Extract Data from PDF to Excel with Python: A Guide
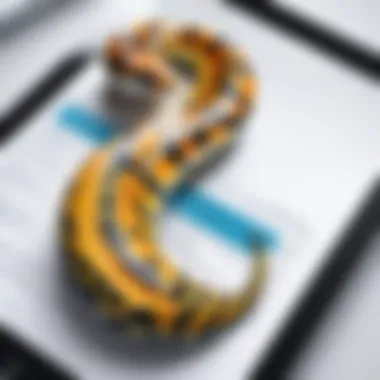
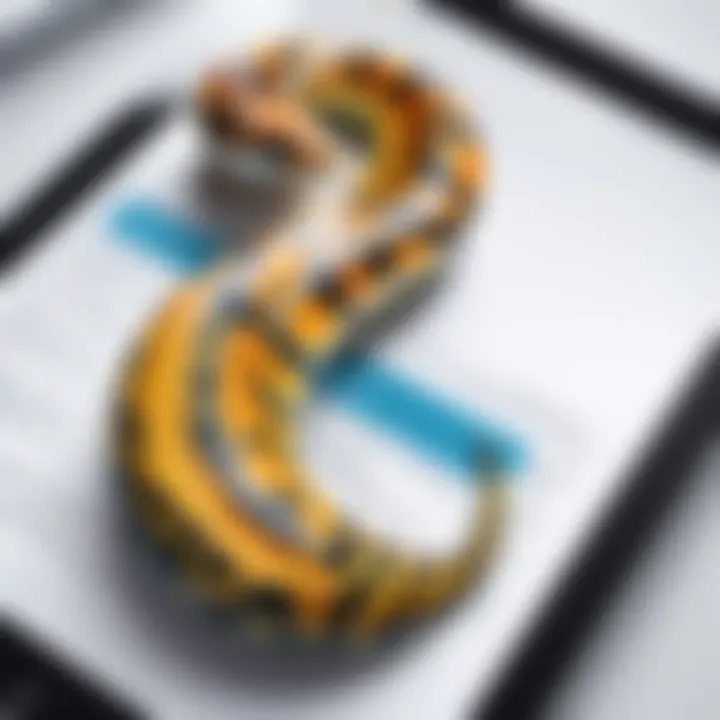
Overview of Topic
This section will introduce the main concept of transforming data from a PDF format into a more manageable Excel spreadsheet. Understanding this process is significant in the tech industry as it improves efficiency in data handling. With data being generated at an unprecedented pace, the ability to extract and transform it effectively becomes essential for professionals in various fields.
Historically, PDF files were created for presentation qualities and not necessarily for data extraction. As Python developed and libraries like PyPDF2 and pandas emerged, so did the potential for easier and faster extraction methods. This evolution marks a shift in data management practices, showing the adaptability of technology in meeting user needs.
Fundamentals Explained
Core Principles and Theories
At its core, the process of extracting data relies on understanding how data is structured within PDFs. Unlike traditional databases or spreadsheets, PDFs do not follow a predictable format. Understanding the structure of PDFs can enhance the extraction process.
Key Terminology
- PDF (Portable Document Format): A file format created by Adobe for document exchange.
- Excel: A spreadsheet software developed by Microsoft, widely used for data manipulation.
- Python: A high-level programming language known for its readability and versatility.
- Library: A collection of pre-written code that simplifies programming tasks.
Basic Concepts
Familiarity with basic Python programming and libraries like Pandas and PyPDF2 is essential. PyPDF2 allows for PDF manipulation, while Pandas provides powerful data manipulation capabilities. Knowing how to navigate arrays, dataframes, and files will aid in understanding the extraction process.
Practical Applications and Examples
The applications for extracting PDF data to Excel are vast. Here are a few domains where this skill is invaluable:
- Finance: Extracting financial statements from PDF reports.
- Healthcare: Compiling patient data from PDF formats.
- Research: Collecting bibliographies or data tables from scholarly articles.
Code Snippets
Here's a basic example of how to use PyPDF2 to read a PDF file:
This code fetches text data from each page of a PDF document. Later, you can process or save this text into an Excel file using the library.
Advanced Topics and Latest Trends
The field of data extraction is always advancing. Newer libraries with enhanced capabilities continue to emerge. Techniques that utilize Optical Character Recognition (OCR), such as Tesseract, are gaining traction. This method can extract data from scanned documents, broadening the scope of what can be accomplished. Expect continued evolution in tools that simplify extraction and ensure accuracy in data processing.
Future Prospects
As machine learning and AI integrate further into data operations, expect tools that make data extraction even more intuitive. The adoption of cloud computing can also enable remote access to extraction tools, allowing for collaborative work across global teams.
Tips and Resources for Further Learning
To deepen your understanding, consider the following resources:
- Books: "Automate the Boring Stuff with Python" by Al Sweigart.
- Courses: Platforms like Coursera and Udemy offer specific courses on Python data manipulation.
- Online Resources: Communities such as Reddit provide forums for discussion.
By leveraging these resources, you can expand your skill set and make the most of Python's capabilities for data extraction from PDF to Excel.
Intro to PDF Data Extraction
Extracting data from PDF files is a crucial skill in today's data-driven world. As organizations and individuals increasingly rely on digital documents, the need to convert data from PDF to Excel has grown tremendously. This is where PDF data extraction comes into play. By mastering this process, one can leverage Python to seamlessly convert data for analysis or record-keeping.
Understanding PDF File Structure
PDF, or Portable Document Format, is designed to present documents consistently across various platforms. The structure of a PDF file is unique. Unlike text files, PDFs store layout-based information. This allows complex documents that include images, text, and vector graphics to be displayed correctly. Understanding how PDFs store this data is crucial for effective extraction.
Key points include:
- Content storage: Text might not be stored in a linear fashion, hard to extract without proper tools.
- Unpredictable layout: Information can be formatted in tables and other configurations, complicating extraction processes.
- Encoding differences: PDFs often contain various encodings, making text recognition challenging.
Recognizing these elements helps in choosing the right extraction method and tool.
Importance of Data Extraction
The importance of data extraction cannot be overstated. Businesses often encounter challenges when attempting to analyze historical data trapped in PDF format. Efficient data extraction can lead to significant benefits:
- Increased productivity: Automating data extraction saves time and reduces human error.
- Enhanced analysis: Converting PDF data to a structured format like Excel enables deeper analysis and insight generation.
- Cost-effectiveness: Automating the manual process of data entry can reduce overhead costs for organizations.
Efficient data extraction transforms inaccessible information into valuable insights.
For students and IT professionals, learning to extract data using Python can provide substantial career advantages. By developing proficiency in extraction techniques, one can improve their programming skills and make a significant impact in their field.
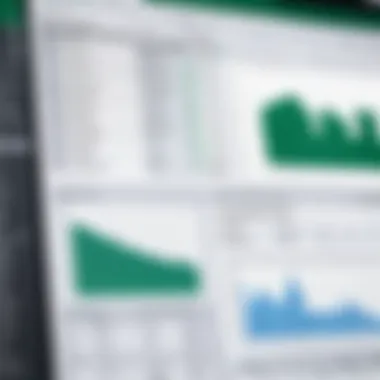
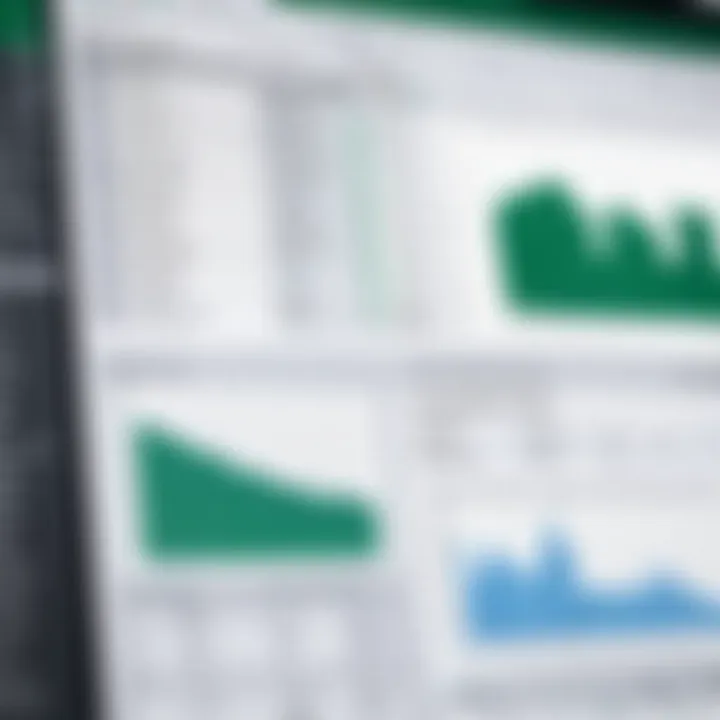
Overview of Tools and Libraries
In the realm of data extraction from PDFs, utilizing the right tools and libraries is crucial. The process of converting data from PDF to Excel using Python requires a robust understanding of various technologies and how they can be leveraged effectively. Each tool serves a specific purpose and offers unique advantages that can simplify complex tasks.
Setting up a Python environment is the first step in this journey. It prepares the workspace where all libraries will be installed, ensuring that dependencies are managed effectively. Proper environment setup can prevent future technical hurdles and streamline the coding experience.
Python Environment Setup
To begin extracting data, one must first establish a functional Python environment. This includes installing Python itself, as well as a package manager like . A common practice is to use a virtual environment, which helps in managing dependencies isolated from other projects. This can be accomplished with the following steps:
- Install Python from the official website.
- Use to install libraries as needed.
- Consider using virtual environments with tools like or .
A well-structured environment not only supports the workflow but also enhances the reliability of the scripts developed.
Key Libraries for PDF and Excel Manipulation
Several Python libraries stand out when it comes to manipulating data within PDF files and exporting it into Excel. Each library comes with its strengths depending on the specific requirements of a project. Below are some of the most commonly used libraries for this task:
PyPDF2
PyPDF2 is primarily used for reading and extracting text from PDF files. Its strength lies in its simplicity and ease of use. One key characteristic is its ability to handle simple text extraction and minor modifications of PDF files. For instance, users can merge, split, and crop PDFs with minimal code.
- Benefits*: It’s lightweight and has a clean, intuitive API, making it popular for quick extractions.
- Disadvantages: PyPDF2 struggles with complex layouts or images within PDFs, leading to incomplete data extraction in certain cases.
PDFMiner
PDFMiner is tailored for more complex documents. It provides a deeper level of parsing than PyPDF2, which is crucial when dealing with intricate layouts and rich content. What sets PDFMiner apart is its ability to extract both text and layout, giving an accurate representation of the original document.
- Benefits: It can accurately capture text positioning and font information, which is vital for extracting data from complex PDFs.
- Disadvantages: However, its steeper learning curve may not make it the first choice for beginners.
Pandas
Pandas is not just for data manipulation in Excel; it plays a significant role in the entire data processing workflow. After extracting data, Pandas allows users to clean and organize data efficiently. It is particularly useful when converting the extracted data into a desirable format for analysis or export.
- Benefits: It provides powerful data manipulation tools and supports various file formats, enabling seamless integration into the data processing workflow.
- Disadvantages: Pandas can be resource-intensive for large datasets, which may slow processing times on lower-end systems.
OpenPyXL
OpenPyXL shines when it comes to creating and modifying Excel files. It offers advanced features like formatting cells, creating charts, and working with various Excel functions. Its integration with Pandas allows for smooth transitions from data cleaning to final output format.
- Benefits: This library supports many Excel features, catering to users looking to produce professional and formatted spreadsheets.
- Disadvantages: Custom configurations can be complex and may require a bit of a learning curve to utilize fully.
Extracting Text from PDF Files
Extracting text from PDF files is a critical step in the process of transforming data into usable formats, especially when transitioning data into Excel using Python. PDFs are widely used for document sharing due to their consistent formatting across different platforms. However, this consistency makes extracting data a bit challenging, as the structure is not inherently designed for easy data manipulation. Understanding how to extract text effectively can greatly enhance data handling capabilities, enabling users to pull relevant information from complex documents.
The benefits of efficient text extraction are numerous. It allows users to automate tedious data entry processes, minimize human errors, and save time. Additionally, having text in a digital format enables further analysis, visualization, and reporting. Given the vast amount of information locked away in PDF files, mastering this skill becomes essential for students, IT professionals, and anyone dealing with data management.
Using PyPDF2 for Text Extraction
PyPDF2 is one of the simplest libraries in Python to extract text from PDF files. Its ease of use makes it suitable for beginners and those who need quick results without delving deep into complex coding structures. This library works by reading the PDF file and allowing access to the text content contained within it.
To get started, install PyPDF2 via pip:
After installation, the following Python code snippet shows how to use PyPDF2 for extracting text:
In this code, we open the given PDF document and employ the to access its pages. The method is then called for each page, gathering the complete text into a single string. While PyPDF2 is effective for simple PDFs, it may struggle with more complex layouts, such as those containing images or unusual formats.
Leveraging PDFMiner for Complex Documents
For more intricate PDF files, PDFMiner emerges as a powerful alternative. Unlike PyPDF2, PDFMiner parses the layout of the document, making it possible to capture texts arranged in more complicated structures, including multiple columns or embedded images. This results in more accurate representations of the original document.
To start using PDFMiner, first install it:
Here is how to use PDFMiner for text extraction:
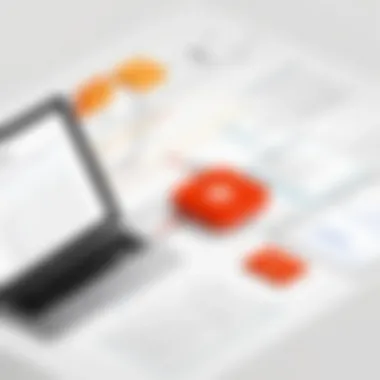
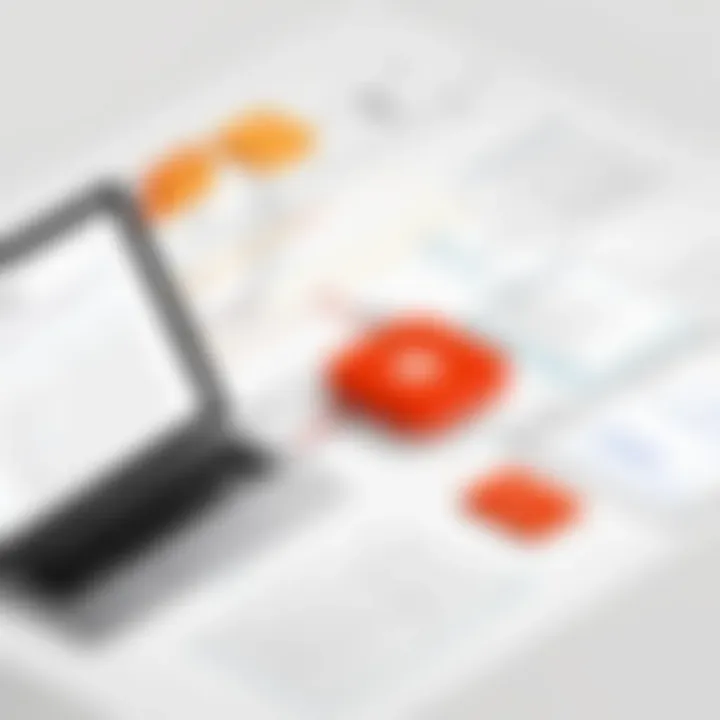
The function is much more capable in rendering text from documents that might otherwise baffle simpler libraries. By converting the text layout into a meaningful output, users can better maintain the intended formatting and structure of the original document.
In summary, extracting text from PDF files is foundational for effective data manipulation and analysis in Python. Whether using PyPDF2 or PDFMiner, selecting the right tool for the job makes a significant impact on the quality of extracted data.
Handling PDF Tables
Handling PDF tables is a critical aspect of working with data extraction, particularly when converting PDF documents into Excel format. PDFs can contain various forms of structured data, including tables, which are often not straightforward to extract. Proper handling of these tables allows for more efficient data manipulation and analysis, essential for any data-driven task.
When working with PDF files, the ability to identify tables effectively is paramount. Tables can vary in complexity, with some containing merged cells, varied row heights, and intricate formatting. Understanding how to approach these tables can significantly impact the quality of the extracted data.
Engaging with tables allows developers and analysts to transform static information into a dynamic format, such as Excel, where further calculations and adjustments can be performed. Misunderstandings in identifying or extracting table data can result in inaccuracies, leading to incorrect analyses or faulty business decisions. Thus, it is vital to approach the extraction of table data with a plan.
Identifying Tables in PDF
The first step in extracting table data from PDFs is identifying where these tables are located within the document. This task can be more challenging than it appears.
- Visual Inspection: Open the PDF file and visually check for tables. This is important because not all tables are formatted uniformly. The table's layout might vary across different documents.
- Pattern Recognition: Learning to spot common indicators of tables, such as grid lines or consistent spacing between data points, can be beneficial. This practice can save time and help in determining extraction methods more effectively.
- Software Tools: Utilizing PDF analysis tools can streamline this process. Tools like Tabula or PDFMiner can assist in outlining tables within the PDF. These tools often provide a graphical interface to help identify table structures for easier extraction.
Extracting Tabular Data Using Tabula
Tabula is a robust library specifically designed for extracting tables from PDFs. It can be a game changer for those needing to convert PDF table data into Excel. Below are the essential steps to use Tabula for effective data extraction:
- Install Tabula: Begin by installing the Tabula library, usually via pip.
- Basic Implementation: With Tabula installed, you can start extracting tables. The following code snippet illustrates the basic usage:
Specify the PDF file path
file_path = 'path/to/your/pdf/document.pdf'
Extract tables into a DataFrame
df = tabula.read_pdf(file_path, pages='all')[0]
Save to Excel
df.to_excel('extracted_data.xlsx', index=False)
In this code, a simple dataset is created and converted into a DataFrame. The method is then used to export this DataFrame to an Excel file named . The parameter ensures that the row indices will not be written in the final spreadsheet, providing a clean output.
Using Pandas not only simplifies the process but also maintains data integrity.
Utilizing OpenPyXL for Advanced Excel Features
While Pandas is excellent for basic exporting, OpenPyXL brings in additional features that enhance Excel file handling. This library allows users to manipulate worksheets, format cells, add charts, and utilize more complex Excel functionalities that are often essential in data reporting.
For instance, you can create an Excel file where you format specific cells, set styles, and even protect worksheets. Here’s a simple example:
In this example, a new workbook is created, worksheet data is filled in, and then saved to an Excel file named . This approach allows for finer control over how the exported data appears, providing both aesthetic and functional advantages.
Utilizing OpenPyXL could remarkably improve the presentation and usability of your Excel files, catering to specific project needs.
Common Challenges in Data Extraction
Extracting data from PDF files is not without its challenges. Understanding these common issues can significantly enhance the effectiveness of the extraction process. When working with PDFs, one often encounters various obstacles that can hinder data capture and transformation efforts. The complexities of PDF file formats and differing data structures can lead to additional complications. This section explores those challenges, particularly focusing on dealing with scanned PDFs and handling malformed PDFs.
Dealing with Scanned PDFs
Scanned PDFs present a unique set of challenges due to the nature of the data they contain. Unlike regular PDFs, which often hold text data, scanned PDFs create images of the text. This means that extracting the data requires Optical Character Recognition (OCR) technology, which can introduce inaccuracies. These inaccuracies can stem from a variety of factors:
- Image Quality: Poor resolution scans can lead to misinterpretation of characters.
- Font Styles: Uncommon fonts or handwritten text can reduce OCR effectiveness.
- Layout Complexity: Text that appears in non-standard formats, such as columns or tables, can complicate extraction.
Using libraries such as Tesseract in conjunction with Python can help alleviate some of these issues. Tesseract allows the conversion of scanned images into editable text, enabling more straightforward subsequent processing. However, it is highly recommended to conduct post-extraction validation of the data to ensure accuracy. Here’s a simple example of how to implement OCR with Tesseract:
This code uses the Python Imaging Library (PIL) and Tesseract to convert an image of a scanned document into text. The quality of results will depend largely on the original image quality.
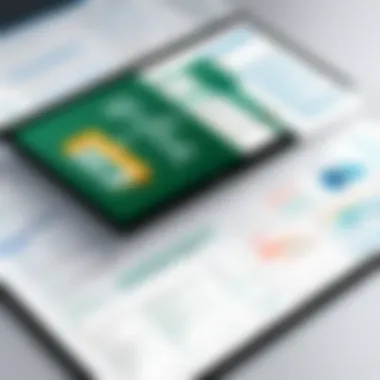
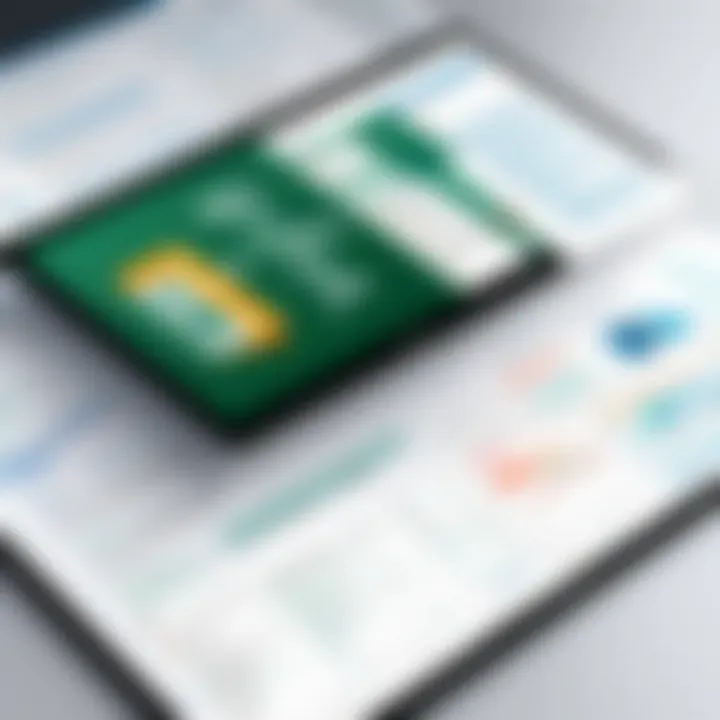
Handling malformed PDFs
Malformed PDFs can be another significant hurdle in the data extraction process. These are PDFs that may not conform to the standard PDF specifications. Malformations can appear for various reasons, including:
- Corrupted Files: Data within the PDF may become corrupted due to incomplete downloading or save processes.
- Unsupported Features: Some PDFs may contain features that certain libraries do not support, like advanced security settings or annotations.
- Inconsistent Structure: A poorly structured or generated PDF may lead to various interpretation issues, making it difficult for extraction tools to handle them properly.
To address these types of issues, the first step is to verify the integrity of the PDF file. If a file is corrupted, recovery options are limited, and re-obtaining the document may be necessary. For PDFs with unsupported features, evaluating different libraries may provide a solution. Tools like PDFMiner or PyPDF2 have different parsing capabilities and can be useful for extracting text or contents from complex structures. In some cases, repairing a PDF using third-party tools may be viable; however, this process can be time-consuming and may not yield guaranteed results.
Addressing these challenges in data extraction is crucial for achieving accurate and usable outputs. Understanding the specific nature of these issues allows developers to better prepare their extraction pipelines and mitigate potential errors, ensuring a smoother data manipulation process throughout.
Best Practices for Effective Data Extraction
Data extraction from PDFs to Excel is a nuanced process that requires attention to detail and a strategic approach. Following best practices ensures that the extraction is both accurate and efficient. These practices not only streamline the workflow but also enhance the overall quality of the data being handled. By implementing sound techniques, users can mitigate common pitfalls and maximize the utility of extraction processes.
Optimizing Extraction Techniques
To begin with, optimizing extraction techniques is crucial. Selecting the right libraries can make a significant difference. Libraries like PyPDF2 for straightforward text extraction or PDFMiner for more complex documents are essential based on the specific type of data in use. Here are several additional techniques to consider:
- Batch Processing: For large PDF files, process pages in batches to save time.
- Setting Parameters: Adjust parameters according to the document’s structure, such as defining the area from which to extract data.
- Sample Runs: Conduct sample runs on smaller sections of data before executing the full extraction.
These techniques may help to identify unpredicted issues early and refine the extraction approach.
Regular Expressions for Improved Data Capture
Regular expressions (regex) provide a powerful method for improving data capture during the extraction process. By defining specific patterns, users can filter and capture relevant information more effectively. This is particularly useful when extracting structured data like emails, phone numbers, or specific keywords. Some key points about using regex include:
- Precision: Regular expressions can capture exact text patterns, reducing the likelihood of errors.
- Flexibility: They can be adapted to various formats, allowing extraction from different types of documents easily.
- Efficiency: Using regex can speed up the process by automating the identification of specific data points.
For example, here is a simple regex pattern to extract email addresses:
Incorporating best practices for effective data extraction not only improves the extraction quality but also equips users with the skills they need to handle diverse documents in their data processing workflows effectively. Following the above recommendations will ensure that the data extracted is relevant and useful for subsequent analysis.
Real-World Applications and Use Cases
The extraction of data from PDF files into Excel has become increasingly relevant across various fields. This process plays a critical role in enhancing efficiency, improving data accuracy, and facilitating easier analysis. Understanding the real-world applications and use cases helps illustrate the importance of mastering these skills, especially for students, individuals learning programming languages, and IT professionals.
Business Intelligence and Analytics
In the realm of business intelligence, organizations often accumulate vast amounts of data in PDF reports, white papers, and financial documents. Extracting data from these sources allows businesses to compile relevant insights and visualize them in Excel. With Excel's powerful analytical tools, data can be transformed into charts and dashboards, leading to more informed decision-making.
Moreover, converting PDF data enhances data integration. This allows companies to merge data from various sources, thus providing a holistic view of their operations. The ability to extract and manipulate this data not only streamlines reporting processes but also fosters a more agile approach to analysis.
Benefits include:
- Improved accuracy in data reporting.
- More efficient data manipulation and analysis.
- Enhanced visualization capabilities through Excel.
Academic Research Data Compilation
In academic settings, researchers often rely on published papers, government reports, and statistical analyses found in PDF format. The ability to extract data from these files enables seamless data compilation for academic research projects. This process is essential for conducting meta-analyses and literature reviews, where synthesizing information from multiple studies is necessary.
By converting PDF data into Excel format, researchers can easily manage datasets, perform statistical analyses, and visualize their findings in a clearer manner. This is particularly relevant for students involved in research projects or theses, as organized data leads to more comprehensive evaluations and conclusions.
Considerations include:
- The importance of accuracy when compiling data to ensure valid results.
- The need for effective data management practices to handle large volumes of information.
Overall, the extraction of data from PDF to Excel serves as a powerful tool in both business intelligence and academic research, enhancing data utility and supporting informed decisions across various domains.
The End
In the realm of data management, extracting data from PDF files to Excel using Python has become increasingly significant. This article highlights the continuous need for effective data processing strategies in various sectors such as business, academia, and technology. Mastering the extraction process not only enhances one's technical skills but also contributes to greater efficiency in data handling.
The melding of Python’s capabilities with PDF manipulation techniques opens up several avenues for automation and analysis. Efficient data extraction methods save time, reduce errors, and allow users to streamline workflows. Hence, having robust knowledge about the extraction process is a valuable asset, especially in today’s data-driven environment.
"In an age where data is more abundant than ever, the ability to convert and manipulate data formats is paramount."
Recap of Key Points
The journey through this guide demonstrates several essential components of the data extraction process:
- Understanding PDF Structure: Knowing how a PDF file is organized is foundational for successfully extracting content.
- Tools and Libraries: Familiarity with Python libraries such as PyPDF2, PDFMiner, Pandas, and OpenPyXL provides the groundwork for effective data manipulation.
- Text and Table Extraction: Techniques for both text and tabular data extraction ensure comprehensive data retrieval styles.
- Data Cleaning: Ensuring the quality and readiness of extracted data before exporting it to Excel is crucial for accuracy.
- Export Techniques: Utilizing Pandas and OpenPyXL maximizes the potential of Excel, allowing for advanced data operations.
- Common Challenges: Recognizing and planning for challenges in PDF data extraction helps to refine strategies.
- Best Practices: Following established guidelines leads to more efficient and reliable data extraction.
Future Directions in PDF Data Processing
As technology evolves, so too do the methods and tools available for extracting PDF data. Future advancements might include:
- Integration with Artificial Intelligence: Leveraging machine learning can lead to smarter data extraction processes, especially from complex or unstructured PDFs.
- Enhanced OCR Technology: Improved optical character recognition systems will make it easier to extract text from scanned documents accurately.
- Cloud-Based Solutions: Moving to cloud-based extraction tools can facilitate collaborative work and improve accessibility.
- Real-Time Data Processing: Developing solutions for real-time data extraction and manipulation would greatly enhance decision-making capabilities in time-sensitive situations.
As the landscape of data continues to change, staying updated with the latest tools and practices will ensure that professionals remain adept and competitive in their fields.