In-Depth Exploration of the Swift Programming Language
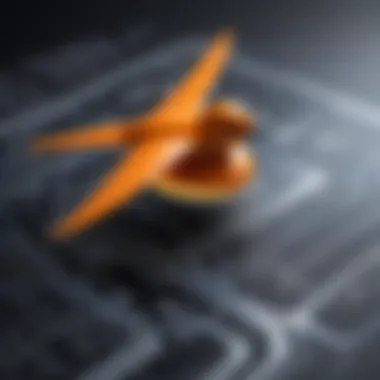
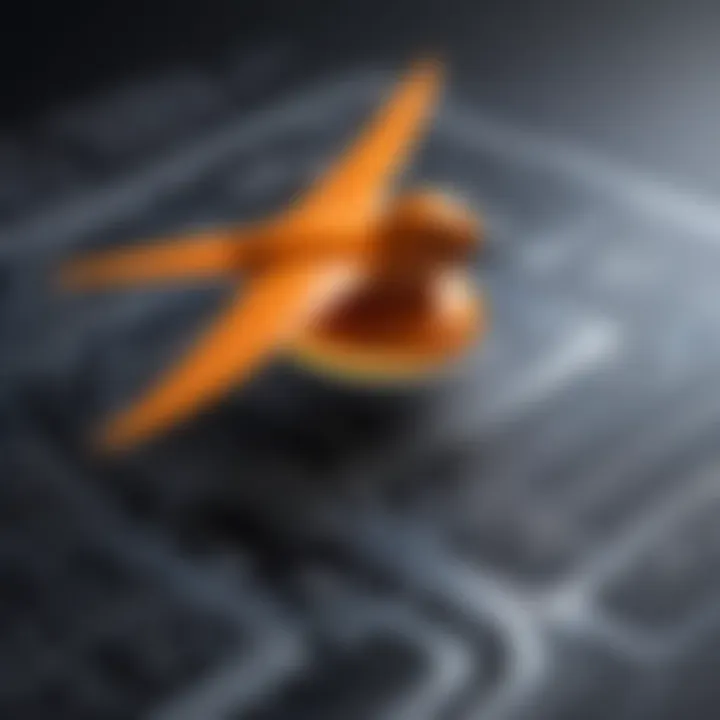
Overview of Topic
The Swift programming language has emerged as a vital player in the software development landscape, establishing itself as a favorite among programmers. This section dives into what Swift is all about, exploring not just the technical aspects but also its significance in today’s tech industry.
Swift first made its debut in 2014, created by Apple Inc. to replace Objective-C as the primary language for iOS and macOS development. Since then, it has evolved considerably, adopting a clean syntax and incorporating modern programming paradigms. The evolution of Swift reflects broader trends in programming, such as a shift towards safety, performance, and developer productivity. The growing popularity of Swift can be attributed to its ability to provide developers with powerful tools while maintaining readability and ease of use.
In the realm of mobile and desktop applications, as well as in server-side development, Swift has solidified its position. This significance cannot be overstated; the demand for application developers proficient in Swift continues to climb, opening up new avenues for career advancement in an era where tech continues to dominate daily life. By analyzing Swift's features and applications, we can better appreciate its impact in an ever-changing industry.
Fundamentals Explained
To truly grasp Swift, one needs to delve into its core principles. At its heart, Swift emphasizes a mix of simplicity and performance. It supports type safety, making it robust yet flexible enough to cater to both novice and experienced developers.
Here are some crucial terminology and concepts:
- Variables and Constants: Swift uses for constants and for variables. This distinction aids in understanding immutability.
- Data types: Swift has a range of built-in data types, including Int, String, Bool, and Float, which form the foundation for more complex types.
- Control Flow: Familiar control structures like statements, cases, and loops are present, allowing for powerful logic implementations.
Understanding these fundamentals sets the stage for any programming journey in Swift. It builds a base from which more advanced concepts can be explored.
Practical Applications and Examples
Swift's real-world applications are vast. Many of the apps we use daily on our iPhones and iPads are built using Swift. For instance, the popular mobile game "Angry Birds 2" utilized Swift to enable a smooth user experience and efficient processing.
Here’s an example that demonstrates the creation of a simple function in Swift:
Such examples highlight the language's capacity to handle fundamental programming tasks effectively.
Advanced Topics and Latest Trends
As Swift continues to evolve, developers are increasingly experimenting with its advanced features like protocol-oriented programming and concurrency. The introduction of SwiftUI marks a significant shift in user interface design, allowing developers to create visually stunning apps with far less code compared to traditional methods. Trends indicate a rising interest in server-side Swift, pushing frameworks like Vapor and Kitura into the spotlight.
Keeping abreast of these advancements is crucial for developers looking to stay ahead of the curve. It’s not just about using Swift; it’s more about leveraging its potent features to create innovative solutions.
Tips and Resources for Further Learning
To enhance your understanding and skills in Swift, here are some recommended resources:
- Books: "The Swift Programming Language" by Apple Books is a must-read for anyone looking to get a thorough overview.
- Online Courses: Websites like Coursera and Udemy offer comprehensive courses covering beginner to advanced Swift programming.
- Developer Communities: Engaging with communities on platforms such as Reddit or Stack Overflow can provide support, tips, and networking opportunities.
As you embark on your Swift programming journey, these resources can significantly bolster your learning experience, leading to a deeper understanding and mastery of this modern language.
Prelims to Swift
Swift is not just another programming language; it's a robust tool that has changed the landscape of coding, especially for iOS and macOS developers. Understanding Swift becomes fundamental for those looking to step into modern software development. It’s built for speed and runs efficiently, which immediately sets it apart from its predecessors. Moreover, Swift encourages safe programming practices, reducing the likelihood of runtime errors that can plague less diligently crafted languages.
Historical Context
The concept of Swift emerged in 2010; however, it was officially introduced to the world in 2014 by Apple during their annual Worldwide Developers Conference (WWDC). Swift was forged out of a need for a more modern, expressive language to replace Objective-C, which had started to show its age. The aim was to create a language that not only performed well but was also approachable. While Objective-C was known for its dynamic runtime and intricate syntax, Swift was designed with clarity and conciseness in mind. The language takes forte from the best practices of both functional and object-oriented programming, helping to usher in a new era in development for the Apple ecosystem.
Design Goals
Swift was conceptualized with several key design goals which reflect a shift towards safety and performance. Here are some central tenets that guided its development:
- Ease of Use: Swift's syntax is easier to learn, making programming more accessible to newcomers while still powerful enough for seasoned developers.
- Performance: Swift was built with speed in mind, often outperforming other languages in common tasks and algorithms.
- Safety: By eliminating certain common programming mistakes, such as dereferencing null pointers, Swift strives to enhance developer productivity and application performance.
- Modern Features: The language incorporates advanced features, such as type inference and functional programming paradigms, allowing developers to write more expressive and maintainable code.
Target Audience
The beauty of Swift lies in its versatility and appeal to a wide range of programmers, from students and hobbyists to seasoned IT professionals. Here are some of the groups who will find Swift not just beneficial but essential:
- New Developers: For those just dipping their toes into programming, Swift's concise syntax and clear structure make it an excellent choice for learning.
- iOS Developers: Given that Swift is deeply integrated into the iOS app development ecosystem, seasoned developers upgrading their skills will find it vital to understand Swift's intricacies.
- Backend Developers: With the rise of server-side Swift, professionals involved in backend services stand to gain from learning the language, broadening their career paths.
"Understanding Swift sets a solid foundation for any developer looking to engage with the Apple ecosystem or advance to modern programming practices."
In essence, the introduction to Swift is about recognizing not only the language’s technical strengths but also its role in shaping a developer's mindset towards safety, performance, and accessibility. Engaging with this dynamic language can shift paradigms, enabling programmers to build applications that are robust, efficient, and scalable.
Core Features of Swift
Understanding the core features of Swift is crucial for anyone aiming to become proficient in this programming language. Each feature contributes not only to the efficiency of coding but also promotes readability and maintainability of the code. In this section, we’ll explore some of the most significant features, namely type safety, optionals and nil-coalescing, and closures and functional programming. These elements can be considered the bedrock upon which Swift developers build robust applications.
Type Safety
Type safety refers to the ability of a programming language to prevent type errors during compilation or runtime. In Swift, type safety is highly emphasized, ensuring that the values of variables are consistent with their data types. This feature reduces potential runtime crashes and enhances the clarity of code.
For instance, consider a scenario where you have to perform mathematical operations. If you mistakenly assign a string to a variable meant for integers, Swift will throw an error at compile-time. This not only saves time during debugging but also encourages developers to write more thoughtful and deliberate code. The use of type inference also simplifies coding, allowing the language to automatically deduce the type of a variable from its initial value. Overall, type safety safeguards the integrity of data in your programs.
Optionals and Nil-Coalescing
Swift introduces a unique approach to handling the absence of a value through optionals. An optional is essentially a variable that can hold either a value or , giving programmers a way to express the absence of data. This might sound trivial, but in practice, it provides a powerful mechanism for avoiding unexpected crashes due to null reference exceptions, which are a common headache in many other languages.
Nil-coalescing is another essential feature revolving around optionals. When dealing with optional values, it can sometimes be necessary to provide a default value. Instead of checking for manually, Swift allows developers to utilize the nil-coalescing operator . Here’s a simple example:
In this case, if is , will default to "Guest". The clean syntax enhances readability while effectively managing optional values, thus making your code more robust and less prone to errors.
Closures and Functional Programming
Closures in Swift are self-contained blocks of functionality that can be passed around and used in your code. They are akin to anonymous functions and are first-class citizens in Swift, meaning they can capture and store references to variables from their surrounding context. This makes them incredibly powerful for implementing completion handlers, callback functions, and functional programming paradigms.
Swift's support for functional programming encourages a different way of thinking about how code is structured. Functions can be treated like variables; they can be assigned to variables, passed as arguments, and returned from other functions. This not only leads to more concise and expressive code but also enables programmers to write cleaner algorithms.
A typical use case could involve filtering an array based on a condition. Instead of looping through an array with verbose control statements, you could employ a closure with the function:
This snippet captures the essence of functional programming, allowing the coder to focus on what to achieve rather than how to do it. The expressive nature of closures significantly simplifies many programming tasks.
In summary, the core features of Swift—type safety, optionals with nil-coalescing, and closures—provide essential building blocks for developing efficient and reliable applications. These features encourage a programming style that is both safe and expressive, paving the way for enhanced productivity and reduced likelihood of bugs in your code.
Swift Syntax and Structure
Understanding Swift Syntax and Structure is fundamental for anyone diving into Swift programming. The syntax serves as the very backbone of the language, providing the rules and conventions that ensure code can be easily read, written, and maintained. A well-structured approach to coding enhances clarity, reduces errors, and increases collaboration among developers. Not only does adherence to syntax improve code quality, but it also accelerates the learning process for newcomers, making them feel less overwhelmed when grasping new concepts.
Basic Syntax Overview
At its core, Swift exhibits a clean and expressive syntax. It is designed to be concise while still being powerful. Variables are declared using the keyword for mutable variables and for constants, making it instantly clear whether a value can change or not. For example:
This simplicity allows programmers to focus on logic rather than getting bogged down by complex declarations. Swift’s preference for readability means that code often resembles natural language.
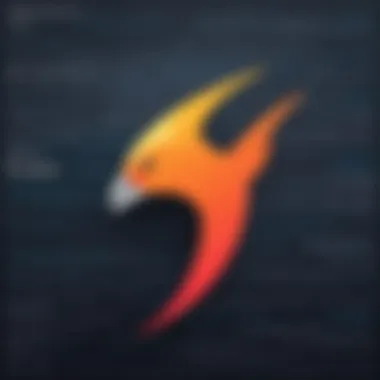
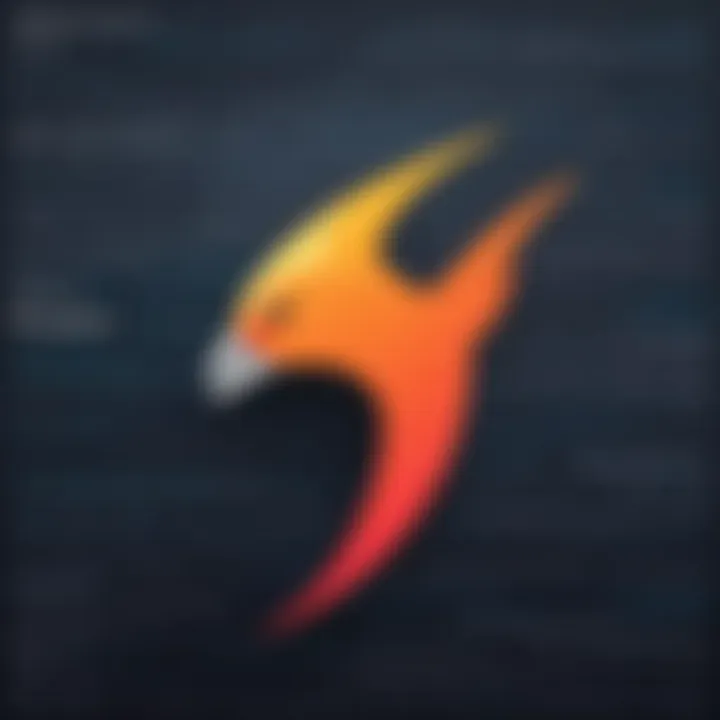
Furthermore, Swift allows type inference, meaning you don't always need to explicitly define the data type:
This capability helps clean up the syntax and lead to more fluid coding.
Control Flow Statements
Control flow is essential in any programming language, providing the means to direct the flow of execution based on conditions or iterations. Swift's control flow statements include , , and loop constructs such as and . This control allows programmers to make decisions during runtime.
For instance, an statement is used to execute code only if a condition is true:
In contrast, the statement is particularly powerful in Swift, accommodating multiple conditions cleanly and efficiently. Using minimizes the need for long chains of statements and makes the code easier to read:
This structure allows developers to tackle complex logic more easily, keeping their code tidy and understandable.
Functions and Methods
Functions and methods are pivotal in structuring code for reusability and clarity. In Swift, functions are first-class citizens, meaning they can be passed around as values, assigned to variables, and returned from other functions.
Defining a simple function in Swift is straightforward:
This clarity allows programmers to focus not just on what the code does but also on how to express it succinctly. Additionally, methods are functions that belong to a certain type (usually classes or structs). Calling a method is as easy as appending it after the instance name:
Understanding functions and methods also prepares learners to work with closures, another advanced topic in Swift, reflecting its functional programming paradigms and promoting cleaner coding practices.
In short, the syntax and structure of Swift are crafted to be intuitive—a quality that stands out in comparison to many other programming languages. As Swift continues to evolve, maintaining this focus on readability and efficiency will undoubtedly attract new generations of programmers.
Data Types and Structures
In Swift programming, data types and structures serve as the backbone of how information is represented and manipulated. Understanding these concepts is crucial because they govern how data is stored, accessed, and transformed throughout your programs. Mastering data types allows for efficient memory utilization and contributes to the overall robustness and readability of your code.
When programmers choose the appropriate data types and structures, they not only streamline processing but also boost performance and prevent common bugs—helpful, right? In this section, we’ll line up the various built-in data types, delve into collections like arrays and dictionaries, and explore how custom types and structs can enhance your code's flexibility and organization.
Built-in Data Types
Swift provides an array of built-in data types that cater to common programming needs. These are the very first building blocks you’ll come across. Familiarizing yourself with them not only speeds up the coding process but also lays a solid foundation for greater topics. The primary built-in data types include:
- Int: Represents integer values, both positive and negative, like 42 or -7.
- Double: Stores floating-point numbers with a higher precision, ideal for scientific computations, e.g., 3.14159.
- String: Manages collections of characters, such as names or sentences, for handling text within your application.
- Bool: This one’s for true or false values, useful in conditional statements and logical expressions.
These data types come with many inherent functionalities that help avoid many common pitfalls in programming. For example, by leveraging Swift's type inference, the compiler often automatically determines the data type based on the assigned value, reducing errors and improving code clarity.
Collections: Arrays and Dictionaries
Collections in Swift take data management a step further. Two of the most prevalent types, arrays and dictionaries, each serve unique purposes and make life easier for a programmer.
- Arrays: This ordered collection holds elements of the same type. You can think of it like a row of books on a shelf—indexed and easy to locate. For instance, if you have an array of integers like , accessing any number is as simple as knowing its position. You could also add or remove items without tedious operations.
- Dictionaries: Think of dictionaries as mini databases. They store key-value pairs allowing you to retrieve values via their keys efficiently. This structure is akin to a contact list on your phone; you look for a name (the key) to get the number (the value). A simple code snippet would be:
This structure is especially beneficial when it comes to quick lookups or when data association is crucial, such as linking user accounts to their preferences or settings.
Custom Types and Structs
While built-in types give a solid grounding, creating custom types and structs elevates your programming game. Swift's support for creating complex types empowers you to define your own data structures tailored to specific needs.
Defining a custom struct allows you to encapsulate related data and functionality, promoting a modular approach to coding. Here’s a quick example:
In this case, the struct models both a person’s name and age. Using custom types not only enhances code readability, making it clear what each piece of data represents, but it also allows for more organized and scalable code—ideal for larger projects.
Furthermore, Swift accommodates protocols and extensions, which can extend the functionality of these custom types seamlessly. This flexibility enables programmers to create richer applications that can evolve as requirements change.
Object-Oriented Programming in Swift
Object-oriented programming (OOP) holds a pivotal spot in computer programming. For Swift, a language built with modern paradigms in mind, OOP isn’t just an afterthought; it’s part of its DNA. OOP helps programmers organize their code in a way that is both flexible and effective, promoting the reuse of components and enhancing collaboration among developers.
It's paramount for those learning Swift to grasp OOP concepts. The shaping of the code using classes and objects lays a sound foundation. Code becomes more maintainable, and when extending applications or debugging, it usually makes finding issues less of a headache.
Classes and Inheritance
Classes form the blueprint from which individual objects are created. Think of a class like a recipe; it outlines the ingredients and steps but doesn't produce a dish until it's executed. In Swift, defining a class can be as simple as this:
Here, we’ve defined a class called with properties and a method. What's striking is the ability to inherit from existing classes. This is where the beauty of OOP shines. For example, if we want to extend to represent a :
In this snippet, inherits from , taking on its properties and methods while being able to override them. This allows programmers to create a hierarchy, thereby promoting code reuse. Inheritance helps to reduce redundancy, ultimately hurting the maintainability of your applications if overused. Keeping an eye on this balance is crucial.
Protocols and Extensions
Protocols give you a powerful way to define a blueprint of methods, properties, and other requirements. Swift's protocol-oriented programming paradigm emphasizes the importance of protocols. When a class conforms to a protocol, it agrees to implement its required methods. This can enhance flexibility because it allows for multiple conformance. For instance:
In this example, conforms to the protocol, thus adopting its requirements. This structure gives you the ability to write code that can work with any object that conforms to a specific protocol, allowing for cleaner and more reusable code.
Moreover, extensions enable you to enhance existing classes, structs, and protocols without altering their original source code. For instance:
By utilizing extensions, you can keep your code modular. Each bit can focus on a specific task, making it easier to read and manage.
Error Handling
When it comes to programming, mistakes are part of the rodeo. Swift has a robust error handling system designed to make it clearer and easier to manage these issues. Instead of letting the program crash, you can use Swift’s error types to handle problems gracefully.
Here’s how an error might be defined and handled:
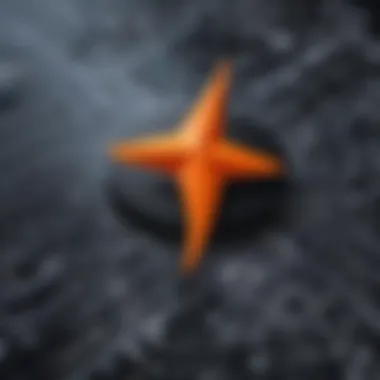
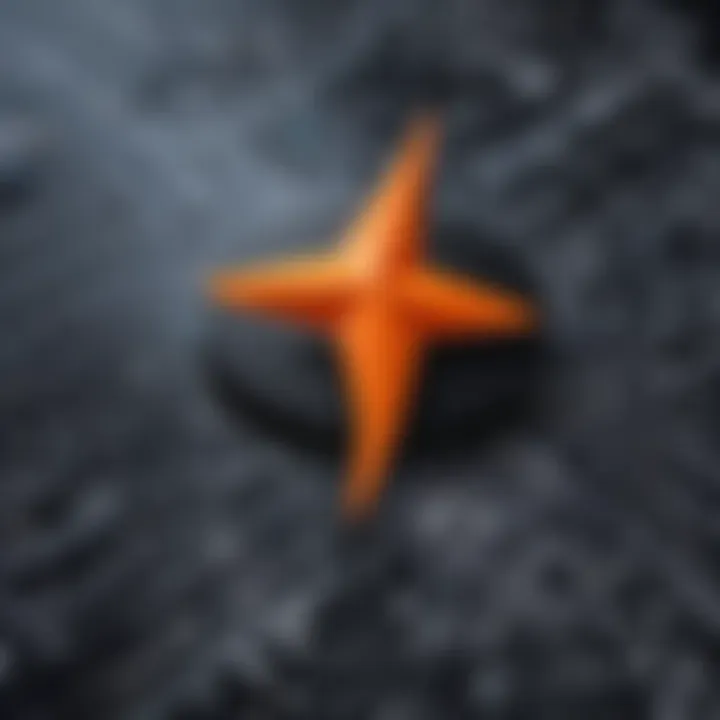
In this example, we’ve defined an error type and a function that could potentially throw an error. By using the statement in Swift, you can handle errors appropriately, ensuring that your program won’t come crashing down. This allows for more robust applications and a smoother user experience.
In object-oriented programming, embracing OOP concepts such as classes, inheritance, protocols, and error handling can crucially enhance your programming toolkit.
Asynchronous Programming in Swift
Asynchronous programming in Swift is a pivotal concept for developers aiming to create fast, responsive applications. In today’s digital landscape, users expect applications that perform seamlessly, without delays. This is where asynchronous programming comes into play. It separates tasks that can happen simultaneously, allowing programs to handle multiple operations at the same time. This not only boosts the performance of applications but also enhances user experience by providing a smooth workflow.
As we venture deeper into this section, we will dissect the core elements of asynchronous programming in Swift, its benefits, and the considerations that developers must bear in mind. By grasping the following concepts, programmers can significantly optimize their coding strategies and application outcomes.
Concurrency Basics
Concurrency is the backbone of asynchronous programming. It enables multiple tasks to coexist and run simultaneously, thus improving efficiency. Understanding how concurrency functions in Swift involves familiarizing oneself with the idea of threads. In Swift, each thread represents a path to execute a sequence of commands.
- Threading: Each thread can run its own tasks independently. When one thread is busy waiting for a resource, others can continue with their operations. This understanding can help developers avoid bottlenecks in processing.
- Synchronization: Managing shared resources among threads is crucial. Without proper management, data can become corrupt when multiple threads attempt to access the same resource concurrently.
Developers must also consider whether tasks are CPU-bound or I/O-bound, as this distinction helps in determining the most effective implementation strategy.
Grand Central Dispatch
Grand Central Dispatch, often referred to as GCD, is a powerful system that allows developers to manage concurrent tasks efficiently. It simplifies the scheduling of code execution, allowing for more straightforward performance optimization.
- Dispatch Queues: GCD uses dispatch queues to handle tasks. There are serial queues, which process tasks one at a time, and concurrent queues, which execute multiple tasks at once. Choosing the right type of queue can greatly affect the responsiveness of applications.
- Background Processing: Offloading tasks to background queues frees up the main thread, keeping the user interface responsive. This is crucial when performing high-load operations like network requests or data parsing.
Utilizing GCD is a practical way to exploit the hardware capabilities of modern devices, especially with multi-core processors.
Swift's Async/Await
Swift’s introduction of async/await syntax marks a prominent advancement in writing asynchronous code. This feature allows developers to write asynchronous code in a more synchronous fashion, bridging the gap between clarity and performance.
- Readability: Code written using async/await is often easier to read and understand because it resembles sequential logic rather than the more complex nested callbacks or closures.
- Error Handling: Swift’s syntax allows for better handling of errors using blocks, making it more intuitive compared to traditional methods.
By employing async/await, developers can streamline their code structure, which can lead to fewer bugs and reduced development time.
"Asynchronous programming is not just about managing tasks, it’s about improving the overall user experience. Swift’s advancements in this area allow developers the luxury of focusing on code clarity without sacrificing performance."
In summary, mastering asynchronous programming in Swift encompasses learning about concurrency fundamentals, leveraging Grand Central Dispatch, and utilizing async/await. These elements come together to empower developers to create highly responsive applications while maintaining clean and understandable code structures.
Integration with Xcode
In the tech landscape, Xcode is the go-to integrated development environment (IDE) for Swift programmers. Its robust capabilities facilitate every step of the application development process, from writing code to testing, debugging, and deploying applications. Understanding how to effectively integrate Swift with Xcode can set you apart in your programming journey. Let's delve into the essential facets of this integration.
Setting Up the Environment
Setting up your development environment in Xcode involves a few straightforward steps. First and foremost, you need to install Xcode from the Mac App Store. Once it’s installed, you’ll want to open it and create a new project.
- On the Xcode welcome screen, click "Create a new Xcode project."
- Choose a template for your project; for most apps, a Single View App is a good starting point.
- Fill in the required information, like project name and language (ensure you select Swift).
- Finally, choose a suitable location on your disk to save your project.
A well-configured environment enhances productivity. You can easily navigate through files, set breakpoints, and use the Interface Builder to design your app's user interface without needing to wrestle with complicated settings.
Debugging Techniques
Debugging is crucial for ensuring that your code runs smoothly. Xcode offers a robust set of debugging tools that help you track down bugs efficiently. Here are some essential techniques:
- Use Breakpoints: Setting breakpoints will pause your program execution at pivotal lines of code. This allows for inspection of variable values and flow control at that moment. It can pinpoint where things go south in your program.
- Debug Area: The Debug area shows the console output and helps view variable values in real time. Utilize the variable inspectors and watch expressions to keep an eye on specific data points.
- View Call Stack: When a crash occurs, the call stack can show the function calls leading to the crash. This gives you a clearer path of what went wrong.
"A good developer is one who writes code that is both simple and understandable, making debugging less of a chore."
Using Playgrounds for Experimentation
Swift Playgrounds are an incredible feature in Xcode that allow you to write and test Swift code in an interactive environment. This tool is especially beneficial for both beginners and seasoned programmers wanting to experiment with new ideas rapidly or test snippets of code. Here’s why you should include Playgrounds in your workflow:
- Immediate Feedback: You can write a line of code and instantly see its result. This interactive loop cultivates a better understanding of programming logic.
- Learning Tool: Playgrounds are ideal for practicing Swift syntax, testing algorithms, and experimenting with different functions without the overhead of setting up full projects.
- Tutorial Integration: Many tutorials are compatible with Playgrounds, which provide challenges that help reinforce your understanding.
Setting your code snippets into Playgrounds allows for a playful yet educational approach to programming, paving the way for deeper understanding without pressure.
In essence, mastering the integration of Swift with Xcode can significantly boost your development prowess. It prepares you to handle real-world applications effectively while establishing a strong foundation for understanding the intricacies of programming with Swift.
Building iOS Applications with Swift
Building applications for iOS using Swift is not just a skill; it’s an essential component of modern software development. This section delves into the fundamentals of creating robust iOS apps, focusing on the languages, frameworks, and tools that make the task both efficient and rewarding. Swift, being designed specifically for Apple’s ecosystems, offers developers a powerful option infused with modern programming sensibilities.
UIKit Basics
UIKit is the backbone of iOS applications, providing essential components, frameworks, and tools to create stunning user interfaces. At its core, UIKit is about building visually appealing interfaces while maintaining functionality. Developers can create views, controls, and event handling in a streamlined environment. This results in smoother interactions—a key factor that impacts user retention.
Key elements of UIKit include:
- View Controllers: They manage a view hierarchy and handle user input.
- Views: Basic building blocks for everything visual, such as buttons, labels, and images.
- Auto Layout: A powerful feature that allows developers to create responsive designs across various device sizes.
It's worth noting that learning UIKit paves the way for mastering more complex iOS applications. Without this foundational knowledge, one may struggle to navigate the broader landscape of iOS development.
SwiftUI Overview
SwiftUI represents a revolutionary shift in how user interfaces are constructed within the Apple ecosystem. Launched as a modern alternative to UIKit, it provides a more declarative approach to UI development. With SwiftUI, programmers describe what their UI should look like, and Swift takes care of the details, allowing for faster prototyping and more readable code.
This innovative framework includes:
- Declarative Syntax: Write less code and get more output, focusing on the UI’s appearance and behavior.
- Integration with Xcode: SwiftUI’s integration with Xcode’s design tools allows developers to visualize changes in real-time.
- Cross-Platform Compatibility: Create interfaces that work across iOS, macOS, watchOS, and tvOS without rewriting code.
As more developers adopt SwiftUI, staying updated with its features is becoming increasingly necessary for those wanting to tap into the latest developing trends in iOS applications.
Data Persistence with Core Data
Data persistence is crucial for iOS applications. Users expect their data to remain consistent, even after the app is closed. Enter Core Data—a robust framework designed to manage object graphs and persist data efficiently. Unlike traditional databases, Core Data integrates seamlessly with Swift, offering powerful functionalities for complex data models.
Key considerations for using Core Data include:
- Object Management: Easily track and modify data using managed objects.
- Migration Support: Core Data provides robust solutions for evolving data models, ensuring smooth transitions as the app grows.
- Performance Optimization: With features like caching and batch processing, Core Data can handle large datasets while maintaining swift app performance.
A sound understanding of Core Data will undoubtedly set developers apart, providing them with the tools to build applications that are not only feature-rich but also maintain a high standard of user experience.
"Incorporating Swift and its frameworks reduces repetitive tasks and enhances productivity, allowing developers to focus on crafting better user experiences."
Overall, mastering the nuances of building iOS applications with Swift unlocks countless opportunities for programmers in the mobile development landscape.
Swift for Server-Side Development
The rise of Swift as a prominent programming language extends beyond the realms of iOS and macOS applications. Its potential for server-side development cannot be overlooked. Swift has tailored its capabilities to fulfill modern web demands that prioritize performance, safety, and simplicity. This section digs into the significance of using Swift for server-side development, discussing its frameworks, building RESTful services, and integrating databases.
Server-Side Frameworks
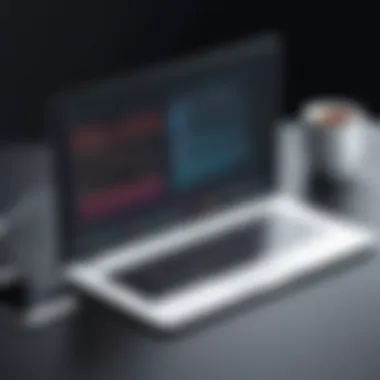
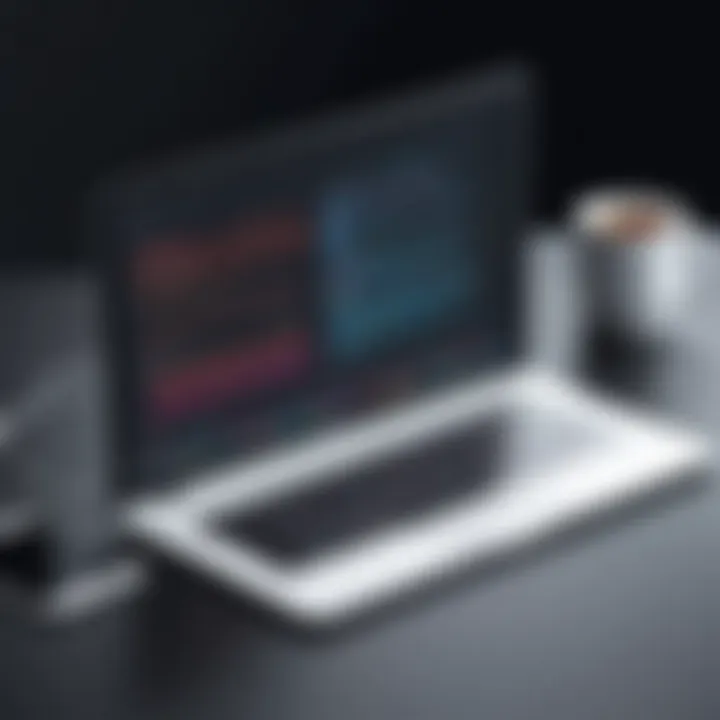
Swift’s server-side frameworks have evolved remarkably. Initially met with skepticism, Swift now boasts solid frameworks like Vapor, Perfect, and Kitura. Vapor, in particular, has become the go-to choice for many developers due to its extensive documentation and privilege features. Each framework offers unique strengths, making it vital for programmers to assess their needs per project.
- Vapor: Known for its expressive syntax, it allows rapid development while maintaining performance. With built-in templating, it brings a modern approach to web development.
- Perfect: A full-featured framework that emphasizes scalability and flexibility, perfect for larger applications.
- Kitura: Developed by IBM, Kitura integrates seamlessly with cloud services, making it suitable for enterprise-level tasks.
Developers opting for these Swift frameworks enjoy type safety, enhanced performance, and an easy transition from Swift's native development on client-side to server-side applications.
RESTful Services in Swift
With the prominence of RESTful services in modern applications, Swift’s ability to handle web APIs efficiently is critical. REST (Representational State Transfer) allows systems to communicate over the web, with JSON being the preferred data format. Swift simplifies creating and handling these services with its clean syntax.
The choice of frameworks facilitates the entire process:
- Creating RESTful APIs: Developers can easily define routes and handle requests and responses, streamlining API development.
- Handling JSON: Swift’s Codable protocol brings ease to JSON encoding and decoding, crucial in RESTful services.
- Asynchronous Callbacks: Using Swift's native support for asynchronous programming, it enables efficient data handling without blocking the main thread, enhancing the user experience.
"Swift’s approach allows developers to write server-side applications that are not only efficient but also intuitive, attracting a broader audience from different backgrounds."
Database Integration
Integrating a database into a server-side application is a cornerstone for many developers. Swift supports several database options compatible with various frameworks, allowing flexibility in choice.
- PostgreSQL and MySQL are among the top contenders for relational databases.
- For NoSQL solutions, integrating with MongoDB or Redis is straightforward.
Using libraries such as Fluent with Vapor, developers can define their data models easily and perform migrations, which is a significant productivity boost. Understanding the underlying database principles and how Swift interfaces with them can lead to efficient data management and retrieval.
To summarize, Swift’s emergence in server-side development offers fresh prospects for developers looking to leverage its robust features while ensuring performance, safety, and maintainability. As the technology landscape keeps evolving, Swift is positioned to be a key player in future server-side solutions.
Comparative Analysis with Other Languages
Understanding Swift's position amidst other programming languages is crucial for several reasons. Comparative analysis allows us to appreciate the unique elements that Swift brings to the table, highlighting its design strengths as well as the challenges it encounters. Programmers often find themselves choosing between languages based on project requirements, team skill sets, or personal preference. By examining Swift in relation to languages like Objective-C and Kotlin, we can uncover insights that help developers make informed decisions about which tools best suit their needs.
Swift vs Objective-C
The historical backdrop of Swift cannot be discussed without acknowledging Objective-C. The latter was the go-to language for Apple’s ecosystem long before Swift entered the scene. Thus, the comparison lies not just in syntax, but in philosophy and approach to coding. Swift is built to be more elegant and easy to read compared to the more verbose Objective-C. This is evident in how it handles memory management, incorporating Automatic Reference Counting (ARC) to reduce the potential for memory leaks.
Opting for Swift means embracing the language’s modern features, such as type safety and optionals, which can prevent many common programming errors encountered in Objective-C. Developers who have transitioned from Objective-C to Swift often remark on the increased productivity due to Swift's cleaner syntax and powerful compiler.
"Swift's expressive syntax makes even the most complex tasks more manageable, something we often struggled with in Objective-C."
However, Objective-C has the advantage of being deeply ingrained in Apple's frameworks. Many libraries, frameworks, and existing projects are written in Objective-C, meaning that while Swift is the newcomer, Objective-C is not going anywhere soon.
Swift vs Kotlin
When comparing Swift to Kotlin, things get a little interesting. Both languages have been engineered with modern programming paradigms in mind, aiming to eliminate common pitfalls found in older languages. Kotlin serves as the preferred language for Android development, while Swift has risen to prominence in iOS development.
Kotlin supports a feature known as null safety, preventing null reference exceptions, much like Swift’s handling of optionals. Both languages also prioritize performance and make use of strong static typing, which helps in maintaining code quality and reducing runtime errors. This means programmers can often find themselves in a familiar territory while working with either language.
However, differences lie in interoperability and platform support. Kotlin can interoperate with Java, allowing for seamless integration into existing Java projects; whereas Swift primarily targets Apple’s ecosystem. Developers may find their choice influenced by the platforms they’re working on. If you’re knee-deep in Apple development, then Swift is likely the way to go.
Performance Considerations
The aspect of performance should not be overlooked when diving into a comparative analysis. Both Swift and its counterparts, like Objective-C and Kotlin, come with their strengths and weaknesses in this regard. Swift is known for its speed and efficiency, often outperforming Objective-C in benchmarks. Its compiler optimizations take advantage of modern hardware, which has become increasingly important in performance-critical applications.
However, as languages continue evolving, performance metrics can change. Benchmarking tests often showcase differences that could impact decision-making for developers. Therefore, it's vital to keep abreast of performance trends and how optimizations play out in both Swift and the languages it competes with.
Best Practices in Swift Development
When diving into Swift programming, it's crucial to consider best practices that not only streamline the development process but also enhance the overall quality of your code. Adhering to established guidelines can facilitate easier maintenance, improve readability, and minimize bugs. Thus, the importance of this topic cannot be understated. This section will cover key elements such as code styling guidelines, version control strategies, and testing and debugging methods—all vital for both new and seasoned programmers.
Code Styling Guidelines
Good code doesn't just work; it shines. Code styling is about making your Swift code easy to read, maintain, and share. Consistency is the name of the game. Here are important tips to follow:
- Follow Swift Naming Conventions: Your variable names should be meaningful, which helps in understanding what the variable represents. For example, instead of , consider .
- Use Spacing and Indentation: Indent your code properly. This makes it easier to follow the flow, especially in complex structures such as loops and conditionals.
- Organize Your Code: Group similar functions or properties together. This makes the logical structure of your code clearer and more intuitive.
Keeping these styling principles in mind can significantly improve efficiency and foster collaboration among team members who might work with your code in the future.
Version Control with Swift
Managing code changes is like keeping your desk tidy; a cluttered workspace leads to confusion. Version control systems help developers track and manage changes. Git is a popular choice in the Swift community. Here are some practices for effective version control:
- Commit Often: Making small, incremental commits helps you keep track of changes without feeling overwhelmed. It’s also easier to identify when a problem arises.
- Write Meaningful Commit Messages: Describe what you changed and why. A message like "Fix typo in the user input method" is far clearer than simply stating "Update code".
- Branching Strategy: Use branches to manage features or hotfixes without disturbing the main codebase. This way, the master branch remains stable while you work on new features in separate branches.
Adopting a robust version control strategy not only saves time but also enhances teamwork and collaboration in software development projects.
Testing and Debugging Strategies
In software development, finding and fixing bugs can feel like searching for a needle in a haystack. Effective testing and debugging strategies can save you a lot of headaches down the line. Here are some strategies to consider:
- Unit Testing: This involves testing individual components in isolation to ensure they function as expected. Swift's XCTest framework makes it straightforward to write and run your tests.
- Continuous Integration: By integrating code changes and running tests automatically, you can catch issues early on. This fosters a culture of accountability and encourages you to write more tests.
- Debugging Tools: Swift comes with powerful debugging tools in Xcode that allow you to step through your code. Don't shy away from utilizing breakpoints and the console.
Utilizing these practices can lead to a more reliable and efficient Swift application, ultimately saving time and resources.
"The best code is the code you never have to write."
By integrating these best practices into your development routine, you establish a strong foundation for yourself as a programmer, enabling not just personal growth but also setting a high standard within your projects. Keep learning, keep improving, and your skills will undoubtedly blossom.
Future Trends in Swift
The swift evolution of programming languages often reflects the demands of technology and developer needs. As Swift continues to gain traction in both mobile and server-side development, understanding its future trends becomes crucial for anyone looking to deepen their expertise. This section dives into not just where Swift is headed, but also why these trends matter, and how they can benefit coders at any level.
Community Contributions
Community plays a pivotal role in the growth of any programming language, and Swift is no exception. With a vibrant ecosystem, developers are continuously introducing libraries, frameworks, and tools that expand Swift's capabilities. The contributions from the community not only enhance what Swift can do but also lower barriers for newcomers.
For instance, many open-source projects like Alamofire, which simplifies HTTP networking, or SwiftNIO, facilitating asynchronous event-driven network applications, showcase how collaborative efforts can improve productivity.
Additionally, engaging in community forums such as Reddit's Swift community can be invaluable for feedback and support. This collective knowledge becomes a powerful resource for solving problems and sharing best practices. Through such channels, developer accessibility to resources increases significantly, adding to Swift's attractiveness as a language of choice.
Emerging Applications
As technology evolves, so too does the application landscape for Swift. Apart from traditional mobile app development, Swift is making strides in areas like machine learning and data analysis. Its performance edge over other languages makes it a suitable candidate for applications that demand speed and efficiency.
Notably, Apple has introduced Create ML, a framework that allows developers to build machine learning models using Swift. This integration makes Swift not just useful for programming but also for engaging with modern, data-driven applications.
Moreover, Swift is gaining ground in the realm of server-side development. With frameworks like Vapor and Perfect, it's becoming easier to build and deploy scalable server applications using Swift, further broadening the audience who may transition from other languages.
Evolving the Language
Swift isn’t static; it’s a living language that undergoes regular updates based on user needs and technological advancements. Each version brings new features that reflect its evolution—enhancements like result builders, property wrappers, and improvements in concurrency seamlessly make it more robust.
Swift's community-driven development model ensures that changes adhere to the expectations of users. For instance, with Swift 5, the introduction of ABI stability represented a significant change. This allows the Swift binaries to be included in applications and improves the support across platforms.
As the programming landscape shifts toward greater reliance on mobile and cloud-based solutions, Swift’s evolution is likely to keep pace. Its compatibility with various architectures and platforms only reinforces its relevance in the coming years.
"Timely advancements in Swift ensure that developers are not only equipped with the tools they need but also stay ahead of the curve in an ever-changing tech world."
Overall, the future of Swift looks promising. The coordinated efforts of the community, the emergence of diverse applications, and the ongoing evolution of the language make it a key player in software development. As programmers gear up to embrace upcoming trends, they can leverage Swift's direction to enhance their profiles and productivity.