Integrating the YouTube API with JavaScript: A Guide
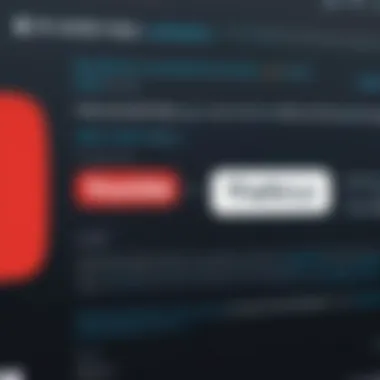
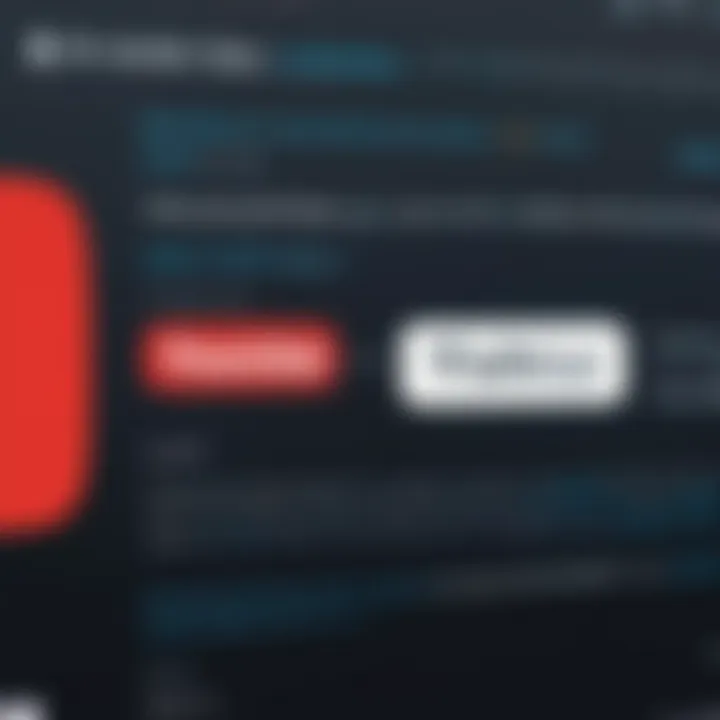
Overview of Topic
Preface to the Main Concept Covered
Integrating the YouTube API with JavaScript allows developers to access the vast repository of videos and data available on the YouTube platform. This integration enables enhanced functionalities and the ability to create unique web applications tailored to user needs. It provides developers the power to use real-time video data, building interfaces for seamless video playback, channel management, and more.
Scope and Significance in the Tech Industry
The significance of this integration is immense in today’s tech landscape. As video content continues to dominate online space, developers’ ability to interact with YouTube data is critical for businesses, marketers, and content creators. Integrating such APIs enhances user engagement, driving traffic, and improving user experience across various platforms. Knowledge of such integration is now vital for aspiring developers and seasoned professionals alike.
Brief History and Evolution
The YouTube API has evolved since its inception, reflecting the changing demands for online video content. Initially focused on simple video playback functions, the API now showcases extensive capabilities including advanced search functionalities, playlists management, and video statistics retrieval. Keeping up with these evolutions is key for developers to leverage ever-growing video standards and viewer preferences.
Fundamentals Explained
Core Principles and Theories Related to the Topic
A deep dive into API integration reveals core principles that dictate how data flows and functionalities are exposed. APIs serve as interfaces between different software components; in this case, JavaScript applications and YouTube. Understanding concepts such as RESTful services and JSON data formats will aid developers in making the most of this integration.
Key Terminology and Definitions
Certain terminology is integral to comprehending API integration:
- API Key: A code passed in the request header to identify and authenticate the user of the API.
- Endpoints: Specific URLs used to access various functionalities of the API.
- Rate Limits: Restrictions placed on the number of requests to the API within a specific time frame.
Basic Concepts and Foundational Knowledge
Familiarity with, at least, fundamental JavaScript and asynchronous programming concepts like Promises and calls is essential. These concepts enable seamless application behavior while interacting with YouTube’s considerable data resources. Understanding call patterns through methods aids in building responsive applications.
Practical Applications and Examples
Real-world Case Studies and Applications
Many organizations utilize YouTube API effectively. Educational platforms can leverage video content within their learning management systems by embedding tutorials. Businesses utilize promotional video content linked directly to their pages for enhancing product visibility. These integrations are common in web applications aiming for innovative user interaction methods.
Demonstrations and Hands-on Projects
Constructing a simple project using YouTube's API can be enlightening. For instance, developing a video search feature assists students or users in finding relevant content based on specified parameters.
Code Snippets and Implementation Guidelines
The code above portrays a basic implementation that retrieves videos related to programming tutorials based on a search query. By crafting functionalities around these queries, developers can enhance their projects significantly.
Advanced Topics and Latest Trends
Cutting-edge Developments in the Field
As technology progresses, the YouTube API continually adapts. It now includes machine learning integrations for better content suggestions and analytic tools to assess video performance, making it invaluable for brands hoping to optimize their approach.
Advanced Techniques and Methodologies
Advanced usage could involve creating applications that cluster data streams or utilize user feedback to refine video retrieval algorithms. Elevating engagement through adaptive user interfaces leveraging JavaScript-driven content is the future of these integrations. This is imperative for maintaining relevance in a fast-evolving tech space.
Future Prospects and Upcoming Trends
We can expect more sophisticated access protocols and better analytics integration to emerge soon. These aspects will drive further interactivity in future user applications, relying heavily on real-time data interaction.
Tips and Resources for Further Learning
Recommended Books, Courses, and Online Resources
Understanding YouTube API
In today's digital world, video content reigns supreme. Integrating YouTube into various applications can deepen user engagement significantly. By understanding the YouTube API, developers can unlock a wealth of possibilities. This enables richer interactions with one of the world's largest video platforms.
Understanding the YouTube API involves recognizing its fundamental purpose. The API provides a means to interact programmatically with YouTube, which expands functionality and embeds it into existing programs and platforms. By using this API, developers can retrieve videos, manage accounts, or even analyze viewership patterns with relative ease.
What is YouTube API?
The YouTube API allows developers to access and interact with various components of YouTube. This includes functionalities to search for videos, retrieve published channels or manage playlists. Access to extensive video information provides vast potential, particularly for applications that require curated video content.
It functions as a bridge between programs and the vast resources YouTube has built over the years. For instance, a developer can use the YouTube API to display recommended videos, automatically update playlists, or gather statistics on video performance.
Key Features of YouTube API
Several key features set the YouTube API apart:
- Video Search: Retrieve videos based on keywords and parameters.
- Comments and Likes: Post and manage comments as well as view like counts.
- Channel Management: Access channel details and manage playlists dynamically.
- User Authentication: Secure interactions with OAuth 2.0 authorization.
These features allow developers to build varied tools and applications that resonate well with user experience expectations. Early adoption of these capabilities tends to yield greater user loyalty and enhance interaction.
Use Cases for YouTube API
The YouTube API can be leveraged in numerous applications, enhancing both user engagement and functionality. Common use cases include:
- Educational Platforms: Integrating video content in learning management systems by pulling relevant videos based on topics.
- Mobile Apps: Creating smartphone apps that allow users to customize video playlists or receive notifications about specific content.
- Web Development: Enabling dynamic content on websites like blogs, where video content enhances written material.

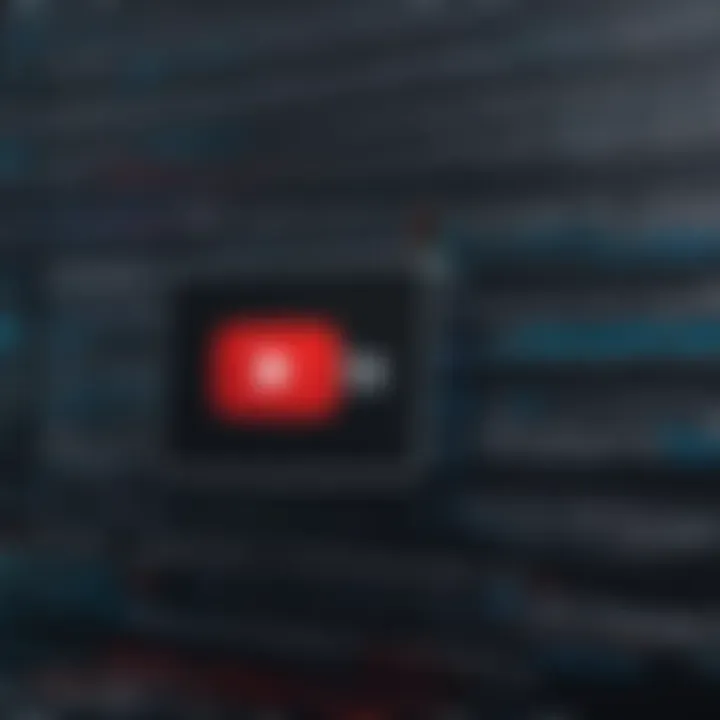
Every case reveals a purpose that enriches the overall user experience. Understanding these uses adds to the relevance of the YouTube API for today's tech-focused developers.
Setting Up Your Environment
Setting up your environment is a crucial first step when working with the YouTube API and JavaScript. Having a correctly configured workspace lays the foundation for a smooth development process. This section emphasizes essential elements needed to begin your journey. Careful consideration of the environment will save you from potential connectivity issues and integration hurdles.
Prerequisites for Using YouTube API
Before you start, you must ensure a few prerequisites are in place. Start with a basic understanding of HTML and JavaScript. Knowing how these languages function will ease your API integration efforts. Here are some key prerequisites to consider:
- Proficiency in JavaScript, as it will be your primary programming language for integration.
- A web browser for testing API responses, where Google Chrome is recommended due to its developer tools.
- Basic knowledge about RESTful APIs can help you grasp how API requests and responses work.
- A Google account to access the Google Developer Console. This account is a gateway to manage your projects and OAuth configurations.
Additionally, installing a code editor is necessary. Options like Visual Studio Code or Sublime Text offer a user-friendly interface to write and manage your JavaScript code effectively. You can also utilize text editors like Atom, which supports a range of plugins for better productivity.
Creating a Google Developer Console Project
Creating a project in the Google Developer Console is mandatory to access the YouTube API. By setting up a project, you will control settings related to your API usage efficiently. Here is how to proceed step by step:
- Sign in to the Google Developer Console.
- Click on the “Create Project” button to initiate a new project.
- Provide a suitable name for your project and check the settings.
- Once everything looks good, click “Create.”
Following these steps, a new project will appear in your console. Always remember that this will allow you to enable APIs and manage credentials, including API keys throughout your development.
Generating API Keys
To interact with the YouTube API, generating API keys is necessary. API keys serve as a unique identifier which allow your application to authenticate itself when requesting resources. Here’s the process:
- In the Google Developer Console, navigate to the “Credentials” section of your project.
- Click “Create Credentials” and select “API Key.”
- A pop-up will show your new API key.
- Make sure to store the key securely but also be informed about user permissions and access levels.
Remember that your API key is sensitive information. Do not share it publicly. To prevent abuse or excessive charges, consider restricting its usage to specific hours or IP addresses if needed. This is an essential practice in managing the security of your application.
API keys provide vital access to the API, but security measures must always accompany usage for safeguarding against misuse.
Authentication Mechanisms
In any interaction with the YouTube API, understanding authentication mechanisms is crucial. These processes help ensure that only authorized users and applications can access the data and features provided by the API. There are clear benefits to employing appropriate authentication methods. First, they protect sensitive information to prevent unauthorized access or data breaches. Second, they enhance the reliability of using the YouTube API within projects. Developers can be confident that the resources they are accessing are secure and trackable. This aspect becomes particularly important since APIs often involve user data, videos, and other personal or private material.
Before delving into the first specific mechanism, OAuth 2.0, it is useful to identify that the type of authentication required generally falls within a few categories. OAuth 2.0 is one of the most commonly used; it permits applications to gain limited access to user accounts on a third-party service without sharing passwords.
Overall, effectively implementing authentication mechanisms will not only protect users but also foster a better development environment.
OAuth 2.
Overview
OAuth 2.0 is the framework that the YouTube API relies on for authenticating and authorizing users. At its core, OAuth 2.0 allows applications to obtain a limited access token. This token can perform operations on behalf of a user without needing their password. One clear advantage is users do not need to share their credentials with the application.
Here are key aspects of OAuth 2.0:
- Authorization Grant: It serves as the application’s ticket to gain an access token.
- Access Token: This token allows the application limited access to the user's account.
- Refresh Token: It is used to obtain new access tokens without re-authenticating the user. This is important since access tokens often have expiration times.
Overall, OAuth 2.0's secure mechanism is beneficial to both users and developers, ensuring the process is manageable yet secure.
Implementing OAuth 2.
in JavaScript
The implementation of OAuth 2.0 in a JavaScript environment involves several steps to orchestrate the authorization flow effectively. Here is a general outline of the approach:
- Create a Client ID and Secret: The first component involves creating a project within the Google Developer Console. Obtain these unique identifiers as they are required during authentication.
- Redirect Users to Authorize: After prompting the user to log in via their Google account, the application then redirects them to the OAuth 2.0 server with necessary parameters, such as scope and redirect URI, included.
- Handle the Authorization Response: Once the user authorizes the app, they receive a code as part of the redirect back to your app. This code is essential for exchanging it later for an access token.
- Exchange the Authorization Code for a Token: A request will now be sent to the token endpoint of the OAuth server, along with the client credentials and obtained code. In response, the server provides an access token and may also return a refresh token.
- Make API Requests: Finally, the API can be called using the access token. Always include it in HTTP headers when making requests to access YouTube resources.
By following these steps, developers can effectively authenticate using OAuth 2.0 within their JavaScript applications. Therefore, having a firm understanding on how to implement OAuth 2.0 will significantly benefit one's integration with the YouTube API.
Tip: Familiarize yourself with the API documentation and ensure to manage token expiration for a smoother user experience.
Making API Requests
Making API requests is a significant aspect of working with the YouTube API in JavaScript. Proper handling of API requests allows developers to fetch, update, or delete data seamlessly. Each request made to the API returns specific data, which can be vital for any application that relies on YouTube content. Knowing this makes it crucial to understand the types of requests and how to handle responses effectively.
API requests are a bridge between an application and YouTube’s resources. Quinn understanding of these requests can help developers utilize the YouTube platform more efficiently. Below are key components of API requests that contribute to a smoother development process.
Types of API Requests
API requests to the YouTube API primarily fall into two categories: GET and POST requests. It is important to note that understanding various types helps to effectively retrieve or manipulate data.
- GET Requests: These are used to request data from the YouTube server. For example, if one asks for a video’s details, a GET request will return essential information such as titles and views.
- POST Requests: These requests are employed to send data to the YouTube server, generally for actions like uploading or editing videos. POST requests may also require various parameters based on the ACH operation being performed.
Understanding these differences assures that developers can craft their API usage more purposefully based on intent.
GET and POST Requests Explained
GET Requests
GET requests serve to retrieve data from the server without making changes to that data. They benefit from ease and speed, providing a direct way to access the required information. Developers often use GET for the following:
- Fetching video statistics
- Obtaining playlist details
- Getting channel information
The syntax for a basic GET request might look like this:
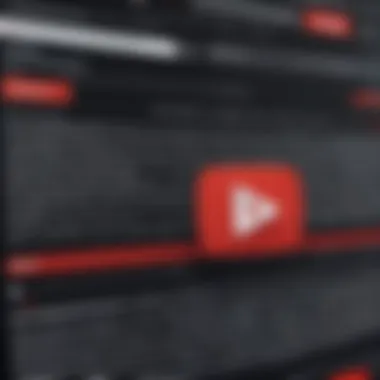
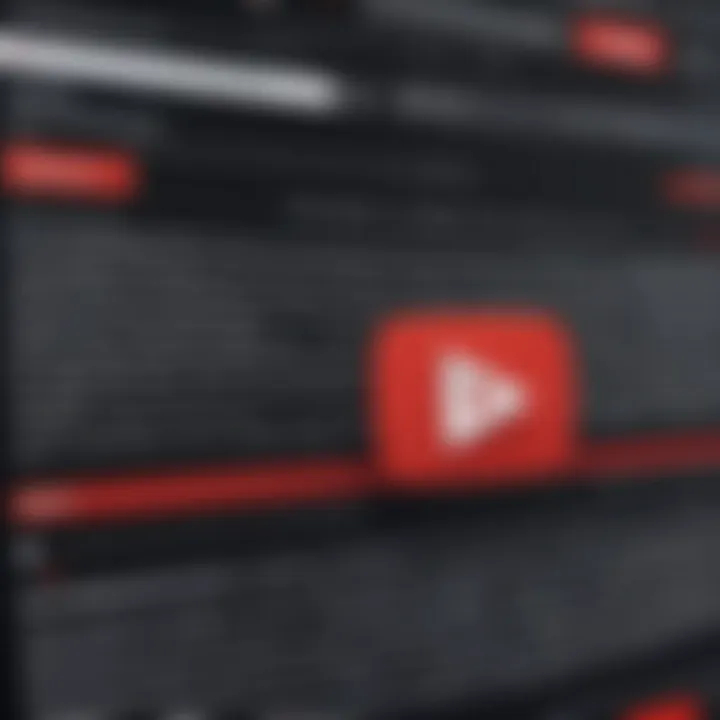
In this code, replace and with actual values to retrieve specific video information.
POST Requests
Post requests are instrumental for adding or updating content on YouTube. They often required structured data formats such as JSON. POST requests engage users more dynamically but come with extra requirements:
- Authorization headers may be needed.
- Data format must align with the server expectations.
An example could be uploading a video by collecting necessary metadata:
Ensure that appropriate permissions are set before attempting upload actions.
Handling API Responses
Understanding how to handle API responses is vital for developers. The process often involves parsing and storing data to make it usable within applications. API responses are generally structured in JSON format, making it a natural fit for JavaScript applications.
Handling requests can often include checking the response (HTTP status codes), extracting data, and accommodating errors. Typical actions taken upon getting a response include:
- Validate Response Status: Ensure that requests return a status code of 200, which indicates success.
- Parse JSON data: Use data structures in JavaScript such as arrays and objects to make sense of the returned information.
- Address Errors: Implement error-handling mechanisms when statuses are other than 200. This can help troubleshoot issues promptly.
A calm yet methodical approach to handling data can lead to improved application performance and user experience.
In summary, mastering API requests along with understanding how to leverage GET and POST methods will empower developers to harness the full potential of the YouTube API.
Common API Endpoints
Common API endpoints serve as fundamental access points to interact with the vast array of functionalities provided by the YouTube API. Understanding these endpoints is vital because they determine how data is organized and how developers can retrieve or manipulate content. This section explores the three essential endpoints: Video Data Retrieval, Playlist Management, and Channel Information Access. Each also provides context for their significance, potential use cases, and how they can optimize your JavaScript applications.
Video Data Retrieval
Video data retrieval is a primary function within the YouTube API, enabling developers to collect essential information about videos hosted on the platform. This might include aspects like title, description, views, likes, or even comments. Accessing this data is crucial for those building applications that seek to present or analyze video content.
Utilizing the endpoint allows for both standalone video details retrieval and batch requests for multiple videos, allowing for efficient data acquisition when necessary. Understanding how to create a correctly formatted API request is imperative. Examples cleanly structured will guide effective usage and minimize request errors. Documentation indicates the specific parameters required, adding to the necessity for precise coding practices.
Usage example
Playlist Management
Managing playlists via the YouTube API opens a vast range of possibilities for users desiring to customize viewing experiences. Developers can create, modify, or delete playlists, alongside adding or removing videos from them.
The and endpoints are particularly relevant, as they allow a structured way to provide users with options tailored to their preferences which means increased engagement and value.
Implementing functionality for users to interact with playlists motivates retention rates; additionally, understanding the playlist management inherently feeds engagement statistics back into the developer’s analysis later on. Devs can emphasize sharing features using these endpoints, thereby leveraging YouTube's communal nature.
Channel Information Access
Access to channel information is vital for applications designed to provide user insights about content creators. The API facilitates retrieving data such as a channel's description, view counts, subscriber numbers, and more, through the endpoint.
By implementing these access mechanisms, developers enhance user engagement with browse tools that can immediately surface popular channels, identified by category or user interest. This allows applications to curate custom lists, enabling heavy user interaction and promoting discoverability. The continuous flow of updates via this channel-centric data is beneficial not only from a user experience perspective but can also tremendously fuel promotional activities if a certain channel becomes prominent.
Extracting and utilizing information effectively from these common API endpoints enable developers to create richer applications that enhance user engagement and drive efficiency. Each endpoint serves a purpose and should be leveraged according to the specific needs of the application being developed.
Data Manipulation and Display
Data manipulation and display is crucial for effectively harnessing the capabilities of the YouTube API within JavaScript applications. Through this process, developers can interpret and present data in a meaningful way to users. This section provides insight into parsing JSON responses, rendering data in HTML, and creating interactive components. Each aspect plays a vital role in building a user-friendly experience that leverages the extensive data offered by YouTube.
Parsing JSON Responses
When working with the YouTube API, data is returned in the form of JSON (JavaScript Object Notation). Understanding how to parse these JSON responses is fundamental for any developer engaging with the API. After making a request, the data typically arrives in nested objects and arrays, which can seem complex at first.
The main objective here is to extract relevant information for use in your applications. For instance, if you retrieve data about videos, the response may include titles, thumbnails, and descriptions. Using JavaScript, the parsed data can be efficiently accessed and manipulated.
The parsing process can use methods, such as:
- : This allows developers to convert a JSON string into an object that can be easily worked upon.
- Accessing object properties: Once parsed, properties can be accessed easily to retrieve various details. This step is fairly simple, yet vital.
Rendering Data in HTML
Once the data is parsed, the next step involves presenting the information through the user interface. Rendering this data in HTML is where a clean and organized format can greatly enhance user experience. Quality rendering considers aesthetic design and functionality.
Through techniques in JavaScript, such as the creation of HTML elements and appending them to the document DOM, developers can reveal data like video titles and views efficiently to users. Additionally, using template literals in JavaScript helps in dynamically inserting values from the JSON data into the HTML structure, ensuring every detail is effortlessly displayed.
For instance, a simple video card representation can look like:
Using such methods allows the data to be not only visible but also engaging for the users.
Creating Interactive Components
In addition to merely displaying information, incorporating interactivity offers a more dynamic experience. Interactive components, such as buttons, modals, and sliders, can make the application more effective and appealing. Such elements allow users to engage directly with the presented data. For instance, enabling users to click to view detailed video information or interact with a playlist expands the functional scope of the application.
Developers can achieve interactivity through event listeners in JavaScript, tailored to respond to user actions. Functions handling clicks might fetch more data from the API or allow comments, enhancing user engagement further.
To engage users effectively, ensure the components are intuitive and straightforward.
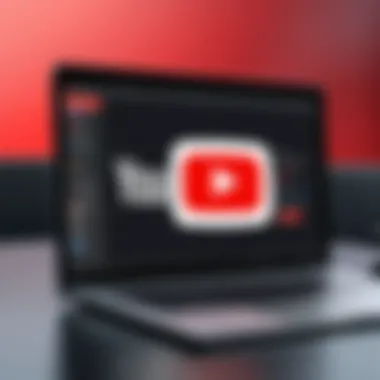
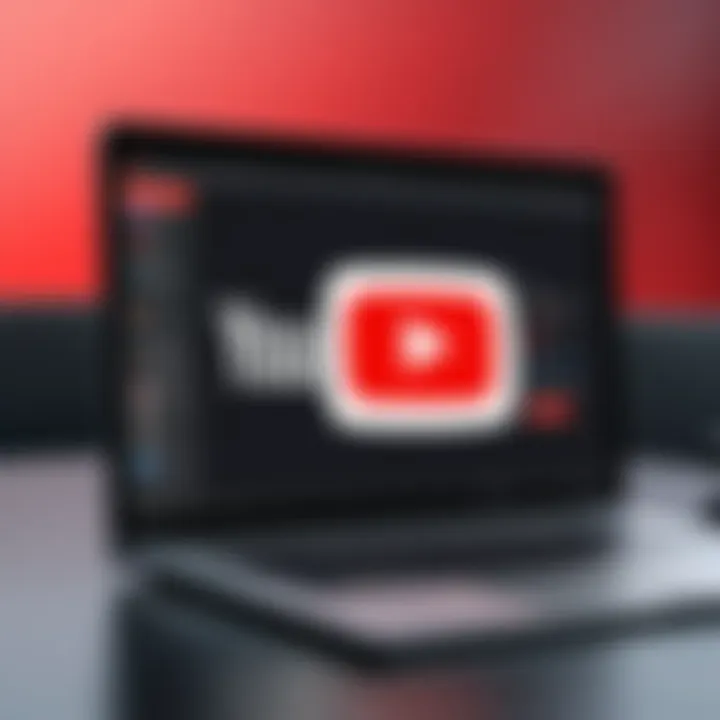
In summary, mastering data manipulation and display is indispensable when integrating the YouTube API with JavaScript. This process simplifies complex data into structured, appealing information that enhances user interaction and satisfaction.
Error Handling Strategies
Error handling is a crucial aspect of working with the YouTube API, influencing both the developer experience and the end user's satisfaction. Effective error management not only helps in isolating problems but also aids in maintaining seamless application performance. Here, we explore common errors that one might encounter when working with the YouTube API, as well as strategies to implement robust error handling in JavaScript.
Common Errors with YouTube API
When integrating YouTube API with your application, understanding potential errors is vital. These errors can arise from several issues, such as network problems, incorrect configurations, or code implementations. The most common errors include:
- 400 Bad Request: This error may be due to malformed syntax or misconfigured requests. This happens often with incorrect parameters passed to the API.
- 401 Unauthorized: This indicates that authentication has failed. Ensure that you are using valid API keys and that your OAuth tokens are correct.
- 403 Forbidden: This error suggests that you lack the necessary permissions, which could relate to the API quotas or insufficient access rights to the requested resource.
- 404 Not Found: Here, it usually means that the particular resource you are trying to access does not exist or was removed from the platform.
- 500 Internal Server Error: This indicates there was a server issue at YouTube’s end. Retry logic is often necessary for this type of error.
- Quota Exceeded: Spending beyond available API quota results in errors as well. Monitoring your API usage is critically important during development.
Recognizing these issues early can prevent costly disruptions in your project's flow.
Effective error handling can significantly improve both user trust and application reliability.
Implementing Error Handling in JavaScript
Writing error handling logic in JavaScript involves implementing codes that can catch and manage these errors gracefully. Here are some structured approaches:
- Try-Catch Blocks: Use try-catch statements to handle exceptions that might occur in synchronous code execution.
- Error Handling Functions: Define functions to manage different error scenarios effectively, allowing for modular and reusable handling.
- Promises and Async/Await: With asynchronous calls, attach .catch to your promises or use try-catch when utilizing async/await syntax to manage errors properly.
By following these outlined strategies, developers can create robust applications that anticipate potential errors and react appropriately, thus fostering a smoother integration experience with the YouTube API.
Best Practices for Efficiency
In the context of integrating the YouTube API with JavaScript, efficiency is a crucial aspect to consider. Proper application of best practices can help developers optimize their projects, reduce potential errors, and enhance user experience. Understanding these practices is central to leveraging the API's capabilities effectively. Through effective performance management, developers can ensure smoother interaction between applications and YouTube’s extensive resources while maintaining the application's responsiveness.
Rate Limiting Awareness
YouTube API has rate limits that restrict the number of requests a client can make in a defined timeframe. Understanding these limitations is vital for any developer. Rate limits prevent abuse and manage traffic to ensure stability for all users. Exceeding these limits often results in denied requests or blocked access to the API for a specified period.
Several points should be understood about rate limiting:
- Types of Rate Limits: YouTube imposes per-project and per-user quotas. A well-designed development strategy should account for these differing constraints.
- Monitoring Usage: Regularly monitor your application's API request counts, adjusting as necessary to avoid reaching these limits. Keeping track of usage patterns will lead to better decision making.
- Graceful Error Handling: When you reach a rate limit, have your code handle this situation gracefully. Include relevant internal messaging in your application.
Developers can leverage strategies to manage requests efficiently, avoiding disruptions to the user's experience. Always incorporate these strategies into your plans.
Optimizing API Calls
Optimizing API calls is essential for maximum performance and minimal downtime. Efficient API interactions can significantly reduce loading times and enhance overall application responsiveness. Here are some guidance tips on optimization:
- Batch Requests: If possible, combine multiple requests into a single call. The YouTube API allows batching certain types of calls. This reduces the number of requests and helps manage rate limits effectively.
- Filter and Specify Data: Make use of the API’s parameters to only fetch necessary fields instead of retrieving unnecessary data. Focusing on specific data will reduce the payload size.
- Caching Responses: Use caching strategies to store previously retrieved data temporarily. This is particularly effective for data that doesn’t change frequently, thus reducing repetitive requests.
- Leverage Webhooks: Whenever applicable, implement webhooks to receive live updates from YouTube instead of frequently polling for changes. This approach minimizes the number of requests made and maximizes the API’s potential.
Implementing these strategies can significantly enhance your application's performance. It's the small details that often bring about the biggest benefits over time.
In summary, being aware of the inefficiencies in request handling can foster thoughtful development. Incorporating optimized practices into your interactions with the YouTube API can lead to robust and efficient results.
Future of YouTube API with JavaScript
As YouTube continues to evolve its platform, the integration of its API with JavaScript becomes increasingly vital. The Future of YouTube API with JavaScript is not just about syntax and implementation. It speaks to the ability of developers and companies to tap into a resource that hosts millions of videos and user interactions globally. This section examines prevailing trends, updates, and innovative approaches to utilizing the YouTube API within contemporary and forthcoming applications.
Emerging Trends and Updates
The landscape surrounding the YouTube API changes constantly. YouTube followers expect enhanced experiences, fresh content, and new features. This results in iterative updates to the API itself. APIs generally evolve in specific directions to meet user needs and technological advancements. Some recent noteworthy trends include:
- Increased Focus on Machine Learning: Leveraging ML for recommendations based on user behavior offers user-personalized experiences, improving engagement over time.
- Integration with Mobile Standards: With the rise of mobile internet usage, the API seeks closer compatibility with platforms like React Native and Progressive Web Apps (PWAs).
- Advanced API Management Tools: Increasingly sophisticated tools make monitoring usage and performance simpler, allowing developers to maintain efficient workflows.
- Live Streaming Capabilities: YouTube expands streaming features allowing developers to integrate live video functionalities into their applications. This can enhance user engagement significantly.
Recent developments indicate a shift towards accommodating developers who favor a more unified ecosystem for all aspects of application development, prioritizing speed and efficiency.
These trends are redefining interaction with the platform, positioning the YouTube API as a cornerstone for modern web applications. Changes promise better performance, user satisfaction, and by extension, greater adoption of services that rely on this data.
Potential Use Cases in New Technologies
As technology advances, the scope of YouTube API applications broadens. Here are some significant pointers regarding its potential utility given significant current trends:
- Virtual and Augmented Reality (VR/AR): Integrating YouTube's video capabilities into VR environments provides immersive experiences for content consumption. Applications here can scale how users enjoy immersive, simulated environments with video content.
- Smart Devices: Increased use of IoT means that developers have a vast opportunity. Smart screens outfitted with YouTube capabilities could serve users on the go, streaming vital information or entertainment.
- E-commerce Applications: Nowadays, shoppers often look for product demonstration videos before purchasing goods. Embedding YouTube for product demonstrations or reviews might enhance shopping experiences, thereby fostering more conversions.
- Educational Platforms: Educators and learners alike have much to gain. YouTube enables the incorporation of multimedia resources to augment classroom instruction. This can lead to enriched learning experiences and content delivery across digital platforms.
Overall, the YouTube API with JavaScript presents an exciting avenue for innovation. As this reach enhances, the potential for wide-ranging applications in various sectors means that developers and organizations remain well-positioned to translate emerging technology choices into impactful applications. This adaptability will be key to thriving in the dynamic tech landscape.
Finale
The conclusion of this article acts as a essential component that captures the main elements discussed throughout. By coherent summarization, it aids reader convenience. It highlights critical takeaways about how the YouTube API integrates efficiently with JavaScript.
Summarizing Key Takeaways
In skimming through everything presented in the article, several notable points stand out:
- The YouTube API is a extensive tool for accessing various functionalities related to video and channel data.
- Understanding how to authentication mechanisms, specifically OAuth 2.0, is crucial for secure communication with the API.
- API requests are not solely limited to retrieving data; they also encompass sending data and managing various elements like playlists and comments.
- Error handling remains integral throughout integration. Knowing common errors and installation of error management in your code simplifies development.
- Practical understanding of manipulative techniques like parsing JSON and rendering data effectively enhances the end-user experience.
Given these insights, a grasp of YouTube API allows for a deeper engagement in software development and projects focusing on video content.
Encouragement for Further Exploration
Venturing beyond the established guidelines of YouTube API can enhance knowledge. As each API receives updates and new features, following the emerging trends sustains project relevance.
- Stay Updated: Keep an eye on YouTube's official documentation for the latest latest features and updates.
- Join Communities: Engage with forums like reddit.com to discuss experiences and challenges faced when using various functions of YouTube API, especially with JavaScript.
- Experimentation: Don’t achieve mastery by mere observation. It benefits to get your hands dirty by building small applications utilizing the API.
- Best Practices: Implement best practices of rate limiting and efficient calls as you develop larger scalable applications.
Exploring these paths not only fortifies understanding, but also nurtures the skills needed in technical fields especially relational to web-based projects in continuance growth.
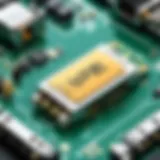
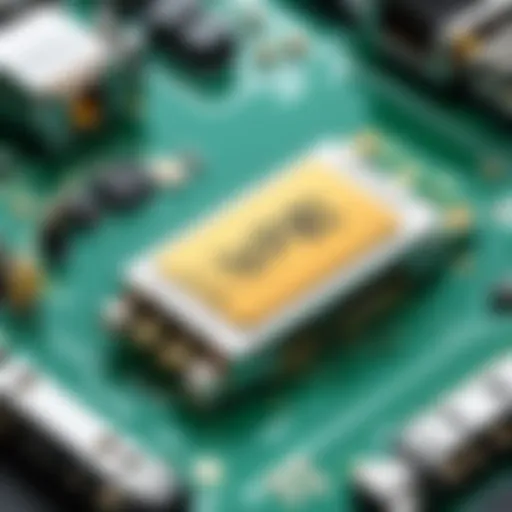