Mastering f-strings in Python: A Complete Guide
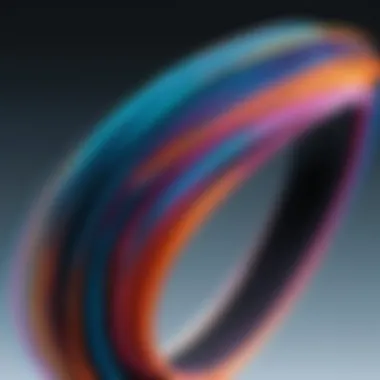
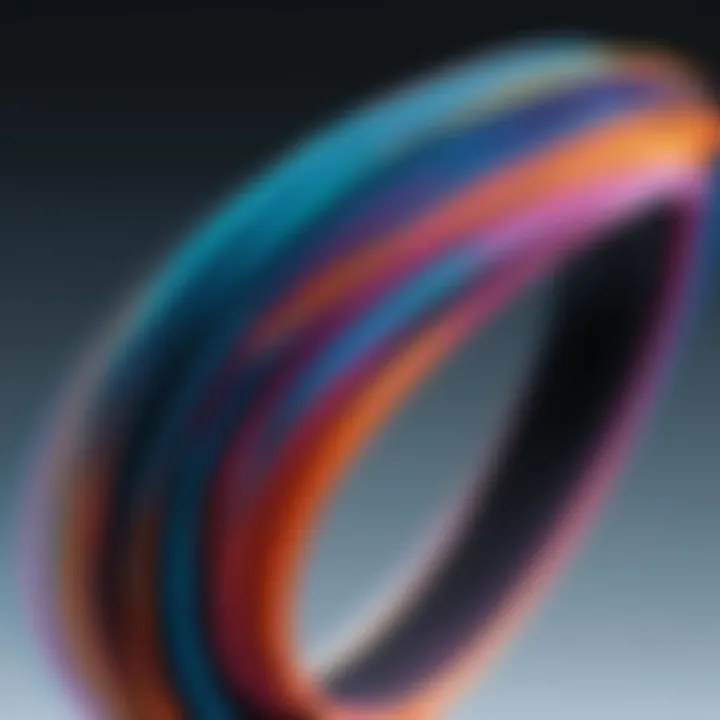
Overview of Topic
Preamble to the Main Concept Covered
Scope and Significance in the Tech Industry
The importance of f-strings cannot be overstated in Python programming. They simplify code, making it less error-prone and offering better performance than older string formatting methods. Given the growing demand for clean, maintainable code, they fit well into contemporary development practices.
Brief History and Evolution
Python has seen various string formatting methods since its inception. Traditional methods included formatting and the function. However, these methods were often cumbersome and less intuitive. The addition of f-strings marked a significant advancement in Python’s idiomatic ways of handling strings, making it easier for new developers to understand and work with.
Fundamentals Explained
Core Principles and Theories Related to the Topic
F-strings are straightforward but powerful. They are prefixed with an or , allowing embedded Python expressions that are evaluated at runtime. This functionality lets the programmer insert variables or expressions directly into the string without complex syntax.
Key Terminology and Definitions
- f-string: A string literal prefixed with 'f' that can contain expressions inside curly braces.
- Embedding: The process of inserting an expression into a string for evaluation during execution.
- Interpolation: The method of replacing placeholders in a string with variable values or expressions.
Basic Concepts and Foundational Knowledge
Understanding f-strings requires an understanding of basic Python syntax. Variables can be included straightforwardly, and operations can happen within the curly braces. For example:
This example demonstrates how f-strings can condense multiple functionalities into one succinct line.
Practical Applications and Examples
Real-world Case Studies and Applications
Many software applications benefit from the clarity of f-strings. For instance, logging frameworks can effectively provide clean output messages that use f-strings to incorporate dynamic information, enhancing debugging.
Demonstrations and Hands-on Projects
Change Example: Logging Future Events
Here's a basic logging example using f-strings to formulate messages:
In this case, the developer can quickly implement insightful log messages with ease.
Code Snippets and Implementation Guidelines
Utilizing f-strings enhances code readability. The following illustrates this point:
The format specifier ensures the float is always displayed with two decimal points.
Advanced Topics and Latest Trends
Cutting-edge Developments in the Field
The ongoing enhancements in Python continually reinforce f-strings' importance. New features are being developed to improve performance and efficiency while addressing the evolving needs of programmers.
Advanced Techniques and Methodologies
Fast checks in large datasets could leverage f-strings effectively to increase speed in reports or data views. For large-scale applications, f-strings reduce execution time compared to slower alternatives without contrived syntax.
Future Prospects and Upcoming Trends
F-strings may further evolve with Python's improvements to type hints and typing. This could introduce more complex embedding abilities while increasing code safety in larger projects.
Tips and Resources for Further Learning
Recommended Books, Courses, and Online Resources
To grasp f-strings deeply, explore these resources:
- Automate the Boring Stuff with Python by Al Sweigart
- Python Crash Course by Eric Matthes
- Online course at Coursera or edX.
Tools and Software for Practical Usage
Using Integrated Development Environments like PyCharm or Jupyter Notebooks can optimize your f-string development and debugging experience. Reliable access to Python's official documentation at en.wikipedia.org) also provides valuable insights.
Preamble to Python Print Function
The print function in Python plays a crucial role in displaying output to the user. It serves as a primary method for providing insight into what the code is doing at various stages. This function is vital for debugging, allowing programmers to check their logic until they reach the desired results.
Moreover, understanding how to efficiently utilize the print function sets the foundation for exploring more advanced printing techniques, such as f-strings. F-strings simplify formatting, making the output more readable and easier to interpret immediately.
Historical Overview
The history of the print function in Python is intertwined with the evolution of the language itself. Introduced in Python 2, the functioning of the print statement required additional care, particularly in differentiating between versions and dealing with different types of output. Whereas earlier Python versions treated print as a statement, newer versions, from Python 3 onward, implemented it as a function. This change presents new advantages, offering optional features such as sep and end, which allow further flexibility
In the early stages of Python, printing was sufficient for basic output needs. However, as the programming community grew, so did the requirement to enhance output formatting capabilities. With the introduction of more complex data structures and types, developers increasingly needed to visualize data effectively. The shift toward f-strings can seen as a resolution to these requirements.
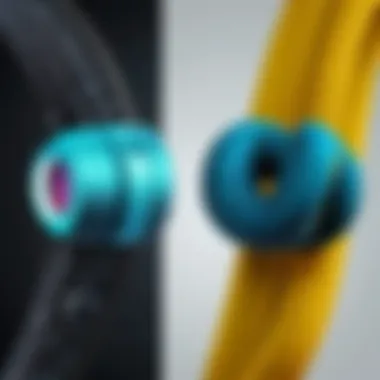
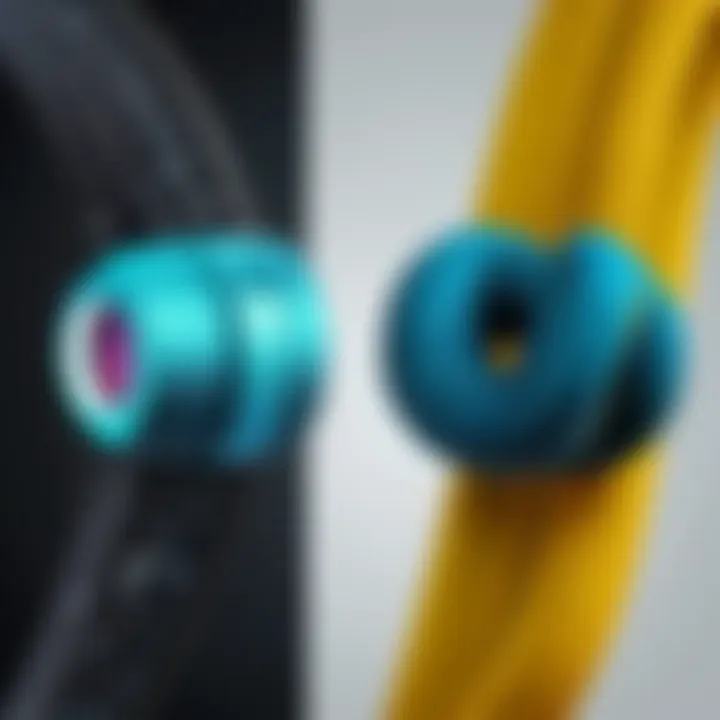
Basic Syntax of the Print Function
The syntax for the print function in Python is quite straightforward. It primarily consists of the word followed by a set of parentheses, encapsulating the objects to be printed.
In this example, the string is displayed in the console. The print function can accept multiple arguments, enabling more complex output combinations:
This prints , using a default space as the separator. The print function also accepts various parameters like , which specifies a custom separator, and , which determines what gets printed after the output.
Understanding Output Formatting
Effective output formatting is essential. The print function provides basic output capabilities, yet there is much flexibility available to developers seeking precise control over their printed content.
- String Concatenation
You can concatenate strings in the print function. - Using Commas for Separation
This is an efficient way to avoid manual concatenation. With commas, the print function inherently adds white spacing - Colorizing Output
While the print function do not directly offer colors, various libraries can add this feature.
In summary, the print function is integral, not just in displaying output but in establishing pathways to advanced formatting options. Considering these principles helps underscore why transitioning to f-strings could be an important enhancement in a developer's toolbox.
Prologue to f-strings
F-strings, introduced in Python 3.6, bring a fascinating evolution in handling string formatting in Python. Understanding them is vital for anyone working with Python, as they enhance clarity, efficiency, and features compared to earlier string methods. Their introduction marked a significant simplification in the methods programmers use for outputting strings, particularly when involving complex variable expressions and outputs.
What are f-strings?
For example:
The syntax is straightforward, enabling programmers to quickly incorporate variable values directly within their strings.
F-strings Syntax Explained
The syntax for f-strings includes the variable names directly placed within curly braces within an f-prefixed string. This allows the strings to seamlessly integrate variables’ values. The presence of curly braces indicates a substitution is taking place. Adjustments can be done directly on the formatting applied within the curly braces.
Here’s an example:
It displays the value formatted to two decimal places using standard formatting options such as for floats.
Key Benefits of Using f-strings
F-strings offer various advantages over other string formatting methods. Some of the key benefits include:
- Efficiency: F-strings are often faster than the method or the old -based formatting. The performance improvement is noticeable especially with longer strings and multiple variables.
- Simplicity: Their syntax is clean and empowers developers to read and write strings that may have multiple embedded expressions with greater ease.
- Flexibility: Programmers can use arbitrary Python expressions directly within f-strings, allowing for dynamic and complex formatting requirements without the need for unpacking.In summary, understanding f-strings is essential due to their robust nature, which complements the need for effective and efficient string handling. As coding applications grow more diverse, exchanging knowledge on precise formatting options, errors, and best practices helps every programmer.
"F-strings have significantly optimized Python's handled string formats, making coding more efficient and maintainable".
Setting Up Your Python Environment
Setting up your Python environment is a fundamental step for anyone who aims to use f-strings effectively in their programming tasks. A conducive development setting can ease the learning process, make coding more intuitive, and help avoid common errors that may arise from an improper setup. Having the right tools ensures that practitioners can maximize the utility of features such as f-strings.
When it comes to working with Python, a variety of environments can be chosen based on needs and preferences. The setup typically includes the installation of Python itself and selecting an appropriate Integrated Development Environment (IDE) or a text editor. Each aspect influences how well the user can understand and apply f-strings, making this choice important for both new and experienced programmers.
Necessary Installations
To get started, you need to ensure that Python is installed on your system. Python can be obtained from the official Python website, which provides the latest version tailored for different operating systems. Here is how you can do it:
- Visit python.org and download the installation package relevant for your operating system.
- Run the installer and accept the licensing agreement.
- Ensure to select "Add Python to PATH" during installation for easier command line access.
- Confirm the successful installation by running or in your terminal.
In addition to Python, some libraries and tools may be necessary, particularly if you plan to experiment with advanced features of f-strings. Pip, Python's package installer, typically comes with the standard Python installation. It enables the installation of various third-party libraries, broadening the programming capabilities. Installing any additional libraries can be achieved via pip with simple terminal commands. For instance, if you need to install the library, you would enter the command:
Choosing an IDE or Text Editor
The selection of an IDE or text editor significantly impacts code writing and debugging experiences. Various options fit different user types, and understanding your needs is essential. If you are looking for simple text editing, Notepad++ or Sublime Text can suffice. More expansive IDEs like PyCharm or Visual Studio Code offer comprehensive features that enhance coding efficiency as well.
Here are a few considerations when making the choice:
- Feature Set: Choose based on your specific needs. An IDE provides advanced features like debugging tools and code suggestions.
- Usability: A user-friendly interface can streamline the coding process, especially for beginners.
- Community and Support: A well-supported tool ensures you can find help when you encounter challenges.
Implementing f-strings in Python
The implementation of f-strings in Python represents a significant leap in string formatting. This section addresses both the ease and versatility they bring to Python programming. F-strings, introduced in Python 3.6, allow for more straightforward embedding of variables and expressions within strings. Their clear and concise syntax makes it easier for developers to read and write their code, aligning with Python's philosophy of simplicity.
By examining various usage examples, the article will elucidate both benefits and potential pitfalls when introducing f-strings in code. Mastery of implementing f-strings empowers programmers to write less verbose and more expressive code, thus leading to a more enjoyable coding experience. Here are specific aspects that you will gain insight on:
- Ease of use and clarity
- Enhanced readability versus older string formatting methods
- Practical examples demonstrating various techniques
Basic Usage of f-strings
To grasp the fundamentals, it is crucial to first learn how to create f-strings. They are prefixed by the letter ‘f’ or ‘F’ and allow you to seamlessly integrate expressions into string literals. The primary purpose is to provide an easily identifiable format for including Python expressions inside string statements.

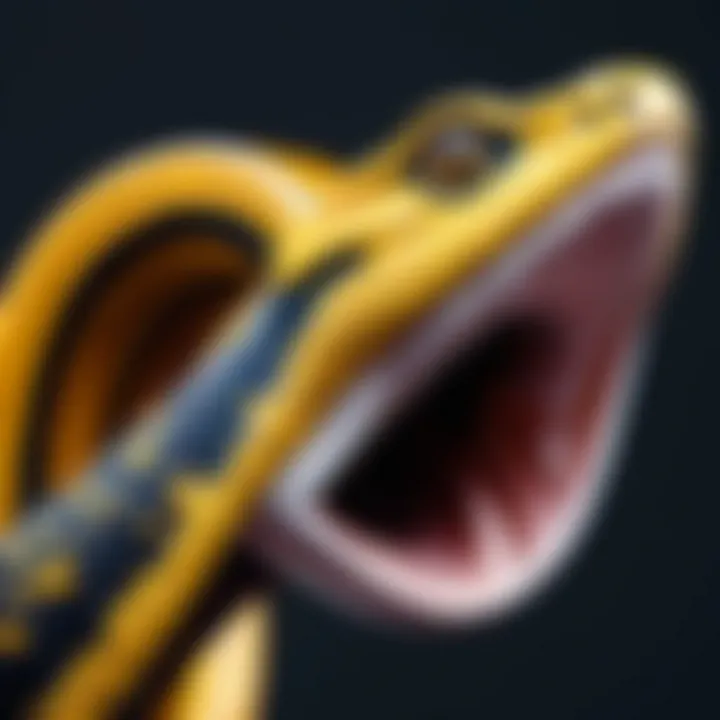
A basic example goes like this:
This code acknowledges that the output will directly represent the current values of and variables, resulting in:
The simplicity of this syntax not only reduces the chance of syntax errors but is also more satisfactory for developers.
f-strings with Expressions
F-strings also support more than just variable substitution. You can evaluate basic arithmetic, call methods, or run function calls directly within the curly braces. The flexibility enhances their utility in practical applications.
For instance:
This outputs:
Using expressions within f-strings helps condense operations that might otherwise clutter code, ensuring readability while merging functionality.
Using f-strings for String Interpolation
String interpolation with f-strings allows embedding of values into strings dynamically. This means you can include complex expressions or computed values in string literals, enhancing the expressiveness of your code. For example:
In this case, the formating restricts the output to two decimal places, which is highly useful for monetary values and gives a provisional presentation to the print function, illustrating precision. The output will be:
The potential for combining varying types of outputs directly within strings defines a new era of possibilities while coding with Python.
Advanced Features of f-strings
F-strings in Python present not only a straightforward syntax for string interpolation but also advanced features that enhance their functionality. Understanding these robust capabilities is essential for any developer who aims to leverage Python’s printing abilities effectively. Advanced features can save time, reduce code complexity and improve both readability and performance.
Formatting Numbers and Dates
F-strings allow for sophisticated formatting of numbers and dates directly within the string construction process. Using them eliminates the need for external functions or complicated formatting techniques previously prevalent in older methods.
To format numbers, one can utilize a colon followed by a specified format type within the curly braces of an f-string. This method can adjust precision, display percentage forms and format currency efficiently.
Examples of Number Formatting:
- Floating Point Precision: You can limit floating-point numbers to a specific number of decimal places by using , where is the count of decimal places, like so:Result: The value rounded to two decimal places is: 123.46
- Percentage Representation: You can represent a fraction as a percentage by appending a symbol, as shown below:Result: The percentage is: 67.99%
- Currency Formatting: For currency values, you might include additional specifications such as alignment or the inclusion of commas:Result: The total amount is: $1,500.00
For manipulating dates, f-strings support functionality that allows for formatted date strings easily. Using the method from the module enables customization of date displays very simply.
Example for Date Formatting:
Result: Today’s date is: 2023-10-25
This inclusiveness of number and date formatting within f-strings greatly enhances readability and correctness of the output. While maintaining a clean and efficient codebase, these functionalities illustrate why anyone working with Python should adopt f-strings.
Calling Functions within f-strings
One notable feature of f-strings is the ability to call functions directly within the string. This capability allows for dynamically generated content without the overhead of auxiliary variables. Interest grows as code conciseness improves while eliminating steps in the process.
For instance, you can directly include function results in an f-string which can significantly streamline the coding process.
Example of Function Calling in f-strings:
Result: The square of 4 is: 16
By integrating logic directly in print statements using f-strings, the readability of the code improves consistently. This facilitates debugging as comparisons between input and result appear side by side.
Additionally, these capabilities promote a more exploratory programming style, where developers can encapsulate logical operations seamlessly within strings. Participants interested in functional programming find this aspect particularly compelling.
As one programmer noted, integrating functions within f-strings provides not just utility, but also elegance, yielding cleaner scripts overall.
Common Pitfalls and Issues
Understanding the potential pitfalls and issues associated with using f-strings in Python is crucial for both new and experienced programmers. Awareness of these challenges ensures better code quality and reduces frustration down the line. F-strings offer a modern and powerful method of string formatting. However, they are not without their drawbacks. Exploring these common problems helps users to navigate potential obstacles efficiently, maximize the advantages f-strings provide, and maintain clean code practices.
Common Syntax Errors
Syntax errors are the most frequent issues developers encounter when working with f-strings. These errors often stem from improper use of curly braces or quotes. An f-string starts with the letter ‘f’ or ‘F’ followed by a string enclosed in quotes. Inside these quotes, any expression should be placed within curly braces. Here are some typical mistakes:
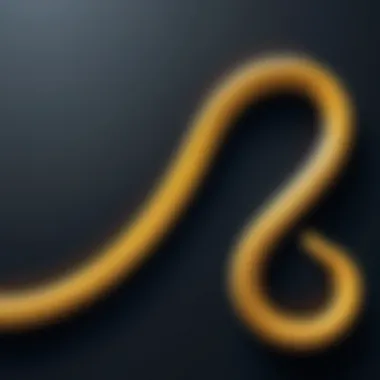
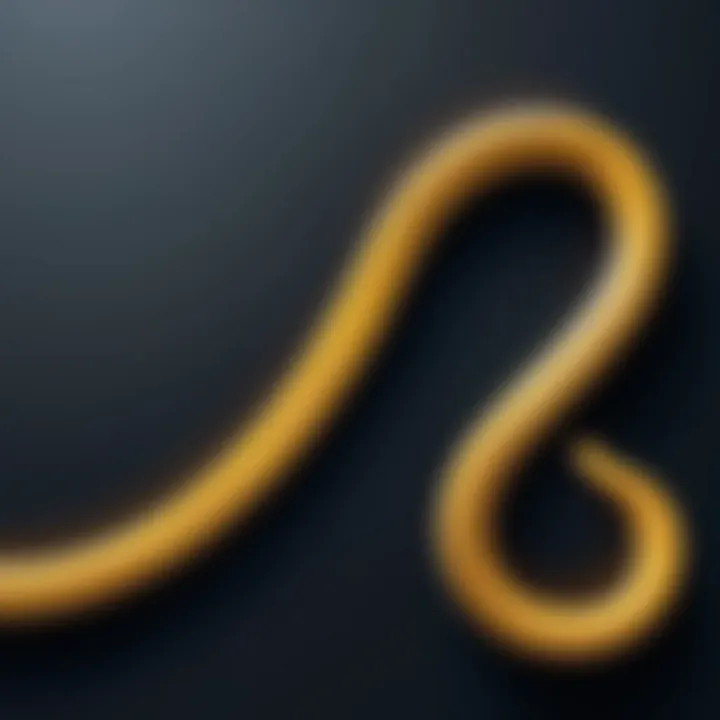
- Omitting the opening or closing curly brace can lead to a .
- Using single quotes inside a f-string formatted with single quotes can confuse the parser, resulting in an error.
- Not escaping special characters can also create problems. For instance, to include a single quote within a single-quoted f-string, you must escape it with a backslash.
Example of Common Syntax Error
Suppose you try this line of code:
This will throw a due to the mismatched curly brace. Standardizing your use of quotes and being vigilant about braces and escape characters will help mitigate these problems. Frequent debugging can create additional developmental overhead if not addressed early.
Compatibility Considerations
Compatibility is another important aspect when exploring f-strings. F-strings were introduced in Python 3.6. Therefore, any systems running an earlier version will not support this feature, leading to errors or no output at all. It is essential to check the deployment environment to ensure that all systems involved are equipped with at least Python 3.6.
When writing code intended for a broader audience, you may need to provide fallback options for earlier versions. Developers can achieve a similar result by using alternative string formatting methods.
Another compatibility concern relates to how strings and unicode are handled across different platforms. Keep this in mind, especially when your application has potential users across various operating systems.
While developing scripts that utilize f-strings, it can be helpful to rely on a version management tool such as . To streamline onboarding new developers mastering f-strings and maintain a uniform environment, integrating these practices early can be beneficial.
Remember, understanding these common pitfalls not only saves time but improves your programming skills.
Best Practices for Using f-strings
Using f-strings can significantly enhance your Python coding experience. By adhering to best practices, you can make your code more efficient and easier to understand. Best practices for f-strings involve careful consideration of readability, performance, and maintainable code. A commitment to these principles aids in producing high-quality Python scripts, which is essential for programmers at any level.
Maintaining Readability
Readability is crucial in programming, especially in collaborative environments. f-strings create clear and concise outputs, but proper implementation is equally important. Here are a few practices to keep code readable:
- Keep Expressions Simple: Avoid embedding overly complicated expressions within f-strings. Instead, compute complex values before the f-string. This makes the f-string easier to read and understand.
- Utilize Multi-line f-strings: When needed, break your f-string into multiple lines. Python allows this if you use parentheses around the values.
- Consistent Naming: Use clear and consistent variable names. This helps others (or your future self) comprehend what the code does.
In short, f-strings should enhance code readability without becoming cluttered with extraneous details.
By focusing on readability, one can ensure that the intended message of your code is conveyed effectively, thereby helping both current and future teammates who will work with the same code.
Performance Considerations
Although f-strings are fast, it's essential to understand their performance in different contexts. Being deliberate about their use can enhance performance greatly. Here are performance pointers:
- Choose f-strings for Concatenation: In many scenarios, f-strings outperform traditional string formatting methods like or . Their syntactic simplicity means they create less overhead, improving performance.
- Consider Scenarios: Evaluate whether an f-string is the best fit, especially when used inside loops. Analyze if its performance maintains efficiency with repetitive tasks. Occasionally, repetitive calculations within f-strings can lead to slower performance.
- Avoid Escape Characters: When formatting strings that include quotes, ensure that you don't rely on backslashes (`"). Instead, explore using single or triple quotes to sidestep the issue and enhance performance.
Implementing these performance considerations allows programmers to take full advantage of the speed benefits f-strings provide, ensuring applications run smoothly. Understanding how to balance performance and readability helps in creating code that's robust and efficient.
End
In summary, f-strings represent a significant evolution in Python's string handling capabilities. This article has explored their many facets, illustrating their practicality and advantages. The benefits of clarity, maintainability, and performance have been highlighted throughout. By enabling expressive interpolation, f-strings streamline the task of presenting data, making it more intuitive for developers to create readable code.
Recapping the key points, one can pinpoint that the journey begins with understanding f-strings and the essential syntax required to harness their power. The advantages of f-strings over traditional methods prove persuasive, particularly when combined with advanced features like formatting and embedded expressions. By recognizing common pitfalls, developers can avoid unnecessary errors that might arise during implementation.
F-strings not only enhance code legibility but also boost performance, serving as a preferred choice for modern Python programming.
Encouragement to engage with f-strings should resonate broadly among tech enthusiasts and professionals. Exploring this functionality leads to greater proficiency in Python coding. Testing various formatting scenarios can unearth unique efficiencies. Moreover, digging deeper into features competes with any curiosity to improve one’s skill set effectively. It is through experimentation that one might grasp the full utility of f-strings in practice, empowering their coding journey in a complex programming landscape.
Venturing into this territory is both exciting and rewarding. настройки Regular engagement with resources like Python's official documentation or communities on platforms such as Reddit could furnish continuous learning opportunities and insights.
By embracing f-strings, developers are not just limiting themselves to mere syntax; they are advancing their method of thinking about Python programming itself.
Further Resources for Python Enthusiasts
The expasion of knowledge in Python, especially regarding its f-strings, can be significantly enhanced through various resources. As the programming landscape continues to evolve, it is valuable for learners, students, and IT professionals to access diverse sources that cater to different learning styles. Properly selected resources ensure that learners can deepen their understanding, gain new insights, and remain updated with contemporary practices.
Recommended Books
Books can serve as an excellent foundation for understanding Python and its features. Here are some trusted titles specifically addressing f-strings and string formatting:
- "Python Crash Course" by Eric Matthes: This book offers an introduction to Python, with sections dedicated to f-strings, providing practical examples and exercises.
- "Fluent Python" by Luciano Ramalho: Delving into advanced features, this book highlights Python's capabilities, including f-strings. The focus is on writing effective code and utilizing language idioms properly.
- "Automate the Boring Stuff with Python" by Al Sweigart: This accessible book is excellent for beginners. Though broad in scope, it does include relevant sections on strings and formatting techniques.
Purchasing or borrowing notable texts can elevate comprehension levels. Joining platforms like reddit.com can yield additional titles, based on community recommendations.
Online Courses and Tutorials
Online learning platforms provide versatile and interactive methods to comprehend Python. Specialized courses can dive deeply into f-strings alongside other Python topics. Here are some notable options:
- Coursera: Offers a range of Python courses like "Python for Everybody". You can see f-strings addressed within the context of essential string formatting and interactive exercises.
- edX: This platform provides professional courses covering various aspects of Python, including courses focused on advanced features like f-strings.
- Codecademy: Their hands-on programming classes help users learn coding efficiently. They include modules specifically using f-strings in context.
Many platforms also offer definite modules of tutorials that focus only on f-strings, which can address specific questions or doubts that learners have.
Exploring these resources can vastly improve an individual’s skills in Python programming and string manipulation through f-strings, equipping a learner well for real-world applications.
Questions and Answerss
Understanding the frequently asked questions (FAQs) section is essential for anyone delving into Python's f-strings. This part of the article encapsulates important inquiries that programmers often have regarding this feature. Here, we address common concerns, clarify misconceptions, and provide clear answers to enhance the reader's knowledge and experience.
The importance of this section lies in its ability to consolidate the relevant information into easily digestible responses. FAQs can bridge the knowledge gap for beginners and those transitioning from different methods of string formatting. By addressing various quirks, advantages, and best practices of f-strings, you empower readers to tackle real-world coding challenges efficiently.
By exploring common questions, we reveal the nuances that set f-strings apart from other assertion techniques. For instance, the performance advantages can change how developers approach project budgets—affecting both load times and memory usage. The FAQs will also explore compatibility issues, providing insights for users running older versions of Python.
This section is a go-to guide for troubleshooting and providing quick resolutions in everyday programming contexts. Diligently curated FAQs can help foster learning and improve the overall productivity of both newcomers and seasoned programmers. Importantly, it encourages active engagement with both the language and coding community.
Common Questions about f-strings
There are several common queries that arise when implementing f-strings in real-world applications. Let’s address some of the most pressing ones:
- What Python versions support f-strings?
F-strings are available starting from Python 3.6. Users of previous versions will need to adopt alternative methods like or the older formatting. - Can I use f-strings for complex expressions?
Yes, f-strings support embedding any expressions within curly braces. This flexibility is one of their unique advantages. - Are f-strings safe for user input?
It's crucial to sanitize any user input before including it in f-strings. This measure helps prevent potential security vulnerabilities such as injection attacks. - What about multi-line f-strings?
F-strings can be spread over multiple lines, however, you should manage multi-line strings carefully. Use parentheses or a backslash to ensure smooth concatenation and avoid syntax errors. - How do f-strings handle types?
F-strings automatically convert types to string format, allowing seamless presentation of integers, floats, and even objects.
These questions are just a glimpse into the considerations that come with using f-strings. Addressing such queries helps solidify the overall understanding and integration of this powerful feature in Python programming.