Mastering Python Classes: A Complete Guide
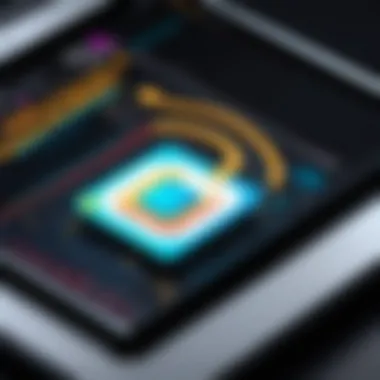
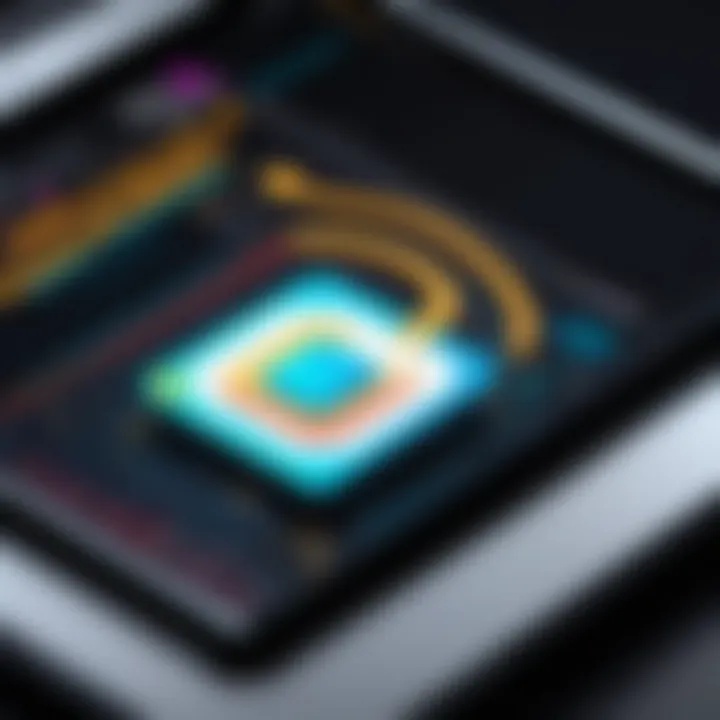
Overview of Topic
In recent years, the importance of understanding object-oriented programming (OOP) has surged, especially for programming languages like Python. Classes sit at the heart of this paradigm, behaving almost like blueprints for building objects. Recognizing how to effectively utilize classes is crucial for any budding developer or seasoned IT professional aiming to enhance their coding proficiency.
Prelims to the Main Concept Covered
Classes provide a way to encapsulate data and behaviors associated with that data. Imagine having a template for creating a car, where features like color and model can be defined, but specifics like those features can vary for each instance of a car created. That right there is the power of classes.
Scope and Significance in the Tech Industry
As modern software development increasingly embraces OOP principles, classes become indispensable. Think of them as the cornerstones of well-structured code. Whether working on small applications or large-scale systems, mastering classes invariably leads to better maintainable and more scalable projects.
Brief History and Evolution
Python emerged in the late 20th century, and its simplicity and readability have made it one of the top choices among developers. The introduction of classes in Python marked a significant step in aligning it with other object-oriented languages like Java and C++. Today, Python's class system continues to evolve, with enhancements that cater to various programming needs.
Fundamentals Explained
Core Principles and Theories Related to the Topic
Understanding classes requires grappling with several key concepts within OOP: encapsulation, inheritance, and polymorphism. Encapsulation refers to bundling data and methods together, protecting them from unauthorized access. Inheritance allows subclasses to inherit attributes and methods from parent classes, promoting code reuse. Polymorphism enables methods to behave differently based on the object calling them.
Key Terminology and Definitions
- Class: A blueprint for creating objects.
- Object: An instance of a class.
- Attribute: A variable that belongs to a class.
- Method: A function defined within a class.
Basic Concepts and Foundational Knowledge
The foundation of working with classes involves knowing how to define them and how to create instances. A class definition generally includes attributes and methods, while instances represent specific realizations of that class with their own unique states.
Practical Applications and Examples
Real-World Case Studies and Applications
Consider a scenario where a weather application displays various data, such as temperature and humidity. Each location can be represented as an instance of a Weather class, capturing both common and unique attributes. Utilizing classes makes it far easier to manage a significant amount of data without getting lost.
Demonstrations and Hands-On Projects
Let's look at a simple demonstration of creating a class in Python:
In this code snippet, we defined a Dog class with attributes and , along with a method . This provides a clear instance of how classes can be structured.
Advanced Topics and Latest Trends
Cutting-Edge Developments in the Field
As the programming landscape evolves, advanced features such as decorators, metaclasses, and context managers have emerged, pushing class functionality to new heights. These tools not only streamline processes but also enhance the readability of code.
Advanced Techniques and Methodologies
Some advanced techniques include multiple inheritance and class composition. These invite a higher level of flexibility in tailoring the behavior of objects. Combined with design patterns such as Singleton or Factory, they can significantly elevate your coding craft.
Future Prospects and Upcoming Trends
With the rise of machine learning frameworks like TensorFlow and PyTorch, the significance of classes in encapsulating complex algorithms and training routines is greater than ever. As technology progresses, the role of classes in these domains is likely to expand.
Tips and Resources for Further Learning
Recommended Books, Courses, and Online Resources
To solidify understanding of classes and OOP in Python, consider diving into:
- "Python Crash Course" by Eric Matthes
- Online platforms like Coursera or edX offering Python courses
- The official Python documentation for deeper insights (https://www.python.org/doc/)
Tools and Software for Practical Usage
Using integrated development environments (IDEs) such as PyCharm or Jupyter Notebook can greatly enhance your coding experience. These tools offer significant features for testing and debugging, making the learning process smoother.
Understanding Object-Oriented Programming
Object-Oriented Programming (OOP) serves as a cornerstone for modern programming languages, including Python. By leveraging the principles of OOP, developers can create code that is not only reusable but also easier to maintain. It allows for more complex systems to be understood through simpler, relatable structures called classes and objects. This reflective mode of thinking fosters a more natural organization of code, which mirrors how we perceive and interact with the real world.
Why should a programmer care about OOP? For starters, it divides the programming life into digestible pieces, reducing the cognitive load. Furthermore, OOP promotes encapsulation, abstraction, inheritance, and polymorphism, making it easier for developers to manage large codebases. A solid grasp of these principles can dramatically improve the efficiency and clarity of your coding practice.
- Encapsulation ensures that an object's state can only be changed by its methods, creating a protective barrier.
- Abstraction allows us to hide complex realities while exposing only the necessary parts.
- Inheritance permits the creation of new classes using existing ones, fostering the reuse of code.
- Polymorphism enables methods to do different things based on the object it is acting upon.
Through the lens of OOP, we can appreciate why knowledge of classes is fundamental in Python programming. It is more than just a tool; it is a paradigm that underpins the language's capabilities, influencing its design and utility in solving real-world problems.
The Principles of Object-Oriented Design
When diving into the principles of object-oriented design, a few key concepts must be understood. First off, letās talk about class design. A class is essentially a blueprint from which individual objects are created. Think of a class as a cookie cutter and the objects as cookies. Each cookie can be slightly differentānot every cookie must have the same toppings or shapeābut they all come from the same cutter.
Another essential principle is modularity, which allows us to break down a program into manageable parts. Each module performs a distinct function or set of functions, boosting the systemās flexibility. This leads right into another critical aspect: loose coupling. Loose coupling means that if one module has to change, it does not force changes in others, minimizing the risk of introducing bugs when updating your code.
A notable design principle is DRYāDonāt Repeat Yourself. Repetition often leads to maintenance nightmares. Writing code in classes promotes reusable code, helping developers stick to this principle. By following these guidelines, programmers can cultivate code that flows logically, is easy to read, and is less prone to errors.
Why Classes are Essential in Python
Many may wonder why classes are heralded as essential in Python. The answer is straightforward: classes provide a framework for organizing code in a way that mirrors real-world behavior. Imagine describing a car. Instead of going into technical details about the vehicle, we can say it has wheels, a steering wheel, and an engine, amongst other characteristics. By using classes, programmers can define their objects in a similar high-level manner, ushering in clarity.
Classes also empower developers to create data structures that encapsulate behavior alongside data. This means not only do we define what an object looks like (its data) but also how it behaves (its methods). For example, when creating a class, you can embed both (data) and (method) within the class structure. Attach behaviors directly to the corresponding data.
In the growing landscape of programming where Python is increasingly popular, understanding classes also opens the door to advanced topics like inheritance and polymorphism. These concepts allow developers to enhance their codeās functionality without needing to start from scratch.
In summary, classes bring a structured way of solving problems, which makes them indispensable for anyone venturing into the realm of Python programming.
Defining a Class in Python
Defining a class in Python holds an essential place in the realm of object-oriented programming. Classes serve as blueprints for creating objects, encapsulating properties and methods that act on those properties. Understanding how to define a class is crucial for anyone looking to delve deeper into Python programming. Whether you're building a simple program or architecting complex systems, mastering class definitions enhances code organization and readability.
A class groups related data and functionality, which makes it easier to manage code and enables code reusability. You might wonder, why exactly is this structure important? The importance is two-fold. First, it simplifies problem-solving by breaking down a larger task into manageable pieces. Second, it fosters a cleaner codebase that can be easily navigated and understood by others or by yourself when revisiting it later. To put it simply, classes are where the magic happens.
The Class Syntax
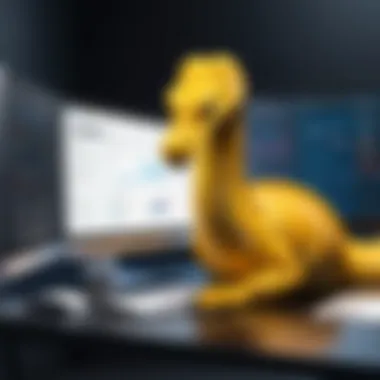
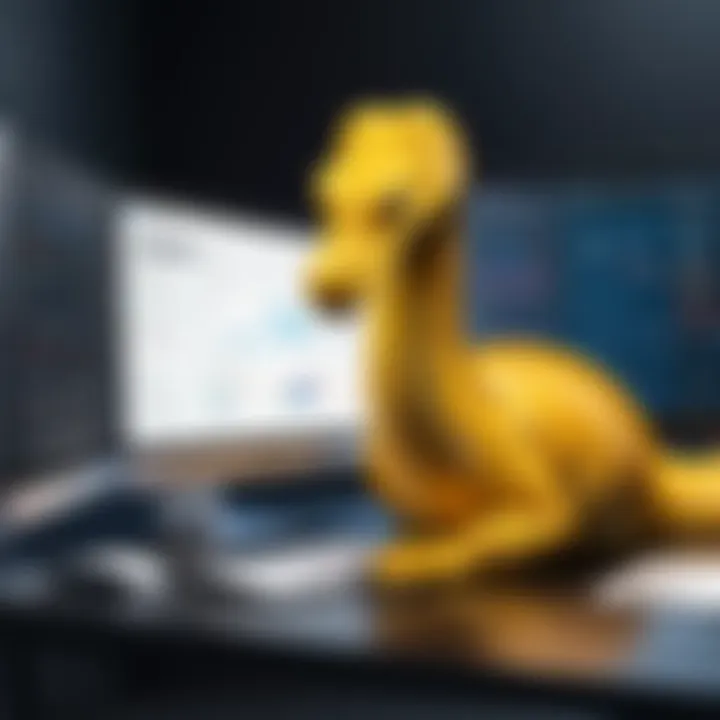
When it comes to defining a class, the syntax is quite straightforward yet powerful. Hereās a skeleton of the class definition in Python:
In this snippet, is the name of your class. The method is a special method that initializes the attributes of the class. The use of refers to the instance of the class itself, which allows you to access variables and methods within the class.
Every class begins with the keyword, followed by the name of the class, conforming to the convention of using CamelCase for readability. The attributes set inside the method are instance variables, tailored to each object created from the class. This sets the stage for making your class more functional and accessible.
Creating the First Class
Now, letās roll up our sleeves and create our first class in Python. Suppose weāre developing a program that manages a library system. You could start by defining a class named that holds all the relevant information about each book:
In this example, we have a class with three attributes: , , and . The method gives us a convenient way to retrieve information about the book.
To create an instance of this class, you simply do:
Here, is an instance of , and when you call the method, it returns a formatted string with the book's details.
Class Attributes and Instance Variables
In the realm of Python programming, understanding the fabric of class attributes and instance variables is crucial. These components form the backbone of how data is stored and managed within classes. Knowing the ins and outs of these characteristics can greatly enhance your programming efficiency and design practices. The separation of class attributes and instance variables provides a better structure for how information is accessed and modified, allowing developers to write clearer and more maintainable code.
Understanding Attributes
Attributes in Python represent the data associated with a class or an instance of a class. Think of them as qualities or properties that define the characteristics of an object. When you create a class, you usually define attributes to hold data pertinent to that class. These attributes can be saved at either the class level or the instance level.
When attributes are defined at the class level, they are shared across all instances of that class. This means that if one instance changes the value of a class attribute, all instances see this change. Conversely, instance variables are specific to an individual object instance and can hold unique data. This distinction allows for both shared and unique properties, making it easier to structure your code according to different use cases.
For example, consider a class named . You might define a class attribute like , which would be common to all cars:
Here, is a class attribute, whereas is an instance variable. Each car object can have a different , while all share the same number of .
Distinction Between Class and Instance Variables
Understanding the differences between class and instance variables is vital to mastering object-oriented programming in Python. Let's break it down further:
- Class Variables (Class Attributes): These are attributes declared within a class but outside of any instance methods. They belong to the class rather than to any specific object. As mentioned earlier, if one instance of the class modifies a class variable, the change is reflected across all instances. This can be ideal for attributes like , where you intend for all instances to share the same property.
- Instance Variables (Instance Attributes): These are attributes that are defined within the method and are prefixed with , referring to the specific instance of the class. Each instance can have different values for these variables, creating a unique state for each object. For example, each object can have a distinct value, operating independently of other instances.
When designing your classes, it's important to consider which variables should be class variables and which should be instance variables. Using class variables unnecessarily can lead to unexpected behavior and state sharing that may not be desirable in all scenarios. Conversely, if everything is only instance variables, you may miss out on efficient, shared properties that class variables offer.
Remember, the distinction between class and instance variables leads to a fundamental understanding of how data is encapsulated within your class structure. Accurately utilizing both attributes sets the stage for effective object-oriented design.
To summarize, the interplay between class attributes and instance variables develops a powerful dynamic within a class. Grasping this concept is a stepping stone towards advancing your skills in Python, allowing you to create classes that are both flexible and well-structured.
Methods in Classes
Methods are essentially the working hands of classes in Python; they bring the attributes to life by performing the necessary operations. They do this by defining specific functions that act upon the instance variables of the class, making them an essential element in any well-designed class. Through methods, you encapsulate behaviors that correspond to the data housed within your classes, allowing for a better-organized code structure while enhancing reusability.
Every time you want to manipulate or process the attributes of a class, itās the methods that come into play. They serve multiple purposes:
- Encapsulation of Behavior: Methods group functionalities related to instances of a class. This promotes cleaner coding by keeping related operations in one location.
- Reusability: Once defined, methods can be used across various instances, reducing redundancy.
- Ease of Maintenance: Changes to methods can be done in one place, and those changes propagate throughout your program wherever the method is utilized.
- Improving Readability: Well-named methods enhance the readability of your code, making it clearer what operations occur under the hood.
Understanding how to define and call methods is foundational to programming with classes. This section outlines the specifics of both.
Defining and Calling Methods
Defining a method in a Python class is uncomplicated. A method is just like a function but within the context of a class. Hereās a simple way to define a method in a class:
In this example, defines a method called . This method utilizes the instance variable to return a playful bark whenever itās called. The init method defines how to create a new instance of with a name. This captures the core idea: methods can operate on data held in the class, using to refer to instance variables.
To call a method, you create an instance of the class and then invoke the method using a dot notation:
Special Methods and dunder Methods
Special methods, often referred to as dunder methods (short for double underscore), are pre-defined methods that control the behavior of your classes. They allow your objects to integrate seamlessly with Python's built-in operations. For example, if you want your class to handle addition, you would define the method.
While you can create regular methods, dunder methods are special because they grant control over significant aspects of your objects. Here are some commonly used dunder methods:
- : Called when an instance is created.
- : Defines a human-readable string representation of the object.
- : Defines an official string representation of the object that can ideally be used to recreate the object.
- : Allows you to define behavior for the addition operator.
Hereās an example of a class utilizing some dunder methods:
The class has a constructor and two dunder methods that facilitate the string representation and addition of point objects. This means you can add two point instances directly, like so:
In summary, methods are not just tools to manage class behavior; they form the backbone of interaction when working with classes. By defining and calling methods appropriately, and understanding the role of special methods, you can harness the power that Python's object-oriented programming offers.
Inheritance in Python
Inheritance is one of the cornerstones of object-oriented programming and a critical concept in Python. It allows a class to inherit characteristics (attributes and methods) from another class. This not only promotes code reusability but also establishes a natural hierarchy between classes.
With inheritance, you can create a new class that expands on an existing one, saving time and effort. Imagine you have a class called , which includes basic attributes like or methods such as . Now, you want to create a class that should have the same traits as , but also add specific behaviors like . Through inheritance, you can create as a subclass of . This is not just beneficial for the present code but also makes future modifications and enhancements easier, providing a solid framework.
Concept of Inheritance
When we talk about the concept of inheritance, we're diving into the relationship between classes. In essence, an inheritance structure signifies that one class, referred to as a child or subclass, inherits from another class, known as a parent or superclass. This relationship allows the child class to utilize all properties and methods of the parent class without having to rewrite them.
For instance, if you define a base class called , it may contain attributes like and , and methods like and . When you create subclasses such as and , both of these classes automatically have access to those base methods and attributes. This structure encourages cleaner code and can significantly enhance maintainability.
Inheritance allows for code reusability, a principle every programmer must embrace.
Creating Subclasses
Creating a subclass in Python is quite straightforward. You simply specify the parent class when defining a new class. Here's a quick example:
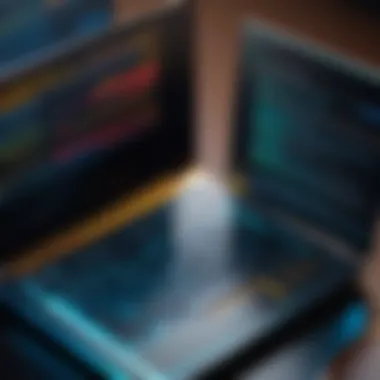
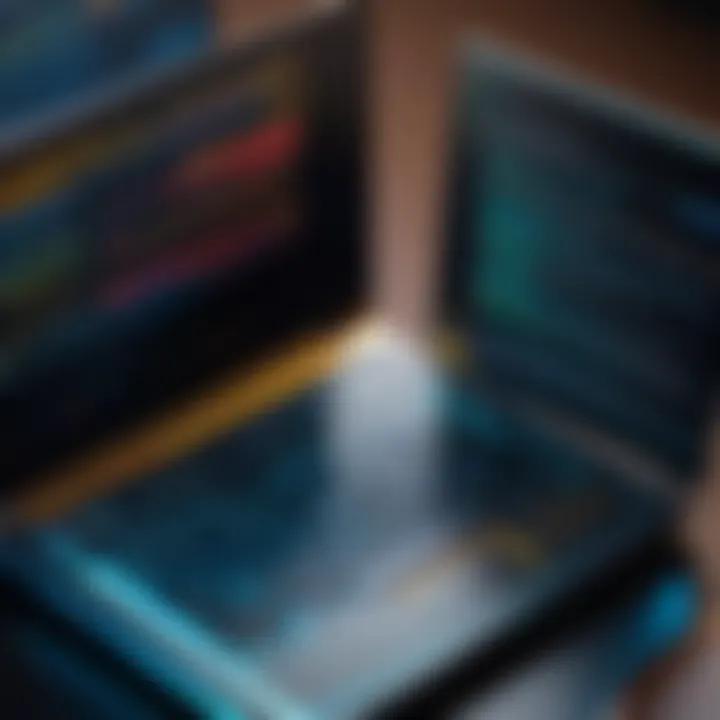
In this code snippet, extends . While inherits the method, it changes the behavior of the method to return "Woof!". This flexibility enables polymorphic behavior, where a subclass can redefine its parent class methods. You can think of subclasses as specialized versions of their parent classes, tailored for specific functionality while retaining the foundational features.
The Super() Function
The function plays a vital role in inheritance. It gives you a way to call methods from the parent class within the child class. This can be particularly useful for accessing the parent class's method to ensure that the parent properties are correctly initialized.
Here's how you would utilize in the example:
In this scenario, is also initializing its own attribute while ensuring the from the class is set correctly. This provides a powerful mechanism to build upon existing functionality while minimizing code duplication, leading to a more organized codebase.
Through proper use of inheritance and the function, developers can enhance the effectiveness of their class designs, making them both adaptable and less prone to errors.
Encapsulation in Classes
Encapsulation is one of the cornerstone principles of object-oriented programming, and it plays a crucial role in structuring your code effectively. Think of encapsulation as a protective layer around your dataālike a secure vault where sensitive information is stored away from prying eyes. It allows you to restrict access to certain components of a class, ensuring that the internal workings are hidden from the outside world. This hides complexity while allowing interaction through well-defined interfaces.
The benefits of encapsulation are manifold. Firstly, it promotes data integrity. By exposing only what is necessary, you safeguard your objects from being inadvertently altered by external factors, preventing chaos in your logic. Additionally, encapsulation enhances code maintainability. When you encapsulate data, making updates or modifications becomes less likely to cause unexpected issues elsewhere in your program.
Lastly, encapsulation simplifies debugging. When errors emerge, you can more easily zero in on specific areas of your code since thereās a clear boundary between what data is exposed and what isnāt. As programmers, we often find ourselves dealing with hundreds or thousands of lines of code; encapsulation provides a way to keep things tidy.
Defining Encapsulation
At its core, encapsulation is about bundling the data (attributes) and the methods (functions) that operate on that data into a single unit or class. Instead of allowing direct access to the particulars of data within a class, encapsulation promotes interaction through public methods known as getters and setters. This encapsulated design offers a controlled means for object interactions.
For instance, if you have a class that manages a bank account, you donāt want just anyone to modify the balance directly. Instead, youāll implement methods that ensure the balance can only be adjusted in a safe, validated manner. Hereās a simple illustration:
In this example, by using , you signal to other programmers that this attribute is intended for internal use. The and methods regulate how the balance can be altered, reinforcing the encapsulation concept.
Private and Protected Attributes
When discussing encapsulation, youāll often hear about private and protected attributes and their implications. In Python, thereās a certain flexibility in these classifications.
Protected attributes are intended to be accessible within the class and its subclasses. They are typically denoted by a single underscore prefix, as seen in the earlier example with . This indicates that the variable should be treated as a non-public part of the API, though it is not enforced strictly.
On the other hand, private attributes are signified with a double underscore prefix and are somewhat more restrictive in access. Python employs name mangling to discourage access from outside the class. Hereās a quick view of how this works:
Here, gives an alert to the developer that this variable is not meant to be accessed from outside the class. While technically still accessible through name mangling, this usage showcases a primary feature of encapsulation: controlling access to class data.
Remember: Encapsulation not only protects data but also clearly defines how data can be accessed and manipulated.
In summary, encapsulation in classes is more than just a programming concept; it's a design philosophy that promotes security, reusability, and maintainability. As we dive deeper into object-oriented programming in Python, understanding these fundamental concepts will empower you to craft more robust and cleaner code.
Polymorphism in Python
Polymorphism stands as a cornerstone in object-oriented programming (OOP), and this principle finds a significant embodiment in Python. Understanding how polymorphism works in Python not only clears the fog around its usage but also enhances the efficiency of code. When a language supports polymorphism, it cultivates flexibility; this becomes paramount as projects grow or requirements shift.
In the raw essence, polymorphism allows objects of different classes to be treated as objects of a common superclass. The real beauty of this lies in its power to implement shared methods for diverse class objects with no need to revise existing code.
Understanding Polymorphism
At its core, polymorphism can be divided into two main types: compile-time and runtime. Python primarily embraces runtime polymorphism, which happens through method overriding and interfaces.
Method Overriding
In a traditional sense, method overriding is where a derived class provides a specific implementation of a method that is already defined in its parent class. This is not merely a programming gimmick; it is the oil that keeps the engine of OOP running smoothly. When you have a parent class called and derive classes like and , you can override methods such as . Hereās an illustrative example:
Here, and define their versions of , showcasing how a call to this method will yield different responses depending on the object invoked.
This leads to a compelling takeaway: Polymorphism enables the use of a unified interface that can extend its functionality depending on the object type. In practical scenarios, this means when functions or methods receive arguments of different class types, they can still operate seamlessly without the need to worry about the underlying object details. This streamlining is what lends itself well to rapid development alongside easier maintainability.
Polymorphism with Methods
With polymorphism, the spotlight particularly shines on methods, specifically through how they adapt to different usages. When invoking a method, the Python interpreter determines which version of the method to execute at runtime based on the object's class, rather than the type referenced, also known as dynamic method resolution. This grants developers a robust toolkit for creating versatile and reusable code.
Consider creating a graphics application, where different shape classes (, , etc.) need to have a method called . The essence of polymorphism shines brightly here:
In this code, is a common method in each shape class, yet it behaves differently based on the type of shape. This allows the code to scale easily as new shapes can be added without changing the interfaces established in the previous implementations.
Culmination of Key Points
Polymorphism is not just a theoretical construct; it is an essential practical feature that enhances development efficiency. By employing method overriding, one can encapsulate behavior while maintaining the interchangeability of different objects. This flexibility encourages a programming approach that is both clean and scalable, making it a fundamental aspect of Python and its rich ecosystem of class-based programming. Thus, appreciating and mastering polymorphism is a key stride towards becoming a proficient Python programmer.
Best Practices in Class Design
Designing classes in Python demands a thoughtful approach. The essence of object-oriented programming lies in organizing code effectively to enhance clarity, reusability, and maintainability. Solid class design isnāt merely a nicety; itās a necessity. When practitioners adhere to best practices, they not only streamline their development process but also build systems that are easier to extend and modify in the future.
Principles of Solid Class Designs
When it comes to solid class designs, a few principles stand out, ensuring that your classes achieve their intended purpose without causing unnecessary complications. These principles can be summarized as follows:
- Single Responsibility Principle: Each class should have one responsibility or purpose. Having multiple unrelated functions in a single class invites confusion. This principle helps in maintaining a cleaner codebase where each class can be understood and tested independently.
- Open/Closed Principle: Classes should be open for extension but closed for modification. This means you should be able to add new functionality without altering existing code. This can often be achieved through inheritance or by using interfaces.
- Liskov Substitution Principle: This principle emphasizes that subclasses should be substitutable for their base classes without affecting the behavior of the program. It ensures that a derived class can stand in for its base class without breaking functionality.
- Interface Segregation Principle: Rather than one fat interface, many slender interfaces are preferred. Clients should not be forced to depend on interfaces they do not use. This keeps the design flexible and easier for changes.
- Dependency Inversion Principle: High-level modules should not depend on low-level modules. Both should depend on abstractions. This helps to reduce the coupling of code and enhances testability.
Utilizing these principles helps create clear and maintainable code. Think about them as a guiding lighthouse when designing your classes.
Avoiding Common Pitfalls
Even experienced developers can stumble into traps when designing classes. Here are some pitfalls to avoid:
- Overcomplicated Structures: While it's admirable to build sophisticated systems, avoid adding layers of complexity that are unnecessary. Aim for simplicity. When things start getting tangled, itās hard to tweak later on.
- Tightly Coupled Classes: Classes that depend heavily on each other become challenging to manage. Strive for loose coupling, allowing classes to interact but not rely heavily on each other. This might mean creating clear interfaces which each class can independently interact with.
- Ignoring Encapsulation: Make sure to keep attributes private or protected unless a good case can be made otherwise. Exposing class internals can inadvertently lead to unintended side effects.
- Neglecting Documentaiton: Failing to document your classes can leave others, or even you in the future, lost in the code. Comments and docstrings might feel like a chore, but they are invaluable for tracking down the intent behind design choices.
Keeping these principles in mind while also being wary of the common pitfalls can greatly enhance your class design, ensure sustainability, and provide direction for future development.
In summary, turning to best practices in class design enriches the robustness and adaptability of your code. Whether you are building a new library or improving an existing system, the structural integrity of your classes will play a pivotal role in the quality of your end product.
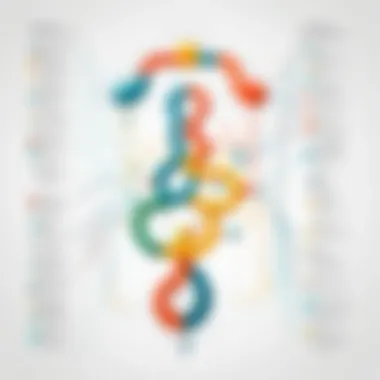
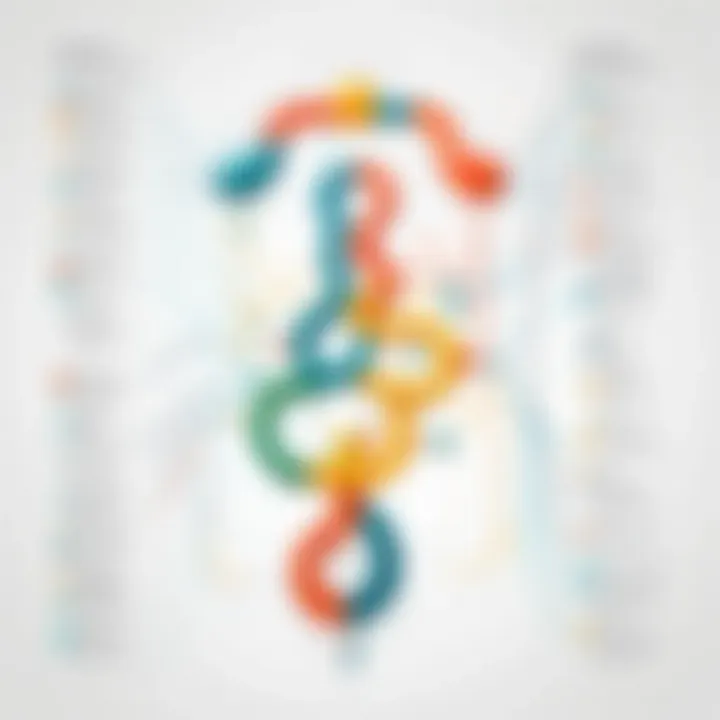
Advanced Class Features
In the realm of Python programming, Advanced Class Features serve as the cherry on top of the object-oriented design cake. These features empower developers to write code thatās not just functional, but also elegant and maintainable. They include aspects like class decorators and metaclasses, which introduce a layer of complexity that can lead to significant improvements in code organization and functionality. Understanding these advanced features can make one stand out in the developer crowd, showcasing a deeper grasp of Pythonās capabilities.
Class Decorators
Class decorators are a nifty way to modify or enhance class behavior without changing its actual code structure. Like a sprinter donning lightweight shoes before a race, decorators boost class performance or add features seamlessly.
To grasp their essence, consider this:
- Definition: A class decorator is simply a function that takes a class as an argument. The decorator function can return a modified version of that class or even a new class altogether.
- Use Cases: They can be remarkably useful for adding logging, enforcing access control, or even registering classes in frameworks. For example, one might need to automatically track the number of instances of a class being created or add additional methods dynamically.
Hereās a brief code snippet to illustrate:
In this example, wraps , adding a print statement every time an instance is created. The beauty here lies in the separation of concerns; the decorator handles additional behavior while can focus on its core logic without clutter.
Metaclasses Explained
Metaclasses are somewhat of the hidden gems in Pythonās toolkit. They define how classes behave, carving the path for their creation. Think of them as blueprints for classes, similar to how a class defines the structure for its objects.
In the simplest terms:
- Definition: A metaclass is essentially a class of a class. If classes create objects, metaclasses create classes. By default, the metaclass for all classes in Python is .
- Customization: Programmers can define their own metaclasses to modify class creation, injection of properties, or validation of certain attributes. Itās akin to having a custom factory that ensures every product meets specific criteria before hitting the shelves.
Suppose you want to enforce that all class attributes are lowercase:
Here, the class ensures that all attributes defined in are transformed to lowercase automatically. While it may raise some eyebrows at first, this method of enforcing rules can foster consistency across larger codebases where uniformity is crucial.
In summary, leveraging Advanced Class Features like class decorators and metaclasses opens doors to a more refined and powerful programming style in Python. These tools encourage a cleaner code structure, promote reuse, and can significantly elevate how developers interact with Python's object-oriented paradigm. Understanding and utilizing these features is not just an advantage; itās a hallmark of programming maturity.
Real-world Applications of Classes
Understanding real-world applications of classes in Python serves as a bridge between theory and practice, allowing programmers to grasp how these concepts manifest in everyday tasks. Classes bring order and structure to programming, making them an invaluable tool across various industries. Utilizing object-oriented programming provides several benefits:
- Modularity: Code structured with classes is easier to manage. Each class acts as a unit of logic, which can be developed, tested, and debugged independently.
- Reusability: Once a class is defined, it can be reused in different parts of an application or in different projects without modification.
- Encapsulation: Classes help in protecting data and methods that shouldn't be exposed to the rest of the code, ensuring that only the necessary aspects are visible.
- Scalability: As applications grow, classes make it simpler to scale features. New classes can easily be added to existing structures without disrupting the overall architecture.
Building a Simple Application
To appreciate the utility of classes, letās consider how to build a simple application. Imagine a contact management system. You can create a class called . This class will encapsulate all relevant details about a person, such as name, phone number, and email address. Hereās a glimpse of how it might look:
In the above example, the class serves as a blueprint for creating contact objects. You can easily instantiate multiple instances with various details, permitting straightforward add, edit, or delete functions. This simplicity captures a critical feature of class-based programming, where you can replicate functionality while maintaining organization and clarity in the codebase.
Besides this, the simple application highlights how you can manage complexity effectively. The class structure cuts down on redundancy and offers a clear pathway to extending functionalities, such as adding methods to search or filter contacts based on various criteria.
Use Cases in Web Development
Web development is a perfect example of real-world class implementations. Frameworks like Django and Flask leverage classes to define models, views, and even forms. These applications underscore their significance in web contexts, such as:
- Django Models: In Django, each database table translates to a class. Special attributes determine the fields necessary while methods on the class can represent actions, enabling a clean and coherent representation of your data.
- Flask Views: Flask makes use of class-based views to streamline routing and associated business logic. By utilizing the , you can define methods such as , , etc., within the same class, making code more organized.
An example of a class-based view in Flask could look like this:
A structured approach aids not just in reducing complexity but also fosters maintainability of the code.
Classes in web development establish a clear architecture, ensuring developers can work independently if the project expands. By working within the framework of classes, maintaining high code quality becomes more manageable, which is crucial in team environments.
Testing Classes in Python
When diving into the nitty-gritty of Python development, testing classes is a cornerstone that cannot be overlooked. Testing is vital not just for catching hidden bugs but also for ensuring that your code behaves as expected. Great testing practice fosters confidence in your code, making it easier to maintain and adapt as needs evolve. Also, carrying out tests brings to light areas where the design can be refined, ultimately enhancing the quality of the code. When you invest time in testing, you save yourself the headache of chasing down elusive bugs later down the road. Itās like putting on a seatbelt before the car rideābetter safe than sorry.
Unit Testing Basics
Unit testing boils down to verifying the smallest parts of your code in isolation. When we talk about units, we are usually referring to individual methods or functions within a class. The beauty of unit testing lies in its simplicityāby scrutinizing one small piece at a time, you can pinpoint issues without the noise of other code impacting your results.
For example, letās say you have a simple class that incorporates basic arithmetic operations. You could write unit tests to check that each calculation method returns the correct results for a range of input values. In Python, the built-in framework makes this process straightforward. Here's a basic outline to illustrate:
In this snippet, we establish a class and a corresponding test class. The module provides handy assertions like , allowing you to compare expected outcomes with actual results. Registering tests in this manner promotes a robust method of validating your classes' functionality.
Testing often helps uncover edge cases, which you might not have considered during development.
Testing with pytest
Pytest has gained notable popularity in the Python community, offering a refreshing take on testing. Its capabilities extend far beyond those of the traditional framework. One key aspect is its ability to handle multiple tests from a single function and its simple assertion methods, which often read relatively clean and intuitive.
To get started with pytest, first, youāll need to install it. If you havenāt yet:
Once installed, testing with pytest can be a welcome shift. Imagine you want to test the same class:
In this concise example, you can see how pytest eliminates the boilerplate code. Instead of defining a class for tests, we define a simple function and use straightforward assertions. Run the tests simply by calling from the command line, and it will automatically discover and execute all the tests in your directory. This feature eliminates the hassle of manual test registration.
Utilizing pytest could not be easier, as its rich features and plugins can help cater to the specific needs of more complex testing scenarios. Overall, testing in Python isn't just a good practice; it's a necessary part of effective development that every programmer should embrace.
Finale
As we reach the end of this exploration into Python classes, it's essential to grasp the significance of the insights shared throughout the article. In a world where software development is constantly evolving, having a solid understanding of classes is no longer a luxury; it's a necessity. Classes are the backbone of object-oriented programming, allowing developers to model real-world entities with precision and simplicity. The advantages of utilizing classes go beyond mere structure; they facilitate cleaner, more maintainable code that can grow with a project.
Recap of Key Takeaways
- Object-oriented programming principles lay the groundwork for creating reusable and efficient code.
- Classes allow for encapsulation, bundling data and behavior that represent real-world objects, making it easier to model complexity in applications.
- Inheritance simplifies code reuse and aids in extending functionalities without repeating code.
- Testing classes through frameworks such as ensures robustness and helps catch bugs early, preventing costly issues down the road.
- Keeping ahead of common pitfalls in class design contributes to a sound architecture and a more seamless coding experience.
"Good code is its own best documentation."
Future Directions in Class Usage
As software technology continues to advance, so does the way we approach class design and usage in programming languages like Python. Future directions might include:
- Increased Focus on Async Programming: Asynchronous programming will continue to shape how classes operate, especially with web applications becoming more responsive.
- Emphasis on Data Classes: With the introduction of data classes in Python 3.7, thereās a growing trend toward utilizing them for simpler, boilerplate-free coding, creating lighter structures to handle data.
- Integration with AI and Machine Learning: Classes are likely to be more deeply integrated into frameworks for AI and machine learning, helping to encapsulate various components of models effectively.
- Greater Use of Mixins and Composition: These design patterns allow for more flexible and reusable code, contrary to strict inheritance which can sometimes lead to complex hierarchies.
Ultimately, mastering the nuances of classes leads to better coding practices and prepares you to tackle the demands of modern programming challenges.