Create a Flutter App from Scratch: A Comprehensive Guide
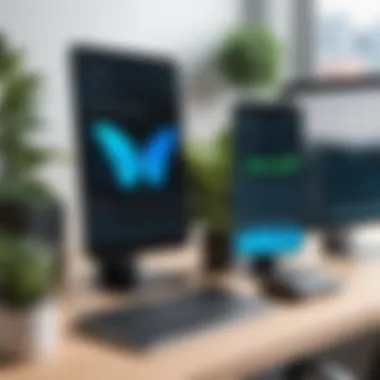
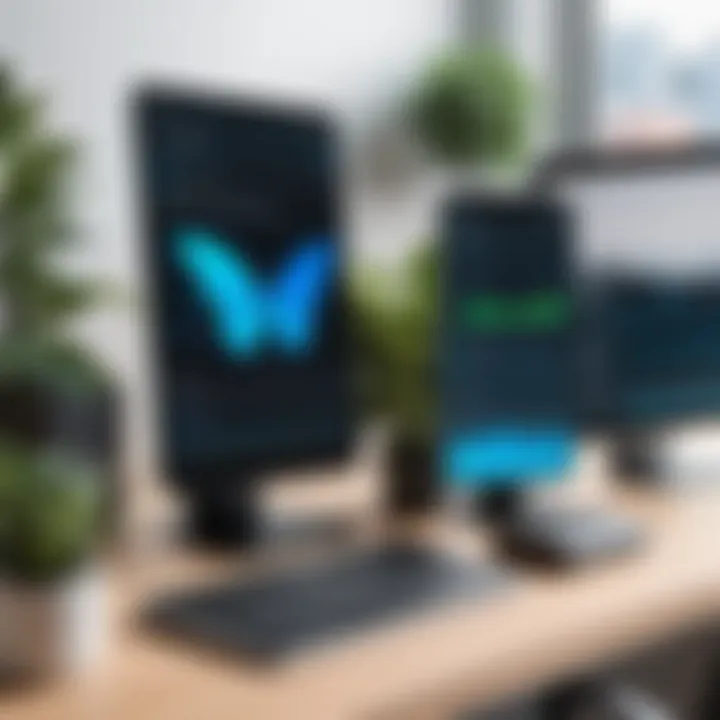
Overview of Topic
Building an application with Flutter is not just a technical endeavor; itās a gateway into the evolving world of mobile development. Flutter, created by Google, has changed the game with its unique architecture and ease of use.
This section lays down the framework for what weāll explore as we move through this guide. The main concept here revolves around the Flutter SDK and Dart programming language, focusing on how they intertwine to facilitate rapid app development.
Scope and Significance in the Tech Industry
The significance of learning Flutter stretches far and wide within the tech industry. As a cross-platform toolkit, it allows developers to target multiple platforms with a single codebase, saving time and resources. With organizations increasingly looking for efficiency, mastering Flutter can be a vital asset.
Brief History and Evolution
Flutter has come a long way since its initial release in 2017. Starting as an alpha version, it has rapidly evolved, earning a higher profile in the developer community. Integrating feedback and improvements, Flutter 2.0 brought in support for web and desktop applications, pushing forward the frontiers of what development tools can achieve.
Fundamentals Explained
Understanding the fundamentals is essential for effective Flutter application development. Hereās a pointer on key principles and terms youāll encounter.
Core Principles and Theories
Flutterās standout feature is the widget tree: everything in Flutter is a widget, whether itās the interface or a simple button. This leads to a highly customizable and organized layout, crucial for crafting a responsive design.
Key Terminology and Definitions
- Widgets: The building blocks of any Flutter app.
- Stateful vs Stateless Widgets: Core concepts that determine whether a widget can hold state over its lifetime.
- Hot Reload: A feature allowing developers to see changes in real-time, drastically speeding up the development cycle.
Basic Concepts and Foundational Knowledge
To effectively work with Flutter, grasping the Dart language is indispensable. Dartās syntax is clean and easy to understand, making it suitable for both new and experienced developers. Understanding async programming is also crucial, as it enhances the appās responsiveness by handling operations without blocking the user interface.
Practical Applications and Examples
Nothing solidifies understanding like practical application.
Real-world Case Studies and Applications
Several companies have leveraged Flutter to create stunning applications. For example, companies like Alibaba and Google Ads utilize Flutter for their mobile interfaces, showcasing the real-world effectiveness of the framework.
Demonstrations and Hands-on Projects
Creating a simple app, say a To-Do list, offers a great starting point. Begin by setting up your Flutter environment with Visual Studio Code or Android Studio, then follow along with the example below:
Code Snippets and Implementation Guidelines
Try to break down your code into widgets to enhance reusability. New developers often try to do everything in one place. Instead, transform complex widgets into simple ones and build from there.
Advanced Topics and Latest Trends
Staying informed about the latest trends is crucial in tech.
Cutting-edge Developments
As of 2023, Flutter continues to adapt, introducing features like integration with machine learning APIs, making it a powerful tool for modern app development. The latest version of Flutter emphasizes performance optimizations that resonate with large-scale applications.
Advanced Techniques and Methodologies
Familiarize yourself with state management solutions such as Provider or Riverpod, as scalability often hinges on how well you manage the app's state.
Future Prospects and Upcoming Trends
The future of Flutter looks promising. With advances in desktop and web integration, developers can expect to develop applications that are not just mobile but cross-platform, enabling a wider reach.
Tips and Resources for Further Learning
To keep your skills sharp, consider diving into these resources:
- Books: "Programming Flutter" offers a comprehensive understanding of the framework.
- Online Courses: Platforms like Udemy have substantial resources focused on Flutter.
- Tools and Software: Get familiar with tools like Flutter DevTools for debugging and performance insights.
Prelude to Flutter
In the realm of mobile app development, Flutter has carved its niche, becoming a go-to framework for many developers. Itās crucial to grasp the core elements of Flutter before diving headfirst into creating your first application. Understanding Flutter entails not just knowing what it is but also recognizing the context in which it thrives. This section will set the stage for your journey into the world of Flutter.
What is Flutter?
Flutter is an open-source UI software development toolkit created by Google. With it, developers can build beautiful, natively compiled applications for mobile, web, and desktop from a single codebase. The emphasis on cross-platform compatibility means that a single code written in Flutter can work on both Android and iOS, making it a time-saver for developers and businesses alike.
But itās not just about functionality; Flutter offers a rich set of customizable widgets that add flair to your applications. From buttons to complex layouts, you have the flexibility to design what suits your appās vision.
Key Features of Flutter
When discussing Flutter, several standout features demand attention:
- Hot Reload: This feature allows developers to see changes in real-time without restarting their application. It dramatically speeds up the development process, making adjustments easier and helping spot issues quickly.
- Rich Set of Widgets: Flutter has a huge catalog of pre-built widgets, which enables developers to craft intricate applications more efficiently. These widgets are designed to follow the specific design languages of iOS and Android.
- Native Performance: Flutter compiles the code into native machine code, enhancing the performance without compromising on the user experience. This is a game-changer for developers focusing on performance-intensive applications.
- Strong Community Support: Being spearheaded by Google, Flutter is backed not only by a vast community but also a myriad of resources and packages that simplify development.
Advantages of Using Flutter
The benefits of adopting Flutter for mobile app development are compelling:
- Unified Codebase: Write once, run anywhere. This means less time wasting on maintaining separate codebases for iOS and Android.
- Faster Development Cycle: With tools like hot reload and extensive libraries at your disposal, you can develop applications more rapidly than with traditional frameworks.
- Customization: The highly customizable widgets mean that your app can maintain a unique look and feel, aligning with brand identity without major hurdles.
- Growing Ecosystem: Flutter's ecosystem is expanding, with constant updates and new packages, making it a future-proof choice.
"Flutter is more than just a technology. It embodies a philosophy of creating highly performant applications with ease and style."
Setting Up the Development Environment
Setting the stage for any software development project calls for a carefully structured environment. The same rings true for building Flutter applications. This section hones in on the essential step of getting your development environment in tip-top shape. By establishing a solid foundation, developers can focus on crafting applications instead of wrestling with configuration issues later on. When an environment is right, it smoothens the workflow, leading to increased productivity and reduced frustration.
So, why is setting up the development environment crucial? First off, it allows for seamless integration with the tools youāll be using to write, test, and run your Flutter apps. Having the Flutter SDK installed, along with a suitable IDE, enables efficient coding practices and enhances real-time testing capabilities. Moreover, understanding how to interact with emulators and devices will significantly impact the development lifecycle.
Installing Flutter SDK
To get the ball rolling, the installation of the Flutter SDK is a non-negotiable step. Think of the SDK as the toolkit that offers all the necessary components needed to develop a Flutter application. The Flutter SDK provides the framework, libraries, and command-line tools to build and deploy your applications.
When diving into the installation process, first head over to the official Flutter install page. Choose your operating systemāWindows, macOS, or Linuxāand follow the very clear instructions laid out there. Once installed, make sure to add the Flutter and Dart SDK to your system's PATH. This ensures you can run Flutter commands directly from the terminal.
After getting it installed, run the following command in your terminal:
This nifty command checks the status of your Flutter installation. It informs you about missing dependencies or issues that could hamper your progress.
Configuring IDEs for Flutter
A key part of the development process is selecting and configuring an appropriate Integrated Development Environment (IDE). Flutter developers tend to favor both Android Studio and Visual Studio Code due to their distinct advantages.
Setting up Android Studio
When it comes to Android development, Android Studio is the heavyweight champion. Its robust features align perfectly with Flutterās requirements. Android Studio allows you to design and develop interactive apps with ease. One of its standout traits is the built-in support for Flutter plugin installations, which adds more functionality.
Not only does this IDE provide a range of tools for debugging and testing, it equally comes equipped with designing UI components. The drag-and-drop interface simplifies UI creation, making it user-friendly even for those who might not have extensive coding experience.
Yet, itās crucial to keep in mind that Android Studio can be a bit resource-heavy. If youāre working on a less powerful machine, it might slow things down.
Configuring Visual Studio Code
On the other side of the coin, we have Visual Studio Code, often cited as the lighter alternative. With its simple interface, Visual Studio Code maintains robust support for Flutter development as well. A key characteristic is the extensive library of extensions available, which can customize your development experience dramatically.
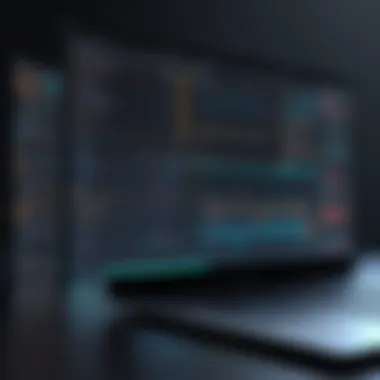
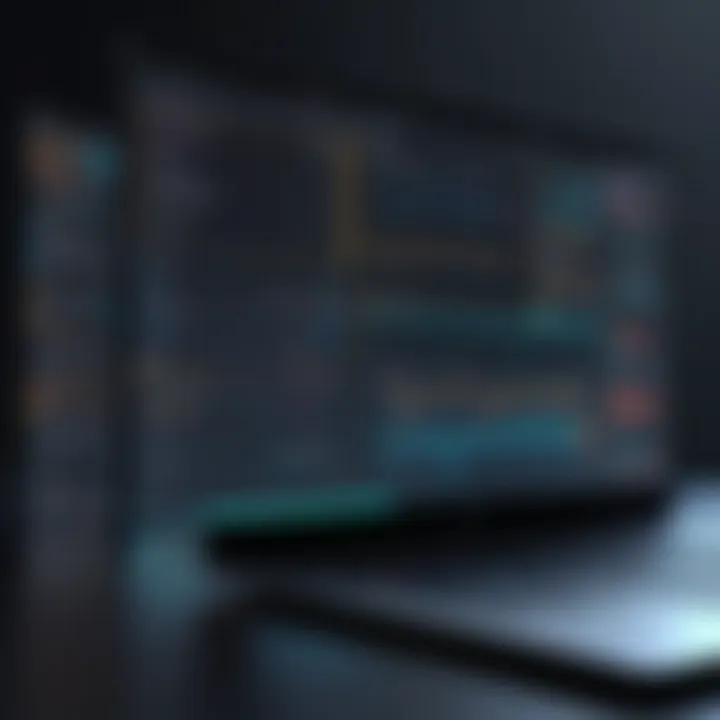
One of the unique features of Visual Studio Code is its integrated terminal, allowing for easy command-line access without needing to switch windows. Additionally, it has excellent debugging capabilities and is less demanding on system resources. This can be a deciding factor for developers working on older hardware or who enjoy a smoother experience.
Both IDEs come with their own pros and consāchoosing the right one often depends on personal preference and project requirements.
Emulators and Device Testing
Lastly, successful app development is incomplete without proper testing, which leads us to the importance of emulators and device testing. Running your app on an emulator gives a preview of how it will function on actual devices. Emulators can simulate various devices, operating systems, and screen sizes, providing versatility in testing different scenarios.
Real device testing is also essential. In real-world situations, users might encounter various issues that fellow developers couldn't replicate on an emulator. Testing on actual devices helps uncover these potential problems, leading to a more polished final product.
To conclude, the development environment is arguably the backbone of your Flutter application project. A thoughtful setup saves time and enhances productivity, ensuring a seamless coding experience as you bring your app ideas to life.
Understanding Dart Language Basics
Dart is the language that underpins Flutter, making it essential for anyone serious about developing applications with this framework. Understanding the basics of Dart not only aids in grasping Flutter more effectively but also enables the developer to write cleaner, more efficient code. It's much like learning to read music before composing; you may have ideas floating around, but it's the fundamental knowledge that will allow you to bring them to life.
In this section, we will cover several core areas within Dart, including its syntax, data types, and control flow statements. Each of these components plays a pivotal role in structuring your Flutter applications and directly impacts performance and maintainability.
Dart Language Syntax
Dart has a syntax that borrows elements from languages like Java and JavaScript while also introducing its own unique features. So if you've dabbled in those languages, Dart should feel somewhat familiar.
Hereās what stands out in Dartās syntax:
- Variables and Functions: Dart allows you to define variables with , , or , giving flexibility in variable assignment. For example:
- Control Structures: Dart uses traditional control structures such as , , and , making it easy to implement logic.
- Classes and Objects: Dart is an object-oriented language, and everything revolves around classes, making it fairly straightforward to encapsulate behaviors and data.
Overall, mastering the syntax will prepare you for writing robust and efficient code, ensuring that your applications function smoothly while being easy to read.
Data Types and Variables
In Dart, data types dictate how variables are stored and manipulated. Understanding how to use these types effectively is a corner-stone of writing functional code.
Dart has several built-in data types:
- Numbers: Both for integers and for floating-point numbers.
- Strings: Textual data enclosed in single or double quotes.
- Booleans: The simplest types, or .
- Lists: Ordered collections of items, akin to arrays in other languages.
- Maps: Key-value pairs that allow you to store more complex data structures.
With variable declarations, Dart also introduces type inference. While you can specify types explicitly, Dart often determines them automatically:
These features enhance your ability to manage data within your applications and facilitate robust programming practices that are less prone to errors.
Control Flow Statements
Control flow statements form the backbone of your application logic, allowing you to dictate what happens based on certain conditions. Understanding these statements is crucial for creating a responsive and functional application.
Here are some key control flow structures:
- If-Else Statements: Useful for branching logic based on conditions.
- Switch Cases: A cleaner way of executing different code blocks depending on the value of a variable compared to a series of if-else statements.
- Loops: 'for', 'while', and 'do-while' loops are essential for executing code multiple times until a condition is met.
Here's a simple example illustrating these concepts:
By mastering control flow statements, you pave the way for dynamic and interactive mobile applications, significantly enhancing user experience.
"Knowledge of Dart's basic principles will ultimately lead to robust Flutter applications, translating abstract ideas into tangible user experiences."
Creating Your First Flutter App
Creating your first Flutter app is not just about coding; itās a rite of passage for many budding developers. It serves as the bridge between understanding Flutterās environment and the practical application of its features. As we navigate through this section, weāll discuss key elements, benefits, and important considerations.
Starting your journey with Flutter is like learning to ride a bike. At first, it might seem daunting. But once you figure it out, the freedom it brings is unparalleled. The process introduces you to Flutter's intricacies, opening the door to a realm of possibilities in mobile app development. Beyond the technical knowledge, there's an emotional wināwatching a vision transform into a working application.
Project Structure Overview
Every Flutter application has a distinct structure that lays the foundation for smooth development. This structure organizes your code, resources, and assets in a manner that developers can easily navigate. Itās crucial to understand this architecture early on, as it aids in readability and maintainability throughout your development journey.
Here's a high-level breakdown of the standard Flutter project structure:
- lib/: Where most of your code will reside, particularly Dart files.
- pubspec.yaml: The project configuration file, managing dependencies and assets.
- assets/: Any media files like images or fonts that your app requires.
- ios/ and android/: Platform-specific configurations for iOS and Android.
This structured layout not only keeps things tidy but also adheres to best coding practicesālike keeping separate files for different functionalities. Moreover, it allows you to scale your projects more easily as they grow.
Building the User Interface
Building the User Interface (UI) is where the magic happens. The UI is often the first impression users get; therefore, it has to be functional yet visually appealing. Flutter provides a rich set of widgets to facilitate this.
Understanding Widgets
Widgets are the building blocks of your Flutter app. They encapsulate everything in your applicationāfrom structural elements like buttons and text to complex layouts. Think of them as the individual pieces of a jigsaw puzzle. Without a complete puzzle, you can't see the full picture.
An important aspect of widgets in Flutter is their reusability. You can build custom widgets tailored to your needs, which promotes cleaner code by reducing duplication.
- Key Characteristic: Lifecycles make managing state and theming significantly easier, thus enhancing performance.
- Unique Feature: Hot reload allows developers to see the changes made instantly without restarting the app, which accelerates the development process.
While widgets offer several advantages, itās essential to keep them well organized to prevent overwhelming the application with too many components at once.
Layout and Design Principles
Once you understand widgets, you can dive into layout and design principles. Good design isn't just about aesthetics; it's about functionality and usability as well. Considering mobile devices, itās vital to create interfaces that are intuitive, responsive, and accessible.
- Key Characteristic: Flutter employs a rich set of layout widgets that adapt to various screen sizes, thus enhancing user experience.
- Unique Feature: The concept of flexible layouts allows for dynamic resizing, which is crucial as users operate across multiple devices.
Understanding how to properly structure your layouts can make or break your app. If not done carefully, your app can turn into a convoluted mess that frustrates users.
Adding Functionality to the App
Adding functionality is where the rubber meets the road. Itās one thing to have a beautiful interface; it's another to make it functional. This section often involves using methods, state management tools, and APIs, making your app alive and interactive. Every tap, swipe, or button press serves a purpose. Each functionality should enhance the user experience and not complicate it.
To sum it up, creating your first Flutter app lays the groundwork necessary for deeper knowledge and more complex projects. Gaining familiarity with project structure, widgets, layout designs, and adding functionalities will make your journey through Flutter smooth and enjoyable.
"A journey of a thousand miles must begin with a single step." - Lao Tzu
Letās take that step toward building our app with these essential cornerstones in place.
State Management Approaches
State management is a foundational aspect of building applications in Flutter. It refers to how a Flutter app handles the data that changes over time, typically in response to user interactions or external events. Managing the state effectively can significantly improve the app's performance, user experience, and maintainability. While it might seem straightforward, state management can become quite intricate, especially as an application scales in size and complexity.
In this guide, we will delve into the primary state management approaches utilized in Flutter, breaking down key advantages, potential challenges, and the ideal contexts for each solution. This exploration will provide developers with a robust understanding which is essential for creating interactive and responsive apps.
Understanding State Management
At its core, state management in Flutter involves the handling of data that influences how the app renders its user interface. When a user's action prompts a change, whether that means updating a form or fetching data from the internet, the application must react accordingly.
Consider this: when a user taps a button to change a setting, the state of that setting must be updated and reflected in the UI immediately. If this doesn't happen seamlessly, users can become frustrated, leading to a poor user experience. Additionally, state management frameworks also help in separating business logic from UI code, making the application easier to test and maintain.
Popular State Management Solutions
Several state management solutions cater to Flutter developers, each bringing its own strengths and weaknesses. Here, we will explore three prominent options: Provider, Riverpod, and Bloc.
Provider
Provider is one of the most popular state management libraries within the Flutter ecosystem. It leverages a simple and elegant approach to dependency injection and state management, making it a solid choice for many developers. One key characteristic of Provider is its ease of use, which allows for quick implementation without overwhelming beginners.
A notable aspect of Provider is its use of ChangeNotifier, which helps Flutter apps automatically rebuild the UI when the state changes. This feature simplifies the code and makes it more readable. However, it does have its limits ā managing complex states can become cumbersome, often necessitating additional layers of abstraction.
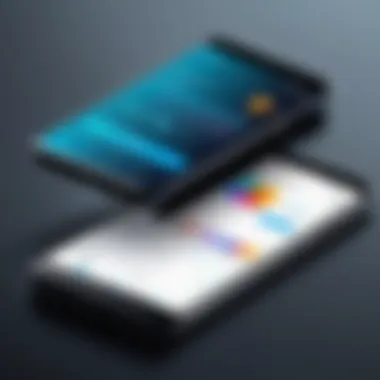
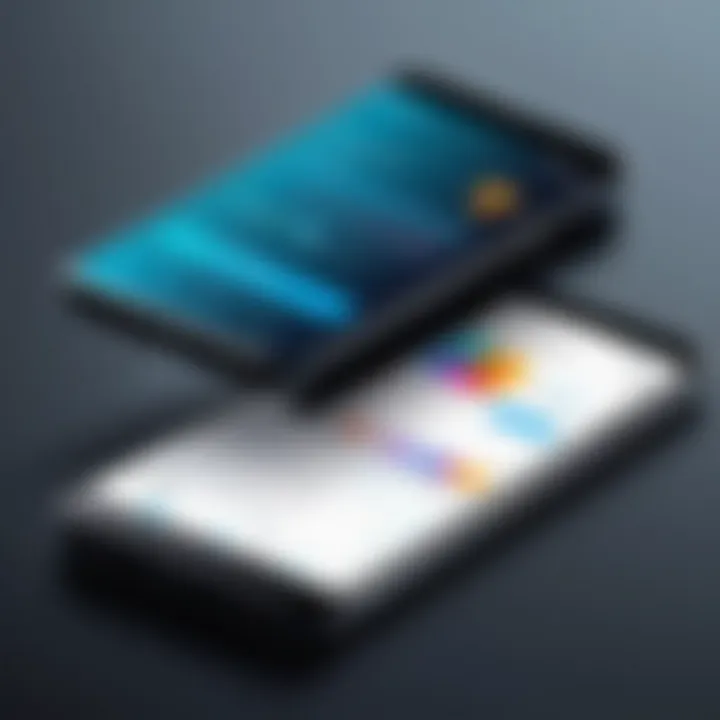
In summary, Provider is beneficial for smaller to medium-sized applications where simplicity and quick implementation are essential. It shines in environments where the app may not have a complex structure, allowing developers to focus on functionality rather than intricate state handling.
Riverpod
Riverpod is a reactive and modern state management solution that emerged as a successor to Provider. It's designed to overcome some limitations of its predecessor by providing better support for modularity and testability. A key characteristic of Riverpod is that it eliminates much of the boilerplate code found in Provider, which leads to cleaner and more maintainable codebases.
One unique feature of Riverpod is its ability to encapsulate state management in a way that it remains independent of the widget tree, enhancing reusability. This approach allows developers to access the same state from different places in the application without tightly coupling components.
Riverpod is an excellent choice for larger applications where modularity and separation of concerns are vital. Despite its enhanced flexibility, some developers may find the learning curve a bit steeper compared to Provider due to its more complex API.
Bloc
Bloc, which stands for Business Logic Component, offers a more structured approach compared to both Provider and Riverpod. It utilizes Streams and Sinks, making it particularly powerful for applications that demand a clearer separation between UI and business logic. This separation can result in improved code organization, making it easier to maintain and test.
A standout feature of Bloc is the ability to utilize Events and States, where users send events and the Bloc responds by emitting new states. This provides a robust way of handling asynchronous activities, making it an appealing choice for applications that deal with rich interactions or complex data flows.
While Bloc is incredibly powerful, it often requires writing more code than the other solutions, which might not be ideal for smaller applications or those with simpler needs. It's generally recommended for larger applications where the investment in learning and using the framework pays off in sustained maintainability and scalability.
"Choosing the right state management approach is critical. It can streamline development and enhance the end-user experience significantly."
Understanding and selecting the appropriate state management approach is crucial for Flutter developers aiming to create responsive, maintainable, and high-performing applications. Each of these solutions presents unique features that cater to different application requirements, making an informed choice all the more vital as one embarks on their app development journey.
Integrating APIs and Backend Services
Integrating APIs and backend services is a cornerstone of mobile application development in todayās digital age. Flutter excels in providing developers with the tools to connect their applications to various external services, enhancing functionality and user experience. With the ability to fetch real-time data, send user inputs to servers, and access third-party services, integrating APIs can make your application not only interactive but also powerful.
Think about it: without APIs, an application would be a standalone entity with limited capabilities. By tapping into APIs, developers can bring the world to their apps, such as pulling in live weather data, displaying social media updates, or even fetching data from a userās favorite database. The possibilities here are endless.
Some benefits of integrating APIs include:
- Enhanced Functionality: Leveraging existing services leads to more robust applications.
- Efficiency: Rather than building system components from scratch, APIs can be integrated with ease, saving time and resources.
- Real-time Interaction: Allows applications to provide real-time data to users, making the app feel more alive.
- Scalability: Integrating with various services means the app can evolve as new technologies and APIs become available.
However, combining external services isnāt without its challenges. Developers must consider security issues, error handling, and the potential for dependency on third-party services that may change or become unavailable. Careful planning, thorough testing, and understanding the APIās capabilities and limitations are key to smooth integration.
"In the digital ecosystem, if you arenāt integrating with others, you might be left alone on an island."
Making HTTP Requests
One of the first steps in integrating APIs is making HTTP requests, a fundamental method for fetching data or sending information to a server. In Flutter, this process is managed through the package, which simplifies working with HTTP requests.
Start by adding this package to your file:
Making a GET request looks something like this:
This snippet shows a basic GET request that retrieves data from an API endpoint. Itās straightforward, but it lays the groundwork for more complex operations. When dealing with APIs, always remember to manage errors gracefully; a userās experience hinges on how well these situations are handled.
Handling JSON Data
When working with APIs, youāll often encounter JSON data, given its popularity for data exchange. JSON (JavaScript Object Notation) is lightweight and easy for humans to read and write, as well as easy for machines to parse and generate. In Flutter, converting JSON to Dart objects is a common task.
You can make use of Dartās built-in library for this purpose. Hereās a simple approach to decode JSON:
In the example above, the method is used to convert a JSON string into a Dart object. From there, you can manipulate or display the data as you see fit. The goal is to ensure a seamless transition between how information is stored on the server and how itās presented to users.
Connecting to Firebase
Firebase is often the go-to solution for developers looking to integrate backend services in their Flutter applications. With a whole suite of tools covering databases, authentication, and storage, Firebase simplifies many backend challenges.
To connect your Flutter app with Firebase, begin by registering your app on the Firebase console and adding the required dependencies to your project.
Hereās a brief insight on how to set up Firebase in Flutter:
- Create a Firebase project on the Firebase Console.
- Add your Flutter app to the Firebase project.
- Follow the steps provided to modify your and files.
- Add the required FlutterFire plugins to your projectās file.
The integration with Firebase allows for streamlined features such as user authentication, cloud storage, and real-time databases. This not only enhances app functionality but also provides a smoother user experience.
By incorporating APIs and backend services, your Flutter application becomes more appealing and effective. It not only fetches real-time data and connects to third-party services but also handles data securely and efficiently. This foundational knowledge is crucial as you delve deeper into Flutter development.
Testing Your Flutter App
In the realm of software development, ensuring that your application works as intended is essential. Testing in Flutter serves not just as a safety net but as a crucial part of the development cycle. When you come across bugs or unwanted behaviors in an application, it typically leads to poor user experiences. Testing helps you pinpoint these issues early in the process, saving you time and resources down the road. This section delves into various testing methodologies within Flutter, laying out the importance of unit testing, widget testing, and integration testing.
Testing is the cornerstone of building reputable applications. It gives you confidence that every change made doesnāt accidentally introduce new problems. Think of it as maintenance for your codebase; without it, you might find yourself in a quagmire trying to fix things last minute. Hereās what you need to know:
- Improves code quality: A well-tested application is usually more robust and easier to maintain.
- Saves time: Catching bugs early reduces the need extensive debugging periods.
- Enhances user satisfaction: If users find bugs consistently, they'll likely uninstall the app, which can lead to a loss in credibility.
"A stitch in time saves nine"āthis old saying couldnāt be more relevant in the world of app development.
Having laid out the importance of testing, letās dig deeper into the types of testing you can implement.
Unit Testing Basics
Unit testing is a process where individual pieces of code, known as units, are tested in isolation. This usually applies to functions and methods, checking if they perform as expected. In Flutter applications, unit tests focus on business logic.
Starting unit tests typically involves the following:
- Choosing a Testing Framework: Flutter comes with a great testing library called that is integrated into your Dart environment.
- Writing Your Tests: You'll often structure tests to cover various scenarios for each function.
- Running Tests: Tests can be run from the command line using .
Here's a simple example of a unit test in Dart:
This code snippet shows how to test the addition of two numbers using Dartās test package. Remember to focus on edge cases that could cause your functions to behave unexpectedly.
Widget Testing
Widget testing dives deeper than unit tests. It involves testing individual widgets in isolation, ensuring they work properly within the app's framework. This aspect is particularly vital in Flutter, as the framework is built around the use of widgets.
The following points outline widget testing:
- Building Widgets: You can create widgets in a test environment to see how they render and function.
- Interaction Testing: This involves user interactions like taps and swipes, ensuring the widget responds accurately to user inputs.
For example, hereās how you might test a button widget:
This test checks if a button click increments the displayed counter. Widget tests can take a little more time but are invaluable for verifying that complex UIs behave as intended.
Integration Testing
This testing type checks entire applications or a significant part of them, focusing on how various elements interact. Integration tests are crucial for ensuring everything works together, from the user interface to the backend connections.
In Flutter, integration testing ensures that your app functions as expected from the perspective of an end user. Consider these strategies:
- Combining Elements: Testing entire user flows from start to finish.
- Simulating User Scenarios: Creating tests that mimic user actions and the expected system reactions.
A simple integration test might look like this:
This snippet tests the counter increment functionality in an integrated application environment. By ensuring compounds of your app work together, you're increasing reliability and a more seamless user experience.
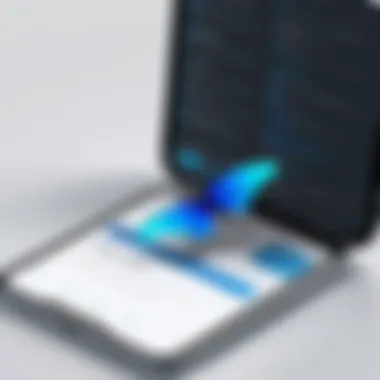
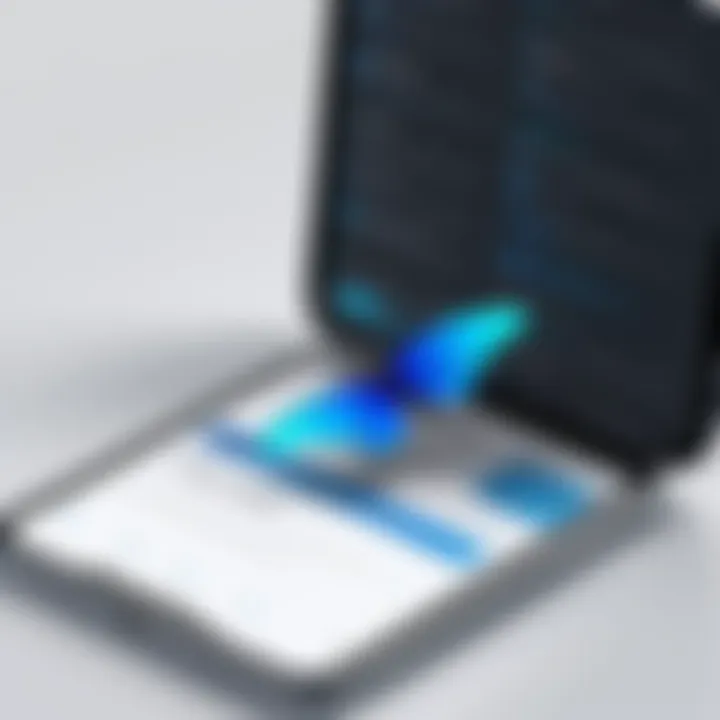
Debugging and Performance Optimization
In the world of mobile app development, mastering debugging and performance optimization is akin to being the captain of a ship navigating through stormy waters. Itās not just about launching an app; it's about ensuring that the app runs smoothly and efficiently in the hands of the users. In this section, we will explore why these elements are crucial in Flutter development and how they contribute to a robust application.
When developing an app, bugs can feel like uninvited guests that show up when you least expect them. They can disrupt the user experience, hinder functionality, or even crash the app entirely. This underscores the importance of effective debugging strategies. Meanwhile, performance optimization focuses on making your app run faster and more responsively, translating to a better experience for the end users. After all, in a world where attention spans are as fleeting as a summer breeze, ensuring that your app performs well can be the difference between user retention and abandonment.
Debugging Techniques in Flutter
Debugging in Flutter involves a mix of tools and practices, which can significantly simplify the bug-fixing process. Below are a few notable techniques to help you dive into the debugging waters:
- Flutter DevTools: This is a powerful suite of performance and debugging tools provided by Flutter. By taking advantage of this toolkit, you can inspect the widget tree, monitor performance metrics, and even debug layout issues. Itās like having a magnifying glass to find those sneaky bugs hiding in your code.
- Print Statements: While it sounds primitive, using print statements can help you trace the logic of your code. By strategically placing these canaries in your code, you can track the flow of execution and catch anomalies early on.
- Hot Reload: One of the best features in Flutter, hot reload allows developers to apply changes to the code quickly without losing the current state of the application. This streamlines the debugging process and speeds up the entire development cycle.
- Asserting Conditions: Using can catch issues during development. If a condition evaluates to false, the app will throw an error. Itās a simple yet effective way to enforce invariants in your code, acting as early warning bells for larger problems down the road.
Implementing these techniques enables developers to tackle issues methodically, improving code quality and stability.
Identifying Performance Bottlenecks
Performance bottlenecks can feel like roadblocks on a smooth highwayāthey impede progress and frustrate drivers. They typically occur due to various factors such as heavy animations, widget rebuilds, or inefficient network calls. Addressing these limitations can elevate your app from mediocre to exceptional. Hereās how you can identify and fix performance issues in your Flutter app:
- Performance Profiling: Using Flutter DevTools, you can analyze the performance of your app and identify where slowdowns occur. It provides valuable insights, much like a mechanic diagnosing issues in a car.
- Widget Rebuilds: Excessive widget rebuilds can drain resources. Keep an eye on which widgets need to rebuild and try to minimize unnecessary updates. Using constructors can help ensure that widgets only rebuild when necessary.
- Lazy Loading: For long lists or heavy content, implementing lazy loading can improve performance. By loading items only as they come into view, you save processing time and memory, effectively reducing the workload on the app.
- Optimize Images and Resources: Large image sizes or unoptimized assets can significantly slow down your app's load time. Ensure that all media files are compressed and tailored to the appropriate size for various devices.
By honing in on these performance issues early in the development cycle, you can smooth out the ride for users, providing them with a faster, more responsive experience.
Remember, a well-optimized app not only stands out in the marketplace but also ensures a loyal user base that keeps coming back.
Publishing Your Flutter App
Publishing a Flutter app isn't just a formality; it's the culmination of hard work, creativity, and technical skill. For developers, understanding the publishing process is as crucial as building the app itself. This section sheds light on key factors surrounding the release of your application. By appreciating these elements, developers can ensure their app reaches the intended audience. This includes regulatory aspects, user expectations, and the competitive landscape. Moreover, discovering effective strategies for app publication can significantly impact downloads and user engagement.
Preparing for Release
Before pushing the proverbial button, it's essential to carry out a thorough review of your app. This phase prepares your application for the outside world, guaranteeing everything runs smoothly. Key activities include:
- Testing: Ensure all functionalities work as intended. Explore edge cases and potential bugs.
- Review App Store Guidelines: Familiarize yourself with the submission criteria, such as UX consistency, data handling, and content restrictions. Different platforms have unique rules!
- Optimize for Performance: It's not just about functionality; app speed and responsiveness matter. Use tools to analyze performance, fix bottlenecks, and enhance the user experience.
An effective pre-release checklist helps avoid the pitfalls that come with launching an app without preparation. It's the difference between a glitchy app and a polished version that reflects professionalism.
Publishing on Google Play Store
Publishing on the Google Play Store opens up vast potential as itās one of the largest mobile app marketplaces. Itās a straightforward process, but a few steps demand attention:
- Create a Developer Account: Registering requires a one-time fee, so have your credentials ready.
- Compile Your Appās Release Version: This differs from your development version. Make sure to strip out debug code and use a release keystore for signing.
- Write a Compelling App Description: This can make or break your appās visibility. Incorporate relevant keywords naturally, while ensuring itās fluid and engaging for potential users.
- Prepare Graphics for the Store Listing: Screenshots and promotional graphics should showcase your appās features effectively; think of these as the first impression.
- Submit and Wait: After everything is in order, submit your app for review. Monitor your emails closely for feedback from Google.
Publishing on Google Play doesn't just mean dropping your app in a bucket; it's about ensuring your app is distinguished among millions.
Distributing Through Other Platforms
While the Google Play Store is a giant, it's wise to consider alternative distribution strategies to capture different audiences. Some options include:
- Apple App Store: If your app is platform-agnostic, expanding to iOS can maximize reach.
- Amazon Appstore: Particularly relevant for Kindle Fire devices, this store can attract a unique user base.
- Direct Distribution: Hosting APK files on your website allows for direct engagement with users and caters to niche markets. However, this comes with overhead in terms of security and ensuring updates reach users.
Each platform has its hurdles, but the potential benefits often outweigh these challenges. Ensure you tailor your distribution strategies based on your target demographic, as user preferences can vary significantly across platforms.
"In the world of apps, visibility is critical. Itās not just about getting your app out there, but making sure it stands out in a crowded field."
Post-Publication Maintenance
Once your Flutter app has been released into the wild, the work doesnāt stop there. Post-publication maintenance is crucial for ensuring your app continues to meet users' needs and adapts to changing technologies. Ignoring this aspect can lead to user dissatisfaction and ultimately, a decline in app usage. Here's a deep dive into what post-publication maintenance entails and why it matters.
Updating Your App
Keeping your app updated is more than just adding new features. It encompasses correcting bugs, enhancing performance, and ensuring compatibility with the latest versions of operating systems and dependencies. Periodic updates serve multiple purposes:
- User Satisfaction: Users expect apps to run smoothly and efficiently. Fixing known issues can enhance their experience dramatically.
- Security Enhancements: As vulnerabilities are discovered, timely updates ensure that your app doesn't become an easy target for malicious attacks.
- Feature Expansion: Regularly introducing new features keeps your app fresh and interesting, prompting users to return. This strategy works to minimize churn.
Focusing on updates can sometimes feel like plugging holes in a leaking boat, but itās a necessary task. A methodical approach to versioning your app, such as Semantic Versioning, can help manage these updates effectively.
Monitoring App Performance
Once your app is live, monitoring its performance is just as vital as the coding and designing phase. Not only does it help catch and resolve issues early, but it also provides insights into areas that can be improved. Here are the key metrics to keep an eye on:
- Crash Analytics: Use tools like Firebase Crashlytics to get detailed reports on crashes. This helps prioritize fixes for the most critical issues.
- User Engagement Metrics: Analyze how often users are engaging with your app, which features are popular, and where users are dropping off. This data is invaluable for future updates or features.
- Load Times: Slow load times can frustrate users. Tools like Google Analytics provide insights on how quickly your app loads in various environments.
"An app's success isn't defined just by its launch; it's sustained through continuous care and improvement."
By regularly monitoring these aspects, you can make informed decisions, ensuring your app remains competitive and appealing in an ever-evolving digital landscape.
Ultimately, post-publication maintenance is not a mere afterthought but a critical component of a successful app lifecycle. Taking proactive steps to maintain and monitor your Flutter application can influence not only its current performance but also its future growth and relevance.
Advanced Flutter Features
In the fast-paced world of mobile app development, staying ahead of the curve is crucial. Advanced Flutter features offer a treasure trove of possibilities that can significantly enhance your application's usability and aesthetic value. Delving into what these features bring can often mean the difference between a mediocre app and one that dazzles users and meets their needs seamlessly. The focus here will revolve around three primary areas: animations, widget customization, and responsive design. Each has its own set of benefits and considerations worth exploring.
Using Flutter's Animation Framework
Animations in mobile applications arenāt just about aesthetics; they can also play a pivotal role in user experience. With Flutter's animation framework, developers can create rich, delightful experiences that react intuitively to user actions. Not only do animations enhance the user interface, but they also provide visual cues that guide users through the app. For instance, imagine an animation that gently shrinks a button when pressed, giving instant feedback that the action has been registered.
Flutter makes it straightforward to implement animations using its built-in classes like and . These tools enable developers to design complex animations that sync beautifully with their app's overall flow and design language.
Here are a few tips for using Flutter's animation framework effectively:
- Keep it Subtle: Overdoing animations can lead to distractions. Aim for fluid movements that enhance rather than detract from the experience.
- Utilize Curves: Experiment with different to make your animations feel more natural. For example, easing in and out gives a soft touch to transitions.
- Test on Devices: Always verify that animations run smoothly on various devices to ensure a consistent user experience.
"Good design is obvious. Great design is transparent." ā Joe Sparano
Customizing Widgets for Enhanced UI
Widgets are the building blocks of any Flutter application. Customizing these widgets allows developers to forge a unique identity for their app. This step can result in heightened user engagement since tailor-made widgets can resonate more with your audience. For instance, a banking app could use custom widgets that visually align with finances, such as a pie chart widget representing expenditure categories.
When customizing widgets, consider the following:
- Reshape Common Widgets: Flutter provides an array of widgets that can be tailored to fit your vision. Adjust elements like color, size, or layout properties.
- Composition Over Inheritance: Favor compositional patterns that utilize existing widgets to create new functionalities rather than heavily extending base classes.
- Manage State Wisely: Consider how state management can affect widget customization, ensuring a thoughtful flow of data.
Implementing Responsive Design
In an era where users access apps across various devices, responsive design is non-negotiable. Flutter's inherent capability of adaptive layouts is a boon for developers aiming to provide a consistent experience, whether on a smartphone, tablet, or even a web interface. Responsive design in Flutter involves understanding a variety of device characteristics and ensuring your UI adjusts accordingly.
Here are some pointers for effective responsive design:
- Use MediaQuery: This helps in getting the device's dimensions and making layout decisions based on screen size.
- Flexible Layouts: Utilize and widgets to adapt your layout elements dynamically based on available space.
- Testing: Always preview your layout on different devices and orientations to ensure consistency and usability.
By diving into these advanced features of Flutter, developers can not just enhance the aesthetic appeal of their applications but also significantly improve user interactions and overall satisfaction. The seamless integration of animations, widget customization, and responsive design can lead to a more engaging and well-rounded user experience, showcasing the true power of Flutter in mobile app development.
Culmination
Bringing a Flutter application to life is a journey filled with numerous learning curves and revelations. In the ever-evolving world of mobile app development, Flutter has carved out a niche for itself, offering developers a rapid and flexible framework that simplifies the complexities often associated with cross-platform development.
Reflecting on the Flutter Journey
As we close the chapter on this article, it's essential to reflect on what the Flutter journey has entailed. From the very first lines of code to the final design touches, the process of building a Flutter app is as rewarding as it is challenging. Developers often find themselves grappling with new concepts, such as widgets and state management, but each challenge comes with its own set of satisfaction upon solving it.
Understanding how Flutterās architecture operates helps to demystify the development process. The frameworkās use of the Dart language, alongside its widget-centric design, encourages a fresh perspective on how applications can be structured. Many programmers have reported substantial increases in productivity, as Flutter minimizes the need for repetitive code while maximizing the UI capabilities.
Moreover, Flutter fosters a community thatās rich with resources and support. From forums on Reddit to documentation pages on Wikipedia) and insightful exchanges on social platforms like Facebook, the Flutter developer community continuously shares knowledge that can aid beginners and seasoned developers alike. This collective wisdom represents an invaluable asset that enhances the learning experience as one navigates through various challenges.
Future of Flutter Development
Looking forward, the trajectory of Flutter development shows no signs of stagnation. The ongoing enhancements in performance and device compatibility reflect the framework's adaptability in an ever-changing tech landscape. As mobile-first approaches gain more traction, Flutter's ability to deliver engaging user experiences will stand out even more. One can anticipate improvements in already existing features, such as the animation framework and comprehensive widget library that only seem to grow richer.
As Flutter continues to expand its reach, the demand for skilled developers in this domain is bound to increase. The potential for building versatile applications not only for mobile but also for the web and desktop is a testament to the frameworkās agility. Developers would do well to stay attuned to Flutter's updates and community trends, as they present opportunities to leverage new tools and methodologies that could further enhance development efficiency.
In summary, engaging with Flutter isnāt merely about writing code; itās about being part of a vibrant ecosystem that encourages exploration and innovation. The path ahead is promising, both for newcomers and those looking to hone their Flutter expertise, positioning themselves at the forefront of mobile application development.
"The future belongs to those who prepare for it today." ā Malcolm X
This sentiment resonates strongly in the realm of Flutter development, as every new project and upgrade takes you one step closer to mastering this dynamic framework.