Java Database Connectivity: Download and Implementation Guide
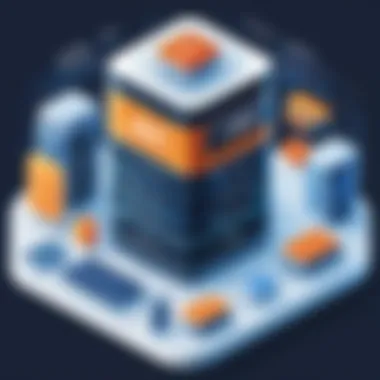
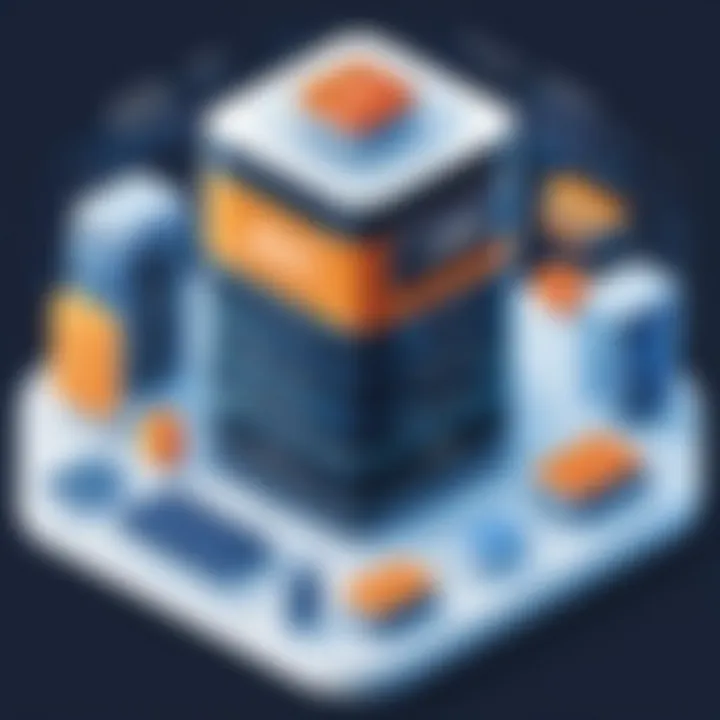
Overview of Topic
Preface to the Main Concept Covered
Java Database Connectivity (JDBC) serves as the cornerstone for efficiently connecting Java applications to relational databases. It acts as an interface, facilitating communication between Java apps and a diverse range of database management systems. Without JDBC, integrating Java applications with databases would be significantly more challenging. This article aims to provide an essential guide for anyone looking to harness JDBC for their development needs.
Scope and Significance in the Tech Industry
In today's digital landscape, database management is a critical skill for Java developers. With an increasing reliance on data-driven applications, the ability to correctly manage and interact with databases is paramount. JDBC stands out in enhancing the efficiency of this process.
Brief History and Evolution
JDBC was introduced in the mid-1990s, coinciding with the rising popularity of Java. Over the years, the specification has evolved, adapting to technological advancements and expanded requirements. It is now a fundamental part of Java's extensive ecosystem, allowing developers to use SQL commands seamlessly.
Fundamentals Explained
Core Principles and Theories Related to the Topic
At its core, JDBC simplifies the process of executing SQL statements, retrieving results, and managing connections. Understanding how JDBC manages these aspects is essential for database-centric Java applications.
Key Terminology and Definitions
- Connection: A session established with a database through which queries are executed.
- Statement: An object representing a SQL statement to be executed.
- ResultSet: A table of data that results from querying the database.
Basic Concepts and Foundational Knowledge
Absorbing foundational knowledge surrounding the architecture, including DriverManager and Connection interfaces, is vital. JDBC supports various drivers, allocating flexibility that maximizes compatibility with different databases.
Practical Applications and Examples
Real-world Case Studies and Applications
Consider a small web application designed for inventory management. In this scenario, JDBC enables the application to query product details and refresh stock levels by directly interacting with a backend database.
Demonstrations and Hands-on Projects
Implementing JDBC in Java is easier with practical examples. Below is a simple guide to establishing a connection:
Code Snippets and Implementation Guidelines
Code snippets are indispensable for successfully implementing JDBC in applications. Follow issues with prepared statements for boosting security and optimizing performance.
Advanced Topics and Latest Trends
Cutting-edge Developments in the Field
Many organizations are investing in cutting-edge frameworks that incorporate JDBC. Implementations like Spring Data allow elegant interactions with data sources while retaining core JDBC functions.
Advanced Techniques and Methodologies
Mastering connection pooling techniques contributes significantly to resource management, reducing latency and enhancing performance.
Future Prospects and Upcoming Trends
As the tech landscape evolves, expect deeper integrations with cloud services, increasing the demand for advanced JDBC skills among developers.
Tips and Resources for Further Learning
Recommended Books, Courses, and Online Resources
- Java Database Programming: A Beginner's Guide (Book)
- Online courses on platforms like Coursera often cover the essentials as well as advanced concepts of JDBC communication.
Tools and Software for Practical Usage
Utilizing tools like Eclipse or IntelliJ IDEA enhances the overall development process. These environments typically support database connections out of the box.
In summary, mastering JDBC is essential for any developer focusing on Java applications. The evolving tech landscape further highlights its importance.
Prelude to Java Database Connectivity
Java Database Connectivity, or JDBC, serves as a critical framework that allows Java applications to communicate with various relational databases. It provides a standard method for Java programs to execute SQL statements, retrieve response data, and manage errors. JDBC is essential because it unifies database access for Java developers, irrespective of the database used. This section outlines the key elements and benefits associated with JDBC, setting a solid foundation for comprehending its utility in Java programming.
Understanding JDBC
Java Database Connectivity is a Java-based API that facilitates connecting applications to database management systems. It establishes a bridge between the Java programming language and a wide array of database solutions. JDBC defines how a client may access a database and execute queries or updates. The primary functions of JDBC include:
- Connecting to databases: The core feature of JDBC involves establishing a connection to a database instance, enabling SQL commands execution.
- Executing queries: Users can send various SQL statements to the DBMS and receive responses in a standardized format, supporting both queries and data modifications.
- Processing results: After executing SQL commands, JDBC handles the fundaments of managing result sets, summarizing data efficiently, and fetching the required information.
To effectively engage with JDBC, understanding its consistency is vital, especially as databases take on increasingly complex roles in applications.
Importance of JDBC in Java Development
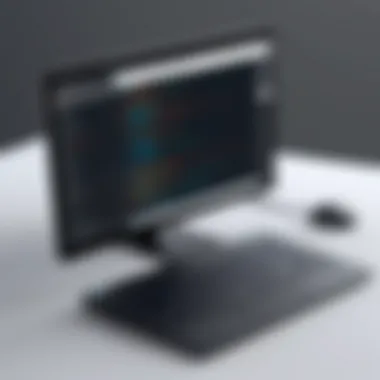
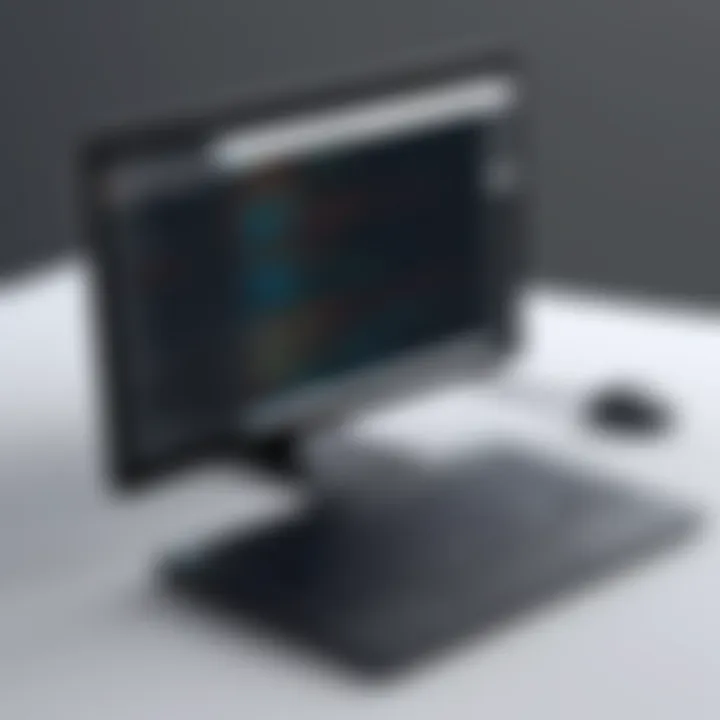
The Role of JDBC in Java Development cannot be overstated. As applications today demand data-driven features and functionalities once only available in standalone systems, JDBC provides a robust framework to fulfill these expectations. Here are several critical aspects that affirm the significance of JDBC in the current landscape:
- Platform Independence: JDBC’s design paradigm allows for operating across different databases consistently through straightforward APIs, simplifying issues of compatibility.
- Interactive Database Management: With JDBC, developers are equipped to create applications that not only read from a database but also perform transactions and manipulate data dynamically based on user input.
- Enhanced Performance Reporting: JDBC enhances the details provided by databases through improved frameworks for logging connection states and queries performance.
> *"Data management in Java applications hinges significantly on JDBC, through ensuring a seamless link with varied databases."
Understanding these principles assists developers in consciously utilizing JDBC as a powerful tool in writing scalable complex applications derived from real-time data interactions. As the use of databases continues to rise, so does the necessity for effective JDBC implementation integrated strategically into Java applications, ensuring efficiency and reliability.
JDBC Download Overview
Understanding the concept of JDBC Download is crucial for software developers and database managers alike. This section delves into the central elements involved in obtaining JDBC drivers, which are essential to establish a link between Java applications and databases. Without the necessary drivers, the ability to execute SQL commands and retrieve data effectively will be hindered. Hence, comprehension of this theme is vital.
Components Required for JDBC
When setting up JDBC, several components need consideration. First are the required JDBC drivers. These can vary based on the type of database the Java application will connect to. For example, different drivers exist for MySQL, Oracle, and PostgreSQL databases. Choosing the correct driver is imperative for compatibility.
Additionally, JDBC libraries come into play. These libraries consist of classes for managing database connections, processing results, and handling SQL procedures. Understanding these components streamlines the implementation process. Here’s a brief overview of these components:
- JDBC Drivers: Interfaces that allow a Java application to communicate with a database.
- JDBC API: A set of classes and interfaces that facilitate database interaction for SQL statements.
- Database Server: The hosting service for the database, providing the necessary infrastructure.
In summary, having knowledge about these components is essential, as it guarantees a smooth installation and implementation procedure in JDBC.
Where to Download JDBC Drivers
Finding the right JDBC drivers is a necessary task for developers initiating a new project or enhancing an existing application. Various online repositories and official websites offer JDBC drivers. One notable source includes the official documentation from the database vendors, such as:
- MySQL Connector/J from MySQL's official site: MySQL Connector
- Oracle JDBC Driver available on Oracle's download page: Oracle JDBC
- PostgreSQL JDBC Driver from the PostgreSQL official site: PostgreSQL JDBC
To successfully ensure compatibility, always download the driver version that corresponds to your database version.
In addition, package management tools, such as Maven and Gradle, also allow for easy downloading and managing of these dependencies. While utilizing mainstream repository systems, one should include a proper artifact with dependency versions set. The benefit of these platforms lies in their ability to manage dependency lifecycle evolution seamlessly.
Using the correct repositories promotes efficiency and prevents potential runtime errors during application execution, especially in diverse system environments.
Types of JDBC Drivers
Understanding the types of JDBC drivers is crucial for developers who interact with databases using Java. Each driver type offers unique features and nuances that can affect performance, ease of use, and compatibility with database management systems. By selecting the correct driver, one can optimize database connections for various applications. The effectiveness of database operations often hinges on this selection because drivers manage how data travels between the Java application and databases.
Type Driver - JDBC-ODBC Bridge Driver
The Type 1 driver allows Java applications to connect to ODBC data sources. This bridge converts JDBC calls to ODBC calls, leveraging the traditional ODBC architecture. Although it offers the benefit of connecting Java applications to any database with an ODBC driver, its reliance on ODBC imposes specific limitations. These include performance overhead and increased compatibility issues across different platforms. Moreover, using this driver is not advisable for production-level applications due to potential reliability concerns. Despite historical relevance, it has largely fallen out of favor in contemporary contexts.
Type Driver - Native API Driver
A Native API driver typically utilizes the client-side library of a specific database. It translates JDBC calls directly into the database-specific calls. As a result, this driver offers improved performance over the Type 1 driver. However, it requires that the client installation even though it enhances connectivity under certain scenarios. For large-scale applications, the requirement of installing native libraries could limit deployment flexibility. Moreover, it remains tied to a specific database, making it less portable compared to other types of JDBC drivers.
Type Driver - Network Protocol Driver
The Type 3 driver communicates with database middleware and then routes requests to the database. This driver type separates itself from others by providing a more flexible and scalable solution. It immediately converts JDBC calls to messages sent across the network to the middleware server. Consequently, this type of driver can connect multiple database types, consolidating the adaptation necessary for varying database architectures. Overall, Type 3 drivers prove to be suitable for applications distributed across central and local management centers, offering adaptability at scale.
Type Driver - Thin Driver
The Type 4 “Thin” driver is a universal JDBC driver that translates JDBC calls directly into the network protocol used by DBMSs (Database Management Systems). Since it does not rely on any client-side library, it is often praised for its simplicity and portability. Application developers find it easier to distribute applications without worrying about driver-specific libraries. This approach leads to faster response times and reduced resource demands. Overall, Type 4 drivers are highly recommended for server-side code where high performance, quick deployment, and ease of configuration prevail.
The right choice of JDBC driver can significantly affect both efficiency and maintainability in database interactions.
Steps to Download JDBC Drivers
Understanding the correct procedures to download JDBC drivers is essential for any developer looking to work with relational databases in Java. These steps function as a foundational block for establishing robust database connections. The right drivers ensure that your Java applications communicate seamlessly with databases, enhancing data management and retrieval processes.
In this section, we focus on specifics such as residing reputable driver websites and leveraging tools like Maven for an efficient download experience.
Locating Official Downloads
The most crucial first step in this process is identifying the official sources for JDBC driver downloads. Many database vendors offer drivers tailored for their systems. For instance, if you are working with MySQL, you can visit the official MySQL website to find the latest Connector/J drivers. These are built specifically to guide Java applications in communicating with MySQL databases effectively.
Key Points to Consider:
- Always download drivers from official vendor sites. This reduces the risk of downloading malware or broken assets.
- Look for the documentation available on these sites. Vendor documentation commonly aids in integrating drivers into your Java projects correctly.
- Ensure the version is compatible with both your Java Development Kit (JDK) version and the database system you are targeting.
Blockquote:
"Choosing the correct JDBC driver can significantly impact the performance and reliability of your database operations. Always prioritize quality sources."
Using Maven for Dependency Management
Maven serves as a powerful tool for various aspects of Java development, including dependency management. With it, developers can automate the handling of libraries such as JDBC drivers within a project.
Getting Started with Maven:
- Add JDBC driver dependencies in your project's file. Here’s an example for including MySQL Connector/J:
By defining this in your Maven configuration, your project ensures that your JDBC driver is always available during build management. Maven offers resilience in development by eliminating the need for manual downloads and updates.
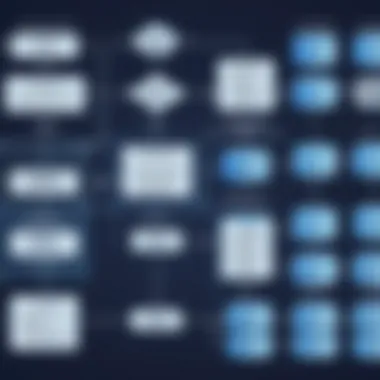
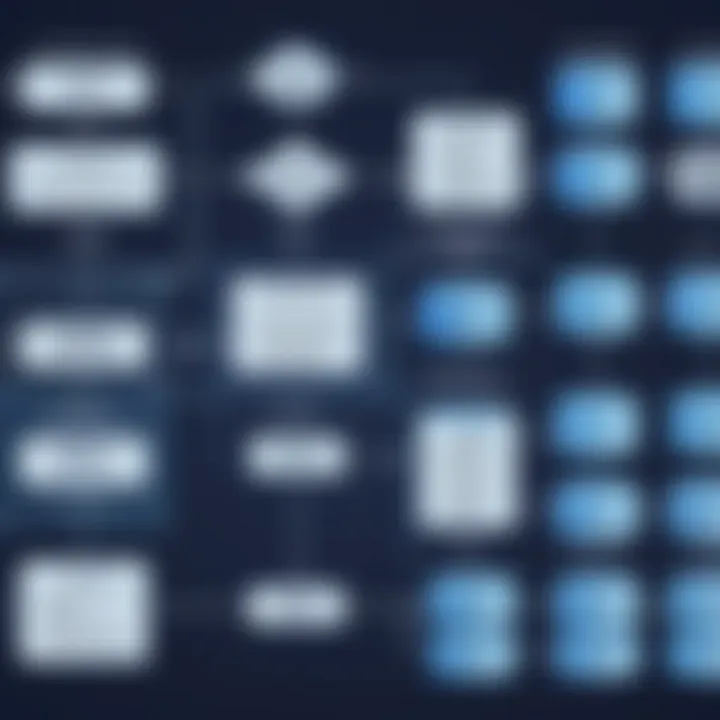
- Using Maven, you can easily change driver versions just by modifying the version number in the XML, streamlining the upgrade process.
Overall, combining official JDBC driver downloads with a robust package manager such as Maven ensures both stability and ease of development. Understanding the sourcing and implementation process adequately prepares developers for effective Java Database Connectivity.
Setting Up JDBC in Your Java Project
Setting up Java Database Connectivity (JDBC) in your Java project is an essential phase in the process of establishing connections between your applications and databases. Understanding this setup enhances overall data handling in applications and enhances performance. The correct incorporation of JDBC helps to streamline database interactions, improving the reliability and efficiency of your application.
Including JDBC Libraries
The first step in setting up JDBC is to include the necessary libraries in your project. Java developers must access the JDBC libraries, which typically come packaged with Java Runtime Environment or can be downloaded as part of a JDBC driver suite.
Using an Integrated Development Environment such as Eclipse or IntelliJ IDEA can simplify this process. If you are using Maven as a build tool, including the libraries requires adding the dependencies to your file. A typical entry might look like:
By integrating these libraries, you allow your project to interact with databases through JDBC. This approach ensures that any required data source has seamless traversal between application code and database operations.
Establishing a Database Connection
The next critical element is establishing a robust database connection. This connection forms the foundation of all database transactions.
To establish a connection, you can use the class provided by JDBC. The key to creating a connection lies in the connection string, which contains details like the database URL, username, and password. A simple connection example can be demonstrated as:
It is important to manage connection states efficiently to ensure that resources are not left hanging and that errors are minimized. Close connections at the conclusion of your operations to maintain proper resource usage.
Setting up JDBC involves directly integrating needed libraries to establish successful connections while ensuring efficiency and reliability throughout the application lifecycle.
In summary, correctly setting up JDBC in your Java project offers clear pathways between your application and databases and sets a strong foundation for reliable database management.
Working with JDBC
Working with JDBC is crucial for Java developers who need to connect their applications with databases. By mastering JDBC, developers gain the capability of performing fundamental database operations like executing queries and managing data efficiently. Moreover, JDBC abstracts the complexities of interacting with the database, making it easier for developers to focus on the application's logic.
Familiarizing oneself with the nuances of JDBC can significantly contribute to strong application performance and reliability. Moreover, understanding the functional aspects of JDBC allows for better error resolution and overall operational assurance.
Executing SQL Statements
Executing SQL statements is one of the primary functionalities provided by JDBC. This process involves submitting SQL commands from a Java application to a database and receiving results for processing. The execution of SQL statements usually breaks down into several steps:
- Creating a Statement Object: This is done using the connection object.
- Sending the SQL Command: Use the or the methods, depending on whether the intention is to retrieve data or affect changes.
- Handling Exceptions: Managing SQL exceptions is essential to ensure that the application does not crash due to unexpected issues.
- Processing the Results: Any data returned through a should be retrieved efficiently to ensure smooth application performance.
Here is an example of how to execute a SQL statement in JDBC:
Such snippets are not only fundamental for the immediate needs of data retrieval but also enhance the people's understanding of how effective database interaction can be executed within Java.
Handling Results Sets
Handling results sets allows developers to capture and manipulate the output returned from executed SQL commands. A object provides methods to navigate through data rows and obtain records of interest. The important aspects of working with ResultSets include:
- Iterating through Results: You can use methods like to traverse the rows of results once the query is executed successfully.
- Retrieving Data Types: JDBC provides a variety of methods like , , and to fetch data in its specific type, respecting database integrity.
- Managing ResultSet States: Understanding the cursors and navigational capabilities of the is vital since it dictates the interaction with the data.
It’s paramount that developers write code that anticipates possible null results or empty data sets, minimizing exceptions that would affect user experience. Matches between expected data types and fetched data ensure reliability as well.
For effective handling, consider organizing a clean retrieval pattern:
- Prepare for potential empty results.
- Use accurate data types when retrieving.
- Ensure proper closure of resources post-retrieval.
By following structured controlling of ResultSets, the robustness of database interaction in Java applications is significantly augmented.
Remember, a well-structured approach to JDBC interaction paves the way for enhanced performance and maintains high application integrity.
Best Practices for JDBC Implementation
Implementing Java Database Connectivity (JDBC) in your applications is not merely a matter of connecting to a database; it encompasses a range of best practices that ensure performance, security, and reliability. Adhering to recommended strategies enhances the efficiency of JDBC usage and mitigates risks that can arise during database interactions. These practices can transform a rudimentary database operation into a scalable and robust process, suitable for modern applications.
Error Handling in JDBC
Effective error handling in JDBC is crucial. It provides developers with control while managing potential issues that may occur when communicating with a database. The nature of communication with a database involves various probable failure points. Thus, capturing and addressing these errors efficiently can save developers significant time and resources.
When implementing error handling, the following strategies should be considered:
- Use try-catch blocks. Wrapping your database code with try-catch statements helps detect JDBC errors and indicates an appropriate response.
- Log detailed error messages. Avoid generic error messages; provide specifics about the error. Detailed logs are invaluable for troubleshooting.
- Use SQLException The SQLException class is fundamental when handling database errors. It enables specific error handling for various database operations. Capture these exceptions to respond effectively.
Implementing these practices propels one toward developing a resilient database layer. For example:
By adopting structured error handling, developers can create applications that gracefully manage exceptions rather than crashing or becoming inoperable.
Closing Connections Properly
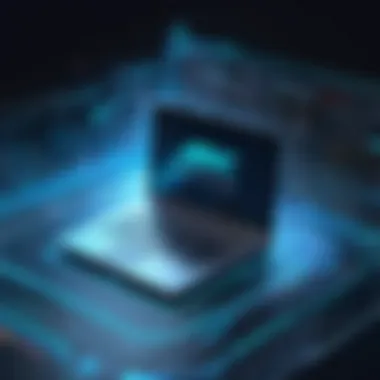
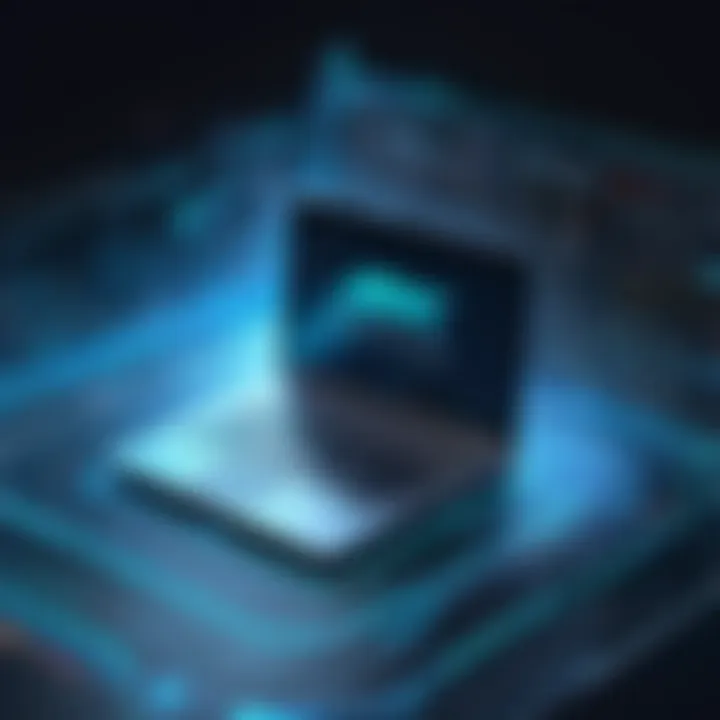
Closing database connections ensures that resources are freed in a timely and orderly manner. Unused connections consuming system resources can lead to slower performance or resource exhaustion. To maintain optimal performance and system reliability, practices regarding connection closure should be routinely acknowledged.
Best efforts should include:
- Always close connections in a finally block. This ensures that connections are terminated even if an exception arises. It solidifies the principle of resource management at all times.
- Utilize connection pooling. Implementing connection pooling not only reduces the overhead of establishing new connections but also helps in effective resource consumption. Libraries such as Apache DBCP or HikariCP can assist significantly here.
- Use the try-with-resources statement where available. This feature, introduced in Java 7, provides a cleaner, more effective way to close connections. Objects declared within the parentheses of try-with-resources are automatically closed after the execution of the try block.
Example using try-with-resources:
In summary, the straightforward work of closing connections is an often overlooked but essential best practice. Fostering these habits will empower developers to produce higher quality and more efficient applications.
Security Considerations with JDBC
When dealing with database connectivity, security rises as a vital aspect that cannot be overlooked. Java Database Connectivity (JDBC) provides a bridge between Java applications and databases, making it crucial to ensure that data exchanged between these platforms remains safe from unauthorized access and breaches. This section discusses core elements related to security in JDBC implementation while underscoring its significance in the overall architecture of applications.
Data Protection and Encryption
Data protection stands as a primary pillar in the realm of JDBC security considerations. JDBC facilitates interaction with databases which store sensitive information, such as personal details and financial data. Ensuring that this data is protected during transit and at rest is crucial.
One efficient way to enhance data protection while using JDBC is by implementing encryption. Encryption algorithms transform readable data into an unreadable format, making it difficult for someone without the proper credentials to access it.
- Some commonly used encryption methods include:
- AES (Advanced Encryption Standard)
- RSA (Rivest–Shamir–Adleman)
- DES (Data Encryption Standard)
While integrating encryption practices, it’s also paramount to keep keys in secure locations, away from exposure during the normal operation of applications. By applying database-level encryption or utilizing SSL/TLS protocols for secure connections, developers can implement an additional layer of safety, ensuring that sensitive data remains protected during transmission.
“Data protection essentially encompasses the methods and practices put in place to safeguard personal and sensitive information.”
This proactive approach towards data security enhances user trust and aligns with compliance frameworks that emphasize the necessity of strong data protection measures.
Access Control and User Permissions
Access control determines who can access and manipulate data within a database system. When implementing JDBC solutions, establishing proper access control mechanisms is essential. Without appropriate access controls, unauthorized entities could manipulate or view sensitive data, leading to data leaks and integrity violations.
Implementing user roles and predefined permissions is vital in JDBC implementations. This allows administrators to control access levels among users based on their specific roles within an organization. Integrity and confidentiality can be assured through these measures. Admins can assign different levels of access, indicating who can execute SQL statements such as INSERT, SELECT, UPDATE, or DELETE.
Some key aspects of user permission management include:
- Role-Based Access Control (RBAC): Assign user roles that encapsulate a set of permissions, ensuring that only individuals with relevant authorization levels can access sensitive data.
- Least Privilege Principle: Provide the minimum access necessary for users to perform their tasks, reducing the risk of accidental or malicious data exposure.
- Auditing: Keep track of user activities through legitimate auditing, which helps in identifying unauthorized attempts at data access.
By applying these access control measures, an organization can maintain the integrity of its data access and safeguard against potential security threats within the JDBC framework.
Troubleshooting Common JDBC Issues
Troubleshooting issues related to Java Database Connectivity (JDBC) is a critical aspect of ensuring seamless database operations within Java applications. Roadblocks can occur during development, deployment, or runtime, and understanding how to address these scenarios can improve efficiency and enhance the development experience. By diagnosing connection problems and SQL syntax errors effectively, developers can maintain robust connections to databases.
Troubleshooting JDBC issues minimizes downtime and fosters maintainable code.
Connection Failures
Connection failures are among the most frequent issues encountered while using JDBC. They can result from various issues including network problems, wrong credentials, or misconfigured properties. Understanding how to identify these issues aids in resolving them more swiftly. Some significant factors to consider include:
- Driver Configuration: Ensure the JDBC driver is set up correctly in your environment. Wrong paths or older driver versions may result in connection errors.
- Connection URL: The format must align with the specifications required by the database. For example, a typical URL for MySQL would look like this:A small typo can lead to a failure.
- Network Availability: Gridlock or configurations in firewalls can inhibit access to the database server. Terminal commands such as ping or telnet can often assess availability.
- Database Server Status: Sometimes the issue stems from the database server itself. If the server is down or restarting, connections will fail until it becomes operational again.
Establish robust methods to log connection attempts and errors. These logs help identify recurring problems which can be resolved with a more systemic approach.
SQL Syntax Errors
SQL syntax errors transpire when queries sent to the database do not adhere to the database's expected language and structure. They're vital to address because reducing SQL errors ensures the application functions correctly, minimizes failures in data handling, and refrains from impacting database integrity. Key points to consider include:
- Error Messages: Pay attention to messages provided by the JDBC driver. They often pinpoint where an error may occur within the SQL.
- Testing Queries: Testing queries directly in a successful database client can help highlight syntax issues before deploying in your Java code.
- Use Keywords Properly: SQL is specific with its wording. For instance, ensuring correct use of SELECT, FROM, JOIN, or ORDER BY is needed, as mistaking these can lead to notable errors.
- Parameter Binding: Employ parameter binding techniques instead of directly inserting variables in queries. Not only does this enhance security against SQL injections, but it also circumvents many common syntax errors by ensuring types match.
By tackling such errors with diligence, developers can leverage efficient debugging methodologies. Gathering regular feedback during the testing phases allows for rapid content refinement and application reliability.
Culmination
The conclusion of this article serves as a critical reflection on the importance of JDBC, emphasizing the key themes discussed throughout. JDBC stands at the nexus of Java programming and database management, ihabilitating seamless interaction between these systems. In a world where data flows incessantly, understanding JDBC becomes not just an advantage, but a requirement for modern developers.
Recap of JDBC Essentials
To summarize the pivotal aspects of JDBC, this section underlines several key points. First, JDBC establishes a standard for connecting Java applications to various databases. It allows for executing SQL statements and managing results in a relational database. By supporting multiple driver types, JDBC provides flexibility depending on user needs.
Second, it embodies principles of robust database handling. By incorporating best practices around error handling and connection management, developers can foster more secure applications. It is imperative that one also keeps in mind aspects such as data protection and authorization when using JDBC.
- Effective usage includes initiating proper connections to avoid leakages of resources.
- Utilize the various driver types that suit your streaming requirements.
- Implementing proper access control ensures safety against SQL injection and other vulnerabilities.
These considerations and practices are paramount for ensuring efficient and secure database transactions.
Future of JDBC in Java Development
Looking ahead, the future of JDBC in Java development is rife with potential for enhancement. The ongoing evolution of both databases and Java itself signifies a digital landscape that will continuously adapt. As technology burgeons, new standards and conventions will likely arise to enhance the functions of JDBC.
Developers may expect more integration with cloud-based services. This incorporates making JDBC driver installations simpler alongside frameworks that automatically alternate drivers based on cloud resources. The push towards microservices architecture could further drive computational efficiency.
In a rapidly changing ecosystem, ensuring JDBC remains relevant hinges on concerted effort toward updates and education. This requires professionals updating their skills to keep up with advancements in JDBC innovations, quickly integrating best practices. Other advancements may address existing limitations, like improvements in load balancing or advancements in JDBC performance through newer drivers.
As a community, the role of JDBC within the wider Java domain cannot be understated. Contin shared engagement and knowledge-sharing will play key roles for its future direction, likely fostering entirely new paradigms in application connections.