Mastering the For Loop Syntax in R Programming
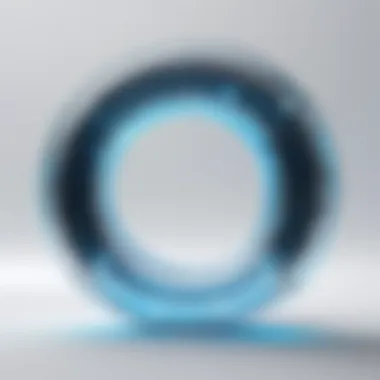
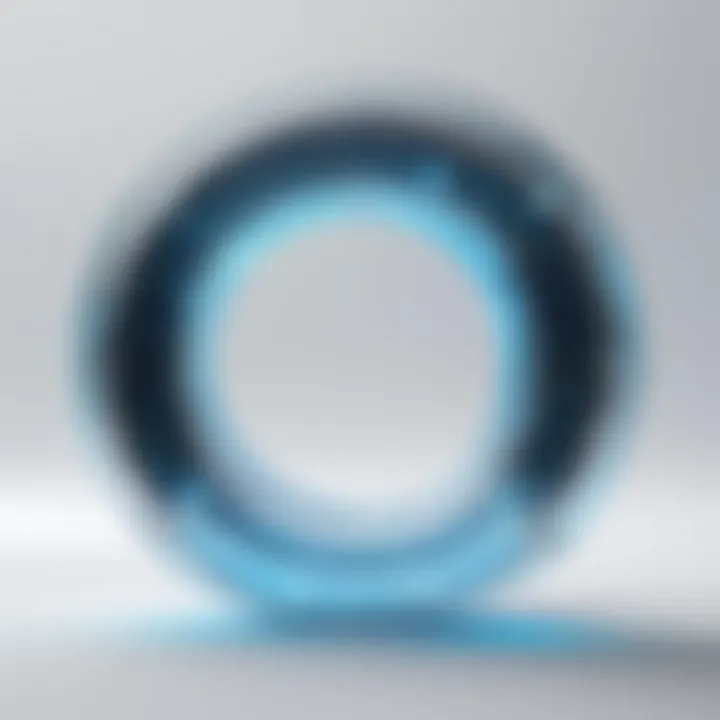
Overview of Topic
Understanding the mechanics and functionality of the for loop in R is crucial for anyone delving into the world of programming within this statistical environment. A for loop allows for the repetition of a block of code multiple times, making it invaluable for automating tasks, processing datasets, and efficiently handling repetitive calculations.
The significance of mastering this syntax cannot be overstated, especially in the tech industry where data analysis and programming intersect. As companies increasingly rely on data-driven decision-making, professionals with robust programming skills, especially in R, hold a competitive edge.
Historically, the concept of looping in programming languages traces back to the early days of computing. R, originally developed by Ross Ihaka and Robert Gentleman at the University of Auckland, has evolved from its inception in the 1990s into one of the most widely used languages for statistical computing. Its for loop structure, while straightforward, offers a gateway to more complex programming logic and data manipulation techniques.
Fundamentals Explained
To grasp the for loop in R, one must first become acquainted with certain core principles:
- Iteration: This is the process of repeating a set of instructions until a certain condition is met.
- Variable: A variable is a storage location in the program that holds values that can change; in a for loop, the iteration variable takes on different values from a specified vector.
Key Terminology and Definitions
- Initialization: This refers to setting up the loop with the starting variable.
- Condition: This determines when the loop should continue to run. For a for loop, it typically iterates over the entire length of a vector.
- Body of Loop: The block of code executed during each iteration.
Basic Concepts and Foundational Knowledge
At its core, a for loop in R is structured as follows:
Here, the takes on the value of each element in a specified sequence during its iterations. Understanding this structure clears the path to employing loops in various applications.
Practical Applications and Examples
Exploring practical instances where a for loop shines can illuminate its utility:
- Data Transformation: Suppose you wish to square each element in a numeric vector. A simple for loop can iterate through the vector elements, allowing efficient modifications.
- Summarizing Data: When working with data frames, a for loop can aggregate values or generate summary statistics based on specific conditions.
Real-World Case Studies and Applications
In a marketing context, data scientists often use for loops to analyze customer data, allowing them to perform tasks such as segmentation and behavioral analysis quickly. This can lead to more tailored marketing approaches, thereby improving customer engagement and retention.
Advanced Topics and Latest Trends
As programming advances, so too does the need for more efficient handling of loops. More complex substitutes like the apply() family functions in R are increasingly popular among seasoned programmers. These functions allow for similar iterations but offer improved performance for larger datasets.
Future Prospects and Upcoming Trends
In the ever-evolving landscape of data science, the adoption of R in conjunction with machine learning frameworks signals a trend toward integrating looping mechanisms with advanced analytics. Understanding for loops prepares programmers for a deeper dive into these increasingly sophisticated methodologies.
Tips and Resources for Further Learning
For those eager to expand their knowledge of for loops and R, several resources prove invaluable:
- Books: Look for R for Data Science by Hadley Wickham for foundational insights and practical applications.
- Courses: Platforms like edX or Coursera offer courses tailored to R programming.
- Online Forums: Engaging with communities on Reddit's r/Rlanguage can provide real-world insights and troubleshooting tips.
In this rich tapestry of learning, the for loop serves as a critical thread, weaving together crucial programming skills and practical data analysis capabilities. As you navigate through advancing your proficiency in R, remember to embrace the little quirks and nuances of loops, for they often hold the key to efficient coding.
Preface to R Programming
Exploring R programming sets a solid foundation for understanding data analysis and statistical computing. This language, with its unique strengths and functionalities, serves as a crucial tool for statisticians, data scientists, and researchers alike. R's ability to handle large datasets and its comprehensive ecosystem of packages make it an indispensable asset for anyone looking to maximize their analytical skills.
Overview of R as a Programming Language
R is specifically designed for statistical analysis and visualizing data. This open-source programming language has rapidly gained traction among professionals in diverse fields, from academia to industry. Not only does R boast a wide range of built-in functions for mathematical computations, but its flexibility allows users to craft customized functions tailored to their specific needs.
Some key features of R include:

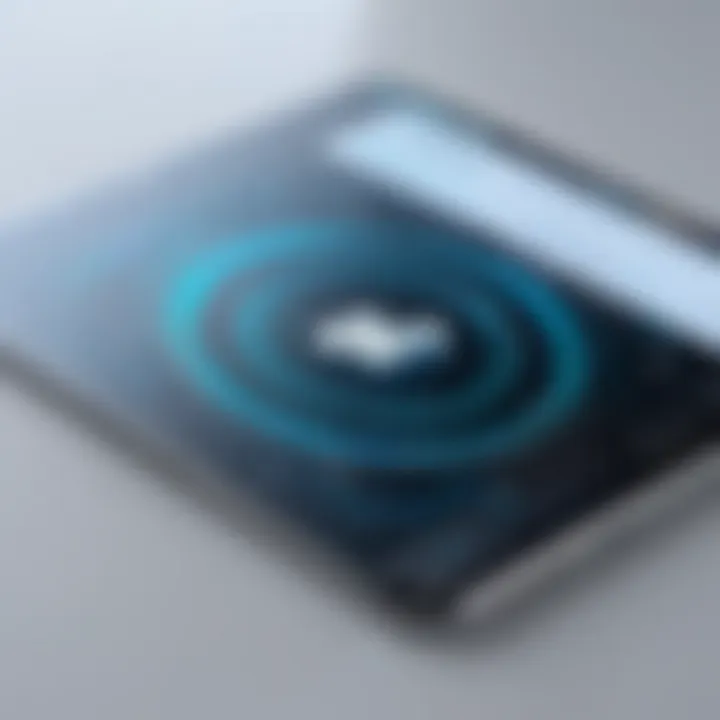
- Rich Package Ecosystem: R has thousands of packages available through CRAN (Comprehensive R Archive Network), covering a plethora of functionalities from data manipulation to machine learning.
- Data Visualization: With libraries like ggplot2 and lattice, R excels at creating informative and aesthetically pleasing graphics. Visual representation of data is crucial in deriving insights effectively.
- Community Support: A thriving community supports R, ensuring that learners and experts alike can easily access help and resources for problem-solving.
R is not merely a tool; it fosters an environment conducive to learning and innovation. Understanding its capabilities can significantly enhance a reader's programming prowess, especially when it comes to statistical programming practices.
Why Use For Loops in R?
For loops are a fundamental aspect of programming in R, allowing for efficient and streamlined iteration through elements in a dataset. These loops provide a structured means to automate repetitive tasks, which can save both time and effort, particularly when working with larger datasets.
Key benefits of using for loops in R:
- Efficiency: By automating repetitive calculations, for loops can drastically reduce the time taken for tasks.
- Flexibility: They can handle variable-length data, making them suitable for a variety of use cases in data analysis.
- Enhanced Readability: Code readability improves when loops structure tasks systematically, making it easier for others (or yourself in the future) to follow.
However, while utilizing for loops can enhance productivity, it is vital to understand how to implement them effectively. Missteps can lead to logical errors that may compromise data integrity or processing time.
"Understanding how to navigate for loops can be the difference between an efficient workflow and a tangled mess of code."
Through this exploration of R programming, we will dive deeper into the syntax and practical applications of for loops. By mastering these concepts, you can enhance your programming agility and elevate your analytical capabilities.
Basic Syntax of the For Loop
The basic structure of a for loop in R is not just a matter of syntax; it is fundamental in effectively controlling the flow of execution within your scripts. When you delve into the intricacies of this structure, you’ll notice how it allows for repeated execution of a block of code, which is incredibly useful for tasks like data processing or simulations. Understanding the basic syntax becomes essential for anyone looking to maximize their efficiency in R programming. The well-defined nature of a for loop can prevent errors and enhance the readability of your code, thus making it a popular choice among programmers.
Understanding the Structure
At its core, a for loop in R has a straightforward structure that consists of three primary parts: the initialization, the condition, and the increment. This simplicity is what makes it accessible for learners while also being powerful enough for seasoned coders to implement complex logic. The body of the loop, which contains the code that will be executed repeatedly, is placed within curly braces, ensuring that clarity is maintained. A clear understanding of how these components interact can profoundly impact code performance and effectiveness.
Components of a For Loop
Initialization
Initialization is where the magic starts. In R, this step usually involves creating a variable that serves as the loop counter. For instance, initializes the variable to take values from 1 to 10. The importance of this initial setup should not be underestimated; it lays the groundwork for what the loop will execute. The beauty of initialization lies in its flexibility: you can set counters to any starting point that makes sense for your logic. Yet, users should remain vigilant, as initializing incorrectly can lead to endless loops or missed iterations—an experience no coder wants.
Condition
Moving on to the condition, this part directs the loop on when to stop executing. In a typical for loop, this is encoded implicitly within the framework of the initialization itself, such as , which indicates the loop should continue until exceeds 10. The efficiency of conditions in for loops is significant. A well-crafted condition ensures that the loop executes just the right number of times without venturing into unnecessary iterations. The simplicity of this approach makes it an appealing choice, allowing users to focus on algorithmic efficiency without getting bogged down in complex logic.
Increment
Finally, the increment takes place automatically at the end of each loop iteration. By default, R increments the counter by one, allowing for a straightforward progression through the defined range. However, more advanced users can configure this step, adjusting it to larger increments or even decrementing if required. The unique advantage of having this control is the ability to adapt the loop's behavior to specific needs, such as skipping certain values for particular calculations. When used judiciously, increment strategies can foster enhanced computational performance without complicating code readability.
Tip: Always test for loops with different initializations and conditions to get a feel for how they operate in various scenarios. This hands-on practice can solidify your understanding and readiness to tackle real data problems.
Understanding these components—the initialization, condition, and increment—creates a solid foundation for mastering for loops in R. These components are not just standalone features but interwoven elements that, when employed wisely, contribute to overall coding success.
Examples of For Loops in R
When delving into R programming, grasping the various examples of for loops is a cornerstone that every learner should focus on. For loops are not just another piece of code; they are powerful constructs that enable programmers to simplify repetitive tasks. By understanding how to implement these loops effectively, you gain a better handle on managing data more efficiently. This section will explore a few key examples to showcase the versatility and functionality of for loops within R.
Simple Iteration Example
At its heart, a for loop in R serves the purpose of iterating over a sequence of values. Here’s a simple demonstration:
In this snippet, the loop cycles through the integers from 1 to 5. Each iteration, it prints the current value of . This straightforward example opens the door to understanding how to automate repetitive tasks in programming. Imagine needing to process a list of numbers or apply a function to a dataset—loops allow you to avoid redundancy and write cleaner code.
Using For Loops with Vectors
Vectors are fundamental data structures in R, and utilizing for loops alongside them allows for efficient data manipulation. Consider this example:
In this case, the loop iterates through each element in the vector. For each iteration, it calculates the square of the number and prints it to the console. This showcases the loop’s capability to interact seamlessly with R’s data structures, making it a crucial concept for anyone looking to perform operations on datasets, be it for statistical purposes or data analysis.
Nested For Loops
Nested for loops might sound complex, but they are simply loops within loops, useful for dealing with multi-dimensional data. Take a look at this example:
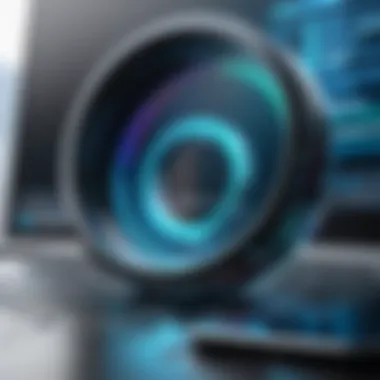
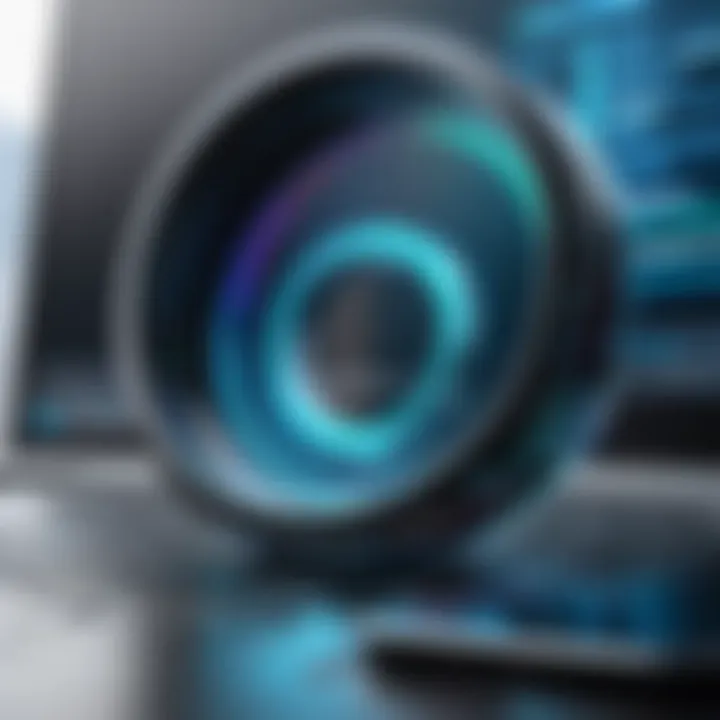
Here, we have a 3x3 matrix, and the outer loop iterates over the rows while the inner loop goes through the columns. Each element of the matrix is printed in order. This example is vital when your data is structured in rows and columns, allowing for operations that require fine control of each element. The combination of loops helps to facilitate operations like matrix manipulation, which holds extensive importance in statistical analysis.
In summary, examples of for loops in R provide learners with practical insights into coding efficiently. They illustrate how to work with iterations, vectors, and even complex data structures like matrices. Grasping these examples not only enhances your programming skills but also empowers you to tackle real-world data challenges with ease.
Advanced For Loop Techniques
In any programming language, mastering advanced techniques can elevate one’s capabilities from simply getting the job done to achieving it with finesse and efficiency. In the realm of R programming, for loops are no exception. These loops become far more powerful when one delves into the intricacies of combining them with other programming constructs or optimizing their execution. By understanding these advanced techniques, programmers can unlock new levels of performance and adaptability in their code.
Combining For Loops with Conditional Statements
When you pair for loops with conditional statements, you can introduce flexibility into your iterations. This combination allows your loop to make decisions on-the-fly, which can save time and resources. Here's a simple example to illustrate:
In this snippet, the loop checks if each number in the vector is even or odd, displaying results accordingly. Such capabilities empower developers to filter data, create meaningful summaries, or dictate specific actions based on conditions. In a nutshell, combining conditionals with for loops can yield cleaner code and higher readability, allowing future programmers to understand logic without second-guessing.
Performance Considerations
Performance is a critical factor every programmer should keep in mind, especially when working with loops over large datasets. As the size of your data grows, inefficiencies within a loop can lead to significantly longer processing times. Consider the following aspects to improve loop performance in R:
- Vectorization: Whenever possible, try to replace loops with vectorized operations. R is optimized for vector calculations, and leveraging this feature can yield considerable speed boosts.
- Preallocation: If you're building a vector within a loop, consider preallocating the vector. This means defining the vector size before the loop runs rather than growing it during each iteration. For example:
result - numeric(length(numbers))# Preallocate for (i in seq_along(numbers)) result[i] - numbers[i]^2# Store squared values
In this situation, the first element is excluded from the sum due to starting the loop at the second element. A logical error like this may go unnoticed, leading to confusion regarding the returned sum. To avoid such mistakes, it’s essential to pay close attention to indexing and ensure the loop iterates over the correct set of elements.
Performance Pitfalls
Apart from logical errors, performance pitfalls can severely diminish the efficiency of a for loop, making the code sluggish and cumbersome. One common performance issue occurs when users modify data structures within the loop. For instance, if you’re appending elements to a vector inside a loop, it leads to unnecessary reallocations of memory during each iteration.
Consider the following:
In the example above, each iteration requires R to allocate more memory for the growing vector, ultimately slowing down execution significantly. A more efficient method is to preallocate space for the vector before the loop starts:
By defining the size of beforehand, the performance of the loop improves markedly, eliminating the memory reassignment overhead. Recognizing this and similar performance issues is crucial for writing clean, effective R code.
To shine in coding, knowing what not to do can often be as valuable as understanding the techniques themselves.
Debugging For Loops in R
Debugging is a critical skill in programming, and it holds particularly true when working with for loops in R. When you're knee-deep in looping through data, even minor errors can cascade into significant issues, making it hard to pin down where things went wrong. Ill-timed mistakes often result in infinite loops or unintended behaviors that can throw off your data analysis. An effective debugging strategy not only saves time but also enhances your understanding of R's workings, which is vital for anyone serious about programming.
Using print() for Tracking Execution
One of the simplest yet effective methods for debugging a for loop is to sprinkle in some statements. This technique can provide valuable insights into the flow and status of your loops. By outputting variable values or loop progress at different stages, you can gain clarity on how your data is being manipulated. For instance, consider a loop that sums up numbers:
Here, the statements offer a running commentary, allowing the programmer to follow along with the computation. It might feel like using a flashlight in a dark room; you can see where you're going instead of stumbling around. However, bear in mind that too many statements can lead to cluttered outputs, which could be distracting. It's about finding a balance.
Employing Debugging Tools
Beyond simple print statements, R has some robust tools specifically designed for debugging complex loops. Functions such as , , and allow you to step into your code and examine its behavior during execution. When combined with your loop, these tools elevate your debugging game.
For instance, if you want to debug a function that includes a for loop, the function activates the debugger within the R environment:
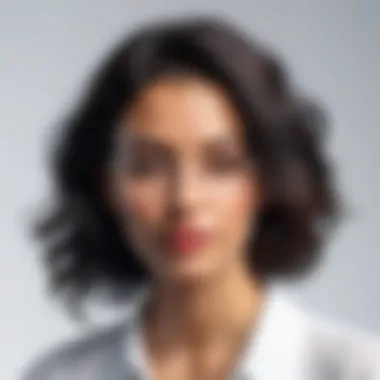
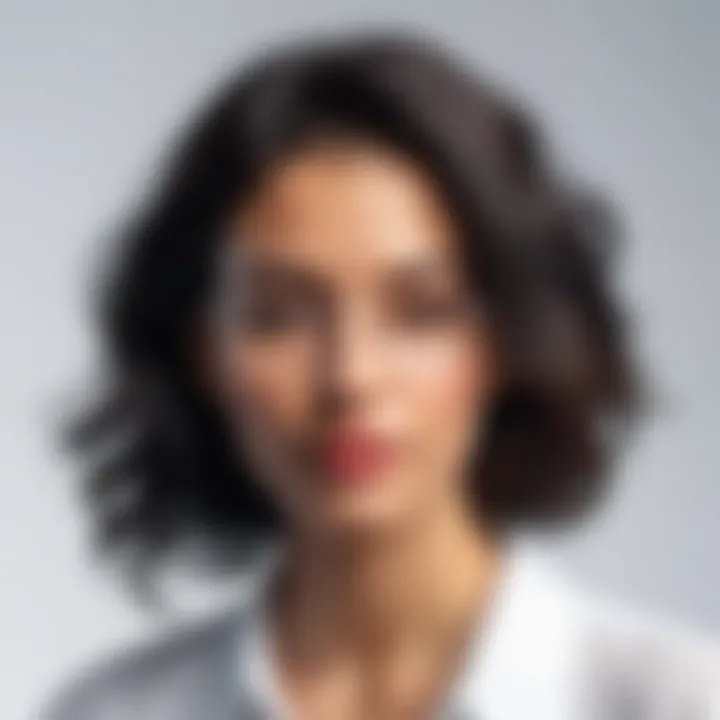
Here, you'll be able to inspect variables at every line before moving on—essentially, it's like watching a movie in slow motion to catch every detail. Alternatively, using the function within your for loop pauses execution at that point, giving you an opportunity to explore the environment and variable values on-the-fly. This approach can significantly reduce the time spent on identifying nuanced bugs.
In summary, debugging for loops is not merely about finding and fixing errors; it’s about refining your comprehension of how R operates under the hood. By blending simple techniques like with powerful debugging tools, you can navigate the complexities of programming and enhance your overall coding skills.
"Debugging is like being the detective in a crime movie where you are also the murderer." - Volker Borges
By investing time into mastering these tools and techniques, you become well-equipped to tackle the challenges that come with programming in R.
Best Practices for Writing For Loops
When delving into the realm of R programming, particularly when dealing with for loops, understanding best practices can significantly enhance coding experiences. Why? Because well-structured code not only operates smoothly but also remains maintainable over time, allowing others to understand it without breaking a sweat. The overarching goal in this section is to instill good habits that optimize not just functionality but also readability and efficiency within your scripts. Here are some key elements to consider:
- Maintainability: Code that is easy to read and understand is also easier to maintain. This becomes vital when you or someone else revisits the code later.
- Debugging Ease: If your loops are easy to follow, debugging becomes less like pulling teeth. Clear logic reduces time spent chasing down elusive bugs.
- Performance Optimization: While for loops can be straightforward, inefficient implementation may lead to bottlenecks in larger datasets or more complex operations.
Adhering to best practices makes for loops optimal and more intuitive.
Code Readability
Code readability is like a well-lit path in a dense forest—benign and inviting. No one wants to work through a labyrinthine mess, especially when multiple loops are at play. Start by naming your variables with clarity in mind. Instead of using vague references like or , consider something descriptive, such as or .
Another aspect worth mentioning is consistent indentation. Keep your for loops structured with proper indentation; this visually represents the hierarchy of your code logic. Here’s a small example:
Note how each line inside the loop is indented. It’s simple, but such details contribute to better readability. Consider adding comments in your code. They offer quick insights into the code's purpose. For instance:
Efficiency Tips
To make your loops fly faster than the speed of light, adopting efficiency tips is paramount. As the saying goes, “work smarter, not harder.” One approach is to minimize the work done inside the loop.
For example, if you have computations that do not change with each iteration, consider moving them outside:
Here, is calculated just once, not ten times.
Whenever possible, look into vectorized operations. R is optimized for operations on whole vectors instead of single elements, which can lead to significant performance gains. Here’s what a vectorized version would look like:
Lastly, avoid modifying data structures within a loop, if you can. This includes growing data frame or list objects dynamically, as R's memory allocation for these types can lead to slowdowns. Instead, preallocate your vectors or matrices whenever feasible.
By blending readability and efficiency, your for loops can be a joy to work with, eliminating headaches for you and others. A touch of clarity and a dash of performance consideration go a long way in making your R code robust and reliable.
"In the world of programming, clarity and efficiency aren’t just goals; they are a necessity."
Implement these best practices in your coding ventures, and see how your projects not only gain in speed but also in transparency.
Ending
In wrapping up our discussion on the syntax and practical applications of for loops in R, it's crucial to underscore their critical role in navigating the complexities of programming. This article has guided readers through the essential structure of for loops, showcasing their versatility and utility in real-world scenarios. Understanding how to effectively implement and utilize for loops can significantly enhance a programmer's ability to manipulate data and streamline tasks.
Recap of For Loop Syntax and Use Cases
To recap, the for loop's basic syntax consists of three main components: the initialization, the condition, and the increment. This structured layout allows you to iterate over elements in a vector or list and execute a block of code repeatedly.
The applications of for loops in R are vast and varied. They can be used for:
- Data processing: Manipulating datasets efficiently by iterating through rows or columns.
- Simulations: Running simulations for statistical models where repeated trials are required.
- Automation: Automating repetitive tasks, thus saving time and reducing the risk of human error.
For instance, imagine you have a massive dataset in R, and you want to perform the same calculation on each row. Instead of manually coding the operation for each element, you can simply set up a for loop to handle that job in one fell swoop.
"A well-placed for loop can save hours of manual coding, transforming tedious tasks into swift, automated workflows."
Future Learning Paths in R
Looking ahead, there are numerous pathways for eager learners to broaden their expertise in R. As you feel more comfortable with for loops, consider diving deeper into more advanced programming concepts that R offers:
- Vectorization: Understand how to leverage R's inherent abilities with vectors for more efficient computations. This can often replace for loops in many cases, yielding faster code.
- Functional Programming: Explore functions like , , and their family to improve readability and sometimes performance.
- Machine Learning Packages: Experiment with packages like or to apply your programming skills in contexts that could analyze big data.
Each of these learning avenues can significantly enhance your programming fluency, equipping you with practical tools that can be applied in various fields, from statistics to data science.