Mastering GCC Compiler Commands for All Levels
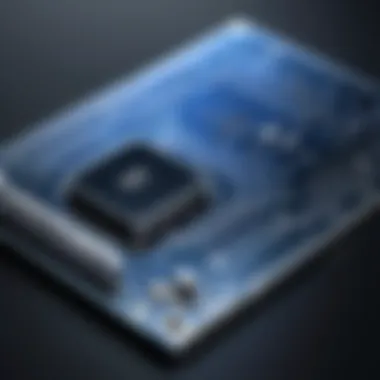
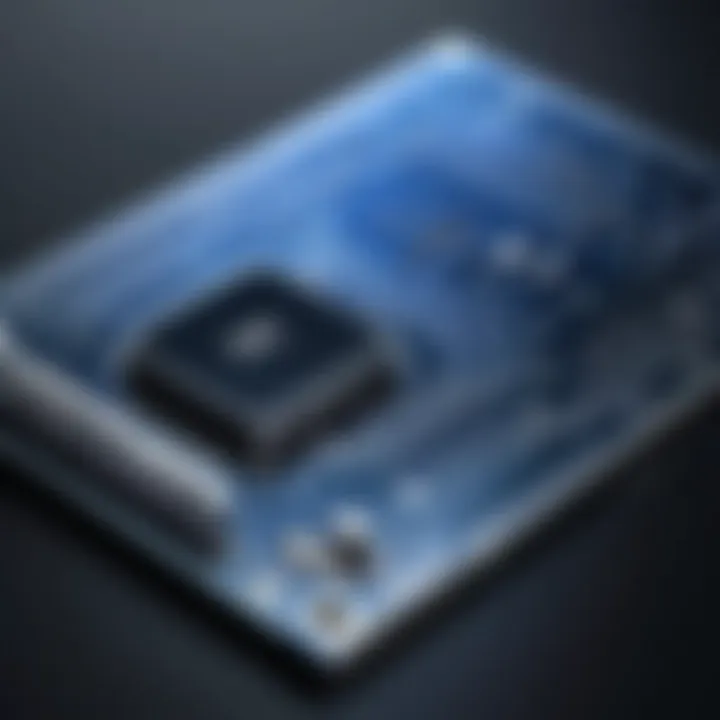
Overview of Topic
The GNU Compiler Collection, commonly referred to as GCC, serves as a crucial tool for software development across various programming languages. GCC is not merely a compiler; it is a versatile suite that supports languages like C, C++, and Fortran, among others. The significance of GCC in the tech industry cannot be overstated. Many of today’s most robust systems and applications are built upon code that has been compiled using this tool.
Historically, GCC emerged in the late 1980s as a part of the GNU Project. It aimed to provide an open-source compiler for free software development. Over the decades, its evolution has cemented its place as a standard compiler in many Unix-like operating systems, reinforcing its relevance in both academic and professional settings.
Fundamentals Explained
To effectively harness the power of GCC, understanding its core principles is paramount. At its essence, GCC translates high-level programming languages into machine code that computers can execute. Key terminology includes:
- Compiler: A program that converts code written in one programming language into another.
- Source Code: The human-readable instructions that developers write.
- Executable: The machine-readable format resulting from the compilation process.
Foundational knowledge includes familiarity with basic commands and how to structure simple compilation processes. This understanding is imperative for both beginners and seasoned developers alike.
Practical Applications and Examples
In practical terms, GCC is widely used to build software across various platforms. One prominent case study is the Linux operating system, which relies heavily on GCC for its compilation needs. Here are some practical applications and examples:
- Building a Simple C Program
To compile a simple C program named , you would execute the following command:This command compiles and outputs an executable file named . - Debugging with GDB
GCC integrates seamlessly with GDB, the GNU Debugger, allowing developers to debug programs effectively. By adding the flag during compilation, you enable debugging: - Using Optimization Flags
Optimization flags can drastically affect performance. For example, the flag activates various optimization techniques intended to enhance the execution speed:
It is important to note that optimization can result in longer compile times, so it must be used judiciously.
Advanced Topics and Latest Trends
With the rise of new programming paradigms and ever-evolving technologies, GCC continues to adapt. Recent trends worth noting include support for new programming standards, such as C17 and C++20. Additionally, the emergence of just-in-time compilation techniques is a notable development, enhancing performance during runtime.
As programming languages diversify, GCC's expansion in supporting languages is also critical. Understanding these advanced techniques is essential for professionals aiming to stay ahead in a competitive field.
Tips and Resources for Further Learning
For those interested in deepening their understanding of GCC, various resources can be helpful:
- Books: "The Definitive C Book Guide and List" on Goodreads provides excellent recommendations.
- Online Courses: Platforms like Coursera and Udacity offer courses specifically tailored to GCC and programming languages.
- Forums and Communities: Engaging with communities on platforms like Reddit can provide valuable insights and peer support.
Preamble to GCC Compiler
The GCC Compiler, short for GNU Compiler Collection, is a powerful tool widely used among developers. Its importance cannot be overstated. As a free and open-source compiler system, GCC supports various programming languages, including C, C++, and Fortran. This versatility makes it an essential component in modern software development environments. Many developers rely on GCC for compiling code, enabling them to run programs on different architectures. This article aims to delve deeply into GCC compiler commands, it lists multiple commands tailored for a range of users, from novices to expert programmers.
What is GCC?
GCC stands for GNU Compiler Collection. It is a set of compilers developed by the GNU Project. The primary purpose of GCC is to convert source code written in high-level programming languages into machine code. This machine code allows programs to be executed by computers. Alexei Panshin has said, "It is the job of the compiler to translate human-readable code into a format the machine can understand."
GCC supports several programming languages like C, C++, Objective-C, Fortran, Ada, and more. It is often praised for its performance and optimization capabilities. Developers can use GCC in various operating systems, including Linux, macOS, and Windows, thus making it flexible and adaptable for different needs.
History and Evolution of GCC
GCC was first released in 1987. Initially, it only supported the C programming language. However, over the years, it has evolved significantly. The introduction of support for C++ in 1990 marked a key milestone in its development. Later versions expanded its capabilities further, accommodating languages such as Ada and Fortran.
This evolution is a response to growing software development needs. Each iteration of GCC improved upon the last, continuing to adapt to new language standards and programming paradigms. Today, GCC is not just a compiler; it’s a programming tool essential for many projects, ranging from small scripts to large-scale applications.
Importance in Modern Development
In today's fast-paced software landscape, GCC plays a crucial role in the development cycle. Programmers depend on its robustness and efficiency. Some key reasons for its importance include:
- Cross-Platform Support: GCC works across multiple platforms, facilitating portability.
- Open Source: Being part of the GNU Project ensures that GCC is continually improved by a global community.
- Comprehensive Language Support: It accommodates a variety of programming languages, making it a versatile choice for developers.
- Optimization Capabilities: GCC offers various optimization flags that improve program performance without requiring extensive modifications.
In summary, GCC is more than a compiler; it is a fundamental tool that shapes the way software is developed and deployed. Its history, adaptability, and continued enhancement ensure it remains relevant, making it a topic of significant interest for anyone engaged in programming.
Getting Started with GCC
Understanding how to effectively use the GCC compiler is essential for anyone venturing into programming or software development. This section provides foundational knowledge necessary for harnessing the power of GCC. It covers important aspects such as installation procedures on various platforms and verifying the installation. These elements are crucial for ensuring that users can compile their code swiftly and accurately.
Installing GCC on Different Platforms
Installing GCC can vary based on operating systems. Below are distilled steps for common platforms:
- Linux: Most Linux distributions come with GCC already installed. However, if it’s not available, you can install it using your package manager. For example, on Ubuntu, run:
- macOS: The recommended way to install GCC on macOS is through Homebrew. If you don’t have Homebrew, install it from the official website. Then, to install GCC, use:
- Windows: On Windows, the simplest approach to installing GCC is to use MinGW or Cygwin. Both provide a Unix-like environment. For MinGW, follow these instructions:
- Download MinGW from MinGW.org.
- During installation, select the and packages.
- Add the MinGW directory to your system PATH.
This variety accommodates different user needs, recognizing that not all developers use the same operating platform.
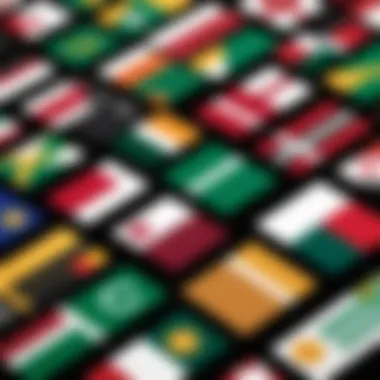
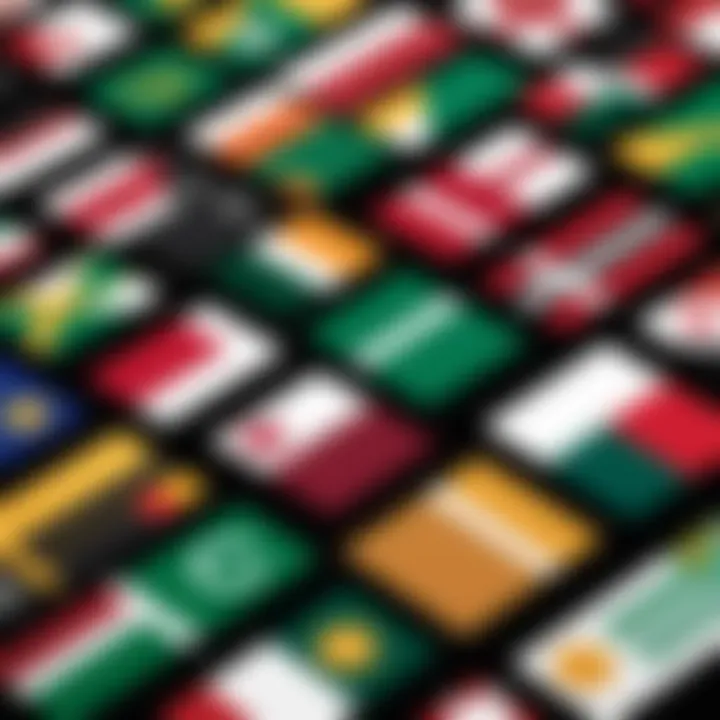
Verifying Your GCC Installation
Once installed, it’s necessary to verify that GCC is operational. You can confirm the installation by checking the version. This helps to ensure that everything was set up correctly and that you are prepared to compile code efficiently. To verify, simply open your terminal or command prompt and type:
This command should return the version of GCC that is installed. If you receive a message indicating that is not recognized, check your installation path or revisit the installation steps.
Proper verification not only establishes that the initial setup was successful but also reassures that you are equipped with the latest features offered by GCC.
Taking these steps to install and verify GCC forms the backbone of using this powerful tool effectively. Without the correct setup, compiling and executing code could become problematic, hindering progress in your programming endeavors.
Basic GCC Compiler Commands
The section on Basic GCC Compiler Commands serves as a fundamental building block in mastering the use of the GCC (GNU Compiler Collection). Understanding these commands is essential, as they establish a solid foundation for both novice and experienced developers. Basic commands allow programmers to compile code, link files, and produce executable outputs, which are crucial steps in the software development process. By grasping these concepts early, users can progress to more complex tasks and optimization techniques.
Compiling a Simple Program
Compiling a simple C program is often the first exercise for many developers exploring GCC. It represents a hands-on understanding of how source code translates into machine language. The fundamental command for this task is simple, yet powerful:
This command instructs the GCC compiler to take , compile it, and produce an executable named by default. This is useful for quickly testing small snippets of code. However, for larger projects, keeping track of generated files becomes important. The structure and format of commands must reflect the extensiveness of the project.
It’s also worth mentioning the need to check for and resolve any syntax errors or warnings during this process. Familiarity with the terminal can be invaluable. Run this simple example to see the translation from high-level code to low-level execution.
Using the -o Flag for Output Files
GCC provides an option called the flag, which specifies the name of the output file. It is quite practical because users often require meaningful file names for exectuables. For instance:
Here, instead of creating the default , GCC produces an executable called . This improves code organization, making it easier to manage and identify multiple compiled programs in a project folder.
Employing the flag also enables better integration with project build systems and scripting methods. When collaborating with teams, distinguishing which executable belongs to which source file is essential for efficiency.
Understanding the -c and -E Flags
The and flags are critical tools that help in the modularization of code. Using the flag allows developers to compile source files without linking them right away:
This command compiles and , generating respective object files ( and ). This method is particularly useful in large projects where separate compilation of modules is necessary. It enhances compile times by allowing partial recompilations.
On the other hand, the flag preprocesses the source code. This can be beneficial for debugging preprocessor macros and included files. The command is as follows:
Executing this command yields the preprocessed output of the C program, allowing users to observe the results of macro expansions and included headers prior to compilation. This detail is invaluable for understanding how the compiler interprets and expands code before it reaches the compilation stage.
Understanding these basic commands and flags is key to effective programming in C using GCC. They not only streamline the compilation process but also empower developers to handle larger projects confidently.
Advanced GCC Compiler Commands
The realm of advanced GCC compiler commands opens a door to optimizing the performance of programs and enhancing the efficiency of the development process. Mastery of these commands is essential for programmers who wish to elevate their coding capabilities and adapt their programs to different environments and requirements. It enables developers to exploit the full potential of the GCC compiler, ensuring their applications run swiftly and effectively on various platforms.
These commands are particularly beneficial for refining the performance of software applications. By using optimization flags, for instance, developers can instruct the compiler to analyze and enhance the code, potentially leading to significant speed improvements. Furthermore, debugging commands allow for identifying and resolving issues efficiently, thereby avoiding costly errors during the software development life cycle. Profiling capabilities help in understanding resource usage. Overall, familiarizing with advanced commands gives developers a strategic edge.
Optimization Flags for Enhanced Performance
Optimization flags are pivotal in GCC for improving program execution speed and minimizing runtime overhead. These flags enable the compiler to perform various analyses that can enhance the resulting binary's efficiency. Some common optimization flags include , , and , each providing a distinct level of optimization.
- : This flag enables basic optimization strategies while maintaining a balance between compilation time and performance.
- : It activates further optimizations—often leading to a noticeable performance enhancement without significantly increasing compile time.
- : This flag compiles the program with aggressive optimization techniques, ideal for compute-intensive applications, yet it may increase compile times.
Using these flags aids in producing faster and more efficient executables, improving the user experience and system performance. It is crucial to consider the nature of the project to select the most appropriate optimization level.
Debugging with GCC: -g Flag
The flag is essential when it comes to debugging applications with GCC. This flag informs the compiler to include debugging information in the generated executable. This information is critical for debugging tools like , allowing developers to diagnose issues effectively by providing line numbers, variable names, and other source-level details that aid in tracing program flow and state.
Including the debug information can take up additional space in the binary, but it is often necessary during development phases. Here is a simple example of how to use the flag:
By enabling debugging, developers can step through code, set breakpoints, and inspect variables. Consequently, the flag empowers developers to identify and fix bugs, enhancing code reliability and performance.
Profiling Your Code using -pg
Profiling is a fundamental aspect of performance tuning that can significantly impact application efficiency. The option in GCC allows developers to analyze the performance of their application by providing call graph information. This profiling data reflects how frequently each function is called, helping identify bottlenecks within the code.
When compiling an application with the flag, you can write:
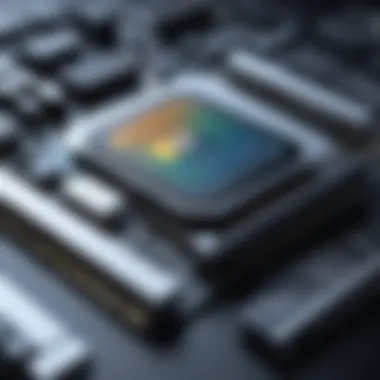
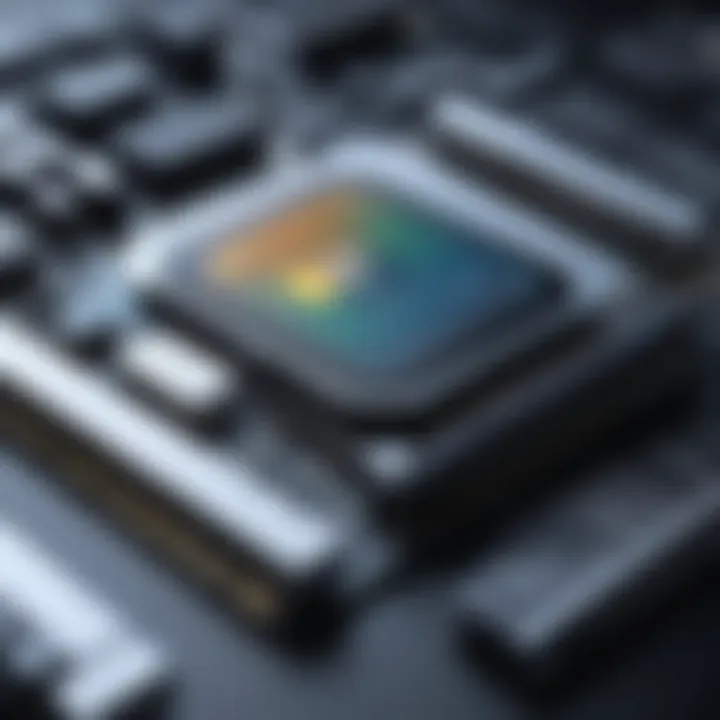
Once the application runs, it generates a file, which contains the profiling data. To visualize this data, developers can use the tool, allowing for deeper insights into the program's runtime behavior.
This process helps in making informed decisions on where to focus optimization efforts, ultimately leading to better software performance. By utilizing profiling tools, developers can pinpoint inefficient portions of their code and make necessary adjustments for enhanced application speed.
GCC Environmental Variables
GCC relies on various environmental variables to manage its operations more effectively. Understanding these variables is crucial for optimizing the compilation process and tailoring it to meet specific project requirements. Environment variables can significantly influence how GCC behaves, from specifying default libraries to setting paths for include files. By configuring these variables correctly, users can streamline their workflows and avoid potential issues during compilation.
Key Environment Variables
Some key environment variables that users need to be aware of include:
- CC: This variable specifies the default C compiler to use. Setting this can be helpful if you want to use an alternative version of GCC or another compiler altogether.
- CXX: Similar to CC, this variable indicates the C++ compiler. This can be useful for projects involving both C and C++ code.
- CFLAGS: Used to set default flags for the C compiler. Flags can control optimization levels, debugging information, and more. Setting proper CFLAGS can improve the performance and debugging of the final executable.
- CXXFLAGS: Works in the same way as CFLAGS but is specifically for C++ files.
- LDFLAGS: This variable is for passing flags to the linker when linking object files into executables.
Configuring these variables can help streamline the build process and avoid repetitive command-line inputs.
Setting Environment Variables for Custom Configurations
To set environment variables in a custom way, you can use shell commands. On a Unix-like system, you can do this directly in your terminal:
This command configures the variables for the current session. For a more permanent change, you might want to add these lines to your or file. This way, every new terminal session will automatically use your configurations.
Setting GCC environmental variables can lead to significant improvements in build efficiency and reduce the risk of configuration errors.
By customizing these environment variables, programmers can ensure that GCC compiles their code with the accuracy and efficiency they need. Understanding and effectively using these variables can elevate a developer’s capability to manage their environment, leading to smoother project executions.
Cross-Compilation with GCC
Cross-compilation refers to the capability of compiling code on one platform, targeting another platform. This feature is an essential aspect of software development, especially when aiming for versatility across various devices and systems. GCC's flexibility facilitates the creation of executables for multiple architectures, making it indispensable for developers working in diverse environments. Understanding this process can lead to significant improvements in development efficiency and a broader range of project capabilities.
Understanding Cross-Compilation
Cross-compilation allows developers to build software on a different machine than the one where the software will be run. This is particularly useful for embedded systems and mobile devices where a native build environment may not be feasible or available. The GCC framework provides tools to specify the target system architecture and operating system so that developers can generate executables suited for environments that differ from their development machine.
Advantages of cross-compilation include:
- Time Efficiency: It can be time-consuming and often impractical to move a development environment to an embedded system for testing. Cross-compilation allows developers to produce builds faster from their main workstations.
- Resource Utilization: Certain target systems may lack the resources needed for a full development environment. By cross-compiling, developers can leverage their primary machine’s capabilities.
- Wider Testing: Developers can target multiple architectures without the need for multiple devices, simplifying the testing pipeline.
Specifying Target Machines
When using GCC for cross-compilation, specifying the target machine becomes key. This can be achieved through the use of various flags that direct GCC on how to handle the compilation process. The flag is an essential part of this specification. It indicates to GCC the specific architecture for which the code should be compiled.
A typical usage might look something like this:
In this command, you instruct GCC to generate binaries for an ARM architecture on an x86_64 Linux machine. Other specific parameters can include:
- --prefix: Defines where the cross-compiled binaries will be installed.
- --with-sysroot: Specifies the location of target-specific libraries and headers, allowing GCC to find the necessary dependencies.
To ensure smooth cross-compilation, validate that the necessary libraries and headers for the target architecture are correctly set up in your environment.
By mastering the cross-compilation capabilities of GCC, developers can enhance the efficiency of their workflows and create applications that are portable across various systems and devices.
Common Errors and Troubleshooting
GCC compiler is a powerful tool, but it is not without its quirks. Understanding how to handle errors and troubleshoot common issues is essential in the development process. Errors can occur at various stages, from compilation to linking. Knowing how to identify and resolve these errors can greatly reduce development time and frustration.
Identifying Compilation Errors
Compilation errors are the most common issues encountered while using GCC. They arise when there is a problem with the code itself. This section outlines how to recognize and address these errors.
First, GCC reports errors in a standardized format. This means that any error message contains crucial information, like the type of error and the line number. For instance, if you see a message like this:
it indicates that there's a missing semicolon before the function returns a value. Always pay attention to the specifics of the message, as they can often lead you directly to the problem.
Apart from syntax errors, logical errors can also lead to compilation failure. However, they might not be caught until the code is executed. This is where debugging comes in. Make sure to run your code in a controlled environment to catch any unexpected behavior.
Debugging Common GCC Issues
GCC provides several tools and flags that can aid in debugging. One of the most helpful is the flag, which includes debug information in the compiled output. To use it, your command would look like:
This allows you to use a debugger like GDB to step through your code and find where things may be going wrong.
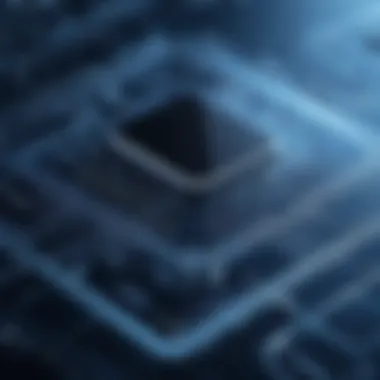
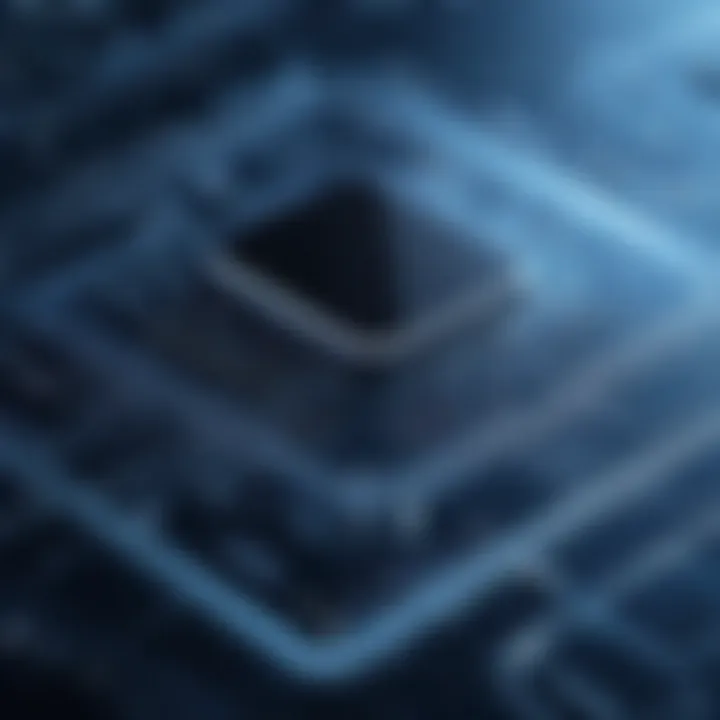
Common GCC issues can also stem from environment misconfigurations or linking errors. If you encounter an error stating something like:
it often indicates that the linker cannot find the definition of a function you have declared. Ensure that all necessary object files are included in your linking command.
Additionally, consider the following tips:
- Check if libraries are correctly linked. If using a library such as for math functions, make sure to include it with .
- Make use of the flag when compiling to enable warnings about questionable constructs. This can help catch issues before they escalate.
- Keep your code organized and modular. A well-structured codebase makes it easier to find and rectify errors.
"The only real mistake is the one from which we learn nothing." - Henry Ford
By becoming adept at identifying and debugging these common errors, you enhance not only your coding efficiency but also your confidence in using GCC effectively.
GCC Compiler Extensions
GCC Compiler Extensions offer a variety of enhancements that extend the capabilities of the GNU Compiler Collection. They allow developers to leverage additional features specific to certain languages, which can optimize performance or introduce functionalities not included in the standard language specifications. Understanding these extensions is crucial for programmers who wish to produce highly efficient, robust, and maintainable code for diverse applications.
Extensions can provide syntactic sugar, specialized data types, or even unique optimizations. They are particularly useful when targeting advanced application domains like embedded systems, kernel development, or high-performance computing. However, developers should tread cautiously because using these extensions can lead to reduced portability. Code that heavily relies on such features might not compile or perform similarly across different platforms or compilers.
Using Language-Specific Extensions
Language-specific extensions expand the existing language capabilities tailored for unique needs. For instance, in C, GCC includes various extensions that cater to inline assembly and certain data types. This can lead to enhanced performance by allowing for manual optimizations that are vital in critical application areas.
Benefits of Language-Specific Extensions:
- Performance Optimization: Developers can fine-tune their code to run faster by utilizing lower-level programming techniques.
- Advanced Features: Extensions like , which control alignment in memory, are useful in system-level programming.
- Increased Control: They allow more control over memory management and data representation.
Despite these benefits, it’s essential to check the compatibility of language-specific extensions with other compilers. Not every compiler supports the same set of extensions, which can hinder cross-platform development efforts.
Prelude to GNU Extensions
GNU C Extensions represent an additional layer of functionality tailored specifically for C programming. The extensions offer features ranging from type-generic macros to nested functions, which aren't present in standard C. These extensions can enhance the expressive power of programs, allowing developers to write cleaner, more maintainable code.
For instance, using the keyword, developers can provide the compiler with information about functions or variables, which can enable better optimization and checks during compilation. These attributes can specify alignment, visibility, and may even include optimizations for specific architectures.
Moreover, programmers can utilize GNU’s support for variadic macros, enhancing the flexibility of function definitions. This is crucial when building complex libraries or APIs where extensibility is a priority.
Key Points about GNU Extensions:
- They provide added flexibility in programming constructs.
- They can improve compilation warnings and errors, helping catch potential issues earlier in the development cycle.
- However, heavy reliance on these extensions can lead to code that is less portable and harder to maintain.
As a rule of thumb, use GCC-specific extensions mindfully. Assess the trade-off between performance improvement and potential portability issues in your development context.
Best Practices for Using GCC
When working with GCC, employing best practices is crucial for both efficiency and effectiveness. These practices not only save time but also enhance code quality. Proper usage of GCC ensures that programmers and developers can manage their projects with greater flexibility and fewer errors. It is essential to approach GCC with a structured mindset to leverage its full potential.
Organizing Your Code for Efficient Compilation
When your code is well-organized, GCC performs more efficiently. Code organization refers to how files and directories are arranged within a project. A clear structure allows for easier navigation, modifications, and compilations. Here are some key points to consider:
- Directory Structure: Create a logical directory hierarchy. Group similar files together, such as source files, header files, and documentation. This makes it easier to locate resources during compilation.
- File Names: Use meaningful names for files. Proper naming conventions improve readability. For example, use descriptive names that indicate the file's purpose, like or .
- Separate Modules: Break down your program into modules. Each module should handle specific tasks. This not only simplifies debugging but also speeds up the compilation process when you only need to recompile part of the codebase.
Proper organization leads to cleaner builds and minimizes compilation time.
Leveraging Makefiles for Project Management
Makefiles can be a game-changer in project management when using GCC. A Makefile is a special file used with the build automation tool to simplify the compilation of programs. Here are the benefits and how to create an effective Makefile:
- Automation: Automatically manage dependencies and recompilation. Instead of manually running GCC commands, a Makefile allows you to run a single command that compiles your entire project correctly.
- Maintainability: Makefiles help maintain your project as it grows. You can easily update build instructions without modifying the compilation command in multiple places.
- Example of a simple Makefile:This example sets a compiler, compiler flags, and outlines how to compile main and math functions into an executable. The target helps remove generated files.
Implementing these best practices will make your interaction with GCC more productive and less cumbersome, ensuring high-quality software delivery.
End
The conclusion plays a vital role in summarizing the essentials of using GCC compiler commands effectively. This article has outlined various aspects from basic commands to complex functionalities, helping readers navigate through the intricacies of the GCC environment.
This section illustrates the significance of what has been discussed. It consolidates knowledge and serves as a reflective tool for students and programming professionals alike. Understanding these commands not only aids in efficient code compilation but also empowers developers to troubleshoot, optimize, and enhance their projects. Benefits from this knowledge include savings in time and effort during the development process, which can exponentially impact overall productivity.
Moreover, a well-rounded grasp of GCC commands fosters greater confidence when faced with diverse programming challenges. It equips a developer with the ability to utilize advanced functions properly, thus opening up avenues for innovative coding practices.
Summarizing Key Takeaways
As we conclude, it is important to reiterate some critical points:
- GCC offers a robust set of commands that simplifies the compilation of C and C++ programs.
- Various compiler flags, such as -o, -g, and -pg, provide developers with flexibility in output management, debugging, and profiling their code.
- Understanding environmental variables can significantly improve customization options within your development environment.
- Common troubleshooting techniques are essential for identifying and correcting errors efficiently.
These takeaways can be beneficial regardless of whether you are a novice programmer or a seasoned IT professional seeking to enhance your skill set.
Further Resources for GCC Command Mastery
To continue developing your knowledge and mastery of GCC commands, consider exploring these resources:
- GNU Compiler Collection Documentation: The official documentation provides thorough insights into commands and flags. https://gcc.gnu.org/onlinedocs/
- Wikipedia - GCC: A comprehensive overview of GCC's history and features. https://en.wikipedia.org/wiki/GNU_Compiler_Collection
- Reddit Programming Communities: Engage with fellow learners and experts, share experiences, and seek help in subreddits dedicated to programming. https://reddit.com/r/programming
- Facebook Developer Groups: Join groups focusing on coding and compiler techniques to enhance your network and knowledge-sharing. https://facebook.com/search/top?q=developer%20groups
These resources can provide additional context, examples, and hands-on projects, further solidifying your command of GCC and its functions.