Java 17 Tutorial: Mastering Modern Java Development
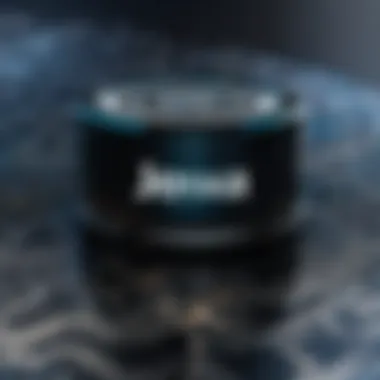
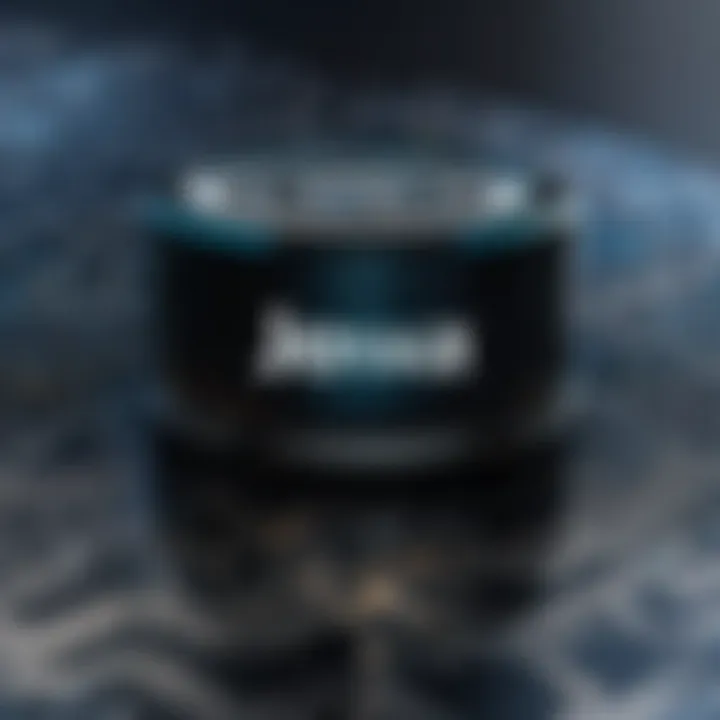
Overview of Topic
In the world of software development, staying current with the latest technology is essential. Java 17 brings numerous advancements that streamline coding efforts and improve application performance. As a long-standing language in the programming community, understanding these changes is crucial for both beginners and experienced developers.
Java has evolved significantly since its inception in the mid-1990s. From the introduction of the object-oriented paradigm to the more recent enhancements in functional programming, each version has contributed uniquely to the language's growth. Java 17, being a Long-Term Support (LTS) release, plays a vital role in this evolution.
The significance of Java 17 cannot be overstated. With new features like pattern matching and sealed classes, it addresses many of the limitations seen in previous versions, making it an attractive option for modern application development.
Fundamentals Explained
To effectively utilize Java 17, developers must grasp key principles that define the language. The core of Java is its object-oriented foundation, which encourages modular code design and reusability.
Key Terminology:
- Java Development Kit (JDK): The software development environment for building Java applications.
- Java Runtime Environment (JRE): A part of the JDK that allows applications to run on a machine.
- Java Virtual Machine (JVM): The engine that implements the Java bytecode and allows Java programs to run.
Understanding these terms will lay a solid groundwork for deeper engagement with Java 17’s features.
Basic Concepts:
- Classes and Objects: The fundamental building blocks of Java, representing real-world entities.
- Inheritance: A mechanism allowing one class to inherit the fields and methods of another.
- Polymorphism: The ability to present the same interface for different underlying forms.
Practical Applications and Examples
Hands-on experience with Java 17’s capabilities can solidify comprehension. Numerous projects and case studies exist showcasing its practical applications. For instance, a banking application can benefit from pattern matching to simplify data verification processes.
Code snippets can also demonstrate the unique features of Java 17. For example:
This snippet illustrates how pattern matching enables clearer and more concise code.
Advanced Topics and Latest Trends
Keeping abreast of advanced topics in Java 17 is imperative for developers looking to harness its full potential. Innovations such as the new Foreign Function Interface (FFI) allow Java applications to interact seamlessly with native libraries, promoting efficiency.
Looking ahead, the Java community is increasingly focused on integrating cloud-native capabilities within applications. This trend is poised to shape the future of Java development.
Tips and Resources for Further Learning
To deepen your understanding of Java 17, consider exploring the following resources:
- Books:
- Online Courses:
- Effective Java by Joshua Bloch
- Java: The Complete Reference by Herbert Schildt
- Coursera: Java Programming and Software Engineering Fundamentals
- Udemy: Mastering Java SE 17
Additionally, visiting platforms such as Wikipedia) or Reddit can provide community insights and discussions on Java.
Java 17 holds significant importance for developers. Familiarizing yourself with its features, applications, and advancements allows for smarter and more efficient coding practices. Don't underestimate the value of ongoing learning and practice in mastering this powerful programming language.
Prelims to Java
Java 17 represents a significant milestone in the Java programming language's long and storied history. Understanding its introduction is essential for developers seeking to harness the full potential of this robust and versatile language. By outlining its core attributes and improvements, this section emphasizes why Java 17 is being adopted widely across various sectors.
The advancements in Java 17 are not merely incremental. They reflect a deeper evolution of the language, addressing both modern programming demands and community feedback. This version offers new features and enhancements that streamline development processes, enhance productivity, and support sophisticated software engineering practices.
Overview of Java's Evolution
Java’s journey began in the mid-1990s. Initially, it was aimed at providing an accessible language for web app development. Over the decades, several versions have added significant features. Each release has brought enhancements in capabilities, security, and performance, allowing it to adapt to the exigencies of modern programming.
Some key milestones include:
- Java 1.0: The inaugural release set the foundation for cross-platform applications.
- Java 5 (2004): Introduced generics, annotations, and the enhanced for-loop, vastly improving code quality.
- Java 8 (2014): Enabled functional programming features through lambda expressions and streams.
These advancements illustrate Java's responsiveness to evolving coding practices. Java 17 continues this trend, addressing existing frustrations and inconsistencies in its predecessors.
Significance of Java Release
The release of Java 17 holds remarkable significance for developers. It marks a Long-Term Support (LTS) version, meaning it will receive updates and support for several years. LTS versions allow organizations to adopt new Java iterations with confidence, eliminating the frequent update burdens that non-LTS versions can incur.
Some of the new highlights include features such as sealed classes which offer a more predictable class hierarchy, pattern matching for switch expressions, and enhanced random number generation. Such features do not only modernize the language but also simplify complex tasks, leading to cleaner and more maintainable code.
"Java 17 is not just an update; it is a commitment to future-proofing the language and ensuring its relevance in software development."
The significance of adopting Java 17 extends beyond individual feature updates. It represents a collective understanding of the community's needs and expectations. For both novice and experienced developers, mastering Java 17 is not just beneficial; it is essential for remaining competitive in a technology landscape that demands agility and innovation.
Setting Up the Development Environment
Setting up the development environment is a critical first step for any Java developer, whether a beginner or a veteran in the programming landscape. The proper configuration of this environment allows for an efficient workflow, facilitating task execution without unnecessary hindrances. Not only does it streamline the coding process, but it also minimizes errors during development. Furthermore, a well-structured environment aids in debugging and testing, ultimately enhancing the quality of the final product. With Java 17, this setup is even more crucial due to the new features and enhancements that require familiarization before diving into application development.
Installing Java
To get started with Java 17, the installation process is the first order of business. This step involves downloading the Java Development Kit (JDK) from Oracle’s official website or an open-source alternative, such as OpenJDK. The installation consists of several straightforward steps:
- Visit the JDK Download Page: Navigate to the official Oracle JDK page or the OpenJDK site to get the latest Java 17 version.
- Choose Your Platform: Depending on your operating system — Windows, macOS, or Linux — select the appropriate package for your setup.
- Download the Installer: Download the executable file for your selected platform. Make sure to verify the checksum to ensure the file is not corrupted.
- Run the Installer: Execute the downloaded file and proceed through the installation wizard, ensuring to set the path variable correctly during the installation process.
- Verify Installation: Finally, use the command prompt or terminal to run to check if Java 17 is installed correctly.
Completing this step successfully sets the groundwork for any development tasks ahead.
Configuring Your IDE
After installing Java 17, the next phase is configuring your Integrated Development Environment (IDE). The choice of IDE can impact both productivity and ease of use. Popular options include IntelliJ IDEA, Eclipse, and NetBeans. Here are the steps to configure your IDE:
- Install the IDE: Download and install your chosen IDE from its official website. Each IDE usually offers a community version, which is suitable for most java development tasks.
- Set JDK Path: Open your IDE settings and point it to the Java 17 JDK you installed. This configuration allows the IDE to utilize the latest features of Java.
- Import or Create a Project: Start a new project or import an existing one, ensuring that the project structure reflects the Java 17 settings. Use the project wizard to define the SDK version.
- Set Up Build Tools: If you plan to use Maven or Gradle, ensure these tools are configured correctly in your project.
A properly configured IDE helps in writing and managing code effectively, enhancing your development experience.
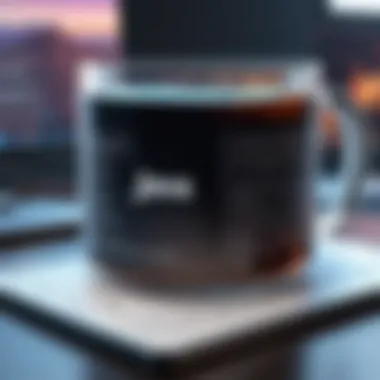
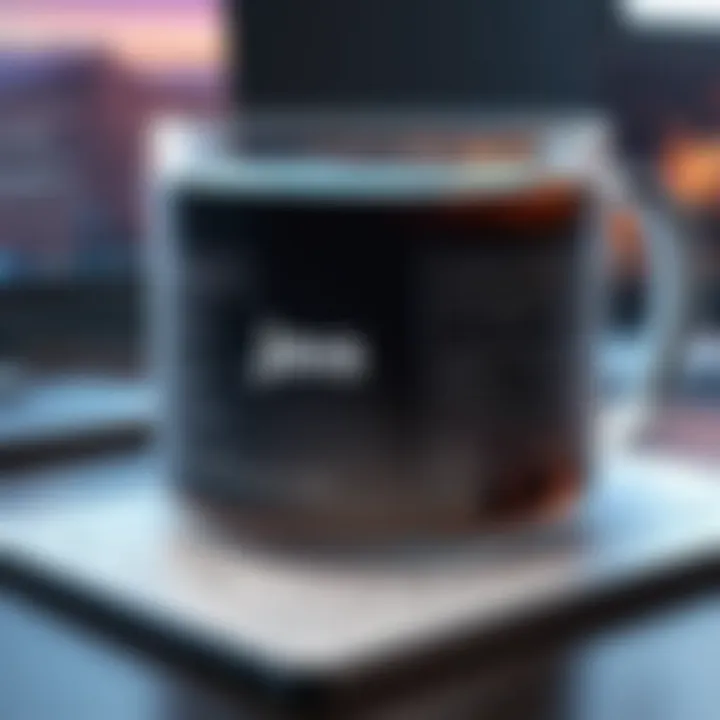
Running Your First Java Program
With the development environment set up and the IDE configured, you can now run your first Java 17 program. This simple step solidifies your foundation in Java programming. Here’s how to do it:
- Create a New File: In your IDE, create a new Java file named .
- Write Your Program: Enter the following code snippet into the file:
- Compile Your Program: Use the build functionality of your IDE to compile the code. It should produce a file without errors.
- Run Your Program: Execute your program using the run command in your IDE. You should see the output:
This initial program illustrates the basic structure of Java applications and confirms that your setup is operational. Each of these steps will help lay a solid groundwork for exploring the advanced features and capabilities of Java 17 in your projects.
New Features in Java
The introduction of new features in Java 17 marks a significant milestone in the journey of the Java programming language. Each feature not only enhances the capabilities of the language but also streamlines development processes. Understanding these features is crucial for both new and seasoned developers as they encourage more efficient coding practices and enable modern software design approaches. The following sections delve into specific new features that contribute to the robust functionality of Java 17.
Sealed Classes
Sealed classes are a notable addition in Java 17, allowing developers to control which classes can inherit from a given superclass. This feature introduces a clear hierarchy and enhances type safety, addressing the frequently encountered issues of unauthorized inheritance. By defining a sealed class, a developer can specify a closed set of subclasses. This allows for better maintainability and predictability in code.
Benefits of Sealed Classes:
- Enhanced Security: Limitations on class inheritance reduce the risk of method overriding and potential security vulnerabilities.
- Clearer Design: By clearly defining subclasses, code readability improves, making it easier for maintainers to understand class relationships.
- Better API Design: APIs can better communicate their intent and usage patterns, fostering a more structured approach to design.
Pattern Matching for Switch
Pattern matching for switch is another significant enhancement introduced in Java 17. It streamlines the process of writing switch expressions by allowing patterns to be used as case labels. This leads to clearer and more concise code, especially in cases involving complex object types and conditions. The feature aims to reduce boilerplate code and minimizes the potential for errors.
Key Advantages:
- Conciseness: Code becomes shorter and easier to understand, eliminating the need for multiple if-else statements.
- Type Safety: It can match the type of an object directly, which reduces the risk of ClassCastExceptions during runtime.
- Flexibility: With this feature, developers can more easily handle multiple types in a single switch statement.
Enhanced Random Number Generation
Java 17 brings enhancements to random number generation with a new API that offers a more flexible and efficient way to generate random numbers. This allows developers to access a wider variety of algorithms for random number generation, accommodating different needs ranging from gaming applications to scientific computations.
Benefits of Enhanced Random Number Generation:
- Improved Performance: New algorithms provide better performance than previous implementations, which is essential in performance-sensitive applications.
- Varied Randomness: Supports multiple types of algorithms to meet different randomness requirements, enabling better application design.
- Ease of Use: The new API is designed to be user-friendly, making it easier for developers to integrate random number generation in their applications.
Language Improvements
Language improvements are a critical focus in Java 17. They enhance code readability and efficiency, making the language more user-friendly and adaptable to modern programming needs. Each update aims to reduce boilerplate code, while increasing expressiveness and versatility. These changes are especially significant for developers who often work with complex systems or manage large codebases.
Text Blocks Enhancements
Text blocks are a feature introduced to simplify the creation of multi-line string literals. In Java 17, this concept is further refined. Developers can now express strings over multiple lines without the need for cumbersome concatenation or escape sequences. The main benefits include:
- Improved Readability: Code becomes cleaner and easier to understand, especially when integrating large blocks of text such as JSON or SQL.
- Ease of Maintenance: Text blocks resist errors that come with frequent manipulation, as the structure remains intact.
For instance, consider the previous approach:
With text blocks, it is simplified to:
This change eliminates the needed escape characters and aligns the text visually, making it clearer. Taking advantage of text blocks can improve the flow of the code, particularly when dealing with structured data.
Improved Type Inference
Improved type inference is another key element introduced in Java 17. This follows the pattern of simplifying the coding process, where developers can write less whilst maintaining type safety. The enhancements allow the compiler to better deduce the types in various contexts, which can lead to fewer explicit type definitions in the code.
Here are some reasons why improved type inference is beneficial:
- Reduced Boilerplate: Developers can spend less time specifying types, leading to cleaner and more concise code.
- Increased Flexibility: Allows for dynamic coding patterns, where the exact type might be ambiguous but still safe.
For example, in earlier versions, it was common to see code like:
In Java 17, it can be simplified to:
This enhancement not only enhances clarity but also aids in maintaining focus on solving problems rather than managing type declarations.
"Java 17’s language improvements ultimately adhere to a philosophy of simplicity and expressiveness, making programming more accessible."
Overall, these language improvements increase productivity while ensuring that Java remains relevant in the rapidly evolving field of software development.
Performance Enhancements
Performance enhancements are crucial in any programming language, especially in a widely-used language like Java. Java 17 introduces several improvements that optimize performance and efficiency, making applications more responsive and less resource-intensive. These enhancements reflect the ongoing commitment of the Java community to provide developers with tools and features that elevate application performance while ensuring stability.
Garbage Collection Improvements
Garbage collection (GC) plays a vital role in Java's performance. It automates the memory management process by reclaiming memory occupied by objects that are no longer needed. The garbage collection improvements in Java 17 focus on efficiency and responsiveness.
Java now has a more advanced garbage collector known as ZGC (Z Garbage Collector), which reduces the pause times significantly. With this improvement, large applications can execute without noticeable downtime. In addition, the improvements in G1 (Garbage-First) GC ensure better handling of memory fragmentation, which can be a performance bottleneck in high-throughput systems.
Some benefits of these enhancements include:
- Reduced Pause Times: Applications can run smoother with less interruption.
- Enhanced Throughput: More tasks can be completed in a given timeframe.
- Better Memory Utilization: Improved algorithms optimize how memory is allocated and freed.
This is especially relevant in an era where performance is often a competitive advantage in software applications.
JVM Performance Tuning
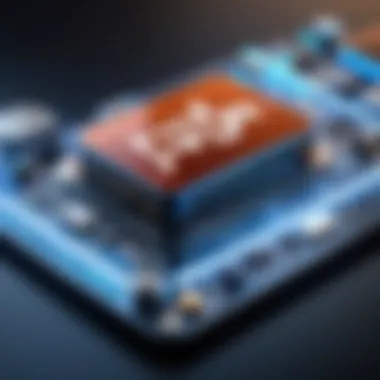
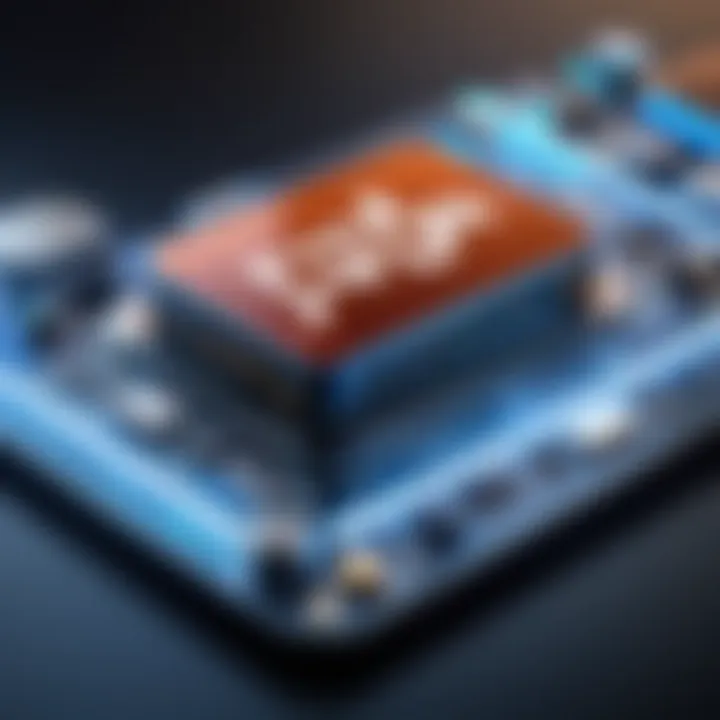
The performance tuning capabilities of the Java Virtual Machine (JVM) have seen notable advancements in Java 17. Developers can now make more informed decisions about memory allocation and garbage collection, directly influencing application performance.
Two primary aspects of JVM performance tuning include:
- Heap Size Configuration: Adjusting the heap size can lead to better performance, especially in memory-intensive applications. By setting the appropriate -Xms (initial heap size) and -Xmx (maximum heap size) flags, developers can tailor memory usage to their specific needs.
- Garbage Collector Selection: Choosing the right garbage collector for the application workload can result in significant performance improvements. Each garbage collector has its strengths, and knowing which to choose based on the application's characteristics is key. For instance, if low latency is critical, ZGC may be the right choice.
Here’s a quick brief on JVM tuning commands:
In this example, you set the initial heap size to 512MB and the maximum to 4GB, while using the ZGC, which is optimized for performance.
"Performance enhancements in Java 17 reflect its adaptability to modern application needs, ensuring smoother execution and effective resource management."
To summarize, the performance enhancements in Java 17, particularly related to garbage collection and JVM tuning, are essential for developers working to build efficient applications. Understanding and leveraging these features can lead to substantial improvements, emphasizing Java's relevance in today's competitive software landscape.
The Java Platform Module System
The Java Platform Module System (JPMS) represents a significant advancement in Java's architecture, introduced with Java 9 and further refined in subsequent versions, including Java 17. This system facilitates better organization of code, enhances dependency management, and promotes encapsulation. With JPMS, developers can structure their applications into individual modules, each with its own set of functionalities and dependencies. This not only simplifies the development process but also makes the applications more secure and maintainable over time.
One of the primary benefits of JPMS is its ability to manage large codebases more efficiently. It encourages developers to think in terms of modules rather than just a collection of classes. Modules can specify which packages they expose for public use, promoting encapsulation and hiding internal implementation details. This leads to cleaner APIs and reduces the likelihood of unintended interactions between different parts of an application.
Another vital aspect of the module system is the increased control over dependencies. Modules can declare their required dependencies explicitly, making it clear what each module needs to function correctly. This feature assists in avoiding the common pitfalls of classpath issues, especially in large projects with many dependencies.
"JPMS encourages a modern approach to application design, focusing on modular code organization and enhancing maintainability."
Understanding Modules in Java
In Java 17, understanding modules is essential for utilizing the full potential of the Java Platform Module System. A module in Java is essentially a named, self-describing collection of related packages and resources. It must contain a file that specifies the module's name, its dependencies, and the packages it exports. This configuration allows for precise control over what parts of a module are visible to other modules, promoting strong encapsulation.
Modules are defined as:
- A Collection of Packages: Each module can contain one or many packages that are logically related.
- Visibility Control: By specifying exported packages in the , developers can control what is visible outside the module.
- Dependencies Management: A module can declare which other modules it depends on, which is essential for resolving dependencies at compile-time and runtime.
Implementing modules requires a clear understanding of how they interact with each other. For example, if a module depends on another, it needs to import it explicitly. This act not only provides clarity but also helps in optimizing the application by ensuring that only the necessary modules are loaded.
Creating Your Own Modules
Creating your own modules in Java 17 starts with defining the module structure. This begins with a file, which must be placed in the root directory of the module. Here is a simple step-by-step guide for creating a module:
- Create the Module Directory: Organize your code files in a dedicated folder named after your module.
- Add the Module Descriptor: Inside this folder, create a file named .
- Define the Module: Use the following structure to define your module:
- Compile the Module: Use the tool to compile your module. Make sure to include the necessary classpath options.
- Run the Module: Finally, use the command to run your module.
Creating modules aligns your applications with modern Java developments. It leads to better-designed applications, reduces complexity, and enhances code reusability.
Working with APIs
APIs, or Application Programming Interfaces, are fundamental in modern software development. They allow different software programs to communicate and share data in a coherent manner. Understanding how to work with APIs in Java 17 is crucial because it enhances the developer's ability to integrate various functionalities and services into their applications.
APIs simplify complex processes, allowing developers to focus on their applications without worrying about the intricacies of the third-party service. Java 17 comes with enhanced API features that support developers in building robust and scalable applications.
New API Features in Java
Java 17 introduced several new API features that streamline development. One significant addition is the updates to the Stream API. This allows for more concise data processing and transformation. Another important feature is the HttpClient API improvements, which make it easier to manage HTTP operations and asynchronous programming.
These updates also include better JSON handling capabilities alongside new classes for representing lexical, severity, and type information in code, which makes working with external data even more efficient. Developers can take advantage of these improvements to create applications that are not only faster but easier to maintain.
Key Features of Java 17 API Enhancements:
- Stream API Enhancements: Improved functionalities that facilitate easier manipulation of collections.
- Improved HttpClient: Streamlined methods for handling HTTP requests, making APIs easier to use.
- JSON Processing: More flexible classes that support various JSON formats and operations.
- Native enhancements: Better file handling, including items such as FileSystem API.
Using the New FileSystem API
The new FileSystem API introduced in Java 17 allows for greater ease and flexibility in handling files. This API enables developers to interact with file systems more intuitively. It abstracts the underlying file system details and provides a more user-friendly interface.
With the updated FileSystem API, developers can manipulate files, directories, and their attributes more effectively. This is particularly useful for applications that require frequent file I/O operations.
Here are some functionalities offered by the new FileSystem API:
- File Access: Simplified methods for reading from and writing to files in various formats.
- File Attributes Management: Easier ways to manage and modify file attributes.
- Cross-Platform Compatibility: Ensures consistency across different operating systems.
Integrating the new FileSystem API will definitely improve the developer's workflow when working with islesystems. While employing these enhancements, the development process becomes smoother.
APIs are the backbone of today's applications, enabling connectivity and enhanced functionalities.
Java Development Best Practices
Developing robust Java applications requires more than just understanding syntax and features; it necessitates a structured approach to coding. Java Development Best Practices are critical for ensuring that code is not only functional but also maintainable, scalable, and efficient. This section will delve into the significance of adhering to best practices, focusing on various elements like code organization and error handling strategies. These practices contribute to a smoother development process and enhance the overall quality of the software.
Code Organization
Effective code organization is a cornerstone of professional Java development. Ensuring that code is arranged logically and intuitively helps developers navigate the project without confusion. Key principles to consider when organizing code include:
- Package Structure: Create a clear hierarchy of packages that reflect the application's architecture. Group related classes and interfaces, which improves discoverability. For instance, placing all your models within a package, and your service classes in a package makes maintenance easier.
- Class Naming Conventions: Use descriptive and clear names for classes and methods. Following Java naming conventions aids in readability. For example, instead of naming a class , a more informative name like provides immediate context.
- Modularity: Break down functionality into smaller, reusable modules. Implementing modules allows for easier testing and debugging, which is essential during development.
"Proper code organization can dramatically reduce technical debt, making future changes simpler and less error-prone."
Error Handling Strategies
Error handling in Java is as crucial as writing the correct implementation of features. Mistakes can occur at any part of the application flow. Thus, employing sound error handling strategies is vital to safeguard against unexpected behavior. Here are some effective strategies:
- Use of Exceptions: Java provides a robust exception handling mechanism. It is essential to differentiate between checked and unchecked exceptions. Checked exceptions like must be declared or handled, while unchecked exceptions like do not have this requirement. Carefully select the type of exception based on the situation.
- Try-Catch Blocks: Wrapping potential error-prone code blocks in try-catch statements helps manage exceptions efficiently. Where necessary, catch specific exceptions instead of a generic one to handle specific conditions accurately.
- Logging: Implement logging to track errors. Using libraries like SLF4J alongside Logback can provide insights into application flow and issues. Allowing developers to debug in hindsight is a crucial aspect of long-term project maintainability.
In summary, following Java Development Best Practices enhances the quality of the codebase significantly. Code organization streamlines work processes, and robust error handling strategies fortify applications against anomalies. By adhering to these practices, developers can ensure that their Java programming efforts are both effective and sustainable.
Building and Managing Java Projects
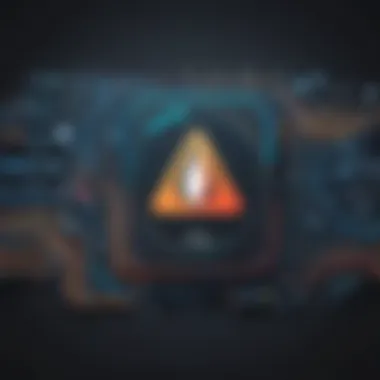
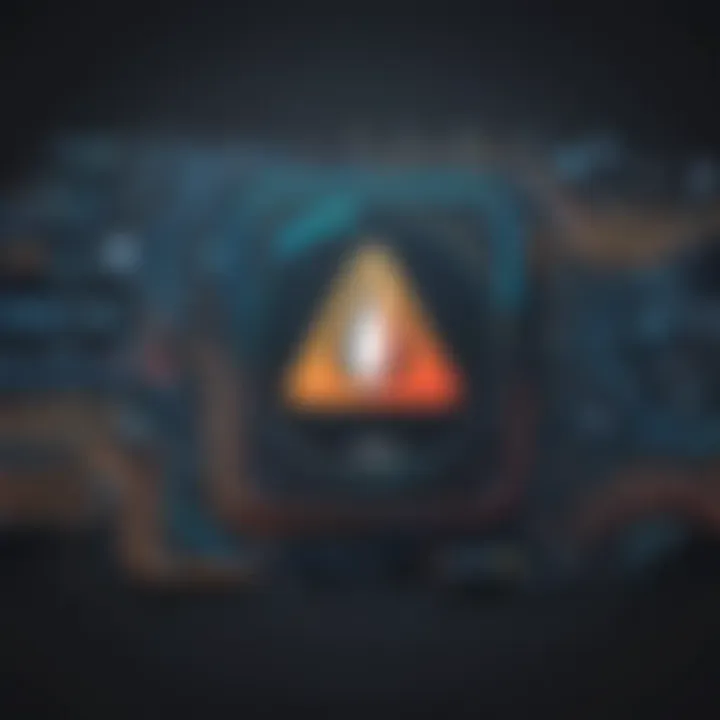
Building and managing Java projects is fundamental in ensuring efficiency and maintainability in modern development environments. Java 17 introduces several features that enhance project management tasks, providing tools that streamline the building process, dependency management, and overall project organization. This section will explore key elements of building and managing Java projects, focusing on specific considerations that every developer should keep in mind.
One of the major benefits of organized project management is the ability to handle dependencies and version control more effectively. A well-structured project allows developers to avoid conflicts that arise from library dependencies. This is essential as projects grow and require multiple libraries or frameworks.
Using Maven with Java
Maven is a project management tool that simplifies the build process and dependency management in Java applications. Utilizing Maven with Java 17 can significantly enhance productivity. Maven's powerful dependency management system allows developers to specify the libraries they need in a simple configuration file, typically called the .
By using Maven, Java developers can easily:
- Manage project dependencies without manual configuration.
- Build, package, and deploy applications with a single command.
- Integrate testing frameworks and continuous integration systems effortlessly.
Maven’s conventions regarding the project structure mean that developers can focus more on coding rather than keeping track of complex configurations. Example configuration in for Java 17 integration would look like this:
This approach saves time and minimizes errors associated with outdated manual configurations, which can become a significant overhead in large projects.
Gradle and its Advantages
Gradle is another popular build automation tool that has gained traction among Java developers. Its emphasis on flexibility and performance makes it a top choice for modern Java projects. Gradle utilizes a domain-specific language based on Groovy or Kotlin, providing a more expressive way to configure projects compared to XML-based tools like Maven.
Key advantages of using Gradle include:
- Incremental Builds: Gradle understands what parts of the project need to be rebuilt, saving time for developers by avoiding unnecessary compilation.
- Rich Ecosystem: With support for a variety of languages and platforms, Gradle can be integrated with almost any other system or library.
- Customizability: Developers can easily create tasks that fit their precise requirements, resulting in a tailored build process.
Using Gradle can help in managing dependencies effectively through a file:
With its modern approach, Gradle offers alternatives that can suit different project needs depending on complexity or developer preferences. Choosing the right tool for managing Java projects is crucial for a smooth development experience.
Testing in Java
Testing is a crucial component of software development, especially in a language as widely used as Java. In this context, Java 17 brings notable advancements that simplify testing and improve developer efficiency. The emphasis on testing goes beyond just ensuring code quality; it is about increasing confidence in the stability and functionality of applications. As the complexity of modern applications grows, robust testing strategies become imperative.
The integration of new testing frameworks, better libraries, and improved support for existing tools in Java 17 highlights the significant role that testing plays in contemporary development. Developers are incentivized to adopt systematic approaches for testing, which can save time and reduce errors during deployment. With Java 17, there is a reinforcement of best practices that can enhance the quality of projects.
JUnit Features
JUnit 5 emerges as a foundational framework for testing in Java 17. This version introduces a modular architecture that supports a range of testing paradigms. Developers can now leverage several features designed to streamline testing processes:
- JUnit Jupiter: This is the programming model that comes with JUnit 5. It allows for expressive and customizable tests.
- Dynamic Tests: A significant feature where tests can be defined at runtime, providing flexibility to test scenarios that are only known during execution.
- New Annotations: Several new annotations like @Nested and @Tag have been introduced, enhancing test organization and categorization.
These features not only promote cleaner code but also allow for better organization of tests. The modularity permits integration with various other testing libraries more easily, making JUnit 5 a go-to choice for Java developers.
Mocking Frameworks
Mocking frameworks have become pivotal in unit testing, allowing developers to simulate the behavior of complex objects. In Java 17, frameworks like Mockito and WireMock continue to gain traction.
- Mockito: This framework is simple yet powerful for creating mock objects in tests. It enables developers to define how mocked interactions should behave, facilitating isolated unit testing without relying on external components.
- WireMock: This tool provides a mocking server that simulates HTTP-based APIs. It is particularly useful for testing web services and microservices in Java applications.
Using these frameworks, developers gain the ability to focus on testing specific parts of their code without the influence of other modules. This isolation is critical for identifying issues early in the development lifecycle.
Deploying Java Applications
Deploying Java applications is a critical aspect of modern software development. Once the development phase is completed, it is essential to ensure that the application operates efficiently in a production environment. Java 17 introduces several enhancements that streamline this process. Understanding deployment methods enhances the effectiveness of Java applications, allowing for scalability, improved performance, and easier maintenance.
Containerization with Docker
Containerization has revolutionized how developers deploy applications. With Docker, developers can create lightweight, portable containers that package applications along with their dependencies. This ensures consistency across different environments.
Using Docker for deploying Java applications offers many benefits:
- Isolation: Each container is isolated from others, reducing potential conflicts with libraries or runtime environments.
- Portability: Applications can be easily moved across various platforms, whether on-premises or in the cloud.
- Scalability: Containers can be quickly scaled up or down based on the demand, optimizing resource usage.
- Simplified CI/CD: Docker integrates seamlessly with continuous integration and continuous deployment tools, streamlining the deployment pipeline.
To deploy a Java application using Docker, follow these steps:
- Create a Dockerfile: This file defines how the application will be built. An example Dockerfile for a Java application might look like this:
- Build the Docker image: This is done using the command:
- Run the container: Finally, run the container with:
This process encapsulates the Java application in a robust, reliable environment.
Cloud Deployment Options
Deploying Java applications in the cloud has gained prominence as more organizations move towards cloud infrastructures. Cloud platforms such as Amazon Web Services, Microsoft Azure, and Google Cloud Platform provide a range of services tailored for Java deployment.
Cloud deployment offers several advantages:
- Flexibility: Choose from various services according to your project’s needs, such as serverless functions or managed container services.
- Global Reach: Applications can serve users across the globe with minimal latency.
- Cost-Effectiveness: Pay-as-you-go models allow for efficient resource management, which can significantly reduce operational costs.
- Automatic Scaling: Many cloud services automate the scaling of applications based on real-time demand, enhancing user experience.
When considering cloud deployment for Java applications, here are some options:
- Amazon Elastic Beanstalk: An easy-to-use service for deploying applications that automatically handles the infrastructure details.
- Google Cloud Run: A fully managed compute platform that automatically scales your containerized applications.
- Microsoft Azure App Service: A platform that allows for deploying Java web apps quickly while managing resources effectively.
Closure
In this article, we explored the significance of Java 17 in modern software development. Understanding the conclusion is essential as it distills the wealth of information discussed throughout the tutorial. This section serves to reinforce the key concepts and takeaways relevant to Java 17 and its practical applications. A proper summary synthesizes ideas, enabling readers to correlate the features with real-world programming tasks.
Recap of Key Concepts
Java 17 introduces several notable features that enhance developer productivity and application performance. Some of the key concepts to remember include:
- Sealed Classes: These provide a way to restrict which classes can extend or implement a certain class or interface. This is crucial for designing secure APIs.
- Pattern Matching for Switch: This feature streamlines the syntax and logic of switch statements, making code cleaner and more intuitive.
- Enhanced Random Number Generation: A new suite of random number generators that offers better performance and a more straightforward API has been added.
- Text Block Improvements: The enhancements in text blocks simplify multi-line string handling, improving readability and maintainability.
- Garbage Collection Improvements: Advanced garbage collection options enable better memory management, especially for applications with high throughput demands.
Future of Java Development
The future of Java development appears promising. As technology evolves, so too does the need for languages that are efficient, secure, and adaptable. Java 17 positions itself well within this framework because:
- Continual Updates: The release showcases Java's commitment to innovation and augmentation of its capabilities while remaining backward compatible for most applications.
- Adoption in Emerging Technologies: With the growing interest in cloud computing and microservices, Java’s adaptable ecosystem is set to thrive in these domains.
- Support for Modern Programming Paradigms: Future updates will likely include enhancements in functional programming, reactive programming, and better integration with modern development frameworks.