Mastering GDB for Java: A Detailed Guide to Debugging
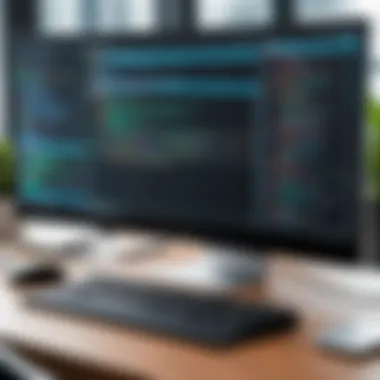
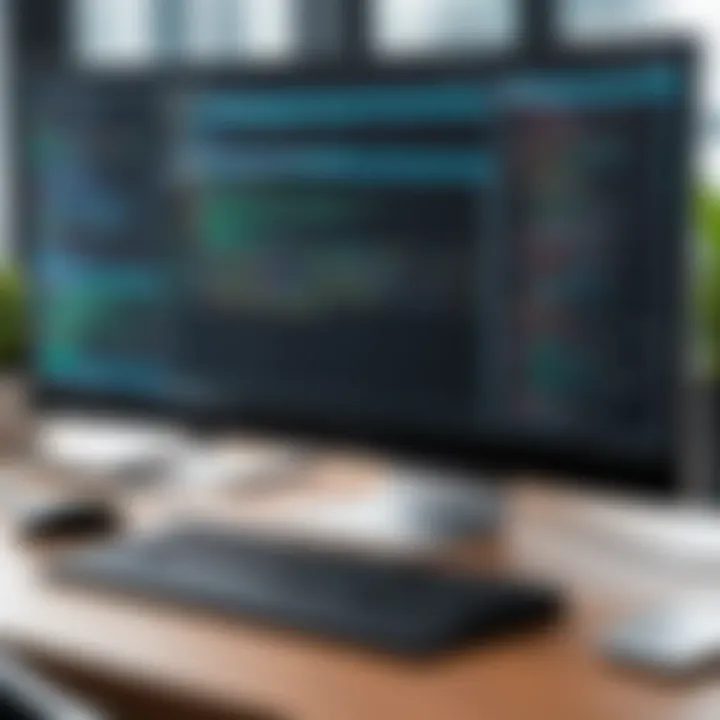
Overview of Topic
Java programming, while robust and widely used, isn't without its challengesâespecially when it comes to debugging. Enter the GNU Debugger, or GDB, a tool traditionally associated with languages like C and C++. However, GDB has increasingly found its place in the Java ecosystem, offering developers a powerful ally for tackling issues that arise in code execution. This guide aims to explore the functionalities of GDB, elucidating its application in Java programming.
Understanding the integration of GDB with Java is paramount in todayâs tech landscape. Debugging is a critical aspect of software development, and effectively employing a robust tool such as GDB can significantly enhance the quality and reliability of Java applications. This guide provides a thorough examination of GDB's features and commands, outlines practical applications, and delves into advanced topics that reflect current trends in the tech world.
The evolution of debugging tools has come a long way since the early days of programming. Initially, programmers relied on manual methods for error tracking, which often resulted in a tedious and time-consuming process. With the advent of sophisticated tools like GDB, developers now have access to an interface that streamlines debuggingâsaving precious time and improving code quality.
Fundamentals Explained
At its core, GDB serves as an intelligent debugger designed to assist developers in identifying and fixing problems within code. It allows for the examination of what happens inside a program while it executes or when it crashes. This debugging practice is crucial for maintaining the integrity of a project.
Key Terminology
- Breakpoint: A marker set by the developer to pause execution at a specific line of code, so they can inspect the program's state.
- Stack Trace: A report that provides information about the active subroutines (functions or methods) at a certain point in time during the execution of a program.
- Watchpoint: Similar to a breakpoint, but specifically triggers when the value of a variable changes, allowing you to monitor changes in the programâs execution.
In Java, the debugging process typically involves compiling code and running it within GDB, much like one would in a different language. Developers often get caught up in the crux of coding without acknowledging the underlying structures and functions that direct how the program behaves. Understanding these foundational elements is crucial to effectively utilizing GDB for debugging.
Practical Applications and Examples
Using GDB in a Java environment might seem unusual at first, but practitioners have already begun to reap the benefits of this tool. Consider the following real-world scenarios:
- Case Study: A software company struggled with a Java application that repeatedly crashed during user login. By setting breakpoints at crucial points in the application flow within GDB, the developers tracked a problematic function that generated excessive retries. Fixing this not only improved user experience but also reduced server load.
- Hands-On Project: Imagine you're building a simple Java application that computes Fibonacci numbers recursively. By integrating GDB, you can set watchpoints to observe how values change through the iterative process, allowing for an opportunity to pinpoint logic flaws that might otherwise go unnoticed.
In the code snippet above, imagine running this through GDB and immediately observing the output during recursion, making it a cakewalk to identify any inefficiencies in the recursive calls.
Advanced Topics and Latest Trends
GDB continues to evolve as debugging paradigms shift. Its integration with advanced IDEs and its growing compatibility with new languages, including Java, reflect ongoing trends in software development. Developers now have access to features such as multi-threading support, allowing for inspections of applications that process tasks concurrentlyâa prominent aspect of modern programming.
Moreover, the advent of graphical interfaces is transforming how developers interact with GDB, offering a more user-friendly experience as opposed to solely command line functionality.
Future Prospects
- Increased support for modern Java frameworks.
- Enhanced collaboration features for teams using GDB.
- Ongoing refinement of GDBâs capabilities for multi-language support.
Tips and Resources for Further Learning
To master GDB's robust capabilities, consider the following resources:
- Books: "Debugging with GDB" provides in-depth knowledge with practical examples.
- Online Courses: Platforms like Coursera and Udemy offer tailored courses focusing on GDB and debugging practices.
- Tools: JDB (Java Debugger) can be useful in tandem with GDB to enhance debugging experience.
Engaging with communities, such as the GDB subreddit, can offer invaluable insights from fellow programmers. Furthermore, articles from Wikipedia can provide a broader historical context to GDB usage across different programming languages.
Preamble to GDB and Java
When diving into the intricate realm of programming, debugging stands out as perhaps one of the most vital components. Itâs that bridge between the dreams you weave in code and the reality that unfolds on screen. For programmers delving into Java, leveraging tools that streamline the debugging process is pivotal. This is where GDB, the GNU Debugger, comes into play.
Overview of GDB
GDB is a powerful debugging tool widely used in various programming languages, and its application within Java brings unique advantages. This command-line tool allows developers to inspect whatâs happening inside a program while it executes or to analyze it after a crash. With GDB, programmers can thoroughly track down errors, manipulate the execution flow, and understand complex data structures in their Java applications.
Imagine working hard on a Java application, only to find it behaving in unexpected ways. Wouldnât it be comforting to have a toolkit that not only identifies where things go wrong but also provides a window into how variables change over time? GDB does just that, helping unravel the threads of execution to reveal the underlying issues.
Why Use GDB with Java?
Using GDB with Java isnât just about convenience; itâs an invitation to delve deeper into the structure of your code. For starters, one could argue that it bridges a gap between high-level language ease and low-level debugging power.
- Powerful Insights: GDB reveals intricate details about your program's performance, from memory usage to variable states, giving you a comprehensive view of its behavior.
- Efficient Bug Tracking: You can pinpoint the exact code line causing malfunctions, thus saving you legwork and possibly preventing future headaches.
- Control Over Execution: GDB grants developers the ability to pause execution, step through code, and examine state at will, akin to having a flashlight in a dimly lit room.
Setting Up GDB for Java
Setting up GDB for Java is a crucial step in leveraging debugging capabilities while working with Java applications. GDB, or the GNU Debugger, provides developers with tools to analyze code execution, inspect memory, and diagnose issues. Proper setup is key to ensuring that GDB functions seamlessly with Java programs, making it easier to identify and resolve bugs that can lead to erratic behavior or application crashes. Understanding the intricacies of setting up GDB for Java can make the debugging process less daunting, ultimately enhancing productivity and software quality.
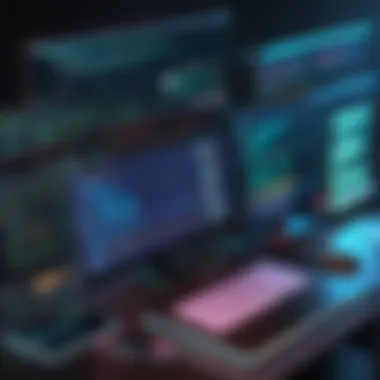
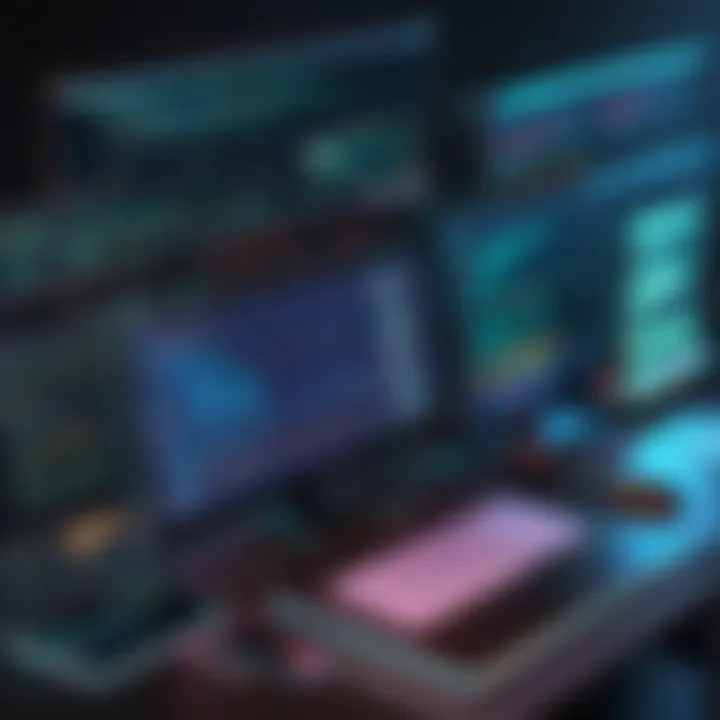
Installation Requirements
Before diving into the installation of GDB for Java, it is important to recognize the prerequisites that ensure a smooth setup process. Here are the key requirements youâll need to keep in mind:
- Operating System: GDB can be installed on various operating systems including Linux, Windows, and macOS. Each OS may have slightly different installation steps or command-line utilities.
- Java Development Kit (JDK): Make sure to have the JDK installed on your system, as GDB requires the Java tools for compiling and executing Java programs. Youâll typically want the latest stable version for compatibility.
- GDB Version: Ensure that you are using a version of GDB that supports Java. Versions post-7.0 have improved Java support, allowing for more effective debugging capabilities.
Verifying these components will help pave the way for a functional debugging environment that minimizes headaches down the line.
Steps for Installing GDB
Installing GDB on your system can differ depending on your operating system. Hereâs a straightforward guide to help you install GDB:
- Linux:
- Windows:
- macOS:
- Open your terminal.
- Update your package list with the command: (for Debian-based systems).
- Install GDB using: .
- Download the GDB installer from the MinGW website.
- Follow the installation prompts to install MinGW and choose the GDB components.
- Once installed, you can access GDB from the command line or through an IDE.
- Open your terminal.
- If you have Homebrew installed, simply run: .
- If not, consider following the official instructions for manual installation as listed on the GDB website.
While installations are often seamless, it's always good to refer to the official documentation for any lingering issues or specific system configurations.
Configuring Java for GDB
Once GDB is installed, configuring it to work with Java programs is the next vital step. This involves a few adjustments to ensure proper functionality:
- Compile with Debugging Options: To utilize GDB with Java, make sure to compile your Java code with debugging options enabled. Use the following command:This ensures that the compiled class files contain necessary debugging information.
- Set Environment Variables: Depending on your operating system, setting environment variables like can enhance compatibility. For instance:
- Test the Setup: Run a simple Java program within GDB to ensure that everything is functioning as expected. Start GDB and load your Java program using:
This provides an opportunity to confirm that GDB interacts correctly with your Java application.
"Setting up GDB is just the tip of the iceberg; the true power lies in understanding how to effectively utilize it for troubleshooting."
A well-configured environment can significantly boost your debugging efficiency. Having GDB ready to go opens doors to a better understanding of your code's behavior, making debugging less of a chore and more of a learning experience.
GDB Commands Specific to Java
Understanding how to leverage GDB commands tailored for Java is crucial for any developer eager to elevate their debugging prowess. When you step into the world of GDB specifically for Java, it pays to be familiar with these commands that serve as your toolkit. They can make a world of difference in catching elusive bugs, optimizing your workflows, and ensuring your code runs as expected.
Basic GDB Commands
When starting out with GDB, some foundational commands can make navigating through the debugger much easier. Hereâs a rundown of the basic commands you should know:
- run: This command starts executing your program. It's where the magic begins, and if you have input arguments, you can specify them right after the command.
- break: Setting breakpoints is essential for pausing execution at specific lines of code. For instance, places a breakpoint at line 10 of the source file. This allows you to inspect the state just before it reaches that point.
- next: As it suggests, this command helps you go to the next line of code, stepping over function calls.
- print: This one is for keeping tabs on variable values. Simply type , and GDB will show you the current value.
These commands are the bread and butter in the debugging process. To illustrate, while debugging a Java application, you might want to set a breakpoint at a method call and use to check how the parameters of that method change as execution moves along.
Advanced GDB Commands
Once you get comfortable with the basics, diving into advanced commands can significantly enhance your debugging capabilities:
- watch: Unlike the break command, pauses the program's execution whenever the specified variable changes. This is particularly valuable in scenarios where a variable being altered has ramifications.
- reverse-continue: A less common yet powerful feature, it allows you to continue from the current point of execution backward in time. If you've made a grave mistake but want to investigate without restarting, this is your best bet.
- commands: This command lets you define a sequence of commands to execute whenever a breakpoint is hit. This can automate tasks and make your debugging smoother.
- info locals: In scenarios complicated by multiple variable scopes, this command provides a list of all local variables at the current breakpoint or execution point.
The key benefit of mastering these commands is to gain a more granular control over your debugging process, enabling you to pinpoint issues quicker and with greater accuracy.
Common Debugging Scenarios
Having a repertoire of GDB commands is one aspect, but knowing when to use them is another story. Here are some common scenarios where GDB shines:
- Null Pointer Exceptions: If you face a Null Pointer Exception, start by setting breakpoints around the suspected lines. Once the breakpoint is hit, use to check your variables, helping you trace back to the root of the problem.
- Infinite Loops: An endless loop can drive any developer up the wall. Utilizing the command can stop your application at crucial points. From there, the command will reveal if variable states changed as expected.
- Thread Issues: Debugging multi-threaded applications is tricky. Using can provide insights into all running threads, while setting breakpoints in different threads helps isolate and identify issues more effectively.
In summary, understanding and properly utilizing GDB commands can save hours of debugging time. Mastering basic commands provides a solid foundation, while learning advanced techniques further empowers you. Always keep these scenarios in mindâhaving a command ready for particular problems makes you a more efficient programmer.
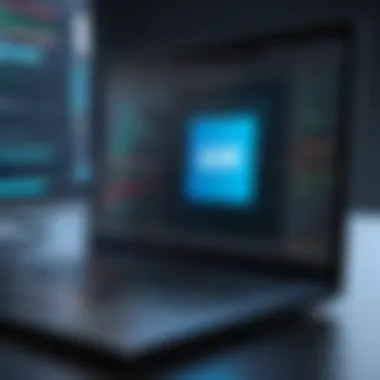
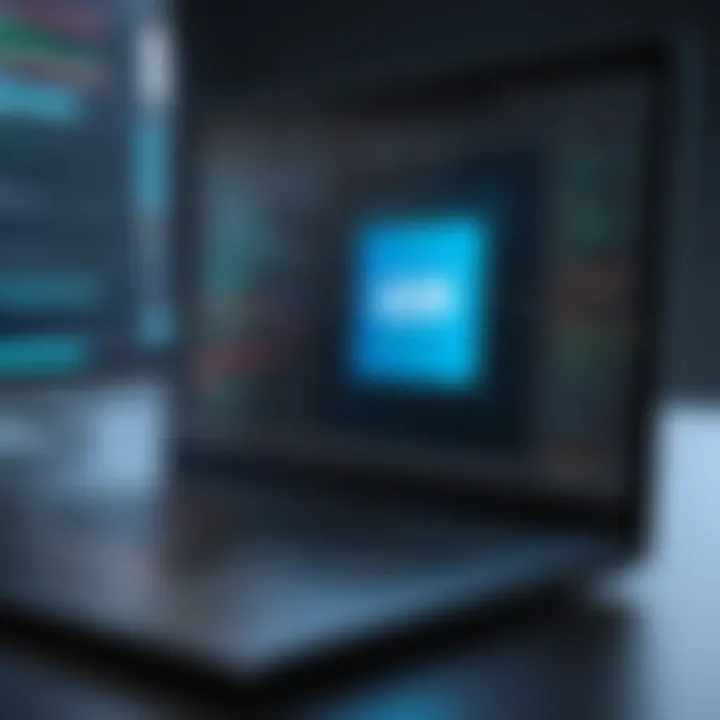
Integrating GDB with Java IDEs
Debugging is oftentimes viewed as a daunting task, but with the right tools, it can become a seamless part of the development process. Integrating GDB with Java IDEs like Eclipse and IntelliJ IDEA offers a range of advantages that can significantly enhance oneâs debugging experience. By combining the powerful capabilities of GDB with user-friendly development environments, programmers can achieve a streamlined workflow.
Using GDB in an IDE helps in managing complex debugging scenarios more effectively. The GUI of these IDEs provides a visual representation of code execution, which can be much easier to comprehend compared to command-line interfaces. Moreover, you gain the ability to set breakpoints, inspect variables, and control program execution all from a singular interface, thus allowing for quicker identification of issues. Here's why this integration is crucial:
- Efficiency: Working within an IDE minimizes context-switching between tools. This keeps developers in the zone, allowing them to work faster and more effectively.
- Visualization: IDEs come with visual debuggers that enhance the understanding of code flow and data structure, making it simpler to catch errors.
- User Experience: The graphical aspects of an IDE can help novice or intermediate developers grasp debugging concepts without getting mired in the technicalities of GDB commands.
Let's dive deeper into how specifically GDB can be utilized with Eclipse and IntelliJ IDEA.
Debugging Techniques Using GDB
Debugging forms the backbone of software development. In the context of GDB and Java, it opens up a realm of opportunities to scrutinize your code with unparalleled precision. Understanding the techniques for effective debugging using GDB is not just beneficial; itâs essential for anyone looking to refine their development skills. By mastering these techniques, programmers can identify issues faster, leading to more robust applications and a smoother coding experience.
Setting Breakpoints
Setting breakpoints is akin to planting flags in the rough terrain of your code. Essentially, a breakpoint allows developers to pause the execution at a specific line, facilitating a closer inspection of the program's state. By doing this, a programmer can clarify what happens around a particular line and identify inaccuracies in logic or values that donât add up.
Establishing a breakpoint with GDB is straightforward and can be accomplished using the command:
For instance, if you're debugging a function in and want to inspect line 10, you'll type:
Doing so pauses your programâs execution right at that line, allowing you to examine variables and control the flow effectively. This kind of probing is particularly vital when dealing with more intricate pieces of code where the flow isn't linear.
Examining Variables
Once you've paused execution with a breakpoint, the next step is examining variables, which is crucial for understanding how data moves through your program. Through GDB, you can check the value of variables and understand the state of your application at the exact moment of interruption. This step helps in pinpointing problems that might arise due to incorrect values or unexpected changes.
Using the command:
allows you to evaluate any specific variable's value. For example, if you want to check the variable while the program is paused, you simply type:
Moreover, GDB also supports another command:
This will show you all local variables within the current scope, which can be instrumental when you're trying to get a broader picture of the situation.
Controlling Execution Flow
Controlling execution flow goes hand-in-hand with breakpoints and variable examination. After pausing your program, you have several options on how to proceed. The ability to step through code line-by-line or to continue execution until the next breakpoint is incredibly powerful. This type of control allows for meticulous examination without losing sight of the overall logic in your program.
Some key commands in GDB include:
- Next: Moves to the next line of code, while skipping over function calls.
- Step: Enters into function calls, allowing you to trace through them one line at a time.
- Continue: Resumes running the program until the next breakpoint is hit.
These methods give a programmer the tools needed to dissect complex issues and understand how data changes through the execution lifecycle. By mastering these techniques, code collapses due to obscure bugs can be resolved more efficiently, ultimately leading to higher quality software.
Debugging is not just about fixing bugs; itâs also about understanding your code deeply.
In summary, the techniques provided by GDB for debugging Java not only streamline the process of identifying issues but also build a foundation for developing cleaner and more efficient code. Each stepâsetting breakpoints, examining variables, and controlling execution flowâworks in tandem, facilitating a comprehensive understanding of what's happening behind the scenes.
Common Pitfalls in Java Debugging with GDB
Debugging with GDB in the context of Java programming can be a bit like trying to catch smoke with your bare hands. Itâs tricky, and some all-too-common missteps can make your life harder than it should be. Understanding these pitfallsâsuch as misconfigured environments and ignoring compiler optimizationsâis key to ensuring a smooth debugging process.
These issues can drastically affect your workflow, increase frustration levels, and ultimately lead to more bugs in your code. So, letâs break down these common snafus youâd want to watch out for in your journey to mastering GDB for Java.
Misconfigured Environments
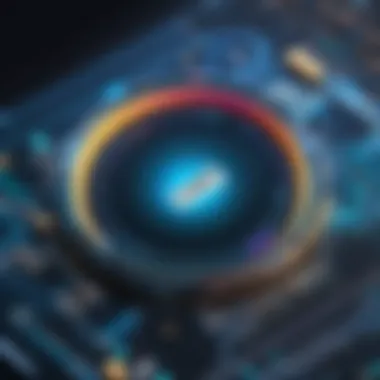
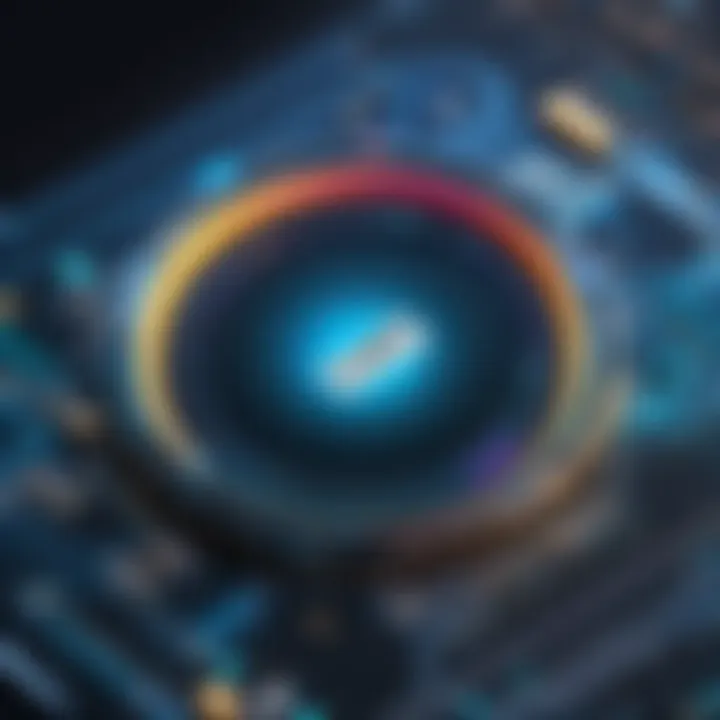
A misconfigured environment can be a thorn in your side when trying to debug Java applications with GDB. Ensuring that your paths are set up correctly, the right libraries are linked, and the environment variables are appropriately configured is critical.
- Java Versions: It's easy to forget that different versions of Java can behave differently. Make sure your GDB and Java versions align as expected. A mismatch might mean certain features or debugging capabilities are simply unavailable.
- GDB Configuration: Remember to set your GDB to recognize Javaâs specific bytecode. If itâs not set, you could end up hunting a ghost in your code, getting misleading information during your debugging session.
- Environment Variables: Things like should point to where Java is installed, and must include all necessary libraries. Misconfiguration here can lead to GDB not being able to locate the files it needs.
Proper configuration can save you a mountain of headaches. Double-checking these settings might just keep you on the path to a smoother debugging experience.
"A stitch in time saves nine." â Old adage, but fitting in this case.
Ignoring Compiler Optimizations
Ignoring compiler optimizations is another common snag in Java debugging with GDB. Java compilers, such as the Java Compiler (javac), often do some pretty heavy lifting regarding optimization. Not accounting for these can throw a wrench into your debugging process.
When compiler optimization flags are enabled, certain expected behaviors in code do not manifest during debugging. For example:
- Code Elimination: Some sections of code may be eliminated completely if the compiler determines theyâre unnecessary, leading to unexpected states that can baffle you.
- Inlining: Methods can be inlined, changing how stack traces appear and making it difficult to trace back through function calls.
To mitigate this issue, a wise approach is to compile your Java code without optimizations when preparing for debugging sessions. You can do this by using the following command:
The option includes debug information in the compiled class files, which is essential for a smoother debugging experience. Failing to account for these optimizations can cause you to chase shadows instead, leaving you struggling to make sense of GDBâs output.
In sum, being aware of the environment youâre working within and the optimizations your Java compiler applies can vastly improve your debugging experience. Both misconfigured setups and ignored compiler settings can whip your workflow into a tailspin, making an already intricate process all the more complicated.
Best Practices for Effective Debugging
When we talk about debugging in Java using GDB, we canât overlook the importance of best practices. These practices help in not only smoothing the debugging process but also in ensuring that you are on the right track to delivering quality software.
One key aspect is keeping a tidy workspace. When your code is organized, itâs easier to spot flaws. For instance, imagine your function names speak to their purpose clearly, or that your variables have descriptive names rather than just being labeled or . Itâs like guiding someone through a maze with a clear map instead of handing them a jumbled assortment of directions.
"An ounce of prevention is worth a pound of cure."
This old adage resonates well in the context of debugging. If you employ effective practices during the coding phase, you will have fewer headaches later while trying to debug using GDB.
Writing Debug-Friendly Code
Creating debug-friendly code starts with a solid foundation. To begin with, always aim for simplicity. Complex structures and large classes can obscure errors. Short functions are easier to test and debug.
Some tips:
- Comment Your Code: Adding commentary can assist not only your future self but also others who may interact with your code later. It reduces time spent figuring out the logic.
- Use Assertions: Applying assertions can catch errors early in the development. If something goes wrong, an assertion will indicate where it occurred, making it easier to trace back.
- Follow a Consistent Style: Consistency in naming conventions and formatting renders your code readable, making it simpler to run through the logic when debugging with GDB.
Incorporating these principles significantly reduces debugging time. Less confusion and more clarity lead to fewer mistakes when it comes time to trace errors.
Regularly Testing and Monitoring
Regular testing and monitoring is crucial. The earlier you catch a bug, the less headache it will in the future. Think of it like maintaining a car. If you ignore small sounds and vibrations, they may lead to major engine problems down the line.
Here's how to ensure you keep on top of testing:
- Unit Testing: Break your application into smaller chunks and test them independently. This way, you spot issues solidly linked to specific components.
- Integration Testing: As modules begin to fit together, testing them as a whole can reveal integration problems that wouldnât show up during unit tests.
- Code Reviews: Collaborating with peers during reviews can surface bugs early. Fresh eyes are often great at noticing whatâs missed by the original coder.
With a consistently monitored environment and regular tests in place, you build a safety net that makes debugging more manageable when you need to fall back on GDB.
In addition, maintain an eye on log files. They serve as a record of what transpired in your applications, often shedding light on issues that arise post-deployment.
Adopting these practices not only paves the way for easier debugging with GDB but also improves overall code quality. Everybody wants to avoid scrambling for solutions in the eleventh hour, and following these guidelines can keep that chaos at bay.
Ending: Enhancing Java Development with GDB
The use of GDB in the realm of Java programming can't be overstated. As developers, we continually seek tools that not only increase our efficiency but also deepen our understanding of the code we write. GDB serves this purpose by presenting a powerful debugger that, when integrated with Java, opens up avenues for meticulous analysis of applications.
One primary advantage of utilizing GDB in Java development is its robust functionality in troubleshooting complex issues. Traditional debuggers often present a limited view. GDB, however, empowers programmers to dive into the nitty-gritty of their execution environment. You can trace through code line by line, inspect variable values at any point, and execute commands interactively. The immediate feedback allows you to rectify issues in real-time, saving not just time but also reducing the potential for downstream errors.
Moreover, GDBâs ability to handle multi-threaded applications is immensely helpful. Developers can monitor multiple threads of execution simultaneously, which is crucial in todayâs software landscape where concurrency is the norm. GDB helps in identifying race conditions, deadlocks, and other threading issues that may otherwise go unnoticed during typical runtime.
To sum up, GDB enhances Java development through:
- Deep Insights: By providing detailed reports on variable states and function calls.
- Multi-threading Support: Enabling developers to debug concurrent code effortlessly.
- Integration Capabilities: Connecting seamlessly with various IDEs, allowing for a smooth workflow.
"Leveraging GDB can turn a challenging debugging experience into a structured, manageable process."
While the learning curve may be steep for some, the benefits far outweigh the initial difficulty. As with any tool, practice and familiarity lead to proficiency. Therefore, engaging with GDB regularly fosters a more profound understanding not just of the debugging process but also of the Java platform itself.