Master Python for Data Analytics: A Comprehensive Guide
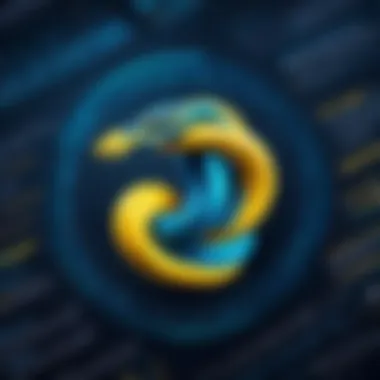
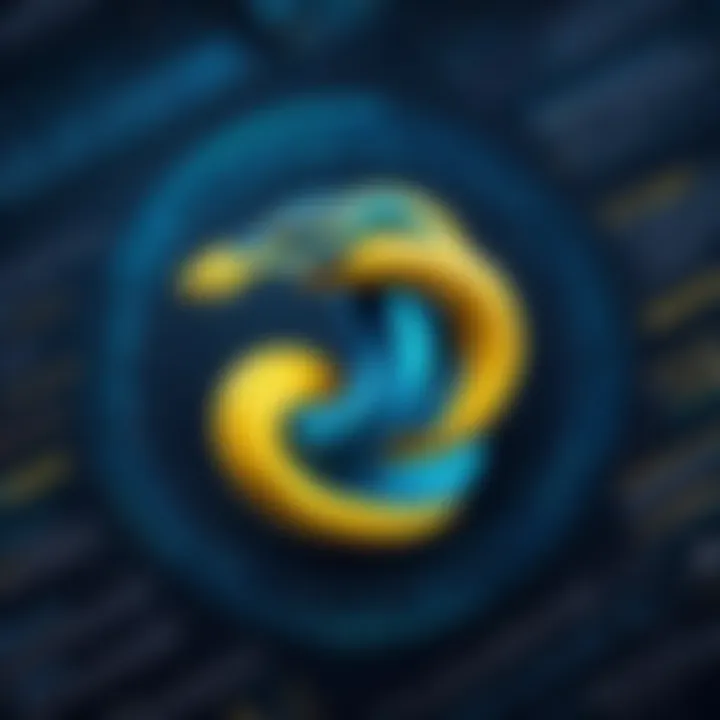
Overview of Topic
Prelude to the main concept covered
Learning Python for data analytics is becoming increasingly important in today’s data-driven world. Python is a versatile and robust programming language, favored for its simplicity and readability. With the exponential growth of data, the ability to analyze and extract insights from this data is crucial. This guide aims to provide a structured approach to mastering Python in the context of data analytics.
Scope and significance in the tech industry
The significance of Python in the tech industry cannot be overstated. Numerous organizations across various sectors utilize Python for tasks ranging from data manipulation to predictive analysis. It democratizes access to data analysis tools for professionals with varying levels of technical proficiency. Understanding Python opens pathways in fields like data science, machine learning, and artificial intelligence.
Brief history and evolution
Python was created by Guido van Rossum and first released in 1991. Over the years, it has evolved significantly, with version 3.0 released in 2008 emphasizing clearer syntax and better functionality. The rise of libraries such as Pandas, NumPy, and Matplotlib in the 2010s marked a milestone that made Python the go-to language for data manipulation and visualization. Today, Python is a mainstay in data analytics, with continued growth in tools and community support.
Fundamentals Explained
Core principles and theories related to the topic
Some foundational concepts in data analytics using Python include data ingestion, data processing, and data visualization. These principles guide organizations in making data-driven decisions through effective analysis. Understanding data types, control structures, and functions are key to leveraging Python in analytics.
Key terminology and definitions
Familiarizing oneself with jargon is essential. Here are some key terms:
- DataFrame: A two-dimensional size-mutable, potentially heterogeneous tabular data structure with labeled axes.
- Numpy: A fundamental package for scientific computing with Python, essential for handling arrays and matrices.
- Pandas: A powerful data manipulation library that provides data structures and functions needed for data analysis.
Basic concepts and foundational knowledge
To begin learning Python for data analytics, one should start with Python syntax, variables, data types, and control flow constructs. Understanding how to manipulate data through arrays and lists forms the bedrock for more complex operations later.
Practical Applications and Examples
Real-world case studies and applications
Many companies are using Python for data analytics. For example, Netflix uses Python for data analysis to drive recommendation engines. Financial institutions use it for risk assessment and fraud detection.
Demonstrations and hands-on projects
It is beneficial to engage in hands-on projects. One can analyze publicly available datasets on websites like Kaggle and demonstrate skills by building models that predict outcomes based on data trends.
Code snippets and implementation guidelines
Here’s an example of a simple code snippet using Pandas to load and display a dataset:
This basic command loads a CSV file and shows the first few rows, a fundamental step in data analysis.
Advanced Topics and Latest Trends
Cutting-edge developments in the field
The field of data analytics is continually evolving. Techniques such as deep learning and complex statistical modeling are becoming more prominent. Familiarizing oneself with these advanced techniques is crucial for any serious data analyst.
Advanced techniques and methodologies
Machine learning and artificial intelligence are at the forefront of analytics. Implementing libraries like TensorFlow or Scikit-learn can greatly enhance analytical capabilities and insights.
Future prospects and upcoming trends
Data storytelling and interpretation will become increasingly important as data continues to grow. The future belongs to those who can interpret data creatively and convey insights effectively.
Tips and Resources for Further Learning
Recommended books, courses, and online resources
Some valuable resources include:
- Automate the Boring Stuff with Python by Al Sweigart.
- Python for Data Analysis by Wes McKinney.
- Online platforms like Coursera, edX, or DataCamp offer courses tailored to data analytics with Python.
Tools and software for practical usage
Consider utilizing tools such as Jupyter Notebook for interactive coding, or Anaconda which offers a suite of tools for data science. Additionally, integrated development environments (IDEs) like PyCharm or Visual Studio Code can enhance productivity with Python programming.
Prelims to Python for Data Analytics
Python has become a cornerstone of the data analytics landscape. This section provides a comprehensive exploration of why Python is not just preferred but essential for data analytics. With the growth of data in many sectors, the demand for strong analytical capabilities has increased dramatically. Understanding data analytics through Python allows individuals and organizations to extract valuable insights from raw data, leading to informed decision-making.
The importance of Python in this context cannot be overstated. Its simplicity and versatility make it accessible to beginners, while also powerful enough for seasoned professionals. This combination allows data analysts to quickly turn data into actionable intelligence without getting mired in the complexities of programming languages. Python’s extensive library ecosystem enhances its utility, providing tools that cater specifically to data analytical tasks.
Definition and Importance of Data Analytics
Data analytics refers to the systematic computational analysis of data. It involves various techniques to cleanse, inspect, transform, and model data with the goal of discovering useful information, informing conclusions, and supporting decision-making. In today's data-driven world, data analytics plays a crucial role across industries. Organizations rely on data analytics for everything from understanding customer behavior to optimizing operational efficiency.
The importance of data analytics lies in its ability to turn vast amounts of data into meaningful insights. Organizations can identify trends, forecast future outcomes, and detect anomalies in their processes. By harnessing data analytics, companies position themselves competitively, leveraging insights into strategic advantages. A well-informed approach to data can lead to improved performance, enhanced customer satisfaction, and increased profitability.
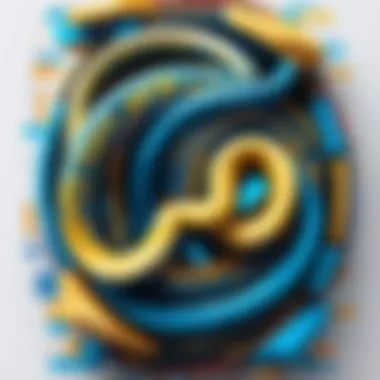
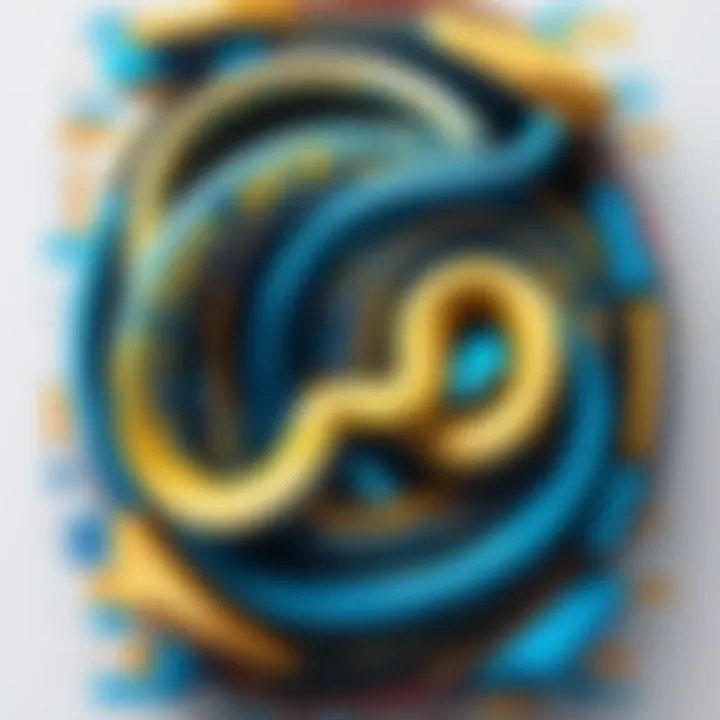
Why Python?
Choosing Python for data analytics comes down to several compelling factors:
- Ease of Learning: Python has a clean and straightforward syntax. This makes it a favorable choice for individuals new to programming.
- Community Support: The Python community is vast and active. This means that help is readily available through forums and user groups such as Reddit or Stack Overflow.
- Extensive Libraries: Libraries such as Pandas, NumPy, and Matplotlib are specifically designed for data analysis and visualization. These tools simplify complex tasks, enabling faster and more efficient analysis.
- Integration with Other Tools: Python integrates seamlessly with other programming languages and technologies, enhancing its applicability in diverse data environments.
- Versatility: Beyond data analytics, Python is used in web development, machine learning, automation, and more, making it a versatile tool for any programmer.
To quote a well-known expert in the field,
"Python is an ideal language for data analytics due to its simplicity and powerful capabilities, allowing even the most complex analysis to be performed with relative ease."
As you proceed through this guide, you will gain a deeper understanding of how Python can transform your approach to data analytics, addressing both its foundational concepts and advanced techniques that will enrich your analytical skill set.
Getting Started with Python
Getting started with Python is a crucial step for anyone looking to explore data analytics. Python's simplicity and versatility make it an ideal choice for beginners. This section will guide you through the initial steps necessary to set up your Python environment and understand its basic syntax. Learning these foundations will pave the way for mastering more complex data analytics tasks later on.
Installation and Setup
Before you can write any Python code, you need to install it on your computer. The installation process is quite straightforward, project can be done on most operating systems including Windows, macOS, and Linux. Here are the steps to follow:
- Visit the official Python website at python.org.
- Download the latest version of Python. Ensure that you choose the version that corresponds to your operating system.
- Run the installer. In the installation wizard, do not forget to check the box that says "Add Python to PATH." This makes it easier to run Python from the command line.
- Once the installation is complete, verify it by opening a terminal or command prompt, then typing . You should see the version number displayed.
In addition to Python, you might want to install an Integrated Development Environment (IDE). IDEs like PyCharm, Visual Studio Code, or Jupyter Notebooks can significantly enhance your coding experience by providing useful tools and features.
Basic Syntax and Structure
Understanding the basic syntax and structure of Python is imperative for new learners. Python code is known for its clean and readable layout. The following concepts form the foundation of Python programming:
- Indentation: Unlike many programming languages, Python uses indentation to define blocks of code. Each block must be consistently indented, which helps improve code readability.
- Variables: Variables are essential for storing data in Python. You can create a variable by simply assigning a value, for instance:
- Data Types: Python includes several built-in data types such as integers, floats, strings, and lists. Understanding these types is necessary for effective data manipulation.
- Comments: Comments in Python are indicated by the symbol. They are essential for explaining your code to others and yourself.
- Basic Operations: Performing operations such as addition, subtraction, multiplication, and division is straightforward in Python. For example:
print(result)# Output: 15
Using clear variable names enhances code readability, especially in data analytics where clarity is vital. Moreover, Python allows dynamic typing, which means you can change the type of a variable as needed, providing flexibility in data handling.
Control Structures
Control structures in Python dictate the flow of the program based on certain conditions. The primary control structures include conditional statements such as , , and , as well as loops like and . These constructions allow for more advanced data manipulation techniques.
For example, you might use a loop to iterate through a dataset:
This loop will print numbers from 0 to 9. In data analytics, control structures grant the capability to execute code based on the specific conditions of your dataset, facilitating operations like filtering, aggregating, or transforming data.
Functions and Modules
Functions in Python are blocks of reusable code that perform a specific task. Functions help to organize code into manageable pieces, allowing you to perform operations without repeating code. When discussing data analytics, creating functions for common tasks can save time and minimize errors.
A simple function might look like this:
Modules are files containing Python code, which can be imported into other code files. Utilizing modules allows you to leverage existing libraries and functionalities without reinventing the wheel. For instance, Python has extensive libraries like Pandas and NumPy that are crucial for data analysis, allowing analysts to work efficiently with complex datasets.
In summary, mastering these core concepts is non-negotiable for success in data analytics. Familiarity with data types, control structures, and functions/modules will empower you to write clean, effective Python code aimed at data-driven decision-making.
Data Handling with Python Libraries
Data handling is a fundamental component of data analytics. With the vast amount of data available today, using effective libraries in Python enables analysts to manipulate, clean, and analyze data efficiently. Python's libraries streamline the process of working with different types of data sources, whether they are numerical, categorical, or textual.
Python's popularity in the data analytics domain can be attributed to its simplicity, readability, and extensive support through libraries like Pandas, NumPy, and Matplotlib. These libraries provide consistent tools that allow data analysts to focus on insights rather than getting bogged down in technical complexities. Understanding these libraries is critical for anyone aiming to work in data analytics, as they form the backbone of data manipulation and visualization tasks.
Foreword to Pandas
Pandas is a powerful library for data manipulation and analysis. It offers data structures like DataFrames, which are well-suited for handling structured data. A DataFrame can be thought of as a table where rows represent observations and columns represent variables. Using Pandas, users can easily perform operations such as:
- Data cleaning and transformation.
- Filtering and indexing data.
- Handling missing data, which is common in real-world datasets.
With Pandas, operations that would typically require extensive code can be executed with concise syntax. Here is an example to demonstrate how to load a dataset:
This code snippet reads a CSV file into a DataFrame and displays the first few rows. The functional approach of Pandas enables a seamless transition from data acquisition to analysis.
Using NumPy for Numerical Data
NumPy is essential for performing numerical computations in Python. It provides support for arrays, matrices, and many mathematical functions. NumPy enhances performance for numerical analysis, as its operations are implemented in C and designed to handle large datasets with high efficiency.
Some advantages of using NumPy include:
- It offers an array interface that allows for fast mathematical computations.
- It supports broadcasting, which renders working with arrays of different shapes easier.
- NumPy integrates well with other scientific libraries, such as SciPy and Matplotlib, enhancing its utility in data analysis.
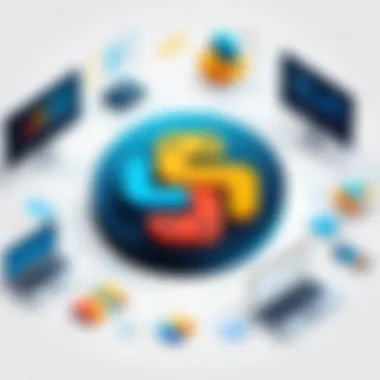
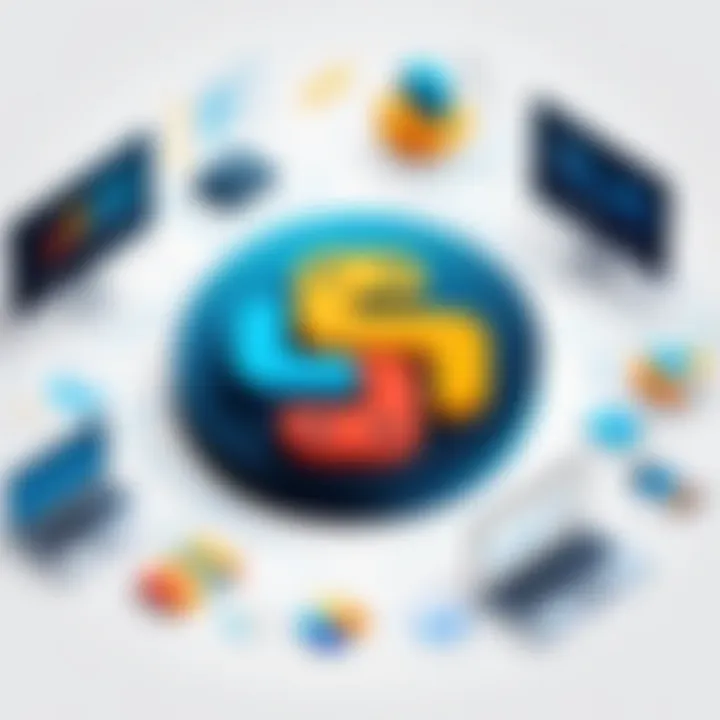
An example of using NumPy arrays for basic calculations is shown below:
This snippet calculates the mean of a set of numbers, illustrating how NumPy simplifies routine data-related tasks.
Data Visualization with Matplotlib and Seaborn
Visual representation of data is a critical step in data analytics, as it aids in revealing patterns, trends, and outliers. Matplotlib is one of the foundational libraries for data visualization in Python. It provides extensive options for creating static, animated, and interactive visualizations.
Seaborn, built on top of Matplotlib, enhances its functionality by offering a higher-level interface for drawing attractive statistical graphics. Using Seaborn, analysts can create informative plots with less code and greater aesthetics.
Some common features include:
- Histograms, scatter plots, and box plots.
- Options for customizing color palettes and styles.
- Integrated functionality with Pandas DataFrames, allowing for direct visualization of data.
Example code to create a simple line plot using Matplotlib:
This code snippet generates a line plot to visualize the relationship between two variables, demonstrating how straightforward it is to create graphs using these libraries.
Understanding these libraries is crucial for effective data analytics and enables analysts to extract meaningful insights from raw data.
Performing Data Analysis
Data analysis is a crucial step in any data-driven project. It transforms raw data into actionable insights. In data analytics, performing analysis effectively holds significant value. This section highlights specific elements that contribute to successful data analysis, such as cleaning, exploring, and applying statistical methods. The benefits of these practices can lead to better decisions, optimized processes, and improved understanding of the underlying data.
Data Cleaning Techniques
Data cleaning is often described as the foundation of data analysis. Accurate results depend heavily on the quality of the data used. Employing robust data cleaning techniques ensures that the data is consistent and free of errors.
Key techniques include:
- Removing duplicates: This prevents skewed results due to repeated data points.
- Handling missing values: Different strategies such as removal or imputation can be applied, depending on the context of the data.
- Standardizing formats: Consistency in date formats, text casing, and numerical values minimizes confusion in analysis.
Cleaning the data allows for a more precise exploration and enhances the reliability of the analytical outcomes. A well-cleaned dataset serves as a trustworthy foundation for subsequent analysis.
Exploratory Data Analysis (EDA)
Exploratory Data Analysis, or EDA, is a crucial method for understanding the characteristics of the data before diving deeper. EDA helps uncover patterns, trends, and anomalies that may not be immediately evident. It provides a preliminary visual perspective of the data.
Key activities in EDA include:
- Descriptive statistics: Measures such as mean, median, mode, and standard deviation provide insights into the dataset's distribution.
- Visualizations: Tools such as histograms, scatter plots, and box plots illustrate relationships and distributions effectively.
- Correlation analysis: Assessing relationships between variables helps in identifying potential predictors for further models.
The essence of EDA lies in its exploratory nature, allowing analysts to formulate hypotheses and direct future analyses. Ultimately, EDA aids in making informed decisions in the analysis process.
Statistical Analysis with Python
Statistical analysis is a powerful aspect of data analysis. Python provides robust libraries such as SciPy and StatsModels that simplify complex statistical operations. Understanding basic statistical principles enhances the educational journey in data analytics.
Key components of statistical analysis include:
- Hypothesis testing: This involves making inferences about populations based on sample data. Methods such as t-tests and chi-square tests determine the strength of relationships.
- Regression analysis: This aspect models relationships between variables. Linear regression is commonly used to predict outcomes.
- ANOVA: Analysis of variance helps in comparing differences between group means.
Integrating statistical methods into analytics using Python empowers analysts to draw meaningful conclusions. Consistent application and exploration of these techniques are essential for deriving insights from data.
Python for Business Intelligence
In today's data-driven environment, the capacity to analyze vast amounts of information is essential for driving strategic decisions and gaining competitive advantages. Python has become a pivotal tool in Business Intelligence (BI), transforming raw data into digestible insights. Its versatility and the availability of numerous libraries make it the go-to language for organizations aiming to implement robust data analysis solutions.
Integration with Data Warehousing Solutions
Integrating Python with data warehousing solutions is critical for organizations looking to consolidate their data management processes. Data warehousing involves collecting and managing data from various sources, making it accessible for analysis. Here, Python plays a vital role. It can connect seamlessly with popular data warehousing systems like Amazon Redshift, Google BigQuery, and Snowflake. This integration allows analysts to query the warehouse, retrieve datasets, and manipulate them using Python’s powerful libraries.
Some key benefits of this integration include:
- Streamlined Data Access: Python scripts can automate data retrieval from warehouses, reducing the time spent on manual data extraction.
- Enhanced Data Management: Using libraries like Pandas enables better manipulation and visualization of data fetched from these sources.
- Scalability: Python can handle increasing volumes of data efficiently, accommodating the growing needs of organizations as data sources expand.
Additionally, it is vital to consider the security and compliance aspects when integrating Python with these systems. Ensuring secure connections and managing permissions properly can help protect sensitive information.
Reporting and Dashboard Creation
Reporting and dashboard creation are fundamental aspects of Business Intelligence. Businesses rely on insightful reports and dashboards to make data-driven decisions. Python simplifies this process through various third-party libraries, facilitating the creation of dynamic and engaging visual representations of data.
Libraries such as Matplotlib, Seaborn, and Plotly are instrumental in crafting appealing visualizations. Moreover, tools like Dash and Streamlit can be used for building interactive dashboards that stakeholders can explore in real-time.
Key elements to consider when creating reports and dashboards using Python:
- User-Focused Design: Understand the audience. Tailoring visualizations to meet specific needs increases engagement and comprehension.
- Automated Reporting: Python scripts can automate the generation and distribution of reports, saving valuable time for data analysts.
- Dynamic Updates: Interactive dashboards can reflect real-time data, providing up-to-date insights that organizations can act upon immediately.
Advanced Data Analytics Techniques
Advanced data analytics techniques represent a pivotal area in the realm of Python for data analytics. As industries expand their reliance on data, these techniques provide sophisticated tools to extract insights and predict outcomes. This section specifically addresses predictive analytics and machine learning applications, which are critical for both academic and professional growth in data analytics.
Predictive Analytics with Python
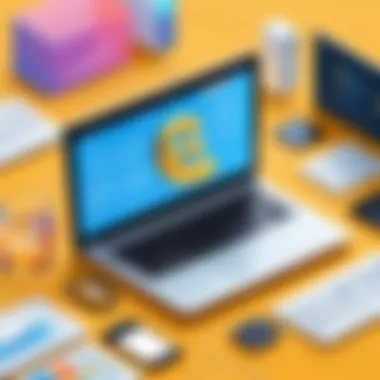
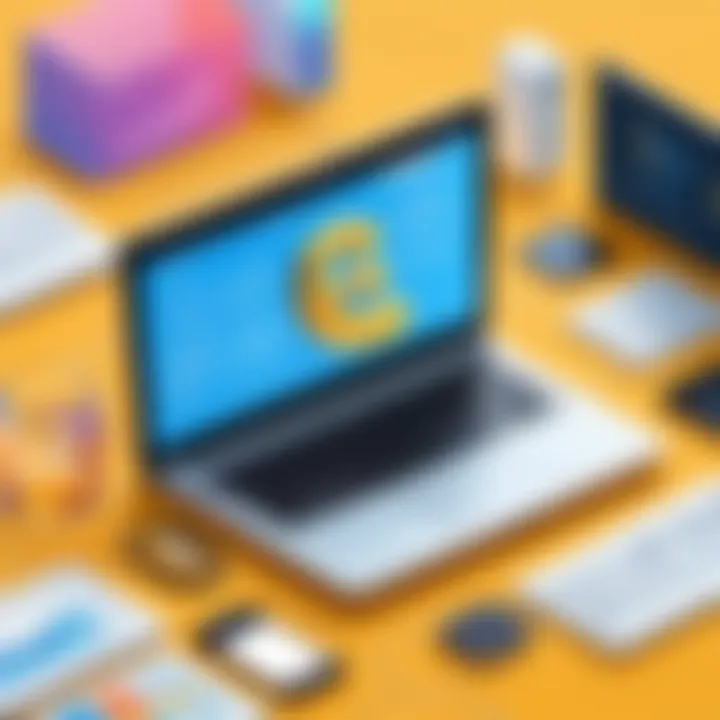
Predictive analytics refers to the branch of advanced analytics that uses historical data, statistical algorithms, and machine learning techniques to identify the likelihood of future outcomes. It enables organizations to make informed decisions based on data-driven predictions. In Python, several libraries play an essential role in predictive analytics, offering streamlined processes for data manipulation, model building, and evaluation.
Important libraries include:
- Scikit-learn: This library provides a robust framework for building predictive models. It offers various algorithms such as regression, classification, and clustering.
- StatsModels: Useful for statistical modeling, this library allows analysts to run tests for hypothesis and create detailed statistical reports.
- TensorFlow and Keras: For more complex models, these libraries are designed for deep learning, enabling the exploration of advanced neural networks.
To illustrate how predictive analytics functions in practice, consider a retail business wishing to forecast sales. By analyzing past sales data, promotional impacts, and customer behavior, an analyst can create predictive models that estimate future sales figures, thus aiding inventory management and marketing strategies.
Machine Learning Applications in Data Analytics
Machine learning is a subset of predictive analytics that employs algorithms to learn from data and improve performance over time. In the context of data analytics, it shines in its ability to recognize patterns and generate insights from large datasets.
Key applications of machine learning in data analytics include:
- Customer Segmentation: Using clustering algorithms to identify distinct customer groups based on purchasing habits.
- Anomaly Detection: Implementing techniques to detect irregularities in data which may indicate fraud or operational issues.
- Recommendation Systems: Utilizing collaborative filtering and content-based filtering to suggest products to users based on their previous interactions.
To begin using machine learning with Python, analysts typically follow these steps:
- Data Collection: Gather relevant datasets from reliable sources.
- Data Preprocessing: Clean and prepare the data for analysis.
- Model Selection: Choose appropriate algorithms based on the problem needs.
- Training: Feed the selected model with training data to optimize its parameters.
- Evaluation: Assess model performance using validation datasets to ensure accuracy and generalizability.
As these advanced techniques continue to evolve, they hold significant potential for transforming the field of data analytics. Understanding and applying these concepts will not only enhance analytical skills but also contribute to more effective decision-making processes.
Real-World Applications of Python in Data Analytics
Understanding how Python is applied in real-world scenarios is crucial. This section provides insight into the practical uses of Python in various industries, highlighting its versatility and effectiveness as a data analytics tool. Key benefits of utilizing Python for data analytics include its simplicity, the rich ecosystem of libraries, and strong community support. By examining concrete case studies, a reader can appreciate not just theoretical applications but also practical implementations that drive decision-making and innovation.
Case Studies in Various Industries
Several industries leverage Python for data analytics, providing numerous examples for learners to analyze.
- Finance: In the finance sector, Python is used for risk management and quantitative analysis. Hedge funds and investment firms employ algorithms for trading which rely on Python libraries like Pandas for data manipulation and NumPy for numerical analysis.
- Healthcare: Data from health records, patient surveys, and clinical trials can be analyzed using Python. Hospitals use Python to predict patient outcomes, optimize staffing, and manage resources based on historical data trends.
- Retail: Retail companies analyze consumer behavior through sales data. By using Python, they create predictive models that help in inventory management and personalized marketing strategies. Data collected from customer interactions can lead to better service and customer retention.
- Social Media: Platforms like Facebook produce massive amounts of data. Python helps in analyzing user interactions, preferences, and trends. Sentiment analysis tools built with Python can assist companies in understanding public perceptions about products or services.
These case studies illustrate that Python is not just a language for programming but a powerful tool for driving strategic decisions across various sectors. Each example serves as evidence of Python’s adaptability and importance.
Future Trends in Data Analytics using Python
With the continuous evolution of technology, Python's role in data analytics is expected to expand further. Key trends include:
- Automation: As businesses strive for efficiency, automation of data processes will become more common. Python scripts can automate repetitive tasks in data collection and analysis, which enhances productivity.
- Integration with Artificial Intelligence and Machine Learning: Python is poised to play a major role in integrating analytics with AI. Tools like TensorFlow and Scikit-learn enable data analysts to build sophisticated models to derive insights from data more effectively.
- Big Data Handling: The growth of big data necessitates advanced analytics tools. Python supports frameworks like PySpark, which allows handling large datasets seamlessly.
- Real-Time Analytics: As businesses become more data-driven, there is a shift toward real-time analytics. Python libraries can process data streams, leading to instant insights that are crucial in industries such as finance and telecommunications.
In summary, understanding real-world applications of Python in data analytics not only enriches the learning experience but also prepares individuals for future advancements in the industry. Python stands as a valuable resource in navigating through vast data landscapes.
"In a data-driven world, practical applications of data analytics using Python can lead to innovative solutions and informed decisions."
Best Practices for Data Analysts using Python
The success of data analytics projects lies not only in tools or frameworks but also in adhering to best practices when using Python. Following best practices helps maintain the clarity, efficiency, and accuracy of code, enabling data analysts to tackle complex problems effectively. It streamlines collaboration among team members, fosters easier maintenance, and enhances the overall quality of analysis, ultimately leading to better insights and decision-making.
Code Efficiency and Optimization
Code efficiency refers to how well a program performs under given constraints such as speed and resource usage. Optimizing code is crucial, particularly in data analytics where large datasets are common. Inefficient code can lead to longer processing times and increased computational costs.
Here are some essential tips to improve code efficiency:
- Use Built-in Functions: Python's built-in functions are often optimized for speed. Leveraging these can reduce code execution time significantly.
- Profiling: Use libraries like cProfile or line_profiler to identify bottlenecks in your code. These tools can help you understand where optimization is necessary.
- Vectorization: Utilizing libraries like NumPy allows you to operate on entire arrays instead of element by element, which enhances performance.
As a data analyst, always look for opportunities to refine your code. This may involve refactoring or rewriting code components to avoid redundancies and improve readability while maintaining or improving performance.
Documentation and Version Control
Documentation is often an overlooked aspect of coding. Proper documentation explains code functionality, making it easier for others (or yourself in the future) to understand the logic behind data analysis processes. A well-documented codebase provides context and rationale for decisions made during development, making troubleshooting and future enhancements simpler.
Some key points include:
- Docstrings: Use docstrings in functions and classes to provide a description of their purpose and parameters.
- Comments: Adhere to best practices for comments. Avoid comments that state the obvious; instead, focus on explaining why something is done, not just what is done.
Version control, typically handled with tools like Git, is essential in collaborative environments. It allows for tracking changes and maintaining a history of edits, which is crucial when multiple analysts work on the same codebase. Here are some advantages of using version control:
- Collaboration: Multiple team members can work on different features or bug fixes simultaneously without conflict.
- Revert Capability: Mistakes are less costly. You can revert to previous versions with ease if something goes wrong.
- Change Documentation: Commit messages serve as a record of the rationale behind code modifications, enhancing clarity.
By committing to these best practices, data analysts can create a robust environment for analysis and project development, improving overall effectiveness in their work.
The End
In this article, we have explored the essential aspects of learning Python specifically for data analytics. The importance of mastering Python cannot be overstated in today's data-driven world. Python offers a unique combination of simplicity and versatility, making it an ideal choice for both beginners and advanced users. Understanding the significance of data analytics and how Python fits into this landscape can provide a substantial advantage in various professional contexts.
Some specific elements we considered include the fundamental concepts, key libraries, and practical applications. As data analytics becomes more critical across industries, familiarizing oneself with tools like Pandas, NumPy, and visualization libraries such as Matplotlib and Seaborn helps leverage data effectively. The benefits of using Python in data analytics extend beyond just coding proficiency; they encompass the ability to extract insights and communicate findings effectively.
Moreover, the best practices for Python programming, such as code efficiency and proper documentation, equip analysts with the skills to work collaboratively in diverse teams. These considerations are crucial for making data-driven decisions that can enhance business outcomes.
Ultimately, Python serves not only as a programming language but as a powerful tool to interpret and analyze data. In a field that is continually evolving, staying informed about new techniques and frameworks will be integral to your success.
Recap of Key Points
- Python's versatility makes it suitable for various data analytics tasks.
- Key libraries like Pandas and NumPy streamline data manipulation processes.
- Visualization tools enhance the understanding of complex datasets.
- Best practices in coding promote collaboration and efficiency.
- Real-world applications demonstrate Python's relevance in different industries.
Next Steps in Your Learning Journey
To continue your path in mastering Python for data analytics, consider the following steps:
- Practice Regularly: Engage in hands-on projects to reinforce your learning.
- Explore Online Resources: Websites like Kaggle and Coursera offer courses and datasets for practice.
- Join Online Communities: Platforms like Reddit and Stack Overflow provide support and networking opportunities.
- Contribute to Open Source: Participating in open source projects can deepen your understanding and improve your skills.
- Experiment with Advanced Topics: Eventually, dive into machine learning and predictive analytics, expanding your capabilities.
By following these steps, you will solidify your competence in Python for data analytics and prepare yourself for various professional opportunities.