Comprehensive Guide to Basic Programming Languages
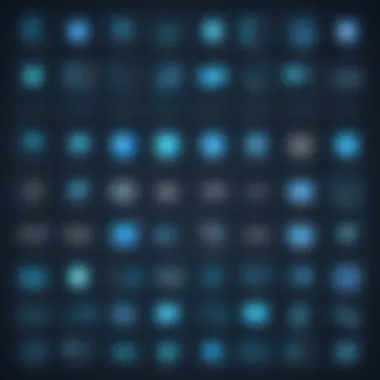
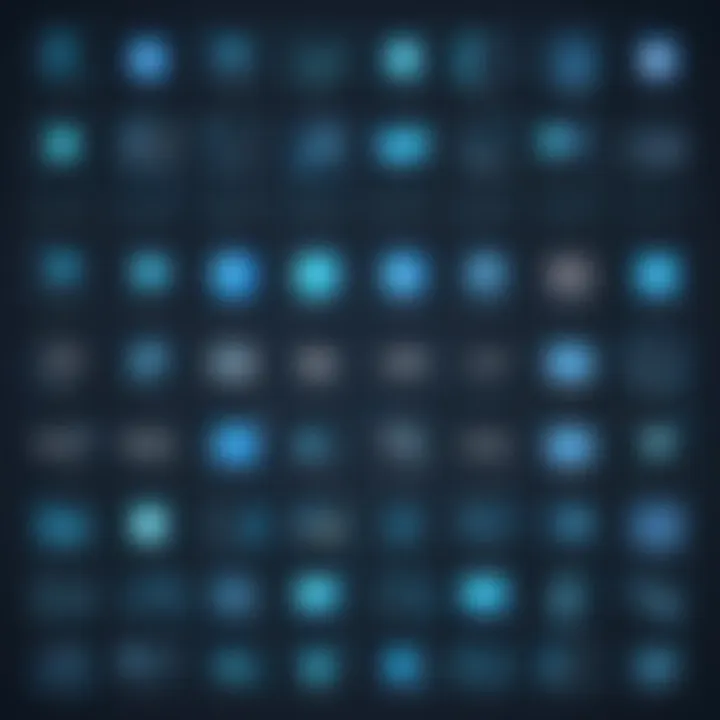
Overview of Topic
Preface to the main concept covered
The world of programming languages is vast and intricate. These languages act as a medium for humans to communicate instructions to computers. Through programming languages, we can develop software, build websites, and automate tasks. This article aims to provide an extensive overview of basic programming languages, focusing on those suitable for beginners and learners wishing to solidify their knowledge.
Scope and significance in the tech industry
In today's digital age, understanding programming languages is invaluable. Tech industry continues to grow rapidly, requiring individuals who can create and manage technology. Familiarity with programming languages not only enhances employability but also aids in solving problems efficiently. Furthermore, it serves as a gateway for those interested in pursuing a career in technology.
Brief history and evolution
Programming languages have evolved significantly since their inception. The first languages, like Assembly language, were complex and machine-specific. As technology progressed, languages such as Fortran and COBOL emerged, allowing for more accessibility and application in various sectors. Today, languages like Python, Java, and JavaScript dominate, each chosen for specific tasks, reflecting both the needs of developers and the demands of the industry.
Fundamentals Explained
Core principles and theories related to the topic
Understanding programming requires a grasp of several fundamental principles. These include concepts like algorithms, data structures, and the syntax of each programming language. Without these foundational elements, one cannot effectively write or understand code.
Key terminology and definitions
Several terms are crucial in programming.
- Variable: A storage location paired with an associated name.
- Function: A block of code designed to perform a particular task.
- Loop: A control structure for repeating actions.
Basic concepts and foundational knowledge
Beginners should focus on the basics such as writing simple programs, understanding how to print output, and learning how to accept user input. Grasping these concepts provides the groundwork for more complex programming tasks.
Practical Applications and Examples
Real-world case studies and applications
Programming has numerous applications in various industries. For example, Python is widely used in data science for analytics and artificial intelligence. Java is favored for building enterprise applications due to its robust and scalable nature.
Demonstrations and hands-on projects
To better understand programming languages, undertaking small projects is beneficial. For example:
- Creating a simple calculator using Python.
- Developing a simple website using HTML, CSS, and JavaScript.
Code snippets and implementation guidelines
Here is a basic Python code snippet to print "Hello, World!":
This simple example illustrates how commands are executed in Python and serves as a starting point for learners.
Advanced Topics and Latest Trends
Cutting-edge developments in the field
As technology advances, programming languages continue to evolve. The rise of machine learning and artificial intelligence has introduced new languages and frameworks. For instance, languages like R and Julia are gaining popularity for data analysis and scientific computing.
Advanced techniques and methodologies
Learning about Object-Oriented Programming (OOP) and Functional Programming can elevate a programmer’s skill set. These paradigms provide different approaches to solving problems and structuring code.
Future prospects and upcoming trends
The future of programming languages looks promising, with trends such as low-code development platforms and artificial intelligence integration shaping the landscape. Staying updated is crucial for aspiring programmers.
Tips and Resources for Further Learning
Recommended books, courses, and online resources
For those looking to deepen their knowledge, consider resources such as:
- Books: "Python Crash Course" by Eric Matthes
- Online Courses: Coursera, edX, and Codecademy offer excellent starting points.
Tools and software for practical usage
Familiarity with integrated development environments (IDEs) like Visual Studio Code and PyCharm can aid in learning programming effectively. Additionally, being aware of version control systems, such as Git, is beneficial for managing projects and collaborations.
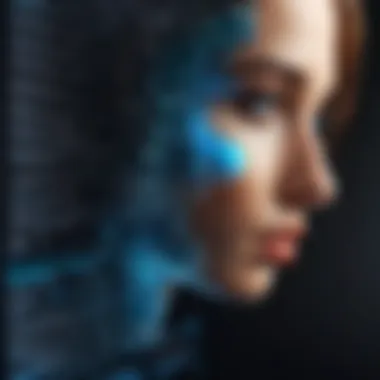
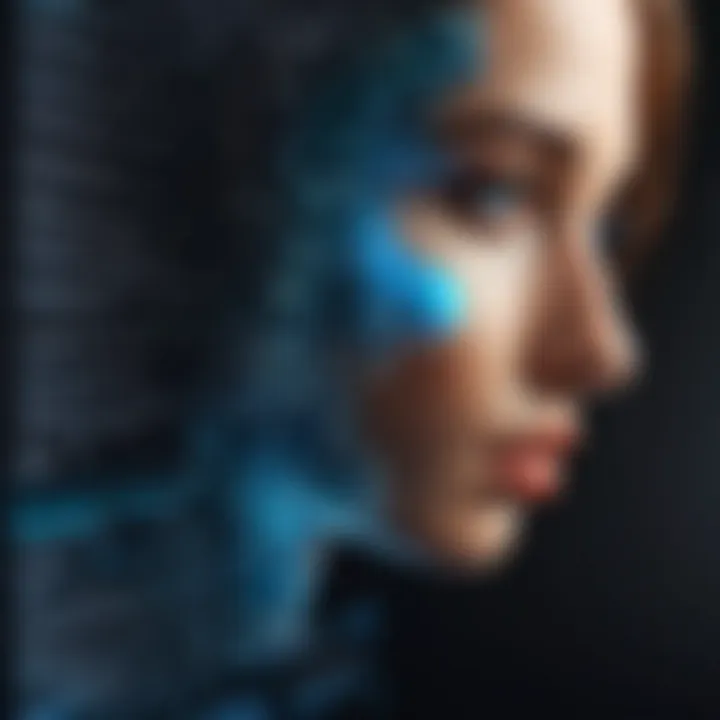
Prelude to Programming Languages
Understanding programming languages is essential, especially in today's technology-driven world. Programming languages serve as the foundation for all software development. They are critical for creating systems, applications, and websites. Each language possesses unique features that cater to various needs and industries.
Importance of Programming Languages
Programming languages are important because they translate human commands into machine-readable instructions. This interaction shapes virtually every aspect of modern technology. Whether a novice or an experienced developer, knowing programming languages allows people to engage deeply in tech-related projects. Moreover, they enhance problem-solving skills and logical thinking.
Definition and Importance
A programming language is a set of rules and syntax used for instructing computers to perform specific tasks. Each language has its own syntax, semantics, and structure, making it suitable for different applications. For example, Python is often favored for its readability, while Java is renowned for its portability across platforms.
Learning a programming language equips individuals with the ability to communicate with computers effectively. This leads to not only job opportunities but also the potential to innovate and automate processes in various domains.
The significance of these languages is not just in coding but in affecting everyday life. From basic website functionality to complex data analysis, every piece relies on effective programming languages.
Historical Context
The evolution of programming languages dates back to the early 1950s. The first high-level language, Fortran, emerged, allowing more straightforward programming of complex scientific computations. Shortly after, COBOL appeared, aimed at business applications. Each new language often addressed limitations in its predecessors, contributing to more efficient coding practices.
The 1980s saw the development of C and its derivatives, laying the groundwork for many modern languages like C++, Java, and C#. The continued evolution reflects the shift toward object-oriented programming, emphasizing code reusability and organization.
In recent years, languages like Python and JavaScript have gained popularity due to their versatility and ease of learning. Such growth exemplifies how programming languages adapt to the needs of developers and the demands of the industry. This context shapes a foundational understanding of programming languages today and prepares learners for future developments.
Understanding Basic Programming Concepts
Understanding basic programming concepts is essential for anyone embarking on a journey in programming. These concepts form the bedrock upon which all programming languages are built. This section will delve into important elements such as syntax and semantics, variables and data types, as well as control structures. Mastering these foundational ideas not only enhances one’s coding skills but also fosters the ability to learn new programming languages efficiently.
Syntax and Semantics
In programming, syntax refers to the set of rules that define the structure of statements in a language. Each programming language has its own unique syntax. For instance, Python uses indentation to indicate code blocks, while Java relies on braces. Proper syntax is crucial because even minor errors can lead to bugs or compilation failures.
Semantics, on the other hand, deals with the meaning of these statements. Understanding the semantics allows programmers to write code that not only runs correctly but also performs the intended operations. For example, using an incorrect operator may result in logical errors, leading to unexpected outcomes. Clear comprehension of both syntax and semantics is vital, as it allows learners to communicate effectively with the computer through code while ensuring that the code operates as intended.
Variables and Data Types
Variables act as storage containers for data in programming. They enable programmers to write flexible code by storing values that may change during program execution. For instance, a variable can hold a user's input or a calculated result. Choosing appropriate variable names improves code readability and helps in maintenance.
Data types define the kind of data a variable can hold. Common data types include integers, floating-point numbers, characters, and strings. Understanding data types is essential because it influences memory allocation and performance. For example, misusing data types may lead to type errors or inefficient memory usage.
Control Structures
Control structures direct the flow of execution within a program. They determine how and when certain parts of code are executed based on specific conditions. The most common control structures are conditional statements and loops.
- Conditional statements allow programmers to execute certain sections of code based on whether a condition evaluates to true or false. Common examples include , , and statements.
- Loops, such as and , enable repetitive execution of code until a certain condition is met.
These structures increase a program's efficiency and sophistication, enabling programmers to implement complex features and interactive functionality.
Overall, understanding these basic programming concepts cultivates a strong foundation for further exploration of programming languages and enhances problem-solving skills. Mastery of syntax and semantics, familiarity with variables and data types, and adeptness in control structures prepare learners for tackling more advanced topics in programming.
Overview of Key Programming Languages
Understanding various programming languages is essential for anyone venturing into the realm of software development. Each language possesses unique characteristics, strengths, and weaknesses that dictate its suitability for particular tasks. By exploring key programming languages, learners become better equipped to make informed decisions on which tools best serve their projects.
Importance of Programming Languages
Programming languages act as the medium through which developers communicate with computers. A well-chosen language enhances productivity and allows for efficient problem-solving. The significance of programming languages is not just academic; it has real-world implications in technology, employment, and innovation.
Python
Python is often the first choice for beginners. Its simple syntax promotes readability and reduces the cognitive load on new learners. Widely used in data science, web development, and automation, Python’s versatility sets it apart. Libraries like NumPy and Pandas complement Python's capabilities, making it a powerful tool for analysis and computation.
JavaScript
JavaScript is indispensable for web development. It brings interactivity to web pages, allowing developers to create dynamic user experiences. With frameworks like React and Node.js, JavaScript has expanded beyond browsers, facilitating full-stack development. Its event-driven nature and non-blocking I/O model offer performance advantages for applications that require real-time user interactions.
Java
Java is a statically typed language known for its portability across platforms. The principle of "write once, run anywhere" has made Java a staple in enterprise applications. Its rich ecosystem, with a vast selection of libraries and frameworks such as Spring and Hibernate, enhances Java's functionality. Additionally, the emphasis on object-oriented programming fosters good design practices.
and ++
C and C++ are foundational languages in computer science. C is lauded for its performance and procedural programming base. Meanwhile, C++ adds features such as classes and object-oriented principles, making it suitable for complex systems. Both languages allow for low-level memory manipulation, making them ideal for system programming and developing high-performance applications.
Ruby
Ruby stands out for its focus on simplicity and productivity. The syntax is elegant and expressive, allowing developers to write less code while achieving more. Ruby on Rails, a widely used web application framework, exemplifies how Ruby simplifies web development. Its emphasis on convention over configuration accelerates the development process without sacrificing flexibility.
"Choosing the right programming language is a crucial step that can significantly affect the success of a project."
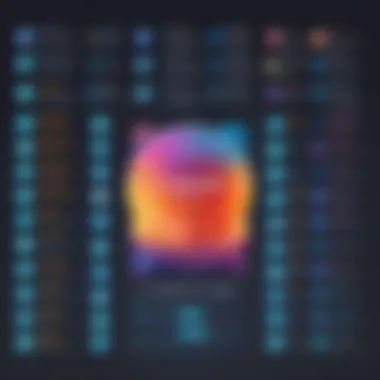
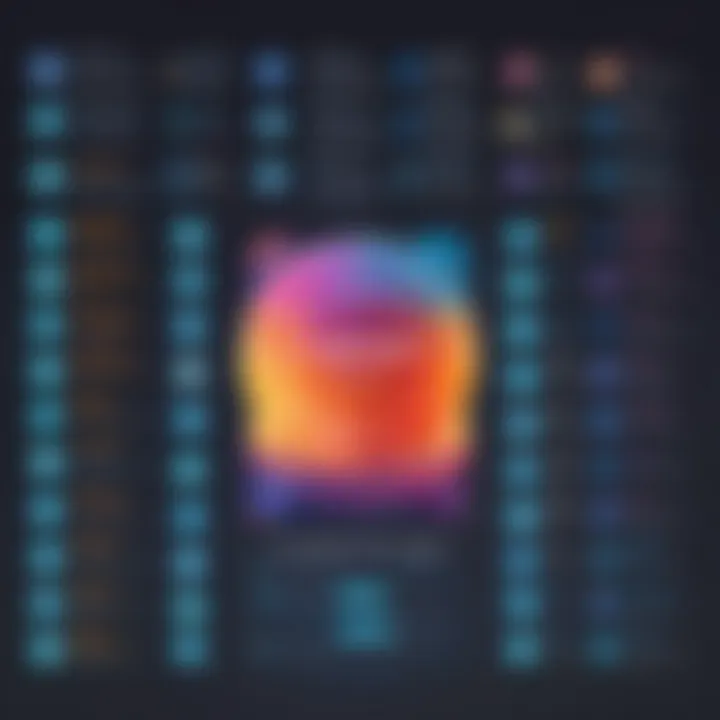
By examining these programming languages, learners gain insight into their various uses andCapabilities. Each language presents unique opportunities and challenges, understanding these can inform a learner's journey in programming.
Selecting the Right Programming Language
Choosing a programming language is a significant decision for beginners and seasoned developers alike. It can influence not only the learning curve but also the types of projects one can effectively undertake. With so many languages available, each with its unique strengths and weaknesses, understanding how to select the right one is crucial. This section explores various factors to consider and outlines common applications for each programming language, allowing individuals to align their choice with their specific goals and needs.
Factors to Consider
When deciding on a programming language, several factors come into play:
- Personal Goals: Begin by defining your objectives. Are you interested in web development, data analysis, software engineering, or mobile app creation? Your chosen field can greatly influence which language is most suitable.
- Ease of Learning: Some languages, like Python, are known for their straightforward syntax and readability. This can be an advantage for beginners. In contrast, languages such as C++ may offer more complexity, requiring a steeper learning curve.
- Community Support: A vibrant community can provide vital assistance when learning. Languages like JavaScript and Python benefit from extensive online forums, resources, and documentation, which can aid in your understanding and troubleshooting.
- Industry Demand: Research the job market to understand which languages are most sought after. For example, Java and JavaScript continue to show strong demand in tech industries, while newer languages like Rust and Go are gaining traction.
- Performance Needs: If performance is a critical factor, languages like C and C++ often excel due to their efficiency. On the other hand, languages like Python are slower but offer rapid development capabilities for less performance-intensive applications.
These considerations will vastly influence not just the learning process but the practical outcomes of your work.
Use Cases and Applications
Different programming languages serve various purposes.
- Python: Often used for web development, data analysis, machine learning, and automation. Its versatility makes it a go-to choice for many startups and enterprises alike. Python’s libraries, such as Pandas and NumPy, are essential for data-centric tasks.
- JavaScript: Critical for front-end web development. It enables interactive elements on websites and is also used on the server-side with frameworks like Node.js. JavaScript is a must for those looking to develop modern web applications.
- Java: Known for its portability across platforms, Java is commonly used in enterprise environments. It powers a wide range of applications, from mobile applications on Android to large-scale web applications.
- C and C++: These languages are often used in systems programming, game development, and applications where performance is paramount. They allow for low-level memory management, making them optimal for resource-intensive applications.
- Ruby: Primarily known for web development, especially with the Ruby on Rails framework. Ruby’s syntax is elegant and straightforward, making it a popular choice for startups.
By understanding these applications and the context in which languages thrive, you can make informed decisions that align with your career aspirations.
Remember: The right programming language can open doors to new career opportunities and enhance productivity in projects.
Learning Methods and Resources
Learning programming languages is a multifaceted endeavor. Understanding the various learning methods and resources available can greatly enhance one’s coding journey. This section dives into the significance of these methods, explores formal education, online courses, and literature that shape programming competency.
Formal Education
Formal education remains a traditional and structured approach to learning programming. Universities and colleges offer computer science degrees that cover programming languages, software engineering, data structures, and algorithms. The advantages of this route include access to experienced instructors, networking with peers, and opportunities for internships.
However, there are considerations for potential students.
- Cost: Tuition fees can be substantial.
- Time: Completing a degree typically takes several years.
Despite these drawbacks, a degree can significantly bolster one’s resume in a competitive job market. It provides a heavy foundation in theoretical concepts, which can be critical for advanced understanding.
Online Courses and Tutorials
The rise of online education has transformed how learners approach programming. Platforms such as Coursera, Udemy, and edX offer a plethora of courses tailored to all skill levels.
Benefits of online learning include:
- Flexibility: Students can learn at their own pace and schedule.
- Variety: A wide array of languages and frameworks are covered, from Python to React.
- Affordability: Many courses are free or low-cost compared to traditional education.
It's crucial to choose reputable sources when selecting online tutorials. Community reviews and ratings can help determine course quality. Additionally, interactive platforms like Codecademy provide hands-on exercises, which can augment theoretical learning.
Books and Literature
Books remain an essential resource for many programmers. They offer in-depth knowledge and can serve as reference guides. Notable texts such as "The Pragmatic Programmer" by Andrew Hunt and David Thomas or "Clean Code" by Robert C. Martin delve into principles of writing efficient code and improving one’s programming style.
Considerations for selecting books include:
- Level of expertise: Some books target novices while others are written for advanced programmers.
- Current relevance: Technology evolves quickly; ensure that the material is up-to-date.
Moreover, documentation and official guides provided by programming languages like Python's or Java's official sites are invaluable for learners seeking accuracy and clarity.
Practical Applications
Understanding practical applications of programming languages is vital. The purpose of programming extends beyond theoretical knowledge; it involves creating tangible solutions in various fields. Practical applications provide insights into how learned programming concepts can be utilized in real-world scenarios. They also enable learners to connect their coding skills to functional projects, yielding tangible outcomes.
Incorporating real-world projects and hands-on exercises helps reinforce knowledge, making it memorable and applicable. These applications encourage experimentation and foster problem-solving abilities, which are crucial for any aspiring programmer.
Moreover, engaging in practical applications strengthens understanding of programming paradigms. It assists learners in navigating complexities of coding challenges, eventually boosting their confidence in tackling larger projects.
"Engaging in real-world scenarios allows learners to apply abstract principles, enhancing retention and comprehension on a deeper level."
Real-world Projects
Real-world projects exemplify programming utility across various industries. These projects often mimic challenges professionals face, providing learners with a taste of actual job demands. Engaging in these projects enhances critical thinking and fosters creativity.
Some examples of real-world projects include:
- Web Development: Creating personal or business websites using HTML, CSS, and JavaScript can illustrate how websites function and how user interaction can be enhanced.
- Data Analysis: Leveraging Python or R for analyzing datasets can help demonstrate how programming plays a key role in drawing insights from data.
- Mobile Apps: Developing applications for iOS using Swift or Android using Kotlin teaches necessary skills in a growing field.

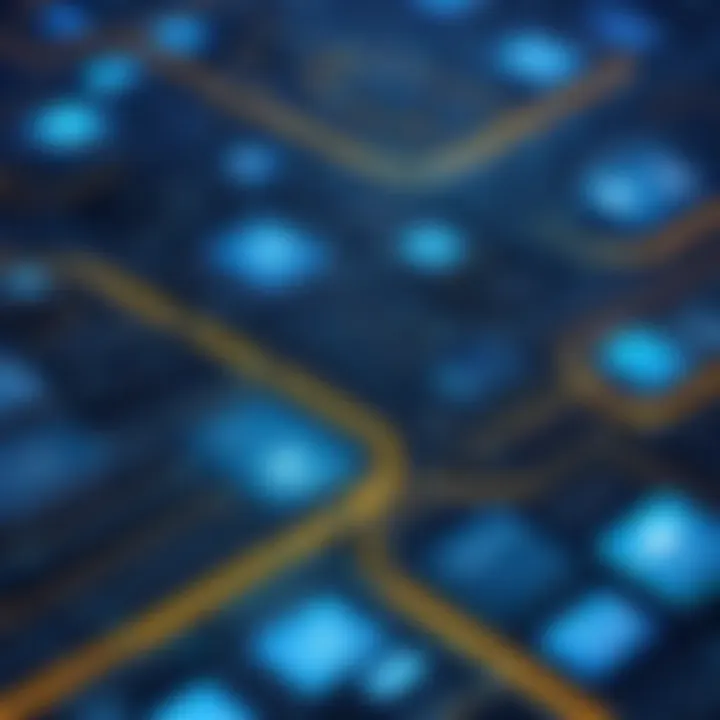
Each of these examples highlights the importance of theory in practice. By immersing themselves in real projects, learners discern how their skills contribute to solving real problems, reinforcing both their knowledge and their interest in programming.
Hands-on Exercises
Hands-on exercises complement theory by offering a practical dimension to learning programming languages. These exercises are designed to challenge learners and ensure they can translate theoretical concepts into practical skills. Regular practice through exercises instills confidence as learners begin to see their progress.
- Code Challenges: Websites like LeetCode and HackerRank provide opportunities to solve coding problems ranging from beginner to advanced levels. These challenges sharpen analytical skills and familiarity with various programming constructs.
- Mini-Projects: Completing small projects, such as a to-do list application or a simple game, provides a sense of accomplishment and makes concepts more tangible.
- Collaborative Coding: Participating in platforms like GitHub allows learners to work on real projects with others. Understanding version control is essential for professional development.
Hands-on exercises also serve to identify gaps in knowledge. When learners encounter difficulties or errors, it prompts them to seek solutions, deepening their understanding. Overall, practical applications through real-world projects and hands-on exercises are essential for cementing programming knowledge and skills.
Common Challenges in Learning Programming
Learning programming is not just about acquiring technical skills; it also involves navigating various challenges that can hinder progress. Recognizing these common challenges is vital as it helps learners prepare and adapt their strategies effectively. The complexities of programming can be overwhelming, but understanding the difficulties can foster resilience and improve learning outcomes.
Cognitive Load
Cognitive load refers to the amount of mental effort being used in the working memory. In the context of programming, this concept becomes crucial for learners. Beginners often face a high cognitive load when trying to grasp new concepts, syntax, and logic simultaneously. When confronted with multiple new ideas, such as variables, loops, and functions, it can become difficult to retain information.
To manage cognitive load, learners should break tasks into smaller, more manageable units. Here are some strategies that can help reduce cognitive overload:
- Prioritize Learning Objectives: Focus on one concept at a time, rather than trying to learn everything at once.
- Utilize Visual Aids: Diagrams and flowcharts can simplify complex logic and help with understanding structures and processes.
- Practice Regularly: Regular hands-on exercises reinforce understanding and memory retention.
By implementing these strategies, learners can mitigate cognitive load and process information more effectively.
Debugging Issues
Debugging is an integral part of programming, yet it often frustrates beginners. Debugging refers to the process of identifying and fixing errors in code, which can be a daunting task, especially for novices. The common belief that errors signify failure can discourage learners. However, it is essential to view debugging as a natural phase of the coding process.
Common issues beginners face in debugging include:
- Syntax Errors: These are typos or mistakes in the code that violate the programming language's rules. They are usually straightforward to fix once discovered.
- Logical Errors: These occur when the code runs without crashing, but the output is not what was expected. Identifying the source of these errors can require more critical thinking and analysis.
- Runtime Errors: These errors happen during execution, often due to unforeseen conditions like invalid input.
"Every programming error is an opportunity to learn. Embrace debugging as a valuable part of the journey."
To enhance debugging skills, learners should develop a systematic approach:
- Read Error Messages Carefully: Error messages often provide clues about what's wrong.
- Divide and Conquer: Isolating code blocks can help identify where the issue lies.
- Use Debugging Tools: Integrated Development Environments (IDEs) offer debugging functionalities that can simplify this process.
Career Opportunities in Programming
The field of programming presents an array of enticing career opportunities. As technology continues to evolve, the demand for skilled programmers grows proportionally. This section explores various job roles within the programming domain, outlines their responsibilities, and provides insights into industry demand. Understanding these elements is crucial for anyone considering a career in programming, as it can guide their education and training choices.
Job Roles and Responsibilities
In the realm of programming, multiple roles exist, each with distinct responsibilities. Here are some of the prominent positions:
- Software Developer: Develops applications and systems software. They design, code, test and maintain software solutions to meet user needs.
- Web Developer: Specializes in building websites and web applications. Their focus is on both the front-end user interface and the back-end server-side application.
- Mobile Application Developer: Focuses on creating apps for mobile devices. They must be familiar with platforms such as Android and iOS, using languages like Java or Swift.
- Data Scientist: Uses programming skills to analyze complex data. They create algorithms and models to extract meaningful insights which help businesses make informed decisions.
- DevOps Engineer: Merges development and operations by automating the development lifecycle. They ensure continuous integration and continuous deployment practices.
Each role requires a different set of skills and specializations. Learning programming languages relevant to these positions is essential. For example, knowing Python may benefit a data scientist, while familiarity with JavaScript might be more relevant for a web developer.
Industry Demand
The tech industry has a strong and continually growing appetite for skilled programmers. According to various reports, sectors such as finance, healthcare, and e-commerce heavily depend on programmers to streamline operations and enhance user experiences. Some key points regarding industry demand include:
- Job Availability: The programming job market is expected to grow significantly in the coming years. According to the U.S. Bureau of Labor Statistics, the employment of software developers is projected to grow by 22% from 2020 to 2030, much faster than the average for all occupations.
- Diverse Industries: Almost every industry now relies on technology, which increases the need for programming professionals. From creating dynamic websites for businesses to developing sophisticated algorithms in healthcare, opportunities abound.
- Freelancing Opportunities: Many businesses seek freelance programmers for specific projects. This creates potential for individuals to work independently, focusing on jobs that match their skills and interests.
"Programming is not just about writing code; it's about problem-solving and creating solutions that impact lives."
Ending
In the realm of programming, the conclusion serves as a vital component that summarizes the journey through the intricacies of basic programming languages. It is essential to reflect on the significance of the knowledge gained and the paths that lie ahead. The role of a conclusion is not merely to wrap up the discussion; it encapsulates the learning experience and reinforces key insights acquired throughout the article.
The conclusion highlights several important elements discussed in the sections above. One aspect is the understanding of different programming languages and their applications. By gaining insight into languages like Python, JavaScript, and Ruby, learners can match their skills with real-world needs. Moreover, recognizing the learning methods available—whether through formal education, online courses, or books—empowers students to choose the best approach suited to their circumstances.
Additionally, the common challenges of learning programming and the career opportunities available in this rapidly evolving field are another focal point. Being aware of potential hurdles, such as cognitive load and debugging issues, prepares aspiring programmers for the realities of the discipline. At the same time, understanding job roles and industry demand illuminates the paths available after mastering these languages.
Altogether, the conclusion strengthens the minds of learners about programming. It sets a thoughtful tone for ongoing development and encourages the pursuit of further learning, ensuring that readers are well-equipped to explore the programming landscape.
Recap of Key Points
- The importance of programming languages in today’s digital ecosystem cannot be overstated. They serve as the foundation for software development, web development, and more.
- Each discussed programming language has unique properties that cater to various applications, from general-purpose utilization to specific domain needs.
- Learning methods vary significantly, from traditional classroom learning to self-directed online studies.
- Understanding the common challenges faced during learning provides practical insights into overcoming obstacles.
- Awareness of career opportunities in programming is crucial for aligning skills with market demands.
Encouragement for Continued Learning
The tech industry is dynamic. Therefore, continuous learning is key also to success in programming. As new languages, tools, and frameworks emerge, programmers must adapt. This adaptive learning helps to stay relevant and competitive.
Engaging with online communities, attending workshops, and reading current literature can greatly enhance knowledge. Platforms like Reddit and educational sites offer resources for self-study.
Setting personal goals can also foster motivation. Whether aiming to contribute to open-source projects or improving specific skillsets, having clear objectives can guide the journey ahead. Remember, the world of programming is vast, and the quest for knowledge is never-ending.
"Programming is not just about writing code; it is about solving problems and creating solutions that impact the world."
In summary, the conclusion does not signify an end. Rather, it marks a new beginning in the programmer's journey, where each step forward brings them closer to mastery.
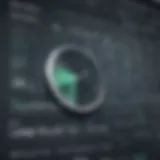
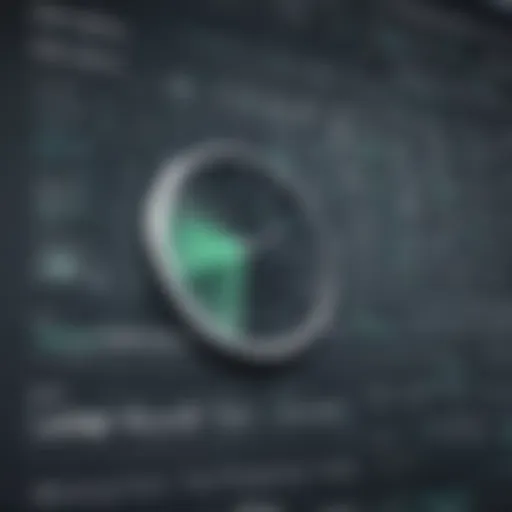