Comprehensive Guide to GUI Development in Java

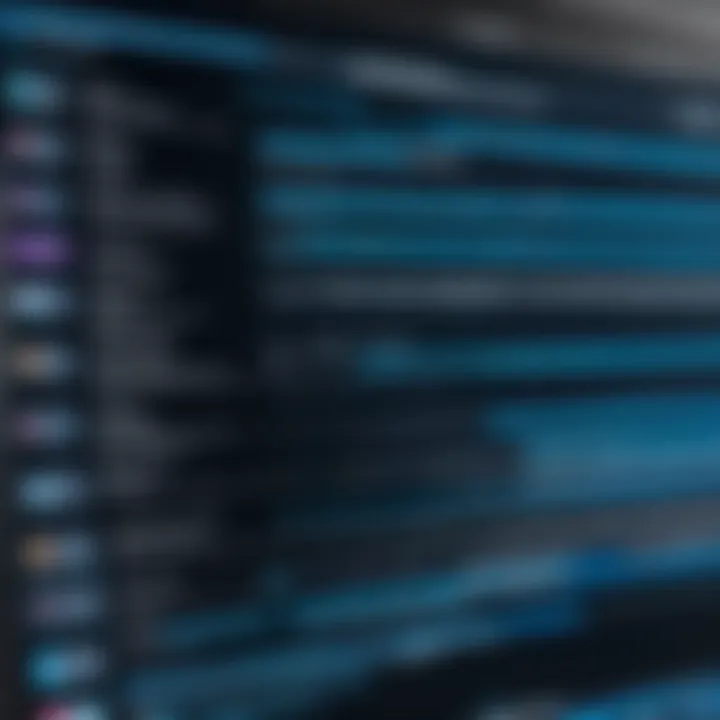
Intro
Graphical User Interfaces (GUIs) have transformed howusers interact with software applications. In the rapidly evolving tech industry, GUI development in the Java programming language holds a significant place. Many developers are drawn to Java due to its portability across platforms and robust libraries for building graphical interfaces. This article intends to provide both a theoretical and practical exploration of GUI development in Java, supporting developers at various skill levels.
Overview of Topic
Understanding the concept of GUI development is crucial in today's software innovation landscape. GUIs offer intuitive user interactions, which enhance user experience and efficiency. Java provides a robust ecosystem with libraries like Swing and JavaFX, designed specifically for building rich GUIs. These libraries have evolved over the years, being pivotal in the development of countless applications across multiple domains, from mobile software to enterprise-level systems.
The scope of GUI development extends beyond mere aesthetics. It involves carefully考虑ing user needs, accessibility, and functional design, which greatly determine the usability and functionality of applications in diverse environments.
Fundamentals Explained
To develop effective GUIs in Java, one must grasp a few key principles:
- Components: These are the building blocks of the GUI, such as buttons, text fields, and labels.
- Layout Management: Determines how components are arranged in the application window.
- Events: Represents actions performed by the user. Handling events is central to making the interface interactive.
Key Terminology:
- Swing: A Java library for creating window-based applications.
- JavaFX: A more modern tool for designing rich GUI applications.
- AWT: The Abstract Window Toolkit, an earlier Java GUI development library.
Understanding these fundamentals lays the groundwork for exploring more complex topics later.
Practical Applications and Examples
In real-world applications, GUI development showcases its necessity:
- Customer Relationship Management (CRM) Systems leverage GUIs for user-friendly interfaces.
- CAD Applications Utilizes creative visual component interactions to build designs.
For a hands-on project, one might start with a simple Swing application. Here is a minimal example to create a basic window:
A result of this code will be a simple window presenting a greeting message. Practical implementation consistently enhances understanding.
Advanced Topics and Latest Trends
As the field develops, new trends emerge:
- Integration of responsive designs for mobile and desktop platforms.
- Increased emphasis on usability and user experience.
- Use of frameworks that simplify complex tasks, such as JavaFX with its powerful property bindings and scene graph.
By analyzing upcoming tools, developers can position themselves at the forefront of GUI technologies in Java.
Tips and Resources for Further Learning
Guidance is vital in mastering Java GUI development:
- Books:
- Online Resources:
- Core Java Volume I by Cay S. Horstmann.
- JavaFX 8: Introduction by Example by Carl de Souza.
- Wikipedia on Java)
- reddit.com Java for community assistance.
Also, join developer forums to stay updated with tools available for GUI design and development. Consider using integrated development environments (IDEs) like IntelliJ IDEA or NetBeans for showcasing GUIs efficiently and effectively and exploring their advanced features.
The uniqueness of GUI development in Java lies in the possibilities it opens for interdisciplinary applications, from educational software to industrial systems. By continuously learning and practicing, developers can cultivate significant skill sets necessary for this domain.
Prologue to GUI in Java
Graphical User Interfaces (GUIs) are a critical component of modern software applications. The capacity to engage users through visual icons, buttons, and layouts has reshaped how applications operate today. As applications evolve, the necessity for well-designed and functional GUIs has never been greater. This section serves as an introduction to the essential aspects of GUIs in Java, exploring their purpose, benefits, and significance in the broader context of application development.
Understanding Graphical User Interfaces
A graphical user interface facilitates human-computer interaction through visually represented elements rather than text-based commands. This interaction paradigm allows for a more intuitive approach to using applications. Java provides diverse frameworks, mainly Swing and JavaFX, which allow developers to create GUI applications effectively. Structuring UIs in Java means leveraging pre-defined components such as buttons, sliders, and forms that reduce the overload of information for users.
The importance of these details cannot be underestimated. Understanding the components enables developers to craft applications that resonate with user expectations and cognitive capabilities. Thus, applications become not just functional but also user-friendly and visually engaging.
Importance of GUI in Modern Applications
In the modern era, applications serve multiple purposes, launching functionalities previously confined to desktop software and transitioning to the web and mobile platforms. Having a well-designed GUI can enhance usability, functionality, and growth.
A few critical points outline this importance:
- User Experience: A well-structured GUI improves user experience (UX) by making tasks easy to perform without extensive training or instructions.
- Customer Retention: Applications with user-friendly interfaces are likely to maintain customer loyalty, as users can navigate the system intuitively.
- Efficiency and Productivity: Streamlined GUIs save time. Users can find information quickly, perform tasks, and achieve goals without delay.
- Accessibility: GUIs support diverse user populations, catering to those with varying levels of technical skills and those requiring assistive technologies.
In a digital age where fast responses win user's hearts, a well-designed GUI becomes crucial for any software application aiming for engagement and success.
Understanding the basics and importance of GUI development in Java enables programmers to create robust applications tailored to user needs. As we will discover in subsequent sections, mastering this development translates to crafting powerful user experiences expected from modern software.
Java GUI Frameworks Overview
Understanding the available GUI frameworks in Java is crucial for developers aiming to create sophisticated visual applications. When it comes to Java, two primary frameworks dominate the landscape: Swing and JavaFX. Each of these frameworks carries specific characteristics that address different needs, offering various functionalities that can enhance user experience.
Using Java GUI frameworks appropriately can greatly impact the functionality, performance, and aesthetic appeal of an application. Familiarity with these frameworks not only boosts development efficiency but also ensures that applications remain adaptable and scalable. Focusing on framework selection based on project requirements is essential in master the art of building user interfaces in Java.
Swing Framework
History of Swing
The Swing framework emerged as a significant advancement over its predecessor, AWT (Abstract Window Toolkit). Swing was developed in the mid-1990s as part of the Java Foundation Classes (JFC). As a lightweight GUI toolkit, its integration with an event-handling model and the pluggable look-and-feel concept truly influenced the landscape of Java development.
One area where Swing excels is in its rich set of components that can be customized for specific needs. This flexibility makes it a favorable choice for many developers. While it does have a somewhat steeper learning curve compared to simpler libraries, its depth compensates for this through its feature-rich offerings.
Key Features of Swing
Swing is favored for its extensive component library, which enables the creation of dynamic and versatile user interfaces. Important features include pluggable look-and-feel that allows changes in appearance without modifying the underlying structure. Additionally, Swing’s lightweight components enhance both performance and interoperability across platforms.
Despite its strengths, some weaknesses include slower rendering times compared to other newer frameworks. However, many projects still find merit in Swing's comprehensive range of tools that cater specifically to varied applications and use cases.
Example Applications Using Swing
Swing is used in numerous applications across different industries. Notable examples include integrated development environments (IDEs) like NetBeans and enterprise-level applications for managing databases, such as Jaspersoft Studio.
The use of Swing allows developers to build applications strong in functionality while maintaining a clean user interface. However, its emerging age and some performance concerns have prompted many to consider other frameworks in recent years.
JavaFX Framework
Intro to JavaFX
JavaFX represents a more modern alternative to Swing, introduced to provide enriched UI features distinct from its predecessors. One of its most celebrated aspects is its built-in support for rich graphics, video playback, and animations. It is designed to provide a reduced learning curve less complicated features as well offer browser app capabilities offered today.
Moreover, JavaFX employs FXML, an XML-based language that simplifies UI design, allowing separation of design from business logic. This framework's upsurge in popularity unterthe developer community is largely due to its capabilities to handle diverse graphical needs alongside standard UI elements.
Advantages of JavaFX Over Swing
Addressing the limitations of Swing, JavaFX provides improved performance and can easily manage multimedia applications. Enhanced maintaining and scaling features are built directly into JavaFX, thereby offering incremental management for modern applications.
The CSS-like styling abilities allow developers to create visually appealing interfaces that easily adhere to modern design principles. While developers are gaining familiarity with JavaFX's performance advantages, older applications may still rely heavily on the traditional functionalities Swing offers for backward compatibility.
Example Applications Using JavaFX
JavaFX powers various applications thanks to its superior multimedia capabilities. Examples span across game development and advanced UI-centric social applications such as JFoenix, a library that provides material design components, presenting modern UI aesthetics to Java users.
An all-encompassing power blend with the contemporary tooling has made JavaFX ideal for projects that require engaging user engagement while maintaining a rapid assembly structure. However, developers migrating from Swing must approach JavaFX with an open mind to grasp the diverse advantages of current UI technology.
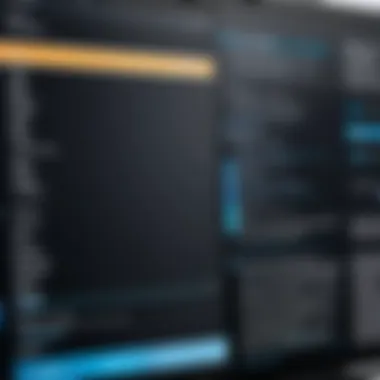
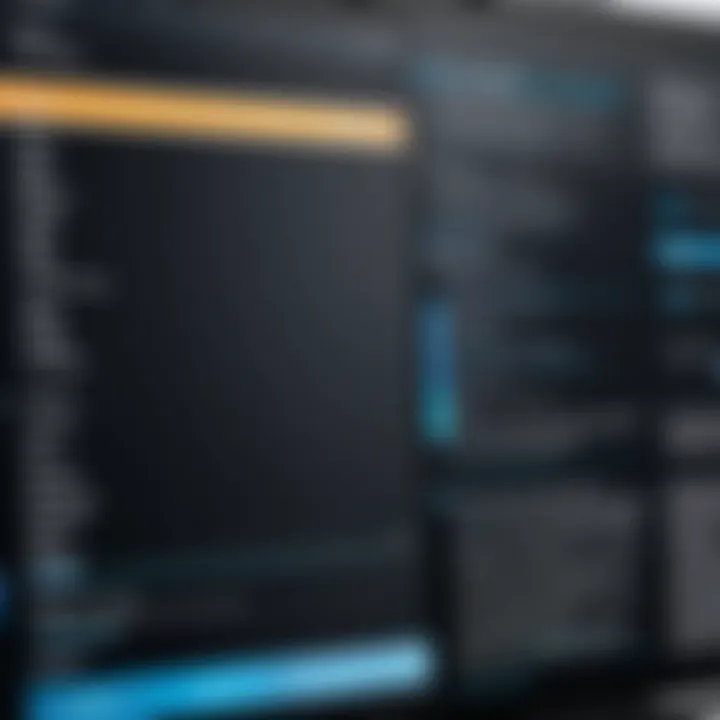
Setting Up Your Development Environment
Creating a solid environment for GUI development in Java is pivotal. The right setup not only enhances productivity, but it also ensures that developers can fully grasp the tools available. A solid foundation can significantly reduce the chances of encountering avoidable errors and problems during the development process. Furthermore, an appropriate setup facilitates easier integration of libraries and frameworks.
Installing Java Development Kit (JDK)
Installing the JDK is the first step in beginning Java development. The JDK contains all the tools necessary for developing in Java, including the Java compiler and various libraries. One must have a compatible version installed to take full advantage of the features of the chosen frameworks.
- Download the JDK: Visit the official Oracle website or OpenJDK site to acquire the latest JDK version.
- Installation Process: Follow the installation prompts specific to your operating system. This typically includes a series of standard steps, such as agreeing to terms, selecting installation paths, and further settings.
- Verify Installation: After installation, it is crucial to verify that JDK is correctly set up by running the command in the terminal.
Choosing the Right IDE for GUI Development
Choosing an Integrated Development Environment (IDE) significantly influences productivity and development experience. Several IDEs offer robust support for Java GUI development. The popular options are Eclipse, IntelliJ IDEA, and NetBeans, each with its respective strengths.
Eclipse
Eclipse is an open-source IDE renowned for its extensibility. The modular architecture allows developers to enhance the IDE with a myriad of plug-ins. For instance, the SWT (Standard Widget Toolkit) frameworks enable native GUI development using Java. One benefit of Eclipse is its large community, which assures ample resources and support.
Advantages of Eclipse:
- Drive extensibility with a wide selection of plugins.
- Facilitate working on multi-project sets efficiently.
Disadvantages of Eclipse:
- The interface can be overwhelming to new users due to its complexity.
IntelliJ IDEA
IntelliJ IDEA is regarded for its intelligent code completion and frequent updates. This IDE provides a user-friendly experience with a systematic support system in place. Developers appreciate the powerful debugging tools available. Additionally, code refactoring is simpler and can save significant time during development.
Advantages of IntelliJ IDEA:
- Excellent performance features and quicker execution.
- Adjust to swift code enhancement with suggestions.
Disadvantages of IntelliJ IDEA:
- Not all features are available in the free version; the ultimate functionality requires purchasing the premium version.
NetBeans
NetBeans is another ideal choice for newcomers and professionals. The easy installation process allows users to promptly get the development environment set up with necessary libraries. The user interface supports rapid development in Java and it provides good integration with other tools.
Advantages of NetBeans:
- Provides a smooth installation and setup experience.
- Good for beginner developers as it integrates quickly and coherently with Java frameworks.
Disadvantages of NetBeans:
- It can be sluggish at times, especially with larger projects.
Choosing the correct IDE is essential for efficient and effective development processes. Each option presents unique benefits but also has specific limitations.
Appropriating the suitable setups within your development environment both simplifies and amplifies the experience of creating Java GUIs. The right combination facilitates productive application development and prepares yourself for excellent outcomes in your work.
Design Principles for Effective GUIs
Designing effective graphical user interfaces is not just an art; it is a skill critical for Java developers aiming to create applications that resonate with users. The key principles that underlie effective GUI design shape user interaction, enhance usability, and ultimately dictate the success of applications. Understanding and applying these principles result in interfaces that not only fulfill functional requirements but also foster an engaging user experience. Effective design principles balance visual appeal with accessibility and usability, ensuring that applications cater to a broad spectrum of users.
User-Centered Design Approach
User-centered design (UCD) is a vital philosophy promoting a deeper understanding of the end user as the focal point of the design process. This ensures that applications are tailored to meet user needs effectively. Drawing insights from user behavior, preferences, and feedback significantly enhances usability.
Key aspects to consider in UCD include:
- User Research: Conducting surveys, interviews, and usability testing helps gather invaluable data about user behavior and preferences.
- Iterative Design: UCD advocates for constant feedback loops. Prototyping and testing allow for the refining of user interfaces based on users input.
- Context of Use: Designers should analyze the environment and situations in which users will interact with the application, which influences overall design choices.
By keeping users at the forefront, applications become intuitive and responsive to real-world needs, negating the common pitfall of imposing the designer's perspective onto the user experience.
Consistency and Simplicity in Design
The design principle of consistency encompasses both design elements and functionality, allowing users to develop a familiarity with the application that facilitates easy navigation. When visual styles, language, and user interface elements remain uniform throughout the application, users spend less time trying to decipher new interactions, leading to a refined experience.
Simplicity is paramount in reducing cognitive load. A straightforward and uncluttered design emphasizes core functionalities, allowing users to focus on task completion. Important elements to consider are:
- Visual Hierarchy: Create a prioritization of information using font sizes, colors, and spacing to guide users visually.
- Limit Interactions: Reduce the number of choices presented to users at each interface stage to avoid overwhelming them.
- Clear Labels and Instructions: Make buttons, menus, and options visible and succinct to convey actions clearly, ensuring comprehension.
Together, consistency and simplicity foster an interface that feels cohesive and reduces friction for the user, enhancing overall satisfaction with the application.
Accessibility in GUI Development
Addressing accessibility in GUI design is not just ethical; it is also practical. Inclusive applications expand your audience to users with various disabilities, enhancing user engagement and satisfaction. Accessibility principles mandate consideration for various physical and cognitive challenges users may have.
Tip>WAI-ARIA (Web Accessibility Initiative – Accessible Rich Internet Applications) guidelines provide frameworks for enhancing accessibility in web applications.Tip>
Key considerations for accessible GUIs might include:
- Keyboard Navigation: Ensure all functionalities are accessible without a mouse for users with limited mobility.
- Color Contrast: Use color schemes that allow for readability by individuals with visual impairments; choosing high contrast between text and background is essential.
- Alternative Text: Employees alternative descriptions for images or icons, so users with screen readers can engage with content meaningfully.
Focusing on accessibility extends the reach of your applications, fostering a user-friendly approach where no one is left out, ensuring that everyone can benefit from the tools you create.
Building a Basic GUI Application in Java
Building a basic GUI application is critical to both learning and mastering Java's capabilities in developing user interfaces. Understanding how to create simple applications provides a foundation upon which more complex functionalities can be constructed. These early stages of development introduce key concepts like listeners, layout management, and event handling, which are essential for any Java programmer.
Working with GUI creates a direct interaction with end-users, enhancing engagement. Furthermore, since Java applications are built to be cross-platform, developers benefit from the insights gained in setting up applications that run effectively on different operating systems.
Creating Your First Swing Application
Creating your first Swing application marks a significant step in your Java journey. Swing provides a robust set of components that can be customized and extended, making it one of the preferred choices for many developers.
Code Structure
The code structure when working with Swing is straightforward yet powerful. Swing uses a Unified Modelling Language (UML) architecture that separates the graphical representation from the business logic. This separates concerns better, allowing developers to maintain and update code more efficiently. This clear separation of files and resources makes it easier to design complex visuals while keeping the logic clean.
A key characteristic of Swing's code structure is its use of components. Each UI element, such as buttons or text fields, is an instance of a class. This is beneficial since it promotes an object-oriented approach where developers can readily create different reusable components across applications.
One unique feature of Swing's code structure is the ability to customize components extensively. This agility allows for tailored user experiences but can lead to challenging debugging processes when numerous components are customized without documentation.
Compiling and Running the Program
Compiling and running a Swing program typically involves using APIs provided by Java. To compile, developers will use the Java Compiler () and to run the produced bytecode, the command is executed. The simplicity of these commands contributes to Swing's user-friendly appeal.
A KEY characteristic of compiling in this environment is conformance with Java's overall platform independence. Developers can swing their applications to any compatible system, promoting versatility assured by Java’s inherent design.
The compiling and executing features within Swing offer unique advantages. For instance, the ability to make changes, recompile swiftly and observe reflections is invaluable during the debugging process. However, errors can arise due to changes made without recompilation, leading to potential confusion.
Creating Your First JavaFX Application
JavaFX modernizes the Java platform’s user interface design by incorporating a more modern rendering engine. Developing with JavaFX enables developers to create more visually appealing interfaces that are in tune with contemporary design patterns.
Code Structure
JavaFX's code structure corresponds closely to the Model-View-Controller (MVC) design pattern, wherein user interface elements are dealt with as nodes in a tree structure. This viewpoint enhances maintainability and scalability.
One of the primary interesting aspect of JavaFX’s Code Structure is that it embraces FXML, an XML-based format. This permits designers to create UIs separately from the Java code itself, which is a practice that developers can find much simpler and manageable.
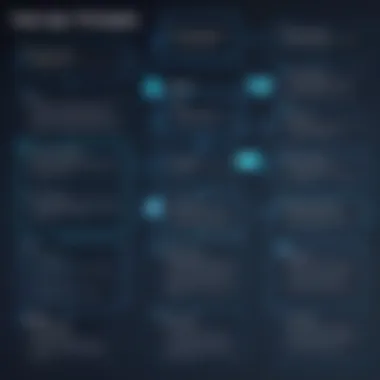
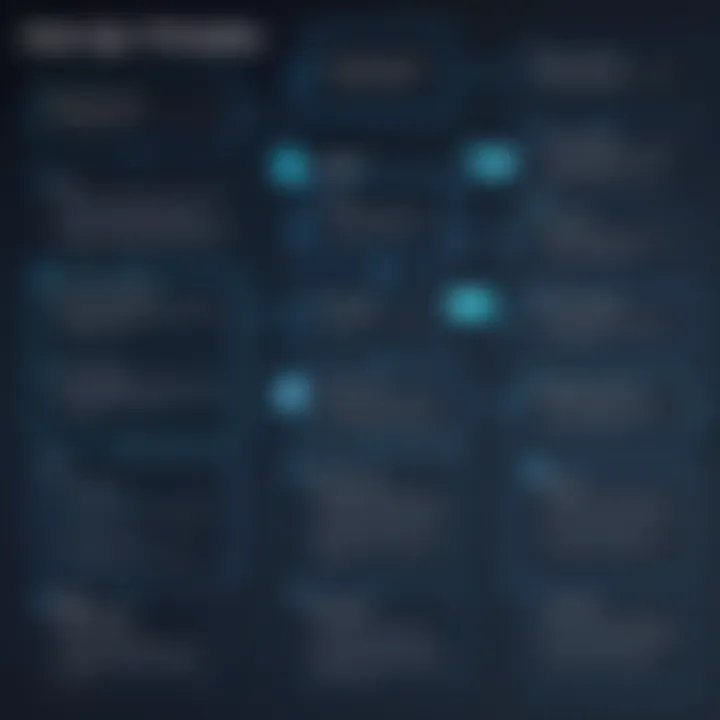
Due to tight interlinking of FXML, CSS, and Java, some developers find integrative design simultaneously simpler and more complex, depending on their experience with UI markup languages. However, its clear separation of UI can save time when teams work on different aspects of a project concurrently.
Compiling and Running the Program
When compiling and executing JavaFX applications, the process complements the Java Language as a whole. Similar to Swing, you can use the Java Compiler and Java Virtual Machine, but JavaFX also leverages several added libraries that simplify animations and transitions.
This dual compilation assurances system makes developing JavaFX highly responsive and interactive. Its extensive Built-in capabilities with scenes and animations are an advantage, allowing developers to enhance User experience effortlessly. However, increased complexity may arise, given the broader continual learning curve associated with managing animations.
Advanced GUI Components and Layouts
The design and functionality of a graphical user interface deeply rely on the use of advanced GUI components and layouts. In this section, we delve into the crucial aspects of these elements and their benefits in creating interfaces that are not only visually appealing but also user-friendly.
Working with Common GUI Components
Buttons
Buttons serve as the primary interaction tool in most applications. Their ubiquity is not without reason; they allow users to execute commands with a single click, enhancing user experience. A key characteristic of buttons is their intuitive nature. They signal an action that will be taken upon selection.
When we consider the design, buttons can be styled extensively. This ability makes them a beneficial choice for developers seeking to create unique interfaces. However, a unique feature of buttons is that their functionality must always be apparent; flashy designs can sometimes confuse the user about their purpose.
Advantages:
- Bolster user interaction.
- Adapt well to various styles and contexts.
Disadvantages:
- Overly creative designs risk losing clarity.
Text Fields
Text fields are essential for data input in any GUI application. They allow users to enter information, making them vital for forms, searches, and data entry interfaces. The key characteristic of text fields is their dynamic nature; they can expand to accommodate varying lengths of input.
Text fields are beneficial because they facilitate seamless user interaction with the underlying application. A unique feature is the integration of placeholder text which advises users on the expected input. Nevertheless, a drawback can be the limitations of validation; unless rigorously implemented, erroneous input can occur.
Advantages:
- User-friendly input method.
- Versatile across applications.
Disadvantages:
- Susceptible to entry errors without validation.
Checkboxes
Checkboxes are fundamental when collecting multiple selections or confirmations. Their defining feature is the binary choice that allows users the option to select or deselect each checkbox independently. This frequent utility makes them a popular choice in forms requiring choices.
Checkboxes encourage user engagement by providing clear visual feedback about selections made. However, they can clutter a simple UI if used excessively. Thoughtful application in forms can promote clarity and encourage accurate submissions.
Advantages:
- Efficient for multi-select tasks.
- Provides clear visibility of user choices.
Disadvantages:
- Overuse can lead to visual noise.
Menus
Menus play a critical role in user navigation within applications. They categorize functions, allowing users to explore multiple features without cluttering the main interface. Their hierarchical design is beneficial, ensuring the application remains organized and streamlined.
A defining aspect of menus is the instinctive browsing they encourage. Users can recognize and access commands readily. However, they may confuse users if the menu structure is inconsistent or too complex, spanning too many levels can frustrate rather than facilitate.
Advantages:
- Superior integration of multiple features.
- Condition users to easily browse functions.
Disadvantages:
- Can become overwhelming if poorly organized.
Layout Managers in Swing
FlowLayout
FlowLayout is a simplistic and flexible layout that arranges components in a left-to-right sequence. This ordering makes it naturally adept for dynamic GUI creation. Its key characteristic lies in the dynamic adjustment of components based on available space.
FlowLayout adapts easily across various display sizes, making it valuable for numerous applications. Its unique feature allows components to wrap as needed, though a downside is that it lacks stringent alignment features, which can impact the look of more complex layouts.
Advantages:
- Highly adaptable and easy to implement.
- Great for simple UIs.
Disadvantages:
- Limited control over precise placements.
BorderLayout
BorderLayout offers a well-defined structural approach by dividing the container into five regions: North, South, East, West, and Center. Its effectiveness in organizing components within these segments makes it a favorable choice for complex UIs. The major feature is that it can stretch components to occupy available space.
This flexibility supports full-screen strategies and dynamic adjustments. Still, its rigid design could pose challenges in terms of component placement ni more complex requirements than what its distributed nature can handle.
Advantages:
- Excellent for organized interfaces.
- Maximizes space use efficiently.
Disadvantages:
- Less control over individual component sizing and alignment.
GridBagLayout
GridBagLayout provides a powerful grid-based system allowing developers to align components in a customizable two-dimensional grid. Its flexibility is its main appeal. Developers can specify the position and size of each component precisely, sports high customization.
This layout excels in creating sophisticated interface designs; however, its complexity can lead to a steeper learning curve for new users. The detailed configuration may result in a tedious setup compared to more straightforward layout managers.
Advantages:
- Offers maximum control over layout structure.
- Highly suitable for complex interfaces.
Disadvantages:
- May be overly complex for simple applications.
JavaFX Layouts and Controls
VBox
VBox provides a vertical layout capable of stacking nodes in a single column. Its contribution to GUI design is pronounced as it enhances the organization of controls. The ability to uniformly space content gives it an edge in promoting simplicity and user experience.
An appealing rationale for its popularity is the ease at which developers can maintain spacing and alignment among contained items. However, it restricts layout to more vertical stacking, which can require added consideration based on the design goals of an application.
Advantages:
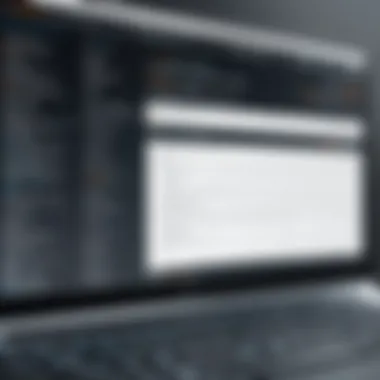
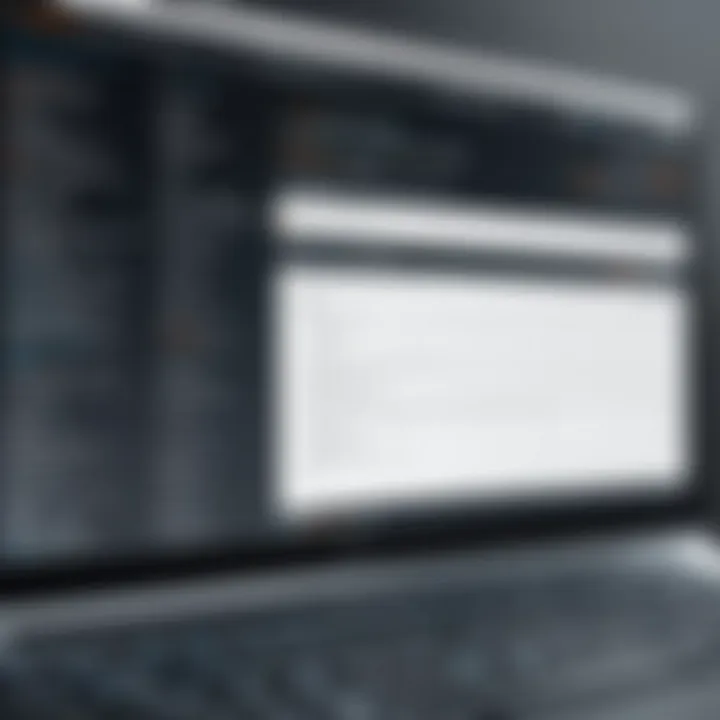
- Simplifies vertical layout management.
- Consistent alignment and spacing.
Disadvantages:
- Limited to a single orientation display.
HBox
Contrarily, HBox arranges its children nodes in a horizontal line. The significance of HBox is its function in creating appealing and intuitive horizontal layouts. This orientation can foster improved usability by emphasizing simultaneous options.
Adding to its allure is the facility for adaptive sizing, which adjusts arrangements to match content size dynamically. However, it may pose challenges for readability and accessibility baring trends in usage accessibility modules heeded in design.”
Advantages:
- Suitable for instances where grouped horizontal actions are functional and appeal.
- Handles resizing with ease.
Disadvantages:
- Readability can suffer with overcrowded side components.
GridPane
GridPane functions similarly to GridBagLayout but with an added benefit of its more natural integration with JavaFX controls. A comprehensive grid system allows sprawling configurations which lead to intricate designs across multi-dimensional sliders.
Its character lies in accommodating dense UI requirements; developers can define specific cells to house UI components seamlessly. A downside can be the confusion it introduces for managing cell properties surpassing more basic layouts.
Advantages:
- Highly versatile with regard to organizing complex content layouts.
- Encompasses a graphical representation enjoyed by users.
Disadvantages:
- Comprehending cell arrangement can be convoluted.
Event Handling in Java GUIs
Event handling is a crucial part of Java GUI development. It allows the user to interact with the application in real time, which enhances usability. Understanding how to manage events makes your application responsive to user actions like button clicks, keyboard input, and mouse movements. If done effectively, it leads to a smoother user experience.
Understanding Event-Driven Programming
Event-driven programming is the paradigm that guides GUI development in Java. In this model, the application responds to various events triggered by user actions. Each component, such as buttons or text fields, listens for user input. When an event occurs, an event object is created and sent to the appropriate handler.
This approach separates the user interface logic from the business logic, allowing for a cleaner and more maintainable codebase. Common types of events include ActionEvents for buttons, MouseEvents for mouse interactions, and KeyEvents for keyboard input. Understanding the lifecycle of these events is vital for creating responsive applications.
Implementing Action Listeners in Swing
In Swing, working with action listeners is fundamental for handling button clicks and other interactive elements. You must implement the interface, which defines the method.
For example:
This code demonstrates adding an to a button. When the button is clicked, it triggers the method, displaying a message in the console. It's pivotal to register the listener after creating the component to ensure it captures the action events.
Handling Events in JavaFX
In JavaFX, event handling operates similarly but offers a more streamlined experience. GUI components, called controls, can handle many types of events. All you need to do is attach an event handler to the control — either by setting it directly in the code or via FXML.
Here's an example using the JavaFX event handling mechanism:
In this example, the method attaches an event handler to the button, enabling it to respond when pressed. JavaFX abstracts much of the tedious listener implementation, allowing developers to focus more on application logic.
Efficient event handling is paramount for building robust and user-friendly applications. Mastering these aspects not only improves user experience but also enhances application performance.
Overall, understanding event handling is essential when developing Java GUIs, as it constitutes the backbone of interactive applications.
Debugging and Testing GUI Applications
Debugging and testing are critical components of GUI development in Java. This phase of the development process ensures that the application operates smoothly and meets quality standards. Given that user-friendly applications are highly reliant on functionalities working correctly, a robust debugging and testing strategy is indispensable.
Common Issues in GUI Development
Developers commonly encounter several issues when creating GUI applications. A key problem can involve responsiveness. Users expect intuitive and responsive interfaces. When a GUI is slow or unresponsive, it can lead to frustration. Other frequent issues include layout problems, such as components not displaying correctly or being misaligned.
Additionally, events not triggering may happen. For instance, if a button does not respond, users become confused about the action flow. Adequate UI validation is also essential, as users may input data incorrectly. Without proper validation, applications can crash or produce unexpected results.
To address these issues, developers should be vigilant during the application-building process. They must systematically test each component as it is created, focusing on input, output, and overall interactions. A methodical approach helps ensure that underlying issues don't cascade into larger, harder-to-solve problems.
Testing Strategies for GUI Applications
Implementing effective testing strategies is crucial for a successful GUI application. First, unit testing plays a significant role here. Each component or class of the application should be tested individually. Tools like JUnit are suitable for unit testing in Java, as they allow for straightforward setup and execution of tests.
Integration testing should follow, where components are tested together. This testing ensures components integrate seamlessly, allowing for holistic functioning. Importantly, automated testing can streamline this process significantly. Automated frameworks help simulate user actions, testing multiple scenarios efficiently.
User acceptance testing is another strategy, whereby end-users evaluate the application. Their interactions can offer insights overlooked by developers. Collecting feedback from users can pinpoint usability issues or desired features.
"A well-tested application fosters trust and enhances user satisfaction." - Anonymous
Each strategy carries its benefits but also considerations. It's crucial to have a blend of methodologies to ensure comprehensive coverage. No area in GUI testing is too small to review, as minutiae can often lead to substantial user impact. Thus, creating a meticulous testing framework is crucial for the long-term success of GUI applications in Java.
Best Practices for GUI Development in Java
For both beginners and seasoned developers, best practices serve as guiding principles that improve the overall effectiveness and user satisfaction of graphical user interfaces. Best practices in GUI development in Java are essential. They help ensure the application runs efficiently, remains maintainable, and meets the needs of users. Utilizing effective design practices also reduces the likelihood of user error and enhances usability across different platforms.
When developing a GUI, prioritize your structure and organization. This enables better collaboration among team members and comprehensive code reviews, which leads to quality production. Ultimately, following best practices boosts the credibility of the applications you develop, enhances user experience, and streamlines maintenance.
Code Reusability and Modularity
Code reusability focuses on writing software components that developers can reuse in different projects. This results in better utilization of resources, faster development times, and a reduction in potential bugs that come from writing new code from scratch.
When thinking practical, modularity becomes vital. Separating GUI components into distinct modules offers various benefits:
- Enhanced Readability: Code that is neatly arranged in specific modules is easier to understand. Comments can be included explaining the purpose and function of each module.
- Easier Debugging: Issues become simpler to identify when they are isolated within individual modules.
- Facilitating Collaboration: Individual developers can work on different modules concurrently, improving overall efficiency.
- Cost Efficiency: Developers save time since they can reuse established modules, reducing effort to recreate familiar functionality.
For Java developers creating GUIs, building classes like or increases the scope for reuse across other frameworks, enhancing the software life cycle. Libraries such as JComponent form the backbone for this practice, allowing generic aspects to fit within specific applications. Librarues commonly available to the Java community further promote this.
Optimizing Performance for GUI Applications
In designing Java GUI applications, performance is pivotal for user engagement. A snappy application improves the overall experience while prospective users typically abandon sluggish interfaces. Here are some key strategies to optimize performance:
- Reduce Resource Consumption : Avoid resource-heavy architectures. Use tools like lightweight controls to enhance speed.
- Handle Events Efficiently: Deal with events smartly. Consider applying event batching rather than addressing each event immediately.
- Utilize Caching: Storing expensive objects for quicker access minimizes processing time. Caching mechanisms drastically reduce repeated work, like rereading data.
- Optimize Redraw: In interfaces, hindering unnecessary screen redraws boosts response times.
- Asynchronous Operations: Benefit from using background tasks that handle lengthy processes, preventing the GUI from freezing during operations like loading large datasets.
To summarize, performance will heavily influence the reception of GUI applications. You can employ various methods available for Java, adjusting as needed based on project requirements. Analyzing your application's needs and optimizing accordingly will drive user satisfaction effectively.
Ending
The conclusion serves as a vital portion of the discourse on GUI development in Java, encapsulating key insights and providing direction for future endeavors in this area. A quality GUI is not just an aesthetic choice; it acts as a significant tool for engaging users. Ensuring users have a positive experience can lead to better retention rates and overall satisfaction
Recap of Key Learning Points
- Understanding GUI Fundamentals: Recognizing the role and importance of Graphical User Interfaces in software development.
- Java Frameworks: Knowing the distinctions and applications of both Swing and JavaFX for building robust user interfaces.
- Design Principles: Applying user-centered design methodologies and structure to create relatable interfaces that benefit end-users.
- Development Process: Familiarity with the steps of installation, coding, testing, and debugging applications to streamline workflows.
- Best Practices: Emphasis on modular code and performance optimization to create efficient applications.
Future Trends in Java GUI Development
Java GUI development is constantly evolving with new practices and technologies emerging. Here are some anticipated trends in this space:
- Increased Use of Libraries: Development frameworks are adapting more components and easing integration with modern cloud services.
- Focus on Web and Mobile Compatibility: More developers will focus on making applications that operate effectively across different domains, especially as users turn to various devices.
- Artificial Intelligence Integration: Automation will take a substantial role, allowing GUIs to adjust and predict user needs through learned behaviors.
- Continuous Framework Updates: Expect ongoing enhancements of frameworks like JavaFX contributing to modern visual architecture fostering richer user experiences.
Continue keeping abreast of these emerging practices to stay competitive in the realm of Java GUI development.
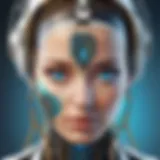
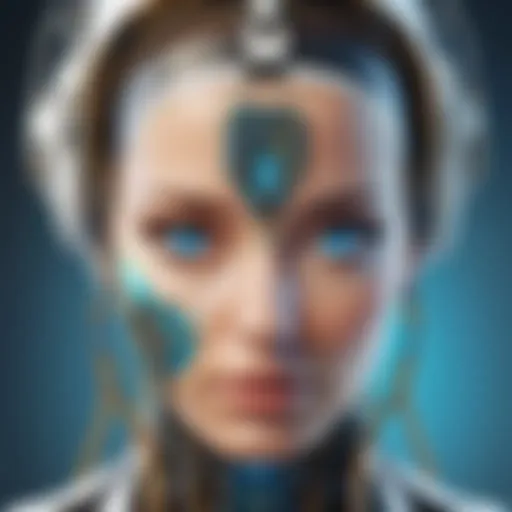