Comprehensive Guide to Learning Ruby Programming
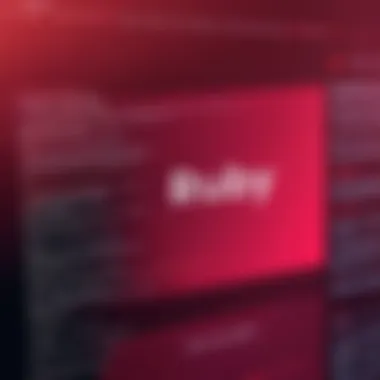
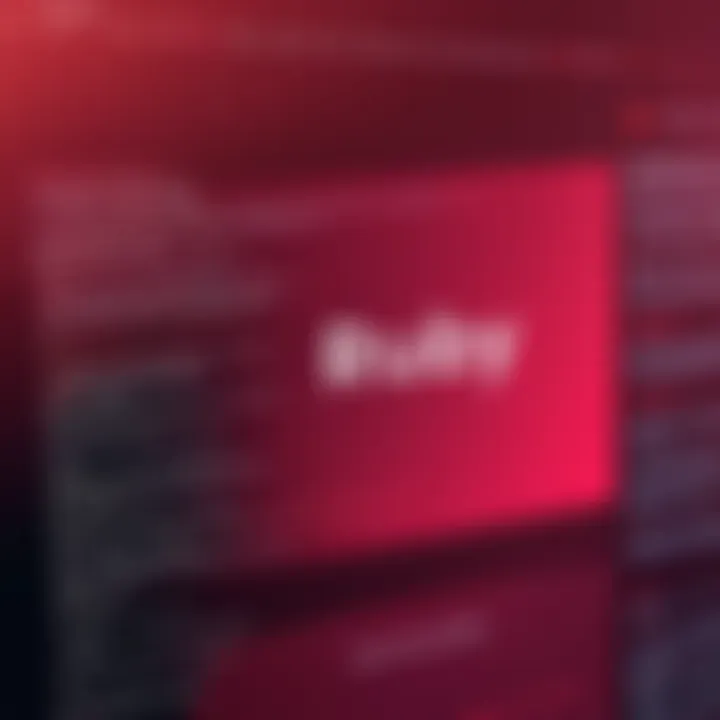
Overview of Topic
Prelude to the Main Concept Covered
Ruby is often described as a language that prioritizes simplicity and productivity. For students and budding IT professionals, understanding Ruby can open doors to various career opportunities in software development. It's a versatile programming language known for its elegant syntax, making it easy to read and write compared to other types of code.
Scope and Significance in the Tech Industry
Given the rapid pace of advancements in technology, Ruby holds a significant place in the industry, particularly in web development. Frameworks such as Ruby on Rails have empowered developers to create robust applications in a fraction of the time, elevating Ruby's status as a staple in the programming world.
Brief History and Evolution
Ruby was created in the mid-1990s by Yukihiro Matsumoto, with the desire to combine parts of his favorite languages: Perl, Smalltalk, Eiffel, and Ada. This mix aimed to produce a language that is both powerful and enjoyable to use. Over the years, Ruby has evolved significantly, bringing in new features and libraries that keep it relevant in today's tech scene.
Fundamentals Explained
Core Principles and Theories Related to the Topic
At its heart, Ruby embodies object-oriented programming principles. Everything in Ruby is an object, which is a unique concept for new programmers. This allows for methods and properties to be easily manipulated, fostering a strong grasp of programming logic.
Key Terminology and Definitions
As you start learning Ruby, it's crucial to familiarize yourself with its unique terminology. Here are a few key terms:
- Object: An instance of a class that holds data and methods.
- Class: A blueprint from which objects are created.
- Method: A set of codes that perform a specific task.
Basic Concepts and Foundational Knowledge
To get started, learners should grasp basic concepts like data types, variables, and control structures. For instance, understanding how to use arrays and hashes is essential, as they are commonly utilized in everyday programming tasks. Knowing how to control program flow with loops and conditionals is equally important.
Practical Applications and Examples
Real-World Case Studies and Applications
Many companies leverage Ruby for their projects. Airbnb is a prime example, having grown its platform using Ruby on Rails. This showcases its capability to support scalable applications. Other organizations like Github and Shopify also illustrate Ruby’s substantial benefits in the web development arena.
Demonstrations and Hands-On Projects
Engaging in hands-on projects can significantly aid in the learning process. For a beginner, building a simple command-line calculator is a practical exercise. This project can help solidify grasp of methods, input/output functions, and basic arithmetic operations.
Code Snippets and Implementation Guidelines
Here’s a simple code snippet illustrating a method in Ruby that adds two numbers:
Advanced Topics and Latest Trends
Cutting-Edge Developments in the Field
As Ruby progresses, new libraries and frameworks emerge. For example, Hanami is gaining attention as a lightweight alternative to Ruby on Rails, providing a more modular approach to web development.
Advanced Techniques and Methodologies
Those seasoned in Ruby often explore metaprogramming, a technique that allows the programmer to write code that can modify itself. Understanding this can unveil a whole new layer of Ruby, allowing you to craft more flexible and reusable code.
Future Prospects and Upcoming Trends
With the rising trend of microservices, Ruby may further adapt to support such architectures, merging with tools like Docker for improved application deployment and scaling.
Tips and Resources for Further Learning
Recommended Books, Courses, and Online Resources
For learners looking to deepen their Ruby knowledge, consider resources like:
- "The Well-Grounded Rubyist" - a solid foundation in Ruby principles.
- Online platforms like Codecademy or Udemy provide interactive courses.
Tools and Software for Practical Usage
Familiarity with tools like RubyMine, Atom, or VS Code can enhance coding efficiency and productivity. These environments cater to Ruby’s intricacies, providing rich features for debugging and testing.
Learning Ruby is not just about mastering syntax; it’s about understanding the underlying principles that govern programming.
With this guide, readers should feel confident in embarking on their journey with Ruby, leveraging the comprehensive insights provided to navigate the world of programming with clarity and purpose.
Preamble to Ruby Programming
The realm of programming languages is vast, with options that vary vastly in syntax, application, and user experience. Among these, Ruby stands out not just for its functionality but also for its elegant simplicity. Understanding Ruby programming is like unraveling a novel with rich character development—each element contributes to the grand narrative of software development today.
History of Ruby
Ruby was conceived in the mid-1990s by Yukihiro Matsumoto, whose vision was to create a language that blended parts of his favorites: Perl, Smalltalk, Eiffel, and Ada. This blend aimed to produce a language that was both powerful and easy to use, making programming accessible to a broader audience. The first public release occurred in 1995, marking the beginning of a journey that would soon gain traction not just among hobbyists but also within the corporate environment.
As Ruby evolved, so did its community. Many contributors, including the developers of Rails—a web framework that became a game-changer—drove Ruby into the limelight. Their input forged a landscape where quick development cycles could coexist with robust, maintainable applications. This evolution highlights an essential aspect of Ruby: it is not just a tool; it reflects a philosophy of making programming more enjoyable.
Importance of Ruby in Modern Development
In the fast-changing world of software development, Ruby has carved its niche, especially in web development. One of the language's most significant advantages lies in its expressiveness. Developers can write less code to achieve more functionality, which translates to faster project delivery—definitely a win-win for companies operating in competitive markets.
Apart from web applications, Rails' conventions and best practices have fostered a culture emphasizing maintainability, something that resonates with tech teams eager to avoid technical debt. Moreover, Ruby provides rich libraries and frameworks, empowering developers to leverage pre-built solutions instead of reinventing the wheel.
Here are a few reasons why Ruby remains relevant today:
- Community Support: The Ruby community is incredibly active, providing continuous updates and resources.
- Versatility: From web apps to data processing, Ruby's applications are diverse.
- Learning Curve: For newcomers, Ruby offers an approachable syntax, making it easier to grasp core programming concepts.
"Ruby is all about making programming fun and accessible."
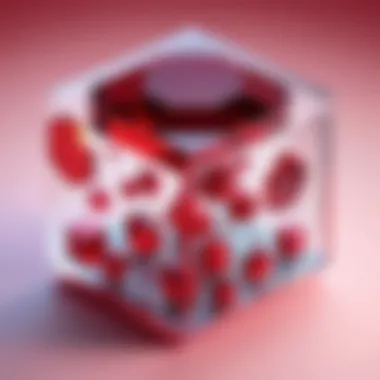
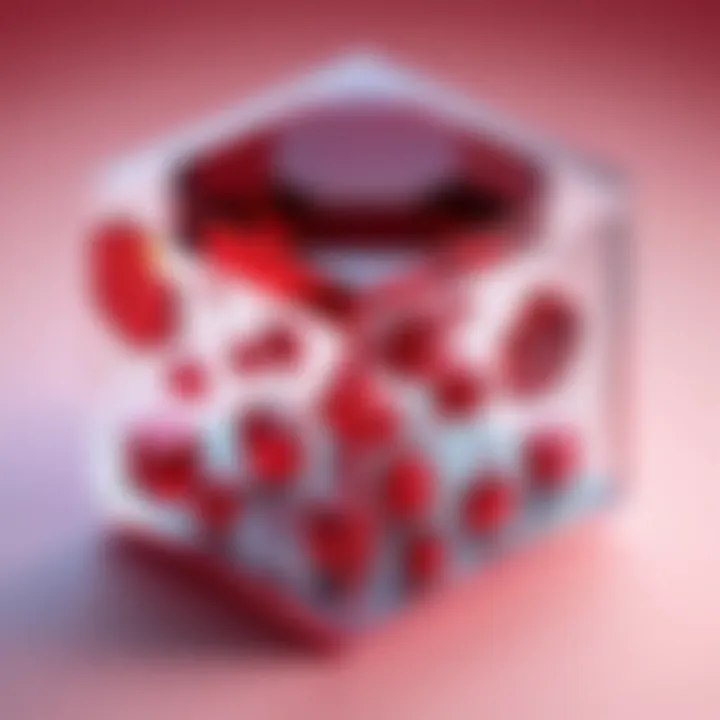
Setting Up the Development Environment
Setting up the development environment is one of the most crucial steps when diving into Ruby programming. This phase involves gathering the essential tools and configuring them appropriately, paving the way for a smoother coding experience. When you have a properly set up environment, it can reduce the friction between your intent and execution. With an array of choices available, it's important to weigh the options carefully. A well-structured environment not only enhances productivity but also minimizes errors. Here’s what you need to know.
Choosing a Text Editor
The text editor is your primary interface with code. Choosing the right one might feel daunting, but it's a personal journey. Consider your comfort level and the features you need. Popular options for Ruby programming include:
- Visual Studio Code: Highly customizable, with a vast library of extensions.
- Sublime Text: Known for its speed and ease of use.
- Atom: Developed by GitHub, it’s open-source with a friendly community.
Each of these editors has its strengths. For instance, if you appreciate extensive customization, Visual Studio Code might be your cup of tea, while Sublime Text provides a straightforward, distraction-free atmosphere. Make sure to explore different themes and plugins to tailor the editor to your preferences.
A well-chosen text editor can serve as a second skin. If you're comfortable using it, you'll find your coding flow much more effective.
Installing Ruby
Once you've settled on a text editor, the next stop is installing Ruby itself. Whether you're on Windows, macOS, or Linux, the installation process has been streamlined significantly over the years.
- Windows Users: Utilize RubyInstaller. Download and install it, ensuring to check the box to add Ruby to your PATH.
- macOS Users: Open the Terminal and use Homebrew by running the command:
- Linux Users: Most distributions have Ruby available via package managers. For Ubuntu, use:
After installation, it’s always a good idea to verify
This command will display the Ruby version installed, confirming that everything went off without a hitch. Make sure that your environment variables point correctly to Ruby’s executable file, as this can often be a source of confusion.
Managing Gems and Dependencies
Ruby's ecosystem is robust and vibrant, primarily due to the concept of gems. Gem management is essential because it allows developers to incorporate existing libraries, avoiding the need to reinvent the wheel for every project.
- Bundler: This is the most popular tool for managing dependencies in Ruby. It ensures that the right gems and versioning are installed, keeping projects organized. To install Bundler, run:
- Gemfile: This file specifies which gems your project depends on. It’s the heart of Bundler operations. After creating a , run:
With the right gems, your Ruby projects can be powerful and fully functional, saving time and effort. Your local development environment can be a breeding ground for productivity when managed effectively.
Understanding Ruby Syntax
Understanding Ruby syntax is the cornerstone to becoming proficient in Ruby programming. It forms the foundation upon which all Ruby applications are built. Mastering the basics of syntax allows programmers to write clear and efficient code, ultimately leading to better software development practices. In addition, knowing the syntax of the language helps in debugging and enhances code readability, which is vital when collaborating on projects or contributing to open-source gems.
Basic Syntax and Structure
Ruby's syntax is often lauded for its elegance and simplicity. For instance, you can define a variable with just a few characters:
This straightforward syntax encourages developers to focus more on logic than on intricate code structures. Also, Ruby omits many mandatory syntactical elements seen in other languages:
- Curly braces around blocks
- Semicolons to end statements (unless on the same line)
- Type declaration
This makes Ruby a friendlier choice for beginners, allowing them to dive right into programming without being bogged down by excessive syntax rules. However, being aware of the conventions, such as using indentation for readability, is essential. It is often said in programming, "Readability counts." Ruby adheres to this philosophy, allowing developers to express their thoughts clearly through code.
Data Types and Variables
In any programming language, understanding data types is critical, and Ruby is no exception. Ruby is dynamically typed, which means you don't have to specify the type of variable when you create one. Here’s a rundown of common data types in Ruby:
- String: Text enclosed in quotes, such as "Hello, World!"
- Integer: Whole numbers, like 42.
- Float: Decimal numbers, such as 3.14.
- Array: A collection of objects, such as .
The ability to change a variable's type dynamically can be advantageous, but it can also lead to confusion if one isn't careful. For example, a variable that starts as an integer can later be reassigned to a string. To avoid any potential pitfalls, it’s suggested to have a firm understanding of these types in different contexts.
Control Flow Statements
Control flow statements enable programmers to dictate the order of operations in their programs. In Ruby, you'll commonly use statements like , , , , and . Here’s an example of an statement:
This checks if a person's age qualifies them as an adult. The use of control flow statements is crucial for creating logical pathways in your code, ultimately allowing it to respond to different conditions dynamically.
In addition, Ruby's expressive syntax can make these statements quite readable, which is often why developers find it a preferred choice for rapid application development, especially in environments that demand agility.
"Brevity is the soul of wit" – This saying extends well into the realm of coding; concise and expressive control flow statements make your Ruby code not just functional but also aesthetically pleasing.
Object-Oriented Programming in Ruby
Object-oriented programming, or OOP, is a paradigm that revolutionized software development, and Ruby is an exemplary language that embodies its principles. In this section, we will dive into the essentials of OOP in Ruby, looking at how it enhances code organization, improves reusability, and fosters a more intuitive way of structuring programs. The core of OOP can be broken down into fundamental concepts such as classes, objects, inheritance, polymorphism, modules, and mixins. Understanding these components not only boosts your proficiency in Ruby, but also nurtures good coding habits that are applicable across various programming languages.
Classes and Objects
At the heart of Ruby's OOP capabilities lie classes and objects. A class can be thought of as a blueprint, defining the structure of an object. For instance, consider a simple class, . This class might incorporate attributes like brand, color, and speed, along with methods such as and . By creating instances of the class, known as objects, we can represent individual cars with specific traits.
Here's an example of a class definition:
Creating objects becomes a cinch with this model:
Key Takeaway: Understanding how to create and utilize classes and objects is essential for effective Ruby programming, providing clarity in your code as you transition from simpler scripts to more complex systems.
Inheritance and Polymorphism
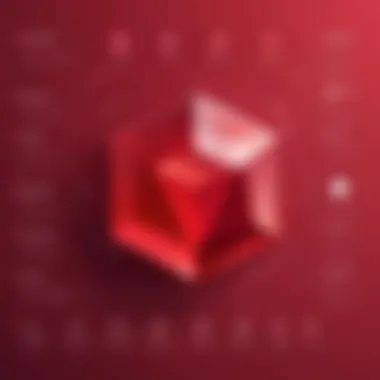
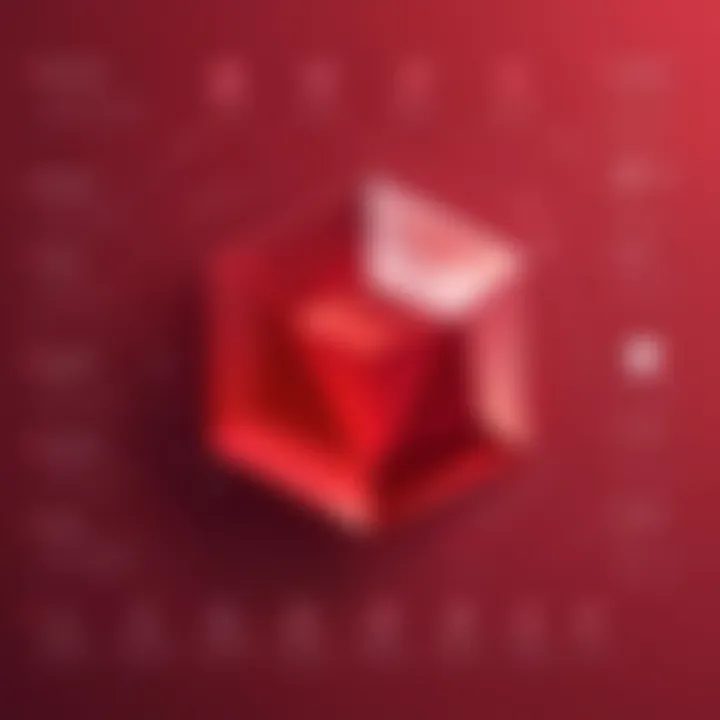
Inheritance allows new classes to inherit the characteristics and behaviors of existing classes. This not only aids in code reusability but also helps maintain consistency. For example, if we have a class with basic properties common to all vehicles, we can create a class that inherits from it. This means all the properties and methods of are now accessible in , streamlining the development process.
Polymorphism, on the other hand, refers to the ability for different classes to be treated as instances of the same class through a common interface. This is especially useful when a method can operate on different types of data. In Ruby, polymorphism is often achieved through method overriding. For instance:
Now, despite and being different classes, calling will yield behavior specific to the class instance while still being invoked in the same way.
"Good design is about making things understandable, and for complex systems, polymorphism plays a trick that designing complex features can be simpler."
Modules and Mixins
Modules in Ruby serve as a mechanism to group related methods, which can then be included in classes as mixins. This is particularly effective for code organization, and it encourages reusability without resorting to inheritance. For instance, you could have a module containing methods like and . Any class representing a vehicle can include this module to gain those methods without being tied to a specific hierarchy. This versatility makes Ruby particularly powerful.
Utilizing modules fosters cleaner code and can effectively reduce duplication across your program.
In summary, mastering object-oriented programming in Ruby is a crucial step for aspiring developers. By grasping concepts such as classes, inheritance, polymorphism, modules, and mixins, you can write sophisticated, maintainable, and reusable code. With these tools in your arsenal, you'll be well-equipped to tackle a wide range of programming challenges.
Advanced Ruby Concepts
Understanding advanced Ruby concepts is essential for any developer looking to unlock the full potential of this programming language. These ideas aren't just buzzwords; they form the backbone of flexibility and efficiency in Ruby. Concepts like metaprogramming allow for dynamic method definitions, while duck typing and dynamic typing contribute to the expressiveness of the language. Gaining proficiency in these areas helps you write cleaner, more maintainable code and facilitates rapid development. Whether you’re digging into complex libraries or building scalable applications, grasping advanced Ruby ideas will give you an edge in the programming world.
Metaprogramming
Metaprogramming in Ruby can seem like magic to newcomers, but it’s fundamentally a powerful aspect of the language. It allows you to write code that modifies other code at runtime, making your applications more dynamic. For instance, developers can create methods and classes on the fly, which can significantly reduce the amount of boilerplate code required.
Here is a simple example of metaprogramming in Ruby:
In this snippet, we're defining a method on the fly using . That’s neat, right? But while metaprogramming provides flexibility, it can also introduce complexity. Therefore, it's crucial to use it judiciously. Overdoing it can lead to code that is difficult to read and maintain, ultimately defeating its purpose.
Duck Typing and Dynamic Typing
One of Ruby's hallmark features is duck typing. This means that an object's suitability is determined by the presence of specific methods and properties rather than its class. The famous saying goes, "If it looks like a duck and quacks like a duck, it probably is a duck." In programming terms, if an object responds to the methods you call, it’s good to go.
Dynamic typing complements this flexibility, allowing variable types to change at runtime. For example:
This duality permits rapid prototyping and efficient coding practices. However, it can also lead to errors that are only caught during execution, making unit tests critical for larger projects.
Lambda and Proc
Lastly, understanding lambdas and Procs is vital for making your Ruby code cleaner and more efficient. Both are types of closures in Ruby, but they have some key differences. Lambdas check the number of arguments you pass, while Procs do not. Here’s a brief touch on how they work:
Lambda example:
Proc example:
In summary, mastering these advanced Ruby concepts not only enhances your programming toolbox but also prepares you to tackle complex software challenges with confidence. The benefits they bring to the table are well worth the effort to understand and apply.
Ruby Libraries and Frameworks
When diving into the world of Ruby programming, understanding the landscape of libraries and frameworks is pivotal. Libraries and frameworks serve as foundational tools that allow developers to harness the power of Ruby without starting from scratch. They provide pre-built functionalities and best practices which can significantly speed up the development process while maintaining code quality.
Ruby’s rich ecosystem of libraries and frameworks enables seamless development for various applications, ranging from web applications to data analysis and automation tasks. Operational efficiency and recreation of existing solutions are just a couple of benefits that can be highlighted. This section shines a light on three essential aspects: Ruby on Rails, popular gems, and testing frameworks like RSpec and Minitest.
Prolusion to Ruby on Rails
Ruby on Rails, often simply called Rails, is one of the most influential frameworks powered by Ruby. Launched in the early 2000s, it stood out for adhering to convention over configuration principles, which significantly reduces the time developers spend configuring their applications. This framework adopts the Model-View-Controller (MVC) architecture, which not only promotes clean code practices but also allows for separation of concerns in web applications.
To illustrate its effectiveness, consider a startup aiming to launch a Minimum Viable Product (MVP). With Ruby on Rails, they can develop a fully functioning web application in remarkably short timeframes. Many major companies, including Twitter and GitHub, owe part of their development success to this framework. A key advantage lies in the vast library of gems specifically designed for Rails, facilitating features such as authentication, authorization, or even complex billing processes with just a few lines of code.
Popular Gems and Their Uses
Gems play an integral role in Ruby's success, acting like plugins that extend the functionality of Ruby applications. Here are some gems that stand out due to their usefulness:
- Devise: A flexible authentication solution. This gem makes it incredibly easy to manage user registrations and sessions.
- Pundit: Used for authorizing user permissions. It provides an easy-to-use API for defining roles and policies in applications.
- Sidekiq: A background processing library, which is crucial for handling long-running tasks without freezing the web server.
Incorporating these gems can simplify development efforts while boosting the application's performance. Furthermore, the Ruby community constantly expands this ecosystem, ensuring developers access to modern tools and capabilities.
Testing Frameworks: RSpec and Minitest
As any seasoned developer can attest, testing is an essential part of the software development lifecycle. Ruby offers outstanding frameworks for testing that cater to different needs and preferences.
RSpec: This behavior-driven development (BDD) framework provides a readable language for writing tests. Developers often appreciate its focus on specification, allowing them to define the expected behavior of applications clearly. RSpec promotes a vibrant ecosystem of matchers and mocks that makes writing comprehensive tests intuitive.
Minitest: On the other hand, Minitest is often praised for its simplicity and speed. It incorporates the basic functionalities of a test suite but requires less overhead. Many Rubyists choose Minitest for its straightforward approach, making it easier to get up and running, particularly in smaller applications.
Both RSpec and Minitest allow developers to write clean, maintainable tests, thus ensuring their Ruby applications operate as intended.
Best Practices in Ruby Programming
In the realm of programming, best practices serve as the backbone of readability, maintainability, and efficiency. In the context of Ruby programming, adhering to these high standards not only helps individual coders write clearer code but also fosters team collaboration and reduces the likelihood of errors. As software systems become more complex, the importance of having established best practices cannot be overstated. They streamline workflows and ensure that all developers, regardless of experience, are on the same page. This section focuses on vital elements like code readability, debugging techniques, and version control, crucial for anyone seeking mastery in Ruby or any programming language.
Code Readability and Style Guides
Readability is king in programming. If the main aim is to write code that others can understand, then investing time in it pays dividends. A well-structured piece of code allows both current and future developers to quickly comprehend its functionality without getting bogged down.
One of the most effective ways to achieve this is through a style guide. Ruby has a community-driven style guide that can be found in GitHub. Here are a few key points to consider:
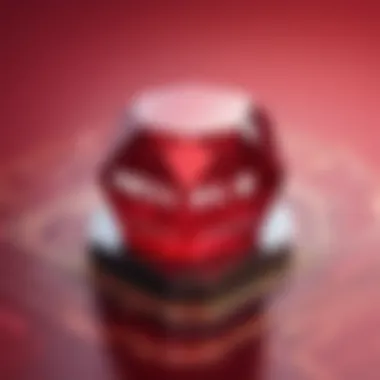
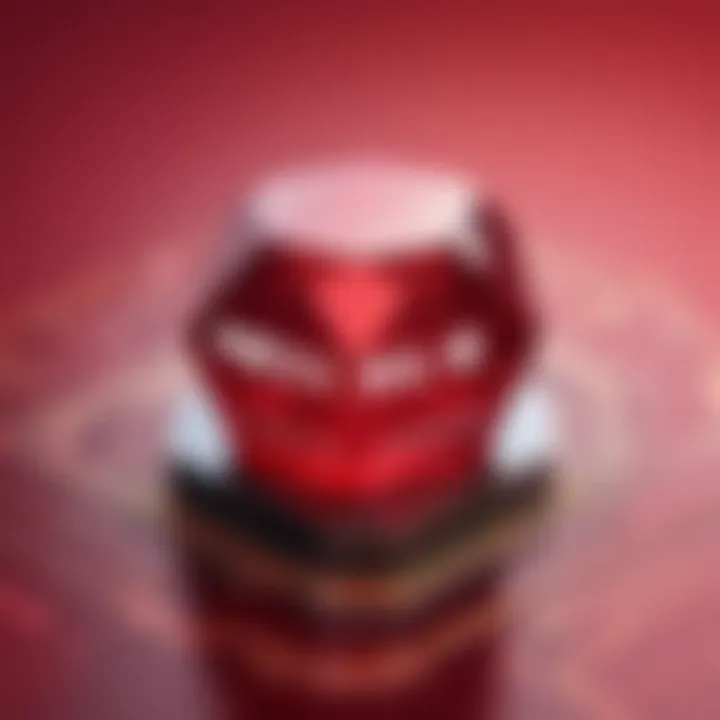
- Consistent Indentation: Use two spaces for indentation. This keeps the visual structure clean and manageable.
- Naming Conventions: Variable and method names should be descriptive. Adopting snake_case for variables and methods—and CamelCase for classes—ensures that their purpose is clear at a glance.
- Comment Wisely: Avoid comments that are obvious. Instead, focus on providing context and explanations for complex logic.
By adhering to these conventions, you significantly reduce cognitive load for others reading your code. It's a step toward more collaborative programming, where everyone contributes efficiently.
Debugging Techniques
Debugging is an inevitable part of the programming process. It’s where code can go from functional to fabulous or from frustrating to functional. Ruby offers several built-in tools and methodology to ease the debugging experience.
Firstly, Ruby programmers often find invaluable. This interactive shell allows developers to pause execution and inspect objects, which can be a lifesaver when trying to understand what’s gone wrong. Here’s how to install and use it:
Subsequently, inserting into your code lets you interact directly at that point in execution. You can check variable states and control flow without disrupting the overall program structure.
Also, log files can be incredibly useful. Rather than relying solely on print statements, consider utilizing the class that Ruby offers. It helps maintain a record of what’s happening in your app in a clean and orderly fashion.
Version Control with Git
Version control is the backbone of modern software development. With Git, developers can track changes, revert to previous states, and collaborate without stepping on each other’s toes. In Ruby programming, leveraging Git efficiently can mean the difference between a chaotic workflow and a seamless collaboration.
Here are a few best practices to consider:
- Frequent Commits: Committing changes often keeps your project history clean and meaningful. It’s easier to track specific changes, and troubleshooting becomes less daunting.
- Descriptive Commit Messages: Each commit should tell a story. Use clear language to describe what changes were made and why, enabling others to grasp the evolution of the project.
- Use Branches: Branching keeps your main project tree clean. Develop new features or fixes in isolated branches and merge them only when they are polished.
By following these practices with Git, you ensure that your Ruby projects remain organized and accessible, allowing for a smoother development process overall.
✅ In summary, incorporating best practices into your Ruby programming can lead to long-term success in software development. Not only do they enhance the quality of your code, but they also facilitate a collaborative environment, creating a solid foundation for teamwork.
Real-World Applications of Ruby
Ruby has carved out a significant niche in the tooling of modern software development. Its applications stretch across various domains, most notably in web development, data analysis, and automation. Understanding these diverse uses is paramount to appreciating the versatility and power of Ruby as a language. These real-world applications do not just showcase how Ruby can be utilized, they also reveal its inherent strengths and weaknesses, making it essential for every budding programmer to comprehend them.
Web Development
When it comes to web development, Ruby is often synonymous with Ruby on Rails, a powerful web application framework that has transformed how developers build websites. Rails promotes convention over configuration and allows for rapid application development, making it attractive for startups and larger enterprises alike.
Using Ruby on Rails, developers can create complex sites in a fraction of the time it would take with other frameworks. For instance, consider a startup aiming to launch an MVP (Minimum Viable Product). With Rails, the team could leverage the framework’s built-in features like scaffolding, which automatically generates the necessary code for various applications, thus speeding up the project.
More than just speed, Rails allows developers to maintain a clean and organized codebase, making it easy to modify and expand upon the application as it grows. This scalability is crucial as the needs of a business evolve. Additionally, the extensive community support and countless gems (one of Ruby's standout features) available make problem-solving more streamlined. However, it's worth noting that while Rails provides numerous advantages, it may not be the best fit for applications requiring highly customized solutions or performance intensive tasks.
Data Analysis Applications
Ruby’s appeal in data analysis is not as widespread as languages like Python, but it certainly has its place. Libraries such as Daru and Statsample allow for effective manipulation and statistical analysis of data.
For example, a data scientist might use Daru to handle large datasets, perform aggregations, or plot data visualizations. The syntax remains relatively straightforward, enabling users to implement complex data manipulations with less cognitive load. This simplicity can streamline the process for professionals coming from non-technical backgrounds or for those who might be new to programming.
Moreover, as organizations continue to depend on data-driven decision-making, having Ruby as a tool in one’s arsenal can yield significant advantages. Not only does it allow for robust analysis, but it also integrates seamlessly with web applications, enabling real-time data interactions. Nevertheless, it is essential to understand its limitations in the statistical realm compared to more specialized environments.
Automation Scripts
Another area where Ruby shines is automation. The language's readable syntax makes it an excellent candidate for writing scripts that handle routine tasks, such as scraping websites or managing files. For instance, a developer might create a simple Ruby script to automate the extraction of job listings from various sites, compile them into a single document, and email the result to interested parties. This could save hours of manual work each week.
Automation with Ruby can lead to increased productivity, allowing programmers and businesses to focus on more critical tasks rather than repetitive ones. Scripts written in Ruby can easily be executed on a variety of systems without heavy degradation in performance, all thanks to Ruby’s cross-platform nature. However, like with other aspects, it's vital to evaluate whether Ruby is the most efficient choice for the specific automation task at hand.
"In the realms of web development, data analysis, and automation, Ruby has proven to be an invaluable asset for professionals looking to enhance their work processes and output."
Overall, the real-world applications of Ruby are as diverse as they are impactful. From facilitating rapid web development to enhancing data analysis and streamlining automation, Ruby continues to hold relevance in a landscape filled with competing technologies. The choice to integrate Ruby into a project ultimately hinges on specific project requirements, available resources, and the goals of the development team.
Further Learning Resources
Learning Ruby, or any programming language for that matter, is an ongoing journey that extends far beyond the initial grasp of basic concepts. Further learning resources play a crucial role in deepening one’s understanding, honing skills, and staying updated with industry trends. This section of the article emphasizes the significance of utilizing various resources—be it books, online courses, or community forums—each offering unique strengths to programmers at any stage of their learning curve.
Books and eBooks
Books and eBooks stand as timeless tools for education. They provide structured insight into Ruby programming, often penned by experts who encapsulate years of experience and knowledge. A few highly recommended titles include:
- "Programming Ruby: The Pragmatic Programmers' Guide" – This classic offers a thorough overview, making it a must-have for beginners and seasoned programmers alike.
- "The Well-Grounded Rubyist" – Aimed at those who already know some basics, this book dives deeper into the intricacies of Ruby. Read it to deepen your understanding of object-oriented principles and Ruby-specific idioms.
- "Eloquent Ruby" – This book focuses on writing idiomatic Ruby and features essential best practices that are vital in the development of functional applications.
Ebooks offer the flexibility of reading on various devices, making them accessible at any time. They are easily searchable and can be updated swiftly to reflect the latest Ruby developments, keeping your knowledge fresh.
Online Courses and Tutorials
With the digital age flourishing, myriad online platforms offer courses tailored for Ruby learners. Websites like Codecademy, Udemy, and Coursera host a spectrum of courses, from beginner to advanced levels. Online courses provide a structured learning environment, often featuring video lectures and downloadable resources. Here’s what to consider when exploring these options:
- Hands-On Practice: Many platforms encourage coding exercises and real-world projects to reinforce learning.
- Community Interaction: Some courses offer forums where you can discuss problems and projects with peers, lending to a richer learning experience.
- Instructor Support: Having access to an expert can clarify doubts and enrich your understanding.
For instance, the Ruby on Rails Specialization course on Coursera walks you through building a robust web application, blending theoretical knowledge with practical application.
Community and Forums
No one learns in isolation. Engaging with others can often clarify doubts that solo learning can't resolve. Community forums such as Stack Overflow, Ruby Forum, and Reddit’s r/ruby subreddit serve as platforms for learners and experienced developers to exchange knowledge and troubleshoot coding issues. Participating in these communities can provide:
- Real-Life Problem Solving: You may find others have faced similar challenges and their solutions can guide you.
- Networking Opportunities: Interaction with fellow students and software developers can lead to potential collaborations or job opportunities.
- Updates and Innovations: Discover new libraries, tools, or trends in the Ruby programming world through other members' experiences.
It’s essential to contribute back. Answering questions can reinforce your understanding and establish you as a knowledgeable member of the community.
The End
In this article, we have navigated through the intricacies of Ruby programming, exploring not just its syntactical elegance but also the vast ecosystem it supports. The conclusion serves as a vital wrapping up of all the threads we've woven throughout our discussions. It’s the moment to reflect on how Ruby stands at the intersection of simplicity and power, making it a top choice for developers across various fields.
Recap of Key Takeaways
When pondering over the key takeaways from this guide, a few salient points emerge:
- Ruby's Readability: Its clean syntax promotes ease of understanding, making it an inviting language for newcomers.
- Object-Oriented Approach: The object-oriented paradigm allows for modular code, enhancing maintainability.
- Robust Libraries and Frameworks: The presence of frameworks like Ruby on Rails encourages rapid application development by providing ready-to-use toolsets.
- Community Support: The vibrant Ruby community offers a wealth of resources, from forums to conferences, fostering continuous learning.
- Metaprogramming: This advanced feature of Ruby allows developers to write code that writes code, showcasing Ruby's flexibility and depth.
Through these elements, one can appreciate the unique position that Ruby occupies in the programming landscape. It's not just about writing code; it's about writing code that is elegant, maintainable, and powerful enough to handle complex real-world applications.
Future of Ruby Programming
Looking ahead, the future of Ruby programming seems promising yet challenges persist. With the rise of languages like JavaScript, which dominate the web development scene, Ruby needs to maintain its relevance by adapting to new trends and technologies.
- Integration with New Technologies: Ruby has begun to embrace emerging technologies like microservices and serverless architectures. Adapting to these practices is crucial to stay relevant.
- Performance Improvements: There’s a noticeable demand for better performance metrics; hence, upcoming versions are focused on speed enhancements without losing Ruby's flair.
- Community Innovation: The community's drive for creativity enables Ruby to evolve. From gem development to contributions in core libraries, the ecosystem remains vibrant.
Overall, while Ruby faces competition, its core attributes of simplicity and power continue to appeal to developers. The language’s adaptability and the community's ongoing efforts will be vital in ensuring its longevity in the programming world.
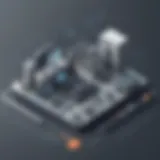
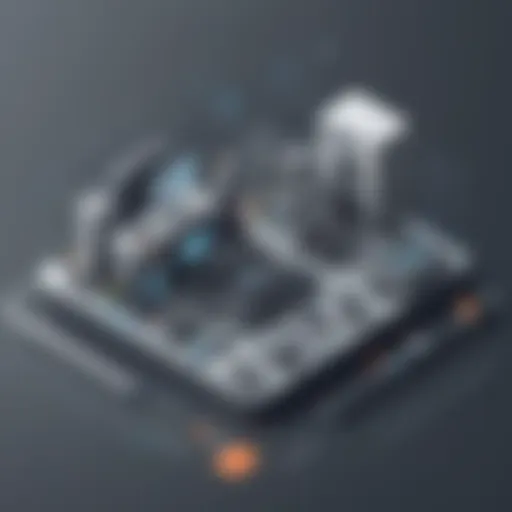