Exploring Python: A Comprehensive Guide to the Language
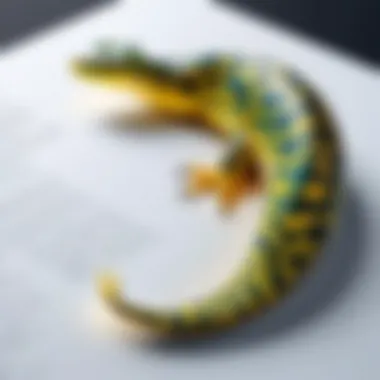
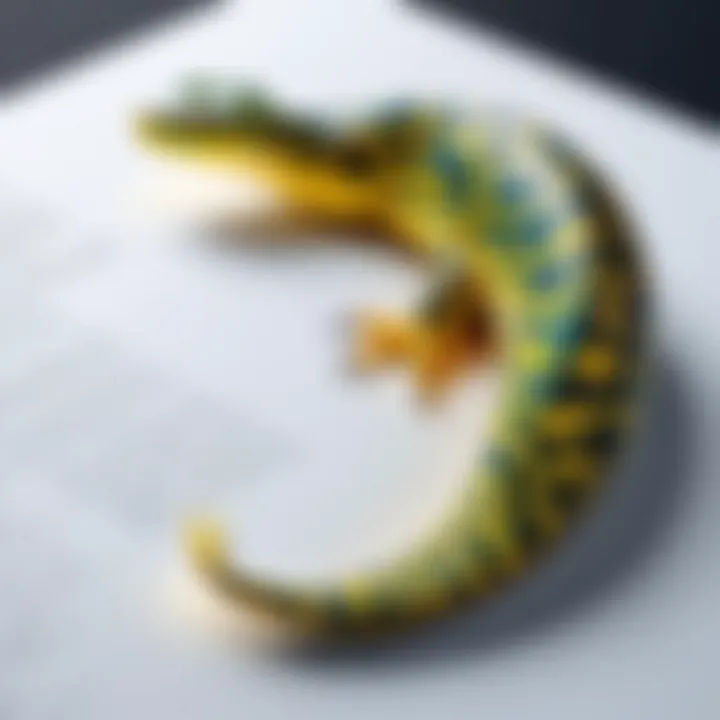
Overview of Topic
Preamble to the main concept covered
Python is a high-level, interpreted programming language. It is known for its simplicity and readability, making it a preferred choice for many developers. This guide aims to dissect Python by examining its core features and unique characteristics.
Scope and significance in the tech industry
In today's rapidly evolving technology landscape, Python holds a significant place. It is widely used in various domains like data science, artificial intelligence, and web development among others. Its versatility allows developers to create applications efficiently, which is essential in a world where time-to-market is critical.
Brief history and evolution
Python was created by Guido van Rossum and first released in 1991. It was designed to emphasize code readability. Over the years, Python has undergone several updates, resulting in improvements in performance and new features, making it a powerful tool in programming.
Fundamentals Explained
Core principles and theories related to the topic
Python's core principles can be summarized in several key areas:
- Code readability: The design philosophy emphasizes making code easy to read, which reduces errors and facilitates collaboration.
- Object-oriented design: Python supports multiple programming paradigms, including object-oriented programming, which helps manage larger projects effectively.
Key terminology and definitions
Understanding Python requires familiarity with certain terms:
- Interpreter: The program that reads and executes Python code.
- Library: A collection of pre-written code that developers can use to expedite their programming tasks.
- Syntax: The set of rules that defines how code is written in Python.
Basic concepts and foundational knowledge
Before diving into programming with Python, one should grasp fundamental concepts:
- Variables store data for use in programs.
- Control structures like loops and conditionals determine the flow of a program.
- Functions encapsulate reusable code, making programs modular.
Practical Applications and Examples
Real-world case studies and applications
Python's applications can be seen in various fields:
- Data Science: Libraries like Pandas and NumPy allow data manipulation and analysis.
- Web Development: Frameworks such as Django and Flask enable robust web application development.
Demonstrations and hands-on projects
For practical understanding, creating simple projects can be beneficial:
- A basic calculator can demonstrate functions and user input.
- A web scraper shows how to extract information from websites.
Code snippets and implementation guidelines
Here is a simple code snippet for a calculator:
Advanced Topics and Latest Trends
Cutting-edge developments in the field
Python continues to evolve. Recent developments include frameworks that enhance machine learning capabilities, like TensorFlow and PyTorch. The language is also making strides in automation and data visualization.
Advanced techniques and methodologies
Techniques such as asynchronous programming are gaining popularity, which allows for handling multiple operations concurrently, making applications more efficient.
Future prospects and upcoming trends
As technologies like artificial intelligence and machine learning grow, Python’s role is likely to expand. Its ability to adapt and integrate with other technologies is a promising aspect looking forward.
Tips and Resources for Further Learning
Recommended books, courses, and online resources
For those starting or advancing their Python skills, consider:
- "Automate the Boring Stuff with Python" by Al Sweigart.
- Codecademy Python Course for interactive learning.
Tools and software for practical usage
Key resources for Python development include:
- PyCharm or VSCode for integrated development environments.
- Jupyter Notebooks for interactive coding and data analysis.
Learn more about Python on Wikipedia).
Preface to Python Programming
Python programming has become a pivotal consideration in the realm of technology and software development. The importance of this topic cannot be overstated, given the language's broad adoption across various domains, such as web development, data science, artificial intelligence, and automation. This section serves as a foundation, preparing readers to understand what makes Python an ideal language for both novice programmers and seasoned professionals.
In this part, we will explore the history of Python and its significance in modern programming. Understanding these elements will provide valuable context for appreciating Python's capabilities and its evolution over time.
History of Python
Python was created by Guido van Rossum and was first released in 1991. The language was designed to be easy to read and simple to implement. Its origin can be traced to the late 1980s when van Rossum began developing Python as a successor to the ABC programming language. He aimed to resolve some of ABC's limitations, particularly in the area of extensibility. The decision to incorporate significant features from many programming languages, such as C and Modula-3, contributed to Python's flexible and versatile nature.
Python has undergone continuous improvement since its inception, with major releases enhancing its functionality. The transition from Python 2 to Python 3 marked a significant evolution, emphasizing cleaner syntax and a robust standard library. This change, however, was not without contention, as Python 2 continued to be widely used long after Python 3's introduction. Nonetheless, Python 3's advantages have led to its growing adoption in recent years, leading to the official end of support for Python 2 in January 2020. The collective advancements have positioned Python as a leading language in programming.
Significance in Modern Programming
Today, Python stands out due to its simplicity and versatility. It is widely regarded as a first programming language for students and beginners, thanks to its straightforward syntax. This accessibility encourages learning without overwhelming new programmers. Additionally, Python's large community of users and developers enhances the support ecosystem, making helpful resources readily available.
Importantly, Python's relevance in modern programming extends beyond education. Major tech companies and organizations, like Google, Facebook, and NASA, utilize Python for various applications. Its role in data science has become particularly vital, with powerful libraries like Pandas, NumPy, and Matplotlib offering extensive tools for data manipulation, analysis, and visualization. Likewise, Python's frameworks for web development, such as Django and Flask, provide robust solutions for building dynamic web applications.
Moreover, Python's capability to integrate with various platforms and languages makes it an attractive choice for developers seeking to build scalable and maintainable systems.
"Python is not just a programming language; it is a powerful tool that bridges gaps between different technologies and provides users with a means to translate complex ideas into simple implementations."
In summary, the Introduction to Python Programming establishes a crucial groundwork for readers. By recognizing Python's history and significance, they enhance their understanding of its current stature in technology. This foundation paves the way for exploring the core features, practical applications, and methodologies inherent in the Python landscape.
Core Features of Python
The core features of Python represent the essence of what makes this programming language both powerful and accessible. These attributes have played an integral role in its widespread adoption across different domains, such as web development, data science, and artificial intelligence. By understanding these features, learners can appreciate why Python consistently ranks among the top programming languages.
Simplicity and Readability
One of the primary strengths of Python is its simplicity and readability. The syntax is designed to be straightforward, allowing programmers to express concepts in fewer lines of code compared to other languages like C++ or Java. This is achieved through the use of clear conventions and an emphasis on whitespace, which enhances code legibility.
- Industries often favor Python for projects requiring rapid development and prototyping.
- A simpler syntax lowers the barrier for newcomers, making it an excellent choice for beginners.
Moreover, the clean and organized structure helps maintain code, reducing the difficulty during future revisions or collaborations. As a result, Python not only simplifies the learning curve for new programmers but also promotes best practices in software development.
Versatile Data Types
Python provides an extensive range of data types, which adds versatility to the language. It allows users to work effectively with integers, floats, strings, lists, tuples, and dictionaries, among others.
- The presence of dynamic typing means that you do not have to declare the data type explicitly, leading to quicker code development.
- Each data type has methods that simplify operations and manipulation. For example, lists allow dynamic resizing and easy insertions, which is beneficial in many applications.
This versatility enables Python to serve diverse applications, adapting to the needs of various fields such as web development, machine learning, and data analysis.
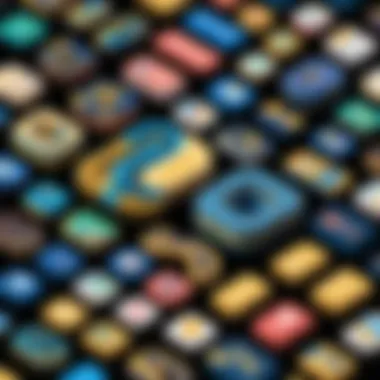
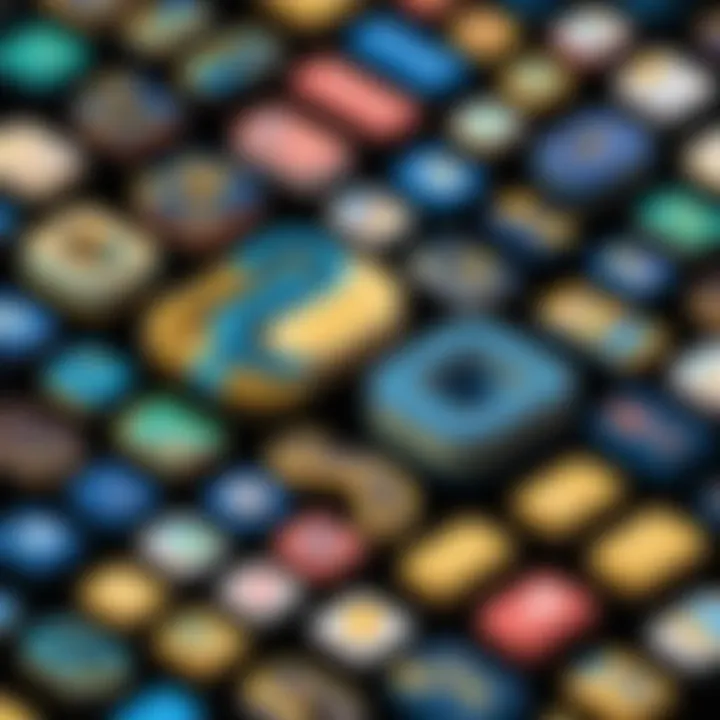
Extensive Standard Libraries
Another noteworthy feature is Python's extensive standard libraries. These libraries provide modules and packages to accomplish common programming tasks with ease, thus saving developers time and effort.
The libraries cover a broad range of functionalities, including:
- Mathematics and Statistics: Useful for calculations and data analysis.
- File Handling: Simplifies reading and writing files.
- Networking: Enables various network protocols for application development.
By leveraging these standard libraries such as , , and , programmers can quickly implement complex functionalities without reinventing the wheel. This efficiency supports Python's adoption in rapidly changing tech environments.
Interpreted Language
Python is an interpreted language, which means code is executed line by line during runtime. This offers several advantages:
- Immediate Feedback: Errors are reported as they occur, allowing for quick debugging. Learning why an error occurred becomes clearer.
- Portability: Python code can run on any platform with a Python interpreter, increasing its flexibility for deployment.
Nonetheless, it is worth noting that interpreted languages are generally slower than compiled languages. However, in many scenarios, the development speed and ease of use outweigh this downside, making Python a preferred choice for many developers.
"Python’s design principles emphasize code readability and a straightforward approach to programming, making it unique in a landscape of more complex languages."
Getting Started with Python
Getting started with Python is crucial for anyone looking to venture into programming. Python has earned a reputation as an accessible language, appealing to novices and veterans alike. This accessibility informs a significant component of its appeal, as it allows users to focus on learning programming principles without getting bogged down by syntactical complexities.
Besides its simplicity, Python supports various applications including web development, data analysis, and artificial intelligence. Consequently, understanding how to get started opens up a multitude of opportunities in various tech domains.
Installation of Python
The installation of Python is a fundamental first step. The official website of Python, python.org, hosts the most up-to-date versions of the language for different operating systems such as Windows, macOS, and Linux.
Once on the website, users should select the appropriate version for their operating system. After downloading, the installer offers options to customize settings. It is recommended to add Python to the system path, which simplifies running the language from the command line.
Indeed, ensuring a smooth installation process is vital, as issues can arise if configurations are altered incorrectly. Familiarizing oneself with installation may prevent future hurdles.
Setting Up an IDE
Following the installation, choosing an Integrated Development Environment (IDE) is critical. Popular choices include PyCharm, Visual Studio Code, and Jupyter Notebook. Each IDE comes with its unique features, aiding in writing, testing, and debugging code.
- PyCharm is favored for larger projects due to its powerful tools.
- Visual Studio Code is lightweight and very customizable, making it suitable for both beginners and experienced developers.
- Jupyter Notebook is excellent for data analysis and supports live code, allowing easy visualizations.
By setting up an IDE, users establish a conducive environment for coding, enhancing productivity and efficiency.
First Python Program
Finally, after installation and setup, writing the first Python program serves as a pivotal moment. A common beginner program is the "Hello, World!" script, which introduces the syntax of Python.
Here is how it looks:
This simple line of code demonstrates the use of the print function, a fundamental structure in programming. Executing this code confirms that the installation was successful and allows users to witness the immediate result of their efforts. Starting small encourages confidence and sets the stage for more complex programming tasks.
"Every programmer was once a beginner."
Taking these initial steps in Python helps build a solid groundwork for future learning and exploration.
Understanding Python Syntax
Understanding Python syntax is fundamental for learning to program effectively in this popular language. The syntax comprises the rules that define the combinations of symbols that are considered correctly structured programs in Python. A solid grasp of Python syntax helps beginners and experienced programmers communicate their logic clearly to the computer, ultimately leading to fewer errors and misunderstandings.
Basic Syntax and Structure
Python is designed to be straightforward. The language emphasizes simplicity and readability, which makes it accessible to new programmers. In Python, the syntax structure includes the use of indentation to define blocks of code, rather than using braces like many other programming languages. Each line of code is generally executed sequentially.
For instance, a basic print statement looks like this:
This simplicity allows the programmer to focus on the logic rather than the complex minutia of code formatting. Key elements of syntax include:
- Keywords: Reserved words like , , and that have special meanings.
- Identifiers: Names you give to variables, functions, and classes.
- Literals: Fixed values like strings and numbers.
Variables and Data Types
In Python, variables act as storage locations for information. Defining a variable is straightforward; it simply requires an assignment operation. For example, to create a variable that holds a number, you use:
Python supports a range of data types, including integers, floats, strings, lists, and dictionaries. This versatility allows programmers to choose the most appropriate type for their specific needs.
- Integers: Whole numbers, e.g., .
- Floats: Decimal numbers, e.g., .
- Strings: Text data enclosed in quotes, e.g., .
- Lists: Ordered collections, e.g., .
- Dictionaries: Key-value pairs, e.g., .
Control Flow Statements
Control flow statements allow the programmer to dictate the order and processing of code execution. They manage the decision-making aspects of a program. The most commonly used flow statements in Python include , , and . These statements provide conditional control over which code blocks are executed.
In addition, loops such as and can control how many times a block of code runs. Here is an example of a loop:
Functions and Modules
Functions encapsulate code into reusable blocks that can be executed when called. Their use fosters a modular programming approach, promoting code reuse. A simple function definition in Python appears as follows:
Modules are separate files that hold related functions and variables, offering an organization method for larger programs. The Python Standard Library comes packed with many built-in modules that aid developers in performing various tasks, such as math operations and file handling.
Important Note: Understanding these syntax elements forms the base for mastering Python effectively. Without a clear comprehension of syntax, it becomes challenging to build complex applications or troubleshoot existing code.
Mastering the Python syntax not only simplifies the learning process for beginners but also enhances productivity for seasoned developers by streamlining their coding practices.
Python Libraries and Frameworks
Python’s extensive ecosystem of libraries and frameworks significantly enhances its utility across various fields, particularly in data science, web development, and machine learning. Libraries and frameworks serve as essential tools that provide pre-written code, which simplifies and accelerates the development process. Leveraging these resources is not only a practice of efficiency but also a key factor in maintaining code quality and consistency.
Data Analysis with Pandas
Pandas is one of the most popular libraries for data analysis in Python. It provides high-performance data structures and data analysis tools. With Pandas, users can effortlessly manipulate structured data. It offers functionalities such as data cleaning, manipulation, and analysis through DataFrames and Series, making it intuitive for beginners. Some key benefits of using Pandas include:
- Easy handling of missing data
- Powerful data transformation functions
- Built-in integration with NumPy
An example of using Pandas could be loading a CSV file, manipulating the data, and then exporting it back to a different format. This versatility makes it a staple in the toolkit of data scientists and analysts alike.
Web Development with Django and Flask
For web development, Python offers robust frameworks like Django and Flask. Django is a high-level framework that promotes rapid development and clean design. It promotes the DRY (Don't Repeat Yourself) principle, which is crucial for writing clean and efficient code. Some of its major features include:
- Built-in admin interface
- ORM for database management
- Security features against SQL injection and cross-site scripting
On the other hand, Flask is a micro-framework, ideal for smaller applications or when developers want more flexibility. It is lightweight and allows developers to build web applications quickly and efficiently, emphasizing simplicity and extensibility. This framework is particularly useful for creating APIs and small-scale applications, as it does not impose a specific project structure or dependencies.
Machine Learning with TensorFlow and Scikit-learn
When it comes to machine learning, TensorFlow and Scikit-learn are two prominent libraries used in the Python community. TensorFlow, developed by Google, is a powerful library for numerical computation and machine learning. It allows users to build complex models and deploy them across multiple platforms. Some features that stand out include:
- Scalability for large datasets
- Support for deep learning algorithms
- Flexibility for model deployment
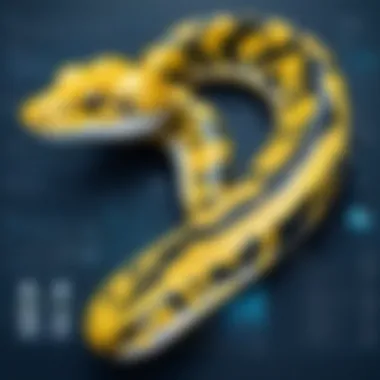
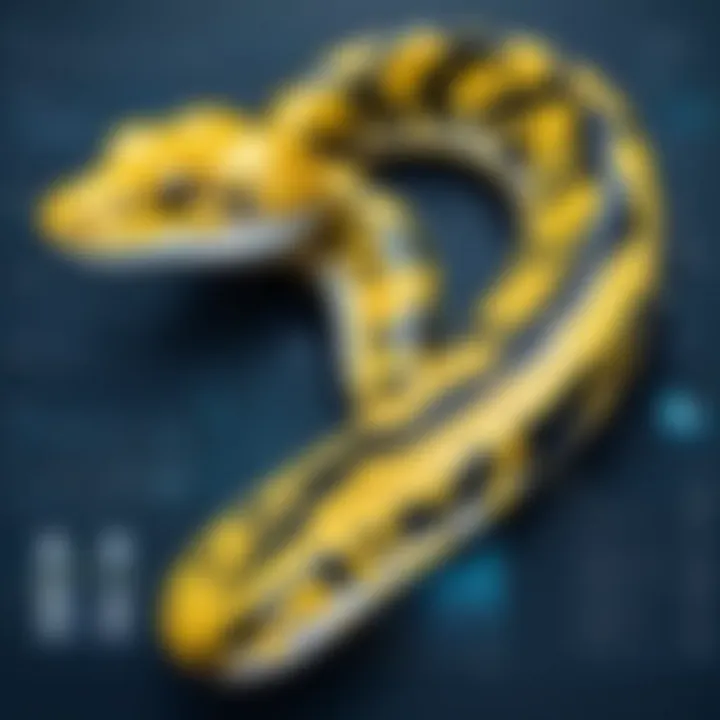
Scikit-learn, however, is focused on traditional machine learning methods. It simplifies the tasks of classification, regression, and clustering, making machine learning accessible to a broader audience. It provides a range of tools for model evaluation and selection, which is vital for developing high-performing models.
Python in Data Science
Python has established itself as a dominant programming language in the field of data science. Its importance arises from its ease of use combined with powerful libraries that simplify complex processes. The language supports a variety of tasks such as data analysis, manipulation, and visualization, which are crucial in making data-driven decisions.
One of the key benefits of using Python in data science is its versatility. It allows data scientists to perform extensive analyses using a single language. Also, Python’s robust support from community libraries means that practitioners can access numerous pre-built functionalities for specific tasks. This lowers the barrier to entry for beginners while providing seasoned data scientists advanced tools at their disposal.
Furthermore, Python promotes reproducibility in data science practices. Using well-structured code and thorough documentation increases the familiarization of others with one's workflows. This quality is essential in collaborative environments, ensuring that results can be consistently interpreted and replicated.
Data Visualization Libraries
Data visualization plays a critical role in data science. It helps convert complex results into easily understandable visual formats. Python features a range of libraries for this purpose. Two of the most prominent libraries are Matplotlib and Seaborn.
Matplotlib
Matplotlib is a foundational library widely used for creating static, interactive, and animated visualizations in Python. One specific aspect of Matplotlib is its capability to produce high-quality charts and figures for publication. It allows users to generate a variety of plots such as line graphs, scatter plots, and bar charts.
A key characteristic of Matplotlib is its flexibility. This library offers extensive options for customizing almost every aspect of a figure, including size, color, and style. This makes Matplotlib a popular choice among data scientists looking to tailor their visual outputs according to audience needs.
One downside, however, could be its steep learning curve. Beginners may find the number of options and commands overwhelming. Nevertheless, once familiarized, the library proves to be immensely powerful in creating detailed graphs.
Seaborn
Seaborn builds on Matplotlib and simplifies the creation of attractive statistical graphics. A specific aspect of Seaborn is its ability to work naturally with Pandas DataFrames and produce informative visualizations with minimal effort.
The key characteristic that sets Seaborn apart is its default aesthetic style. This means that plots created are visually appealing without needing as much customization as Matplotlib figures require. Because of this, Seaborn is often favored for rapid exploratory data analysis.
However, while Seaborn is excellent for specific types of statistical plots, it might not cover all visual requirements. Advanced visualizations may still necessitate falling back on Matplotlib functionality. This aspect highlights a consideration in the choice between these libraries; understanding their strengths helps utilize them effectively.
Statistical Analysis Tools
In addition to visualization, Python boasts powerful tools for statistical analysis, with NumPy and SciPy standing out as leading libraries.
NumPy
NumPy is a fundamental package for numerical computation in Python. It provides support for large multi-dimensional arrays and matrices. One vital aspect of NumPy is its efficient operations using vectorization, which accelerates data processing significantly.
The ability to perform complex mathematical calculations using straightforward syntax makes NumPy a beneficial choice for data scientists. This reduces developmental time and increases productivity. Moreover, its vast array of mathematical functions allows for a wide range of statistical computations, which further enhances its utility.
However, using NumPy effectively may require familiarity with its array-oriented programming model. New users might initially find accessing particular data structures a bit challenging, leading to learning overhead.
SciPy
SciPy extends NumPy's capabilities by providing additional modules for optimization, integration, interpolation, and more. A specific aspect of SciPy is its focus on advanced mathematical functions that include linear algebra and calculus.
One key characteristic is the seamless integration it offers with NumPy. This connection allows users to perform sophisticated scientific computations efficiently. This makes SciPy a preferred library in academia and industry for research purposes.
A potential disadvantage might be the complexity involved in its advanced features. While those in need of basic statistical functions may find it straightforward, the more complex operations can require a deeper understanding of both SciPy and mathematics overall.
The integration of libraries like NumPy, SciPy, Matplotlib, and Seaborn illustrates Python's extensive ecosystem in data science, highlighting its importance in transitioning raw data into actionable insights.
Application of Python in Machine Learning
Python has become the language of choice for many professionals working in the field of machine learning. The language’s simple syntax and flexibility make it highly accessible. Moreover, Python supports a wide range of libraries and frameworks that streamline the implementation of machine learning algorithms. This section explores the significance of Python in machine learning, the core concepts involved, and how to implement various algorithms effectively.
Prolusion to Machine Learning Concepts
Machine learning is a subset of artificial intelligence that focuses on the development of algorithms that allow computers to learn from and make predictions based on data. This involves training models using historical data so they can identify patterns and make informed decisions about new, unseen data.
To grasp the importance of Python in machine learning, consider the following core concepts:
- Data Preprocessing: Cleaning and organizing data is crucial for effective model training. Python provides libraries, like Pandas, that simplify data manipulation tasks.
- Model Selection: Choosing the right algorithm for a specific problem is essential. Python offers numerous machine learning libraries, such as Scikit-learn, that include a diverse range of algorithms.
- Training and Testing: In machine learning, a model is trained on a portion of the dataset and tested on another. Python's tools help automate and validate this process easily.
Python's syntax allows practitioners to express complex algorithms in a clear manner, bridging the gap between theoretical concepts and practical implementation.
Implementing Algorithms with Python
With Python’s extensive libraries, implementing machine learning algorithms becomes straightforward and effective. Let's go over some steps involved in this process:
- Import Necessary Libraries: To start, import libraries like Scikit-learn, NumPy, and Pandas that provide the fundamental functionalities.
- Load and Prepare Data: Load your dataset. Clean it as needed and split it into features (X) and labels (y).
- Split the Data: Divide the data into training and testing sets. This helps evaluate model performance.
- Train the Model: Select a machine learning algorithm, such as Random Forest, and train it on the training data.
- Evaluate the Model: Finally, test the model's accuracy on the testing set to ensure its effectiveness.
Python for Web Development
Web development has become an essential skill in today's digital world. With the growing demand for web applications, Python plays a pivotal role due to its simplicity and efficiency. It allows developers to create powerful applications quickly. Python's versatile frameworks offer a robust solution that can meet the requirements of diverse projects. Though static websites are common, many organizations wish to create dynamic web applications that interact with users and handle data more effectively. Python excels in this area, making it a popular choice.
One major benefit of using Python for web development is its readable and concise syntax. It reduces the learning curve for beginners and allows experienced developers to write code efficiently. Furthermore, Python has a vast repository of libraries and frameworks that enhance productivity. Two of the most popular frameworks are Flask and Django, each serving unique needs for web development.
Building Web Applications with Flask
Flask is a lightweight framework that is easy to get started with. It is often referred to as a micro-framework. This means that it provides the essential features but does not impose heavy structure or tools upon the developer. This flexibility makes Flask particularly appealing for small applications or for developers who prefer to build their architecture from scratch.
Flask allows for rapid prototyping, enabling developers to quickly iterate on their ideas. It supports extensions that provide additional functionalities, such as form validation and user authentication. These extensions can integrate smoothly into the app structure.
To create a basic web application using Flask, developers can start with the following simple code:
This code sets up a basic web server that responds with "Hello, World!" when accessed. It exemplifies the simplicity of Flask, allowing developers to delve into the core functionalities with minimal setup.
Creating Dynamic Websites with Django
Django, on the other hand, is a full-featured, high-level framework designed for larger applications. It follows the "batteries included" philosophy, meaning it comes with various built-in features that reduce the need for third-party add-ons. This framework includes an ORM (Object-Relational Mapping), form handling, and an authentication system.
One notable feature of Django is its "admin panel," which makes it easy to manage application data directly. Developers find it valuable when creating back-end applications for managing user accounts or content submission. Django is also known for its security features, which help to create secure web applications. It offers protections against common vulnerabilities, such as SQL injection and cross-site scripting.
Using Django, a developer can set up a project with just a few commands. After installation, the following command creates a new project:
By simplifying the web development process, Python frameworks like Flask and Django allow developers to focus on building innovative applications rather than getting bogged down in technical details.
Overall, Python's application in web development demonstrates its adaptability and effectiveness in real-world scenarios. Whether opting for Flask's simplicity or Django's robust functionalities, Python provides tools to build dynamic and scalable web applications.
Testing and Debugging in Python
In software development, ensuring code quality is paramount. Testing and debugging are integral parts of this process. They help developers identify issues early, ensure functionality, and improve the overall reliability of applications. In Python, these practices are not only essential but also facilitated by various tools and frameworks. They lead to cleaner and more maintainable code.
Testing is often the first line of defense. It involves running specific code segments to evaluate correctness. Debugging follows, which is the process of finding and fixing bugs or issues in the code. Together, they provide a comprehensive approach to quality assurance in Python programming. The benefits of these practices are vast: they increase confidence in code, reduce the time spent on fixing problems later, and enhance team collaboration by establishing clear expectations around code functionality.
Unit Testing Framework
Unit testing is a practice that focuses on testing individual components of code. It helps ensure that each unit works as expected. In Python, the framework is a powerful tool to facilitate this process.
Here are some key features and benefits of using unit tests in Python:
- Automated Testing: Tests can be easily automated. This is beneficial for continuous integration and deployment workflows.
- Immediate Feedback: Developers receive instant feedback on their code. If a change breaks something, it can be addressed immediately.
- Code Coverage: Unit tests help assess how much of the code is tested. This provides insights into potential problem areas.
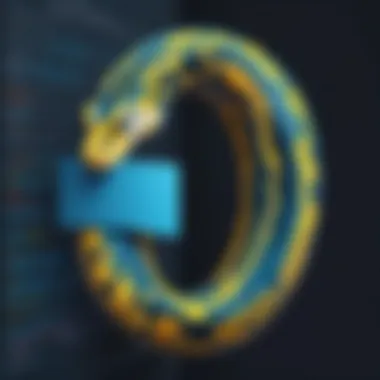
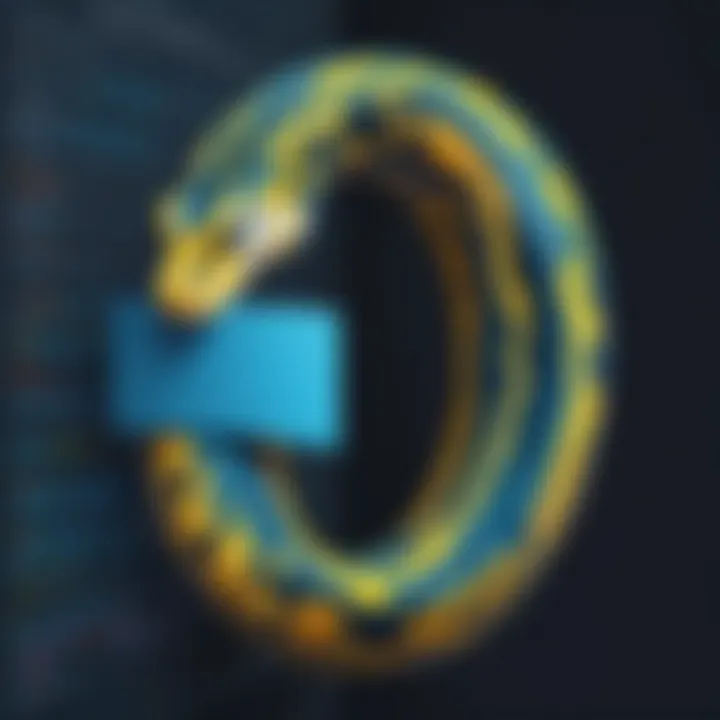
A sample unit test using the framework could look like this:
In this example, a simple function is tested to check its accuracy. The module offers a structured way to create and run tests, enhancing code reliability.
Debugging Techniques
Debugging can be a complex task, especially in larger projects. Python provides several tools to simplify the debugging process. Here are some effective debugging techniques:
- Print Statements: The simplest form of debugging. Inserting print statements helps track the flow and values of variables during execution.
- Logging: Unlike print statements, logging offers more control and can be adjusted to different verbosity levels. Using the module can help maintain records without cluttering the output.
- Using a Debugger: Python’s built-in debugger, , allows for interactive debugging. You can set breakpoints, step through code, and inspect variables.
- Integrated Development Environment (IDE) Tools: Many IDEs like PyCharm and Visual Studio Code come with built-in debugging tools. They provide a graphical interface to set breakpoints and inspect the call stack.
"Debugging is like being the detective in a crime movie where you are also the murderer."
— Unknown
Best Practices in Python Programming
Best practices in Python programming are essential for maintaining high-quality code and ensuring that projects are manageable and scalable over time. They provide guidelines that help programmers avoid common pitfalls and inefficiencies. By adhering to these best practices, developers can improve code readability, enhance performance, and facilitate collaboration across teams. This section will explore key elements of these practices, focusing on code style, documentation, and performance optimization.
Code Style and Documentation
Using a consistent code style is vital for any programming project. It makes the code easier to read and understand, which is important for both the original developer and others who may work with the code later. The PEP 8 style guide is widely regarded as the standard for Python code. It provides a comprehensive set of guidelines regarding naming conventions, line length, indentation, and spacing. By following these recommendations, developers can create clean and organized codebases.
Documentation is equally as important as style. Clear comments and documentation strings enhance understanding. Each function should explain its purpose, inputs, outputs, and any exceptions that may arise. Using tools such as Sphinx can help automate the documentation process, making it easier to keep documentation up-to-date. Good documentation not only aids in maintaining the code but also benefits new team members or contributors who need to understand the project quickly.
"Clear code is better than clever code."
Incorporating both code style and documentation into programming workflows can positively impact the overall success of a project.
Optimizing Performance
Performance optimization in Python consists of several strategies that can significantly enhance application efficiency. Given Python's interpreted nature, its performance may not match that of compiled languages like C or Java. However, there are techniques to improve performance.
One common method is to minimize the use of global variables, as accessing them can be slower than working with local variables. Additionally, using built-in functions is generally faster than writing custom implementations. For example, list comprehensions are often more efficient than using traditional for-loops.
To analyze performance, profiling tools such as cProfile can help identify bottlenecks. Understanding which part of the code consumes the most resources can guide developers in focusing optimization efforts where they will be most impactful.
It is also essential to be aware of memory usage. Using generators instead of lists can reduce memory overhead. With , one can produce items one at a time instead of storing them all in memory.
In summary, Python programming best practices are critical for creating effective and maintainable code. By emphasizing code style, documentation, and performance optimization, developers can build robust applications that stand the test of time.
Advanced Python Concepts
In the realm of programming, understanding advanced concepts is vital for developing robust and scalable applications. In Python, this includes Object-Oriented Programming (OOP), decorators, and context managers. Each of these elements plays a significant role in making Python a powerful tool for developers. Mastering these concepts can enhance code quality, increase efficiency, and streamline complex tasks.
Object-Oriented Programming
Object-Oriented Programming, or OOP, is one of the core principles behind Python’s design. It allows developers to model real-world entities using classes and objects. A class defines the structure of objects, while objects are instances of classes. OOP promotes greater code reusability and organization. By grouping related functionality together, it simplifies maintenance and debugging.
Key benefits of using OOP in Python include:
- Encapsulation: This principle restricts direct access to some components and protects object integrity. It allows developers to hide the internal state and only expose a controlled interface.
- Inheritance: This feature enables new classes to inherit properties and methods from existing ones. This leads to cleaner code and reduces redundancy by allowing specifications and implementations to be extended.
- Polymorphism: With polymorphism, one interface can represent different data types. This promotes flexibility and allows for easier code updates in response to changing requirements.
Overall, utilizing OOP in Python facilitates writing scalable and maintainable code, which is essential in professional programming environments.
Decorators and Context Managers
Decorators are a key feature in Python that helps modify the behavior of a function or method without changing its code. They allow for wrapping another function, adding useful behaviors, while keeping the original interface intact. Common use cases for decorators include logging, access control, and performance testing.
Benefits of decorators include:
- Code Reusability: They allow you to generalize behavior across multiple functions without repeating code.
- Encapsulation of Logic: By separating concerns, decorators keep your codebase cleaner and easier to understand.
Here is an example of a simple decorator in Python:
Context managers, on the other hand, provide a way to allocate and release resources precisely when you want them. The most common example is file handling. With context managers, you can ensure that resources are managed appropriately. This means that files will automatically close after being processed, preventing potential resource leaks.
Using the statement creates a context where the resource is in use. Here is an example of using a context manager for file I/O:
Both decorators and context managers add significant value to Python programming by making the code cleaner and reducing the risk of errors.
Python in Cybersecurity
Python has become a significant tool in the field of cybersecurity due to its versatility and ease of use. The rapid growth of cyber threats has pushed professionals to seek efficient and effective solutions for security operations. Python's wide array of libraries and frameworks makes it particularly suited for this domain, enabling programmers to develop scripts and tools that enhance security measures.
Benefits of Python in Cybersecurity
- Simplicity: Python's clear syntax allows security professionals to quickly write and understand code, making it ideal for those who may not have a programming background.
- Library Support: Libraries like Scapy for network packets manipulation, and Requests for HTTP requests, provide robust tools that facilitate numerous security tasks.
- Cross-Platform Compatibility: Python scripts can run on various operating systems, which is essential for handling diverse technical environments.
"Using Python in cybersecurity enables rapid development of tools needed to address pressing security needs."
Considerations for Using Python in Security Operations
While Python offers many advantages, there are also significant considerations:
- Performance: For resource-intensive tasks, Python may not match the speed of lower-level languages like C. It is essential to assess the requirements of a given task before implementation.
- Security of Scripts: Just like any other coding language, Python scripts must be secured properly to prevent vulnerabilities.
- Continuous Learning: The dynamic nature of cybersecurity requires programmers to remain up to date with the latest libraries and tools.
Scripting for Security Operations
Scripting is a fundamental aspect of cybersecurity, and Python shines in this aspect. Security professionals use Python scripts for various operations, including:
- Automating Routine Tasks: Automating repetitive tasks such as log analysis, vulnerability assessment, and reporting can save valuable time.
- Developing Exploits and Penetration Testing Tools: Python is widely used to create tools that simulate attacks on systems to test their defenses. This is vital for identifying weaknesses.
- Data Extraction and Analysis: Scripting allows for effective parsing of large log files or network traffic, making it easier to identify patterns or anomalies that could indicate a security issue.
Here is a simple example of a Python script that can be used for monitoring a system log for failed login attempts:
This script continuously checks the specified log file for failed login attempts, providing real-time monitoring of potential unauthorized access attempts.
Future of Python
The future of Python programming language is a topic ripe for discussion, considering its widespread adoption and versatility in various fields. This section explores some of the significant trends influencing its trajectory. Understanding these factors is essential for aspiring programmers and IT professionals alike, as it shapes how they should approach learning and applying Python.
Python's growing role in data science, machine learning, and artificial intelligence is of particular note. These areas are expanding rapidly, and Python's ease of use coupled with its robust libraries positions it as a top choice for developers. Tools like TensorFlow and PyTorch facilitate complex computations, making Python a preferred language for researchers and engineers in these domains.
Moreover, the increasing demand for automation and scripting in various industries bolsters Python's relevance. As businesses strive for efficiency, Python’s simple syntax makes it accessible for automating mundane tasks. This pattern not only attracts newcomers but also encourages industry professionals to integrate Python into their workflow.
Python's ability to adapt to emerging technologies ensures its relevance as the tech landscape evolves.
Trends and Predictions
In looking at the trends that will shape Python’s future, several key observations can be made:
- Increased Adoption in Education: Python is becoming the go-to language for teaching programming in schools due to its readability and straightforward syntax. This trend is likely to continue, producing a generation of developers proficient in Python.
- Expansion in AI and Machine Learning: Companies are investing heavily in AI capabilities. Python’s frameworks and libraries will see continuous updates to maintain their usability in cutting-edge AI research and development.
- Focus on Performance Improvements: As Python is not as fast as some compiled languages, there are ongoing efforts to improve its performance. Projects like PyPy aim to offer faster implementations and make Python more efficient for large-scale applications.
- Community and Ecosystem Growth: The Python community remains vibrant and supportive, facilitating knowledge sharing and innovation. Continued contributions from developers around the world will lead to new libraries and frameworks, enhancing Python's capabilities.
Finale
The conclusion of this guide serves to encapsulate the various elements that have been discussed throughout the article, emphasizing the significance of the Python programming language in today's tech landscape. Python is not just another programming language. It represents a powerful tool that combines simplicity with versatility, making it accessible to newcomers while also appealing to experienced developers.
In our analysis, it becomes clear that Python's clear syntax and robust libraries are key factors behind its broad adoption across industries ranging from web development to artificial intelligence. This language empowers users to focus on problem-solving rather than being bogged down by complex syntax.
Furthermore, the framework of this guide highlights how Python fits into various domains such as data science, web development, and machine learning. Each application offers unique benefits, such as extensive functionalities in data manipulation with libraries like Pandas, or rapid prototyping capabilities through frameworks like Flask and Django.
"Python continues to expand its influence across sectors, simplifying tasks while enabling advanced functionality."
The consideration of best practices in Python programming, which were discussed, further reinforces the importance of writing efficient and maintainable code. This is essential in professional settings where team collaboration and code longevity matter.
As we synthesize the information, it’s also essential to note Python's commitment to community and support. The vibrant ecosystem encourages learning and collaboration, which is vital for anyone looking to enhance their programming skills.
In summary, Python stands as a foundation in the tech industry today due to its functionality, ease of use, and a plethora of applications. Understanding its importance provides an outstanding advantage for both students and IT professionals navigating this continually evolving tech landscape. Engaging with this language means choosing a path rich in possibilities and innovation.