In-Depth Guide to React JS with Practical Examples
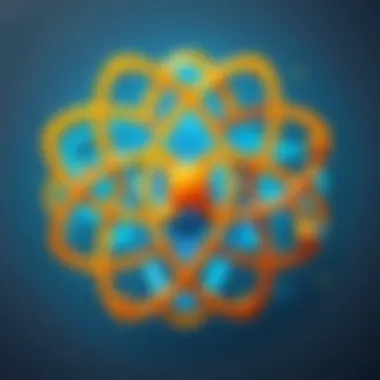
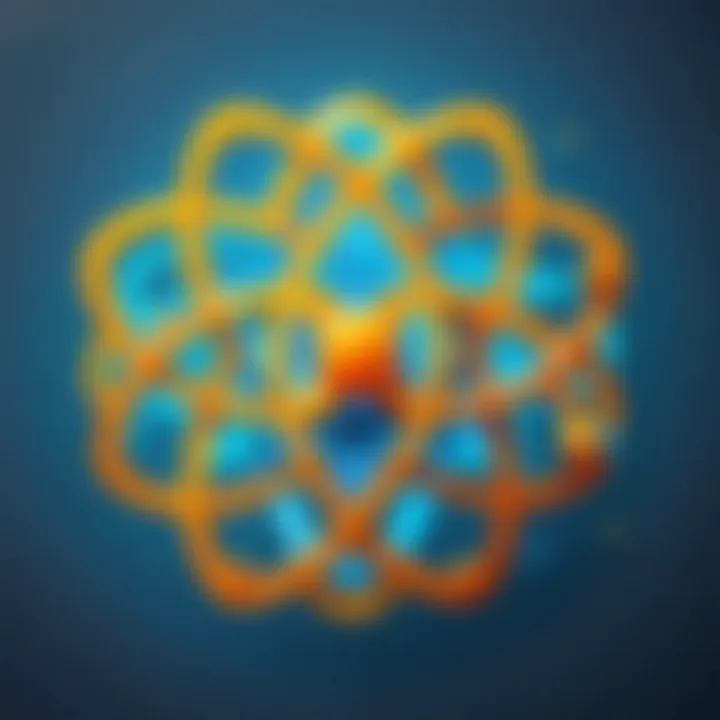
Overview of Topic
In todayās fast-paced digital landscape, the need for dynamic web applications is more pronounced than ever. Enter React JS, a JavaScript library that makes creating user interfaces a smoother ride than the open road. Developed by the team at Facebook, React JS has evolved into a foundational tool for front-end developers. This section will delve into what React JS is, its impact on the tech industry, and a brief look at its historical journey.
Medley of tools and frameworks clutter the development space, but React JS stands out for its component-based architecture. It allows developers to craft rich, interactive UIs with a fraction of the effort required by other libraries. Instead of rewriting code over and over, developers can utilize existing components, leading to not only time savings but also more manageable and scalable projects.
"React has changed the game for developers, making complex interfaces simpler to build and maintain."
The significance of React in the modern programming arena cannot be overstated. From facilitating agile development to enhancing the user experience, it has become a go-to choice for organizations worldwide. This library isnāt just a flash in the pan; itās grown into a mature ecosystem with an abundance of resources and community support.
Brief History and Evolution
Launched in 2013, React JS took its first steps as a way to handle the growing complexity of user interface development. Its introduction brought a breath of fresh air into the world of coding, encouraging the use of declarative programming. Following its release, React underwent several iterations, shaped by user feedback and the evolving needs of the industry. This adaptability has propelled its status among developers.
In subsequent years, continuous improvements, including features like hooks and the concurrent mode, have been rolled out. React JS has positioned itself not only as a library but also as a tool to address challenges that developers face in the quest for seamless user interfaces and efficient state management.
Scope and Significance in the Tech Industry
The tech world is rife with choices, and developers often find themselves at a crossroads when deciding which tools to use. React JS has carved out a significant niche, being embraced by companies ranging from startups to tech giants. Its capabilities allow for rapid iteration in development, which is vital in meeting todayās quick development cycles.
React isnāt just about building applications; itās also about enhancing the overall user experience. Users encounter more complex needs in their interactions with digital interfaces, and Reactās component-based approach caters to this need effectively.
In the following sections, we will explore the core components that make React tick, the best practices to utilize, and common pitfalls that can trip up even the most seasoned developers. Whether you're just starting your coding journey or youāre an experienced developer looking to polish your skills, this guide aims to sharpen your understanding and broaden your toolkit.
Intro to React JS
In the world of web development, React JS stands tall as a popular choice for many developers aiming to create engaging and dynamic user interfaces. The importance of understanding React cannot be overstated, particularly in a landscape where single-page applications have become the norm. Its component-based structure and the ease of managing state make React a favored framework, suitable for newcomers and seasoned developers alike. This article delves deep into React's fundamentals while providing practical code examples and techniques that surface its nuances and versatility.
What is React JS?
React JS is an open-source JavaScript library primarily utilized for building user interfaces, particularly single-page applications where swift interactions and updates without reloading the webpage are crucial. Developed by Facebook, it emphasizes the creation of reusable UI components that can be managed independently. This modularity is what allows developers to build complex applications more efficiently. By embracing React, developers leverage a tool that fits seamlessly into the React ecosystem, promoting code reuse and consistency across projects.
Key Features of React
React's framework is distinguished by several key features that enhance its usability and effectiveness as a front-end solution.
Declarative UI
At its core, React employs a declarative approach to designing user interfaces. This means developers can describe how the UI should look for any given state, and React takes care of updating the DOM to match that state automatically. This characteristic simplifies coding as it reduces the chances of bugs that often arise from manual DOM manipulations. Furthermore, it fosters maintainability, making it easier for developers to track changes and understand how different states affect the UI.
For instance, take the following example of a toggle button:
This example showcases the simplicity of the declarative style in React. The benefit here is clear; as the button is clicked, the state changes, and React takes the wheel to update the UI accordingly. This not only makes it easier to read and understand but also reduces the overhead related to maintaining the UI.
Component-Based Architecture
Another significant feature worth mentioning is the component-based architecture of React. This pattern encourages developers to build encapsulated components that manage their own state, then compose them to create complex user interfaces. The advantages are multifold. Components are reusable, leading to efficient development as they can be used across various parts of an application without duplicating code. This encapsulation also enhances testability since individual components can be tested separately.
To illustrate how this might work, consider the following structure for a simple application:
Each part can be treated as a component, which makes understanding the application's structure much easier, as components can be updated and modified independently. This accessibility lowers the barrier to entry for new developers coming into the project, helping them grasp the codebase faster.
Virtual DOM Concept
React's use of a virtual DOM is perhaps one of its most compelling characteristics. The virtual DOM acts as a lightweight copy of the actual DOM, allowing React to optimize updates by reducing the frequency and scope of direct manipulations to the real DOM. When a component's state changes, React computes the difference between the new state and the previous virtual DOM representation. Only the changed parts are updated in the real DOM, minimizing performance costs.
This approach not only speeds up the process of rendering components but also enhances the user experience by ensuring smooth interactions. Consider web applications that have high user activity; without the virtual DOM, lag or sluggish updates could hinder performance, making React's concept a critical factor in its widespread adoption by developers.
"React emphasizes the need for clear concepts, and a sound knowledge of its foundational elements is key to mastering it."
This article is comprehensive, aimed at students, learners, and IT professionals, ensuring that we dig into React with simplicity and depth.
Setting Up the Development Environment
Setting up the development environment is akin to laying the foundation of a house. Before diving into the nitty-gritty of building applications with React, one has to create an ecosystem where all the necessary tools and libraries can exist harmoniously. This section focuses on the fundamental steps to prepare your machine for React development, highlighting the essential components and techniques that will enhance your overall productivity.
A well-configured environment not only streamlines the coding process but also ensures that any emerging issues are caught early, saving time down the line. This is especially critical in React, where state and component interactions can become quite intricate as applications scale. Next up, weāll discuss specific steps on setting up Node.js, NPM, and the creation of a new React application.
Installing Node.js and NPM
Node.js serves as the backbone for running React applications. It allows JavaScript to operate outside the browser, which is a game changer for developers, enabling server-side programming as well. Along with Node.js comes NPM (Node Package Manager), which manages the various packages and libraries that can help in development.
To install Node.js, visit the official Node.js website (nodejs.org). Youāll usually find two download options: LTS (Long Term Support) and Current. Opting for the LTS version is often a prudent choice as it boasts more stability, perfect for those venturing into React for the first time. Runner-up to this scenario, one could install Node.js using a package manager relevant to their operating system, whether it's Homebrew for macOS or Chocolatey for Windows.
Once Node.js is installed, you can check if both Node and NPM are correctly set up by running the following commands in your terminal:
If those commands yield version numbers, congratulations! Youāre ready to move ahead.
Creating a New React App
With Node.js and NPM in place, it's time to create your first React application, and there's no better way to do this than with the Create React App tool.
Using Create React App
Create React App is an official script developed to streamline the setup process of a React application. Imagine it like a ready-made template that takes care of configuration for you, leaving more room for you to focus on coding. This aspect contributes significantly to its reputation in the React ecosystem.
One key characteristic of Create React App is its simplicity. You can generate a new React project in a matter of minutes with a single command:
As the project initializes, it sets up a directory with a standardized structure, complete with all the necessary dependencies. This feature is beneficial for newcomers who may be overwhelmed by the myriad of configurations often involved in setting up frameworks independently.
However, this approach does have some limitations. Since it abstracts the configuration, if you want customization or fine-tuning later on, you may find it cumbersome to eject the settings. Yet, for the vast majority of developersāespecially beginnersāthis starter pack remains a highly practical choice, ensuring a smooth entry into the world of React.
Folder Structure Overview
Understanding the folder structure is crucial when starting a new React project. The Create React App generates a specific set of folders and files that configure how your project will be structured. At first glance, it might seem a tad complex, yet with perspective, it can be seen as a logical organization.
Among the folders you'll encounter, the folder is where youāll spend most of your time. This is where all your components, styles, and application logic live. A notable characteristic of this setup is that all JavaScript files are housed within , while the folder contains static assets.
It's beneficial to become fluid with this structure, as navigating it will become second nature as you progress. However, the initial setup can feel a bit constraining if one wishes to stray from the pre-defined paths and conventions. Regardless, once mastered, it fortifies the development process, ensuring all components interact in a cohesive manner.
"A good structure lays the groundwork for success; itās like putting a good wheel on an old rusty car.ā
In summary, setting up your development environment is a crucial first step for anyone looking to dive into React. With Node.js and NPM installed, and the Create React App up and running, youāre setting the stage for a productive coding experience, one where logic and creativity can flourish side by side.
Understanding Basic Components
Grasping the concept of basic components in React is like understanding the building blocks of a well-structured house. Components are primarily the heart of React, where everything revolves. Each component is a small, reusable piece of UI that functions independently but can elegantly combine to create more complex interfaces. Itās all about modularity that simplifies code management and enhances readability.
Functional Components
Functional components are like the bread and butter of React. They are simple functions that accept props to return React elements. Compared to their class counterparts, they are often more concise and less verbose. This simplicity allows developers to focus on the core functionality without getting bogged down in the intricacies of state management. Additionally, with the advent of React Hooks, functional components can now handle state and side effects, merging the powers of functional programming with React.
One of the standout features of functional components is their ability to create stateless UI. These components are less likely to carry unnecessary baggage, making them lightweight and efficient. Thus, when creating dozens of similar components, functional ones can keep your codebase clean and easy to follow.
Class Components
Class components come into play when you need more features than what functional components offer. They have a more extensive setup, as they require the declaration of a class and can be equipped with lifecycle methods. With the use of state and the keyword, class components offer a more traditional object-oriented approach to building React UI.
Although class components have their merits, they can sometimes lead to cumbersome code. The additional responsibilities of maintaining state and lifecycle methods can become unwieldy, particularly in larger applications. Still, they remain an essential aspect of React, especially for those who prefer OOP practices.
Props and State
Props and state are crucial in making components dynamic and responsive to user interaction. Props, short for properties, are immutable and serve as the data flow mechanism into components. They are passed down from parent to child components, effectively allowing data to flow in one direction, thus maintaining a predictable data structure.
Passing Data with Props
Passing data with props is fundamentally how components communicate with each other in React. This flow not only facilitates data sharing but also contributes to the reusability of components. One effective characteristic of props is their ability to ensure that the data remains consistent and predictable, as they cannot be manipulated within the child component.
This approach highlights a significant advantage: by keeping the data immutable, you reduce side effects and bugs often associated with changing state within components. Furthermore, the reusability factor is crucial; it allows you to craft components that can be easily reused in varying contexts, enhancing both the efficiency and maintainability of the codebase.
Managing State in Components
Managing state in components deals with the internal data that the component can change over time. This internal state is mutable and serves as a local data store for the component. The common practice is to use the hook in functional components or in class components.
An essential characteristic of state management is its localized nature. Each component has its own state, making them self-sufficient. However, it gets trickier when sharing state across multiple componentsāthis is where state lifting techniques or context API come into play. Managing state effectively can lead to responsive applications that provide users with a seamless experience while minimizing prop drilling issues, which is when you pass props through many levels of components unnecessarily.
In summary, understanding the distinctions and functionalities of functional and class components, as well as the roles of props and state, is paramount in mastering React. Embracing these concepts not only enhances oneās coding skills but also fosters the development of robust, scalable applications.
"Components are the foundation of any React application, where clean design and effective data flow set the stage for success."
By delving into these foundational aspects, developers can create applications that are both robust and maintainable, ensuring a smooth journey in their React development adventure.
Lifecycle Methods
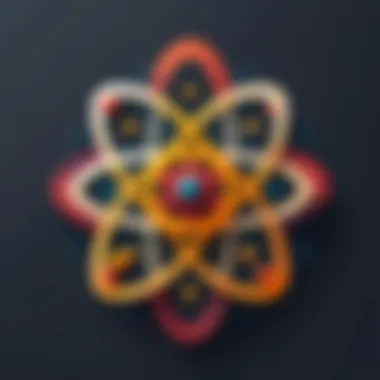
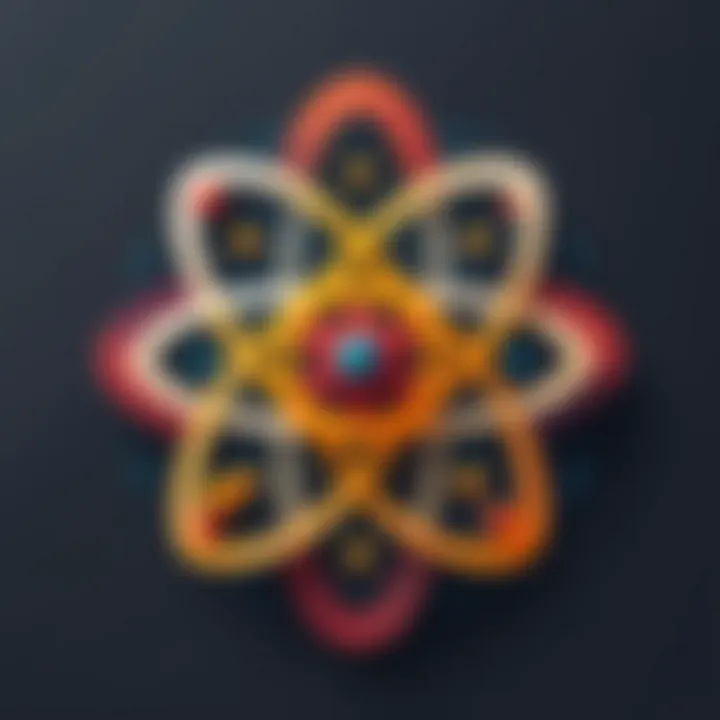
Lifecycle methods are fundamental in React as they govern how components behave throughout their existence. Understanding these methods gives developers the tools to manage performance, control side effects, and ultimately deliver a smooth user experience. React components undergo specific phasesāmounting, updating, and unmountingāand each phase has associated lifecycle methods that allow developers to tap into these changes.
The importance of these methods cannot be emphasized enough. They enable developers to respond to the various stages of a component's lifecycle. For instance, when an component is first added to the webpage, there might be data fetching or subscriptions that need to take place. When updates happen, adjustments in state management can be streamlined, and it's crucial to clean up resources when a component is removed from the DOM.
Preamble to Lifecycle Methods
Every React component has a lifecycle, from creation to destruction. Lifecycle methods offer hooks into these transitions, allowing developers to execute code at specific points. These hooks facilitate integrating external libraries, optimizing performance, and managing state efficiently. While it might seem a lot to digest, mastering these lifecycle methods can drastically improve how one interacts with their components and results.
Common Lifecycle Methods
componentDidMount
The method is called immediately after a component is mounted. This characteristic makes it ideal for fetching data from an API, initiating subscriptions, or integrating with third-party libraries. Developers often find it a go-to choice due to its reliability and timing; it occurs once, ensuring that any side-effects or asynchronous tasks begin only after the component renders.
A unique aspect of is its function as a one-time setup. This can be particularly powerful when you want to load data that a user needs right away without the need for manual refreshes. However, it's essential to be cautious about any memory leaks that can arise if subscriptions or listeners aren't cleaned up appropriately later in the component's lifecycle.
componentDidUpdate
As the name suggests, runs after every update. This method provides a great opportunity to compare previous props and state against new ones and perform logic for data fetching or updates accordingly. Its charm lies in being able to react to changing inputs, thereby allowing components to be dynamic in ways that align with users' needs.
The catch with is that it can lead to performance hitches if not handled properly. It's crucial to implement conditions that prevent unnecessary re-renders. Otherwise, you might find the component entering an infinite update loop.
componentWillUnmount
is executed right before a component is removed from the DOM. This method serves as the final chance for developers to clean up resourcesālike cancelling network requests, clearing timers, or unsubscribing from data streams. Its utility in maintaining performance and avoiding memory leaks makes it a must-know for serious developers.
The beauty of lies in its preventive nature. Neglecting to use it correctly could lead to resource wastage, which directly impacts application performance, especially in larger applications. The key takeaway is that managing your componentās lifecycle with these methods paves the way for responsive, efficient applications.
Lifecycle methods are not just hooks; they're gateways to better performance and user experience.
Event Handling in React
Event handling is a cornerstone in any interactive web application, and in the context of React, its importance cannot be overstated. The framework's ability to manage user interactions in a fluid and responsive manner gives developers the power to create dynamic interfaces. Understanding how to handle events competently leads to smoother applications that significantly enhance user experience.
In this section, we will explore the mechanics of event handling in Reactāfrom managing user inputs to crafting custom event handlers. This journey not only sheds light on the techniques available but also lays bare the considerations and best practices developers should adopt.
Handling User Inputs
Handling user inputs is one of the pivotal tasks every developer faces when building applications. In React, this is often achieved through controlled components. When you decide to control your inputs via state, you gain a significant amount of power. Every change a user makes translates directly into state changes in your application. This makes it easy to reflect the current value of the input in the UI and thus lets users see their actions unfold in real-time.
Hereās a simple example of managing an input field using Reactās state management:
This snippet captures input as the user types, showcasing how easy it is to synchronize component state with user input.
Custom Event Handlers
Custom event handlers offer flexibility and reusability in managing actions triggered by user interactions. Rather than relying solely on built-in event handlers, you can define your own methods, enabling more complex interactions tailored to your applicationās needs. The beauty of custom handlers lies in their ability to encapsulate logic that can be reused across various components without redundancy.
Defining and Using Custom Events
Defining and using custom events can greatly simplify your React application, especially for larger projects. By creating robust handlers that manage specific behaviors, you can keep your components clean and easy to maintain. A prime characteristic of this approach is the decoupling of event logic from component logic, leading to better separation of concerns within your codebase.
For example, you might define a custom event to handle form submissions:
This simple handler can be passed into forms to execute defined steps when a user submits data, making it a powerful choice to streamline your React components.
Event Pooling Concept
Understanding the event pooling concept in React is crucial for developers aiming to optimize performance. React employs a synthetic event system, which pools events to minimize memory usage. When an event occurs, React constructs a single event object and reuses it for all events fired in a given lifecycle stage.
This design choice not only leads to efficiency but also ensures that garbage collection isnāt a bottleneck for your application. One key consideration here is that the event properties are cleared after the event handler executes, meaning you cannot access the properties asynchronously after the handler runs. Therefore, it's vital to extract any data you need from the event object right away:
In summary, while event pooling offers substantial benefits, developers should be aware of its nuances to maintain functional integrity.
"Mastering event handling in React not only streamlines application performance but enhances the overall user experience considerably."
Conditional Rendering Techniques
In React, conditional rendering is a fundamental concept which allows your application to display certain elements based on specific conditions. This practice enhances the user experience by showing or hiding components according to the application state or user actions. It serves not just for aesthetic purposes, but it also plays a critical role in workflows, allowing developers to handle various scenarios dynamically. You can think of it as the gatekeeper, determining what a user sees depending on what theyāve done.
When it comes to building interfaces that respond to user input or application states, understanding how to effectively use conditional rendering techniques can offer significant advantages. Whether itās showing a loader when data is fetching or presenting a different message based on user permissions, it can make your applications more intuitive and user-friendly.
Using if-else Statements
The most straightforward way to implement conditional rendering in React is through if-else statements. This method allows developers to write clear and understandable conditions that define what should be displayed. For instance, suppose you want to display a message for logged-in users and another for those who are logged out.
This approach is not just simple; it also enables developers to scale their conditions easily as the application grows. However, keep in mind that excessive use of nested if-else statements might lead to harder-to-read code. Therefore, strive for balance and clarity.
Ternary Operator
Another popular method for conditional rendering is the ternary operator. This operator enables a more concise way to handle conditional logic directly within your JSX. It can save some lines of code compared to traditional if-else statements and can be especially handy for inline conditionals.
Hereās how the previous example might look using a ternary operator:
While the ternary operator is great for short conditions, itās essential to avoid putting too many conditions within the same expression. Complexity can make it tough to maintain or understand, leading to potential confusion later on.
Logical AND Operator
The logical AND operator offers yet another method of conditional rendering, particularly useful when you want to display something only if a condition is true, without a fallback for false. This technique can be particularly beneficial when rendering components or elements that should appear based on specific user actions.
For example, if you want to show a favorite button only when a user is logged in, you can do it like this:
In this case, if the prop is true, the button renders. If itās false, nothing is shown. This method provides a clean way to conditionally display components without needing to create an explicit else case. However, one should be mindful of readability, as multiple logical conditions can lead to complex structures.
Conditional rendering techniques are essential for creating dynamic user interfaces that enhance user experiences.
In summary, mastering conditional rendering techniques is crucial for any React developer. Each of these methods has its use cases and benefits, and knowing when to apply each can save a great deal of time while improving code clarity. As you build out your applications, keep these strategies in mind to handle the various states your app might encounter.
Working with Forms
Forms are the backbone of user interaction in many web applications. In React, managing forms can either be a piece of cake or a complicated task, depending on how you approach it. Because forms often collect essential data from users ā think of signing up for a newsletter, entering payment info, or just posting a comment ā they carry significant weight in any application.
Controlled vs Uncontrolled Components
When it comes to forms in React, you essentially have two options: controlled components and uncontrolled components. Controlled components are the ones where the form data is handled by the componentās state. You can think of it as keeping a tight leash on whatās happening. Every input field's value is synced with the componentās state, which offers full control over the form data. This tight integration can be beneficial in terms of real-time validation and making sure your data is in a predictable format.
Contrasting this, uncontrolled components allow the form elements to manage their own state. What's interesting here is that the data isn't stored in the state of the component, but rather in the DOM itself. If youāre in a scenario where you need quick and straightforward form handling and don't require the overhead of managing everything through state, uncontrolled components can save you some hassle.
Itās all a question of what fits your use-case best. In applications where precise data fetching, validation, and overall form control are crucial, controlled components are the way to go. On the other hand, if your forms are as simple as pie, hitting the ground running with uncontrolled ones could suit you just fine.
Validating Form Data
Validating form data is an absolute must. You want to ensure the information being entered by users is legitimate and useful. Not only does proper validation create a smoother experience for the user, but it also protects your application from potential issues like incorrect data submissions.
Basic Validation Techniques
Basic validation techniques involve implementing checks at the input level. This could include checks for empty fields, ensuring email formats are right, or even basic password strength validations. One key characteristic of this approach is simplicity; it doesn't require external libraries or complex configurations under the hood. This makes it appealing for smaller projects or when a straightforward solution is needed.
However, itās crucial to note that while this technique is popular for its ease of use, it may lack the depth required for larger applications. It might not be enough for dealing with complex data types or nuanced validations.
Using Validation Libraries
On the contrary, using validation libraries can take things to another level. Many developers turn to libraries like Formik or Yup for their extensive capabilities. These libraries provide elegant solutions for handling forms in React along with powerful validation features out of the box. They often enable you to set complex validation rules easily and can handle scenarios that basic validation techniques just can't touch on.
A notable benefit of using validation libraries is the community support. You typically get regular updates, fixes, and community-contributed examples, which means you arenāt reinventing the wheel. Yet, itās wise to be aware of potential overhead; these additional libraries could bloat your application in terms of size and complexity. Consider how critical validation is for your application before going all-in with third-party libraries.
Bottom line: Choose controlled components for tight data management while validating input straightforwardly or using libraries for more complex forms. The balance between control and simplicity could be your workaround in many cases.
State Management Approaches
State management is a critical aspect of React applications. It deals with how data is stored and manipulated within an app, addressing how components access and maintain this data. With the rise of complex applications, having a solid grasp of state management becomes essential. Effective state management aids in keeping your application organized, predictable, and as bug-free as possible. By managing state properly, components can communicate changes seamlessly, providing a smoother user experience, and ultimately leading to more maintainable code.
Lifting State Up
Lifting state up is a technique that many React developers use to share data between components. In scenarios where two or more components need to access the same state, the best route is to lift the state to their closest common ancestor.
For instance, imagine we have a parent component that houses both a and component. If both components rely on a shared piece of data, like user input, keeping that state in the parent and passing it down as props is a clear choice. This not only makes the state accessible to both children, but it also centralizes control over that data.
The benefit of this approach is that it simplifies state management by ensuring that the source of truth for that piece of state resides in one place. However, one should keep an eye on complexity; too much lifting might lead to cumbersome props drilling, making the code less readable.
Prelude to Context API
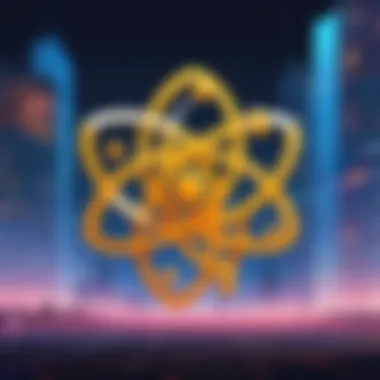
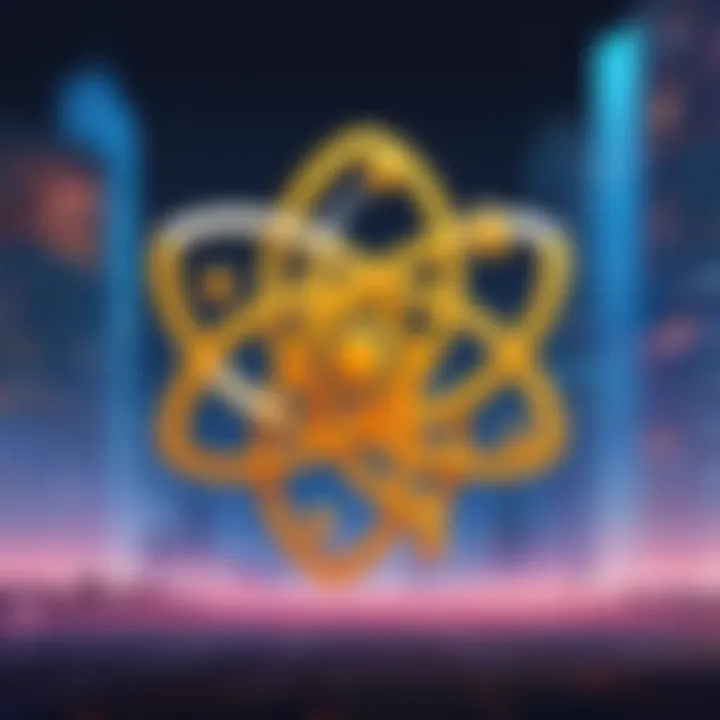
The Context API comes into play when lifting state feels tedious or overdone. It allows developers to share values across the component tree, without the hassle of passing props through each level.
Using the Context API is straightforward: you create a context, provide it at a higher level, and then consume it in child components. For example, if you were building a theme toggler for your app, the selected theme could be set in a Context Provider. Any component within the tree can access the selected theme directly, avoiding multiple levels of propping.
While powerful, one must use the Context API judiciously. Overusing it can lead to components re-rendering unnecessarily, which may affect performance.
Exploring Redux
Redux is an established library that adds a specific approach to handling state globally in an application. It revolves around a single store, holding all the state for your app in one location. This can be a game changer, especially for large-scale applications where numerous components may need to access or modify shared data.
Setting Up Redux
Setting up Redux involves creating actions, reducers, and the store itself. Each of these components plays a unique roleāactions define whatās happening, reducers dictate how the state changes in response to those actions, and the store holds the current state.
A remarkable characteristic of Redux is its predictable state container. Knowing what state exists and how it transitions makes debugging much easier, which is invaluable in complex apps. While Redux can seem daunting, its benefits shine in larger projects where state needs to be managed across multiple components. However, it does introduce some boilerplate code that may not always feel necessary.
Understanding Actions and Reducers
Actions and reducers are integral to Redux's operation. An action is essentially a plain JavaScript object that has a type and an optional payload, indicating what has happened. Reducers are the functions that handle these actionsādeciding how the state should change based on the action dispatched.
It's crucial to appreciate that actions and reducers are the backbone of Redux's unidirectional data flow. This further enforces predictability in how your application's state interacts with UI components. While Redux is beneficial for handling complex state transitions, some developers may find its strict structure limiting or a bit heavy-handed for smaller applications.
Understanding these concepts can significantly elevate the way developers manage state in React applications, leading to cleaner, more maintainable codebases.
Routing in React Applications
Routing is a crucial aspect in modern web applications, particularly in single-page applications (SPAs) like those built with React. By implementing routing, developers can create smooth, dynamic experiences for users as they navigate through different views without the need for full-page reloads. With a plethora of benefits, understanding routing enhances the overall architecture and user experience of React applications.
Using React Router
React Router is the go-to library for handling routing in React apps. This tool simplifies the navigation process, enabling developers to manage routes declaratively. In essence, React Router allows you to define routes within your components, making it easier to control what is displayed based on the URL path.
To get started, you need to install React Router:
Once you have it installed, you can set up a simple routing structure like so:
In this example, the component wraps around the app, encapsulating all route definitions. The component ensures that only one route is rendered at a time, which is particularly useful when dealing with nested routes. Each specifies a and the corresponding component to render. This setup allows for a declarative way to manage different views, keeping your code organized and clean.
Implementing Dynamic Routing
Dynamic routing significantly enhances flexibility by allowing routes to change according to user interaction or data changes dynamically. This is especially handy in scenarios where content is fetched from APIs, and the website needs to display varying content based on user selections.
A typical example would be an application displaying user profiles. The URL may need to reflect each userās unique identifier. Hereās how you can implement this:
In the above code, the is a route parameter that the component will use to fetch and display the respective user's data. Hereās a concise look at how you might define the component:
In this setup, whenever someone navigates to , the component will render, displaying content relevant to the user with ID 123. This makes your application more dynamic, responsive, and user-focused.
As you dive deeper into React, mastering routing will become invaluable as it directly affects user interactions and application behavior.
"A smooth user experience is often the heart of a successful web application. Implementing effective routing is a step in that direction."
In summary, effective routing in React applications lays the foundation for a cohesive and interactive user experience. Not only does it streamline navigation, but it also prepares your application for varying content and user demands.
Performance Optimization Techniques
Performance optimization in React applications is a critical aspect to ensure smooth user experiences and efficient resource management. When applications scale, having an understanding of how to boost performance doesnāt just serve the viewers well; it enhances the overall architecture of the application. Slow-loading pages can lead users to abandon your app faster than you can say "JavaScript". Hence, it is vital to integrate performance optimization techniques into your development process. Here are some of the most significant techniques to keep your React app running smoothly.
Memoization with React.memo
Memoization is a technique where the results of expensive function calls are cached and reused when the same inputs occur again. In the context of React, is used to optimize functional components by memoizing their output. This means that if the component receives the same props as the previous render, React skips rendering the component and reuses the last result instead.
This can significantly reduce rendering times especially in larger applications where many components might rerender unnecessarily.
Consider the following example:
Now, if the prop does not change, won't re-render. This way, you can improve performance in certain scenarios. However, bear in mind that unnecessary memoization can lead to more complexity than performance gains, so itās essential to be prudent and target components that warrant performance improvement.
Lazy Loading Components
Lazy loading is another powerful strategy to enhance performance. This involves loading components only when they are needed, thereby reducing the initial load time of the application. By splitting your code into chunks and loading parts of your application on demand, you can significantly cut down on resource consumption.
React provides a built-in way to implement lazy loading with the function. Hereās how you could use it:
In this example, is only loaded when itās needed, wrapped in a component that shows a fallback UI while waiting. This not only improves the performance but also enhances the user experience with quicker initial load times.
Using React Profiler
The React Profiler is an invaluable tool that helps developers track and understand the performance of their applications. By measuring how often a component renders and how long it takes, profiling can expose inefficiencies and render bottlenecks.
To use the Profiler, you can wrap it around the part of your app you want to measure, then utilize its callback to log performance data:
You can analyze the logged data to gauge which components take the most time to render. This information helps in identifying which components to optimize further or whether they can be simplified, leading to overall better performance in your React application.
Key Takeaway: Regular profiling and optimization can prevent performance issues from piling up, ensuring a responsive experience.
In a nutshell, employing these performance optimization techniques not only makes your React applications snappier but also enhances user satisfaction. Taking the time to understand and implement these strategies can pay off immensely, especially as your application matures and scales.
Building and Deploying React Applications
Building and deploying React applications plays a pivotal role in ensuring that your hard work translates into interactive and user-friendly web experiences. The process is not just about getting a product onto the internet; it's about making it accessible, efficient, and functional for users. When developers create applications, they need to focus on optimizing their code, simplifying deployment processes, and choosing the right hosting options.
Key Elements in Building and Deploying:
- Performance Optimization: It is essential to reduce application load times and boost performance. Your users will likely leave if a page takes too long to load.
- Security Considerations: Protecting your users' data and providing a secure experience fosters trust.
- Scalability: Ensuring that your app can grow as user demands increase is vital.
- Continuous Deployment: The ability to release updates efficiently allows for better user engagement and satisfaction.
- Monitoring and Maintenance: Keeping an eye on the applicationās performance and addressing issues is a crucial ongoing task.
Creating a Production Build
Creating a production build is the first step towards making your React app ready for deployment. A production build optimizes your code by making it minified and more efficient. When you run the command , it packages your application files for optimal loading in a web environment.
- Code Splitting: Splitting the code into manageable chunks ensures that only the necessary code is loaded.
- Asset Optimization: Images, fonts, and other media are also optimized during this process to reduce load times and enhance performance.
- Environment Variables: In this step, you can also define environment variables that are specific to the production environment.
Overall, creating a production build is like laying the foundation for a strong house, ensuring everything is in order before welcoming visitors.
Hosting Options
Deciding where to host your React application is almost as important as building it. You want to choose a platform that is both reliable and fast. Let's look at two popular options:
Using Netlify
Netlify is renowned for its simple setup and integrated features, catering particularly well to front-end developers. With its ability to automatically deploy apps when you push code changes to your repository, it streamlines the deployment process significantly.
One standout characteristic of Netlify is its integrated Continuous Deployment, allowing for seamless integration with GitHub, GitLab, or Bitbucket. This means every commit triggers a new deployment, ensuring your users always have the latest version.
Benefits of Using Netlify:
- Instant Rollbacks: If an update causes issues, you can revert to a previous version with a single click.
- Serverless Functions: You can create backend operations platformlessly, which can be a game changer for smaller projects.
However, itās crucial to note that while Netlify is great for front-end applications, its serverless functions might not support heavy computational tasks efficiently.
Using Vercel
Vercel has gained attention for its simplicity and speed. Focused on front-end frameworks, it specializes in optimizing the deployment process for React apps. One key aspect is Vercel's ability to serve static assets with remarkable speed and reliability, making it a popular choice among developers who prioritize performance.
A unique feature of Vercel is its Preview Deployments. This allows you to see your changes in a production-like environment before finalizing them, ensuring everything works as intended.
Advantages of Using Vercel:
- Improved Performance: Thanks to its global Content Delivery Network (CDN), applications hosted on Vercel load quickly all around the world.
- Automatic SSL Setup: Security features are handled automatically, making sure that your application is always served over HTTPS.
On the downside, Vercel might come with limitations on the number of serverless functions in their free tier, which may not accommodate all project needs.
Building and deploying your React application is not merely about making it available. It's about ensuring that it performs well, remains secure, and scales effectively. The groundwork you lay in this phase will profoundly affect the final user experience.
Exploring Advanced Concepts
Delving into advanced concepts in React JS is like wandering into a treasure trove filled with tools that extend the basic functionalities of components and allow developers to craft cleaner, more reusable code. These topics build upon the foundational principles of React and cater to both the novice and the seasoned developer.
Understanding advanced concepts such as Higher-Order Components and Custom Hooks can markedly elevate a developer's skill set. These concepts encourage a mindset focused on composition rather than inheritance, fostering code that is modular and easy to maintain. The ability to create generic solutions that can be reused across applications becomes increasingly valuable in larger codebases, where avoiding duplication not only enhances readability but also minimizes the potential for bugs.
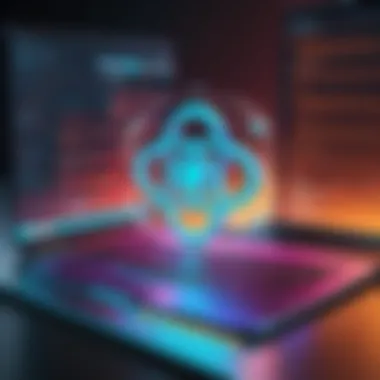
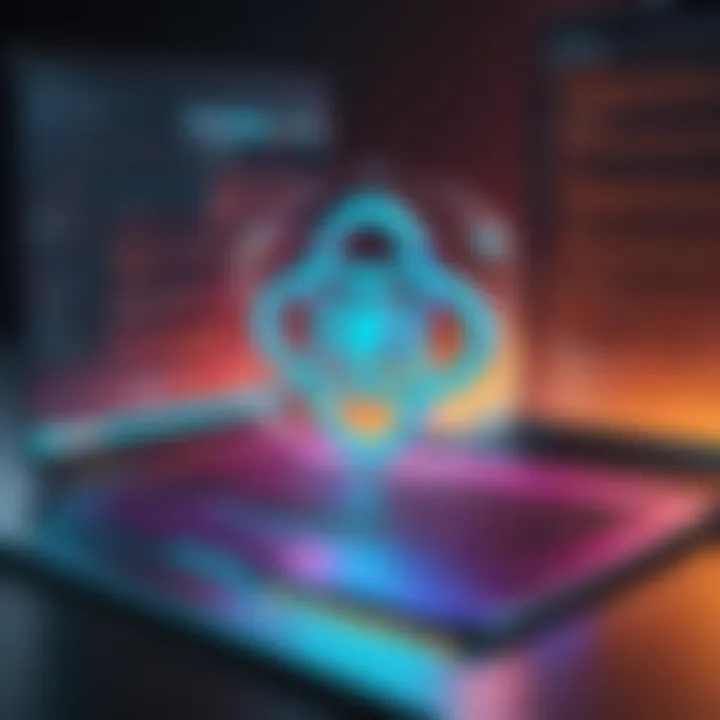
Higher-Order Components
Higher-Order Components (HOCs) are a powerful pattern in React that allows for the enhancement of components without modifying their structures directly. Simply put, an HOC is a function that takes a component as its argument and returns a new component. This technique facilitates code reuse, particularly in scenarios where multiple components share similar logic.
One of the main benefits of HOCs is their capability to encapsulate cross-cutting concerns, such as data fetching or handling authentication. By moving these functionalities out of the specific components, developers can keep their code cleaner and more focused on what matters most. However, the challenge lies in ensuring that the HOC does not overly complicate the component tree, which can make debugging a chore.
Key takeaway: Higher-Order Components enable code reuse and enhance component capabilities without altering their direct implementation.
Custom Hooks
Custom Hooks provide a way to extract reusable logic from components and, like HOCs, are pivotal for maintaining a clean codebase. They are basically JavaScript functions whose names start with "use" which can call other hooks. When developers want to share stateful logic across multiple components, Custom Hooks come into play.
Creating Your Own Hooks
Creating your own hooks allows a developer to encapsulate logic related to state or effects that are not tied to a specific component. For example, if you find that multiple components in your app need to perform similar data fetching, you can create a Custom Hook that handles the data fetching logic. This method is not just cleaner; it's also significantly more manageable as the app scales. One key feature of these Custom Hooks is their ability to simplify complex component logic into reusable functions.
Yet, while beneficial, one must be cautious about over-engineering. Sometimes a simple component state is sufficient, and introducing a Custom Hook may add unnecessary complexity to the code. In cases where the logic is fine-grained and component-specific, the use of Custom Hooks should be evaluated.
Using Existing Hooks
Using existing hooks taps into the rich ecosystem already provided by Hook APIs that React offers. Hooks like , , and come pre-configured to manage state and effects in a way that ensures your application remains responsive to user inputs. They provide the benefit of direct use cases, making it straightforward for developers to implement common functionalities without reinventing the wheel.
For instance, leveraging the existing hook can easily manage local component state without the hassle of setup. Still, developers must understand the intricacies of each hook, as improper usage can lead to performance issues or bugs that are hard to track down. Thus, when adding these existing hooks into components, careful attention should be paid to their dependency arrays and lifecycle implications.
Integrating External APIs
Integrating external APIs is a cornerstone of modern web application development, particularly within the React ecosystem. This topic deserves close attention because leveraging APIs can significantly expand the capabilities of your application. When you're building complex applications, relying solely on internal data sources can be limiting. Integrating APIs allows you to tap into a wealth of external data and services, giving your application richer functionality and more diverse user experiences.
Importance of Integrating External APIs
There are numerous benefits to incorporating external APIs. For one, it saves development time and resources. Instead of building every feature from scratch, developers can use existing services like payment gateways, social media authentication, or data retrieval services. Moreover, APIs are designed to be flexible; they allow for easy updates and seamless new feature additions.
However, while the advantages are clear, there are also considerations to keep in mind. Itās vital to choose stable and well-documented APIs. A poorly designed API can lead to integration nightmares, making updates and maintenance a hassle. Similarly, keep an eye on rate limits and data privacy policies of the APIs you work with. It's also prudent to ensure that you handle errors gracefully so that your application can provide a good user experience even when things go wrong.
"APIs are like the internetās service elevators; they enable complex systems to communicate seamlessly."
In the following sections, we will dive into the nitty-gritty of fetching data through two popular methods in React: the Fetch API and Axios, examining their respective strengths and weaknesses.
Fetching Data with Fetch API
The Fetch API is a built-in JavaScript function that allows you to make network requests. Unlike older techniques like , it operates on Promises, making it a naturally more elegant solution that integrates well with modern JavaScript practices. The syntax for fetching data is straightforward, allowing developers to quickly initiate requests.
Hereās a quick example to illustrate how the Fetch API works:
Above is a simple request to an external API. The function returns a Promise that resolves to the Response object representing the response to the request. It's important to handle both successful and failed responses correctly, as they contribute to the robustness of your application. Fetch API is a great choice for straightforward use cases, but it may not be suited for complex handling.
Axios for HTTP Requests
Axios is a popular library for making HTTP requests, offering a more feature-rich set of functionalities compared to the Fetch API. With Axios, you get interceptors, automatic JSON data transformation, and an array of other versatile tools. This makes it a favorite among many developers working with React.
Hereās how you can leverage Axios for similar functionality:
With just a few lines, Axios allows for concise, readable code that can also be extended for more complex scenarios. One of the standout features is its ability to create an instance of Axios with custom configuration, making it easier to scale your applications and manage settings for various APIs.
In summary, integrating external APIs into your React applications not only enhances functionality but also opens a realm of possibilities for enriching user experiences. Both Fetch API and Axios have their unique merits, and the choice largely depends on the project needs and preferences of the developers.
Testing in React
In the realm of software development, testing holds a place of great significance. Testing in React is no exception. As applications become increasingly complex, ensuring that each component behaves as intended is crucial. Testing serves as a safety net, catching bugs before they reach production. Relying on visual inspections or manual testing alone can lead to missed issues. Thus, an effective testing strategy allows developers to deliver high-quality code, leading to a better user experience and minimized maintenance costs.
There are several reasons why testing should be considered integral to React development:
- Confidence in Code Changes: When adjustments are needed, having tests in place provides assurance that existing functionality will remain intact.
- Identifying Edge Cases: Rigorous testing uncovers scenarios that may not be immediately obvious during development.
- Collaboration Across Teams: With comprehensive tests, different teams can confidently work on various parts of the app without fear of inadvertently breaking each other's code.
As we delve deeper into specific techniques, two popular tools often come into the spotlight: Jest and React Testing Library. These tools aid in creating a robust test suite that ensures components remain reliable over time.
Unit Testing with Jest
Jest is a widely used testing framework for JavaScript, particularly in the React ecosystem. Developed by Facebook, Jest offers a user-friendly interface and comes bundled with powerful features that simplify the process of writing tests.
The beauty of Jest lies in its ability to perform unit tests, allowing developers to isolate and evaluate specific functionalities of components without the influence of external dependencies. This isolation guarantees that any potential issues are rooted in the component itself, rather than external factors.
To illustrate the power of Jest, consider the following steps to set up a unit test for a simple React component:
- Install Jest: Typically, if you create a React app using Create React App, Jest comes pre-configured. If not, it can be added with npm:
- Create a Test File: Name it with a extension in the same directory as your component. For instance, if your component is , create .
- Write a Test Case: Within this file, you can define your test cases. Hereās an example:
- Run Tests: Execute the tests by running the command:
By following these steps, you ensure that not only does your component render properly, but it also displays the intended content. The simplicity of writing tests using Jest empowers developers and elevates the quality of the overall application.
Using React Testing Library
While Jest is crucial for unit testing, React Testing Library complements it by providing a user-centric approach to testing. Instead of focusing on the implementation details, this library encourages testing how users interact with your app.
When you use React Testing Library, you focus more on the behavior of your components rather than their internal mechanics. This method gives you a clear picture of how well your components simulate real-world usage.
Key advantages include:
- Realistic Testing: Tests written with this library simulate actual user interactions, ensuring components behave correctly when interacted with.
- Less Fragility: Since the tests focus on outputs rather than implementation, changes to the componentās structure often donāt require a complete rewrite of the tests.
Here's a simple example of how to utilize the React Testing Library alongside Jest:
- Install the Library: If you're using Create React App, itās already included. Otherwise, you can get it via npm:
- Write a Test Case: Like before, you can use the same test file format. Hereās how a test might look:
- Execute Your Tests: Much like with Jest, you can run this test using forward command:
By blending Jest with React Testing Library, you create a formidable duo that enhances your testing approach, ensuring your React components are not only functional but are also user-friendly.
Keep in mind, thorough testing is not just about finding errors; it's about promotion peace of mind during development, fostering confidence in the software you deliver.
Common Challenges and Solutions
In the realm of React development, challenges are as inevitable as they are instructive. Understanding and addressing these difficulties is crucial for anyone looking to harness the true potential of React. This section delves into some common hurdles developers encounter and suggests effective strategies to overcome them. Not only does this enhance your coding acumen, but it also prepares you for real-world scenarios in software development where adaptability is key.
Debugging React Applications
React applications, while powerful, can sometimes resemble a tangled ball of yarn. The user interface might flicker, or an event might not trigger as intended. Identifying the source of errors can feel like searching for a needle in a haystack.
Tools for Debugging
First off, one pivotal aspect of debugging involves using specialized tools designed for the task at hand. For instance, the React Developer Tools extension for Chrome and Firefox is highly regarded among developers.
- Key Characteristic: This tool allows you to inspect the React component hierarchy in the virtual DOM, making it easier to track changes in state and props.
- Unique Feature: A unique capability of these tools is the ability to view component state in real time. This is invaluable for pinpointing issues during runtime.
- Advantages: It simplifies processes such as performance analysis and helps catch errors that may be overlooked in the code. However, one downside is that these tools can sometimes be overwhelming for new users due to the plethora of options available.
Common Pitfalls
When developing with React, developers often stumble into a few habitual traps. These pitfalls can lead to significant headaches if not recognized early on.
- Key Characteristic: One of the most reported issues is improper state management, where state changes do not reflect in the UI seamlessly.
- Unique Feature: This issue often stems from misunderstanding how data flows within React. As such, failing to lift state when needed can create confusion in component hierarchies.
- Advantages/Disadvantages: While using local state in components might simplify some tasks, it can also lead to redundancy. A holistic understanding of the component lifecycle and proper state management is crucial for avoiding these common pitfalls.
Handling Errors Gracefully
An essential skill in React development is the ability to handle errors gracefully. A user encountering a broken interface could quickly lose confidence in the application. Proper error handling ensures that users are informed about issues without detracting from their experience.
One effective method of handling errors is through the use of Error Boundaries. These special components catch JavaScript errors in their child component tree.
- An Error Boundary captures errors and renders a fallback UI, so the entire application doesnāt crash.
- Implementing this feature helps isolate problems and maintains a smoother user experience. Developers should leverage constructs like to log error details for later analysis and debugging.
Utilizing these strategies can significantly ease the development journey with React. By familiarizing oneself with debugging tools, recognizing common pitfalls, and adeptly managing errors, developers can save considerable time and frustration.
"In software, the most difficult challenges are those that test both your ingenuity and perseverance. Navigating through obstacles sharpens your skills and fosters growth."
As we wrap up this section, keep in mind that every challenge tackled adds to your prowess as a developer. Embrace these experiences, for they will become the building blocks of your expertise in React.
Concluding Thoughts
When delving into React JS, it's vital to step back and understand the broader implications and significance of the framework as a whole. Concluding Thoughts serves as a cornerstone to synthesize previous discussions, highlighting the journey through the complex landscape of React.
First, one must recognize how React JS has transformed the way developers approach UI development. It combines a component-based structure with a declarative mindset. This approach not only fosters reusability but also enhances maintainability. What developers find appealing is that they can compartmentalize their code into small, manageable piecesācomponentsāthat can be reused throughout their projects. This fundamental characteristic streamlines collaboration across teams, enabling them to write cleaner code and significantly reducing bugs.
Another crucial element to consider is the future trajectory of React. With its vibrant community and ongoing updates, React is not just a temporary solution; itās evolving. New features, improved performance, and enhanced tooling continue to make React a preferred choice among professionals. This is central to understanding why learning React is not just beneficial, but necessary in the fast-paced world of web development.
To stay relevant and competitive, developers should continuously engage with the React ecosystem, adapting to new releases and community practices.
The capability to integrate easily with various libraries and frameworks amplifies Reactās utility. Tools like Redux for state management and React Router for navigation are just top of the iceberg. They empower developers to build sophisticated applications while keeping their code efficient.
In summary, Concluding Thoughts isnāt merely about summation; itās about reflection and foresight. As the landscape of web technologies shifts, React JS stands firm, thanks to its robust architecture and adaptability. Emphasizing continuous learning and engagement with resources ensures developers remain equipped to navigate these changes effectively.