as data distribution and task concurrency.
Aspiring programmers should know concepts such as:
- Cluster Computing: Familiarity with running operations across multiple nodes.
- Parallel Processing: This denotes processing multiple tasks at the same time, which is advantageous when running MapReduce jobs.
- Fault Tolerance: Knowing how systems recover from failures is essential for reliability in operations.
Setting Up the Python Environment
Setting up the Python environment is a pivotal step toward using MapReduce effectively. Proper configuration can significantly enhance productivity and streamline the development process. This section will delve into installing necessary libraries, configuring the environment, and validating the setup. Doing so lays the groundwork for successful implementation of the MapReduce model in Python.
Installation of Required Libraries
Before diving into programming, you need the right tools. Python has several libraries that facilitate MapReduce programming. These tools are essential in handling data transformation and processing. Commonly used libraries include:
- Pandas for data manipulation and analysis.
- NumPy for numerical operations.
- PySpark for building distributed applications based on the Hadoop Model.
To install any of these libraries, you can use the following command in your terminal:
This command installs essential components needed to handle and run MapReduce tasks. Ensure you have a development environment set up, such as Anaconda or a virtual environment, to manage dependencies efficiently.
Configuring the Development Environment
Configuring your development environment requires careful planning. It affects productivity and may influence the success of your projects with MapReduce. You will want to ensure your Integrated Development Environment (IDE) is ready. Visual Studio Code, PyCharm, or even Jupyter Notebooks can be advantageous depending on your preferences.
Consider the following aspects while setting up your IDE:
- Environment Variables: Properly set PATH and other required variables.
- Version Control: Git can be invaluable for collaborative projects or keeping track of changes.
- Resources: Make sure you have sufficient RAM and a good processor for running heavy jobs.
For most libraries or frameworks you install, some configurations might be required—be mindful of compatibility issues between Python and libraries. This process ensures maximum efficiency and minimizes issues during development.
Testing the Installation
Before jumping into practical implementations, it is essential to test whether everything is functioning smoothly. Create a simple script that utilizes the installed libraries to run a basic MapReduce job. A
Implementing MapReduce in Python
Implementing MapReduce in Python is a crucial topic in this article as it bridges theory with practical application. The MapReduce framework handles large datasets efficiently, making it essential for both beginners and experienced programmers. By utilizing Python for these tasks, developers can harness a powerful and flexible language designed for rapid development. Python offers a simple syntax and extensive libraries, which makes it a favored choice for creating MapReduce jobs. These features allow users to write cleaner and more maintainable code.
The section outlines how to effectively implement and execute MapReduce logic, enhancing understanding of the process as a whole.
Example Use Case: Word Count
Script Overview
In our word count example, the script serves as a foundational illustration of how MapReduce works. The primary focus is on analyzing a set of text data to determine how many times each word appears. This specific example resonates because counting words is a common task in text analysis, and it showcases MapReduce's efficiency.
One key characteristic of this word count script is its simplicity and straightforward logic. Even those with basic programming skills can grasp the concept quickly. The word count problem allows for easy modifications, such as filtering special characters or analyzing input from different sources.
However, the limitation of using a word count example lies in its scope; for very large datasets, it may perform less efficiently without optimizations.
Map Function Implementation
The map function plays a pivotal role in this process, transforming input data into key-value pairs. In the case of word count, each word becomes a key, and the association of the word is the count of its occurrences.
The primary advantage of the map function is its parallel processing capability. Since many words are processed independently, speed optimization becomes possible when distributing tasks across multiple nodes.
On the other hand, its limitation is the static nature of input expectations. Errors can arise if the input data follows an unexpected structure.
Reduce Function Implementation
Once the map function produces intermediate results, the reduce function consolidates them. The reduce phase sums up the counts for each unique word generated during the map step. Thus, it centralizes the logic needed to derive a final count.
A major characteristic of reduce functions is their ability to form comprehensive results from intermediate challenges. Users can suitably compose more complex aggregations beyond sums, such as creating averages or filtering results based on specific criteria.
While advantageous, a potential hurdle is that potential bottlenecks may appear if there are too many unique keys. Thus, planning toolsets becomes essential when deploying the reduce phase.
Example Use Case: Log Analysis
Data Preparation
Data Preparation involves cleaning and structuring the log data before moving to the map phase. The log files often have various formats, which must be standardized. Clean and structured data leads to more reliable results.
This aspect is critical in increasing the reliability of subsequent processing in MapReduce. Users can benefit from thorough parsing, such as removing duplicates and handling exceptions effectively.
Despite the advantages, preparing data can require substantial effort when handling vast amounts of unrelated information.
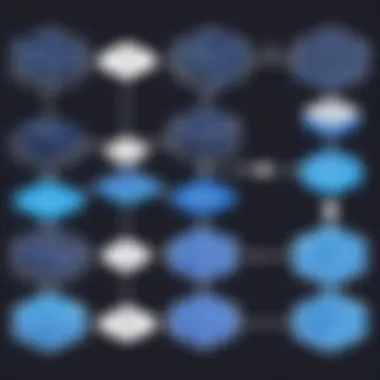
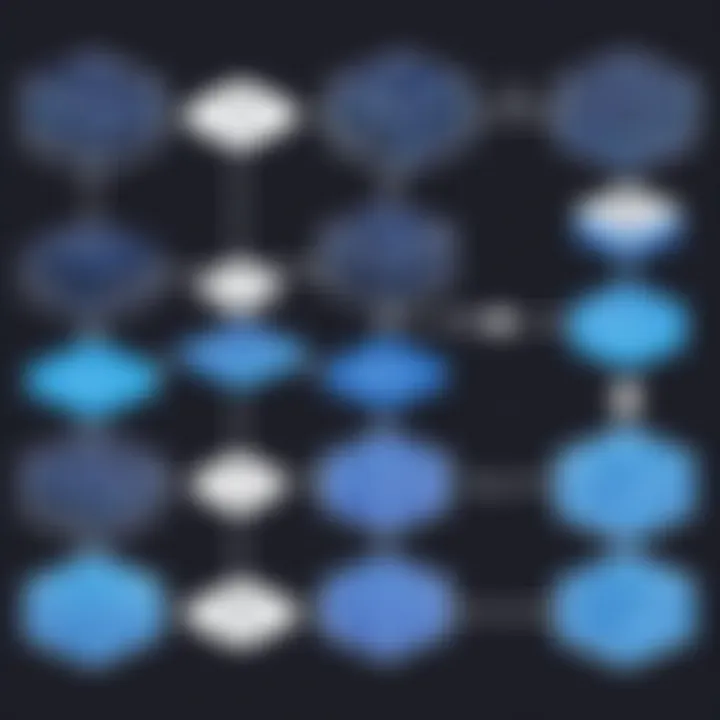
Map Function Details
In the log analysis example, the map function focuses on extracting meaningful pieces of information from the logs. It might parse timestamps or user identifiers depending on project needs. Besides standardizing unique index keys, the map function can enrich the logs' datasets.
The advantage of this method is applying the same mapping logic consistently across log formats and structures when parsing. This allows broader applications to emerge from similar datasets.
However, misconfigurations at this point can result in loss of vital information, underscoring the need for rigorous testing during development.
Reduce Function Details
As log data aggregates through the reduce function, metrics of interest become visible, such as the average number of daily site visits or error message frequencies. This operational focus underscores the reduce phase’s integral side in yielding business insights based on past operation data.
One key feature is customizing results types; the functionality can produce outputs based on specific filters, allowing extensions and deeper analysis. This helps in generating reports according to varying managerial needs.
Nonetheless, one disadvantage is dictating output formats ahead of time may restrict flexibility or encourage unify indices, which can skip significant breakdown options.
Ultimately, the successful application of MapReduce with meaningful Python implementations enables organizations to harness their data efficiently. Finding flaws during the implementation can promote a learning atmosphere for developers.
In delving into the implementation of MapReduce, we meet both versatile challenges and high reward potentials, pointing us toward optimized frameworks in data-centric approaches.
Optimizing MapReduce Jobs
Optimizing MapReduce jobs is crucial in ensuring that large data sets are processed efficiently and effectively. The ability to refine your MapReduce jobs can significantly reduce execution time, save resource consumption, and ultimately enhance performance. It ensures the effective use of distributed computing frameworks, improving the scalability of applications built using MapReduce.
Performance Considerations
When optimizing MapReduce jobs, several performance factors come into play. Firstly, the choice of data input formats greatly influences overall performance. Using formats like Avro or Parquet can enhance input and output efficiency. It's important to take advantage of compression techniques. Techniques such as Snappy, LZO, or Gzip help in reducing disk I/O and can result in quicker data processing.
Furthermore, the distribution of data across the cluster impacts performance. Understanding data locality—where computations are performed near the data rather than transferring data over the network—can reduce network traffic. Thus, optimizing how data is partitioned in HDFS is essential in achieving better performance;
- Assess job configuration parameters, such as and , to ensure that each task has enough resources without over-allocating, which could hinder other jobs.
- Monitor cluster resource utilization frequently to adjust resource allocation effectively.
Performance improvements often yield rapid payoffs when even small adjustments are made in job configurations and data management approaches.
Best Practices for Efficiency
To construct efficient MapReduce jobs, it is beneficial to follow best practices that allow you to achieve better performance consistently:
- Tune the Number of Mapper and Reducer Tasks: Adjusting the number of maps and reduces can have a major effect on overall efficiency. A higher number of tasks can lead to improved resource utilization, but too many tasks might trigger excessive overhead.
- Use Combiner Functions: When applicable, utilizing combiner functions can aggregate data at the mapper level, thus reducing data volume sent to reducers and speeding up the overall process.
- Select the Right Libraries and Frameworks: Using well-suited libraries and frameworks for your task can save time. For instance, using PySpark over standard Python files can leverage distributed processing by the Apache Spark framework.
- Testing and Benchmarking: Continual testing ensures job optimization. Through unit tests and benchmarking, you identify bottlenecks while making performance enhancements incremental and less risky.
بنوم خاص: Cold caching beneficial for recurrent reads that resist data loading every job, ultimately shortening job runtime.
By synthesizing performance considerations and adhering to best practices, developers can significantly enhance their MapReduce processes in Python. Patience and careful implementation are key to improving outcomes and was funding performances to discover hemenesses faults across data frameworks.
Common Pitfalls and Troubleshooting
The art of implementing MapReduce with Python is not without obstacles. Understanding common pitfalls and knowing how to troubleshoot issues is essential for both novice and advanced programmers. Without these skills, one may waste significant resources and time; this understanding ultimately leads to more efficient job executions. Addressing the issues proactively ensures successful data processing and fosters greater confidence in using MapReduce.
Debugging MapReduce Jobs
Debugging in MapReduce can become a daunting task due to the distributed nature of the framework. When a job fails, the reasons can be a range of complexities, from network issues to incorrect code logic. To tackle issues effectively, consider the following strategies:
- Error Logs: Always start with scrutinizing the output logs. They can often highlight where the errors originated. Familiarize yourself with the common types of logs generated by your MapReduce framework relative to your code.
- Local Mode Testing: Prior to deploying in a distributed environment, run jobs in local mode wherever possible. This allows for quicker iterations and isolates errors effectively without the overhead of deploying the entire distributed system.
- Unit Tests: Implement unit tests for your map and reduce functions. This serves as an early detection mechanism for logic errors, enabling simpler and more straightforward validation before moving to a clustered environment.
Utilizing these debugging strategies reduces confusion, saving time and focusing efforts in correcting problematic areas. Often, it is the small overlooked details that can lead to a employment breakdown.
Handling Data Skew
Data skew occurs when certain keys have significantly more data than others during the Map phase. This causes imbalances in resource utilization when it reaches the Reduce phase. Handling data skew is vital for achieving optimal performance in MapReduce jobs. Here are steps to address data skew:
- Identify Skewed Keys: Regular analysis of data distributions prior to processing helps to identify skewed keys. Tools are available to visualize data distributions and pinpoint which keys need more focus.
- Partitioning Techniques: Adjusting the partitioning strategy can balance the load across different reducers. Use custom partition functions to ensure more equity in data distribution. The goal is for all reducers to process relatively equal workloads.
- Additional Map Reduce Jobs: In certain situations, you might consider chaining additional MapReduce jobs that preprocess or redistribute skewed datasets. This ensures that large datasets do not overwhelm a single reducer, thus enhancing the efficiency.
In summary, navigating common pitfalls in MapReduce is critical to success. Whether it's through effective debugging techniques or managing data skew, these practices are essential in maintaining a smoothly running application. Continuous learning from past experiences enhances one's ability to tackle challenges in MapReduce.
End
The conclusion serves as a critical component of this article, summing up key elements surrounding the implementation of MapReduce in Python. The importance of effective summarization should not be underestimated; it reinforces learning by reminding the reader of essential concepts discussed earlier. It encapsulates the practical applications of MapReduce, highlighting its strength in efficiently processing extensive datasets. This ensures that the reader leaves with a compelling understanding of how to apply the principles to real-world problems.
A valid acknowledgment of several main benefits includes efficiency gains, ease of handling large volumes of data, and enhanced performance. Moreover, a well-crafted conclusion can also guide users toward extending their learning beyond the article. Readers who grasped the content may feel motivated to dive deeper into distributed computing, improving their understanding and enhancing their technical capabilities further.
Summary of Key Points
In reviewing MapReduce with Python, several key points emerge:
- Understanding MapReduce: It is crucial to grasp the fundamentals of the Map and Reduce phases. These steps involve data processing and reduction, forming a complete cycle.
- Programming Model: A solid understanding of the programming model assists in creating optimized solutions with Python.
- Environment Setup: Successful implementation hinges on a well-configured development environment and correctly installed libraries.
- Common Issues: Tradershoooting common pitfalls, such as data skew and debugging some jobs, is essential to a successful MapReduce application.
The points mentioned encapsulate the foundation on which further inquiries and projects may be built.
Future Directions in MapReduce with Python
As technology advances, the future of MapReduce with Python appears promising. Potential directions include:
- Integration with Advanced Tools: There is growing interest in integrating MapReduce frameworks with existing data platforms like Apache Hadoop and Apache Spark, utilized for processing large datasets efficiently.
- Machine Learning Applications: As machine learning becomes popular, there could be specialized use cases where MapReduce aids in data preparation and pre-processing steps for training models.
- Improvements in Tools: The continued development of tools that simplify the implementation of MapReduce with Python is inevitable. This would enhance accessibility for beginners and deepen functions for advanced practitioners.
- Research: Ongoing research may contribute to refining the MapReduce algorithms, improving bugs, and optimization to foster better performance.
Engaging with these future directions could lead practitioners and researchers alike into innovative applications within the vast field of data processing.