The JavaScript Console: A Key Tool for Development
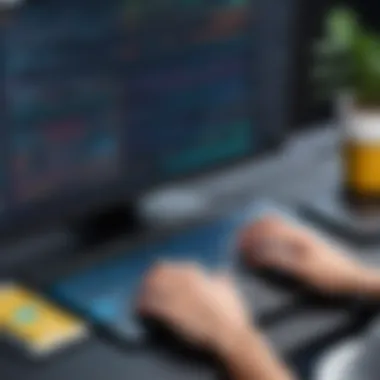
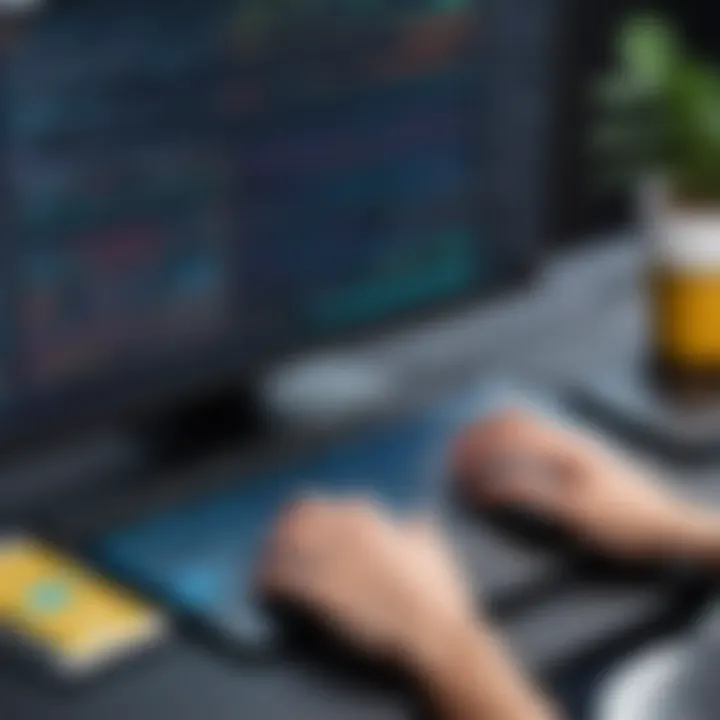
Overview of Topic
The JavaScript console represents a pivotal innovation within modern web development. Initially, it served as a basic tool for developers to log information and debug scripts quickly. Over the years, it has evolved into a comprehensive environment used for error handling, testing, and improving overall web application performance. This makes it a crucial aspect of the development workflow, influencing how developers interact with their web applications.
Its significance in the tech industry cannot be overstated, as the console aids in identifying bugs and enhancing the functionality of web applications. As the demand for web applications continues to surge, the need for effective debugging and testing tools like the JavaScript console becomes ever more pertinent.
The history of the JavaScript console dates back to the early days of web programming. Originally part of the Netscape browser, the console has grown through various iterations and enhancements across different browsers, including Google Chrome, Mozilla Firefox, and Microsoft Edge. Each iteration reflected the changing needs of developers, integrating more robust features and capabilities.
Fundamentals Explained
Understanding the core principles governing the JavaScript console is essential for effective use. The primary function of the console is to facilitate communication between developers and the web browser. This interaction enables developers to execute commands, inspect elements, and view logs generated during runtime.
Key terminology includes:
- Console.log: A method to print messages to the console.
- Debugging: The process of identifying and rectifying errors in code.
- Error Handling: A strategy to gracefully manage errors in applications.
These basic concepts form the foundation upon which advanced functionalities are built. Recognizing how to effectively use commands and interpret feedback from the console can significantly enhance a developer's workflow.
Practical Applications and Examples
The JavaScript console is not just theoretical; it has real-world applications that can improve development processes. For instance, using console.log to debug a JavaScript function allows developers to track variable values and flow without interrupting the workflow.
Real-world case studies show that teams who leverage the console efficiently can reduce the debugging time by substantial margins, leading to faster project completion rates. For example, consider a scenario where a developer uses the console to investigate an unexpected behavior in a web app. By systematically checking variable outputs, they can pinpoint where in the code things go wrong, allowing for quicker resolutions.
Below is a simple code snippet demonstrating how to use the console to log variable values:
This usage illustrates one of the most fundamental capabilities of the console while showcasing how easily errors can be detected and corrected.
Advanced Topics and Latest Trends
As web development continues to evolve, new trends emerge regarding the use of the JavaScript console. Advanced topics include asynchronous debugging and utilizing the console for performance analytics. Modern tools enhance functionality; for example, many browsers support live editing and real-time feedback through their consoles.
Developers are increasingly engaging in methodologies such as source map debugging, which facilitates breakpoints in minified code. This allows for an efficient debugging process that saves time and promotes cleaner code practices.
Looking ahead, the future of JavaScript consoles may incorporate artificial intelligence for automated bug detection, potentially revolutionizing how developers approach debugging.
Tips and Resources for Further Learning
For those eager to deepen their understanding of the JavaScript console, several resources are essential:
- Books: "You Don’t Know JS" by Kyle Simpson offers deep insights into the JavaScript language, including effective debugging techniques.
- Online Courses: Platforms like Udemy and Coursera provide courses specifically focusing on web development tools, including the JavaScript console.
- Tools: Tools such as Chrome DevTools provide a rich environment for practical usage beyond basic commands.
Engagement with community forums, such as Reddit and various online developer communities, can also provide valuable insights and tips from experienced developers.
Intro to the JavaScript Console
The JavaScript console is a crucial element within web development that many developers often overlook until they encounter issues. This section aims to clarify the significance and practical utility of the console. Understanding its use can profoundly impact a programmer's ability to debug and interact with web applications efficiently.
Definition and Purpose
The JavaScript console serves as an interface that allows developers to execute JavaScript code snippets and view the output. Often built into web browsers, it is accessible through developer tools. The primary purpose of the console is to provide a platform for logging messages, running commands, and displaying errors and warnings. This tool acts as a bridge between the code written and the outcome of that code.
In addition to logging, the console can be a powerful debugging tool. By inspecting objects and variables, a developer can pinpoint issues more effectively, reducing the time spent searching for problems. This capability enhances overall productivity, as developers can quickly identify where their code diverges from expected behavior.
Historical Context
The concept of a console in programming has existed for decades, evolving alongside programming languages and environments. In earlier stages of web development, developers relied heavily on alert dialogs for debugging, which provided minimal information and disrupted user experience. As JavaScript matured, so did the tools available for developers.
The introduction of the console as part of browser developer tools allowed for a more integrated approach. Browsers like Chrome, Firefox, and Safari have continuously improved their consoles with features such as syntax highlighting, command history, and contextual debugging information. Understanding this evolution underscores the importance of the console in modern web development and illustrates its role as a key component in the workflow of both novice and experienced developers.
Accessing the JavaScript Console
Accessing the JavaScript console is a foundational skill for developers engaged in web development. The console serves as the primary interface for executing JavaScript code, diagnosing issues, and viewing real-time outputs. A deep understanding of how to access and utilize this tool can significantly enhance a developer's efficiency while debugging or testing scripts. In this section, we will explore the methods for accessing the JavaScript console and the benefits of mastering this crucial resource.
Using Developer Tools in Browsers
Most modern web browsers come equipped with built-in developer tools that include the JavaScript console. These tools allow developers to inspect web pages, modify HTML and CSS in real-time, and execute JavaScript code on-the-fly. To access the console, one can typically navigate to the 'Developer Tools' menu or use a quick keyboard shortcut.
- Google Chrome: Right-click on the page and select "Inspect" or press . After that, click on the "Console" tab.
- Mozilla Firefox: Similar to Chrome, right-click and choose "Inspect Element", then go to the "Console" tab.
- Microsoft Edge: Access by right-clicking and selecting "Inspect" or pressing . Navigate to the "Console" tab afterward.
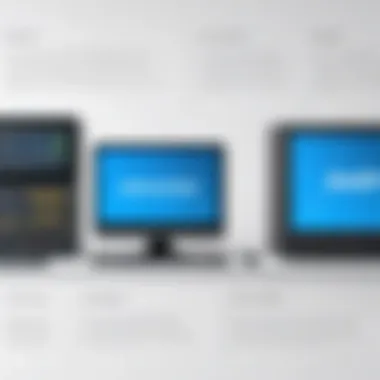
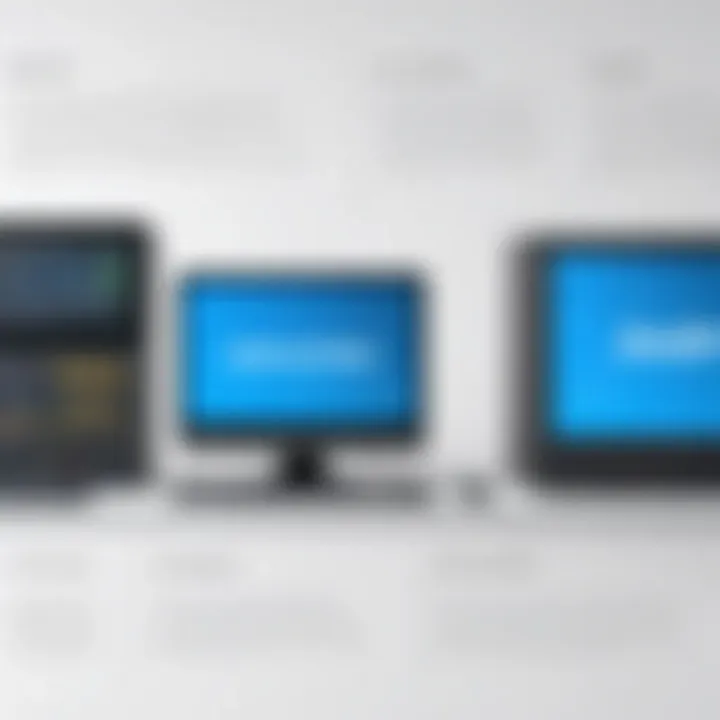
Using the developer tools not only grants access to the console, each browser also provides other panels that allow for in-depth inspection of network requests, performance issues, and security settings. This makes the console an even more powerful feature within the context of overall web development.
Basic Functions of the Console
The JavaScript console serves various crucial functions in web development. Understanding these basic functions is vital to utilizing the console effectively. This section focuses on how the primary capabilities of the console enhance debugging processes and improve the development workflow.
Logging Information
Using console.log
The function is one of the most fundamental tools for developers. It allows the output of information to the console, providing insights into code execution and variables. By logging essential data points, developers can trace the flow of their applications, making it easier to identify issues.
A key characteristic of is its versatility. It can log strings, numbers, objects, and arrays. This feature makes it a popular choice among developers. It helps them visualize what data is being manipulated during the execution of programs. One unique aspect is that it automatically converts objects to a readable format in the console.
However, overusing can clutter the console output and make debugging cumbersome. It's beneficial in development but should be used judiciously in production.
Different logging levels
In addition to , there are other logging methods that denote different severities. These include , , and . Utilizing different logging levels helps differentiate between types of messages, enhancing the clarity of the output.
The key advantage of this approach is that it allows developers to implement effective filtering in the console. For instance, using for warnings puts emphasis on potential issues without marking them as critical errors.
The unique feature here lies in the communication of message severity to the developer, providing a better understanding of application issues. Still, relying on these log levels effectively requires discipline, as misclassifying messages can confuse the development process.
Displaying Errors and Warnings
console.error
The function explicitly logs errors occurring in JavaScript. This method provides a standardized way to report issues, which is invaluable for debugging. When an error is logged, it typically appears in red text, making it stand out in the console.
Its primary characteristic lies in its visibility. Errors are hard to miss, which allows developers to address problems quickly. can take arguments, just like , making it adaptable for printing complex error messages.
However, a potential downside is that excessive reliance on error logs can create an overwhelming amount of information if many issues are present.
console.warn
The function serves to highlight warnings—situations that do not necessarily stop execution but should be noted. It provides a mechanism for developers to flag sections of code that may require attention.
The key characteristic of is its ability to communicate caution without indicating a serious problem. The output is typically shown in yellow, providing a clear visual distinction from regular log messages.
This function is advantageous for identifying code that may lead to errors in certain conditions. Nonetheless, one challenge is ensuring that warnings don’t get ignored; developers may dismiss frequent warnings as negligible, which can lead to larger problems down the line.
Debugging with the Console
Debugging is a crucial part of web development, and the JavaScript console serves as a pivotal instrument in this process. It allows developers to execute code snippets, view the output, and promptly identify issues within their scripts. When working on complex applications, integrating console commands into your debugging workflow can greatly enhance productivity. The console not only helps in tracking down bugs but also provides insights into how the code is executed in real-time. Understanding its features can significantly reduce the time spent on troubleshooting and improve the overall application performance.
Understanding Stack Traces
When an error occurs in JavaScript, it generates a stack trace that provides important information about where the problem lies. A stack trace lists the sequence of function calls that led to the error. The top of the stack represents the most recent call, while the bottom represents the initial entry point of the execution.
Here’s a basic outline of the process:
- Error Logging: When an error is detected, the console outputs the stack trace along with the error message. This detail helps developers pinpoint the exact location of the issue.
- Navigating the Trace: By following the lines in the stack trace, one can backtrack through the function calls to determine the source of the error. Each entry typically shows the function name and the line number in the script where it resides.
- Identifying Patterns: Repeated stack traces can highlight systematic issues within the code. Understanding common patterns can help in rewriting or refactoring the code to avoid similar problems in the future.
Using console.error effectively facilitates this process by generating clear, informative logs that could serve as key points for analysis.
Setting Breakpoints
Breakpoints are instrumental in managing the flow of execution in your code. They allow developers to pause the execution at a specific line, facilitating an in-depth inspection of variables at that point. Setting breakpoints in the browser’s developer tools is straightforward:
- Locating the file: Navigate to the source file within the developer tools.
- Adding a breakpoint: Click to the left of the line number where you wish to pause execution.
- Resuming execution: When paused, you can examine variable values, step through code line-by-line, or even modify the state of your application if needed.
This feature makes it easier to diagnose logic errors and understand the flow of the program. Developers often leverage breakpoints to navigate through loops or complex functions where multiple conditions exist. By pausing at critical stages, insights can be gained that may not be evident when executing the entire script at once.
"Understanding how to effectively debug your code using breakpoints and stack traces can eliminate frustration and streamline your development process."
Utilizing the JavaScript console for debugging is an indispensable skill for any developer aiming to create robust and efficient web applications. The ability to explore stack traces and control execution flow through breakpoints elevates debugging efforts, making the overall development experience smoother and more effective.
Advanced Console Features
The advanced console features offer web developers tools that elevate the debugging process beyond basic logging. They provide a structured way to analyze performance, organize output, and streamline development workflows. Utilizing these features can significantly enhance the efficiency of troubleshooting and performance monitoring. Developers who master these tools can gain fine-grained insights into their code, which is crucial in a fast-paced web development environment.
Timing Events
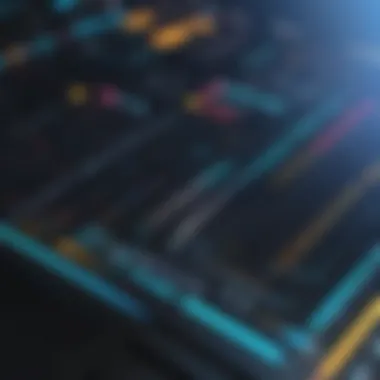
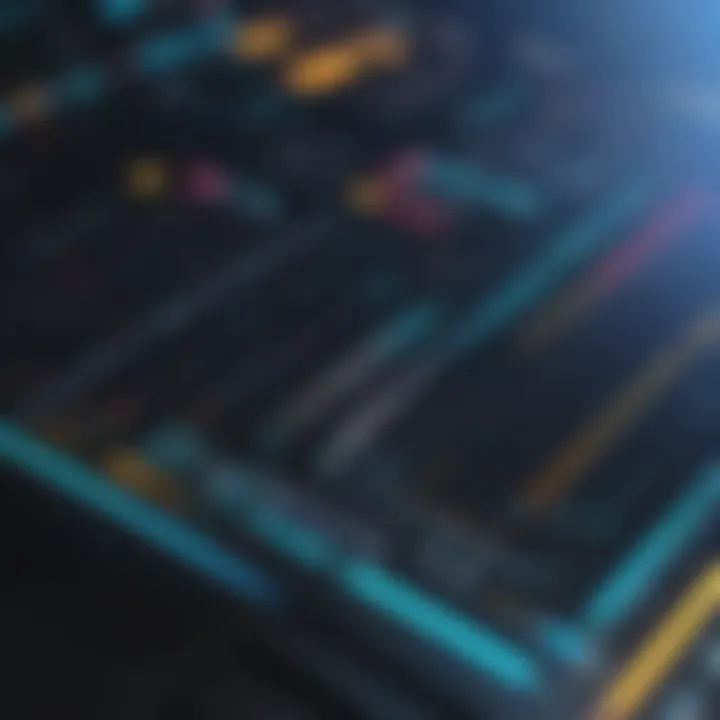
console.time
The method is essential for performance measurement in JavaScript. It allows developers to mark the beginning of a timer associated with a specific label. It is a straightforward tool that helps track how long a block of code takes to execute. Using enables developers to benchmark their functions effectively. The key characteristic that stands out is its simplicity and ease of use.
A significant advantage of lies in its ability to track multiple timers simultaneously by using unique labels. This feature permits the comparison of performance across different functions or methods. However, it's worth noting that relying solely on this method without context can lead to misunderstandings regarding performance metrics, especially when external factors might affect execution time.
console.timeEnd
Complementing , the method concludes a timer set by . This method not only gives users the duration it took for the code execution but also associates the output directly with the corresponding label. By providing immediate feedback, helps in understanding performance gaps. The immediate output is what makes this method particularly beneficial in a development environment.
A unique feature of is its ability to provide instant visibility into performance issues. However, it lacks advanced features such as detailed profiling or visual representation of the timing data. If developers need more nuanced insights, they may explore other profiling tools that offer visualization capabilities. Nonetheless, for quick checks, it is advantageous and widely used.
Grouping Log Messages
console.group
The method allows developers to group related log messages in a collapsible and organized structure. This method improves readability and helps maintain focus when working with multiple console outputs. Its key characteristic is the ability to visually separate and categorize logs, making it easier to correlate output with different sections of code.
One distinct advantage of utilizing is that it dramatically enhances the clarity of console outputs. By organizing logs into groups, developers can trace execution paths more easily. However, excessive use of grouping without necessity can lead to cluttered consoles, making it challenging to find essential information. Careful usage is recommended for maximum effectiveness.
console.groupEnd
Following , the method closes the current group of log messages. This closure is vital as it maintains the organization established by . The key characteristic here is the ability to effectively signal the end of a logging group, ensuring the output remains structured and coherent.
The unique feature of lies in its simplicity, allowing for easy management of grouping operations. However, not using it in tandem with can lead to logical inconsistencies in the console output. Developers must be disciplined in their usage to fully leverage the benefits of grouping without creating confusion in their log data.
"Effective use of advanced console features not only improves workflow efficiency but shapes a developer's problem-solving approach."
By understanding and applying these advanced console features, developers can significantly enhance their debugging experience. These functionalities provide substantial advantages, leading to clearer insights and more efficient coding practices.
Error Handling in JavaScript
Error handling is a critical aspect of programming in JavaScript. Effective error management increases the robustness and reliability of applications. When developers can anticipate and manage errors gracefully, users experience fewer disruptions. This section will explore common error types and the utility of the statement.
Common Error Types
JavaScript defines several error types that developers must be aware of:
- SyntaxError: Occurs when the code does not follow the proper syntax. For example, missing parentheses or misnamed variables can lead to this error.
- ReferenceError: Happens when a code attempts to reference a variable that is not declared. Using an undeclared variable will reliably trigger this error.
- TypeError: This error surfaces when a variable is not of the expected type. For instance, treating a string like an array can lead to inconsistencies.
- RangeError: Arises when a value is not within the acceptable range. For example, passing an invalid array length will result in a range error.
Understanding these error types aids in diagnosing issues during development. In many cases, it allows developers to implement tailored error-handling strategies.
Using trycatch
The statement serves as a mechanism for handling exceptions. The basic structure is simple.
In the block, you place any code that might fail. If an error occurs, control jumps to the block. Here, you can manage the error appropriately, perhaps logging it or informing the user. This separation of concern enhances code readability and organization.
The construct promotes a structured approach to error handling in JavaScript, allowing for smoother development workflows and better user experiences.
Using this feature, a developer can prevent entire applications from crashing due to unhandled errors. Proper use of allows the developer to log errors to the console while maintaining program flow. This practice proves invaluable in debugging tasks and maintaining a quality user interface.
Console APIs and Extensions
Console APIs and extensions serve as vital components in optimizing the usage of the JavaScript console. These tools enhance how developers interact with the console, providing them with advanced functionalities that streamline the debugging process and improve productivity. Understanding these APIs is essential for anyone looking to maximize their efficiency when working with JavaScript. They open up new possibilities for manipulating output, tracking asynchronous processes, and monitoring network requests. By leveraging these APIs, developers can gain deeper insights into their applications, leading to better performance and user experience.
Async Callbacks
Async callbacks are a fundamental part of modern JavaScript programming, especially with the rise of asynchronous programming patterns. These callbacks allow developers to handle operations that take an unpredictable amount of time, such as fetching data from a server or manipulating files. The console provides methods to log, time, and keep track of these asynchronous operations.
Using async callbacks with the console aids in understanding the flow of an application. By logging the start and end times of callbacks, one can easily monitor how long each operation takes. This insight is crucial during development, as it helps identify bottlenecks.
Example of using async callbacks might look like this:
In the example above, the method starts a timer before the fetch operation. Once the data is fetched, the method logs the duration taken to complete the operation. This not only improves debugging processes but also promotes better code efficiency.
Network Requests Monitoring
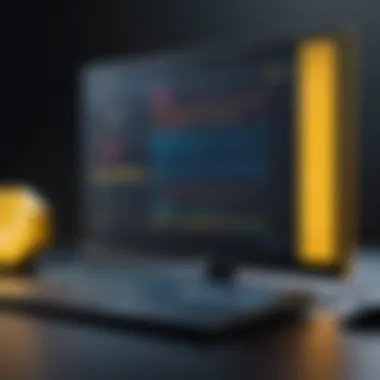
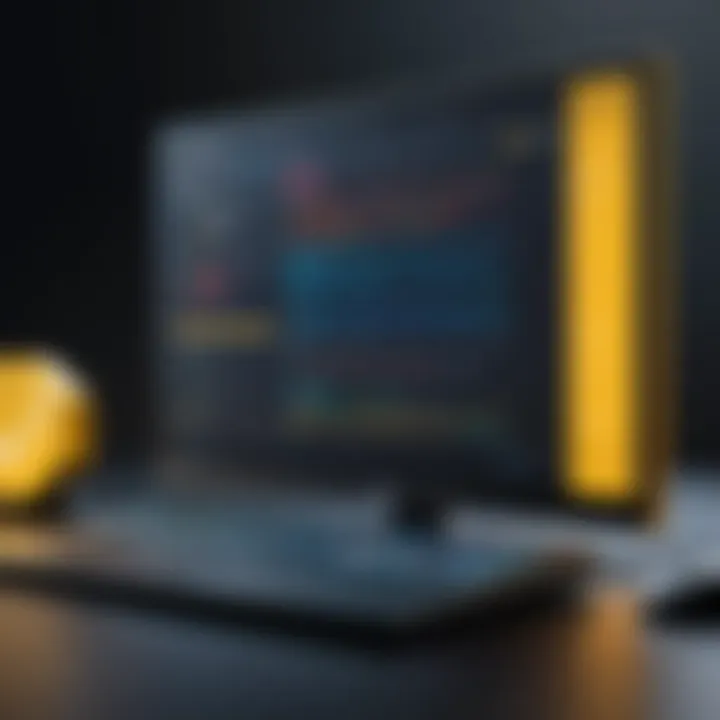
Monitoring network requests is another critical feature offered by the console APIs. This capability allows developers to track all network activities occurring within their applications. From monitoring API calls to third-party resource loading, the console provides a valuable overview of the data being transmitted.
With the method, developers can output relevant data about these requests, such as response times and any errors encountered. This information helps in diagnosing issues related to loading times or failed requests. For example:
In this code snippet, any issues during the fetch operation are logged directly to the console using . This provides immediate feedback for the developer, making it easier to troubleshoot problems efficiently.
"Understanding the console's capabilities enables developers to assert their command over the development process, paving the way for innovative and robust web applications."
In summary, utilizing Console APIs and extensions, such as async callbacks and network requests monitoring, is imperative for modern web development. These functionalities not only aid in debugging but also empower developers to write more efficient and insightful code.
Best Practices for Console Usage
The JavaScript console is a powerful tool that can enhance the web development process significantly. However, using it without care can lead to confusion and inefficiency. Therefore, understanding and implementing best practices for console usage is essential for developers, whether they are beginners or seasoned professionals. Proper use of the console aids in debugging, tracking application performance, and improving code clarity. It can serve as a bridge between understanding complex code behavior and maintaining clean communication in a development team.
Writing Clear Logs
When developers use the console to log information, clarity is crucial. Writing clear logs can help others understand what the log represents. This reduces the time spent deciphering messages during debugging sessions. Clear logs often include meaningful messages, relevant data, and proper categorization.
- Descriptive messages: Logs should clearly state what is being logged. Instead of , a more descriptive entry could be . This approach provides context.
- Use log levels appropriately: JavaScript offers different logging methods, such as and . Using these methods to categorize logs by severity helps prioritize issues.
- Include the relevant data: Whenever possible, include the necessary context in your logs. This might mean logging the state of an application or key variable contents at the time of logging.
As a part of this practice:
"Clarity in logs can prevent future headaches in debugging situations."
The use of colors for logging can also help differentiate levels and importance, making logs easier to digest at a glance.
Minimizing Console Usage in Production
While the console is invaluable during development, its usage in production environments must be approached with caution. Excessive console output can lead to performance issues and expose sensitive information.
Here are several considerations to keep in mind:
- Remove unnecessary logs: Before deploying applications, ensure that only essential logs remain. This can help enhance performance and security.
- Limit sensitive data: Avoid logging personal information or sensitive variables to prevent security vulnerabilities. Logs might be stored and exposed unintentionally.
- Use feature flags: Implementing feature flags can allow you to control logging levels in production without needing to change the codebase frequently. This allows for better monitoring while minimizing clutter.
By keeping console usage minimal in production, developers can maintain a focus on application performance and security without compromising system integrity.
In summary, adhering to best practices for console usage is essential for developers seeking to enhance their web development processes. Using clear logs facilitates understanding, while minimizing console use in production ensures a more secure and performant application.
The Future of the JavaScript Console
As web development continuously evolves, so does the JavaScript console. This tool plays a crucial role in debugging and development processes, and its future entails enhancements that will further streamline these tasks. Understanding what these changes may bring allows developers to leverage the console more effectively, yielding better results in their workflows. The significance of maximizing the console's potential cannot be overstated; as the complexity of web applications grows, developers must be equipped with advanced features that can simplify their debugging tasks.
Evolving Features
New features in the JavaScript console can make a substantial impact on how developers approach coding. One significant evolution is the integration of more detailed error reporting. Advanced environments offer clearer context around errors, including the values of variables at the time an error occurs. Furthermore, the introduction of new commands can enhance productivity, enabling developers to execute tasks with fewer keystrokes.
Additionally, improvements in performance monitoring within the console are key. Developers increasingly rely on optimizing their code for speed and efficiency. Features that provide real-time insights into execution time for functions can be incredibly beneficial. These tools help identify bottlenecks in code more quickly.
Another upcoming trend is enhanced collaboration features, allowing teams to share console logs and insights directly. In a world where remote teamwork is becoming the norm, these enhancements could streamline communication and lesson the back-and-forth that often complicates debugging discussions.
Integration with Other Tools
The future also holds promise in how the JavaScript console will integrate with other development tools. As the landscape of web development progresses, the need for seamless connections between different platforms becomes apparent. For instance, linking the console with version control systems like Git can provide immediate feedback on ongoing changes. This could prevent common issues related to merging code by offering real-time console data that can flag potential problems.
Moreover, integration with design tools such as Figma could enable live previews of changes as they happen, enhancing the developer experience. The ability to debug layout issues directly from the console while referencing design specifications will boost efficiency.
Lastly, there is a growing emphasis on automation. Future iterations of the console may support advanced scripting capabilities, where developers can automate routine tasks within the console itself. This would enable rapid iterations and testing, ultimately leading to more efficient development cycles.
The evolution of the JavaScript console is not just about adding features; it reflects a deeper understanding of developers' needs in a changing technological landscape.
In summary, the future of the JavaScript console is bright. With evolving features and better integration within development ecosystems, programmers can expect a rise in productivity, enhanced collaboration, and more robust debugging capabilities. Keeping an eye on these developments will be crucial for anyone aiming to excel in web development.
Closure
The conclusion of this article serves to encapsulate the essential discussions regarding the JavaScript console. It is critical to recognize its role as a fundamental tool in web development. The console provides a direct interface for developers to interact with their code, troubleshoot issues, and optimize performance. By engaging with the console, developers can identify errors, manipulate variables, and even assess the timing of various operations.
Recap of Key Points
In summary, the article delineates several important aspects:
- Definition and Purpose: The console is not just a tool; it is a robust platform for executing JavaScript in real-time.
- Accessing the Console: Understanding how to access the console through browser developer tools is vital for effective use.
- Basic Functions: Key functions like logging information and displaying errors are foundational for any debugging process.
- Debugging Techniques: Skills such as setting breakpoints and interpreting stack traces enhance debugging efficiency.
- Advanced Features: Timing functions and log message organization are powerful features to refine workflows.
- Error Handling: Being adept at using trycatch mechanisms prevents the application from crashing.
- Best Practices: Writing clear logs and managing console usage in a production environment maximizes effectiveness.
- Future Trends: Anticipating the evolution of console features can inform better practices in upcoming projects.
Encouragement for Further Exploration
Readers should be inspired to delve deeper into the JavaScript console and its extensive capabilities. There is always more to discover. It is worthwhile to experiment with advanced console APIs and to grasp new functionalities as they are developed. Engaging in forums on websites like Reddit, or contributing to discussions on platforms like Facebook, can open avenues for learning from experienced peers.
Through consistent exploration and practice, one can significantly enhance programming skills and debugging prowess. The console is a powerful ally in any developer's toolkit, making it essential to integrate its use into daily programming tasks. Continuously updating one's knowledge about the tools at one's disposal is key to becoming an adept web developer.