Simple Java Programs to Enhance Coding Skills
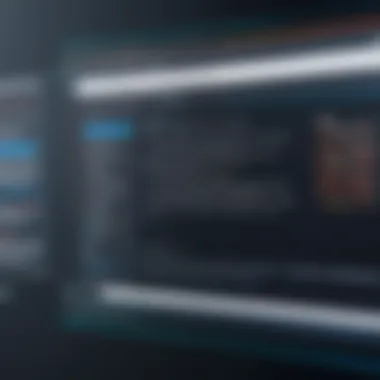
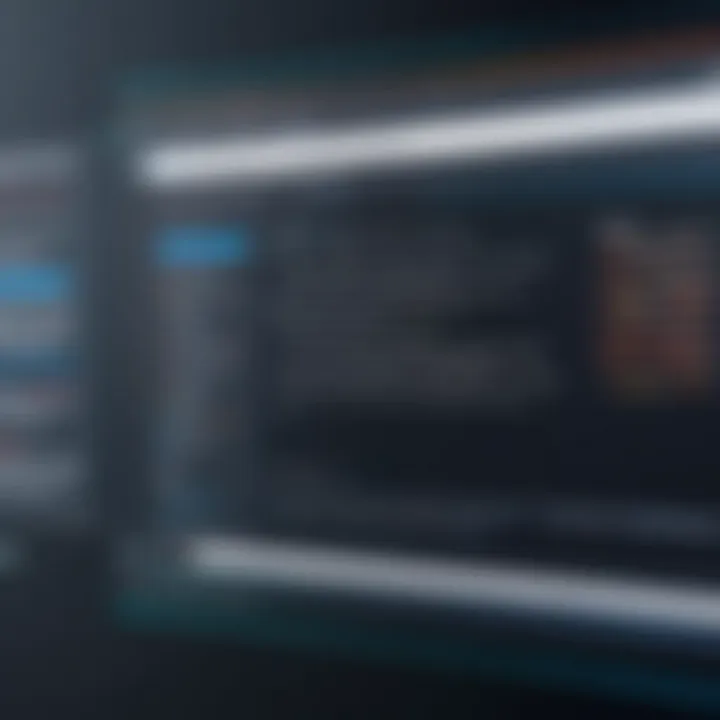
Overview of Topic
Java is a powerful programming language known for its versatility and widespread use. As a core language in many tech applications, mastering Java can significantly boost oneโs career in the IT industry. This guide seeks to provide a structured environment for learners, focusing on simple Java programs that facilitate practice and reinforce key concepts.
Java was introduced in the mid-1990s and has undergone numerous evolutions to keep pace with advancements in technology. Today, it powers a vast array of applications, from desktop software to web services. Understanding its fundamentals can lead to improved problem-solving skills and a deeper appreciation of software design.
Fundamentals Explained
At the core of Java programming lies an array of fundamental principles that are essential for both beginners and more experienced coders. Some key concepts include:
- Object-oriented programming (OOP): Java is fundamentally designed around OOP principles, which help in structuring code more efficiently.
- Syntax and semantics: Understanding Javaโs syntax is crucial for writing error-free code.
- Data types: Knowing how to use various data types effectively can greatly enhance coding practices.
Key terms to grasp include:
- Classes and Objects: The building blocks of Java programs.
- Inheritance: A mechanism to create a new class from an existing class, promoting code reuse.
- Polymorphism: Allowing objects to be processed in multiple forms and providing flexibility.
Practical Applications and Examples
Practical application of Java skills solidifies learning. Here are examples of simple programs to practice:
- Calculator: Create a console-based calculator that can perform basic mathematical operations. This program will help you understand user input and control structures.
- Number Guessing Game: Develop a game where the user must guess a randomly generated number. This will enhance your grasp of loops and conditionals.
- Basic Banking System: Simulate banking operations such as deposits and withdrawals. This project introduces you to classes and object interaction.
Code Snippet Example
This code demonstrates how to take user input and perform arithmetic operations.
"Consistent practice with simple Java programs can enhance your coding skills significantly."
Tips and Resources for Further Learning
To advance your Java skills further, consider the following resources:
- Books: "Head First Java" by Kathy Sierra and Bert Bates offers a comprehensive introduction.
- Online Courses: Platforms like Coursera and Udemy offer targeted courses for Java learners.
- Tools: Download Java Development Kit (JDK) and an Integrated Development Environment (IDE) like IntelliJ IDEA or Eclipse to streamline coding efforts.
Utilizing these resources can yield a structured path to mastering Java programming, enjoying both the learning process and its applications.
Prologue to Java Programming
Java programming stands as a critical gateway in the world of software development. For many learners, it serves as the first step into a vast ecosystem of programming languages and paradigms. Understanding Java is not just about learning syntax or writing code; it is about grasping the fundamental concepts of programming that apply across languages. This framework is built on solid principles, and as such, Java is both beginner-friendly and robust enough for experienced developers.
Importance of Practice
Practicing programming is essential for mastering any language, and Java is no exception. Repeated exposure to problems and coding scenarios reinforces learning. When students engage in simple Java programs, they gain practical experience that solidifies theoretical knowledge. This process aids in:
- Deepening understanding of Java's syntax and structure.
- Cultivating problem-solving skills.
- Developing debugging proficiency, as encountering and resolving errors is part of the learning journey.
Hands-on practice also builds confidence. As learners tackle various challenges, they become more comfortable with Java, which makes it easier to adopt more advanced coding concepts later on. The key to success is consistent effort and practice.
Overview of Java Features
Java possesses a variety of features that make it a popular choice in the programming world. These attributes contribute to its usefulness in diverse applications, from web development to enterprise software. Some noteworthy features include:
- Platform Independence: Java is designed to be platform-independent at both the source and binary levels. The concept of "write once, run anywhere" signifies that Java code can run on any device that has a Java Virtual Machine (JVM).
- Object-Oriented Programming: Java emphasizes an object-oriented approach. This paradigm fosters code reusability and modular design, simplifying programming tasks and promoting cleaner code.
- Strongly Typed Language: The strong typing of Java aids in catching errors at compile time rather than at runtime, enhancing the reliability of applications.
- Rich Standard Library: Java includes a comprehensive library that provides numerous APIs for various functions, making it easier to build complex applications without starting from scratch.
These features combine to form a robust environment for practitioners of all levels. By exploring these characteristics through hands-on experience, learners can better appreciate and leverage the full potential of Java in their projects.
"Practicing simple coding tasks is the key to unlocking deeper understanding and proficiency in programming languages like Java."
Engaging with the language through practical exercises enhances retention and understanding, paving the way for more complex programming challenges.
Fundamental Concepts
Fundamental concepts form the backbone of any programming language, including Java. Understanding these concepts is crucial for anyone looking to build their skills in coding. By mastering basic principles, learners can create a solid foundation that benefits them as they delve into more complex topics. Moreover, these concepts aid in developing problem-solving skills, which are vital in coding.
Variables and Data Types
In Java, variables are used to store data that can be manipulated throughout a program. A variable acts as a label for a memory location where data is kept. Understanding variables is essential because it helps programmers manage data efficiently.
Data types in Java define the kind of data that can be stored in a variable. Common data types include for integers, for floating-point numbers, for single characters, and for text. Selecting the correct data type is important to conserve memory and optimize performance.
Control Structures
Control structures govern the flow of a program and determine how different parts of code execute. They are vital for implementing logic and decision-making in programs.
Conditional Statements
Conditional statements allow a program to execute different code based on specific conditions. The , , and statements are essential for enabling this functionality. A key characteristic of conditional statements is their ability to handle multiple pathways in program execution, making them indispensable for complex software logic.
The benefit of using conditional statements is their straightforwardness. They allow programmers to write clear and logical decisions in their code.
One unique feature of conditional statements is the statement, which is useful when dealing with multiple possible conditions. However, one drawback is that they might lead to complicated nested structures if not managed well.
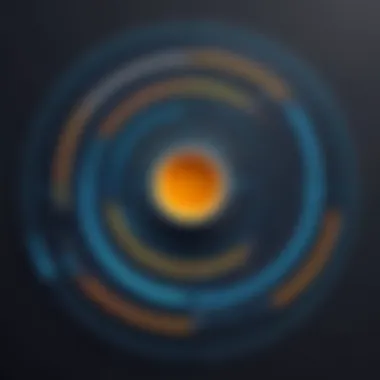
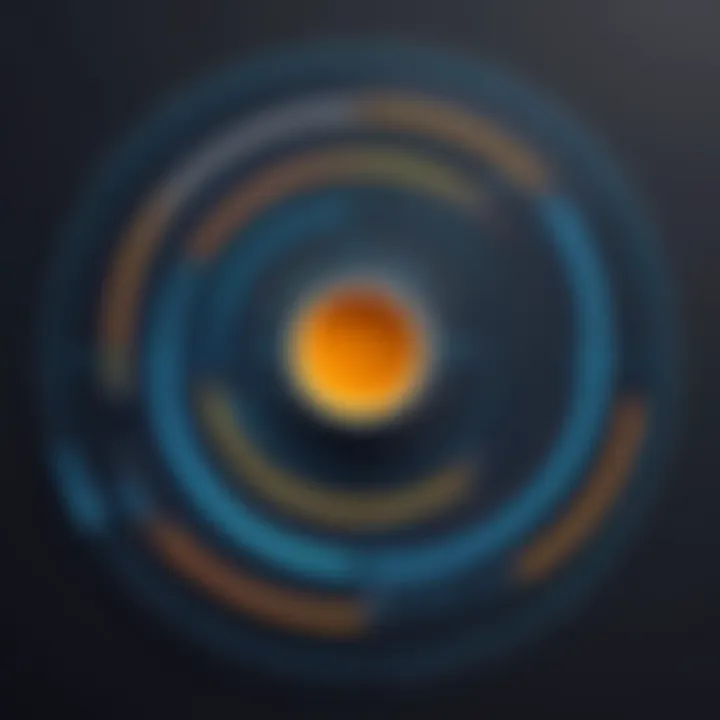
Loops
Loops are structures that repeat a block of code until a specific condition is met. They significantly contribute to reducing code redundancy. Java has several looping mechanisms, such as , , and loops.
A key characteristic of loops is their ability to handle repetitive tasks efficiently. This makes them a popular choice for various programming situations, particularly when processing large amounts of data.
The unique feature of loops lies in their control over iterations. However, they can lead to infinite loops if the exit condition is never met, which is a significant disadvantage.
"Loops and Conditionals together provide the necessary tools for implementing logic in programming. Understanding them thoroughly leads to better code quality and performance."
By honing skills in these fundamental concepts, learners can more effectively tackle challenges in Java, ultimately leading to improved coding abilities.
Simple Java Programs
Simple Java programs form the backbone of practical coding skills for learners in this field. Engaging with these exercises allows both beginners and intermediate coders to reinforce essential programming concepts. These programs stand as gateways, not just into the Java language but also into the logical thinking required for coding.
The main benefits of focusing on simple Java programs include the following:
- Foundational Learning: These programs teach fundamental concepts such as variables, data types, and control structures, which are crucial in building more intricate systems in the future.
- Confidence Building: Successfully completing straightforward tasks boosts self-confidence and motivates learners to advance to more complex challenges.
- Practical Application: Each program serves as a practical example. This real-world application reinforces theoretical knowledge, making learning more effective.
When approaching these programs, it is crucial to understand the underlying concepts thoroughly. Practicing coding can significantly enhance cognitive skills related to problem-solving and logical analysis.
"Practice is the best teacher."
By consistently tackling simple problems, learners can improve their coding fluency and prepare themselves for tackling bigger projects in the future.
Print Hello World
"Hello, World!" is often the first program written by beginners. It serves as a rite of passage into the world of programming. This program is deceptively simple; it introduces key principles of Java syntax. The objective is to display the text on the console, teaching users about the basic structure of a Java application.
Here is the code for the Hello World program:
In this program, the class contains the main method that Java looks for when executing the program. Inside this method, is called to output the string "Hello, World!" to the console. By completing this exercise, learners familiarize themselves with Java's structure and syntax. This foundational step is crucial for further learning.
Basic Calculator
The Basic Calculator program is an excellent way to apply concepts of operations and control structures. This program will allow users to perform simple arithmetic operations such as addition, subtraction, multiplication, and division. Such exercises provide hands-on experience with input handling and decision-making processes.
The following is an example code for a Basic Calculator:
In this code, we use a to accept user input. Based on the user's choice of operation, a switch statement determines which mathematical operation to perform. By practicing this program, learners can explore basic principles of user interaction, conditional logic, and arithmetic operations in Java.
Data Structures in Java
Data structures form a fundamental part of programming, enabling the efficient organization and management of data. In Java, understanding data structures is crucial as they provide the basic mechanisms needed to store and manipulate information effectively. This section highlights the importance of two primary data structures: arrays and ArrayLists. Both serve distinct purposes and demonstrate different characteristics, which are relevant for the development of various applications in Java.
Arrays
Arrays are one of the simplest data structures in Java, designed to store a fixed-size sequential collection of elements of the same type. They contribute significantly to the overall goal of efficient data management due to their straightforwardness and efficient access time.
Declaring Arrays
Declaring arrays is a fundamental step when working with them in Java. It involves specifying the type of elements you want to store and the size of the array. For example:
In this example, an integer array named of size 5 is declared. The key characteristic of array declaration is that you need to know the size of the array at the time of declaration. This static nature is a beneficial feature, as it allocates memory efficiently, allowing fast access and retrieval of elements by using their index.
The unique feature of declaring arrays is that even though they are fixed in size, they are simple to implement and understand, making them popular among beginners in programming. However, a disadvantage is that once an array's size is defined, it cannot be altered, which can be limiting when dealing with dynamic data.
Accessing Array Elements
Accessing array elements in Java is straightforward and efficient. Each element can be reached using its index, which starts from zero.
For example:
This line retrieves the first element of the array. The key characteristic of accessing elements is that it provides constant-time complexityโO(1)โfor retrieval. This efficiency means that no matter how large the array, accessing an element remains very quick.
One unique aspect of accessing array elements is the ability to loop through the elements efficiently using or loops. This capability is particularly advantageous when processing a collection of data. However, care must be taken to avoid , which occurs if you try to access an invalid index.
ArrayList
ArrayList is a part of the Java Collections Framework and represents a resizable array implementation. It allows for dynamic resizing, which makes it quite different from the static arrays.
Its flexibility is one of the primary reasons ArrayList is favored in many programming scenarios. You can add or remove elements without worrying about the underlying array size. This feature makes them a very useful structure when the total number of elements is not known in advance or may change.
Understanding when to use arrays over ArrayLists boils down to the specific requirements of the application. If you need a simple fixed-size collection, arrays are adequate. However, for more complex scenarios where operations on the collection occur frequently, ArrayList provides a more versatile approach.
Program Challenges
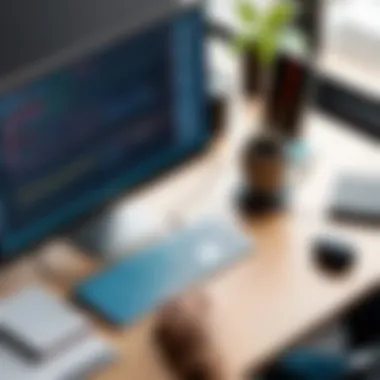
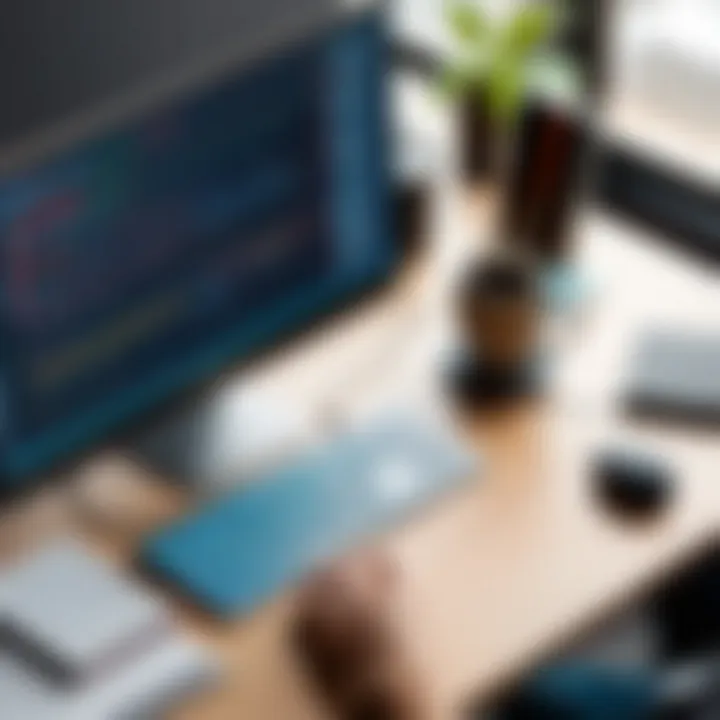
In the realm of programming, challenges serve as essential tools for growth. The section on Program Challenges is vital in this context, as it encourages learners to engage in practical problem-solving. By tackling these challenges, students will find opportunities to apply theoretical knowledge in a tangible manner. Not only does this lead to a deeper understanding of Java, but it also fosters critical thinking and logical reasoning.
Moreover, programming challenges help reinforce concepts learned in earlier sections, such as data structures and algorithms. Addressing distinct problems exposes learners to various approaches and techniques. It is important to keep in mind that failing to solve a problem is not a setback. Instead, it represents a chance to analyze oneโs thought process, seek solutions, and grow more resilient.
Find the Largest Element
Finding the largest element in an array is a common programming challenge that hones problem-solving skills. This exercise requires the use of loops and conditionals, crucial elements of Java programming. The goal is to iterate through each item in the array, compare it to the current maximum value, and update the maximum if a larger value is found.
To illustrate, consider the following aspect of this program:
- Initialization: Start with an array of integers and set a variable to hold the largest value.
- Iteration: Use a loop to examine each element one-by-one.
- Comparison: For each element, compare it against the current largest. If it is larger, update the largest.
This simple exercise not only improves syntax skills but also strengthens the understanding of arrays. Here is a basic code snippet to demonstrate:
This code not only solves the problem but also helps identify areas for further exploration, such as optimizing for efficiency.
Count Vowels in a String
The challenge of counting vowels in a string is another fundamental exercise for Java learners. This task develops skills in string manipulation and character analysis. It requires a keen attention to detail, as the program must recognize both uppercase and lowercase vowels.
In order to achieve this, the steps include:
- Input Handling: Retrieve or define a string to analyze.
- Character Looping: Iterate through each character in the string.
- Vowel Checking: For each character, determine if it is a vowel and maintain a count.
A sample implementation could look like this:
This simple program solidifies the understanding of strings and reinforces essential coding concepts. Counting vowels also presents an opportunity to reflect on character encoding and text processing, which are significant aspects of programming in Java.
Object-Oriented Programming
Object-Oriented Programming (OOP) is a core paradigm in Java that emphasizes the use of objects to represent data and methods that operate on that data. Understanding OOP is crucial for anyone looking to demonstrate proficiency in Java. The importance of this concept spans from code organization to enhanced reusability. In this section, weโll explore the essentials of OOP, focusing on its primary components: classes and the principles of inheritance and polymorphism.
Creating Classes
A class acts as a blueprint for creating objects. It encapsulates data for the object and methods to manipulate that data. This encapsulation is not just for organization but also for data protection. Hereโs how to define a basic class in Java:
In this example, the class includes two attributes: and , along with a method . This method prints the car's details. By creating instances of the class, we can initialize these variables and use the method to display the information. This is a powerful tool as it allows for creating complex structures while maintaining clarity in how the functionality is executed.
Tip: Classes should model real-world entities. This makes them easier to understand and use, especially for beginners.
Inheritance and Polymorphism
Inheritance allows a new class to inherit properties and methods from an existing class. This promotes code reusability. In Java, the keyword is used to achieve inheritance. Consider the following example:
The class extends , gaining its attributes and methods. Now, can also define additional behavior specific to sports cars, such as . This shows how classes can build on one another.
Polymorphism refers to the ability of a single method to behave differently based on the object that invokes it. This can be achieved in Java through method overriding and method overloading. Here's a simple example of method overriding:
Here, the method in the class has a different implementation than in the parent class. Polymorphism allows developers to implement dynamic method invocation at runtime, making programs more flexible and easier to extend.
In summary, embracing the principles of object-oriented programming in Java not only aids in writing clean and manageable code but also encourages a systematic approach to problem-solving. This foundation is essential for developing complex software systems.
Handling Exceptions
Handling exceptions is a critical topic in Java programming. It enables developers to create robust applications that can manage errors effectively while maintaining a seamless user experience. Inherent in software development are the possibilities of unexpected behaviors, such as input mismatches, file-not-found errors, or network connectivity issues. Addressing these situations proactively through exception handling is vital.
Effective exception handling enhances the reliability of your code. It provides a mechanism to catch potential errors and respond accordingly, instead of allowing the program to crash. This makes it easier to debug and improve application performance. It also plays an important role in maintaining clean code structure, thus leading to fewer bugs in the final product.
Beyond basic functionality, handling exceptions allows for clearer communication of errors back to the user or other parts of the system. This ensures that the end user is aware of any issues and assists in troubleshooting resulting problems. Understanding how to handle exceptions is, therefore, an essential skill that anyone working on Java projects must master.
Understanding Exceptions
Exceptions in Java are events that disrupt the normal flow of execution in a program. They comprise various conditions, including runtime errors and logical errors, which the system recognizes as significant issues during program execution. These exceptions can originate from various sources, including user input, hardware malfunctions, or even logic within the code itself.
Java categorizes exceptions into two main types: checked exceptions and unchecked exceptions. Checked exceptions are those which the programmer is forced to handle during compilation, typically using try-catch blocks. Unchecked exceptions, on the other hand, usually occur at runtime. They can often be avoided through proper coding practices but are not necessarily subject to mandatory handling.
When exceptions arise, Java provides an intricate stack trace, detailing the source of the error. This trace aids developers in debugging processes by pinpointing the exact line where the error occurred, thus simplifying the correction process. Understanding these elements is crucial for anyone looking to write effective Java code that anticipates and mitigates potential failures.
Try-Catch Block
The try-catch block is a fundamental construct in Java used to handle exceptions. It allows a program to maintain control during error occurrences rather than terminating unexpectedly. The syntax for a try-catch block is straightforward, and it is essential for creating resilient programs.
A typical structure includes a block where code that might throw an exception is placed. Following this is one or multiple blocks that specify how to handle different types of exceptions.
Here is a simple example:
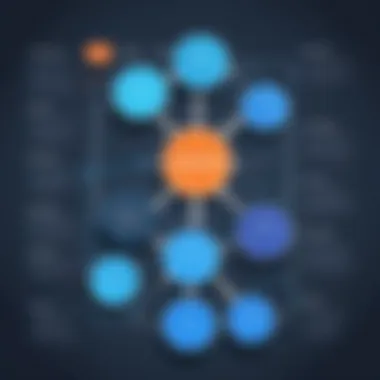
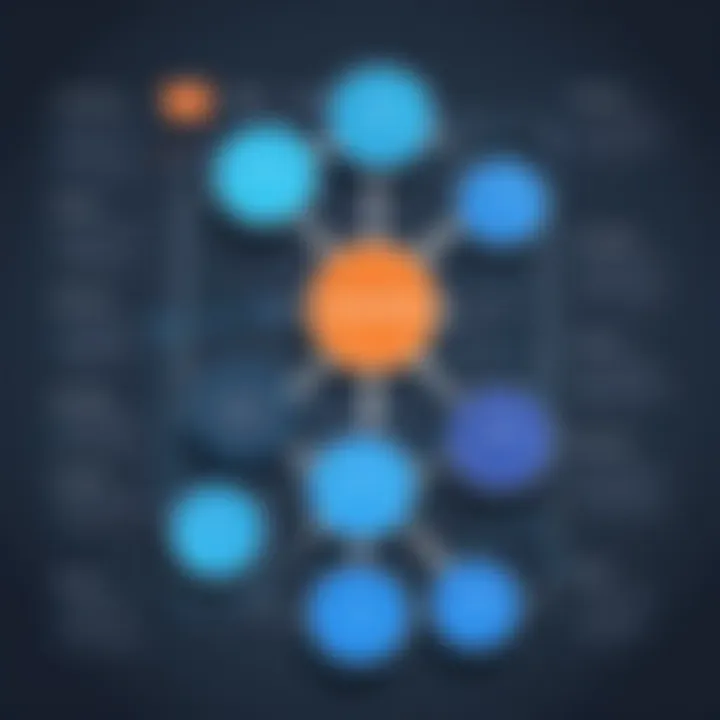
In this code, attempting to divide by zero will raise an . Instead of stopping the program, the catch block captures this exception and prints a user-friendly message.
Using try-catch blocks effectively can save time during later stages of development. It fosters an environment where errors are debugged quickly and allows for specific responses to different exceptions. This makes each program more user-friendly and encourages maintainability in the long run.
Advanced Programming Techniques
The field of programming is consistently evolving, presenting new paradigms and methodologies that extend beyond the fundamental concepts. In this article, the section on Advanced Programming Techniques aims to elevate your skill set and reinforce your understanding of Java programming through pertinent exercises. This section introduces two critical areas: File I/O Operations and Multithreading Basics which are often essential in real-world applications.
Mastering these concepts opens doors to more complex applications. Engaging with advanced programming techniques enhances your ability to write efficient and scalable code. This can lead to better performance in applications and improved user experiences. It also lays the groundwork for understanding more complex frameworks and libraries you may encounter as you advance in your programming journey.
File /O Operations
File I/O Operations are an essential part of Java programming that deals with the reading and writing of files. Being proficient in managing files can greatly benefit programs that rely on persistent data storage. You may need to store user data, configuration settings, or logs. Java provides a robust API for file handling that supports various formats like text and binary files.
Handling files in Java generally involves the following steps:
- Importing Necessary Classes: You will often work with classes like , , and .
- Creating File Objects: It begins with creating a object that references the desired file in your file system.
- Reading & Writing Data: Utilize to read data and for writing data. You may also use and for efficient reading and writing operations.
- Error Handling: Implementing proper error handling is crucial. Using try-catch blocks can help manage exceptions while working with files, ensuring that your program remains robust.
Here is a basic example of reading a text file using :
This code illustrates the foundation of file reading in Java. Understanding file I/O will enable you to develop applications that can store and manipulate data effectively.
Multithreading Basics
Multithreading is a critical concept in Java that allows concurrent execution of two or more threads. It enhances performance significantly, especially in applications requiring multitasking or parallel processing. Java's built-in support for multithreading enables developers to create highly responsive applications.
- Threads: A thread is a lightweight process. Each Java application has at least one thread, the main thread, which can spawn additional threads for performing tasks such as handling user interfaces, processing data, or managing network operations.
- Creating Threads: You can create a thread by extending the class or implementing the interface. The second approach is generally preferred for better scalability.
- Synchronization: While multithreading boosts performance, it can lead to concurrency issues, such as data inconsistency. Utilizing synchronization methods helps to control access to shared resources between threads, ensuring thread safety.
Here's a simple example demonstrating thread creation using the interface:
Understanding these advanced techniques enhances your capability as a Java programmer, preparing you for larger and more complex projects. Embrace these skills and continue to apply and refine them as you grow in your coding journey.
Effective Debugging Strategies
Effective debugging strategies are essential for mastering Java programming. Debugging is not just about finding errors; it is about understanding the code deeply and enhancing the overall coding process. Employing good debugging techniques can save time, reduce frustration, and improve code quality. Developers must go beyond simple error identification to comprehend the logic of their programs. Understanding where and why errors occur leads to better programming practices and skill development.
Common Errors in Java
Java, like many programming languages, has its share of common errors that new programmers encounter. Some of these errors include:
- Syntax Errors: These happen because of typos or mistakes in the structure of code. Example: missing semicolons or mismatched parentheses.
- Runtime Errors: These are often unexpected issues that occur during program execution. For instance, accessing an array index that does not exist.
- Logical Errors: These are harder to detect because the code runs without crashing, but it produces incorrect results due to flawed logic.
Understanding these common errors is the first step in effective debugging. It enables learners to recognize patterns in their mistakes, allowing them to adjust their approach to writing code. By acknowledging these frequent pitfalls, programmers can formulate better testing and debugging strategies tailored to their specific coding habits.
Using Debugger Tools
Debugger tools are invaluable assets in the programming workflow. In Java, tools like Eclipse, IntelliJ IDEA, and Visual Studio Code include integrated debugging features. These tools allow developers to step through their code line by line. Benefits of using a debugger tool include the following:
- Breakpoints: Programmers can set breakpoints to pause execution at specific points. This is useful for inspecting variable states.
- Variable Inspection: Debuggers allow users to view the values of variables in real-time. This can help identify where things are going wrong.
- Call Stack Monitoring: Understanding the call stack can shed light on the sequence of method calls leading to an error.
The intuitive interfaces and functionalities of these tools make debugging a more manageable and less stressful experience. Without proper tools, it can be hard to trace the source of complex errors.
"Effective debugging is about understanding the underlying logic of code, not just fixing errors."
By integrating the use of debugging tools and understanding common errors, learners can enhance their coding practice. As they become efficient at debugging, they are not only able to produce error-free code but also develop a clearer understanding of Java programming principles.
Epilogue and Next Steps
In reflecting upon your journey through the various Java programming exercises, it is essential to understand the significance of this conclusion and what lies ahead. The completion of simple Java programs not only solidifies your understanding of foundational concepts but also sets the stage for continuous improvement. As coding is a skill honed through practice, recognizing your accomplishments encourages further engagement with more complex topics.
Here, we will highlight the importance of each component covered in this article, including data structures, control flow, and object-oriented programming. A solid grasp of these topics is crucial for any programmer, as they form the backbone of many real-world applications. The exercises were structured to progressively build your coding ability, allowing you to tackle challenges with confidence.
It is pivotal to remember that coding is iterative. Each program you create, each error you debug, and each concept you master builds towards a greater understanding. As you conclude this exercise framework, consider not just your progress but the multitude of directions your coding journey can take. Here are a few considerations:
- Assess Your Knowledge: Regularly evaluate your grasp of key programming concepts. This can be done through self-quizzing or peer discussions.
- Explore Advanced Topics: Transitioning into topics such as multithreading or network programming can open new avenues for your skills.
- Engage with Communities: Interacting with platforms such as Reddit can provide insight from fellow learners and professionals and expose you to different problem-solving methods.
Thus, while this article provides a map, your coding journey is uniquely yours. Each step taken prepares you for subsequent challenges.
"Programming can be viewed as a craft; dedicating time for practice refines that craft into expertise."
Recap of Key Concepts
Throughout this article, we engaged with essential Java concepts that are pivotal in any programmer's toolkit. Here is a recap:
- Java Syntax: Understanding the basic structure of Java code is fundamental. This includes proper use of semicolons, curly braces, and method declarations.
- Control Structures: We explored how conditional statements, like , influence program flow and how loops can automate repetitive tasks.
- Data Structures: Familiarity with arrays and ArrayLists enhances your ability to store and manipulate collections of data effectively.
- Object-Oriented Programming: Knowledge of classes and inheritance is crucial for building scalable and maintainable applications.
- Error Handling: Utilizing try-catch blocks ensures robust programs that can gracefully handle unexpected issues.
By revisiting these core areas, you solidify your foundation for when you decide to dive into advanced topics, ensuring you're well-prepared.
Resources for Continued Learning
To further your understanding and mastery of Java programming, a multitude of resources are available. Here are some valuable options:
- Online Platforms: Websites like Codecademy and Coursera offer structured courses and interactive coding environments.
- Books: Consider "Effective Java" by Joshua Bloch for nuanced insights into good practices or "Java: The Complete Reference" by Herbert Schildt for comprehensive coverage.
- Community Forums: Engaging in forums such as Stack Overflow or Reddit's r/learnprogramming can provide direct access to advice and troubleshooting.
- Documentation: Don't underestimate the power of official Java documentation at oracle.com. It offers clear explanations and examples for deeper exploration.
- YouTube Channels: Channels like The Net Ninja and Derek Banas can deliver visual and practical coding guidance.
By utilizing these resources, learners not only sustain their engagement with programming but also encourage ongoing challenges that sharpen their skills. Your commitment today lays the groundwork for the proficient developer you aspire to become.