Master JavaScript in Just 30 Days: A Complete Guide
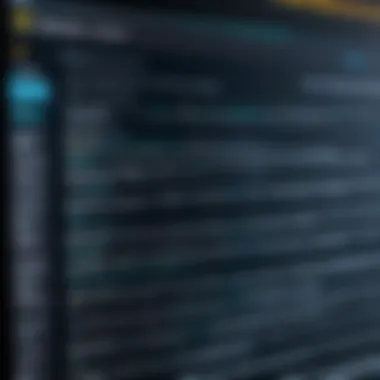
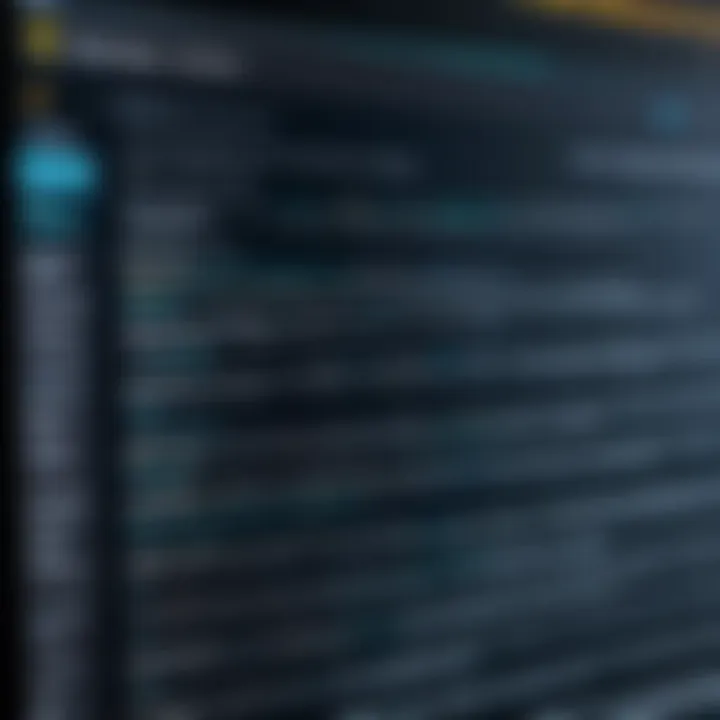
Overview of Topic
Prelims to the Main Concept Covered
Learning JavaScript is a fundamental step for anyone interested in web development. JavaScript is the language that drives interactivity on the web. It allows developers to create dynamic websites, build web applications, and enhance user experience. This guide aims to provide a structured approach to learning the essentials of JavaScript in a month, breaking down complex concepts into manageable parts.
Scope and Significance in the Tech Industry
JavaScript holds a crucial role in the tech industry. It's used in frontend development as well as increasingly in backend environments, especially with the rise of frameworks like Node.js. Understanding JavaScript opens up various career possibilities, from web developer roles to software engineering positions. This importance makes it essential for learners to grasp its fundamentals quickly and effectively.
Brief History and Evolution
JavaScript was created in 1995 by Brendan Eich during his time at Netscape. It was initially designed to add simple interactive elements to websites. Over the years, it has evolved significantly. Modern JavaScript includes advanced features such as async programming, modules, and classes, which enhance its capabilities and applications in web development.
Fundamentals Explained
Core Principles and Theories Related to the Topic
At its core, JavaScript follows certain principles that guide its functioning. Among these are the concepts of variables, data types, functions, and event handling. Understanding these principles provides a solid foundation for working with JavaScript.
Key Terminology and Definitions
It is important to familiarize yourself with some specific terminologies such as:
- Variable: A storage location for data.
- Function: A block of code designed to perform a particular task.
- Event: Actions that occur due to user interactions.
Basic Concepts and Foundational Knowledge
Basic concepts include:
- Syntax: The set rules that define the structure of JavaScript code.
- Data Types: These include strings, numbers, booleans, etc.
- Control Structures: Ways to dictate the flow of program execution, like loops and conditionals.
Practical Applications and Examples
Real-World Case Studies and Applications
JavaScript is used in countless applications we engage with daily. Websites like Facebook and Twitter rely heavily on JavaScript for their functionality. Learning to use this language can help you contribute to similar projects.
Demonstrations and Hands-On Projects
To solidify your learning, hands-on projects are essential. Consider building a simple to-do list app or a personal blog using JavaScript. These projects reinforce concepts and develop problem-solving skills.
Code Snippets and Implementation Guidelines
Advanced Topics and Latest Trends
Cutting-Edge Developments in the Field
The JavaScript ecosystem constantly evolves. New frameworks like React, Vue, and Angular are popular among developers. These frameworks help simplify and enhance the development process.
Advanced Techniques and Methodologies
Techniques such as asynchronous programming and functional programming are becoming standards in JavaScript. Understanding these concepts can set you apart from others in the field.
Future Prospects and Upcoming Trends
JavaScript's future looks bright, with ongoing advancements in both its capabilities and its applications across various fields. WebAssembly is one emerging area that shows promise for enhancing JavaScript performance in the near future.
Tips and Resources for Further Learning
Recommended Books, Courses, and Online Resources
Consider exploring these resources:
- "Eloquent JavaScript" by Marijn Haverbeke.
- Codecademy offers interactive JavaScript courses.
- FreeCodeCamp provides valuable exercises and projects.
Tools and Software for Practical Usage
For a smooth learning experience, utilize tools like:
- Visual Studio Code as your code editor.
- GitHub for version control and collaboration.
- Browser developer tools to test and debug your code.
"The best way to learn JavaScript is to write JavaScript."
Overall, this structured approach aims to provide learners a thorough understanding of JavaScript in just 30 days. By mastering these concepts, students not only gain proficiency but also prepare themselves for various real-world applications.
Preface to JavaScript
JavaScript is an essential programming language in the realm of web development, significantly enhancing the capabilities and interactivity of websites. Recognized for its versatility, it is used by both amateur and professional developers to create dynamic user interfaces. Learning JavaScript enables individuals to write code that executes in web browsers, providing a backbone for client-side programming. Furthermore, JavaScript is integral for back-end development through environments like Node.js, thus expanding its utility beyond traditional front-end tasks.
Understanding JavaScript
JavaScript is a high-level, interpreted programming language that is key to web technology. It falls within the category of dynamic languages, allowing for rapid development and deployment. The language supports object-oriented, imperative, and functional programming styles, granting developers a flexible approach to solving problems. A primary advantage of JavaScript is its asynchronous capabilities, which allow for non-blocking operations. This means web applications can perform tasks like fetching data from a server without freezing the user interface.
JavaScript is also widely supported across all modern browsers, making it a cornerstone for building applications that reach a broad audience. With JavaScript frameworks and libraries available, such as React, Angular, or Vue.js, development can be more streamlined and modular, encouraging efficiency and code reuse.
History and Evolution of JavaScript
JavaScript was created by Brendan Eich in 1995 at Netscape. Originally designed to add interactivity to web pages, it quickly gained traction, influencing the development of web standards. Over the years, JavaScript has evolved significantly. Its early iterations faced limitations, prompting the need for standardization. In 1997, the first version of ECMAScript was introduced, formalizing the language and facilitating wider use.
As web applications grew more complex, JavaScript underwent essential upgrades. The introduction of AJAX in the early 2000s allowed for asynchronous data loading, creating more dynamic web experiences. Subsequent updates have introduced features like promises, async/await, and modules through ECMAScript 6 and beyond. This evolution reflects changing development practices and user expectations, showcasing JavaScript's adaptability.
JavaScript has transitioned from a simple scripting language to a sophisticated tool essential for modern web development.
Overall, understanding JavaScript, its historical context, and its potential applications is critical for anyone looking to become proficient in web technologies. The next sections will delve deeper into the technical aspects, focusing on setting up an environment conducive to effective learning.
Setting Up the Development Environment
Setting up the development environment is one of the most important steps in starting your journey with JavaScript. The right environment can greatly improve productivity and enhance your coding experience. It refers to all the tools and applications that you will use to write, run, and test your JavaScript code.
A well-structured environment increases efficiency and allows ease of debugging and testing. This is especially crucial when you begin to work on more complex projects. You need a space where you can focus on coding without dealing with unnecessary issues related to configurations or tool compatibility.
The primary components you will need include a code editor and a runtime environment. Each of these components brings specific features that enhance your coding experience. In this section, we will discuss how to choose the best code editor and install essential runtime tools like Node.js and NPM.
Choosing the Right Code Editor
Your code editor is where you will spend significant time writing and editing your JavaScript code. Therefore, selecting a suitable code editor is crucial. Different editors have varying features, and the right choice can make a substantial difference in productivity.
Here are some popular options:
- Visual Studio Code: It is highly customizable, supports many extensions, and has integrated Git commands.
- Sublime Text: Known for its speed and excellent performance, it supports many programming languages.
- Atom: An open-source text editor that allows for collaboration with features like Teletype.
When choosing a code editor, consider the following:
- Ease of Use: It should have an intuitive interface. A complicated interface can hamper your learning process.
- Performance: The editor should not slow down as your project grows.
- Extensions: Look for an editor that allows you to expand its functionalities through plugins according to your needs.
In essence, choose one that feels comfortable to you, as the best code editor is the one that maximizes your effectiveness.
Installing Node.js and NPM
Node.js is a runtime environment that allows you to run JavaScript outside of a web browser. It is important for developing server-side applications and provides the ability to leverage JavaScript on the backend. Along with Node.js, you will also need NPM (Node Package Manager), which is a tool that allows you to install and manage libraries and packages that are essential for your development.
To install Node.js:
- Visit the official Node.js website: nodejs.org.
- Download the version recommended for most users. It comes with NPM pre-installed.
- Run the installer and follow the instructions.
After installation, you can verify your setup by running the following commands in your terminal:
If both commands return version numbers, you have successfully installed Node.js and NPM.
Setting up your development environment is a foundational step in learning JavaScript effectively, enabling a seamless transition into practical coding and project development. Once this is completed, you can move forward with confidence as you dive deeper into JavaScript.
JavaScript Basics
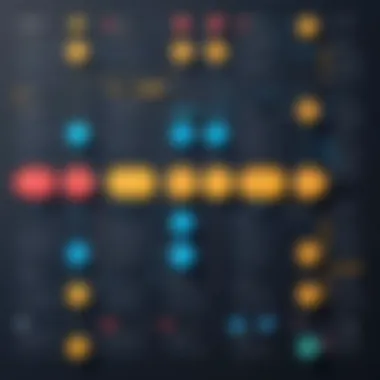
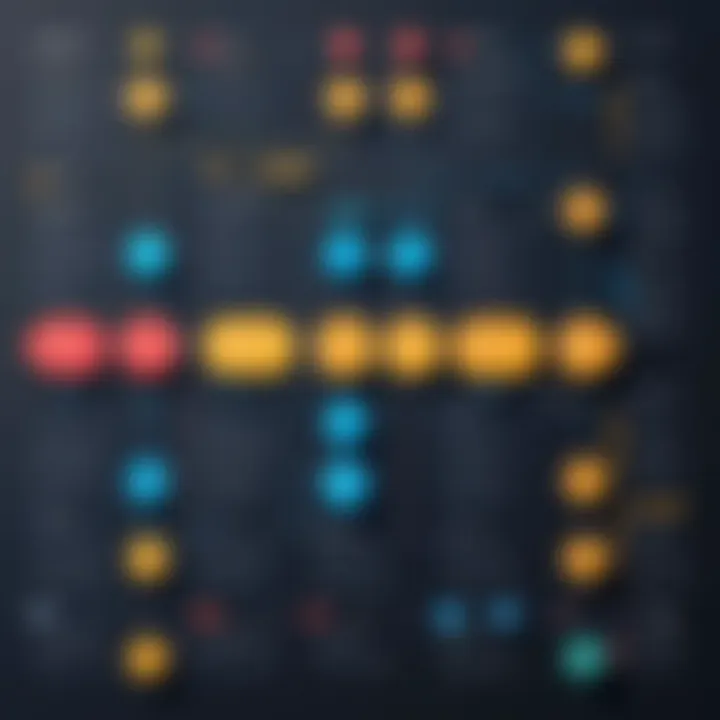
Understanding the basics of JavaScript is fundamental for anyone embarking on a journey to learn this versatile programming language. This section will explore Variables and Data Types as well as Operators and Expressions, which are essential cornerstones of JavaScript programming. Having a solid grasp of these basic concepts allows learners to write effective code, solve problems, and build more complex applications later on.
Variables and Data Types
Variables are containers for storing data values. In JavaScript, variable declarations utilize the keywords , , and , each serving distinct purposes. Choosing the correct keyword affects the variable's scope and mutability. This decision-making is crucial for code maintainability and reduction of unforeseen errors.
When dealing with variables, understanding data types is equally important. JavaScript has several data types:
- String: Represents textual data, like names or messages.
- Number: Represents both integer and floating-point numbers.
- Boolean: Can be either or .
- Object: Used to store collections of data and more complex entities.
- Array: A special type of object for working with lists of items.
- Undefined and Null: Represent the absence of value.
Each data type plays a role in how data is manipulated in programs. Therefore, knowing when to utilize these types is vital for effective coding.
Operators and Expressions
Operators in JavaScript are symbols that perform operations on variables and values. Understanding operators is crucial as they help in data manipulation and calculation. The primary categories of operators include:
- Arithmetic Operators: Such as , , , and , for performing basic mathematical operations.
- Assignment Operators: Such as , , and , to assign values to variables.
- Comparison Operators: Such as , , ``, and , for comparing values, which is essential for conditional statements.
Expressions combine variables, operators, and values to produce a result. A simple example of an expression can be seen below:
The understanding of both variables and data types, along with operators and expressions, sets the stage for more advanced concepts in JavaScript. Mastering these foundational elements makes for proficient code writing and helps avoid common pitfalls encountered by beginners in programming.
"The beauty of coding lies in the ability to solve problems creatively and efficiently."
Control Structures
Control structures form a fundamental part of any programming language, including JavaScript. They provide the framework to build logic in your code, allowing for decision-making and repetitive tasks. Understanding control structures is essential as they enable you to execute different blocks of code based on variable conditions or repeat instructions until a certain condition is met. In this section, we shall explore two key categories of control structures: conditional statements and loops.
Conditional Statements
Conditional statements are vital in programming since they control the flow of execution based on certain conditions. The most common conditional statements in JavaScript are , , and . These structures allow the programmer to define specific conditions under which certain blocks of code should execute.
Using conditional statements efficiently can significantly enhance the functionality of your applications. They enable more complex decision trees and branching logic. For instance, an online shopping site can use these statements to determine if a user is eligible for a discount based on criteria like cart value or membership status. Therefore, mastering conditional statements is essential to create versatile and interactive applications. A simple structure could look like this:
Loops
Loops allow a block of code to be executed repeatedly as long as a specified condition is true. They are crucial for tasks that require iteration, such as processing items in an array or generating repetitive behavior.
For Loops
The loop is a commonly used loop in JavaScript. It is ideal for scenarios where the number of iterations is known ahead of time. Its syntax succinctly combines initialization, condition-checking, and incrementing in one line, making it easy to read and manage.
A key characteristic of a loop is its simplicity and clarity when iterating through arrays or numbers. This makes it a popular choice among programmers.
For instance, when you want to iterate through an array of names, a loop allows you to access each element efficiently. However, if not used correctly, particularly with large datasets, you can run into performance issues. Hereโs a simple example:
While Loops
The loop continues to execute a block of code as long as the specified condition remains true. This kind of loop is beneficial when the number of iterations is not predetermined. A loop keeps checking the condition before each iteration, which can lead to infinite loops if the condition never becomes false.
This loop is particularly useful for user input validation and scenarios where the execution must continue until a certain state is reached. Here's a basic structure:
Do-While Loops
The loop operates similarly to a loop, but with one crucial difference: it guarantees that the block of code will execute at least once, regardless of the condition being true or false. This is particularly useful in cases where you want to ensure that a certain task is performed before making any checks.
The structure looks like this:
While the loop offers usefulness, it can sometimes lead to unexpected results if not carefully managed, especially if the condition is expected to be false based on some premise.
In summary, control structures are paramount in developing functional and responsive applications in JavaScript. By mastering the different types of loops and conditional statements, programmers can write more efficient and robust code.
Functions and Scope
Understanding functions and scope is pivotal in mastering JavaScript. Functions allow for the encapsulation of code, enabling reusability and modularity in programming. This leads to cleaner, more manageable code, which is essential as projects grow larger and more complex. Scope, on the other hand, is the context within which variables are defined and accessible. It governs how variable names are resolved, influencing how data is handled in an application. Both aspects are not only fundamental concepts in JavaScript but also critical to writing effective and efficient code. As you delve into these topics, you will discover how they interrelate and the advantages they offer in real-world applications.
Defining Functions
In JavaScript, a function can be defined as a block of reusable code designed to perform a specific task. Functions help streamline programming by allowing developers to invoke a single code snippet from multiple locations. They can take inputs, process them, and return an output, making them versatile tools.
To define a function, you can use the following syntax:
In this example, is the identifier for the function, while allows you to pass values into the function for processing. When the function runs, it can return a value that can be utilized elsewhere.
There are several prominent types of functions in JavaScript:
- Function Declarations: These are defined using the keyword, as shown in the example above.
- Function Expressions: Functions can also be defined as expressions and assigned to variables. For instance:
- Arrow Functions: Introduced in ES6, these provide a more concise syntax:
Utilizing functions effectively plays a significant role in enhancing programming efficiency.
Understanding Scope
Scope in JavaScript is the concept that determines the accessibility of variables. It is essential to grasp the differences between various scopes, as they dictate where variables can be accessed and modified. JavaScript uses lexical scoping, meaning it defines scopes based on the physical structure of the written code.
There are mainly three types of scope in JavaScript:
- Global Scope: Variables declared outside any function are in the global scope. They can be accessed from anywhere in the code.
- Function Scope: Variables declared within a function are contained within that functionโs scope and not accessible from outside.
- Block Scope: Introduced with ES6, and keywords create scope based on curly braces, limiting access to the variables defined within that block.
Understanding scope is vital for preventing unintended variable access and conflicts in your code.
Objects and Arrays
Objects and arrays are fundamental concepts in JavaScript, serving as essential building blocks for structuring and manipulating data. Mastering these elements is crucial for anyone on the path to becoming proficient in JavaScript. They allow programmers to manage complex data types efficiently, enabling more organized and scalable applications.
The benefits of using objects in JavaScript include the ability to store related data and functions together. Objects are collections of key-value pairs, where each key is a string (or Symbol) and each value can be of any type, including other objects or functions. This flexibility allows programmers to model real-world entities effectively. Arrays, on the other hand, are ordered lists of values, providing a simple way to manage collections of data. The inherent simplicity of arrays makes them invaluable for storing sequences and managing groups of items dynamically.
Considerations when working with objects and arrays in JavaScript also include understanding mutability, object references, and the ways in which these structures can impact performance. Grasping these nuances can enhance a developer's coding practice, leading to more efficient and readable code.
In summary, the importance of objects and arrays in JavaScript cannot be overstated. They empower developers to create robust applications and manage data in an organized manner, facilitating a deeper understanding of JavaScript programming.
Object Basics
Objects in JavaScript are created using either object literals or the syntax. The object literal syntax is more common due to its simplicity and readability. For example:
In this example, we create an object called with properties for name and breed, along with a method called . Accessing object properties can be done using dot notation or bracket notation, offering flexibility in how data is retrieved and manipulated. Understanding how to create, access, and modify objects is crucial for effective programming.
One important aspect of objects is their dynamic nature. You can easily add new properties or methods to an existing object at any time:
Working with Arrays
Arrays in JavaScript are dynamic arrays, meaning they can hold elements of any type and can grow or shrink in size. The creation of an array is straightforward, done either via an array literal or the constructor. A typical way to define an array looks like this:
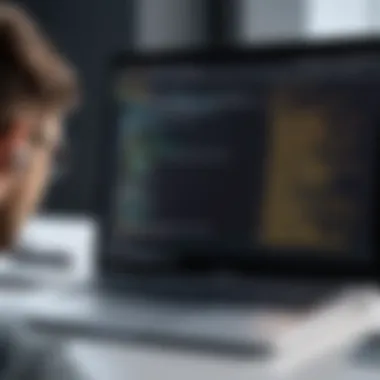
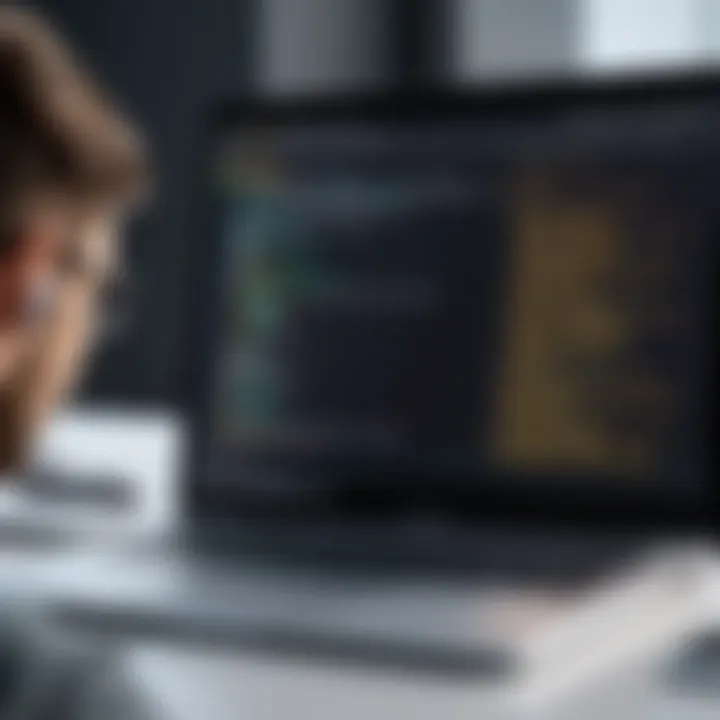
With arrays, developers can access elements using their index, starting from zero:
Arrays come equipped with numerous built-in methods that facilitate data manipulation without requiring elaborate code. For example:
- adds a new element to the end of the array.
- removes the last element.
- creates a new array with the results of calling a provided function on every element.
These methods provide powerful ways to manage and interact with collections of data, making arrays versatile tools in a programmer's toolkit. Understanding how to leverage these features effectively can lead to cleaner, more efficient code.
Advanced JavaScript Concepts
Advanced JavaScript concepts are crucial for anyone looking to deepen their understanding of the language. This section explores key topics like asynchronous JavaScript and error handling, which are pivotal in developing more complex applications. Mastering these concepts enhances a programmer's capability to create efficient and responsive web applications, thereby pushing the boundaries of what can be developed using JavaScript.
Asynchronous JavaScript
Asynchronous programming is a powerful feature of JavaScript, allowing for tasks to run without blocking the main thread. This characteristic is vital in scenarios where tasks may take an unpredictable amount of time, such as fetching data from a server. Understanding this concept can improve performance, making your applications responsive to user actions.
Callbacks
Callbacks are functions passed as arguments to another function. They are executed after the completion of a task. Callbacks enable non-blocking code execution, which is essential when working with asynchronous operations. The main advantage of callbacks is their simplicity. They allow developers to define what should happen after a task completes.
However, a key characteristic often discussed is callback hell, which arises when multiple nested callbacks make the code harder to read and maintain. This can lead to difficulty in debugging as the code structure becomes complex.
In this code block, notice how fetchData takes a callback function that executes once the simulated asynchronous operation completes.
Promises
Promises represent a value that may be available now, or in the future, or never. They provide a cleaner alternative to callbacks by allowing chaining, which results in more readable code. The primary benefit of promises is their ability to simplify handling asynchronous operations, making the code easier to understand.
Key characteristics of promises include three states: pending, fulfilled, and rejected. This structure helps in clearly delineating when the particular task is completed or failed. However, they can also introduce additional complexity, especially when multiple promises need to be managed together.
Here, the promise will resolve after one second, providing an easy way to manage the asynchronous result.
Async/Await
Async/Await is syntactic sugar built on top of promises that allows writing asynchronous code in a more synchronous fashion. It enables cleaner, more straightforward code by eliminating chaining syntax, making it easier to read and maintain. The highlight of using async/await is the ability to write code that looks synchronous but runs asynchronously in the background.
While it provides better code clarity, using async/await effectively requires understanding of promises. This can be a hurdle for beginners. It's also important to handle exceptions properly with try/catch to manage errors that might arise during asynchronous operations.
This example illustrates how async/await can create concise and readable asynchronous code, significantly improving error management through traditional try/catch blocks.
Error Handling
Error handling is a fundamental aspect of robust JavaScript applications. In asynchronous programming, errors can arise from various sources, such as network issues or unexpected data formats. It is vital to anticipate these issues and manage them effectively to avoid disrupting the user experience. Proper error handling ensures that your application can handle unexpected scenarios gracefully, maintaining its functionality and reliability.
In summary, mastering advanced JavaScript concepts expands one's programming toolkit. Asynchronous programming enables smoother operations while error handling promotes application stability. Both are essential for anyone aiming to build sophisticated web applications.
Working with the DOM
Working with the Document Object Model, or DOM, is crucial for anyone learning JavaScript. The DOM provides a structured representation of the document, which web developers can manipulate using JavaScript. This manipulation is essential to create dynamic and interactive web pages. By understanding how to work with the DOM, learners can change the content, structure, and style of a website in real-time.
The benefits of mastering the DOM include enhancing user experience and making applications more responsive. For instance, you can update the content of a page dynamically without needing to reload it, which improves performance and speed. Important considerations include understanding the hierarchy of elements within the DOM and how changes to one element can affect others. Keeping track of these relationships can be complex but is necessary for effective manipulation.
DOM Manipulation
DOM manipulation refers to changing the document structure through JavaScript. Various methods allow developers to add, remove, or edit HTML elements. The most common methods include , , and . These functions enable interactions with elements identified by their IDs, classes, or other attributes.
Here are some key DOM manipulation actions:
- Adding elements: You can create new elements and insert them into the document anywhere. For example, using can add a new element at the end of a specified parent element.
- Removing elements: If elements are no longer needed, you can use to take them out of the DOM, freeing up resources and reducing clutter.
- Changing attributes: Using allows you to change attributes for any element, like updating the of an tag or the of a link.
Knowing these methods is important for creating interactive web applications. A simple example of adding a new item to a list is provided below:
By manipulating the DOM, developers can drastically affect how users interact with their applications.
Event Handling
Event handling is another significant component when working with the DOM. It allows JavaScript to respond to user interactions such as clicks, key presses, and mouse movements. Understanding event handling is vital because it adds a layer of interactivity to web pages. Without it, websites would be static and less engaging.
To handle events, developers can use methods like , which listens for specified events on target elements. Whether it is a button click or an input change, using event listeners makes your application responsive to user actions.
Here are some common types of events:
- Click events: These are triggered when an element is clicked, commonly applied to buttons.
- Keyboard events: These monitor user input from the keyboard, useful for forms or shortcuts.
- Mouse events: These detect actions like hovering over an element or moving the mouse cursor.
For a clearer understanding, see the following example that shows how to handle a button click:
By mastering DOM manipulation and event handling, learners can create rich, interactive experiences on the web. This knowledge not only enhances their programming skill set but also prepares them for working on complex web projects.
Prelims to JavaScript Frameworks
JavaScript frameworks play a critical role in modern web development. As you progress in your learning journey, understanding these frameworks becomes essential. Frameworks provide a structured and efficient way to build applications, helping developers to be more productive. They offer pre-written code that simplifies common tasks, allowing you to focus on the unique aspects of your projects.
Using a framework can lead to faster development cycles and more maintainable code. Consider how a framework like React, Angular, or Vue.js can streamline your workflow. Each framework varies in its approach and features, but they all share the goal of enhancing development efficiency. It's definitely advisable for beginners to familiarize themselves with at least one framework to stay competitive in the job market.
Overview of Popular Frameworks
Many frameworks stand out due to their popularity and utility:
React
Developed by Facebook, React focuses on building user interfaces. It uses a component-based architecture, allowing developers to create reusable UI components. React's virtual DOM improves performance, making it suitable for dynamic web applications.
Angular
Angular, maintained by Google, is a full-fledged framework. It provides a complete solution for building scalable applications. With a focus on a structured approach, Angular uses TypeScript, which can help catch errors during development. The framework's powerful features make it ideal for complex applications but may have a steeper learning curve.
Vue.js
Vue.js is known for its simplicity and versatility. It combines the best features of both React and Angular. Vue is progressively adoptable, meaning you can integrate it into projects gradually. This makes it appealing for developers looking to enhance existing applications without complete rewrites.
jQuery
Though not a framework in the traditional sense, jQuery is significant. It simplifies HTML document traversing and event handling, making it easier to manipulate the DOM. While newer frameworks have gained traction, jQuery remains relevant in many projects today.
By understanding these frameworks, you can make informed decisions about which tool fits best for your needs.
Choosing Your First Framework
Selecting the right framework can be daunting. However, several factors can guide your decision:
- Project Requirement: Identify the requirements of the project you wish to build. For instance, if you need real-time updates, React could be a strong candidate.
- Learning Curve: Consider how much time you're willing to invest in learning a new framework. If you're looking for rapid development, Vue.js may be beneficial.
- Community Support: A larger community often means more resources, tutorials, and forums. This can be crucial when you encounter challenges.
- Future Projects: Think long-term. If you plan on working in a specific industry or job, research which frameworks are popular in that field.
- Frameworks can significantly boost your productivity when building applications. It is essential to choose wisely to align with your personal and professional goals.
Hands-On Projects
Engaging in hands-on projects is a critical phase of the learning process when mastering JavaScript. This section serves as a bridge between theoretical concepts and real-world application; it enables learners to consolidate their understanding while developing practical skills. Through these projects, students become proficient in coding, gain confidence, and cultivate a deeper grasp of JavaScript functionalities.
One key benefit of hands-on projects is that they allow learners to see immediate results from their code. This instant feedback loop can reinforce learning and motivate individuals to experiment more. Moreover, practical experience fosters problem-solving skills, enabling students to approach technical challenges efficiently. It is also essential to select projects that align with one's current learning stage, which promotes incremental growth.
Other elements to consider include collaboration. Working on projects with peers or engaging within coding communities can enhance the learning experience. Feedback from others can provide new insights and differing perspectives, while group efforts can lead to creative solutions that may not have emerged individually.
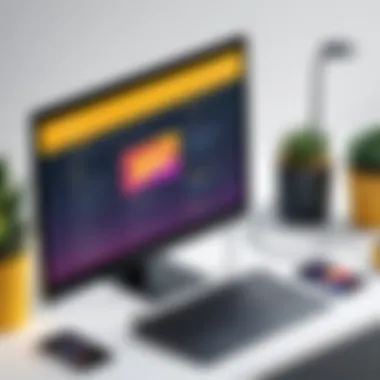
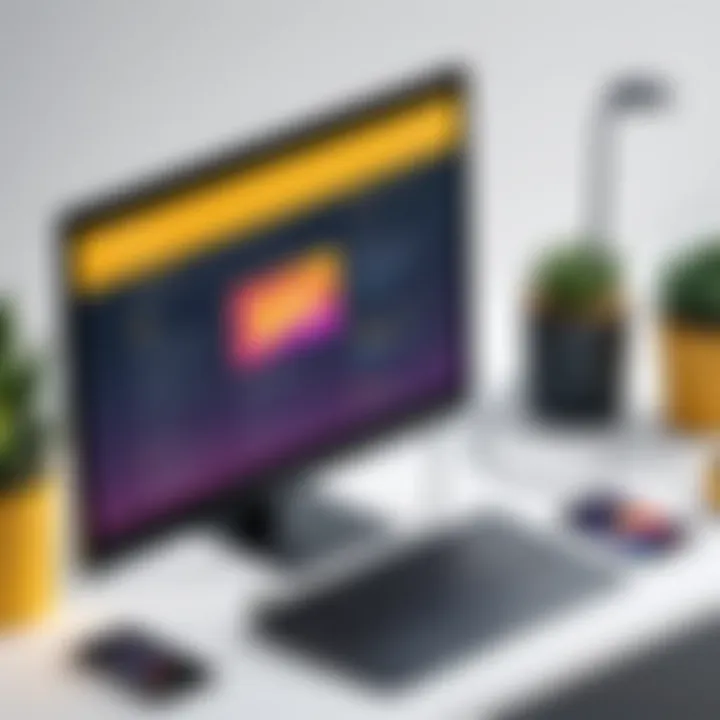
These projects should not be merely functional but should also incorporate best practices in coding and design. This includes code organization and optimization, making it easier to maintain and scale. Additionally, understanding the user experience is crucial when developing applications that will be used in real-world scenarios.
Building a Simple Website
When embarking on the journey to build a simple website, the learner synthesizes their foundational JavaScript knowledge. This project often starts with HTML and CSS frameworks, integrating JavaScript as the dynamic component. The goal is to create a responsive web experience that engages users and showcases essential skills such as DOM manipulation and event handling.
Key steps include:
- Planning the Structure: Decide the website's purpose, layout, and content.
- Setting Up the Environment: Use a code editor and local server to develop.
- Creating HTML: Draft the content and structure using HTML elements.
- Styling with CSS: Add styles to improve aesthetics and user engagement.
- Implementing JavaScript: Program interactive features like form validation, content toggles, or animations.
- Testing: Ensure functionality across different web browsers.
Building a simple website consolidates various skills and serves as a tangible result of the learning process. It allows learners to showcase their understanding of programming languages and web technologies while providing a platform for further exploration.
Creating a JavaScript Game
Developing a JavaScript game offers an exhilarating way to apply coding skills in a creative environment. This project typically involves game loops, user input management, and rendering graphics through the HTML5 Canvas API.
The process can be broken down into several stages:
- Conceptualizing the Game: Define the game type, rules, and mechanics. A small puzzle or a classic arcade game can be appropriate for beginners.
- Setting Up the Environment: Prepare a coding workspace and decide if additional libraries (like Phaser.js) are needed.
- Designing Game Assets: Create or source images and sounds necessary for the game.
- Coding the Game Logic: Implement the core functionalities, including player movements, scoring, and levels.
- Testing and Refinement: Playtest the game repeatedly to find and fix bugs, tweak difficulties, or enhance user experience.
Creating a JavaScript game not only makes the learning process enjoyable but also encourages perseverance. Game design encompasses many complexities, from logic to visual design, which fosters both creativity and critical thinking.
Learning through projects enriches your coding journey. Practical experience bridges gaps and transforms knowledge into skill.
Debugging JavaScript Code
Debugging is a critical aspect of coding in JavaScript. As one progresses in learning the language, the ability to identify and fix errors in code becomes essential. Debugging ensures code runs efficiently and achieves the desired output. Mistakes in code can lead to unexpected results, which can be frustrating, especially for those new to programming. By mastering debugging techniques, learners can improve their problem-solving skills and enhance their understanding of how JavaScript operates. It also promotes confidence in coding projects, which is vital for both personal and professional development.
Common Debugging Techniques
Effective debugging requires familiarity with various techniques. Here are a few common methods:
- Console Logging: Using statements is one of the simplest ways to track down issues. It allows one to inspect variables and understand the flow of the code. By logging different parts of the code, developers can see where things go wrong.
- Step-Through Debugging: This method involves running the code step by step. Many code editors offer this functionality, allowing users to pause execution and inspect the state of the application. This helps in identifying where errors occur.
- Error Messages: Reading and interpreting error messages can provide insight into what went wrong. JavaScript provides descriptive errors that often indicate the line and context of the problem. Understanding these messages can greatly aid in debugging efforts.
- Assertions: Using assertions in code can help verify assumptions made throughout the coding process. If an assertion fails, it indicates that something is wrong, often pointing to a specific logic error.
Using Browser Development Tools
Most modern web browsers come with built-in development tools that are crucial for debugging JavaScript code. These tools provide an environment where developers can inspect elements, monitor network requests, and analyze performance.
- Inspect Element: This feature allows users to see the HTML and CSS of a webpage in real-time. It is especially helpful when debugging how scripts interact with the Document Object Model (DOM).
- Console Tab: This is a primary debugging area. It displays error logs and allows for the execution of JavaScript commands directly in the browser. Users can test snippets of code and see immediate results.
- Sources Panel: Here, users can view and edit JavaScript files directly from the browser. It also allows for setting breakpoints, providing a way to pause execution and examine variables at a specific point in time.
- Network Monitor: This tool tracks all network requests made by a webpage. It aids in debugging issues related to API calls or resource loading failures.
Debugging is not just about fixing code; it is a skill that nurtures a deeper understanding of programming principles. As one becomes proficient in identifying and resolving issues, it will lead to more polished and maintainable code.
Debugging is a crucial skill; it transforms errors into learning opportunities.
For those on their journey of learning JavaScript, mastering these debugging techniques will be invaluable.
Resources for Continued Learning
In the journey of mastering JavaScript, utilizing the right resources is paramount. When you immerse yourself in this programming language, continuing education is essential. The tech industry evolves rapidly, so keeping skills updated is necessary. This section will explore valuable resources that will help learners deepen their understanding of JavaScript and apply their knowledge effectively.
Online Courses and Tutorials
Online courses have become a popular method for learning programming languages. They provide structured learning paths that guide students through the basics and beyond. Websites like Coursera, Udemy, and edX offer courses taught by industry professionals. These platforms not only present information clearly but also provide interactive exercises. This practical aspect reinforces learning and allows users to experiment with JavaScript in real-time.
Consider the following benefits of online courses:
- Flexibility: Learners can schedule classes according to their time and pace.
- Community: Many platforms have forums for students to ask questions and share knowledge.
- Variety: Courses range from beginner to advanced levels, catering to all learners.
Additionally, many courses come with certificates upon completion, which can be useful for showcasing skills to potential employers. Engaging with tutorials on platforms like freeCodeCamp can provide alternate methods for developing JavaScript knowledge. These free resources offer a no-risk way to start coding.
Books on JavaScript
Books remain a valuable resource for many learners. They provide depth and insight that online resources may not always cover. For example, titles like "You Donโt Know JS" by Kyle Simpson offer an in-depth exploration of JavaScript concepts. This series breaks down complex topics, making them easily digestible. Such dedicated works are essential for those who like to learn through reading, as they can read and re-read sections to fully grasp complicated elements.
Some essential JavaScript books include:
- JavaScript: The Good Parts by Douglas Crockford: This book focuses on key features of JavaScript while discarding the less-known aspects.
- Eloquent JavaScript by Marijn Haverbeke: A comprehensive guide that goes from the basics to advanced concepts, interspersed with exercises for practice.
Books often encourage deep thinking about problems, which is crucial for a programmer. This reflective aspect can help learners develop critical thinking and problem-solving abilities. While online resources are great for quick knowledge, books provide a more thorough foundation, which will be beneficial in the long run.
"The greatest benefit of continuous learning is the constant adaptation to the increasing complexity and dynamism of technology."
In summary, leveraging both online courses and books will enrich the JavaScript learning experience. They collectively address various learning styles and preferences, helping each learner progress more effectively.
Understanding Best Practices
Learning JavaScript effectively requires not only grasping its syntax and features but also understanding best practices. These are guidelines that help developers write clean, maintainable, and efficient code. Best practices foster collaboration among teams and improve overall workflow. They ensure that your code is scalable and easy to read, which benefits both you and anyone who works with your code in the future.
In this section, we explore two important aspects of best practices in JavaScript: code organization and performance optimization techniques.
Code Organization
Code organization refers to the structuring of your JavaScript code base in a way that enhances readability and maintainability. A well-organized codebase allows developers to navigate through files easily and understand how functions and modules interact with one another.
There are several strategies to follow for effective code organization:
- Modularization: Break code into smaller, reusable modules. This makes it simple to manage and test individual components.
- Use of Clear Naming Conventions: Choose descriptive names for variables and functions. This helps others (and your future self) to comprehend the intention behind the code more quickly.
- File Structure: Organize files in a logical manner. Group related files together, and separate concerns by keeping different functionalities in specific directories.
- Consistency: Maintain consistent coding styles across the codebase. Adopting a standard style guide can help here.
Good code organization not only leads to enhanced productivity but also makes it easier to collaborate with others. By following these principles, developers can minimize confusion and decrease the onboarding time for new members of the team.
Performance Optimization Techniques
Performance optimization techniques are essential in JavaScript to ensure that your applications run smoothly. Poorly optimized code can lead to slow loading times, unresponsive interfaces, and a negative user experience. To avoid these pitfalls, consider the following techniques:
- Reduce DOM Manipulations: Minimize direct manipulation of the Document Object Model (DOM) since it can be costly in terms of performance. Instead, batch changes and update the DOM in fewer operations.
- Use Event Delegation: Rather than attaching event listeners to numerous individual elements, attach a single event listener to a common ancestor. This reduces memory consumption and improves performance.
- Optimize Loops and Iterations: Analyze and optimize your loops for best efficiency. For instance, storing the length of an array in a variable before a loop can improve performance, especially for large arrays.
- Leverage Caching: Cache results of function calls or data lookups to avoid repeated computations. This reduces processing time and enhances performance.
Employing these techniques will yield a fast and responsive application, which is crucial in todayโs fast-paced digital environment. Remember, user experience heavily depends on how well your application performs.
"Good code is its own best documentation." - Steve McConnell
In summary, understanding best practices in JavaScript enhances the quality of your code, making it easier to read and maintain while also ensuring optimal performance. By focusing on code organization and performance optimization techniques, developers can create applications that are not only functional but also efficient.
Building a Portfolio
Building a portfolio is a crucial step for anyone serious about entering the field of web development and programming. It serves as a tangible representation of your skills and experiences, making it easier for potential employers or clients to evaluate your abilities. A well-structured portfolio not only highlights your technical skills but also demonstrates your thought process, creativity, and capability to solve problems through code. It can differentiate you in a competitive job market.
To be effective, your portfolio should showcase a range of projects that reflect your journey in learning JavaScript. This includes both completed works and projects that are still in progress, providing insight into your growth and learning curve. Here are some considerations to enhance the quality and impact of your portfolio:
- Diversity of Projects: Include various types of projects such as web applications, interactive features, or integrations that demonstrate different JavaScript capabilities. This shows your range and versatility.
- Quality Over Quantity: It is better to have a few excellent projects than many mediocre ones. Make sure each piece you include is polished and effectively showcases your skill set.
- Explain Your Process: Add context to your projects by including descriptions, challenges faced, and how you solved them. This helps viewers understand your thinking and problem-solving skills.
- Keep It Updated: Regularly refresh your portfolio with new projects or improvements on existing ones. This prevents it from becoming stale and reflects your ongoing commitment to learning.
"Your portfolio is often the first impression potential employers have of your work. Make it count."
Showcasing Your Projects
When it comes to showcasing your projects, it's important to provide a clear and structured presentation. Each project in your portfolio should consist of the following elements:
- Project Title: A brief and descriptive name that gives a clear indication of what the project is about.
- Live Demo: Whenever possible, link to a live version of your project. This allows viewers to interact with your work and experience its functionality firsthand.
- Source Code Access: Include a link to the project's GitHub repository or another platform where others can view your code. This transparency helps build trust and allows others to see your coding style.
- Description and Context: Provide a brief overview of the project, including its objectives, the technologies used, and key functionalities. Also, mention any challenges you faced and how you addressed them.
- Visuals: Use screenshots, short videos, or GIFs to make your project engaging. Visual content can quickly capture attention and make your work more appealing.
By presenting your projects thoughtfully, you effectively communicate your abilities and understanding of JavaScript and programming.
Leveraging GitHub for Visibility
GitHub is an essential platform, especially for developers, as it allows you to host projects, track changes, and collaborate with others. Leveraging GitHub can significantly enhance your visibility in the tech community. Here are some strategies to maximize its potential:
- Active Participation: Join coding communities and contribute to open-source projects. This connects you with other developers and expands your network.
- Consistent Commits: Regularly commit code to your repositories to show activity. This is an indicator of your ongoing engagement in coding and project development.
- Write Clear README Files: Each repository should have a README file that explains its purpose, installation instructions, usage, and examples. A well-documented README can make your project more inviting.
- Networking: Follow and interact with other developers. GitHub is not only a place for sharing code but also for building relationships within the developer community.
- Showcase Your GitHub Profile: Add your GitHub link to your resume, LinkedIn profile, and portfolio. Visibility on GitHub enhances your credibility and can lead to job opportunities.
By strategically utilizing GitHub, you not only improve your portfolio but also create pathways for collaboration and professional growth within the programming realm.
Finale
The conclusion of this article plays a significant role in reinforcing the knowledge and skills acquired during your JavaScript learning journey. It encapsulates the core learnings and emphasizes their relevance in real-world applications. When learners reflect on what they have discovered, it can deepen their understanding and encourage the ongoing pursuit of knowledge.
First, a recap of key takeaways is essential. This summary not only consolidates the material learned but also highlights the practical skills gained. Whether itโs control structures, functions, or working with the DOM, each topic interlinks to create a comprehensive understanding of JavaScript. Remembering these key points can help solidify the learnerโs foundation and prepare them for more complex concepts.
Additionally, exploring the next steps in your JavaScript journey is crucial. The journey does not end here. Equipped with the knowledge gained from this guide, you should consider advancing your skills through projects, frameworks, or even diving into backend development with Node.js. Engaging in the developer community, such as participating in discussions on sites like reddit.com or following tutorials on platforms mentioned, can enhance learning and expose new opportunities.
"Learning JavaScript is like building a toolbox. With each concept, you add a new tool, enabling you to tackle more complex problems."
Recap of Key Takeaways
- Understand key JavaScript concepts like variables, operators, and control structures.
- Grasp the importance of working with functions, objects, and arrays.
- Familiarize yourself with asynchronous operations and error handling.
- Experience hands-on projects to deepen practical skills and confidence.
Next Steps in Your JavaScript Journey
- Consider enrolling in advanced online courses to enhance your knowledge.
- Build a portfolio showcasing projects that demonstrate your skills.
- Explore popular frameworks like React or Angular to create modern web applications.
- Engage with community forums and local coding meetups for networking and learning.