Exploring Inter-Process Communication in Computing
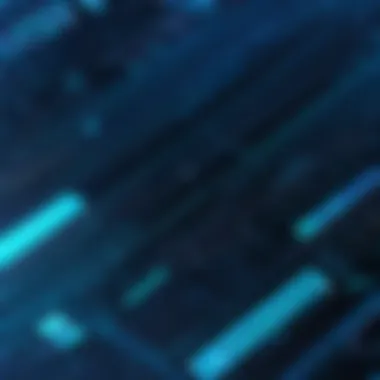
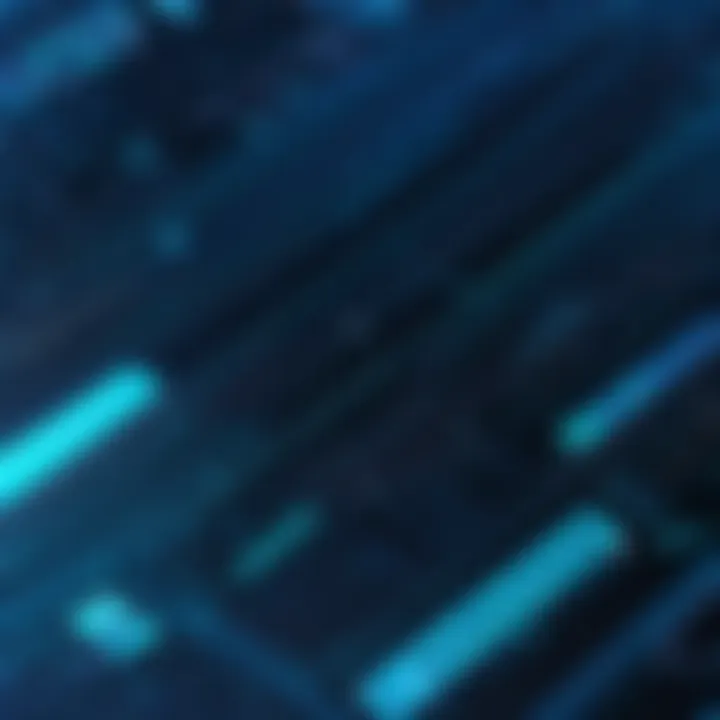
Overview of Topic
Preface to the main concept covered
Inter-Process Communication (IPC) constitutes a fundamental component in the realm of computing, enabling processes (which can be thought of as individual tasks) to communicate and operate in cohesion. Whether it’s a simple desktop application sharing data with another or complex systems communicating in a distributed environment, IPC serves as the vital bridge facilitating this connection.
Scope and significance in the tech industry
In today’s tech landscape, where efficiency and speed reign supreme, IPC is more relevant than ever. It ensures that applications can operate seamlessly within operating systems, making it especially crucial in multi-core processing and distributed systems. The effectiveness of any computing environment often hinges on how well IPC is implemented.
Brief history and evolution
Tracing back to the earlier days of computing, IPC began as rudimentary methods of sharing data between processes. Over the decades, it has evolved significantly alongside advances in multicore processors and distributed architecture. These developments have culminated in sophisticated methods such as message queues, semaphores, and shared memory mechanisms, each catering to different needs in computational tasks.
Fundamentals Explained
Core principles and theories related to the topic
At its heart, IPC hinges on several core principles. The idea is to provide mechanisms through which processes can exchange data safely and efficiently. Key principles include synchronization, to ensure processes do not interfere with each other, and communication, to ensure the timely exchange of information.
Key terminology and definitions
Familiarity with the lexicon of IPC is crucial for understanding its workings. Here are some essential terms:
- Process: An instance of a program in execution.
- Message Passing: A communication method where data is sent between processes as messages.
- Shared Memory: A segment of memory accessible by multiple processes.
Basic concepts and foundational knowledge
IPC encompasses various forms of communication methods. Each has its use cases depending on the requirements at hand.
- Pipes: One-way communication channels between processes.
- FIFOs: Named pipes that allow data to flow bi-directionally.
- Sockets: Commonly used for communication between processes on different machines over a network.
Practical Applications and Examples
Real-world case studies and applications
IPC is used broadly in real life. For instance, web browsers use IPC to separate different tabs as distinct processes, enhancing both performance and security. Similarly, in microservices architecture, different services communicate via IPC methods, often utilizing RESTful APIs.
Demonstrations and hands-on projects
Imagine you have two simple processes: one that generates random numbers and another that processes these numbers. You could employ a message queue to send data from one to the other, ensuring that the consumer process runs only when data is available. Here’s a small pseudocode snippet for clarity:
Code snippets and implementation guidelines
Here's a simple example of using named pipes in Python:
Advanced Topics and Latest Trends
Cutting-edge developments in the field
The landscape of IPC is continuously shifting, with new techniques emerging to meet the demands of modern computing. Technologies like ZeroMQ and gRPC are gaining traction for their efficient data transmission capabilities in distributed systems, offering features that traditional methods lack.
Advanced techniques and methodologies
With the rise of microservices, service mesh frameworks like Istio have come into play, orchestrating communication between services to ensure reliability and security. These techniques not only enhance performance but also provide a layer of management over processes.
Future prospects and upcoming trends
Looking ahead, there is a palpable shift towards utilizing asynchronous communication models and event-driven architectures, which promise greater flexibility in handling a myriad of processes. As cloud computing expands, we anticipate even more intricate IPC methods designed to optimize performance in heterogeneous systems that span across multiple geographic locations.
Tips and Resources for Further Learning
Recommended books, courses, and online resources
For those keen on diving deeper, consider the following resources:
- "Operating System Concepts" by Abraham Silberschatz
- Online courses from platforms like Coursera or edX focused on Operating Systems.
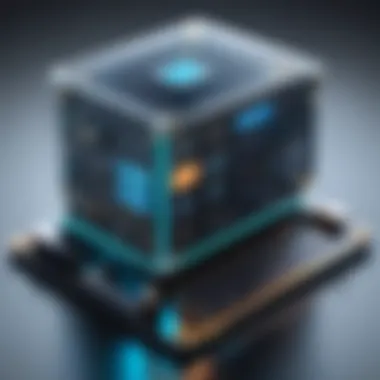
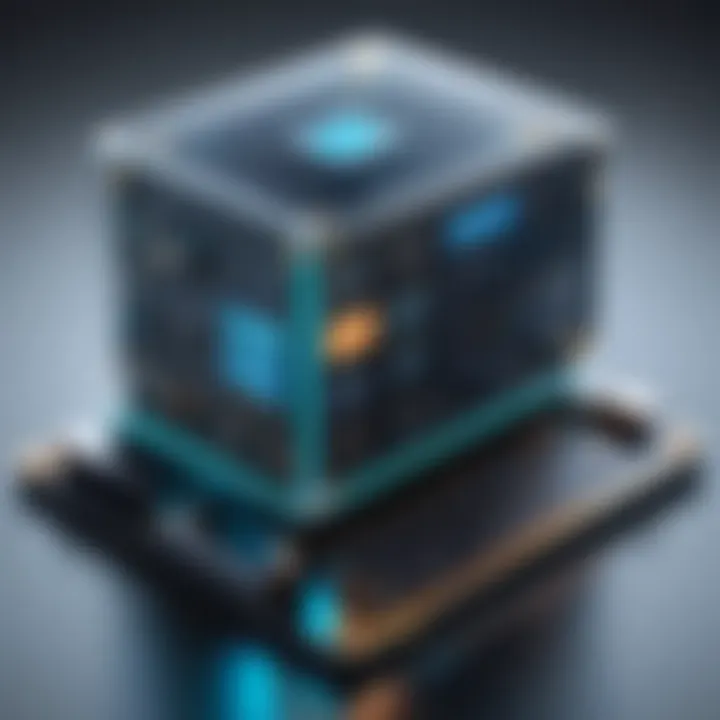
Tools and software for practical usage
Some notable tools that aid in IPC development include RabbitMQ for message queuing, and Docker for containerized processes, permitting simplified management and communication between microservices.
Remember, grasping IPC is essential not only for students and budding programmers but also for seasoned IT professionals looking to refine their skills in designing efficient and reliable systems.
Preface to IPC
Inter-Process Communication (IPC) serves as a vital backbone in the architecture of modern computing. It's the unsung hero that facilitates interaction between processes, promoting efficient data exchange and coordination. Without IPC, processes would remain isolated, rendering many multitasking functionalities of operating systems ineffective.
Definition of Inter-Process Communication
Inter-Process Communication fundamentally refers to the mechanisms that allow processes to communicate and synchronize their actions. This includes exchanging data and signaling events. Picture two chefs in a busy kitchen; they need to communicate about dish components to avoid confusion in meal preparation. Similarly, in computing, IPC ensures smooth collaboration among diverse software components, be it across a single machine or a network of machines. This coordination can take many forms, such as sharing data, passing messages, or managing resources concurrently. Each of these processes requires effective IPC methods to operate harmoniously.
Importance of IPC in Computing
IPC plays a pivotal role in ensuring that complex applications run efficiently. Here are some key points that highlight its significance:
- Resource Sharing: Processes often need to share resources, such as memory or data files. IPC provides the means to manage these shared environments effectively, ensuring no two processes step on each other's toes.
- Collaboration: Various components of software applications need to work together. IPC facilitates collaborative efforts by allowing processes to send messages to each other, thus enabling teamwork in computational tasks.
- Data Integrity: In a multi-process environment, it’s crucial to maintain data integrity. IPC methods help in coordinating access to shared data, preventing conflicts and ensuring that processes can read and write data without corruption.
- Scalability: As systems grow, good IPC mechanisms enable efficient scaling. With adequate IPC designs, more processes can be added to a system without significant performance degradation.
Overall, understanding IPC is key for anyone involved in software development or IT. It is essential for crafting responsive and efficient applications that can harness the power of multi-core processors and distributed systems effectively. IPC is not just a technical concept; it's about enabling communication, enhancing efficiency, and ensuring that processes can work together without stepping on each other's toes.
Effective Inter-Process Communication can significantly improve application responsiveness and reliability, making it a critical aspect of system design.
As we delve deeper into the various types of IPC mechanisms and their applications, you'll see how these strategies form the foundation of robust computing environments.
Types of IPC Mechanisms
Understanding different types of IPC mechanisms is crucial for anyone working in computing. These mechanisms serve as the backbone for communication between processes, allowing them to efficiently share data and respond to various computational demands. Each method has its strengths and weaknesses, shaped by the specific needs of different applications and environments. As we delve into these mechanisms, we’ll uncover how each one plays a distinct role in the grand scheme of inter-process communication.
Message Passing
Message passing is a fundamental IPC mechanism that facilitates communication between processes through discrete messages. Unlike shared memory, where processes can access common data, message passing encapsulates data in messages that must be sent and received explicitly. This method is particularly effective in distributed systems where processes may not share a common address space.
Benefits:
- Isolation: Each process operates in its own memory space, reducing the chance of data corruption.
- Simplicity: Message passing can simplify the design of concurrent systems.
- Scalability: Suitable for distributed applications, allowing processes on different machines to communicate seamlessly.
Despite its advantages, message passing can also introduce latency and overhead due to message creation and delivery. For instance, think of an email chain; while everyone can contribute to the conversation, responses can sometimes take a while to arrive.
Shared Memory
Shared memory is another widely used IPC mechanism. In this approach, processes communicate by reading and writing to a shared area of memory. This method can enhance performance, as it eliminates the overhead of message passing. However, it also introduces complexities related to synchronization and data consistency.
Key Considerations:
- Speed: Accessing shared memory is generally faster than passing messages.
- Control: Requires careful management to avoid race conditions and ensure data integrity.
To illustrate, imagine a cooking scenario—multiple chefs working in the same kitchen (shared memory). They must coordinate and manage their tasks effectively to prevent chaos, just as processes must synchronize their access to shared data.
Pipes
Pipes provide a unidirectional flow of data between processes. They work by allowing one process to write data while another reads it, acting like a channel. This method is particularly useful for applications that follow a producer-consumer model.
Characteristics:
- Simplicity: Pipes can be easier to implement for simple producer-consumer tasks.
- Limitation: Typically, they are restricted to communication between related processes, such as those spawned from the same parent process.
With pipes, think of a water pipeline—crucial for transporting water from one point to another but only functioning effectively if the source and destination are connected directly.
Sockets
Sockets represent a versatile IPC mechanism that allows communication over a network. They can be used for both local and remote communications, making them invaluable in networked applications.
Advantages of Sockets:
- Flexibility: Can facilitate communication between processes on the same computer or across the internet.
- Robustness: Built on established protocols, they can support complex interactions between multiple machines.
When you consider a web server responding to browser requests, sockets are the unsung heroes managing that traffic. They establish a connection, enabling seamless data exchange that fuels the modern web experience.
In summary, each IPC mechanism has its own unique benefits and considerations that suit various computational needs. As you deepen your understanding of these methods, it becomes easier to choose the right IPC mechanism for a given scenario, enhancing the efficiency and effectiveness of your software solutions.
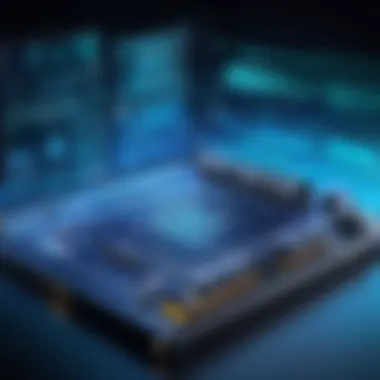
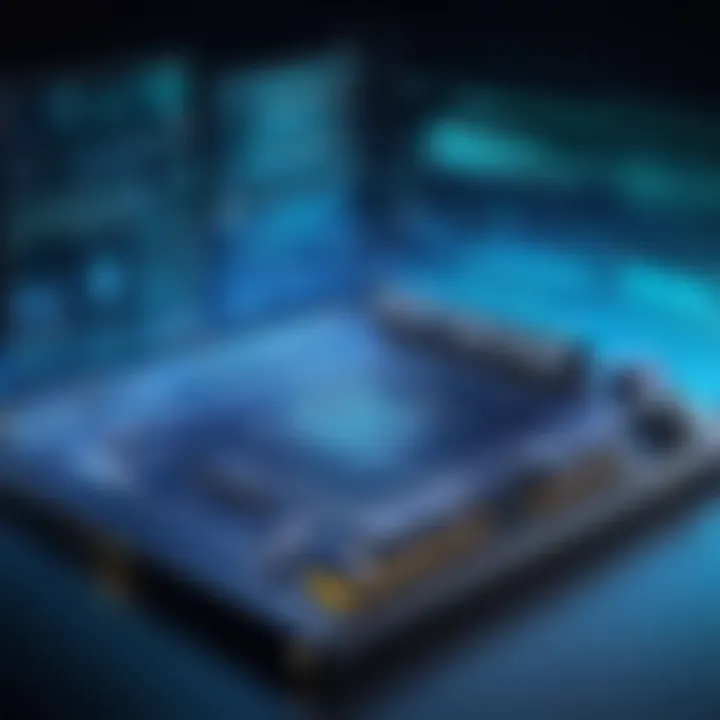
IPC in Different Operating Systems
Inter-Process Communication (IPC) is not just a one-size-fits-all concept; it varies significantly depending on the operating system being used. This section delves into how different environments implement IPC, highlighting unique characteristics, benefits, and considerations that arise in Unix/Linux, Windows, and even cross-platform scenarios. Understanding these differences is crucial for developers and IT professionals looking to optimize communication between processes in their respective systems.
IPC in Unix/Linux
Unix and its derivatives, like Linux, offer a rich suite of IPC mechanisms. The design philosophy here often emphasizes simplicity and modularity. In these systems, IPC is deeply integrated into the kernel, enabling high efficiency when processes share data and signals. Some common methods include:
- Pipes: These allow for a unidirectional data flow between processes. They're straightforward for parent-child process communication, making them perfect for scripting and automation.
- Message Queues: This method provides asynchronous communication, where messages are stored in a queue until processed. It’s handy for managing data flow without overwhelming processes.
- Semaphores and Shared Memory: These mechanisms are utilized to synchronize access to shared resources, critical in multi-threaded applications.
The Unix/Linux approach to IPC benefits from being lightweight and efficient, but it also demands a good grasp of system calls and data structures. Therefore, new programmers might initially find these aspects daunting.
IPC in Windows
Windows approaches IPC with a focus on ease of use and integration into its GUI-centric environment. It provides several mechanisms that are different in terms of their API and intricacies:
- Named Pipes: These are similar to Unix pipes but can be used for communication between unrelated processes, typically across networked machines.
- Message Queues: Like in Unix, Windows also supports message queues, allowing for the sending of messages between processes safely and in an orderly fashion.
- Windows Sockets: This allows processes to communicate over network protocols, which is extremely beneficial in distributed applications.
The Windows API's documentation is often more user-friendly, making it simpler for developers, especially those familiar with Microsoft's development tools. But this approach may come with some overhead, impacting performance in resource-sensitive applications.
Cross-Platform IPC Solutions
As software systems become more complex and diverse, the need for cross-platform IPC solutions has become necessary. Here, developers seek methods that can operate seamlessly across Unix, Windows, and other systems. Some noteworthy solutions include:
- ZeroMQ: Often dubbed the "socket library on steroids," it provides a high-level messaging abstraction that can handle various transport protocols.
- gRPC: This involves using remote procedure calls, allowing for efficient communication between various applications written in different programming languages on different platforms.
- RabbitMQ or Kafka: Both are robust message broker systems that facilitate communication between distributed applications or microservices architecture.
These solutions often abstract the underlying IPC mechanisms, providing a developer-friendly interface while still maintaining high performance, albeit sometimes at the cost of increased complexity in setup and configuration.
"The choice of IPC mechanism is often dictated by the application requirements, the operating system environment, and performance expectations. Each method has its nuances and best practices to harness its full potential."
In summary, IPC methods differ greatly across operating systems, and understanding these variances can significantly impact application design and efficiency. Knowing the ins and outs of each system’s capabilities helps in making informed decisions in software architecture and design.
Challenges in Implementing IPC
Implementing Inter-Process Communication (IPC) presents a series of hurdles that can greatly impact system functionality. Understanding these challenges is essential for anyone involved in systems design and software development. When processes need to communicate, several specific problems can arise, which not only complicate the implementation but can also hinder performance and reliability of applications. As we unravel this section, it’s clear that addressing these issues is not just about making IPC work—it's about making it work effectively and securely.
Synchronization Issues
Synchronization is critical in IPC to ensure that processes do not interfere with each other when accessing shared data or resources. Without proper synchronization, you can run into situations where one process might read or write data at the same time another is modifying it, leading to unexpected behaviors or corrupt data. For example, imagine two bank applications trying to update the same account balance concurrently. If synchronization is poorly managed, it might result in an incorrect balance being displayed or even loss of funds.
To mitigate these issues, developers often use mechanisms like mutexes, semaphores, or other locking techniques that enforce a strict order of access to shared resources. Each of these solutions has its drawbacks, and finding the balance between performance and safety becomes a key consideration. Overly complex synchronization methods can cause bottlenecks, while too lax an approach might lead to race conditions.
Deadlocks
Deadlocks often appear as the dark horse of IPC challenges. A deadlock occurs when two or more processes are waiting indefinitely for each other to release resources that they need to continue executing. Take a simple analogy: Picture two people at a dinner table, each holding a fork, but they need both forks to eat. If both refuse to relinquish their forks, they will just sit there, getting hungrier and hungrier without any resolution. The same principles apply to processes in a computing environment.
Preventing deadlocks involves careful design, like avoiding circular wait conditions, using timeout mechanisms, or employing resource ordering strategies. However, these measures often increase the complexity of the IPC system, as they require a deep understanding of the interdependencies of various processes. Developers have to think two steps ahead, predicting how processes will interact under various circumstances, which can be a tall order.
Security Concerns
When designing an IPC system, security can’t take a backseat. Processes running in the same system may not necessarily trust one another. If one malicious process is able to intercept or manipulate the communication between other processes, the implications can be catastrophic. Envision a situation where an application that processes payment information receives altered data due to an insecure IPC mechanism—this could lead to financial fraud or data breaches.
To address these security concerns, it’s important to implement a robust set of checks and balances. Secure data transmission can be facilitated through protocols that ensure encryption and validation. Moreover, access control measures must be put in place to prevent unauthorized processes from sending or receiving messages. The challenge lies in integrating these security measures without adding too much overhead, which can affect the performance of the IPC mechanism.
Keeping an eye on synchronization, deadlocks, and security is essential for building a sturdy IPC framework. Each element intertwines with the others, making it imperative to tackle these challenges holistically.
Optimizing IPC Performance
Optimizing the performance of Inter-Process Communication (IPC) is a key factor in ensuring that processes communicate swiftly and effectively. In any computing environment, particularly in complex and dynamic systems, the way processes share data can greatly impact overall performance. An efficient IPC system minimizes delays, maximizes throughput, and ultimately enhances the user experience through faster data handling.
Reducing Latency
Latency is a term that refers to the delay between a request being sent and the response being received. Higher latency can slow down the entire system, making applications feel sluggish. There are several ways to tackle latency issues in IPC:
- Direct Communication: Opting for direct communication between processes can cut down on unnecessary layers that might add delay. For instance, using sockets instead of a more convoluted messaging framework can trim the fat and get data where it needs to go faster.
- Efficient Buffering: Implementing efficient buffering techniques ensures that data is ready to go when a process calls for it, avoiding stop-and-go conditions. Utilizing ring buffers or queue systems can help maintain a smooth flow of information.
- Asynchronous Techniques: Switching to asynchronous communication allows processes to continue working while waiting for a response, reducing the apparent waiting time from a user's perspective. This might involve callbacks, promises, or event streams which give a non-blocking feel to the operations.
By focusing on reducing latency, systems become not only faster but also more responsive to user demands, leading to a more satisfying experience overall.
Efficient Data Serialization
Data serialization is the process of converting data into a format suitable for transmission. It's a crucial part of IPC since data needs to be packed tightly for efficient transport. Inefficient serialization can lead to bloated messages and hog bandwidth, dragging performance down.
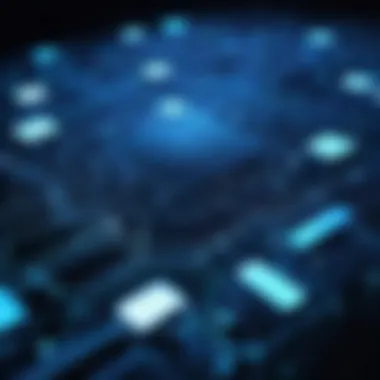
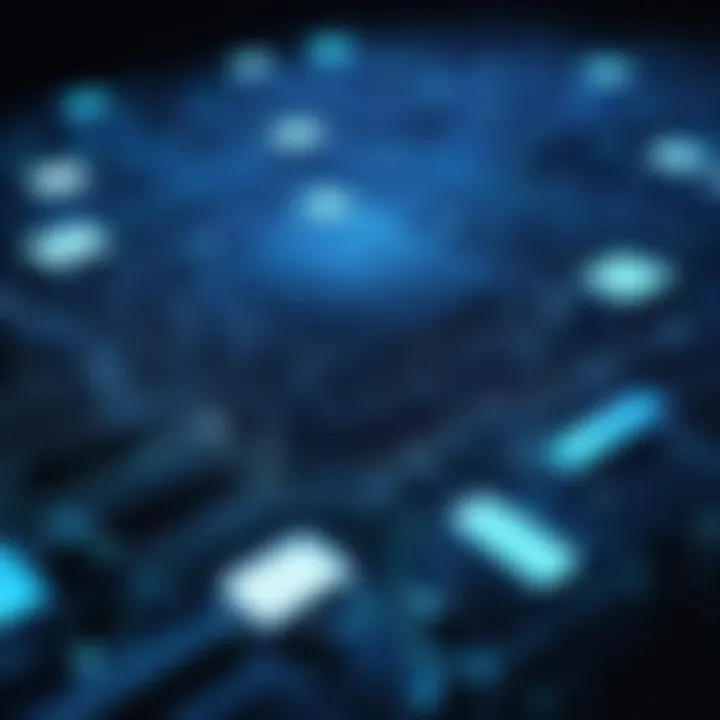
A few strategies to streamline serialization include:
- Use of Binary Formats: Compared to textual formats like JSON or XML, binary formats are generally more compact and faster to process. Protocol Buffers and MessagePack are examples of often-used serialization formats that are both speedy and lightweight.
- Versioning Control: As data evolves, especially in dynamic environments, controlling the version of serialized data can prevent compatibility issues. Adapting data structures gracefully can maintain a stable and efficient communication layer.
- Streamlined Encoding: Avoid redundant information within serialized data. This can involve using identifiers instead of repeating field names or opting for shorter representations of common data types.
By improving data serialization processes, one can achieve significant gains in IPC performance, aiding better resource utilization and quicker information transfer between processes.
Load Balancing Techniques
Load balancing is about distributing workloads across multiple resources effectively. In IPC, it helps avoid bottlenecks and ensures no single component is overwhelmed with requests. Here are methods to enhance load balancing:
- Round Robin Scheduling: This straightforward technique gives each process an equal share of the communication resources, preventing any one process from getting swamped with tasks while others sit idle.
- Dynamic Load Monitoring: Continuously observing process loads can allow automated systems to dynamically reroute tasks to underutilized resources, maintaining efficiency across systems.
- Prioritization: Not all processes have the same urgency. Implementing a priority-based system can ensure that critical tasks receive the bandwidth they require during peak times.
Good load balancing ultimately smooths out the overall operation of IPC, leading to faster processing times and improved reliability in data transmission.
IPC in Distributed Systems
Inter-Process Communication plays a pivotal role in the architecture of distributed systems. As the need for systems to communicate and share resources has grown, particularly in cloud computing and large-scale enterprise applications, IPC methods have become crucial to ensuring data integrity and operational efficiency across various processes, often located on different machines.
Distributed systems are characterized by multiple independent processes that run on different servers or nodes. They require robust communication mechanisms to sync data and coordinate actions. Without effective IPC, these systems risk running amok, leading to data inconsistencies or loss. Therefore, understanding IPC in the context of distributed systems is not just beneficial; it’s critical.
The key elements and benefits of IPC in distributed systems include:
- Scalability: Processes can run independently, increasing the system’s ability to handle numerous requests simultaneously. This means as system demand increases, additional nodes can be integrated without much disruption.
- Fault Tolerance: If one node fails, others can still function normally. Processes can communicate and check on each other, which safeguards the overall system performance.
- Resource Sharing: Efficient IPC enables processes to share resources, including data and services, while maintaining isolation. This is imperative in multi-tenant environments common in cloud services.
- Improved Performance: The right IPC methods can significantly enhance system performance, reducing latency and increasing throughput, which is paramount when dealing with high traffic or complex computations.
The importance of IPC cannot be overstated when working with distributed systems. As these systems grow and evolve, recognizing how processes communicate helps developers design better applications.
Best Practices for IPC
In the realm of Inter-Process Communication, adhering to best practices can significantly enhance the efficiency and reliability of data exchange. As technology continues to evolve, the need for robust methods to ensure smooth communication between processes becomes paramount. This section will explore three key aspects: designing robust interfaces, choosing the right IPC method, and the strategies for testing and debugging IPC implementations.
Designing Robust Interfaces
Designing robust interfaces is the cornerstone of effective Inter-Process Communication. Think of it as creating a bridge between two islands; each side needs to know what to expect. A well-designed interface minimizes misunderstandings and enhances the interaction between different processes.
- Clear Protocols: Establish clear protocols for communication. These should outline the formats of the messages, types of data exchanged, and the expected behaviors of both sender and receiver. This clarity reduces the chances of miscommunication.
- Error Handling: It’s critical to incorporate error detection and handling mechanisms. If one process doesn’t receive the expected data or a timeout occurs, it should have a plan B to handle the situation gracefully rather than crashing or hanging.
- Versioning: When developing interfaces, consider versioning. As systems evolve, maintaining backward compatibility can often be a challenge. Having version numbers allows processes to communicate without halting because of schema changes. This is especially helpful in large systems where many processes depend on each other.
"A robust interface is like a well-oiled machine, ensuring that each component runs smoothly without unnecessary friction."
Choosing the Right IPC Method
Selecting an appropriate IPC method is crucial for the effective communication between processes. Each method has its benefits and trade-offs, which must be carefully weighed based on the specific application needs.
- Message Passing: This method is suitable for loosely coupled systems. It doesn’t require shared memory, which often simplifies synchronization but may introduce higher latency. It’s generally a good choice for systems where processes may not need to share data frequently.
- Shared Memory: This is ideal when high-speed communication is required, as it allows processes to access the same memory space directly. However, it also introduces complexity in managing access to the shared data, requiring proper synchronization strategies.
- Sockets: If processes are running on different machines, sockets provide a reliable method of communication over networks. They’re versatile and support different protocols, catering to a wide range of applications.
Incorporating the right IPC method can significantly boost the performance and reliability of inter-process communications. One must take into account the specific requirements of the application, such as speed, complexity, and data volume.
Testing and Debugging IPC
Testing and debugging Inter-Process Communication can be tricky, primarily because issues often arise from the asynchronous nature of process interactions. To tackle these challenges effectively:
- Simulation Tools: Use simulation tools to mimic different scenarios. This will help you uncover potential weaknesses in communication. For example, testing how processes behave under heavy load can reveal bottlenecks in the system.
- Logging: Implement comprehensive logging for all IPC interactions. This will help in tracing issues when something goes amiss. By reviewing logs, you can determine whether the problem lies in data formatting, unexpected behaviors, or synchronization issues.
- Unit Tests: Create unit tests specifically for your IPC mechanisms. This helps ensure that each communication method functions as intended in isolation before being integrated into larger systems. Consider edge cases during testing to catch unexpected behaviors before deployment.
By following these best practices, you can ensure that the Inter-Process Communication mechanisms you design and deploy are not only effective but also resilient to the challenges that may arise during their operation.
Future of IPC
The landscape of Inter-Process Communication (IPC) continues to evolve at a rapid pace, driven by advancements in technology and changes in how we architect our computing systems. To understand the future implications of IPC, one must grasp the transformative elements that are reshaping methods of communication between processes.
Trends in Technology
As we tread further into a technologically driven age, there are several trends that directly influence IPC. For starters, the rise of cloud computing is revolutionizing data handling and storage. Processes must communicate not just locally but also across geographical barriers. This necessitates more efficient and robust IPC methods. Technologies such as containerization and orchestration tools like Kubernetes have emerged, allowing processes to run in isolated environments while still being able to easily share data when needed.
The prominence of microservices architecture is another critical trend impacting IPC. Rather than monolithic applications, developers are now favoring small, independently deployable services that communicate over networks. In this framework, protocols like REST (Representational State Transfer) and gRPC (Google Remote Procedure Call) are becoming commonplace, enhancing efficiency and responsiveness in communication. With this shift, developers must be astute in selecting the right IPC methods that align well with their architecture.
Additionally, the push towards edge computing is reshaping data transfer dynamics. By processing data closer to the source, latency is reduced significantly. This presents new challenges for IPC as processes at the edge require high-speed communication protocols. Such developments underscore the necessity for scalability and adaptability of IPC mechanisms in upcoming technologies.
Machine Learning and IPC
The integration of machine learning into IPC is an area ripe with potential. As systems become more autonomous, they will require advanced means of communicating insights and adjustments between processes. Here, IPC can provide a backbone for sophisticated data exchange, enabling intelligent systems to react and learn from environments with minimal lag.
Moreover, machine learning algorithms themselves can be influenced by IPC. When it comes to distributing tasks among processes, IPC mechanisms can optimize workloads, ensuring that data flows efficiently where it's needed most. This creates a dynamic where processes not only communicate but also learn from each other, adapting their methods based on real-time feedback and data.
For instance, consider a scenario where an application utilizes IPC to distribute training tasks among multiple machines. The result? More accurate models emerging faster than ever. The interplay of machine learning with IPC enhances both performance and effectiveness, leading to breakthroughs in processing power.
"The future of IPC will not just be about communication but mastering the art of real-time data negotiation and collective intelligence."
As we continue to peel back the layers of this evolving field, it becomes clear that the future of IPC will be shaped by an intricate dance of technology and innovation. Adapting to these changes is not just advantageous, but perhaps necessary for those seeking to remain at the forefront of computing.